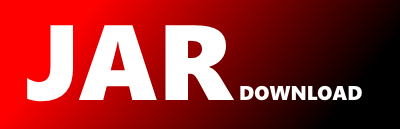
com.tinypass.client.publisher.model.TermConversionData Maven / Gradle / Ivy
package com.tinypass.client.publisher.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
public class TermConversionData {
/* The application ID */
private String aid = null;
/* The offer ID */
private String offerId = null;
/* The term ID */
private String termId = null;
/* The template ID */
private String offerTemplateId = null;
/* The template ID */
private String templateId = null;
/* The user ID */
private String uid = null;
/* The country of the user who converted the term */
private String userCountry = null;
/* The region of the user who converted the term */
private String userRegion = null;
/* The city of the user who converted the term */
private String userCity = null;
/* The zip code of the user who converted the term */
private String zip = null;
/* The latitude of the user's position */
private String latitude = null;
/* The longitude of the user's position */
private String longitude = null;
/* The user agent of the user who converted the term */
private String userAgent = null;
/* The user's locale */
private String locale = null;
/* The hour of the term conversion */
private String hour = null;
/* The URL of the page */
private String url = null;
/* The browser with which the conversion was made */
private String browser = null;
//public enum browserEnum { chrome, firefox, safari, ie, opera, bot, misc, };
/* The platform with which the conversion was made */
private String platform = null;
//public enum platformEnum { desktop, mobile, tablet, game_console, dmr, wearable, bot, other, };
/* The operating system with which the conversion was made */
private String operatingSystem = null;
//public enum operatingSystemEnum { windows, ios, android, mac, linux, other, others_desktop, other_mobile, bot, };
/* The tags of the page */
private String tags = null;
/* When the content was published */
private String contentCreated = null;
/* The author of the content */
private String contentAuthor = null;
/* The section for the content */
private String contentSection = null;
private List campaigns = new ArrayList();
public String getAid() {
return aid;
}
public void setAid(String aid) {
this.aid = aid;
}
public String getOfferId() {
return offerId;
}
public void setOfferId(String offerId) {
this.offerId = offerId;
}
public String getTermId() {
return termId;
}
public void setTermId(String termId) {
this.termId = termId;
}
public String getOfferTemplateId() {
return offerTemplateId;
}
public void setOfferTemplateId(String offerTemplateId) {
this.offerTemplateId = offerTemplateId;
}
public String getTemplateId() {
return templateId;
}
public void setTemplateId(String templateId) {
this.templateId = templateId;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getUserCountry() {
return userCountry;
}
public void setUserCountry(String userCountry) {
this.userCountry = userCountry;
}
public String getUserRegion() {
return userRegion;
}
public void setUserRegion(String userRegion) {
this.userRegion = userRegion;
}
public String getUserCity() {
return userCity;
}
public void setUserCity(String userCity) {
this.userCity = userCity;
}
public String getZip() {
return zip;
}
public void setZip(String zip) {
this.zip = zip;
}
public String getLatitude() {
return latitude;
}
public void setLatitude(String latitude) {
this.latitude = latitude;
}
public String getLongitude() {
return longitude;
}
public void setLongitude(String longitude) {
this.longitude = longitude;
}
public String getUserAgent() {
return userAgent;
}
public void setUserAgent(String userAgent) {
this.userAgent = userAgent;
}
public String getLocale() {
return locale;
}
public void setLocale(String locale) {
this.locale = locale;
}
public String getHour() {
return hour;
}
public void setHour(String hour) {
this.hour = hour;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getBrowser() {
return browser;
}
public void setBrowser(String browser) {
this.browser = browser;
}
public String getPlatform() {
return platform;
}
public void setPlatform(String platform) {
this.platform = platform;
}
public String getOperatingSystem() {
return operatingSystem;
}
public void setOperatingSystem(String operatingSystem) {
this.operatingSystem = operatingSystem;
}
public String getTags() {
return tags;
}
public void setTags(String tags) {
this.tags = tags;
}
public String getContentCreated() {
return contentCreated;
}
public void setContentCreated(String contentCreated) {
this.contentCreated = contentCreated;
}
public String getContentAuthor() {
return contentAuthor;
}
public void setContentAuthor(String contentAuthor) {
this.contentAuthor = contentAuthor;
}
public String getContentSection() {
return contentSection;
}
public void setContentSection(String contentSection) {
this.contentSection = contentSection;
}
public List getCampaigns() {
return campaigns;
}
public void setCampaigns(List campaigns) {
this.campaigns = campaigns;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TermConversionData {\n");
sb.append(" aid: ").append(aid).append("\n");
sb.append(" offerId: ").append(offerId).append("\n");
sb.append(" termId: ").append(termId).append("\n");
sb.append(" offerTemplateId: ").append(offerTemplateId).append("\n");
sb.append(" templateId: ").append(templateId).append("\n");
sb.append(" uid: ").append(uid).append("\n");
sb.append(" userCountry: ").append(userCountry).append("\n");
sb.append(" userRegion: ").append(userRegion).append("\n");
sb.append(" userCity: ").append(userCity).append("\n");
sb.append(" zip: ").append(zip).append("\n");
sb.append(" latitude: ").append(latitude).append("\n");
sb.append(" longitude: ").append(longitude).append("\n");
sb.append(" userAgent: ").append(userAgent).append("\n");
sb.append(" locale: ").append(locale).append("\n");
sb.append(" hour: ").append(hour).append("\n");
sb.append(" url: ").append(url).append("\n");
sb.append(" browser: ").append(browser).append("\n");
sb.append(" platform: ").append(platform).append("\n");
sb.append(" operatingSystem: ").append(operatingSystem).append("\n");
sb.append(" tags: ").append(tags).append("\n");
sb.append(" contentCreated: ").append(contentCreated).append("\n");
sb.append(" contentAuthor: ").append(contentAuthor).append("\n");
sb.append(" contentSection: ").append(contentSection).append("\n");
sb.append(" campaigns: ").append(campaigns).append("\n");
sb.append("}\n");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy