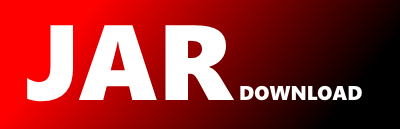
io.permazen.ConvertedIndex1 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
The newest version!
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Converter;
import com.google.common.base.Preconditions;
import io.permazen.index.Index1;
import io.permazen.tuple.Tuple2;
import io.permazen.util.Bounds;
import io.permazen.util.ConvertedNavigableMap;
import io.permazen.util.ConvertedNavigableSet;
import java.util.NavigableMap;
import java.util.NavigableSet;
/**
* Converter for {@link Index1}es.
*
* @param value type of this index
* @param target type of this index
* @param value type of wrapped index
* @param target type of wrapped index
*/
class ConvertedIndex1 implements Index1 {
private final Index1 index;
private final Converter valueConverter;
private final Converter targetConverter;
ConvertedIndex1(Index1 index, Converter valueConverter, Converter targetConverter) {
Preconditions.checkArgument(index != null, "null index");
Preconditions.checkArgument(valueConverter != null, "null valueConverter");
Preconditions.checkArgument(targetConverter != null, "null targetConverter");
this.index = index;
this.valueConverter = valueConverter;
this.targetConverter = targetConverter;
}
@Override
public NavigableSet> asSet() {
return new ConvertedNavigableSet, Tuple2>(this.index.asSet(),
new Tuple2Converter(this.valueConverter, this.targetConverter));
}
@Override
public NavigableMap> asMap() {
return new ConvertedNavigableMap, WV, NavigableSet>(this.index.asMap(),
this.valueConverter, new NavigableSetConverter(this.targetConverter));
}
@Override
public Index1 withValueBounds(Bounds bounds) {
return this.convert(this.index.withValueBounds(ConvertedIndex1.convert(bounds, this.valueConverter)));
}
@Override
public Index1 withTargetBounds(Bounds bounds) {
return this.convert(this.index.withTargetBounds(ConvertedIndex1.convert(bounds, this.targetConverter)));
}
private Index1 convert(Index1 boundedIndex) {
return boundedIndex == this.index ? this :
new ConvertedIndex1(boundedIndex, this.valueConverter, this.targetConverter);
}
static Bounds convert(Bounds bounds, Converter converter) {
final T2 lowerBound = bounds.hasLowerBound() ? converter.convert(bounds.getLowerBound()) : null;
final T2 upperBound = bounds.hasUpperBound() ? converter.convert(bounds.getUpperBound()) : null;
return new Bounds(lowerBound, bounds.getLowerBoundType(), upperBound, bounds.getUpperBoundType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy