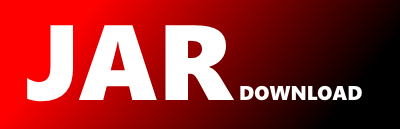
io.permazen.index.Index4 Maven / Gradle / Ivy
Show all versions of permazen-util Show documentation
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen.index;
import io.permazen.tuple.Tuple2;
import io.permazen.tuple.Tuple3;
import io.permazen.tuple.Tuple4;
import io.permazen.tuple.Tuple5;
import io.permazen.util.Bounds;
import java.util.NavigableMap;
import java.util.NavigableSet;
/**
* An index on a four fields.
*
*
* Indexes are read-only and "live", always reflecting the current transaction state.
*
* @param first indexed value type
* @param second indexed value type
* @param third indexed value type
* @param fourth indexed value type
* @param index target type
* @see io.permazen.index
*/
public interface Index4 extends Index {
@Override
default int numberOfFields() {
return 4;
}
/**
* View this index as a {@link NavigableSet} of tuples.
*
* @return {@link NavigableSet} of tuples containing indexed values and target value
*/
NavigableSet> asSet();
/**
* View this index as a {@link NavigableMap} of target values keyed by indexed value tuples.
*
* @return {@link NavigableMap} from indexed value tuple to the corresponding set of target objects
*/
NavigableMap, NavigableSet> asMap();
/**
* View this index as a {@link NavigableMap} of {@link Index1}s keyed by the first three values.
*
* @return {@link NavigableMap} from first three values to {@link Index1}
*/
NavigableMap, Index1> asMapOfIndex1();
/**
* View this index as a {@link NavigableMap} of {@link Index2}s keyed by the first two values.
*
* @return {@link NavigableMap} from first two values to {@link Index2}
*/
NavigableMap, Index2> asMapOfIndex2();
/**
* View this index as a {@link NavigableMap} of {@link Index3}s keyed by the first value.
*
* @return {@link NavigableMap} from first value to {@link Index3}
*/
NavigableMap> asMapOfIndex3();
/**
* Get the prefix of this instance that only includes the first four indexed fields.
*
* @return prefix of this index
*/
Index3 asIndex3();
/**
* Get the prefix of this instance that only includes the first three indexed fields.
*
* @return prefix of this index
*/
Index2 asIndex2();
/**
* Get the prefix of this instance that only includes the first two indexed fields.
*
* @return prefix of this index
*/
Index1 asIndex1();
/**
* Impose {@link Bounds} that restrict the range of the first indexed value.
*
* @param bounds bounds to impose on the first indexed value
* @return a view of this index in which only first values within {@code bounds} are visible
*/
Index4 withValue1Bounds(Bounds bounds);
/**
* Impose {@link Bounds} that restrict the range of the second indexed value.
*
* @param bounds bounds to impose on the second indexed value
* @return a view of this index in which only second values within {@code bounds} are visible
*/
Index4 withValue2Bounds(Bounds bounds);
/**
* Impose {@link Bounds} that restrict the range of the third indexed value.
*
* @param bounds bounds to impose on the third indexed value
* @return a view of this index in which only third values within {@code bounds} are visible
*/
Index4 withValue3Bounds(Bounds bounds);
/**
* Impose {@link Bounds} that restrict the range of the fourth indexed value.
*
* @param bounds bounds to impose on the fourth indexed value
* @return a view of this index in which only fourth values within {@code bounds} are visible
*/
Index4 withValue4Bounds(Bounds bounds);
/**
* Impose {@link Bounds} that restrict the range of the target value.
*
* @param bounds bounds to impose on the target value
* @return a view of this index in which only target values within {@code bounds} are visible
*/
Index4 withTargetBounds(Bounds bounds);
}