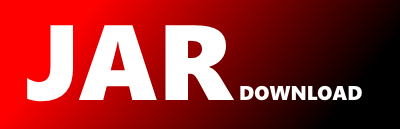
io.permazen.PermazenCollectionField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-main Show documentation
Show all versions of permazen-main Show documentation
Permazen classes that map Java model classes onto the core API.
The newest version!
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen;
import com.google.common.base.Preconditions;
import com.google.common.reflect.TypeToken;
import io.permazen.core.CollectionField;
import io.permazen.core.ObjId;
import io.permazen.core.Transaction;
import io.permazen.schema.CollectionSchemaField;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* Represents a collection field in a {@link PermazenClass}.
*/
public abstract class PermazenCollectionField extends PermazenComplexField {
final PermazenSimpleField elementField;
// Constructor
PermazenCollectionField(String name, int storageId, Annotation annotation,
PermazenSimpleField elementField, String description, Method getter) {
super(name, storageId, annotation, description, getter);
Preconditions.checkArgument(elementField != null, "null elementField");
this.elementField = elementField;
}
// Public Methods
/**
* Get the element sub-field.
*
* @return this instance's element sub-field
*/
public PermazenSimpleField getElementField() {
return this.elementField;
}
@Override
public abstract Collection getValue(PermazenObject pobj);
@Override
public List getSubFields() {
return Collections.singletonList(this.elementField);
}
@Override
public CollectionField getSchemaItem() {
return (CollectionField)super.getSchemaItem();
}
// Package Methods
@Override
CollectionSchemaField toSchemaItem() {
final CollectionSchemaField schemaField = (CollectionSchemaField)super.toSchemaItem();
schemaField.setElementField(this.elementField.toSchemaItem());
return schemaField;
}
@Override
abstract CollectionSchemaField createSchemaItem();
@Override
public TypeToken getTypeToken() {
return this.buildTypeToken(this.elementField.getTypeToken().wrap());
}
abstract TypeToken> buildTypeToken(TypeToken elementType);
@Override
void addChangeParameterTypes(List> types, Class targetType) {
this.addChangeParameterTypes(types, targetType, this.elementField.getTypeToken());
}
abstract void addChangeParameterTypes(List> types, Class targetType, TypeToken elementType);
// POJO import/export
@Override
@SuppressWarnings("unchecked")
void importPlain(ImportContext context, Object obj, ObjId id) {
// Get POJO collection
final Collection objCollection;
try {
objCollection = (Collection)obj.getClass().getMethod(this.getter.getName()).invoke(obj);
} catch (Exception e) {
return;
}
if (objCollection == null)
return;
// Get PermazenCollectionField collection
final Collection