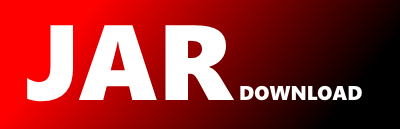
io.permazen.util.UnionNavigableSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of permazen-util Show documentation
Show all versions of permazen-util Show documentation
Common utility classes used by Permazen.
The newest version!
/*
* Copyright (C) 2015 Archie L. Cobbs. All rights reserved.
*/
package io.permazen.util;
import com.google.common.collect.Iterators;
import com.google.common.collect.Lists;
import java.util.Collection;
import java.util.Comparator;
import java.util.List;
import java.util.NavigableSet;
/**
* Provides a read-only view of the union of two or more {@link NavigableSet}s.
*/
class UnionNavigableSet extends AbstractMultiNavigableSet {
/**
* Constructor.
*
* @param sets the sets to union
*/
UnionNavigableSet(Collection extends NavigableSet> sets) {
super(sets);
}
/**
* Internal constructor.
*
* @param sets the sets to union
* @param comparator common comparator
* @param bounds range restriction
* @throws IllegalArgumentException if {@code bounds} is null
*/
protected UnionNavigableSet(Collection extends NavigableSet> sets, Comparator super E> comparator, Bounds bounds) {
super(sets, comparator, bounds);
}
@Override
protected NavigableSet createSubSet(boolean reverse, Bounds newBounds, List> newList) {
final Comparator super E> newComparator = this.getComparator(reverse);
return new UnionNavigableSet<>(newList, newComparator, newBounds);
}
@Override
public boolean contains(Object obj) {
for (NavigableSet set : this.list) {
if (set.contains(obj))
return true;
}
return false;
}
@Override
public CloseableIterator iterator() {
final Comparator super E> comparator = this.getComparator(false);
return new UniqueIterator(
Iterators.mergeSorted(Lists.transform(this.list, NavigableSet::iterator), comparator),
comparator);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy