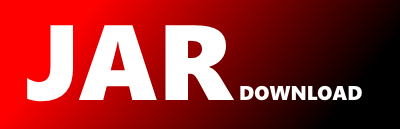
io.permazen.util.ConvertedSpliterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-util Show documentation
Show all versions of permazen-util Show documentation
Common utility classes used by Permazen.
The newest version!
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen.util;
import com.google.common.base.Converter;
import com.google.common.base.Preconditions;
import java.util.Comparator;
import java.util.Spliterator;
import java.util.function.Consumer;
/**
* Provides a transformed view of a wrapped {@link ConvertedSpliterator} using a strictly invertable {@link Converter}.
*
* @param element type of this instance
* @param element type of the wrapped instance
*/
public class ConvertedSpliterator implements Spliterator {
private final Spliterator inner;
private final Converter converter;
/**
* Constructor.
*
* @param inner wrapped instance
* @param converter element converter
* @throws IllegalArgumentException if either parameter is null
*/
public ConvertedSpliterator(Spliterator inner, Converter converter) {
Preconditions.checkArgument(inner != null, "null inner");
Preconditions.checkArgument(converter != null, "null converter");
this.inner = inner;
this.converter = converter;
}
/**
* Get the wrapped {@link Spliterator}.
*
* @return the wrapped {@link Spliterator}.
*/
public Spliterator getWrappedSpliterator() {
return this.inner;
}
/**
* Get the {@link Converter} associated with this instance.
*
* @return associated {@link Converter}
*/
public Converter getConverter() {
return this.converter;
}
// Spliterator
@Override
public int characteristics() {
return this.inner.characteristics();
}
@Override
public long estimateSize() {
return this.inner.estimateSize();
}
@Override
public void forEachRemaining(Consumer super E> consumer) {
this.inner.forEachRemaining(element -> consumer.accept(this.converter.convert(element)));
}
@Override
public Comparator getComparator() {
final Comparator super W> comparator = this.inner.getComparator();
return comparator != null ? new ConvertedComparator(comparator, this.converter.reverse()) : null;
}
@Override
public long getExactSizeIfKnown() {
return this.inner.getExactSizeIfKnown();
}
@Override
public boolean hasCharacteristics(int characteristics) {
return this.inner.hasCharacteristics(characteristics);
}
@Override
public boolean tryAdvance(Consumer super E> action) {
return this.inner.tryAdvance(element -> action.accept(this.converter.convert(element)));
}
@Override
public Spliterator trySplit() {
final Spliterator split = this.inner.trySplit();
return split != null ? new ConvertedSpliterator<>(split, this.converter) : null;
}
// Object
@Override
public boolean equals(Object obj) {
if (obj == this)
return true;
if (obj == null || obj.getClass() != this.getClass())
return false;
final ConvertedSpliterator, ?> that = (ConvertedSpliterator, ?>)obj;
return this.inner.equals(that.inner) && this.converter.equals(that.converter);
}
@Override
public int hashCode() {
return this.getClass().hashCode()
^ this.inner.hashCode()
^ this.converter.hashCode();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy