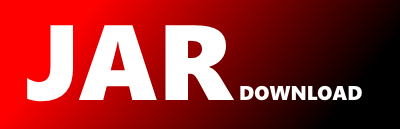
io.permazen.OnVersionChangeScanner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.reflect.TypeToken;
import io.permazen.annotation.OnVersionChange;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
/**
* Scans for {@link OnVersionChange @OnVersionChange} annotations.
*/
class OnVersionChangeScanner extends AnnotationScanner
implements Comparator.MethodInfo> {
private final TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy