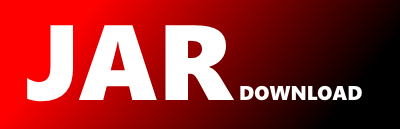
io.permazen.index.package-info Maven / Gradle / Ivy
Show all versions of permazen-util Show documentation
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
/**
* Permazen index classes. These classes define the Java interface to database indexes.
*
*
* Indexes provide fast lookup of objects based on field value(s). The {@code Index*} interfaces in this package have
* generic type parameters that correspond to the field value type(s), plus a final generic type parameter
* corresponding to the "target type". For example, an index on field {@code int }{@code getAccountNumber()} of type
* {@code User} will be represented by a {@link io.permazen.index.Index1}{@code }, and may be viewed
* either as a {@link java.util.NavigableSet}{@code <}{@link io.permazen.tuple.Tuple2}{@code >}
* or a {@link java.util.NavigableMap}{@code >}.
*
*
* Indexes are accessible through the {@link io.permazen.PermazenTransaction} API:
*
* - {@link io.permazen.PermazenTransaction#querySimpleIndex(Class, String, Class) PermazenTransaction.querySimpleIndex()}
* - Access the index associated with a simple field
* - {@link io.permazen.PermazenTransaction#queryListElementIndex PermazenTransaction.queryListElementIndex()}
* - Access the composite index associated with a list field that includes corresponding list indices
* - {@link io.permazen.PermazenTransaction#queryMapValueIndex PermazenTransaction.queryMapValueIndex()}
* - Access the composite index associated with a map value field that includes corresponding map keys
* - {@link io.permazen.PermazenTransaction#queryCompositeIndex(Class, String, Class, Class)
* PermazenTransaction.queryCompositeIndex()} - Access a composite index defined on two fields
* - {@link io.permazen.PermazenTransaction#queryCompositeIndex(Class, String, Class, Class, Class)
* PermazenTransaction.queryCompositeIndex()} - Access a composite index defined on three fields
* - {@link io.permazen.PermazenTransaction#queryCompositeIndex(Class, String, Class, Class, Class, Class)
* PermazenTransaction.queryCompositeIndex()} - Access a composite index defined on four fields
*
* - {@link io.permazen.PermazenTransaction#querySchemaIndex PermazenTransaction.querySchemaIndex()}
* - Get database objects grouped according to their schema versions
*
*
*
* Simple and Composite Indexes
*
*
* A simple index on a single field value is created by setting {@code indexed="true"} on the
* {@link io.permazen.annotation.PermazenField @PermazenField} annotation.
*
*
* Composite indexes on multiple fields are also supported. These are useful when the target type needs to be sorted
* on multiple fields; for simple searching on multiple fields, it suffices to have independent, single-field indexes,
* which can be intersected via {@link io.permazen.util.NavigableSets#intersection NavigableSets.intersection()}, etc.
*
*
* A composite index on two fields {@code String getUsername()} and {@code float getBalance()} of type {@code User}
* will be represented by a {@link io.permazen.index.Index2}{@code }; a composite index on
* three fields of type {@code X}, {@code Y}, and {@code Z} by a {@link io.permazen.index.Index3}{@code }, etc.
*
*
* A composite index may be viewed as a set of tuples of indexed and target values, or as various mappings from one
* or more indexed field values to subsequent values.
*
*
* A composite index may always be viewed as a simpler index on any prefix of its indexed fields; for example, see
* {@link io.permazen.index.Index2#asIndex1}.
*
*
* Complex Sub-Fields
*
*
* Only simple fields may be indexed, but the indexed field can be either a normal object field or a sub-field of
* a complex {@link java.util.Set Set}, {@link java.util.List List}, or {@link java.util.Map Map} field. However,
* complex sub-fields may not appear in composite indexes.
*
*
* For those complex sub-fields that can contain duplicate values (namely, {@link java.util.List} element and
* {@link java.util.Map} value), the associated distinguishing value (respectively, {@link java.util.List} index
* and {@link java.util.Map} key) becomes a new value that is appended to the index.
* The resulting index types associated with indexes on complex sub-fields of some object type {@code Foobar} are as follows:
*
*
*
* Index Types
*
* Complex Field
* Indexed Sub-Field
* Distinguising Value
* Distinguising Type
* Index Type
*
*
* {@link java.util.Set}{@code }
* Set element
* n/a
* n/a
* {@link io.permazen.index.Index1}{@code }
*
*
* {@link java.util.List}{@code }
* List element
* List index
* {@link java.lang.Integer}
* {@link io.permazen.index.Index2}{@code }
*
*
* {@link java.util.Map}{@code }
* Map key
* n/a
* n/a
* {@link io.permazen.index.Index1}{@code }
*
*
* {@link java.util.Map}{@code }
* Map value
* Map key
* {@code K}
* {@link io.permazen.index.Index2}{@code }
*
*
*
*
*
* To ignore the distinguishing values, convert the {@link io.permazen.index.Index2} back into an {@link io.permazen.index.Index1}
* via {@link io.permazen.index.Index2#asIndex1}.
*/
package io.permazen.index;