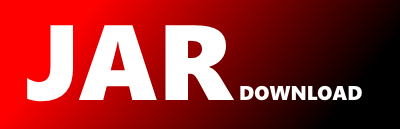
com.tinypass.client.publisher.model.SubscriptionRestrictions Maven / Gradle / Ivy
package com.tinypass.client.publisher.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
public class SubscriptionRestrictions {
/* Whether the app is allowed to change the next billing date of a subscription */
private Boolean allowChangeNextBillDate = null;
/* Whether the app can disable auto renew but cannot enable a previously disabled auto renew */
private Boolean allowEnableAutoRenew = null;
/* Whether the app is allowed to change a user's payment method for a subscriptions */
private Boolean allowSwitchPaymentMethod = null;
/* Whether the app is allowed to manually run a scheduled renewal for a subscription */
private Boolean allowSchedulerRenewals = null;
/* Whether the app is allowed to manually renew subscriptions having their next billing date in the future */
private Boolean allowFutureRenewals = null;
/* Whether the app is allowed to manually verify subscriptions having their next verification date in the future */
private Boolean allowVerifyNow = null;
/* Whether the app is allowed to activate subscription manually having their activation date in the future */
private Boolean allowActivateNow = null;
public Boolean getAllowChangeNextBillDate() {
return allowChangeNextBillDate;
}
public void setAllowChangeNextBillDate(Boolean allowChangeNextBillDate) {
this.allowChangeNextBillDate = allowChangeNextBillDate;
}
public Boolean getAllowEnableAutoRenew() {
return allowEnableAutoRenew;
}
public void setAllowEnableAutoRenew(Boolean allowEnableAutoRenew) {
this.allowEnableAutoRenew = allowEnableAutoRenew;
}
public Boolean getAllowSwitchPaymentMethod() {
return allowSwitchPaymentMethod;
}
public void setAllowSwitchPaymentMethod(Boolean allowSwitchPaymentMethod) {
this.allowSwitchPaymentMethod = allowSwitchPaymentMethod;
}
public Boolean getAllowSchedulerRenewals() {
return allowSchedulerRenewals;
}
public void setAllowSchedulerRenewals(Boolean allowSchedulerRenewals) {
this.allowSchedulerRenewals = allowSchedulerRenewals;
}
public Boolean getAllowFutureRenewals() {
return allowFutureRenewals;
}
public void setAllowFutureRenewals(Boolean allowFutureRenewals) {
this.allowFutureRenewals = allowFutureRenewals;
}
public Boolean getAllowVerifyNow() {
return allowVerifyNow;
}
public void setAllowVerifyNow(Boolean allowVerifyNow) {
this.allowVerifyNow = allowVerifyNow;
}
public Boolean getAllowActivateNow() {
return allowActivateNow;
}
public void setAllowActivateNow(Boolean allowActivateNow) {
this.allowActivateNow = allowActivateNow;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SubscriptionRestrictions {\n");
sb.append(" allowChangeNextBillDate: ").append(allowChangeNextBillDate).append("\n");
sb.append(" allowEnableAutoRenew: ").append(allowEnableAutoRenew).append("\n");
sb.append(" allowSwitchPaymentMethod: ").append(allowSwitchPaymentMethod).append("\n");
sb.append(" allowSchedulerRenewals: ").append(allowSchedulerRenewals).append("\n");
sb.append(" allowFutureRenewals: ").append(allowFutureRenewals).append("\n");
sb.append(" allowVerifyNow: ").append(allowVerifyNow).append("\n");
sb.append(" allowActivateNow: ").append(allowActivateNow).append("\n");
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy