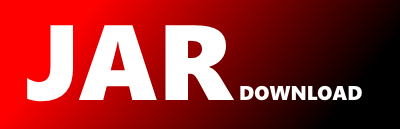
io.permazen.ArrayConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
The newest version!
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Converter;
import com.google.common.base.Preconditions;
import java.lang.reflect.Array;
class ArrayConverter extends Converter {
private final Converter converter;
private final Class aType;
private final Class bType;
@SuppressWarnings("unchecked")
ArrayConverter(Class aType, Class bType, Converter converter) {
Preconditions.checkArgument(aType != null, "null aType");
Preconditions.checkArgument(bType != null, "null bType");
Preconditions.checkArgument(converter != null, "null converter");
this.aType = aType;
this.bType = bType;
this.converter = converter;
}
@Override
protected B[] doForward(A[] value) {
return ArrayConverter.convert(this.aType, this.bType, this.converter, value);
}
@Override
protected A[] doBackward(B[] value) {
return ArrayConverter.convert(this.bType, this.aType, this.converter.reverse(), value);
}
@SuppressWarnings("unchecked")
private static Y[] convert(Class xType, Class yType, Converter converter, X[] value) {
if (value == null)
return null;
final int length = Array.getLength(value);
final Y[] result = (Y[])Array.newInstance(yType, length);
for (int i = 0; i < length; i++)
result[i] = converter.convert(value[i]);
return result;
}
// Object
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (obj == null || obj.getClass() != this.getClass())
return false;
final ArrayConverter, ?> that = (ArrayConverter, ?>)obj;
return this.aType == that.aType
&& this.bType == that.bType
&& this.converter.equals(that.converter);
}
@Override
public int hashCode() {
return this.getClass().hashCode()
^ this.aType.hashCode()
^ this.bType.hashCode()
^ this.converter.hashCode();
}
@Override
public String toString() {
return this.getClass().getSimpleName() + "[" + this.aType.getName() + "[]->" + this.bType.getName() + "[]]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy