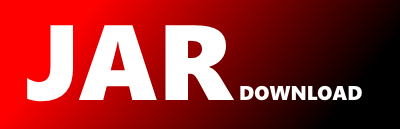
com.tinypass.client.publisher.model.OfferTemplate Maven / Gradle / Ivy
package com.tinypass.client.publisher.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import com.tinypass.client.publisher.model.OfferTemplateContentField;
import com.tinypass.client.publisher.model.OfferTemplateVariant;
import com.tinypass.client.publisher.model.User;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class OfferTemplate {
/* The template ID */
private String offerTemplateId = null;
/* Is template token type */
private String tokenType = null;
//public enum tokenTypeEnum { NON_STATIC, STATIC, BOILERPLATE, };
/* The application ID */
private String aid = null;
/* The application name */
private String appName = null;
/* The name */
private String name = null;
/* The description */
private String description = null;
/* Whether the object is deleted */
private Boolean deleted = null;
/* The creation date */
private Integer createDate = null;
private User createBy = null;
/* The update date */
private Integer updateDate = null;
private User updateBy = null;
/* publish_date */
private Integer publishDate = null;
/* The version */
private Integer version = null;
/* The type */
private String type = null;
//public enum typeEnum { Default Offer, Checkout, Checkout Style, Checkout Components, Error screen, Payment Components, Promotion Components, Provider Components, Term Components, Receipt, Already has access, External Verification Components, Confirmation screen, Custom login/register, Print address collect screen, Consent Components, Gift Components, Redemption Components, 3d Bank secure components, Shared subscription components, Site Licensing Landing Page, Site Licensing Contract List, Site Licensing Redeem Result, Upgrade components, Reactivate subscription components, Upgrade authentication components, Future Start Components, My Account Style, My Account Help Components, My Account common components templates, My Account layout, My Account Library Components, My Account Payments Components, My Account Wallet Components, My Account Voucher Components, My Account User Profile Components, My Account Cash Payment Components, My Account error page message, My Account Transactions Components, My Account Licensing Components, Piano ID layout, Piano ID login page, Piano ID register page, Piano ID initiate password reset, Piano ID new password page, Piano ID generic error page, Piano ID profile in My Account, Piano ID login confirm page, Piano ID register confirm page, My Account PianoId Components, Piano ID custom form, Piano ID email is not confirmed, Piano ID email confirmation required, Piano ID email confirmation view, Piano ID passwordless login page, Piano ID SSO confirm page, Piano ID Digital code, Piano ID newsletters, Piano ID layout GM SSO, Piano ID SSO confirm before, Piano ID Phone number is not confirmed, Piano ID Phone number Update, Piano ID custom form, Piano ID register page, Piano ID 2FA Digital code, Piano ID Alias login, Zuora offer, Newscycle offer, Newsletter signup, Push signup, Lost value offer, Final confirmation, Upgrade offer, Template, };
/* The type */
private String typeId = null;
//public enum typeIdEnum { default_offer, states, checkout_style, components, error, payment_components, promo_components, provider_components, term_components, receipt_template, already_has_access, external_verification_components, confirmation, auth, print_address_components, consent_components, gift_components, redemption_components, bank_secure_components, shared_subscription_components, licensing_landing_page, licensing_contract_list, licensing_redeem_result, upgrade_components, reactivate_subscription, upgrade_authentication_components, future_start_components, myaccount_style, myaccount_help, myaccount_common, myaccount_app, myaccount_library, myaccount_payments, myaccount_wallet, myaccount_voucher, myaccount_user_profile, myaccount_cash_payments, myaccount_error, myaccount_transactions, myaccount_licensing, piano_id_layout, piano_id_login, piano_id_register, piano_id_initiate_password_reset, piano_id_new_password, piano_id_error_page, piano_id_profile, piano_id_login_confirm, piano_id_register_confirm, piano_id_my_account, piano_id_custom_form, piano_id_email_is_not_confirmed, piano_id_email_confirmation_required, piano_id_email_confirmation, piano_id_passwordless_confirmation, piano_id_sso_confirm, piano_id_digital_code, piano_id_newsletters, piano_id_layout_gm_sso, piano_id_sso_confirm_before, piano_id_phone_is_not_confirmed, piano_id_phone_update, piano_id_custom_form_exp, piano_id_registration_exp, piano_id_2fa_email_digital_code, piano_id_alias_login, zuora_offer, newscycle_offer, newsletter_signup, push_signup, lost_value_offer, final_confirmation, upgrade_offer, template, };
/* The boilerplate type */
private String boilerplateType = null;
//public enum boilerplateTypeEnum { Offer list modal, Offer grid modal, Subscription prompt modal, Basic template modal, Zuora, Newscycle, Soft adblocker intercept modal, Hard adblocker intercept modal, Meter reminder modal, Meter expired modal, Newsletter signup modal, Frictionless Offer, Site Licensing Landing Page, Push Enrollment, Subscription modal prompt 1 - Upgrades, Banner - Upgrades, Subscription modal prompt 2 - Upgrades, Grid modal 2 - Upgrades, Grid modal 3 - Upgrades, LinkedIn Premium, LinkedIn Banner, LinkedIn Free, LinkedIn Offer, Credit redemption, Credit counter, OpenPass, Lost value - Churn prevention, Final confirmation, Upgrade offer 1, Upgrade offer 2, };
/* The ID of the boilerplate type */
private String boilerplateTypeId = null;
//public enum boilerplateTypeIdEnum { offer_list, offer_grid, subscription_prompt, basic, zuora, newscycle, soft_adblocker, hard_adblocker, meter_reminder, meter_expired, newsletter_signup, passwordless_offer, licensing_landing_page, push_enrollment, upgrade_downgrade_offer_subscription_prompt_modal_1, upgrade_downgrade_offer_banner, upgrade_downgrade_offer_subscription_prompt_modal_2, upgrade_downgrade_offer_grid_modal_2, upgrade_downgrade_offer_grid_modal_3, linkedin_premium, linkedin_banner, linkedin_free, linkedin_offer, credit_redemption, credit_counter, open_pass, churn_prevention_lost_value, churn_prevention_final_confirmation, upgrade_offer_1, upgrade_offer_2, };
/* The category ID */
private String categoryId = null;
//public enum categoryIdEnum { offer, social, registration, metering, newsletter, adblocker, licensing, push_enrollment, exp_piano_id, upgrade_offer, checkout, my_account, system, piano_id, affiliate, churn_prevention, };
/* The URL of the thumbnail image */
private String thumbnailImageUrl = null;
/* The URL of the live thumbnail image */
private String liveThumbnailImageUrl = null;
/* The status */
private String status = null;
//public enum statusEnum { active, archived, };
/* Published or not */
private Boolean isPublished = null;
/* The number of the template variants */
private Integer countVariants = null;
private List variantList = new ArrayList();
/* The archived date */
private Integer archivedDate = null;
private User archivedBy = null;
private List contentFieldList = new ArrayList();
/* Whether the object can be converted to global */
private Boolean canBeGlobal = null;
/* Whether the object is a global template */
private Boolean isGlobal = null;
/* Whether the object is an inherited template */
private Boolean isInherited = null;
public String getOfferTemplateId() {
return offerTemplateId;
}
public void setOfferTemplateId(String offerTemplateId) {
this.offerTemplateId = offerTemplateId;
}
public String getTokenType() {
return tokenType;
}
public void setTokenType(String tokenType) {
this.tokenType = tokenType;
}
public String getAid() {
return aid;
}
public void setAid(String aid) {
this.aid = aid;
}
public String getAppName() {
return appName;
}
public void setAppName(String appName) {
this.appName = appName;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Boolean getDeleted() {
return deleted;
}
public void setDeleted(Boolean deleted) {
this.deleted = deleted;
}
public Integer getCreateDate() {
return createDate;
}
public void setCreateDate(Integer createDate) {
this.createDate = createDate;
}
public User getCreateBy() {
return createBy;
}
public void setCreateBy(User createBy) {
this.createBy = createBy;
}
public Integer getUpdateDate() {
return updateDate;
}
public void setUpdateDate(Integer updateDate) {
this.updateDate = updateDate;
}
public User getUpdateBy() {
return updateBy;
}
public void setUpdateBy(User updateBy) {
this.updateBy = updateBy;
}
public Integer getPublishDate() {
return publishDate;
}
public void setPublishDate(Integer publishDate) {
this.publishDate = publishDate;
}
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getTypeId() {
return typeId;
}
public void setTypeId(String typeId) {
this.typeId = typeId;
}
public String getBoilerplateType() {
return boilerplateType;
}
public void setBoilerplateType(String boilerplateType) {
this.boilerplateType = boilerplateType;
}
public String getBoilerplateTypeId() {
return boilerplateTypeId;
}
public void setBoilerplateTypeId(String boilerplateTypeId) {
this.boilerplateTypeId = boilerplateTypeId;
}
public String getCategoryId() {
return categoryId;
}
public void setCategoryId(String categoryId) {
this.categoryId = categoryId;
}
public String getThumbnailImageUrl() {
return thumbnailImageUrl;
}
public void setThumbnailImageUrl(String thumbnailImageUrl) {
this.thumbnailImageUrl = thumbnailImageUrl;
}
public String getLiveThumbnailImageUrl() {
return liveThumbnailImageUrl;
}
public void setLiveThumbnailImageUrl(String liveThumbnailImageUrl) {
this.liveThumbnailImageUrl = liveThumbnailImageUrl;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public Boolean getIsPublished() {
return isPublished;
}
public void setIsPublished(Boolean isPublished) {
this.isPublished = isPublished;
}
public Integer getCountVariants() {
return countVariants;
}
public void setCountVariants(Integer countVariants) {
this.countVariants = countVariants;
}
public List getVariantList() {
return variantList;
}
public void setVariantList(List variantList) {
this.variantList = variantList;
}
public Integer getArchivedDate() {
return archivedDate;
}
public void setArchivedDate(Integer archivedDate) {
this.archivedDate = archivedDate;
}
public User getArchivedBy() {
return archivedBy;
}
public void setArchivedBy(User archivedBy) {
this.archivedBy = archivedBy;
}
public List getContentFieldList() {
return contentFieldList;
}
public void setContentFieldList(List contentFieldList) {
this.contentFieldList = contentFieldList;
}
public Boolean getCanBeGlobal() {
return canBeGlobal;
}
public void setCanBeGlobal(Boolean canBeGlobal) {
this.canBeGlobal = canBeGlobal;
}
public Boolean getIsGlobal() {
return isGlobal;
}
public void setIsGlobal(Boolean isGlobal) {
this.isGlobal = isGlobal;
}
public Boolean getIsInherited() {
return isInherited;
}
public void setIsInherited(Boolean isInherited) {
this.isInherited = isInherited;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class OfferTemplate {\n");
sb.append(" offerTemplateId: ").append(offerTemplateId).append("\n");
sb.append(" tokenType: ").append(tokenType).append("\n");
sb.append(" aid: ").append(aid).append("\n");
sb.append(" appName: ").append(appName).append("\n");
sb.append(" name: ").append(name).append("\n");
sb.append(" description: ").append(description).append("\n");
sb.append(" deleted: ").append(deleted).append("\n");
sb.append(" createDate: ").append(createDate).append("\n");
sb.append(" createBy: ").append(createBy).append("\n");
sb.append(" updateDate: ").append(updateDate).append("\n");
sb.append(" updateBy: ").append(updateBy).append("\n");
sb.append(" publishDate: ").append(publishDate).append("\n");
sb.append(" version: ").append(version).append("\n");
sb.append(" type: ").append(type).append("\n");
sb.append(" typeId: ").append(typeId).append("\n");
sb.append(" boilerplateType: ").append(boilerplateType).append("\n");
sb.append(" boilerplateTypeId: ").append(boilerplateTypeId).append("\n");
sb.append(" categoryId: ").append(categoryId).append("\n");
sb.append(" thumbnailImageUrl: ").append(thumbnailImageUrl).append("\n");
sb.append(" liveThumbnailImageUrl: ").append(liveThumbnailImageUrl).append("\n");
sb.append(" status: ").append(status).append("\n");
sb.append(" isPublished: ").append(isPublished).append("\n");
sb.append(" countVariants: ").append(countVariants).append("\n");
sb.append(" variantList: ").append(variantList).append("\n");
sb.append(" archivedDate: ").append(archivedDate).append("\n");
sb.append(" archivedBy: ").append(archivedBy).append("\n");
sb.append(" contentFieldList: ").append(contentFieldList).append("\n");
sb.append(" canBeGlobal: ").append(canBeGlobal).append("\n");
sb.append(" isGlobal: ").append(isGlobal).append("\n");
sb.append(" isInherited: ").append(isInherited).append("\n");
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy