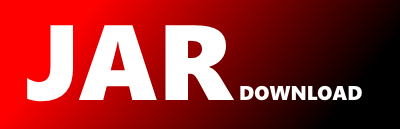
io.pinecone.proto.Core Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pinecone-client Show documentation
Show all versions of pinecone-client Show documentation
The Pinecone.io Java Client
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: core.proto
package io.pinecone.proto;
public final class Core {
private Core() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ServiceControlRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.ServiceControlRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name of the function.
*
*
* string function = 1;
* @return The function.
*/
java.lang.String getFunction();
/**
*
* Name of the function.
*
*
* string function = 1;
* @return The bytes for function.
*/
com.google.protobuf.ByteString
getFunctionBytes();
/**
*
* Unique id of the function (if any).
*
*
* uint64 function_id = 2;
* @return The functionId.
*/
long getFunctionId();
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
* @return The status.
*/
io.pinecone.proto.Core.Status getStatus();
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
io.pinecone.proto.Core.StatusOrBuilder getStatusOrBuilder();
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return The service.
*/
java.lang.String getService();
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return The bytes for service.
*/
com.google.protobuf.ByteString
getServiceBytes();
}
/**
*
**
*Request from the controller to individual service for monitoring, updating, and terminating running services.
*
*
* Protobuf type {@code core.ServiceControlRequest}
*/
public static final class ServiceControlRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.ServiceControlRequest)
ServiceControlRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ServiceControlRequest.newBuilder() to construct.
private ServiceControlRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ServiceControlRequest() {
function_ = "";
service_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ServiceControlRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ServiceControlRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
function_ = s;
break;
}
case 16: {
functionId_ = input.readUInt64();
break;
}
case 26: {
io.pinecone.proto.Core.Status.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(io.pinecone.proto.Core.Status.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
service_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_ServiceControlRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_ServiceControlRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.ServiceControlRequest.class, io.pinecone.proto.Core.ServiceControlRequest.Builder.class);
}
public static final int FUNCTION_FIELD_NUMBER = 1;
private volatile java.lang.Object function_;
/**
*
* Name of the function.
*
*
* string function = 1;
* @return The function.
*/
@java.lang.Override
public java.lang.String getFunction() {
java.lang.Object ref = function_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
function_ = s;
return s;
}
}
/**
*
* Name of the function.
*
*
* string function = 1;
* @return The bytes for function.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFunctionBytes() {
java.lang.Object ref = function_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
function_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FUNCTION_ID_FIELD_NUMBER = 2;
private long functionId_;
/**
*
* Unique id of the function (if any).
*
*
* uint64 function_id = 2;
* @return The functionId.
*/
@java.lang.Override
public long getFunctionId() {
return functionId_;
}
public static final int STATUS_FIELD_NUMBER = 3;
private io.pinecone.proto.Core.Status status_;
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
* @return The status.
*/
@java.lang.Override
public io.pinecone.proto.Core.Status getStatus() {
return status_ == null ? io.pinecone.proto.Core.Status.getDefaultInstance() : status_;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.StatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int SERVICE_FIELD_NUMBER = 4;
private volatile java.lang.Object service_;
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return The service.
*/
@java.lang.Override
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
service_ = s;
return s;
}
}
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return The bytes for service.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getFunctionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, function_);
}
if (functionId_ != 0L) {
output.writeUInt64(2, functionId_);
}
if (status_ != null) {
output.writeMessage(3, getStatus());
}
if (!getServiceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, service_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getFunctionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, function_);
}
if (functionId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, functionId_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStatus());
}
if (!getServiceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, service_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.ServiceControlRequest)) {
return super.equals(obj);
}
io.pinecone.proto.Core.ServiceControlRequest other = (io.pinecone.proto.Core.ServiceControlRequest) obj;
if (!getFunction()
.equals(other.getFunction())) return false;
if (getFunctionId()
!= other.getFunctionId()) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!getService()
.equals(other.getService())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getFunction().hashCode();
hash = (37 * hash) + FUNCTION_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFunctionId());
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.ServiceControlRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.ServiceControlRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
*Request from the controller to individual service for monitoring, updating, and terminating running services.
*
*
* Protobuf type {@code core.ServiceControlRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.ServiceControlRequest)
io.pinecone.proto.Core.ServiceControlRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_ServiceControlRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_ServiceControlRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.ServiceControlRequest.class, io.pinecone.proto.Core.ServiceControlRequest.Builder.class);
}
// Construct using io.pinecone.proto.Core.ServiceControlRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
function_ = "";
functionId_ = 0L;
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
service_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_ServiceControlRequest_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.ServiceControlRequest getDefaultInstanceForType() {
return io.pinecone.proto.Core.ServiceControlRequest.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.ServiceControlRequest build() {
io.pinecone.proto.Core.ServiceControlRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.ServiceControlRequest buildPartial() {
io.pinecone.proto.Core.ServiceControlRequest result = new io.pinecone.proto.Core.ServiceControlRequest(this);
result.function_ = function_;
result.functionId_ = functionId_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
result.service_ = service_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.ServiceControlRequest) {
return mergeFrom((io.pinecone.proto.Core.ServiceControlRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.ServiceControlRequest other) {
if (other == io.pinecone.proto.Core.ServiceControlRequest.getDefaultInstance()) return this;
if (!other.getFunction().isEmpty()) {
function_ = other.function_;
onChanged();
}
if (other.getFunctionId() != 0L) {
setFunctionId(other.getFunctionId());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (!other.getService().isEmpty()) {
service_ = other.service_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.ServiceControlRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.ServiceControlRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object function_ = "";
/**
*
* Name of the function.
*
*
* string function = 1;
* @return The function.
*/
public java.lang.String getFunction() {
java.lang.Object ref = function_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
function_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the function.
*
*
* string function = 1;
* @return The bytes for function.
*/
public com.google.protobuf.ByteString
getFunctionBytes() {
java.lang.Object ref = function_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
function_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the function.
*
*
* string function = 1;
* @param value The function to set.
* @return This builder for chaining.
*/
public Builder setFunction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
function_ = value;
onChanged();
return this;
}
/**
*
* Name of the function.
*
*
* string function = 1;
* @return This builder for chaining.
*/
public Builder clearFunction() {
function_ = getDefaultInstance().getFunction();
onChanged();
return this;
}
/**
*
* Name of the function.
*
*
* string function = 1;
* @param value The bytes for function to set.
* @return This builder for chaining.
*/
public Builder setFunctionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
function_ = value;
onChanged();
return this;
}
private long functionId_ ;
/**
*
* Unique id of the function (if any).
*
*
* uint64 function_id = 2;
* @return The functionId.
*/
@java.lang.Override
public long getFunctionId() {
return functionId_;
}
/**
*
* Unique id of the function (if any).
*
*
* uint64 function_id = 2;
* @param value The functionId to set.
* @return This builder for chaining.
*/
public Builder setFunctionId(long value) {
functionId_ = value;
onChanged();
return this;
}
/**
*
* Unique id of the function (if any).
*
*
* uint64 function_id = 2;
* @return This builder for chaining.
*/
public Builder clearFunctionId() {
functionId_ = 0L;
onChanged();
return this;
}
private io.pinecone.proto.Core.Status status_;
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Status, io.pinecone.proto.Core.Status.Builder, io.pinecone.proto.Core.StatusOrBuilder> statusBuilder_;
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
* @return The status.
*/
public io.pinecone.proto.Core.Status getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? io.pinecone.proto.Core.Status.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
public Builder setStatus(io.pinecone.proto.Core.Status value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
public Builder setStatus(
io.pinecone.proto.Core.Status.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
public Builder mergeStatus(io.pinecone.proto.Core.Status value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
io.pinecone.proto.Core.Status.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
public io.pinecone.proto.Core.Status.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
public io.pinecone.proto.Core.StatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
io.pinecone.proto.Core.Status.getDefaultInstance() : status_;
}
}
/**
*
* Status of the function.
*
*
* .core.Status status = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Status, io.pinecone.proto.Core.Status.Builder, io.pinecone.proto.Core.StatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Status, io.pinecone.proto.Core.Status.Builder, io.pinecone.proto.Core.StatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private java.lang.Object service_ = "";
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return The service.
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
service_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return The bytes for service.
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @param value The service to set.
* @return This builder for chaining.
*/
public Builder setService(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
service_ = value;
onChanged();
return this;
}
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @return This builder for chaining.
*/
public Builder clearService() {
service_ = getDefaultInstance().getService();
onChanged();
return this;
}
/**
*
* Name of the service the function belongs to.
*
*
* string service = 4;
* @param value The bytes for service to set.
* @return This builder for chaining.
*/
public Builder setServiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
service_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.ServiceControlRequest)
}
// @@protoc_insertion_point(class_scope:core.ServiceControlRequest)
private static final io.pinecone.proto.Core.ServiceControlRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.ServiceControlRequest();
}
public static io.pinecone.proto.Core.ServiceControlRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ServiceControlRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ServiceControlRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.ServiceControlRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NdArrayOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.NdArray)
com.google.protobuf.MessageOrBuilder {
/**
*
* the actual array data, in bytes
*
*
* bytes buffer = 1;
* @return The buffer.
*/
com.google.protobuf.ByteString getBuffer();
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return A list containing the shape.
*/
java.util.List getShapeList();
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return The count of shape.
*/
int getShapeCount();
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @param index The index of the element to return.
* @return The shape at the given index.
*/
int getShape(int index);
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return The dtype.
*/
java.lang.String getDtype();
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return The bytes for dtype.
*/
com.google.protobuf.ByteString
getDtypeBytes();
/**
*
* whether lz4 compression is used on buffer
*
*
* bool compressed = 4;
* @return The compressed.
*/
boolean getCompressed();
}
/**
*
**
* Represents the a numpy ndarray
*
*
* Protobuf type {@code core.NdArray}
*/
public static final class NdArray extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.NdArray)
NdArrayOrBuilder {
private static final long serialVersionUID = 0L;
// Use NdArray.newBuilder() to construct.
private NdArray(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NdArray() {
buffer_ = com.google.protobuf.ByteString.EMPTY;
shape_ = emptyIntList();
dtype_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NdArray();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NdArray(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
buffer_ = input.readBytes();
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
shape_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
shape_.addInt(input.readUInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
shape_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
shape_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
dtype_ = s;
break;
}
case 32: {
compressed_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
shape_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_NdArray_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_NdArray_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.NdArray.class, io.pinecone.proto.Core.NdArray.Builder.class);
}
public static final int BUFFER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString buffer_;
/**
*
* the actual array data, in bytes
*
*
* bytes buffer = 1;
* @return The buffer.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBuffer() {
return buffer_;
}
public static final int SHAPE_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.IntList shape_;
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return A list containing the shape.
*/
@java.lang.Override
public java.util.List
getShapeList() {
return shape_;
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return The count of shape.
*/
public int getShapeCount() {
return shape_.size();
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @param index The index of the element to return.
* @return The shape at the given index.
*/
public int getShape(int index) {
return shape_.getInt(index);
}
private int shapeMemoizedSerializedSize = -1;
public static final int DTYPE_FIELD_NUMBER = 3;
private volatile java.lang.Object dtype_;
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return The dtype.
*/
@java.lang.Override
public java.lang.String getDtype() {
java.lang.Object ref = dtype_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dtype_ = s;
return s;
}
}
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return The bytes for dtype.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDtypeBytes() {
java.lang.Object ref = dtype_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dtype_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPRESSED_FIELD_NUMBER = 4;
private boolean compressed_;
/**
*
* whether lz4 compression is used on buffer
*
*
* bool compressed = 4;
* @return The compressed.
*/
@java.lang.Override
public boolean getCompressed() {
return compressed_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!buffer_.isEmpty()) {
output.writeBytes(1, buffer_);
}
if (getShapeList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(shapeMemoizedSerializedSize);
}
for (int i = 0; i < shape_.size(); i++) {
output.writeUInt32NoTag(shape_.getInt(i));
}
if (!getDtypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, dtype_);
}
if (compressed_ != false) {
output.writeBool(4, compressed_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!buffer_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, buffer_);
}
{
int dataSize = 0;
for (int i = 0; i < shape_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(shape_.getInt(i));
}
size += dataSize;
if (!getShapeList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
shapeMemoizedSerializedSize = dataSize;
}
if (!getDtypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, dtype_);
}
if (compressed_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, compressed_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.NdArray)) {
return super.equals(obj);
}
io.pinecone.proto.Core.NdArray other = (io.pinecone.proto.Core.NdArray) obj;
if (!getBuffer()
.equals(other.getBuffer())) return false;
if (!getShapeList()
.equals(other.getShapeList())) return false;
if (!getDtype()
.equals(other.getDtype())) return false;
if (getCompressed()
!= other.getCompressed()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BUFFER_FIELD_NUMBER;
hash = (53 * hash) + getBuffer().hashCode();
if (getShapeCount() > 0) {
hash = (37 * hash) + SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getShapeList().hashCode();
}
hash = (37 * hash) + DTYPE_FIELD_NUMBER;
hash = (53 * hash) + getDtype().hashCode();
hash = (37 * hash) + COMPRESSED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getCompressed());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.NdArray parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.NdArray parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.NdArray parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.NdArray parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.NdArray parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.NdArray parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.NdArray prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents the a numpy ndarray
*
*
* Protobuf type {@code core.NdArray}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.NdArray)
io.pinecone.proto.Core.NdArrayOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_NdArray_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_NdArray_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.NdArray.class, io.pinecone.proto.Core.NdArray.Builder.class);
}
// Construct using io.pinecone.proto.Core.NdArray.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
buffer_ = com.google.protobuf.ByteString.EMPTY;
shape_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
dtype_ = "";
compressed_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_NdArray_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.NdArray getDefaultInstanceForType() {
return io.pinecone.proto.Core.NdArray.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.NdArray build() {
io.pinecone.proto.Core.NdArray result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.NdArray buildPartial() {
io.pinecone.proto.Core.NdArray result = new io.pinecone.proto.Core.NdArray(this);
int from_bitField0_ = bitField0_;
result.buffer_ = buffer_;
if (((bitField0_ & 0x00000001) != 0)) {
shape_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.shape_ = shape_;
result.dtype_ = dtype_;
result.compressed_ = compressed_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.NdArray) {
return mergeFrom((io.pinecone.proto.Core.NdArray)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.NdArray other) {
if (other == io.pinecone.proto.Core.NdArray.getDefaultInstance()) return this;
if (other.getBuffer() != com.google.protobuf.ByteString.EMPTY) {
setBuffer(other.getBuffer());
}
if (!other.shape_.isEmpty()) {
if (shape_.isEmpty()) {
shape_ = other.shape_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureShapeIsMutable();
shape_.addAll(other.shape_);
}
onChanged();
}
if (!other.getDtype().isEmpty()) {
dtype_ = other.dtype_;
onChanged();
}
if (other.getCompressed() != false) {
setCompressed(other.getCompressed());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.NdArray parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.NdArray) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString buffer_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* the actual array data, in bytes
*
*
* bytes buffer = 1;
* @return The buffer.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBuffer() {
return buffer_;
}
/**
*
* the actual array data, in bytes
*
*
* bytes buffer = 1;
* @param value The buffer to set.
* @return This builder for chaining.
*/
public Builder setBuffer(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
buffer_ = value;
onChanged();
return this;
}
/**
*
* the actual array data, in bytes
*
*
* bytes buffer = 1;
* @return This builder for chaining.
*/
public Builder clearBuffer() {
buffer_ = getDefaultInstance().getBuffer();
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList shape_ = emptyIntList();
private void ensureShapeIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
shape_ = mutableCopy(shape_);
bitField0_ |= 0x00000001;
}
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return A list containing the shape.
*/
public java.util.List
getShapeList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(shape_) : shape_;
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return The count of shape.
*/
public int getShapeCount() {
return shape_.size();
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @param index The index of the element to return.
* @return The shape at the given index.
*/
public int getShape(int index) {
return shape_.getInt(index);
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @param index The index to set the value at.
* @param value The shape to set.
* @return This builder for chaining.
*/
public Builder setShape(
int index, int value) {
ensureShapeIsMutable();
shape_.setInt(index, value);
onChanged();
return this;
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @param value The shape to add.
* @return This builder for chaining.
*/
public Builder addShape(int value) {
ensureShapeIsMutable();
shape_.addInt(value);
onChanged();
return this;
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @param values The shape to add.
* @return This builder for chaining.
*/
public Builder addAllShape(
java.lang.Iterable extends java.lang.Integer> values) {
ensureShapeIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, shape_);
onChanged();
return this;
}
/**
*
* the shape (dimensions) of the array
*
*
* repeated uint32 shape = 2;
* @return This builder for chaining.
*/
public Builder clearShape() {
shape_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private java.lang.Object dtype_ = "";
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return The dtype.
*/
public java.lang.String getDtype() {
java.lang.Object ref = dtype_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
dtype_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return The bytes for dtype.
*/
public com.google.protobuf.ByteString
getDtypeBytes() {
java.lang.Object ref = dtype_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dtype_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @param value The dtype to set.
* @return This builder for chaining.
*/
public Builder setDtype(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
dtype_ = value;
onChanged();
return this;
}
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @return This builder for chaining.
*/
public Builder clearDtype() {
dtype_ = getDefaultInstance().getDtype();
onChanged();
return this;
}
/**
*
* the data type of the array
*
*
* string dtype = 3;
* @param value The bytes for dtype to set.
* @return This builder for chaining.
*/
public Builder setDtypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
dtype_ = value;
onChanged();
return this;
}
private boolean compressed_ ;
/**
*
* whether lz4 compression is used on buffer
*
*
* bool compressed = 4;
* @return The compressed.
*/
@java.lang.Override
public boolean getCompressed() {
return compressed_;
}
/**
*
* whether lz4 compression is used on buffer
*
*
* bool compressed = 4;
* @param value The compressed to set.
* @return This builder for chaining.
*/
public Builder setCompressed(boolean value) {
compressed_ = value;
onChanged();
return this;
}
/**
*
* whether lz4 compression is used on buffer
*
*
* bool compressed = 4;
* @return This builder for chaining.
*/
public Builder clearCompressed() {
compressed_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.NdArray)
}
// @@protoc_insertion_point(class_scope:core.NdArray)
private static final io.pinecone.proto.Core.NdArray DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.NdArray();
}
public static io.pinecone.proto.Core.NdArray getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NdArray parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NdArray(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.NdArray getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RouteOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.Route)
com.google.protobuf.MessageOrBuilder {
/**
*
* the name of the Service
*
*
* string function = 1;
* @return The function.
*/
java.lang.String getFunction();
/**
*
* the name of the Service
*
*
* string function = 1;
* @return The bytes for function.
*/
com.google.protobuf.ByteString
getFunctionBytes();
/**
*
* the id of the Service
*
*
* int32 function_id = 2;
* @return The functionId.
*/
int getFunctionId();
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
* @return Whether the startTime field is set.
*/
boolean hasStartTime();
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
* @return The startTime.
*/
com.google.protobuf.Timestamp getStartTime();
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder();
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
* @return Whether the endTime field is set.
*/
boolean hasEndTime();
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
* @return The endTime.
*/
com.google.protobuf.Timestamp getEndTime();
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
com.google.protobuf.TimestampOrBuilder getEndTimeOrBuilder();
}
/**
*
**
* Represents the route paths of this message
*
*
* Protobuf type {@code core.Route}
*/
public static final class Route extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.Route)
RouteOrBuilder {
private static final long serialVersionUID = 0L;
// Use Route.newBuilder() to construct.
private Route(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Route() {
function_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Route();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Route(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
function_ = s;
break;
}
case 16: {
functionId_ = input.readInt32();
break;
}
case 26: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (startTime_ != null) {
subBuilder = startTime_.toBuilder();
}
startTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(startTime_);
startTime_ = subBuilder.buildPartial();
}
break;
}
case 34: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (endTime_ != null) {
subBuilder = endTime_.toBuilder();
}
endTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(endTime_);
endTime_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Route_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Route_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Route.class, io.pinecone.proto.Core.Route.Builder.class);
}
public static final int FUNCTION_FIELD_NUMBER = 1;
private volatile java.lang.Object function_;
/**
*
* the name of the Service
*
*
* string function = 1;
* @return The function.
*/
@java.lang.Override
public java.lang.String getFunction() {
java.lang.Object ref = function_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
function_ = s;
return s;
}
}
/**
*
* the name of the Service
*
*
* string function = 1;
* @return The bytes for function.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFunctionBytes() {
java.lang.Object ref = function_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
function_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FUNCTION_ID_FIELD_NUMBER = 2;
private int functionId_;
/**
*
* the id of the Service
*
*
* int32 function_id = 2;
* @return The functionId.
*/
@java.lang.Override
public int getFunctionId() {
return functionId_;
}
public static final int START_TIME_FIELD_NUMBER = 3;
private com.google.protobuf.Timestamp startTime_;
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
* @return Whether the startTime field is set.
*/
@java.lang.Override
public boolean hasStartTime() {
return startTime_ != null;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
* @return The startTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStartTime() {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
return getStartTime();
}
public static final int END_TIME_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp endTime_;
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
* @return Whether the endTime field is set.
*/
@java.lang.Override
public boolean hasEndTime() {
return endTime_ != null;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
* @return The endTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getEndTime() {
return endTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : endTime_;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getEndTimeOrBuilder() {
return getEndTime();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getFunctionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, function_);
}
if (functionId_ != 0) {
output.writeInt32(2, functionId_);
}
if (startTime_ != null) {
output.writeMessage(3, getStartTime());
}
if (endTime_ != null) {
output.writeMessage(4, getEndTime());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getFunctionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, function_);
}
if (functionId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, functionId_);
}
if (startTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStartTime());
}
if (endTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getEndTime());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.Route)) {
return super.equals(obj);
}
io.pinecone.proto.Core.Route other = (io.pinecone.proto.Core.Route) obj;
if (!getFunction()
.equals(other.getFunction())) return false;
if (getFunctionId()
!= other.getFunctionId()) return false;
if (hasStartTime() != other.hasStartTime()) return false;
if (hasStartTime()) {
if (!getStartTime()
.equals(other.getStartTime())) return false;
}
if (hasEndTime() != other.hasEndTime()) return false;
if (hasEndTime()) {
if (!getEndTime()
.equals(other.getEndTime())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getFunction().hashCode();
hash = (37 * hash) + FUNCTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getFunctionId();
if (hasStartTime()) {
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getStartTime().hashCode();
}
if (hasEndTime()) {
hash = (37 * hash) + END_TIME_FIELD_NUMBER;
hash = (53 * hash) + getEndTime().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.Route parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Route parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Route parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Route parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Route parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Route parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Route parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Route parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Route parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Route parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Route parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Route parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.Route prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents the route paths of this message
*
*
* Protobuf type {@code core.Route}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.Route)
io.pinecone.proto.Core.RouteOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Route_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Route_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Route.class, io.pinecone.proto.Core.Route.Builder.class);
}
// Construct using io.pinecone.proto.Core.Route.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
function_ = "";
functionId_ = 0;
if (startTimeBuilder_ == null) {
startTime_ = null;
} else {
startTime_ = null;
startTimeBuilder_ = null;
}
if (endTimeBuilder_ == null) {
endTime_ = null;
} else {
endTime_ = null;
endTimeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_Route_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.Route getDefaultInstanceForType() {
return io.pinecone.proto.Core.Route.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.Route build() {
io.pinecone.proto.Core.Route result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.Route buildPartial() {
io.pinecone.proto.Core.Route result = new io.pinecone.proto.Core.Route(this);
result.function_ = function_;
result.functionId_ = functionId_;
if (startTimeBuilder_ == null) {
result.startTime_ = startTime_;
} else {
result.startTime_ = startTimeBuilder_.build();
}
if (endTimeBuilder_ == null) {
result.endTime_ = endTime_;
} else {
result.endTime_ = endTimeBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.Route) {
return mergeFrom((io.pinecone.proto.Core.Route)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.Route other) {
if (other == io.pinecone.proto.Core.Route.getDefaultInstance()) return this;
if (!other.getFunction().isEmpty()) {
function_ = other.function_;
onChanged();
}
if (other.getFunctionId() != 0) {
setFunctionId(other.getFunctionId());
}
if (other.hasStartTime()) {
mergeStartTime(other.getStartTime());
}
if (other.hasEndTime()) {
mergeEndTime(other.getEndTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.Route parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.Route) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object function_ = "";
/**
*
* the name of the Service
*
*
* string function = 1;
* @return The function.
*/
public java.lang.String getFunction() {
java.lang.Object ref = function_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
function_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the name of the Service
*
*
* string function = 1;
* @return The bytes for function.
*/
public com.google.protobuf.ByteString
getFunctionBytes() {
java.lang.Object ref = function_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
function_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the name of the Service
*
*
* string function = 1;
* @param value The function to set.
* @return This builder for chaining.
*/
public Builder setFunction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
function_ = value;
onChanged();
return this;
}
/**
*
* the name of the Service
*
*
* string function = 1;
* @return This builder for chaining.
*/
public Builder clearFunction() {
function_ = getDefaultInstance().getFunction();
onChanged();
return this;
}
/**
*
* the name of the Service
*
*
* string function = 1;
* @param value The bytes for function to set.
* @return This builder for chaining.
*/
public Builder setFunctionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
function_ = value;
onChanged();
return this;
}
private int functionId_ ;
/**
*
* the id of the Service
*
*
* int32 function_id = 2;
* @return The functionId.
*/
@java.lang.Override
public int getFunctionId() {
return functionId_;
}
/**
*
* the id of the Service
*
*
* int32 function_id = 2;
* @param value The functionId to set.
* @return This builder for chaining.
*/
public Builder setFunctionId(int value) {
functionId_ = value;
onChanged();
return this;
}
/**
*
* the id of the Service
*
*
* int32 function_id = 2;
* @return This builder for chaining.
*/
public Builder clearFunctionId() {
functionId_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp startTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> startTimeBuilder_;
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
* @return Whether the startTime field is set.
*/
public boolean hasStartTime() {
return startTimeBuilder_ != null || startTime_ != null;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
* @return The startTime.
*/
public com.google.protobuf.Timestamp getStartTime() {
if (startTimeBuilder_ == null) {
return startTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
} else {
return startTimeBuilder_.getMessage();
}
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
public Builder setStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startTime_ = value;
onChanged();
} else {
startTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
public Builder setStartTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (startTimeBuilder_ == null) {
startTime_ = builderForValue.build();
onChanged();
} else {
startTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
public Builder mergeStartTime(com.google.protobuf.Timestamp value) {
if (startTimeBuilder_ == null) {
if (startTime_ != null) {
startTime_ =
com.google.protobuf.Timestamp.newBuilder(startTime_).mergeFrom(value).buildPartial();
} else {
startTime_ = value;
}
onChanged();
} else {
startTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
public Builder clearStartTime() {
if (startTimeBuilder_ == null) {
startTime_ = null;
onChanged();
} else {
startTime_ = null;
startTimeBuilder_ = null;
}
return this;
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
public com.google.protobuf.Timestamp.Builder getStartTimeBuilder() {
onChanged();
return getStartTimeFieldBuilder().getBuilder();
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
public com.google.protobuf.TimestampOrBuilder getStartTimeOrBuilder() {
if (startTimeBuilder_ != null) {
return startTimeBuilder_.getMessageOrBuilder();
} else {
return startTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : startTime_;
}
}
/**
*
* receiving time
*
*
* .google.protobuf.Timestamp start_time = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getStartTimeFieldBuilder() {
if (startTimeBuilder_ == null) {
startTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getStartTime(),
getParentForChildren(),
isClean());
startTime_ = null;
}
return startTimeBuilder_;
}
private com.google.protobuf.Timestamp endTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> endTimeBuilder_;
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
* @return Whether the endTime field is set.
*/
public boolean hasEndTime() {
return endTimeBuilder_ != null || endTime_ != null;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
* @return The endTime.
*/
public com.google.protobuf.Timestamp getEndTime() {
if (endTimeBuilder_ == null) {
return endTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : endTime_;
} else {
return endTimeBuilder_.getMessage();
}
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
public Builder setEndTime(com.google.protobuf.Timestamp value) {
if (endTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endTime_ = value;
onChanged();
} else {
endTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
public Builder setEndTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (endTimeBuilder_ == null) {
endTime_ = builderForValue.build();
onChanged();
} else {
endTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
public Builder mergeEndTime(com.google.protobuf.Timestamp value) {
if (endTimeBuilder_ == null) {
if (endTime_ != null) {
endTime_ =
com.google.protobuf.Timestamp.newBuilder(endTime_).mergeFrom(value).buildPartial();
} else {
endTime_ = value;
}
onChanged();
} else {
endTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
public Builder clearEndTime() {
if (endTimeBuilder_ == null) {
endTime_ = null;
onChanged();
} else {
endTime_ = null;
endTimeBuilder_ = null;
}
return this;
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
public com.google.protobuf.Timestamp.Builder getEndTimeBuilder() {
onChanged();
return getEndTimeFieldBuilder().getBuilder();
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
public com.google.protobuf.TimestampOrBuilder getEndTimeOrBuilder() {
if (endTimeBuilder_ != null) {
return endTimeBuilder_.getMessageOrBuilder();
} else {
return endTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : endTime_;
}
}
/**
*
* sending (out) time
*
*
* .google.protobuf.Timestamp end_time = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getEndTimeFieldBuilder() {
if (endTimeBuilder_ == null) {
endTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getEndTime(),
getParentForChildren(),
isClean());
endTime_ = null;
}
return endTimeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.Route)
}
// @@protoc_insertion_point(class_scope:core.Route)
private static final io.pinecone.proto.Core.Route DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.Route();
}
public static io.pinecone.proto.Core.Route getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Route parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Route(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.Route getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.Request)
com.google.protobuf.MessageOrBuilder {
/**
*
* unique id of the request
*
*
* uint64 request_id = 1;
* @return The requestId.
*/
long getRequestId();
/**
*
* timeout in second until this message is dropped
*
*
* uint32 timeout = 2;
* @return The timeout.
*/
int getTimeout();
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return The path.
*/
java.lang.String getPath();
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* request schema version
*
*
* string version = 4;
* @return The version.
*/
java.lang.String getVersion();
/**
*
* request schema version
*
*
* string version = 4;
* @return The bytes for version.
*/
com.google.protobuf.ByteString
getVersionBytes();
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
java.util.List
getRoutesList();
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
io.pinecone.proto.Core.Route getRoutes(int index);
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
int getRoutesCount();
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
java.util.List extends io.pinecone.proto.Core.RouteOrBuilder>
getRoutesOrBuilderList();
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
io.pinecone.proto.Core.RouteOrBuilder getRoutesOrBuilder(
int index);
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
* @return The status.
*/
io.pinecone.proto.Core.Status getStatus();
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
io.pinecone.proto.Core.StatusOrBuilder getStatusOrBuilder();
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
* @return Whether the query field is set.
*/
boolean hasQuery();
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
* @return The query.
*/
io.pinecone.proto.Core.QueryRequest getQuery();
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
io.pinecone.proto.Core.QueryRequestOrBuilder getQueryOrBuilder();
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
* @return Whether the index field is set.
*/
boolean hasIndex();
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
* @return The index.
*/
io.pinecone.proto.Core.IndexRequest getIndex();
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
io.pinecone.proto.Core.IndexRequestOrBuilder getIndexOrBuilder();
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
* @return Whether the delete field is set.
*/
boolean hasDelete();
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
* @return The delete.
*/
io.pinecone.proto.Core.DeleteRequest getDelete();
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
io.pinecone.proto.Core.DeleteRequestOrBuilder getDeleteOrBuilder();
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
* @return Whether the info field is set.
*/
boolean hasInfo();
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
* @return The info.
*/
io.pinecone.proto.Core.InfoRequest getInfo();
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
io.pinecone.proto.Core.InfoRequestOrBuilder getInfoOrBuilder();
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
* @return Whether the fetch field is set.
*/
boolean hasFetch();
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
* @return The fetch.
*/
io.pinecone.proto.Core.FetchRequest getFetch();
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
io.pinecone.proto.Core.FetchRequestOrBuilder getFetchOrBuilder();
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return The namespace.
*/
java.lang.String getNamespace();
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return The bytes for namespace.
*/
com.google.protobuf.ByteString
getNamespaceBytes();
/**
*
* Integer ID that represents the client connection. Assigned by gateway.
*
*
* uint32 client_id = 13;
* @return The clientId.
*/
int getClientId();
/**
*
* Offset of request within client connection. Assigned by gateway.
*
*
* uint32 client_offset = 14;
* @return The clientOffset.
*/
int getClientOffset();
/**
*
* Shard the message came from. Used by aggregator
*
*
* uint32 shard_num = 15;
* @return The shardNum.
*/
int getShardNum();
/**
*
* Tail of gateway
*
*
* uint32 gateway_num = 16;
* @return The gatewayNum.
*/
int getGatewayNum();
/**
*
**
*For tracing only
*
*
* uint64 telemetry_trace_id = 17;
* @return The telemetryTraceId.
*/
long getTelemetryTraceId();
/**
* uint64 telemetry_parent_id = 18;
* @return The telemetryParentId.
*/
long getTelemetryParentId();
/**
* string service_name = 19;
* @return The serviceName.
*/
java.lang.String getServiceName();
/**
* string service_name = 19;
* @return The bytes for serviceName.
*/
com.google.protobuf.ByteString
getServiceNameBytes();
/**
*
* Send receipts from every function back to the gateway in case the DAG fails
*
*
* bool traceroute = 20;
* @return The traceroute.
*/
boolean getTraceroute();
public io.pinecone.proto.Core.Request.BodyCase getBodyCase();
}
/**
*
**
* Represents an execution plane Request
*
*
* Protobuf type {@code core.Request}
*/
public static final class Request extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.Request)
RequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use Request.newBuilder() to construct.
private Request(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Request() {
path_ = "";
version_ = "";
routes_ = java.util.Collections.emptyList();
namespace_ = "";
serviceName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Request();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Request(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
requestId_ = input.readUInt64();
break;
}
case 16: {
timeout_ = input.readUInt32();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
version_ = s;
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
routes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
routes_.add(
input.readMessage(io.pinecone.proto.Core.Route.parser(), extensionRegistry));
break;
}
case 50: {
io.pinecone.proto.Core.Status.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(io.pinecone.proto.Core.Status.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 58: {
io.pinecone.proto.Core.QueryRequest.Builder subBuilder = null;
if (bodyCase_ == 7) {
subBuilder = ((io.pinecone.proto.Core.QueryRequest) body_).toBuilder();
}
body_ =
input.readMessage(io.pinecone.proto.Core.QueryRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.QueryRequest) body_);
body_ = subBuilder.buildPartial();
}
bodyCase_ = 7;
break;
}
case 66: {
io.pinecone.proto.Core.IndexRequest.Builder subBuilder = null;
if (bodyCase_ == 8) {
subBuilder = ((io.pinecone.proto.Core.IndexRequest) body_).toBuilder();
}
body_ =
input.readMessage(io.pinecone.proto.Core.IndexRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.IndexRequest) body_);
body_ = subBuilder.buildPartial();
}
bodyCase_ = 8;
break;
}
case 74: {
io.pinecone.proto.Core.DeleteRequest.Builder subBuilder = null;
if (bodyCase_ == 9) {
subBuilder = ((io.pinecone.proto.Core.DeleteRequest) body_).toBuilder();
}
body_ =
input.readMessage(io.pinecone.proto.Core.DeleteRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.DeleteRequest) body_);
body_ = subBuilder.buildPartial();
}
bodyCase_ = 9;
break;
}
case 82: {
io.pinecone.proto.Core.InfoRequest.Builder subBuilder = null;
if (bodyCase_ == 10) {
subBuilder = ((io.pinecone.proto.Core.InfoRequest) body_).toBuilder();
}
body_ =
input.readMessage(io.pinecone.proto.Core.InfoRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.InfoRequest) body_);
body_ = subBuilder.buildPartial();
}
bodyCase_ = 10;
break;
}
case 90: {
io.pinecone.proto.Core.FetchRequest.Builder subBuilder = null;
if (bodyCase_ == 11) {
subBuilder = ((io.pinecone.proto.Core.FetchRequest) body_).toBuilder();
}
body_ =
input.readMessage(io.pinecone.proto.Core.FetchRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.FetchRequest) body_);
body_ = subBuilder.buildPartial();
}
bodyCase_ = 11;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
namespace_ = s;
break;
}
case 104: {
clientId_ = input.readUInt32();
break;
}
case 112: {
clientOffset_ = input.readUInt32();
break;
}
case 120: {
shardNum_ = input.readUInt32();
break;
}
case 128: {
gatewayNum_ = input.readUInt32();
break;
}
case 136: {
telemetryTraceId_ = input.readUInt64();
break;
}
case 144: {
telemetryParentId_ = input.readUInt64();
break;
}
case 154: {
java.lang.String s = input.readStringRequireUtf8();
serviceName_ = s;
break;
}
case 160: {
traceroute_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
routes_ = java.util.Collections.unmodifiableList(routes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Request_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Request_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Request.class, io.pinecone.proto.Core.Request.Builder.class);
}
private int bodyCase_ = 0;
private java.lang.Object body_;
public enum BodyCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
QUERY(7),
INDEX(8),
DELETE(9),
INFO(10),
FETCH(11),
BODY_NOT_SET(0);
private final int value;
private BodyCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BodyCase valueOf(int value) {
return forNumber(value);
}
public static BodyCase forNumber(int value) {
switch (value) {
case 7: return QUERY;
case 8: return INDEX;
case 9: return DELETE;
case 10: return INFO;
case 11: return FETCH;
case 0: return BODY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public BodyCase
getBodyCase() {
return BodyCase.forNumber(
bodyCase_);
}
public static final int REQUEST_ID_FIELD_NUMBER = 1;
private long requestId_;
/**
*
* unique id of the request
*
*
* uint64 request_id = 1;
* @return The requestId.
*/
@java.lang.Override
public long getRequestId() {
return requestId_;
}
public static final int TIMEOUT_FIELD_NUMBER = 2;
private int timeout_;
/**
*
* timeout in second until this message is dropped
*
*
* uint32 timeout = 2;
* @return The timeout.
*/
@java.lang.Override
public int getTimeout() {
return timeout_;
}
public static final int PATH_FIELD_NUMBER = 3;
private volatile java.lang.Object path_;
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 4;
private volatile java.lang.Object version_;
/**
*
* request schema version
*
*
* string version = 4;
* @return The version.
*/
@java.lang.Override
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
}
}
/**
*
* request schema version
*
*
* string version = 4;
* @return The bytes for version.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROUTES_FIELD_NUMBER = 5;
private java.util.List routes_;
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
@java.lang.Override
public java.util.List getRoutesList() {
return routes_;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
@java.lang.Override
public java.util.List extends io.pinecone.proto.Core.RouteOrBuilder>
getRoutesOrBuilderList() {
return routes_;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
@java.lang.Override
public int getRoutesCount() {
return routes_.size();
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
@java.lang.Override
public io.pinecone.proto.Core.Route getRoutes(int index) {
return routes_.get(index);
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
@java.lang.Override
public io.pinecone.proto.Core.RouteOrBuilder getRoutesOrBuilder(
int index) {
return routes_.get(index);
}
public static final int STATUS_FIELD_NUMBER = 6;
private io.pinecone.proto.Core.Status status_;
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
* @return The status.
*/
@java.lang.Override
public io.pinecone.proto.Core.Status getStatus() {
return status_ == null ? io.pinecone.proto.Core.Status.getDefaultInstance() : status_;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
@java.lang.Override
public io.pinecone.proto.Core.StatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int QUERY_FIELD_NUMBER = 7;
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
* @return Whether the query field is set.
*/
@java.lang.Override
public boolean hasQuery() {
return bodyCase_ == 7;
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
* @return The query.
*/
@java.lang.Override
public io.pinecone.proto.Core.QueryRequest getQuery() {
if (bodyCase_ == 7) {
return (io.pinecone.proto.Core.QueryRequest) body_;
}
return io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
@java.lang.Override
public io.pinecone.proto.Core.QueryRequestOrBuilder getQueryOrBuilder() {
if (bodyCase_ == 7) {
return (io.pinecone.proto.Core.QueryRequest) body_;
}
return io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
}
public static final int INDEX_FIELD_NUMBER = 8;
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
* @return Whether the index field is set.
*/
@java.lang.Override
public boolean hasIndex() {
return bodyCase_ == 8;
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
* @return The index.
*/
@java.lang.Override
public io.pinecone.proto.Core.IndexRequest getIndex() {
if (bodyCase_ == 8) {
return (io.pinecone.proto.Core.IndexRequest) body_;
}
return io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
@java.lang.Override
public io.pinecone.proto.Core.IndexRequestOrBuilder getIndexOrBuilder() {
if (bodyCase_ == 8) {
return (io.pinecone.proto.Core.IndexRequest) body_;
}
return io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
}
public static final int DELETE_FIELD_NUMBER = 9;
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
* @return Whether the delete field is set.
*/
@java.lang.Override
public boolean hasDelete() {
return bodyCase_ == 9;
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
* @return The delete.
*/
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequest getDelete() {
if (bodyCase_ == 9) {
return (io.pinecone.proto.Core.DeleteRequest) body_;
}
return io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequestOrBuilder getDeleteOrBuilder() {
if (bodyCase_ == 9) {
return (io.pinecone.proto.Core.DeleteRequest) body_;
}
return io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
}
public static final int INFO_FIELD_NUMBER = 10;
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
* @return Whether the info field is set.
*/
@java.lang.Override
public boolean hasInfo() {
return bodyCase_ == 10;
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
* @return The info.
*/
@java.lang.Override
public io.pinecone.proto.Core.InfoRequest getInfo() {
if (bodyCase_ == 10) {
return (io.pinecone.proto.Core.InfoRequest) body_;
}
return io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
@java.lang.Override
public io.pinecone.proto.Core.InfoRequestOrBuilder getInfoOrBuilder() {
if (bodyCase_ == 10) {
return (io.pinecone.proto.Core.InfoRequest) body_;
}
return io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
}
public static final int FETCH_FIELD_NUMBER = 11;
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
* @return Whether the fetch field is set.
*/
@java.lang.Override
public boolean hasFetch() {
return bodyCase_ == 11;
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
* @return The fetch.
*/
@java.lang.Override
public io.pinecone.proto.Core.FetchRequest getFetch() {
if (bodyCase_ == 11) {
return (io.pinecone.proto.Core.FetchRequest) body_;
}
return io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
@java.lang.Override
public io.pinecone.proto.Core.FetchRequestOrBuilder getFetchOrBuilder() {
if (bodyCase_ == 11) {
return (io.pinecone.proto.Core.FetchRequest) body_;
}
return io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
}
public static final int NAMESPACE_FIELD_NUMBER = 12;
private volatile java.lang.Object namespace_;
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return The namespace.
*/
@java.lang.Override
public java.lang.String getNamespace() {
java.lang.Object ref = namespace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
namespace_ = s;
return s;
}
}
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return The bytes for namespace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNamespaceBytes() {
java.lang.Object ref = namespace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
namespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_ID_FIELD_NUMBER = 13;
private int clientId_;
/**
*
* Integer ID that represents the client connection. Assigned by gateway.
*
*
* uint32 client_id = 13;
* @return The clientId.
*/
@java.lang.Override
public int getClientId() {
return clientId_;
}
public static final int CLIENT_OFFSET_FIELD_NUMBER = 14;
private int clientOffset_;
/**
*
* Offset of request within client connection. Assigned by gateway.
*
*
* uint32 client_offset = 14;
* @return The clientOffset.
*/
@java.lang.Override
public int getClientOffset() {
return clientOffset_;
}
public static final int SHARD_NUM_FIELD_NUMBER = 15;
private int shardNum_;
/**
*
* Shard the message came from. Used by aggregator
*
*
* uint32 shard_num = 15;
* @return The shardNum.
*/
@java.lang.Override
public int getShardNum() {
return shardNum_;
}
public static final int GATEWAY_NUM_FIELD_NUMBER = 16;
private int gatewayNum_;
/**
*
* Tail of gateway
*
*
* uint32 gateway_num = 16;
* @return The gatewayNum.
*/
@java.lang.Override
public int getGatewayNum() {
return gatewayNum_;
}
public static final int TELEMETRY_TRACE_ID_FIELD_NUMBER = 17;
private long telemetryTraceId_;
/**
*
**
*For tracing only
*
*
* uint64 telemetry_trace_id = 17;
* @return The telemetryTraceId.
*/
@java.lang.Override
public long getTelemetryTraceId() {
return telemetryTraceId_;
}
public static final int TELEMETRY_PARENT_ID_FIELD_NUMBER = 18;
private long telemetryParentId_;
/**
* uint64 telemetry_parent_id = 18;
* @return The telemetryParentId.
*/
@java.lang.Override
public long getTelemetryParentId() {
return telemetryParentId_;
}
public static final int SERVICE_NAME_FIELD_NUMBER = 19;
private volatile java.lang.Object serviceName_;
/**
* string service_name = 19;
* @return The serviceName.
*/
@java.lang.Override
public java.lang.String getServiceName() {
java.lang.Object ref = serviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serviceName_ = s;
return s;
}
}
/**
* string service_name = 19;
* @return The bytes for serviceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getServiceNameBytes() {
java.lang.Object ref = serviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRACEROUTE_FIELD_NUMBER = 20;
private boolean traceroute_;
/**
*
* Send receipts from every function back to the gateway in case the DAG fails
*
*
* bool traceroute = 20;
* @return The traceroute.
*/
@java.lang.Override
public boolean getTraceroute() {
return traceroute_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (requestId_ != 0L) {
output.writeUInt64(1, requestId_);
}
if (timeout_ != 0) {
output.writeUInt32(2, timeout_);
}
if (!getPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, path_);
}
if (!getVersionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, version_);
}
for (int i = 0; i < routes_.size(); i++) {
output.writeMessage(5, routes_.get(i));
}
if (status_ != null) {
output.writeMessage(6, getStatus());
}
if (bodyCase_ == 7) {
output.writeMessage(7, (io.pinecone.proto.Core.QueryRequest) body_);
}
if (bodyCase_ == 8) {
output.writeMessage(8, (io.pinecone.proto.Core.IndexRequest) body_);
}
if (bodyCase_ == 9) {
output.writeMessage(9, (io.pinecone.proto.Core.DeleteRequest) body_);
}
if (bodyCase_ == 10) {
output.writeMessage(10, (io.pinecone.proto.Core.InfoRequest) body_);
}
if (bodyCase_ == 11) {
output.writeMessage(11, (io.pinecone.proto.Core.FetchRequest) body_);
}
if (!getNamespaceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, namespace_);
}
if (clientId_ != 0) {
output.writeUInt32(13, clientId_);
}
if (clientOffset_ != 0) {
output.writeUInt32(14, clientOffset_);
}
if (shardNum_ != 0) {
output.writeUInt32(15, shardNum_);
}
if (gatewayNum_ != 0) {
output.writeUInt32(16, gatewayNum_);
}
if (telemetryTraceId_ != 0L) {
output.writeUInt64(17, telemetryTraceId_);
}
if (telemetryParentId_ != 0L) {
output.writeUInt64(18, telemetryParentId_);
}
if (!getServiceNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, serviceName_);
}
if (traceroute_ != false) {
output.writeBool(20, traceroute_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (requestId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, requestId_);
}
if (timeout_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, timeout_);
}
if (!getPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, path_);
}
if (!getVersionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, version_);
}
for (int i = 0; i < routes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, routes_.get(i));
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getStatus());
}
if (bodyCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (io.pinecone.proto.Core.QueryRequest) body_);
}
if (bodyCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, (io.pinecone.proto.Core.IndexRequest) body_);
}
if (bodyCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (io.pinecone.proto.Core.DeleteRequest) body_);
}
if (bodyCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (io.pinecone.proto.Core.InfoRequest) body_);
}
if (bodyCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (io.pinecone.proto.Core.FetchRequest) body_);
}
if (!getNamespaceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, namespace_);
}
if (clientId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(13, clientId_);
}
if (clientOffset_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(14, clientOffset_);
}
if (shardNum_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(15, shardNum_);
}
if (gatewayNum_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(16, gatewayNum_);
}
if (telemetryTraceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(17, telemetryTraceId_);
}
if (telemetryParentId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(18, telemetryParentId_);
}
if (!getServiceNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, serviceName_);
}
if (traceroute_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(20, traceroute_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.Request)) {
return super.equals(obj);
}
io.pinecone.proto.Core.Request other = (io.pinecone.proto.Core.Request) obj;
if (getRequestId()
!= other.getRequestId()) return false;
if (getTimeout()
!= other.getTimeout()) return false;
if (!getPath()
.equals(other.getPath())) return false;
if (!getVersion()
.equals(other.getVersion())) return false;
if (!getRoutesList()
.equals(other.getRoutesList())) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!getNamespace()
.equals(other.getNamespace())) return false;
if (getClientId()
!= other.getClientId()) return false;
if (getClientOffset()
!= other.getClientOffset()) return false;
if (getShardNum()
!= other.getShardNum()) return false;
if (getGatewayNum()
!= other.getGatewayNum()) return false;
if (getTelemetryTraceId()
!= other.getTelemetryTraceId()) return false;
if (getTelemetryParentId()
!= other.getTelemetryParentId()) return false;
if (!getServiceName()
.equals(other.getServiceName())) return false;
if (getTraceroute()
!= other.getTraceroute()) return false;
if (!getBodyCase().equals(other.getBodyCase())) return false;
switch (bodyCase_) {
case 7:
if (!getQuery()
.equals(other.getQuery())) return false;
break;
case 8:
if (!getIndex()
.equals(other.getIndex())) return false;
break;
case 9:
if (!getDelete()
.equals(other.getDelete())) return false;
break;
case 10:
if (!getInfo()
.equals(other.getInfo())) return false;
break;
case 11:
if (!getFetch()
.equals(other.getFetch())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRequestId());
hash = (37 * hash) + TIMEOUT_FIELD_NUMBER;
hash = (53 * hash) + getTimeout();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
if (getRoutesCount() > 0) {
hash = (37 * hash) + ROUTES_FIELD_NUMBER;
hash = (53 * hash) + getRoutesList().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (37 * hash) + NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getNamespace().hashCode();
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId();
hash = (37 * hash) + CLIENT_OFFSET_FIELD_NUMBER;
hash = (53 * hash) + getClientOffset();
hash = (37 * hash) + SHARD_NUM_FIELD_NUMBER;
hash = (53 * hash) + getShardNum();
hash = (37 * hash) + GATEWAY_NUM_FIELD_NUMBER;
hash = (53 * hash) + getGatewayNum();
hash = (37 * hash) + TELEMETRY_TRACE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTelemetryTraceId());
hash = (37 * hash) + TELEMETRY_PARENT_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTelemetryParentId());
hash = (37 * hash) + SERVICE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getServiceName().hashCode();
hash = (37 * hash) + TRACEROUTE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTraceroute());
switch (bodyCase_) {
case 7:
hash = (37 * hash) + QUERY_FIELD_NUMBER;
hash = (53 * hash) + getQuery().hashCode();
break;
case 8:
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + getIndex().hashCode();
break;
case 9:
hash = (37 * hash) + DELETE_FIELD_NUMBER;
hash = (53 * hash) + getDelete().hashCode();
break;
case 10:
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
break;
case 11:
hash = (37 * hash) + FETCH_FIELD_NUMBER;
hash = (53 * hash) + getFetch().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.Request parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Request parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Request parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Request parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Request parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Request parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Request parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Request parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Request parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Request parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Request parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Request parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.Request prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents an execution plane Request
*
*
* Protobuf type {@code core.Request}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.Request)
io.pinecone.proto.Core.RequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Request_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Request_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Request.class, io.pinecone.proto.Core.Request.Builder.class);
}
// Construct using io.pinecone.proto.Core.Request.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRoutesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
requestId_ = 0L;
timeout_ = 0;
path_ = "";
version_ = "";
if (routesBuilder_ == null) {
routes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
routesBuilder_.clear();
}
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
namespace_ = "";
clientId_ = 0;
clientOffset_ = 0;
shardNum_ = 0;
gatewayNum_ = 0;
telemetryTraceId_ = 0L;
telemetryParentId_ = 0L;
serviceName_ = "";
traceroute_ = false;
bodyCase_ = 0;
body_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_Request_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.Request getDefaultInstanceForType() {
return io.pinecone.proto.Core.Request.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.Request build() {
io.pinecone.proto.Core.Request result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.Request buildPartial() {
io.pinecone.proto.Core.Request result = new io.pinecone.proto.Core.Request(this);
int from_bitField0_ = bitField0_;
result.requestId_ = requestId_;
result.timeout_ = timeout_;
result.path_ = path_;
result.version_ = version_;
if (routesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
routes_ = java.util.Collections.unmodifiableList(routes_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.routes_ = routes_;
} else {
result.routes_ = routesBuilder_.build();
}
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (bodyCase_ == 7) {
if (queryBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = queryBuilder_.build();
}
}
if (bodyCase_ == 8) {
if (indexBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = indexBuilder_.build();
}
}
if (bodyCase_ == 9) {
if (deleteBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = deleteBuilder_.build();
}
}
if (bodyCase_ == 10) {
if (infoBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = infoBuilder_.build();
}
}
if (bodyCase_ == 11) {
if (fetchBuilder_ == null) {
result.body_ = body_;
} else {
result.body_ = fetchBuilder_.build();
}
}
result.namespace_ = namespace_;
result.clientId_ = clientId_;
result.clientOffset_ = clientOffset_;
result.shardNum_ = shardNum_;
result.gatewayNum_ = gatewayNum_;
result.telemetryTraceId_ = telemetryTraceId_;
result.telemetryParentId_ = telemetryParentId_;
result.serviceName_ = serviceName_;
result.traceroute_ = traceroute_;
result.bodyCase_ = bodyCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.Request) {
return mergeFrom((io.pinecone.proto.Core.Request)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.Request other) {
if (other == io.pinecone.proto.Core.Request.getDefaultInstance()) return this;
if (other.getRequestId() != 0L) {
setRequestId(other.getRequestId());
}
if (other.getTimeout() != 0) {
setTimeout(other.getTimeout());
}
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (!other.getVersion().isEmpty()) {
version_ = other.version_;
onChanged();
}
if (routesBuilder_ == null) {
if (!other.routes_.isEmpty()) {
if (routes_.isEmpty()) {
routes_ = other.routes_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRoutesIsMutable();
routes_.addAll(other.routes_);
}
onChanged();
}
} else {
if (!other.routes_.isEmpty()) {
if (routesBuilder_.isEmpty()) {
routesBuilder_.dispose();
routesBuilder_ = null;
routes_ = other.routes_;
bitField0_ = (bitField0_ & ~0x00000001);
routesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRoutesFieldBuilder() : null;
} else {
routesBuilder_.addAllMessages(other.routes_);
}
}
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (!other.getNamespace().isEmpty()) {
namespace_ = other.namespace_;
onChanged();
}
if (other.getClientId() != 0) {
setClientId(other.getClientId());
}
if (other.getClientOffset() != 0) {
setClientOffset(other.getClientOffset());
}
if (other.getShardNum() != 0) {
setShardNum(other.getShardNum());
}
if (other.getGatewayNum() != 0) {
setGatewayNum(other.getGatewayNum());
}
if (other.getTelemetryTraceId() != 0L) {
setTelemetryTraceId(other.getTelemetryTraceId());
}
if (other.getTelemetryParentId() != 0L) {
setTelemetryParentId(other.getTelemetryParentId());
}
if (!other.getServiceName().isEmpty()) {
serviceName_ = other.serviceName_;
onChanged();
}
if (other.getTraceroute() != false) {
setTraceroute(other.getTraceroute());
}
switch (other.getBodyCase()) {
case QUERY: {
mergeQuery(other.getQuery());
break;
}
case INDEX: {
mergeIndex(other.getIndex());
break;
}
case DELETE: {
mergeDelete(other.getDelete());
break;
}
case INFO: {
mergeInfo(other.getInfo());
break;
}
case FETCH: {
mergeFetch(other.getFetch());
break;
}
case BODY_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.Request parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.Request) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bodyCase_ = 0;
private java.lang.Object body_;
public BodyCase
getBodyCase() {
return BodyCase.forNumber(
bodyCase_);
}
public Builder clearBody() {
bodyCase_ = 0;
body_ = null;
onChanged();
return this;
}
private int bitField0_;
private long requestId_ ;
/**
*
* unique id of the request
*
*
* uint64 request_id = 1;
* @return The requestId.
*/
@java.lang.Override
public long getRequestId() {
return requestId_;
}
/**
*
* unique id of the request
*
*
* uint64 request_id = 1;
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(long value) {
requestId_ = value;
onChanged();
return this;
}
/**
*
* unique id of the request
*
*
* uint64 request_id = 1;
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = 0L;
onChanged();
return this;
}
private int timeout_ ;
/**
*
* timeout in second until this message is dropped
*
*
* uint32 timeout = 2;
* @return The timeout.
*/
@java.lang.Override
public int getTimeout() {
return timeout_;
}
/**
*
* timeout in second until this message is dropped
*
*
* uint32 timeout = 2;
* @param value The timeout to set.
* @return This builder for chaining.
*/
public Builder setTimeout(int value) {
timeout_ = value;
onChanged();
return this;
}
/**
*
* timeout in second until this message is dropped
*
*
* uint32 timeout = 2;
* @return This builder for chaining.
*/
public Builder clearTimeout() {
timeout_ = 0;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
*
* path in DAG (defined ahead of time) to follow
*
*
* string path = 3;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private java.lang.Object version_ = "";
/**
*
* request schema version
*
*
* string version = 4;
* @return The version.
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
version_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* request schema version
*
*
* string version = 4;
* @return The bytes for version.
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* request schema version
*
*
* string version = 4;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
return this;
}
/**
*
* request schema version
*
*
* string version = 4;
* @return This builder for chaining.
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
onChanged();
return this;
}
/**
*
* request schema version
*
*
* string version = 4;
* @param value The bytes for version to set.
* @return This builder for chaining.
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
version_ = value;
onChanged();
return this;
}
private java.util.List routes_ =
java.util.Collections.emptyList();
private void ensureRoutesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
routes_ = new java.util.ArrayList(routes_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Route, io.pinecone.proto.Core.Route.Builder, io.pinecone.proto.Core.RouteOrBuilder> routesBuilder_;
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public java.util.List getRoutesList() {
if (routesBuilder_ == null) {
return java.util.Collections.unmodifiableList(routes_);
} else {
return routesBuilder_.getMessageList();
}
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public int getRoutesCount() {
if (routesBuilder_ == null) {
return routes_.size();
} else {
return routesBuilder_.getCount();
}
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public io.pinecone.proto.Core.Route getRoutes(int index) {
if (routesBuilder_ == null) {
return routes_.get(index);
} else {
return routesBuilder_.getMessage(index);
}
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder setRoutes(
int index, io.pinecone.proto.Core.Route value) {
if (routesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRoutesIsMutable();
routes_.set(index, value);
onChanged();
} else {
routesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder setRoutes(
int index, io.pinecone.proto.Core.Route.Builder builderForValue) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.set(index, builderForValue.build());
onChanged();
} else {
routesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder addRoutes(io.pinecone.proto.Core.Route value) {
if (routesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRoutesIsMutable();
routes_.add(value);
onChanged();
} else {
routesBuilder_.addMessage(value);
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder addRoutes(
int index, io.pinecone.proto.Core.Route value) {
if (routesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRoutesIsMutable();
routes_.add(index, value);
onChanged();
} else {
routesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder addRoutes(
io.pinecone.proto.Core.Route.Builder builderForValue) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.add(builderForValue.build());
onChanged();
} else {
routesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder addRoutes(
int index, io.pinecone.proto.Core.Route.Builder builderForValue) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.add(index, builderForValue.build());
onChanged();
} else {
routesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder addAllRoutes(
java.lang.Iterable extends io.pinecone.proto.Core.Route> values) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, routes_);
onChanged();
} else {
routesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder clearRoutes() {
if (routesBuilder_ == null) {
routes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
routesBuilder_.clear();
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public Builder removeRoutes(int index) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.remove(index);
onChanged();
} else {
routesBuilder_.remove(index);
}
return this;
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public io.pinecone.proto.Core.Route.Builder getRoutesBuilder(
int index) {
return getRoutesFieldBuilder().getBuilder(index);
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public io.pinecone.proto.Core.RouteOrBuilder getRoutesOrBuilder(
int index) {
if (routesBuilder_ == null) {
return routes_.get(index); } else {
return routesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public java.util.List extends io.pinecone.proto.Core.RouteOrBuilder>
getRoutesOrBuilderList() {
if (routesBuilder_ != null) {
return routesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(routes_);
}
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public io.pinecone.proto.Core.Route.Builder addRoutesBuilder() {
return getRoutesFieldBuilder().addBuilder(
io.pinecone.proto.Core.Route.getDefaultInstance());
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public io.pinecone.proto.Core.Route.Builder addRoutesBuilder(
int index) {
return getRoutesFieldBuilder().addBuilder(
index, io.pinecone.proto.Core.Route.getDefaultInstance());
}
/**
*
* a list of routes this message goes through
*
*
* repeated .core.Route routes = 5;
*/
public java.util.List
getRoutesBuilderList() {
return getRoutesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Route, io.pinecone.proto.Core.Route.Builder, io.pinecone.proto.Core.RouteOrBuilder>
getRoutesFieldBuilder() {
if (routesBuilder_ == null) {
routesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Route, io.pinecone.proto.Core.Route.Builder, io.pinecone.proto.Core.RouteOrBuilder>(
routes_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
routes_ = null;
}
return routesBuilder_;
}
private io.pinecone.proto.Core.Status status_;
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Status, io.pinecone.proto.Core.Status.Builder, io.pinecone.proto.Core.StatusOrBuilder> statusBuilder_;
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
* @return The status.
*/
public io.pinecone.proto.Core.Status getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? io.pinecone.proto.Core.Status.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
public Builder setStatus(io.pinecone.proto.Core.Status value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
public Builder setStatus(
io.pinecone.proto.Core.Status.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
public Builder mergeStatus(io.pinecone.proto.Core.Status value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
io.pinecone.proto.Core.Status.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
public io.pinecone.proto.Core.Status.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
public io.pinecone.proto.Core.StatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
io.pinecone.proto.Core.Status.getDefaultInstance() : status_;
}
}
/**
*
* status info, e.g. error
*
*
* .core.Status status = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Status, io.pinecone.proto.Core.Status.Builder, io.pinecone.proto.Core.StatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Status, io.pinecone.proto.Core.Status.Builder, io.pinecone.proto.Core.StatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.QueryRequest, io.pinecone.proto.Core.QueryRequest.Builder, io.pinecone.proto.Core.QueryRequestOrBuilder> queryBuilder_;
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
* @return Whether the query field is set.
*/
@java.lang.Override
public boolean hasQuery() {
return bodyCase_ == 7;
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
* @return The query.
*/
@java.lang.Override
public io.pinecone.proto.Core.QueryRequest getQuery() {
if (queryBuilder_ == null) {
if (bodyCase_ == 7) {
return (io.pinecone.proto.Core.QueryRequest) body_;
}
return io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
} else {
if (bodyCase_ == 7) {
return queryBuilder_.getMessage();
}
return io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
}
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
public Builder setQuery(io.pinecone.proto.Core.QueryRequest value) {
if (queryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
queryBuilder_.setMessage(value);
}
bodyCase_ = 7;
return this;
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
public Builder setQuery(
io.pinecone.proto.Core.QueryRequest.Builder builderForValue) {
if (queryBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
queryBuilder_.setMessage(builderForValue.build());
}
bodyCase_ = 7;
return this;
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
public Builder mergeQuery(io.pinecone.proto.Core.QueryRequest value) {
if (queryBuilder_ == null) {
if (bodyCase_ == 7 &&
body_ != io.pinecone.proto.Core.QueryRequest.getDefaultInstance()) {
body_ = io.pinecone.proto.Core.QueryRequest.newBuilder((io.pinecone.proto.Core.QueryRequest) body_)
.mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
if (bodyCase_ == 7) {
queryBuilder_.mergeFrom(value);
}
queryBuilder_.setMessage(value);
}
bodyCase_ = 7;
return this;
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
public Builder clearQuery() {
if (queryBuilder_ == null) {
if (bodyCase_ == 7) {
bodyCase_ = 0;
body_ = null;
onChanged();
}
} else {
if (bodyCase_ == 7) {
bodyCase_ = 0;
body_ = null;
}
queryBuilder_.clear();
}
return this;
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
public io.pinecone.proto.Core.QueryRequest.Builder getQueryBuilder() {
return getQueryFieldBuilder().getBuilder();
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
@java.lang.Override
public io.pinecone.proto.Core.QueryRequestOrBuilder getQueryOrBuilder() {
if ((bodyCase_ == 7) && (queryBuilder_ != null)) {
return queryBuilder_.getMessageOrBuilder();
} else {
if (bodyCase_ == 7) {
return (io.pinecone.proto.Core.QueryRequest) body_;
}
return io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
}
}
/**
*
* A query request object
*
*
* .core.QueryRequest query = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.QueryRequest, io.pinecone.proto.Core.QueryRequest.Builder, io.pinecone.proto.Core.QueryRequestOrBuilder>
getQueryFieldBuilder() {
if (queryBuilder_ == null) {
if (!(bodyCase_ == 7)) {
body_ = io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
}
queryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.QueryRequest, io.pinecone.proto.Core.QueryRequest.Builder, io.pinecone.proto.Core.QueryRequestOrBuilder>(
(io.pinecone.proto.Core.QueryRequest) body_,
getParentForChildren(),
isClean());
body_ = null;
}
bodyCase_ = 7;
onChanged();;
return queryBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.IndexRequest, io.pinecone.proto.Core.IndexRequest.Builder, io.pinecone.proto.Core.IndexRequestOrBuilder> indexBuilder_;
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
* @return Whether the index field is set.
*/
@java.lang.Override
public boolean hasIndex() {
return bodyCase_ == 8;
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
* @return The index.
*/
@java.lang.Override
public io.pinecone.proto.Core.IndexRequest getIndex() {
if (indexBuilder_ == null) {
if (bodyCase_ == 8) {
return (io.pinecone.proto.Core.IndexRequest) body_;
}
return io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
} else {
if (bodyCase_ == 8) {
return indexBuilder_.getMessage();
}
return io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
}
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
public Builder setIndex(io.pinecone.proto.Core.IndexRequest value) {
if (indexBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
indexBuilder_.setMessage(value);
}
bodyCase_ = 8;
return this;
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
public Builder setIndex(
io.pinecone.proto.Core.IndexRequest.Builder builderForValue) {
if (indexBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
indexBuilder_.setMessage(builderForValue.build());
}
bodyCase_ = 8;
return this;
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
public Builder mergeIndex(io.pinecone.proto.Core.IndexRequest value) {
if (indexBuilder_ == null) {
if (bodyCase_ == 8 &&
body_ != io.pinecone.proto.Core.IndexRequest.getDefaultInstance()) {
body_ = io.pinecone.proto.Core.IndexRequest.newBuilder((io.pinecone.proto.Core.IndexRequest) body_)
.mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
if (bodyCase_ == 8) {
indexBuilder_.mergeFrom(value);
}
indexBuilder_.setMessage(value);
}
bodyCase_ = 8;
return this;
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
public Builder clearIndex() {
if (indexBuilder_ == null) {
if (bodyCase_ == 8) {
bodyCase_ = 0;
body_ = null;
onChanged();
}
} else {
if (bodyCase_ == 8) {
bodyCase_ = 0;
body_ = null;
}
indexBuilder_.clear();
}
return this;
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
public io.pinecone.proto.Core.IndexRequest.Builder getIndexBuilder() {
return getIndexFieldBuilder().getBuilder();
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
@java.lang.Override
public io.pinecone.proto.Core.IndexRequestOrBuilder getIndexOrBuilder() {
if ((bodyCase_ == 8) && (indexBuilder_ != null)) {
return indexBuilder_.getMessageOrBuilder();
} else {
if (bodyCase_ == 8) {
return (io.pinecone.proto.Core.IndexRequest) body_;
}
return io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
}
}
/**
*
* An upsert items request object
*
*
* .core.IndexRequest index = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.IndexRequest, io.pinecone.proto.Core.IndexRequest.Builder, io.pinecone.proto.Core.IndexRequestOrBuilder>
getIndexFieldBuilder() {
if (indexBuilder_ == null) {
if (!(bodyCase_ == 8)) {
body_ = io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
}
indexBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.IndexRequest, io.pinecone.proto.Core.IndexRequest.Builder, io.pinecone.proto.Core.IndexRequestOrBuilder>(
(io.pinecone.proto.Core.IndexRequest) body_,
getParentForChildren(),
isClean());
body_ = null;
}
bodyCase_ = 8;
onChanged();;
return indexBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.DeleteRequest, io.pinecone.proto.Core.DeleteRequest.Builder, io.pinecone.proto.Core.DeleteRequestOrBuilder> deleteBuilder_;
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
* @return Whether the delete field is set.
*/
@java.lang.Override
public boolean hasDelete() {
return bodyCase_ == 9;
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
* @return The delete.
*/
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequest getDelete() {
if (deleteBuilder_ == null) {
if (bodyCase_ == 9) {
return (io.pinecone.proto.Core.DeleteRequest) body_;
}
return io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
} else {
if (bodyCase_ == 9) {
return deleteBuilder_.getMessage();
}
return io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
}
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
public Builder setDelete(io.pinecone.proto.Core.DeleteRequest value) {
if (deleteBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
deleteBuilder_.setMessage(value);
}
bodyCase_ = 9;
return this;
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
public Builder setDelete(
io.pinecone.proto.Core.DeleteRequest.Builder builderForValue) {
if (deleteBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
deleteBuilder_.setMessage(builderForValue.build());
}
bodyCase_ = 9;
return this;
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
public Builder mergeDelete(io.pinecone.proto.Core.DeleteRequest value) {
if (deleteBuilder_ == null) {
if (bodyCase_ == 9 &&
body_ != io.pinecone.proto.Core.DeleteRequest.getDefaultInstance()) {
body_ = io.pinecone.proto.Core.DeleteRequest.newBuilder((io.pinecone.proto.Core.DeleteRequest) body_)
.mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
if (bodyCase_ == 9) {
deleteBuilder_.mergeFrom(value);
}
deleteBuilder_.setMessage(value);
}
bodyCase_ = 9;
return this;
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
public Builder clearDelete() {
if (deleteBuilder_ == null) {
if (bodyCase_ == 9) {
bodyCase_ = 0;
body_ = null;
onChanged();
}
} else {
if (bodyCase_ == 9) {
bodyCase_ = 0;
body_ = null;
}
deleteBuilder_.clear();
}
return this;
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
public io.pinecone.proto.Core.DeleteRequest.Builder getDeleteBuilder() {
return getDeleteFieldBuilder().getBuilder();
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequestOrBuilder getDeleteOrBuilder() {
if ((bodyCase_ == 9) && (deleteBuilder_ != null)) {
return deleteBuilder_.getMessageOrBuilder();
} else {
if (bodyCase_ == 9) {
return (io.pinecone.proto.Core.DeleteRequest) body_;
}
return io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
}
}
/**
*
* A delete items request object
*
*
* .core.DeleteRequest delete = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.DeleteRequest, io.pinecone.proto.Core.DeleteRequest.Builder, io.pinecone.proto.Core.DeleteRequestOrBuilder>
getDeleteFieldBuilder() {
if (deleteBuilder_ == null) {
if (!(bodyCase_ == 9)) {
body_ = io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
}
deleteBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.DeleteRequest, io.pinecone.proto.Core.DeleteRequest.Builder, io.pinecone.proto.Core.DeleteRequestOrBuilder>(
(io.pinecone.proto.Core.DeleteRequest) body_,
getParentForChildren(),
isClean());
body_ = null;
}
bodyCase_ = 9;
onChanged();;
return deleteBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.InfoRequest, io.pinecone.proto.Core.InfoRequest.Builder, io.pinecone.proto.Core.InfoRequestOrBuilder> infoBuilder_;
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
* @return Whether the info field is set.
*/
@java.lang.Override
public boolean hasInfo() {
return bodyCase_ == 10;
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
* @return The info.
*/
@java.lang.Override
public io.pinecone.proto.Core.InfoRequest getInfo() {
if (infoBuilder_ == null) {
if (bodyCase_ == 10) {
return (io.pinecone.proto.Core.InfoRequest) body_;
}
return io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
} else {
if (bodyCase_ == 10) {
return infoBuilder_.getMessage();
}
return io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
}
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
public Builder setInfo(io.pinecone.proto.Core.InfoRequest value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
infoBuilder_.setMessage(value);
}
bodyCase_ = 10;
return this;
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
public Builder setInfo(
io.pinecone.proto.Core.InfoRequest.Builder builderForValue) {
if (infoBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
bodyCase_ = 10;
return this;
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
public Builder mergeInfo(io.pinecone.proto.Core.InfoRequest value) {
if (infoBuilder_ == null) {
if (bodyCase_ == 10 &&
body_ != io.pinecone.proto.Core.InfoRequest.getDefaultInstance()) {
body_ = io.pinecone.proto.Core.InfoRequest.newBuilder((io.pinecone.proto.Core.InfoRequest) body_)
.mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
if (bodyCase_ == 10) {
infoBuilder_.mergeFrom(value);
}
infoBuilder_.setMessage(value);
}
bodyCase_ = 10;
return this;
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
if (bodyCase_ == 10) {
bodyCase_ = 0;
body_ = null;
onChanged();
}
} else {
if (bodyCase_ == 10) {
bodyCase_ = 0;
body_ = null;
}
infoBuilder_.clear();
}
return this;
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
public io.pinecone.proto.Core.InfoRequest.Builder getInfoBuilder() {
return getInfoFieldBuilder().getBuilder();
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
@java.lang.Override
public io.pinecone.proto.Core.InfoRequestOrBuilder getInfoOrBuilder() {
if ((bodyCase_ == 10) && (infoBuilder_ != null)) {
return infoBuilder_.getMessageOrBuilder();
} else {
if (bodyCase_ == 10) {
return (io.pinecone.proto.Core.InfoRequest) body_;
}
return io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
}
}
/**
*
* An object for a get info request
*
*
* .core.InfoRequest info = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.InfoRequest, io.pinecone.proto.Core.InfoRequest.Builder, io.pinecone.proto.Core.InfoRequestOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
if (!(bodyCase_ == 10)) {
body_ = io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
}
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.InfoRequest, io.pinecone.proto.Core.InfoRequest.Builder, io.pinecone.proto.Core.InfoRequestOrBuilder>(
(io.pinecone.proto.Core.InfoRequest) body_,
getParentForChildren(),
isClean());
body_ = null;
}
bodyCase_ = 10;
onChanged();;
return infoBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.FetchRequest, io.pinecone.proto.Core.FetchRequest.Builder, io.pinecone.proto.Core.FetchRequestOrBuilder> fetchBuilder_;
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
* @return Whether the fetch field is set.
*/
@java.lang.Override
public boolean hasFetch() {
return bodyCase_ == 11;
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
* @return The fetch.
*/
@java.lang.Override
public io.pinecone.proto.Core.FetchRequest getFetch() {
if (fetchBuilder_ == null) {
if (bodyCase_ == 11) {
return (io.pinecone.proto.Core.FetchRequest) body_;
}
return io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
} else {
if (bodyCase_ == 11) {
return fetchBuilder_.getMessage();
}
return io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
}
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
public Builder setFetch(io.pinecone.proto.Core.FetchRequest value) {
if (fetchBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
} else {
fetchBuilder_.setMessage(value);
}
bodyCase_ = 11;
return this;
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
public Builder setFetch(
io.pinecone.proto.Core.FetchRequest.Builder builderForValue) {
if (fetchBuilder_ == null) {
body_ = builderForValue.build();
onChanged();
} else {
fetchBuilder_.setMessage(builderForValue.build());
}
bodyCase_ = 11;
return this;
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
public Builder mergeFetch(io.pinecone.proto.Core.FetchRequest value) {
if (fetchBuilder_ == null) {
if (bodyCase_ == 11 &&
body_ != io.pinecone.proto.Core.FetchRequest.getDefaultInstance()) {
body_ = io.pinecone.proto.Core.FetchRequest.newBuilder((io.pinecone.proto.Core.FetchRequest) body_)
.mergeFrom(value).buildPartial();
} else {
body_ = value;
}
onChanged();
} else {
if (bodyCase_ == 11) {
fetchBuilder_.mergeFrom(value);
}
fetchBuilder_.setMessage(value);
}
bodyCase_ = 11;
return this;
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
public Builder clearFetch() {
if (fetchBuilder_ == null) {
if (bodyCase_ == 11) {
bodyCase_ = 0;
body_ = null;
onChanged();
}
} else {
if (bodyCase_ == 11) {
bodyCase_ = 0;
body_ = null;
}
fetchBuilder_.clear();
}
return this;
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
public io.pinecone.proto.Core.FetchRequest.Builder getFetchBuilder() {
return getFetchFieldBuilder().getBuilder();
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
@java.lang.Override
public io.pinecone.proto.Core.FetchRequestOrBuilder getFetchOrBuilder() {
if ((bodyCase_ == 11) && (fetchBuilder_ != null)) {
return fetchBuilder_.getMessageOrBuilder();
} else {
if (bodyCase_ == 11) {
return (io.pinecone.proto.Core.FetchRequest) body_;
}
return io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
}
}
/**
*
* Payload for a request to fetch vectors by id
*
*
* .core.FetchRequest fetch = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.FetchRequest, io.pinecone.proto.Core.FetchRequest.Builder, io.pinecone.proto.Core.FetchRequestOrBuilder>
getFetchFieldBuilder() {
if (fetchBuilder_ == null) {
if (!(bodyCase_ == 11)) {
body_ = io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
}
fetchBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.FetchRequest, io.pinecone.proto.Core.FetchRequest.Builder, io.pinecone.proto.Core.FetchRequestOrBuilder>(
(io.pinecone.proto.Core.FetchRequest) body_,
getParentForChildren(),
isClean());
body_ = null;
}
bodyCase_ = 11;
onChanged();;
return fetchBuilder_;
}
private java.lang.Object namespace_ = "";
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return The namespace.
*/
public java.lang.String getNamespace() {
java.lang.Object ref = namespace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
namespace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return The bytes for namespace.
*/
public com.google.protobuf.ByteString
getNamespaceBytes() {
java.lang.Object ref = namespace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
namespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @param value The namespace to set.
* @return This builder for chaining.
*/
public Builder setNamespace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
namespace_ = value;
onChanged();
return this;
}
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @return This builder for chaining.
*/
public Builder clearNamespace() {
namespace_ = getDefaultInstance().getNamespace();
onChanged();
return this;
}
/**
*
* Namespace to perform operation in (if data is divided by namespace, default "")
*
*
* string namespace = 12;
* @param value The bytes for namespace to set.
* @return This builder for chaining.
*/
public Builder setNamespaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
namespace_ = value;
onChanged();
return this;
}
private int clientId_ ;
/**
*
* Integer ID that represents the client connection. Assigned by gateway.
*
*
* uint32 client_id = 13;
* @return The clientId.
*/
@java.lang.Override
public int getClientId() {
return clientId_;
}
/**
*
* Integer ID that represents the client connection. Assigned by gateway.
*
*
* uint32 client_id = 13;
* @param value The clientId to set.
* @return This builder for chaining.
*/
public Builder setClientId(int value) {
clientId_ = value;
onChanged();
return this;
}
/**
*
* Integer ID that represents the client connection. Assigned by gateway.
*
*
* uint32 client_id = 13;
* @return This builder for chaining.
*/
public Builder clearClientId() {
clientId_ = 0;
onChanged();
return this;
}
private int clientOffset_ ;
/**
*
* Offset of request within client connection. Assigned by gateway.
*
*
* uint32 client_offset = 14;
* @return The clientOffset.
*/
@java.lang.Override
public int getClientOffset() {
return clientOffset_;
}
/**
*
* Offset of request within client connection. Assigned by gateway.
*
*
* uint32 client_offset = 14;
* @param value The clientOffset to set.
* @return This builder for chaining.
*/
public Builder setClientOffset(int value) {
clientOffset_ = value;
onChanged();
return this;
}
/**
*
* Offset of request within client connection. Assigned by gateway.
*
*
* uint32 client_offset = 14;
* @return This builder for chaining.
*/
public Builder clearClientOffset() {
clientOffset_ = 0;
onChanged();
return this;
}
private int shardNum_ ;
/**
*
* Shard the message came from. Used by aggregator
*
*
* uint32 shard_num = 15;
* @return The shardNum.
*/
@java.lang.Override
public int getShardNum() {
return shardNum_;
}
/**
*
* Shard the message came from. Used by aggregator
*
*
* uint32 shard_num = 15;
* @param value The shardNum to set.
* @return This builder for chaining.
*/
public Builder setShardNum(int value) {
shardNum_ = value;
onChanged();
return this;
}
/**
*
* Shard the message came from. Used by aggregator
*
*
* uint32 shard_num = 15;
* @return This builder for chaining.
*/
public Builder clearShardNum() {
shardNum_ = 0;
onChanged();
return this;
}
private int gatewayNum_ ;
/**
*
* Tail of gateway
*
*
* uint32 gateway_num = 16;
* @return The gatewayNum.
*/
@java.lang.Override
public int getGatewayNum() {
return gatewayNum_;
}
/**
*
* Tail of gateway
*
*
* uint32 gateway_num = 16;
* @param value The gatewayNum to set.
* @return This builder for chaining.
*/
public Builder setGatewayNum(int value) {
gatewayNum_ = value;
onChanged();
return this;
}
/**
*
* Tail of gateway
*
*
* uint32 gateway_num = 16;
* @return This builder for chaining.
*/
public Builder clearGatewayNum() {
gatewayNum_ = 0;
onChanged();
return this;
}
private long telemetryTraceId_ ;
/**
*
**
*For tracing only
*
*
* uint64 telemetry_trace_id = 17;
* @return The telemetryTraceId.
*/
@java.lang.Override
public long getTelemetryTraceId() {
return telemetryTraceId_;
}
/**
*
**
*For tracing only
*
*
* uint64 telemetry_trace_id = 17;
* @param value The telemetryTraceId to set.
* @return This builder for chaining.
*/
public Builder setTelemetryTraceId(long value) {
telemetryTraceId_ = value;
onChanged();
return this;
}
/**
*
**
*For tracing only
*
*
* uint64 telemetry_trace_id = 17;
* @return This builder for chaining.
*/
public Builder clearTelemetryTraceId() {
telemetryTraceId_ = 0L;
onChanged();
return this;
}
private long telemetryParentId_ ;
/**
* uint64 telemetry_parent_id = 18;
* @return The telemetryParentId.
*/
@java.lang.Override
public long getTelemetryParentId() {
return telemetryParentId_;
}
/**
* uint64 telemetry_parent_id = 18;
* @param value The telemetryParentId to set.
* @return This builder for chaining.
*/
public Builder setTelemetryParentId(long value) {
telemetryParentId_ = value;
onChanged();
return this;
}
/**
* uint64 telemetry_parent_id = 18;
* @return This builder for chaining.
*/
public Builder clearTelemetryParentId() {
telemetryParentId_ = 0L;
onChanged();
return this;
}
private java.lang.Object serviceName_ = "";
/**
* string service_name = 19;
* @return The serviceName.
*/
public java.lang.String getServiceName() {
java.lang.Object ref = serviceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serviceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string service_name = 19;
* @return The bytes for serviceName.
*/
public com.google.protobuf.ByteString
getServiceNameBytes() {
java.lang.Object ref = serviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string service_name = 19;
* @param value The serviceName to set.
* @return This builder for chaining.
*/
public Builder setServiceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
serviceName_ = value;
onChanged();
return this;
}
/**
* string service_name = 19;
* @return This builder for chaining.
*/
public Builder clearServiceName() {
serviceName_ = getDefaultInstance().getServiceName();
onChanged();
return this;
}
/**
* string service_name = 19;
* @param value The bytes for serviceName to set.
* @return This builder for chaining.
*/
public Builder setServiceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serviceName_ = value;
onChanged();
return this;
}
private boolean traceroute_ ;
/**
*
* Send receipts from every function back to the gateway in case the DAG fails
*
*
* bool traceroute = 20;
* @return The traceroute.
*/
@java.lang.Override
public boolean getTraceroute() {
return traceroute_;
}
/**
*
* Send receipts from every function back to the gateway in case the DAG fails
*
*
* bool traceroute = 20;
* @param value The traceroute to set.
* @return This builder for chaining.
*/
public Builder setTraceroute(boolean value) {
traceroute_ = value;
onChanged();
return this;
}
/**
*
* Send receipts from every function back to the gateway in case the DAG fails
*
*
* bool traceroute = 20;
* @return This builder for chaining.
*/
public Builder clearTraceroute() {
traceroute_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.Request)
}
// @@protoc_insertion_point(class_scope:core.Request)
private static final io.pinecone.proto.Core.Request DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.Request();
}
public static io.pinecone.proto.Core.Request getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Request parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Request(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.Request getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StatusOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.Status)
com.google.protobuf.MessageOrBuilder {
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return The enum numeric value on the wire for code.
*/
int getCodeValue();
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return The code.
*/
io.pinecone.proto.Core.Status.StatusCode getCode();
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return The description.
*/
java.lang.String getDescription();
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
java.util.List
getDetailsList();
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
io.pinecone.proto.Core.Status.Details getDetails(int index);
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
int getDetailsCount();
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
java.util.List extends io.pinecone.proto.Core.Status.DetailsOrBuilder>
getDetailsOrBuilderList();
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
io.pinecone.proto.Core.Status.DetailsOrBuilder getDetailsOrBuilder(
int index);
/**
*
* number of messages sent by the function
*
*
* uint64 msg_sent = 4;
* @return The msgSent.
*/
long getMsgSent();
/**
*
* number of messages received by the function
*
*
* uint64 msg_recv = 5;
* @return The msgRecv.
*/
long getMsgRecv();
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
int getAvgTimeCount();
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
boolean containsAvgTime(
java.lang.String key);
/**
* Use {@link #getAvgTimeMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getAvgTime();
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
java.util.Map
getAvgTimeMap();
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
int getAvgTimeOrDefault(
java.lang.String key,
int defaultValue);
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
int getAvgTimeOrThrow(
java.lang.String key);
/**
*
* size of a function's dataset if stateful
*
*
* uint64 size = 7;
* @return The size.
*/
long getSize();
}
/**
* Protobuf type {@code core.Status}
*/
public static final class Status extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.Status)
StatusOrBuilder {
private static final long serialVersionUID = 0L;
// Use Status.newBuilder() to construct.
private Status(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Status() {
code_ = 0;
description_ = "";
details_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Status();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Status(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
code_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
details_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
details_.add(
input.readMessage(io.pinecone.proto.Core.Status.Details.parser(), extensionRegistry));
break;
}
case 32: {
msgSent_ = input.readUInt64();
break;
}
case 40: {
msgRecv_ = input.readUInt64();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
avgTime_ = com.google.protobuf.MapField.newMapField(
AvgTimeDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
avgTime__ = input.readMessage(
AvgTimeDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
avgTime_.getMutableMap().put(
avgTime__.getKey(), avgTime__.getValue());
break;
}
case 56: {
size_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
details_ = java.util.Collections.unmodifiableList(details_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Status_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetAvgTime();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Status_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Status.class, io.pinecone.proto.Core.Status.Builder.class);
}
/**
* Protobuf enum {@code core.Status.StatusCode}
*/
public enum StatusCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* success
*
*
* SUCCESS = 0;
*/
SUCCESS(0),
/**
*
* ready to use
*
*
* READY = 1;
*/
READY(1),
/**
*
* error
*
*
* ERROR = 2;
*/
ERROR(2),
/**
*
* already a existing service
*
*
* ERROR_DUPLICATE = 3;
*/
ERROR_DUPLICATE(3),
UNRECOGNIZED(-1),
;
/**
*
* success
*
*
* SUCCESS = 0;
*/
public static final int SUCCESS_VALUE = 0;
/**
*
* ready to use
*
*
* READY = 1;
*/
public static final int READY_VALUE = 1;
/**
*
* error
*
*
* ERROR = 2;
*/
public static final int ERROR_VALUE = 2;
/**
*
* already a existing service
*
*
* ERROR_DUPLICATE = 3;
*/
public static final int ERROR_DUPLICATE_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StatusCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static StatusCode forNumber(int value) {
switch (value) {
case 0: return SUCCESS;
case 1: return READY;
case 2: return ERROR;
case 3: return ERROR_DUPLICATE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
StatusCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StatusCode findValueByNumber(int number) {
return StatusCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return io.pinecone.proto.Core.Status.getDescriptor().getEnumTypes().get(0);
}
private static final StatusCode[] VALUES = values();
public static StatusCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private StatusCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:core.Status.StatusCode)
}
public interface DetailsOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.Status.Details)
com.google.protobuf.MessageOrBuilder {
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return The function.
*/
java.lang.String getFunction();
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return The bytes for function.
*/
com.google.protobuf.ByteString
getFunctionBytes();
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return The functionId.
*/
java.lang.String getFunctionId();
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return The bytes for functionId.
*/
com.google.protobuf.ByteString
getFunctionIdBytes();
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return The exception.
*/
java.lang.String getException();
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return The bytes for exception.
*/
com.google.protobuf.ByteString
getExceptionBytes();
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return The traceback.
*/
java.lang.String getTraceback();
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return The bytes for traceback.
*/
com.google.protobuf.ByteString
getTracebackBytes();
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
* @return Whether the time field is set.
*/
boolean hasTime();
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
* @return The time.
*/
com.google.protobuf.Timestamp getTime();
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
com.google.protobuf.TimestampOrBuilder getTimeOrBuilder();
}
/**
* Protobuf type {@code core.Status.Details}
*/
public static final class Details extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.Status.Details)
DetailsOrBuilder {
private static final long serialVersionUID = 0L;
// Use Details.newBuilder() to construct.
private Details(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Details() {
function_ = "";
functionId_ = "";
exception_ = "";
traceback_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Details();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Details(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
function_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
functionId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
exception_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
traceback_ = s;
break;
}
case 42: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (time_ != null) {
subBuilder = time_.toBuilder();
}
time_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(time_);
time_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Status_Details_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Status_Details_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Status.Details.class, io.pinecone.proto.Core.Status.Details.Builder.class);
}
public static final int FUNCTION_FIELD_NUMBER = 1;
private volatile java.lang.Object function_;
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return The function.
*/
@java.lang.Override
public java.lang.String getFunction() {
java.lang.Object ref = function_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
function_ = s;
return s;
}
}
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return The bytes for function.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFunctionBytes() {
java.lang.Object ref = function_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
function_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FUNCTION_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object functionId_;
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return The functionId.
*/
@java.lang.Override
public java.lang.String getFunctionId() {
java.lang.Object ref = functionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
functionId_ = s;
return s;
}
}
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return The bytes for functionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFunctionIdBytes() {
java.lang.Object ref = functionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
functionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXCEPTION_FIELD_NUMBER = 3;
private volatile java.lang.Object exception_;
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return The exception.
*/
@java.lang.Override
public java.lang.String getException() {
java.lang.Object ref = exception_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exception_ = s;
return s;
}
}
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return The bytes for exception.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExceptionBytes() {
java.lang.Object ref = exception_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exception_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRACEBACK_FIELD_NUMBER = 4;
private volatile java.lang.Object traceback_;
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return The traceback.
*/
@java.lang.Override
public java.lang.String getTraceback() {
java.lang.Object ref = traceback_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
traceback_ = s;
return s;
}
}
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return The bytes for traceback.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTracebackBytes() {
java.lang.Object ref = traceback_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
traceback_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIME_FIELD_NUMBER = 5;
private com.google.protobuf.Timestamp time_;
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
* @return Whether the time field is set.
*/
@java.lang.Override
public boolean hasTime() {
return time_ != null;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
* @return The time.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getTime() {
return time_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : time_;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getTimeOrBuilder() {
return getTime();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getFunctionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, function_);
}
if (!getFunctionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, functionId_);
}
if (!getExceptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, exception_);
}
if (!getTracebackBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, traceback_);
}
if (time_ != null) {
output.writeMessage(5, getTime());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getFunctionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, function_);
}
if (!getFunctionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, functionId_);
}
if (!getExceptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, exception_);
}
if (!getTracebackBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, traceback_);
}
if (time_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getTime());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.Status.Details)) {
return super.equals(obj);
}
io.pinecone.proto.Core.Status.Details other = (io.pinecone.proto.Core.Status.Details) obj;
if (!getFunction()
.equals(other.getFunction())) return false;
if (!getFunctionId()
.equals(other.getFunctionId())) return false;
if (!getException()
.equals(other.getException())) return false;
if (!getTraceback()
.equals(other.getTraceback())) return false;
if (hasTime() != other.hasTime()) return false;
if (hasTime()) {
if (!getTime()
.equals(other.getTime())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getFunction().hashCode();
hash = (37 * hash) + FUNCTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getFunctionId().hashCode();
hash = (37 * hash) + EXCEPTION_FIELD_NUMBER;
hash = (53 * hash) + getException().hashCode();
hash = (37 * hash) + TRACEBACK_FIELD_NUMBER;
hash = (53 * hash) + getTraceback().hashCode();
if (hasTime()) {
hash = (37 * hash) + TIME_FIELD_NUMBER;
hash = (53 * hash) + getTime().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Status.Details parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Status.Details parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Status.Details parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.Status.Details prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code core.Status.Details}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.Status.Details)
io.pinecone.proto.Core.Status.DetailsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Status_Details_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Status_Details_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Status.Details.class, io.pinecone.proto.Core.Status.Details.Builder.class);
}
// Construct using io.pinecone.proto.Core.Status.Details.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
function_ = "";
functionId_ = "";
exception_ = "";
traceback_ = "";
if (timeBuilder_ == null) {
time_ = null;
} else {
time_ = null;
timeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_Status_Details_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.Status.Details getDefaultInstanceForType() {
return io.pinecone.proto.Core.Status.Details.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.Status.Details build() {
io.pinecone.proto.Core.Status.Details result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.Status.Details buildPartial() {
io.pinecone.proto.Core.Status.Details result = new io.pinecone.proto.Core.Status.Details(this);
result.function_ = function_;
result.functionId_ = functionId_;
result.exception_ = exception_;
result.traceback_ = traceback_;
if (timeBuilder_ == null) {
result.time_ = time_;
} else {
result.time_ = timeBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.Status.Details) {
return mergeFrom((io.pinecone.proto.Core.Status.Details)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.Status.Details other) {
if (other == io.pinecone.proto.Core.Status.Details.getDefaultInstance()) return this;
if (!other.getFunction().isEmpty()) {
function_ = other.function_;
onChanged();
}
if (!other.getFunctionId().isEmpty()) {
functionId_ = other.functionId_;
onChanged();
}
if (!other.getException().isEmpty()) {
exception_ = other.exception_;
onChanged();
}
if (!other.getTraceback().isEmpty()) {
traceback_ = other.traceback_;
onChanged();
}
if (other.hasTime()) {
mergeTime(other.getTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.Status.Details parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.Status.Details) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object function_ = "";
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return The function.
*/
public java.lang.String getFunction() {
java.lang.Object ref = function_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
function_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return The bytes for function.
*/
public com.google.protobuf.ByteString
getFunctionBytes() {
java.lang.Object ref = function_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
function_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @param value The function to set.
* @return This builder for chaining.
*/
public Builder setFunction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
function_ = value;
onChanged();
return this;
}
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @return This builder for chaining.
*/
public Builder clearFunction() {
function_ = getDefaultInstance().getFunction();
onChanged();
return this;
}
/**
*
* the name of that problematic service
*
*
* string function = 1;
* @param value The bytes for function to set.
* @return This builder for chaining.
*/
public Builder setFunctionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
function_ = value;
onChanged();
return this;
}
private java.lang.Object functionId_ = "";
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return The functionId.
*/
public java.lang.String getFunctionId() {
java.lang.Object ref = functionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
functionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return The bytes for functionId.
*/
public com.google.protobuf.ByteString
getFunctionIdBytes() {
java.lang.Object ref = functionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
functionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @param value The functionId to set.
* @return This builder for chaining.
*/
public Builder setFunctionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
functionId_ = value;
onChanged();
return this;
}
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @return This builder for chaining.
*/
public Builder clearFunctionId() {
functionId_ = getDefaultInstance().getFunctionId();
onChanged();
return this;
}
/**
*
* the id of that problematic service
*
*
* string function_id = 2;
* @param value The bytes for functionId to set.
* @return This builder for chaining.
*/
public Builder setFunctionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
functionId_ = value;
onChanged();
return this;
}
private java.lang.Object exception_ = "";
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return The exception.
*/
public java.lang.String getException() {
java.lang.Object ref = exception_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exception_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return The bytes for exception.
*/
public com.google.protobuf.ByteString
getExceptionBytes() {
java.lang.Object ref = exception_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exception_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @param value The exception to set.
* @return This builder for chaining.
*/
public Builder setException(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
exception_ = value;
onChanged();
return this;
}
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @return This builder for chaining.
*/
public Builder clearException() {
exception_ = getDefaultInstance().getException();
onChanged();
return this;
}
/**
*
* the class name of the exception
*
*
* string exception = 3;
* @param value The bytes for exception to set.
* @return This builder for chaining.
*/
public Builder setExceptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
exception_ = value;
onChanged();
return this;
}
private java.lang.Object traceback_ = "";
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return The traceback.
*/
public java.lang.String getTraceback() {
java.lang.Object ref = traceback_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
traceback_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return The bytes for traceback.
*/
public com.google.protobuf.ByteString
getTracebackBytes() {
java.lang.Object ref = traceback_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
traceback_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @param value The traceback to set.
* @return This builder for chaining.
*/
public Builder setTraceback(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
traceback_ = value;
onChanged();
return this;
}
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @return This builder for chaining.
*/
public Builder clearTraceback() {
traceback_ = getDefaultInstance().getTraceback();
onChanged();
return this;
}
/**
*
* the reason of the exception
*
*
* string traceback = 4;
* @param value The bytes for traceback to set.
* @return This builder for chaining.
*/
public Builder setTracebackBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
traceback_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp time_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> timeBuilder_;
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
* @return Whether the time field is set.
*/
public boolean hasTime() {
return timeBuilder_ != null || time_ != null;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
* @return The time.
*/
public com.google.protobuf.Timestamp getTime() {
if (timeBuilder_ == null) {
return time_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : time_;
} else {
return timeBuilder_.getMessage();
}
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
public Builder setTime(com.google.protobuf.Timestamp value) {
if (timeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
time_ = value;
onChanged();
} else {
timeBuilder_.setMessage(value);
}
return this;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
public Builder setTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (timeBuilder_ == null) {
time_ = builderForValue.build();
onChanged();
} else {
timeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
public Builder mergeTime(com.google.protobuf.Timestamp value) {
if (timeBuilder_ == null) {
if (time_ != null) {
time_ =
com.google.protobuf.Timestamp.newBuilder(time_).mergeFrom(value).buildPartial();
} else {
time_ = value;
}
onChanged();
} else {
timeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
public Builder clearTime() {
if (timeBuilder_ == null) {
time_ = null;
onChanged();
} else {
time_ = null;
timeBuilder_ = null;
}
return this;
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
public com.google.protobuf.Timestamp.Builder getTimeBuilder() {
onChanged();
return getTimeFieldBuilder().getBuilder();
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
public com.google.protobuf.TimestampOrBuilder getTimeOrBuilder() {
if (timeBuilder_ != null) {
return timeBuilder_.getMessageOrBuilder();
} else {
return time_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : time_;
}
}
/**
*
* the timestamp when error occurs
*
*
* .google.protobuf.Timestamp time = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getTimeFieldBuilder() {
if (timeBuilder_ == null) {
timeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getTime(),
getParentForChildren(),
isClean());
time_ = null;
}
return timeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.Status.Details)
}
// @@protoc_insertion_point(class_scope:core.Status.Details)
private static final io.pinecone.proto.Core.Status.Details DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.Status.Details();
}
public static io.pinecone.proto.Core.Status.Details getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Details parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Details(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.Status.Details getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return The enum numeric value on the wire for code.
*/
@java.lang.Override public int getCodeValue() {
return code_;
}
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return The code.
*/
@java.lang.Override public io.pinecone.proto.Core.Status.StatusCode getCode() {
@SuppressWarnings("deprecation")
io.pinecone.proto.Core.Status.StatusCode result = io.pinecone.proto.Core.Status.StatusCode.valueOf(code_);
return result == null ? io.pinecone.proto.Core.Status.StatusCode.UNRECOGNIZED : result;
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DETAILS_FIELD_NUMBER = 3;
private java.util.List details_;
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
@java.lang.Override
public java.util.List getDetailsList() {
return details_;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
@java.lang.Override
public java.util.List extends io.pinecone.proto.Core.Status.DetailsOrBuilder>
getDetailsOrBuilderList() {
return details_;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
@java.lang.Override
public int getDetailsCount() {
return details_.size();
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.Status.Details getDetails(int index) {
return details_.get(index);
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.Status.DetailsOrBuilder getDetailsOrBuilder(
int index) {
return details_.get(index);
}
public static final int MSG_SENT_FIELD_NUMBER = 4;
private long msgSent_;
/**
*
* number of messages sent by the function
*
*
* uint64 msg_sent = 4;
* @return The msgSent.
*/
@java.lang.Override
public long getMsgSent() {
return msgSent_;
}
public static final int MSG_RECV_FIELD_NUMBER = 5;
private long msgRecv_;
/**
*
* number of messages received by the function
*
*
* uint64 msg_recv = 5;
* @return The msgRecv.
*/
@java.lang.Override
public long getMsgRecv() {
return msgRecv_;
}
public static final int AVG_TIME_FIELD_NUMBER = 6;
private static final class AvgTimeDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.Integer> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
io.pinecone.proto.Core.internal_static_core_Status_AvgTimeEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.UINT32,
0);
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Integer> avgTime_;
private com.google.protobuf.MapField
internalGetAvgTime() {
if (avgTime_ == null) {
return com.google.protobuf.MapField.emptyMapField(
AvgTimeDefaultEntryHolder.defaultEntry);
}
return avgTime_;
}
public int getAvgTimeCount() {
return internalGetAvgTime().getMap().size();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public boolean containsAvgTime(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetAvgTime().getMap().containsKey(key);
}
/**
* Use {@link #getAvgTimeMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getAvgTime() {
return getAvgTimeMap();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public java.util.Map getAvgTimeMap() {
return internalGetAvgTime().getMap();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public int getAvgTimeOrDefault(
java.lang.String key,
int defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetAvgTime().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public int getAvgTimeOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetAvgTime().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int SIZE_FIELD_NUMBER = 7;
private long size_;
/**
*
* size of a function's dataset if stateful
*
*
* uint64 size = 7;
* @return The size.
*/
@java.lang.Override
public long getSize() {
return size_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (code_ != io.pinecone.proto.Core.Status.StatusCode.SUCCESS.getNumber()) {
output.writeEnum(1, code_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
for (int i = 0; i < details_.size(); i++) {
output.writeMessage(3, details_.get(i));
}
if (msgSent_ != 0L) {
output.writeUInt64(4, msgSent_);
}
if (msgRecv_ != 0L) {
output.writeUInt64(5, msgRecv_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetAvgTime(),
AvgTimeDefaultEntryHolder.defaultEntry,
6);
if (size_ != 0L) {
output.writeUInt64(7, size_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (code_ != io.pinecone.proto.Core.Status.StatusCode.SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, code_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
for (int i = 0; i < details_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, details_.get(i));
}
if (msgSent_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, msgSent_);
}
if (msgRecv_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, msgRecv_);
}
for (java.util.Map.Entry entry
: internalGetAvgTime().getMap().entrySet()) {
com.google.protobuf.MapEntry
avgTime__ = AvgTimeDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, avgTime__);
}
if (size_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(7, size_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.Status)) {
return super.equals(obj);
}
io.pinecone.proto.Core.Status other = (io.pinecone.proto.Core.Status) obj;
if (code_ != other.code_) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (!getDetailsList()
.equals(other.getDetailsList())) return false;
if (getMsgSent()
!= other.getMsgSent()) return false;
if (getMsgRecv()
!= other.getMsgRecv()) return false;
if (!internalGetAvgTime().equals(
other.internalGetAvgTime())) return false;
if (getSize()
!= other.getSize()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + code_;
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
if (getDetailsCount() > 0) {
hash = (37 * hash) + DETAILS_FIELD_NUMBER;
hash = (53 * hash) + getDetailsList().hashCode();
}
hash = (37 * hash) + MSG_SENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMsgSent());
hash = (37 * hash) + MSG_RECV_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMsgRecv());
if (!internalGetAvgTime().getMap().isEmpty()) {
hash = (37 * hash) + AVG_TIME_FIELD_NUMBER;
hash = (53 * hash) + internalGetAvgTime().hashCode();
}
hash = (37 * hash) + SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.Status parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Status parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Status parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Status parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Status parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Status parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Status parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Status parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Status parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Status parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Status parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Status parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.Status prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code core.Status}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.Status)
io.pinecone.proto.Core.StatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Status_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetAvgTime();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 6:
return internalGetMutableAvgTime();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Status_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Status.class, io.pinecone.proto.Core.Status.Builder.class);
}
// Construct using io.pinecone.proto.Core.Status.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDetailsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
code_ = 0;
description_ = "";
if (detailsBuilder_ == null) {
details_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
detailsBuilder_.clear();
}
msgSent_ = 0L;
msgRecv_ = 0L;
internalGetMutableAvgTime().clear();
size_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_Status_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.Status getDefaultInstanceForType() {
return io.pinecone.proto.Core.Status.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.Status build() {
io.pinecone.proto.Core.Status result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.Status buildPartial() {
io.pinecone.proto.Core.Status result = new io.pinecone.proto.Core.Status(this);
int from_bitField0_ = bitField0_;
result.code_ = code_;
result.description_ = description_;
if (detailsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
details_ = java.util.Collections.unmodifiableList(details_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.details_ = details_;
} else {
result.details_ = detailsBuilder_.build();
}
result.msgSent_ = msgSent_;
result.msgRecv_ = msgRecv_;
result.avgTime_ = internalGetAvgTime();
result.avgTime_.makeImmutable();
result.size_ = size_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.Status) {
return mergeFrom((io.pinecone.proto.Core.Status)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.Status other) {
if (other == io.pinecone.proto.Core.Status.getDefaultInstance()) return this;
if (other.code_ != 0) {
setCodeValue(other.getCodeValue());
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (detailsBuilder_ == null) {
if (!other.details_.isEmpty()) {
if (details_.isEmpty()) {
details_ = other.details_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDetailsIsMutable();
details_.addAll(other.details_);
}
onChanged();
}
} else {
if (!other.details_.isEmpty()) {
if (detailsBuilder_.isEmpty()) {
detailsBuilder_.dispose();
detailsBuilder_ = null;
details_ = other.details_;
bitField0_ = (bitField0_ & ~0x00000001);
detailsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDetailsFieldBuilder() : null;
} else {
detailsBuilder_.addAllMessages(other.details_);
}
}
}
if (other.getMsgSent() != 0L) {
setMsgSent(other.getMsgSent());
}
if (other.getMsgRecv() != 0L) {
setMsgRecv(other.getMsgRecv());
}
internalGetMutableAvgTime().mergeFrom(
other.internalGetAvgTime());
if (other.getSize() != 0L) {
setSize(other.getSize());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.Status parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.Status) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int code_ = 0;
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return The enum numeric value on the wire for code.
*/
@java.lang.Override public int getCodeValue() {
return code_;
}
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @param value The enum numeric value on the wire for code to set.
* @return This builder for chaining.
*/
public Builder setCodeValue(int value) {
code_ = value;
onChanged();
return this;
}
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return The code.
*/
@java.lang.Override
public io.pinecone.proto.Core.Status.StatusCode getCode() {
@SuppressWarnings("deprecation")
io.pinecone.proto.Core.Status.StatusCode result = io.pinecone.proto.Core.Status.StatusCode.valueOf(code_);
return result == null ? io.pinecone.proto.Core.Status.StatusCode.UNRECOGNIZED : result;
}
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @param value The code to set.
* @return This builder for chaining.
*/
public Builder setCode(io.pinecone.proto.Core.Status.StatusCode value) {
if (value == null) {
throw new NullPointerException();
}
code_ = value.getNumber();
onChanged();
return this;
}
/**
*
* status code
*
*
* .core.Status.StatusCode code = 1;
* @return This builder for chaining.
*/
public Builder clearCode() {
code_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* error description of the very first exception
*
*
* string description = 2;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.util.List details_ =
java.util.Collections.emptyList();
private void ensureDetailsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
details_ = new java.util.ArrayList(details_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Status.Details, io.pinecone.proto.Core.Status.Details.Builder, io.pinecone.proto.Core.Status.DetailsOrBuilder> detailsBuilder_;
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public java.util.List getDetailsList() {
if (detailsBuilder_ == null) {
return java.util.Collections.unmodifiableList(details_);
} else {
return detailsBuilder_.getMessageList();
}
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public int getDetailsCount() {
if (detailsBuilder_ == null) {
return details_.size();
} else {
return detailsBuilder_.getCount();
}
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public io.pinecone.proto.Core.Status.Details getDetails(int index) {
if (detailsBuilder_ == null) {
return details_.get(index);
} else {
return detailsBuilder_.getMessage(index);
}
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder setDetails(
int index, io.pinecone.proto.Core.Status.Details value) {
if (detailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDetailsIsMutable();
details_.set(index, value);
onChanged();
} else {
detailsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder setDetails(
int index, io.pinecone.proto.Core.Status.Details.Builder builderForValue) {
if (detailsBuilder_ == null) {
ensureDetailsIsMutable();
details_.set(index, builderForValue.build());
onChanged();
} else {
detailsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder addDetails(io.pinecone.proto.Core.Status.Details value) {
if (detailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDetailsIsMutable();
details_.add(value);
onChanged();
} else {
detailsBuilder_.addMessage(value);
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder addDetails(
int index, io.pinecone.proto.Core.Status.Details value) {
if (detailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDetailsIsMutable();
details_.add(index, value);
onChanged();
} else {
detailsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder addDetails(
io.pinecone.proto.Core.Status.Details.Builder builderForValue) {
if (detailsBuilder_ == null) {
ensureDetailsIsMutable();
details_.add(builderForValue.build());
onChanged();
} else {
detailsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder addDetails(
int index, io.pinecone.proto.Core.Status.Details.Builder builderForValue) {
if (detailsBuilder_ == null) {
ensureDetailsIsMutable();
details_.add(index, builderForValue.build());
onChanged();
} else {
detailsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder addAllDetails(
java.lang.Iterable extends io.pinecone.proto.Core.Status.Details> values) {
if (detailsBuilder_ == null) {
ensureDetailsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, details_);
onChanged();
} else {
detailsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder clearDetails() {
if (detailsBuilder_ == null) {
details_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
detailsBuilder_.clear();
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public Builder removeDetails(int index) {
if (detailsBuilder_ == null) {
ensureDetailsIsMutable();
details_.remove(index);
onChanged();
} else {
detailsBuilder_.remove(index);
}
return this;
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public io.pinecone.proto.Core.Status.Details.Builder getDetailsBuilder(
int index) {
return getDetailsFieldBuilder().getBuilder(index);
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public io.pinecone.proto.Core.Status.DetailsOrBuilder getDetailsOrBuilder(
int index) {
if (detailsBuilder_ == null) {
return details_.get(index); } else {
return detailsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public java.util.List extends io.pinecone.proto.Core.Status.DetailsOrBuilder>
getDetailsOrBuilderList() {
if (detailsBuilder_ != null) {
return detailsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(details_);
}
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public io.pinecone.proto.Core.Status.Details.Builder addDetailsBuilder() {
return getDetailsFieldBuilder().addBuilder(
io.pinecone.proto.Core.Status.Details.getDefaultInstance());
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public io.pinecone.proto.Core.Status.Details.Builder addDetailsBuilder(
int index) {
return getDetailsFieldBuilder().addBuilder(
index, io.pinecone.proto.Core.Status.Details.getDefaultInstance());
}
/**
*
* the details of the error
*
*
* repeated .core.Status.Details details = 3;
*/
public java.util.List
getDetailsBuilderList() {
return getDetailsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Status.Details, io.pinecone.proto.Core.Status.Details.Builder, io.pinecone.proto.Core.Status.DetailsOrBuilder>
getDetailsFieldBuilder() {
if (detailsBuilder_ == null) {
detailsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Status.Details, io.pinecone.proto.Core.Status.Details.Builder, io.pinecone.proto.Core.Status.DetailsOrBuilder>(
details_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
details_ = null;
}
return detailsBuilder_;
}
private long msgSent_ ;
/**
*
* number of messages sent by the function
*
*
* uint64 msg_sent = 4;
* @return The msgSent.
*/
@java.lang.Override
public long getMsgSent() {
return msgSent_;
}
/**
*
* number of messages sent by the function
*
*
* uint64 msg_sent = 4;
* @param value The msgSent to set.
* @return This builder for chaining.
*/
public Builder setMsgSent(long value) {
msgSent_ = value;
onChanged();
return this;
}
/**
*
* number of messages sent by the function
*
*
* uint64 msg_sent = 4;
* @return This builder for chaining.
*/
public Builder clearMsgSent() {
msgSent_ = 0L;
onChanged();
return this;
}
private long msgRecv_ ;
/**
*
* number of messages received by the function
*
*
* uint64 msg_recv = 5;
* @return The msgRecv.
*/
@java.lang.Override
public long getMsgRecv() {
return msgRecv_;
}
/**
*
* number of messages received by the function
*
*
* uint64 msg_recv = 5;
* @param value The msgRecv to set.
* @return This builder for chaining.
*/
public Builder setMsgRecv(long value) {
msgRecv_ = value;
onChanged();
return this;
}
/**
*
* number of messages received by the function
*
*
* uint64 msg_recv = 5;
* @return This builder for chaining.
*/
public Builder clearMsgRecv() {
msgRecv_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.Integer> avgTime_;
private com.google.protobuf.MapField
internalGetAvgTime() {
if (avgTime_ == null) {
return com.google.protobuf.MapField.emptyMapField(
AvgTimeDefaultEntryHolder.defaultEntry);
}
return avgTime_;
}
private com.google.protobuf.MapField
internalGetMutableAvgTime() {
onChanged();;
if (avgTime_ == null) {
avgTime_ = com.google.protobuf.MapField.newMapField(
AvgTimeDefaultEntryHolder.defaultEntry);
}
if (!avgTime_.isMutable()) {
avgTime_ = avgTime_.copy();
}
return avgTime_;
}
public int getAvgTimeCount() {
return internalGetAvgTime().getMap().size();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public boolean containsAvgTime(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetAvgTime().getMap().containsKey(key);
}
/**
* Use {@link #getAvgTimeMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getAvgTime() {
return getAvgTimeMap();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public java.util.Map getAvgTimeMap() {
return internalGetAvgTime().getMap();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public int getAvgTimeOrDefault(
java.lang.String key,
int defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetAvgTime().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
@java.lang.Override
public int getAvgTimeOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetAvgTime().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearAvgTime() {
internalGetMutableAvgTime().getMutableMap()
.clear();
return this;
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
public Builder removeAvgTime(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableAvgTime().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableAvgTime() {
return internalGetMutableAvgTime().getMutableMap();
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
public Builder putAvgTime(
java.lang.String key,
int value) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableAvgTime().getMutableMap()
.put(key, value);
return this;
}
/**
*
* average time spent by messages in function
*
*
* map<string, uint32> avg_time = 6;
*/
public Builder putAllAvgTime(
java.util.Map values) {
internalGetMutableAvgTime().getMutableMap()
.putAll(values);
return this;
}
private long size_ ;
/**
*
* size of a function's dataset if stateful
*
*
* uint64 size = 7;
* @return The size.
*/
@java.lang.Override
public long getSize() {
return size_;
}
/**
*
* size of a function's dataset if stateful
*
*
* uint64 size = 7;
* @param value The size to set.
* @return This builder for chaining.
*/
public Builder setSize(long value) {
size_ = value;
onChanged();
return this;
}
/**
*
* size of a function's dataset if stateful
*
*
* uint64 size = 7;
* @return This builder for chaining.
*/
public Builder clearSize() {
size_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.Status)
}
// @@protoc_insertion_point(class_scope:core.Status)
private static final io.pinecone.proto.Core.Status DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.Status();
}
public static io.pinecone.proto.Core.Status getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Status parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Status(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.Status getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScoredResultsOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.ScoredResults)
com.google.protobuf.MessageOrBuilder {
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
java.util.List
getIdsList();
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
int getIdsCount();
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
java.lang.String getIds(int index);
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
com.google.protobuf.ByteString
getIdsBytes(int index);
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return A list containing the scores.
*/
java.util.List getScoresList();
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return The count of scores.
*/
int getScoresCount();
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @param index The index of the element to return.
* @return The scores at the given index.
*/
float getScores(int index);
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
* @return Whether the data field is set.
*/
boolean hasData();
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
* @return The data.
*/
io.pinecone.proto.Core.NdArray getData();
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder();
}
/**
* Protobuf type {@code core.ScoredResults}
*/
public static final class ScoredResults extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.ScoredResults)
ScoredResultsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ScoredResults.newBuilder() to construct.
private ScoredResults(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ScoredResults() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
scores_ = emptyFloatList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ScoredResults();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ScoredResults(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
ids_.add(s);
break;
}
case 21: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
scores_ = newFloatList();
mutable_bitField0_ |= 0x00000002;
}
scores_.addFloat(input.readFloat());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
scores_ = newFloatList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
scores_.addFloat(input.readFloat());
}
input.popLimit(limit);
break;
}
case 26: {
io.pinecone.proto.Core.NdArray.Builder subBuilder = null;
if (data_ != null) {
subBuilder = data_.toBuilder();
}
data_ = input.readMessage(io.pinecone.proto.Core.NdArray.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(data_);
data_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
scores_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_ScoredResults_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_ScoredResults_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.ScoredResults.class, io.pinecone.proto.Core.ScoredResults.Builder.class);
}
public static final int IDS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList ids_;
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_;
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
public static final int SCORES_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.FloatList scores_;
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return A list containing the scores.
*/
@java.lang.Override
public java.util.List
getScoresList() {
return scores_;
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return The count of scores.
*/
public int getScoresCount() {
return scores_.size();
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @param index The index of the element to return.
* @return The scores at the given index.
*/
public float getScores(int index) {
return scores_.getFloat(index);
}
private int scoresMemoizedSerializedSize = -1;
public static final int DATA_FIELD_NUMBER = 3;
private io.pinecone.proto.Core.NdArray data_;
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return data_ != null;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
* @return The data.
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArray getData() {
return data_ == null ? io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder() {
return getData();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < ids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, ids_.getRaw(i));
}
if (getScoresList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(scoresMemoizedSerializedSize);
}
for (int i = 0; i < scores_.size(); i++) {
output.writeFloatNoTag(scores_.getFloat(i));
}
if (data_ != null) {
output.writeMessage(3, getData());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ids_.size(); i++) {
dataSize += computeStringSizeNoTag(ids_.getRaw(i));
}
size += dataSize;
size += 1 * getIdsList().size();
}
{
int dataSize = 0;
dataSize = 4 * getScoresList().size();
size += dataSize;
if (!getScoresList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
scoresMemoizedSerializedSize = dataSize;
}
if (data_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getData());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.ScoredResults)) {
return super.equals(obj);
}
io.pinecone.proto.Core.ScoredResults other = (io.pinecone.proto.Core.ScoredResults) obj;
if (!getIdsList()
.equals(other.getIdsList())) return false;
if (!getScoresList()
.equals(other.getScoresList())) return false;
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getIdsCount() > 0) {
hash = (37 * hash) + IDS_FIELD_NUMBER;
hash = (53 * hash) + getIdsList().hashCode();
}
if (getScoresCount() > 0) {
hash = (37 * hash) + SCORES_FIELD_NUMBER;
hash = (53 * hash) + getScoresList().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.ScoredResults parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.ScoredResults parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.ScoredResults parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.ScoredResults prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code core.ScoredResults}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.ScoredResults)
io.pinecone.proto.Core.ScoredResultsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_ScoredResults_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_ScoredResults_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.ScoredResults.class, io.pinecone.proto.Core.ScoredResults.Builder.class);
}
// Construct using io.pinecone.proto.Core.ScoredResults.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
scores_ = emptyFloatList();
bitField0_ = (bitField0_ & ~0x00000002);
if (dataBuilder_ == null) {
data_ = null;
} else {
data_ = null;
dataBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_ScoredResults_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.ScoredResults getDefaultInstanceForType() {
return io.pinecone.proto.Core.ScoredResults.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.ScoredResults build() {
io.pinecone.proto.Core.ScoredResults result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.ScoredResults buildPartial() {
io.pinecone.proto.Core.ScoredResults result = new io.pinecone.proto.Core.ScoredResults(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ids_ = ids_;
if (((bitField0_ & 0x00000002) != 0)) {
scores_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.scores_ = scores_;
if (dataBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = dataBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.ScoredResults) {
return mergeFrom((io.pinecone.proto.Core.ScoredResults)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.ScoredResults other) {
if (other == io.pinecone.proto.Core.ScoredResults.getDefaultInstance()) return this;
if (!other.ids_.isEmpty()) {
if (ids_.isEmpty()) {
ids_ = other.ids_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIdsIsMutable();
ids_.addAll(other.ids_);
}
onChanged();
}
if (!other.scores_.isEmpty()) {
if (scores_.isEmpty()) {
scores_ = other.scores_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureScoresIsMutable();
scores_.addAll(other.scores_);
}
onChanged();
}
if (other.hasData()) {
mergeData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.ScoredResults parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.ScoredResults) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList(ids_);
bitField0_ |= 0x00000001;
}
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_.getUnmodifiableView();
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param index The index to set the value at.
* @param value The ids to set.
* @return This builder for chaining.
*/
public Builder setIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.set(index, value);
onChanged();
return this;
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param value The ids to add.
* @return This builder for chaining.
*/
public Builder addIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param values The ids to add.
* @return This builder for chaining.
*/
public Builder addAllIds(
java.lang.Iterable values) {
ensureIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ids_);
onChanged();
return this;
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @return This builder for chaining.
*/
public Builder clearIds() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* String ids of the item results of a query
*
*
* repeated string ids = 1;
* @param value The bytes of the ids to add.
* @return This builder for chaining.
*/
public Builder addIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
private com.google.protobuf.Internal.FloatList scores_ = emptyFloatList();
private void ensureScoresIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
scores_ = mutableCopy(scores_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return A list containing the scores.
*/
public java.util.List
getScoresList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(scores_) : scores_;
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return The count of scores.
*/
public int getScoresCount() {
return scores_.size();
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @param index The index of the element to return.
* @return The scores at the given index.
*/
public float getScores(int index) {
return scores_.getFloat(index);
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @param index The index to set the value at.
* @param value The scores to set.
* @return This builder for chaining.
*/
public Builder setScores(
int index, float value) {
ensureScoresIsMutable();
scores_.setFloat(index, value);
onChanged();
return this;
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @param value The scores to add.
* @return This builder for chaining.
*/
public Builder addScores(float value) {
ensureScoresIsMutable();
scores_.addFloat(value);
onChanged();
return this;
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @param values The scores to add.
* @return This builder for chaining.
*/
public Builder addAllScores(
java.lang.Iterable extends java.lang.Float> values) {
ensureScoresIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, scores_);
onChanged();
return this;
}
/**
*
* Corresponding pairwise scores between the query to each item
*
*
* repeated float scores = 2;
* @return This builder for chaining.
*/
public Builder clearScores() {
scores_ = emptyFloatList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private io.pinecone.proto.Core.NdArray data_;
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder> dataBuilder_;
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
* @return Whether the data field is set.
*/
public boolean hasData() {
return dataBuilder_ != null || data_ != null;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
* @return The data.
*/
public io.pinecone.proto.Core.NdArray getData() {
if (dataBuilder_ == null) {
return data_ == null ? io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
} else {
return dataBuilder_.getMessage();
}
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
public Builder setData(io.pinecone.proto.Core.NdArray value) {
if (dataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
dataBuilder_.setMessage(value);
}
return this;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
public Builder setData(
io.pinecone.proto.Core.NdArray.Builder builderForValue) {
if (dataBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
dataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
public Builder mergeData(io.pinecone.proto.Core.NdArray value) {
if (dataBuilder_ == null) {
if (data_ != null) {
data_ =
io.pinecone.proto.Core.NdArray.newBuilder(data_).mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
dataBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
public Builder clearData() {
if (dataBuilder_ == null) {
data_ = null;
onChanged();
} else {
data_ = null;
dataBuilder_ = null;
}
return this;
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
public io.pinecone.proto.Core.NdArray.Builder getDataBuilder() {
onChanged();
return getDataFieldBuilder().getBuilder();
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
public io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder() {
if (dataBuilder_ != null) {
return dataBuilder_.getMessageOrBuilder();
} else {
return data_ == null ?
io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
}
}
/**
*
* Corresponding data for all of these items, if requested
*
*
* .core.NdArray data = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>
getDataFieldBuilder() {
if (dataBuilder_ == null) {
dataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>(
getData(),
getParentForChildren(),
isClean());
data_ = null;
}
return dataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.ScoredResults)
}
// @@protoc_insertion_point(class_scope:core.ScoredResults)
private static final io.pinecone.proto.Core.ScoredResults DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.ScoredResults();
}
public static io.pinecone.proto.Core.ScoredResults getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ScoredResults parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ScoredResults(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.ScoredResults getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IndexRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.IndexRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
java.util.List
getIdsList();
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
int getIdsCount();
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
java.lang.String getIds(int index);
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
com.google.protobuf.ByteString
getIdsBytes(int index);
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
* @return Whether the data field is set.
*/
boolean hasData();
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
* @return The data.
*/
io.pinecone.proto.Core.NdArray getData();
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder();
}
/**
*
**
* Represents an index request
*
*
* Protobuf type {@code core.IndexRequest}
*/
public static final class IndexRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.IndexRequest)
IndexRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use IndexRequest.newBuilder() to construct.
private IndexRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IndexRequest() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IndexRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IndexRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
ids_.add(s);
break;
}
case 18: {
io.pinecone.proto.Core.NdArray.Builder subBuilder = null;
if (data_ != null) {
subBuilder = data_.toBuilder();
}
data_ = input.readMessage(io.pinecone.proto.Core.NdArray.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(data_);
data_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_IndexRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_IndexRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.IndexRequest.class, io.pinecone.proto.Core.IndexRequest.Builder.class);
}
public static final int IDS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList ids_;
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_;
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
public static final int DATA_FIELD_NUMBER = 2;
private io.pinecone.proto.Core.NdArray data_;
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return data_ != null;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
* @return The data.
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArray getData() {
return data_ == null ? io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder() {
return getData();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < ids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, ids_.getRaw(i));
}
if (data_ != null) {
output.writeMessage(2, getData());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ids_.size(); i++) {
dataSize += computeStringSizeNoTag(ids_.getRaw(i));
}
size += dataSize;
size += 1 * getIdsList().size();
}
if (data_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getData());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.IndexRequest)) {
return super.equals(obj);
}
io.pinecone.proto.Core.IndexRequest other = (io.pinecone.proto.Core.IndexRequest) obj;
if (!getIdsList()
.equals(other.getIdsList())) return false;
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getIdsCount() > 0) {
hash = (37 * hash) + IDS_FIELD_NUMBER;
hash = (53 * hash) + getIdsList().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.IndexRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.IndexRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.IndexRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.IndexRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents an index request
*
*
* Protobuf type {@code core.IndexRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.IndexRequest)
io.pinecone.proto.Core.IndexRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_IndexRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_IndexRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.IndexRequest.class, io.pinecone.proto.Core.IndexRequest.Builder.class);
}
// Construct using io.pinecone.proto.Core.IndexRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (dataBuilder_ == null) {
data_ = null;
} else {
data_ = null;
dataBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_IndexRequest_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.IndexRequest getDefaultInstanceForType() {
return io.pinecone.proto.Core.IndexRequest.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.IndexRequest build() {
io.pinecone.proto.Core.IndexRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.IndexRequest buildPartial() {
io.pinecone.proto.Core.IndexRequest result = new io.pinecone.proto.Core.IndexRequest(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ids_ = ids_;
if (dataBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = dataBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.IndexRequest) {
return mergeFrom((io.pinecone.proto.Core.IndexRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.IndexRequest other) {
if (other == io.pinecone.proto.Core.IndexRequest.getDefaultInstance()) return this;
if (!other.ids_.isEmpty()) {
if (ids_.isEmpty()) {
ids_ = other.ids_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIdsIsMutable();
ids_.addAll(other.ids_);
}
onChanged();
}
if (other.hasData()) {
mergeData(other.getData());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.IndexRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.IndexRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList(ids_);
bitField0_ |= 0x00000001;
}
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_.getUnmodifiableView();
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param index The index to set the value at.
* @param value The ids to set.
* @return This builder for chaining.
*/
public Builder setIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.set(index, value);
onChanged();
return this;
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param value The ids to add.
* @return This builder for chaining.
*/
public Builder addIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param values The ids to add.
* @return This builder for chaining.
*/
public Builder addAllIds(
java.lang.Iterable values) {
ensureIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ids_);
onChanged();
return this;
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @return This builder for chaining.
*/
public Builder clearIds() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* ids of items to be inserted
*
*
* repeated string ids = 1;
* @param value The bytes of the ids to add.
* @return This builder for chaining.
*/
public Builder addIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
private io.pinecone.proto.Core.NdArray data_;
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder> dataBuilder_;
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
* @return Whether the data field is set.
*/
public boolean hasData() {
return dataBuilder_ != null || data_ != null;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
* @return The data.
*/
public io.pinecone.proto.Core.NdArray getData() {
if (dataBuilder_ == null) {
return data_ == null ? io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
} else {
return dataBuilder_.getMessage();
}
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
public Builder setData(io.pinecone.proto.Core.NdArray value) {
if (dataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
dataBuilder_.setMessage(value);
}
return this;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
public Builder setData(
io.pinecone.proto.Core.NdArray.Builder builderForValue) {
if (dataBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
dataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
public Builder mergeData(io.pinecone.proto.Core.NdArray value) {
if (dataBuilder_ == null) {
if (data_ != null) {
data_ =
io.pinecone.proto.Core.NdArray.newBuilder(data_).mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
dataBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
public Builder clearData() {
if (dataBuilder_ == null) {
data_ = null;
onChanged();
} else {
data_ = null;
dataBuilder_ = null;
}
return this;
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
public io.pinecone.proto.Core.NdArray.Builder getDataBuilder() {
onChanged();
return getDataFieldBuilder().getBuilder();
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
public io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder() {
if (dataBuilder_ != null) {
return dataBuilder_.getMessageOrBuilder();
} else {
return data_ == null ?
io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
}
}
/**
*
* vectors of items to be inserted
*
*
* .core.NdArray data = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>
getDataFieldBuilder() {
if (dataBuilder_ == null) {
dataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>(
getData(),
getParentForChildren(),
isClean());
data_ = null;
}
return dataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.IndexRequest)
}
// @@protoc_insertion_point(class_scope:core.IndexRequest)
private static final io.pinecone.proto.Core.IndexRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.IndexRequest();
}
public static io.pinecone.proto.Core.IndexRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndexRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IndexRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.IndexRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.QueryRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Number of results to return for each query
*
*
* uint32 top_k = 1;
* @return The topK.
*/
int getTopK();
/**
*
* Whether to include the vectors and raw data in response as well as ids
*
*
* bool include_data = 2;
* @return The includeData.
*/
boolean getIncludeData();
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
* @return Whether the data field is set.
*/
boolean hasData();
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
* @return The data.
*/
io.pinecone.proto.Core.NdArray getData();
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder();
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
java.util.List
getMatchesList();
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
io.pinecone.proto.Core.ScoredResults getMatches(int index);
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
int getMatchesCount();
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
java.util.List extends io.pinecone.proto.Core.ScoredResultsOrBuilder>
getMatchesOrBuilderList();
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
io.pinecone.proto.Core.ScoredResultsOrBuilder getMatchesOrBuilder(
int index);
}
/**
*
**
* Represents a query request
*
*
* Protobuf type {@code core.QueryRequest}
*/
public static final class QueryRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.QueryRequest)
QueryRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryRequest.newBuilder() to construct.
private QueryRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryRequest() {
matches_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new QueryRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
topK_ = input.readUInt32();
break;
}
case 16: {
includeData_ = input.readBool();
break;
}
case 26: {
io.pinecone.proto.Core.NdArray.Builder subBuilder = null;
if (data_ != null) {
subBuilder = data_.toBuilder();
}
data_ = input.readMessage(io.pinecone.proto.Core.NdArray.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(data_);
data_ = subBuilder.buildPartial();
}
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
matches_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
matches_.add(
input.readMessage(io.pinecone.proto.Core.ScoredResults.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
matches_ = java.util.Collections.unmodifiableList(matches_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_QueryRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_QueryRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.QueryRequest.class, io.pinecone.proto.Core.QueryRequest.Builder.class);
}
public static final int TOP_K_FIELD_NUMBER = 1;
private int topK_;
/**
*
* Number of results to return for each query
*
*
* uint32 top_k = 1;
* @return The topK.
*/
@java.lang.Override
public int getTopK() {
return topK_;
}
public static final int INCLUDE_DATA_FIELD_NUMBER = 2;
private boolean includeData_;
/**
*
* Whether to include the vectors and raw data in response as well as ids
*
*
* bool include_data = 2;
* @return The includeData.
*/
@java.lang.Override
public boolean getIncludeData() {
return includeData_;
}
public static final int DATA_FIELD_NUMBER = 3;
private io.pinecone.proto.Core.NdArray data_;
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return data_ != null;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
* @return The data.
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArray getData() {
return data_ == null ? io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder() {
return getData();
}
public static final int MATCHES_FIELD_NUMBER = 4;
private java.util.List matches_;
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
@java.lang.Override
public java.util.List getMatchesList() {
return matches_;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
@java.lang.Override
public java.util.List extends io.pinecone.proto.Core.ScoredResultsOrBuilder>
getMatchesOrBuilderList() {
return matches_;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
@java.lang.Override
public int getMatchesCount() {
return matches_.size();
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
@java.lang.Override
public io.pinecone.proto.Core.ScoredResults getMatches(int index) {
return matches_.get(index);
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
@java.lang.Override
public io.pinecone.proto.Core.ScoredResultsOrBuilder getMatchesOrBuilder(
int index) {
return matches_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (topK_ != 0) {
output.writeUInt32(1, topK_);
}
if (includeData_ != false) {
output.writeBool(2, includeData_);
}
if (data_ != null) {
output.writeMessage(3, getData());
}
for (int i = 0; i < matches_.size(); i++) {
output.writeMessage(4, matches_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (topK_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, topK_);
}
if (includeData_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, includeData_);
}
if (data_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getData());
}
for (int i = 0; i < matches_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, matches_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.QueryRequest)) {
return super.equals(obj);
}
io.pinecone.proto.Core.QueryRequest other = (io.pinecone.proto.Core.QueryRequest) obj;
if (getTopK()
!= other.getTopK()) return false;
if (getIncludeData()
!= other.getIncludeData()) return false;
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (!getMatchesList()
.equals(other.getMatchesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TOP_K_FIELD_NUMBER;
hash = (53 * hash) + getTopK();
hash = (37 * hash) + INCLUDE_DATA_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIncludeData());
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
if (getMatchesCount() > 0) {
hash = (37 * hash) + MATCHES_FIELD_NUMBER;
hash = (53 * hash) + getMatchesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.QueryRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.QueryRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.QueryRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.QueryRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents a query request
*
*
* Protobuf type {@code core.QueryRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.QueryRequest)
io.pinecone.proto.Core.QueryRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_QueryRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_QueryRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.QueryRequest.class, io.pinecone.proto.Core.QueryRequest.Builder.class);
}
// Construct using io.pinecone.proto.Core.QueryRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMatchesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
topK_ = 0;
includeData_ = false;
if (dataBuilder_ == null) {
data_ = null;
} else {
data_ = null;
dataBuilder_ = null;
}
if (matchesBuilder_ == null) {
matches_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
matchesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_QueryRequest_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.QueryRequest getDefaultInstanceForType() {
return io.pinecone.proto.Core.QueryRequest.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.QueryRequest build() {
io.pinecone.proto.Core.QueryRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.QueryRequest buildPartial() {
io.pinecone.proto.Core.QueryRequest result = new io.pinecone.proto.Core.QueryRequest(this);
int from_bitField0_ = bitField0_;
result.topK_ = topK_;
result.includeData_ = includeData_;
if (dataBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = dataBuilder_.build();
}
if (matchesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
matches_ = java.util.Collections.unmodifiableList(matches_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.matches_ = matches_;
} else {
result.matches_ = matchesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.QueryRequest) {
return mergeFrom((io.pinecone.proto.Core.QueryRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.QueryRequest other) {
if (other == io.pinecone.proto.Core.QueryRequest.getDefaultInstance()) return this;
if (other.getTopK() != 0) {
setTopK(other.getTopK());
}
if (other.getIncludeData() != false) {
setIncludeData(other.getIncludeData());
}
if (other.hasData()) {
mergeData(other.getData());
}
if (matchesBuilder_ == null) {
if (!other.matches_.isEmpty()) {
if (matches_.isEmpty()) {
matches_ = other.matches_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMatchesIsMutable();
matches_.addAll(other.matches_);
}
onChanged();
}
} else {
if (!other.matches_.isEmpty()) {
if (matchesBuilder_.isEmpty()) {
matchesBuilder_.dispose();
matchesBuilder_ = null;
matches_ = other.matches_;
bitField0_ = (bitField0_ & ~0x00000001);
matchesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMatchesFieldBuilder() : null;
} else {
matchesBuilder_.addAllMessages(other.matches_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.QueryRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.QueryRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int topK_ ;
/**
*
* Number of results to return for each query
*
*
* uint32 top_k = 1;
* @return The topK.
*/
@java.lang.Override
public int getTopK() {
return topK_;
}
/**
*
* Number of results to return for each query
*
*
* uint32 top_k = 1;
* @param value The topK to set.
* @return This builder for chaining.
*/
public Builder setTopK(int value) {
topK_ = value;
onChanged();
return this;
}
/**
*
* Number of results to return for each query
*
*
* uint32 top_k = 1;
* @return This builder for chaining.
*/
public Builder clearTopK() {
topK_ = 0;
onChanged();
return this;
}
private boolean includeData_ ;
/**
*
* Whether to include the vectors and raw data in response as well as ids
*
*
* bool include_data = 2;
* @return The includeData.
*/
@java.lang.Override
public boolean getIncludeData() {
return includeData_;
}
/**
*
* Whether to include the vectors and raw data in response as well as ids
*
*
* bool include_data = 2;
* @param value The includeData to set.
* @return This builder for chaining.
*/
public Builder setIncludeData(boolean value) {
includeData_ = value;
onChanged();
return this;
}
/**
*
* Whether to include the vectors and raw data in response as well as ids
*
*
* bool include_data = 2;
* @return This builder for chaining.
*/
public Builder clearIncludeData() {
includeData_ = false;
onChanged();
return this;
}
private io.pinecone.proto.Core.NdArray data_;
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder> dataBuilder_;
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
* @return Whether the data field is set.
*/
public boolean hasData() {
return dataBuilder_ != null || data_ != null;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
* @return The data.
*/
public io.pinecone.proto.Core.NdArray getData() {
if (dataBuilder_ == null) {
return data_ == null ? io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
} else {
return dataBuilder_.getMessage();
}
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
public Builder setData(io.pinecone.proto.Core.NdArray value) {
if (dataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
dataBuilder_.setMessage(value);
}
return this;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
public Builder setData(
io.pinecone.proto.Core.NdArray.Builder builderForValue) {
if (dataBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
dataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
public Builder mergeData(io.pinecone.proto.Core.NdArray value) {
if (dataBuilder_ == null) {
if (data_ != null) {
data_ =
io.pinecone.proto.Core.NdArray.newBuilder(data_).mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
dataBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
public Builder clearData() {
if (dataBuilder_ == null) {
data_ = null;
onChanged();
} else {
data_ = null;
dataBuilder_ = null;
}
return this;
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
public io.pinecone.proto.Core.NdArray.Builder getDataBuilder() {
onChanged();
return getDataFieldBuilder().getBuilder();
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
public io.pinecone.proto.Core.NdArrayOrBuilder getDataOrBuilder() {
if (dataBuilder_ != null) {
return dataBuilder_.getMessageOrBuilder();
} else {
return data_ == null ?
io.pinecone.proto.Core.NdArray.getDefaultInstance() : data_;
}
}
/**
*
* The batch of vectors to query
*
*
* .core.NdArray data = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>
getDataFieldBuilder() {
if (dataBuilder_ == null) {
dataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>(
getData(),
getParentForChildren(),
isClean());
data_ = null;
}
return dataBuilder_;
}
private java.util.List matches_ =
java.util.Collections.emptyList();
private void ensureMatchesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
matches_ = new java.util.ArrayList(matches_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.ScoredResults, io.pinecone.proto.Core.ScoredResults.Builder, io.pinecone.proto.Core.ScoredResultsOrBuilder> matchesBuilder_;
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public java.util.List getMatchesList() {
if (matchesBuilder_ == null) {
return java.util.Collections.unmodifiableList(matches_);
} else {
return matchesBuilder_.getMessageList();
}
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public int getMatchesCount() {
if (matchesBuilder_ == null) {
return matches_.size();
} else {
return matchesBuilder_.getCount();
}
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public io.pinecone.proto.Core.ScoredResults getMatches(int index) {
if (matchesBuilder_ == null) {
return matches_.get(index);
} else {
return matchesBuilder_.getMessage(index);
}
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder setMatches(
int index, io.pinecone.proto.Core.ScoredResults value) {
if (matchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMatchesIsMutable();
matches_.set(index, value);
onChanged();
} else {
matchesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder setMatches(
int index, io.pinecone.proto.Core.ScoredResults.Builder builderForValue) {
if (matchesBuilder_ == null) {
ensureMatchesIsMutable();
matches_.set(index, builderForValue.build());
onChanged();
} else {
matchesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder addMatches(io.pinecone.proto.Core.ScoredResults value) {
if (matchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMatchesIsMutable();
matches_.add(value);
onChanged();
} else {
matchesBuilder_.addMessage(value);
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder addMatches(
int index, io.pinecone.proto.Core.ScoredResults value) {
if (matchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMatchesIsMutable();
matches_.add(index, value);
onChanged();
} else {
matchesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder addMatches(
io.pinecone.proto.Core.ScoredResults.Builder builderForValue) {
if (matchesBuilder_ == null) {
ensureMatchesIsMutable();
matches_.add(builderForValue.build());
onChanged();
} else {
matchesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder addMatches(
int index, io.pinecone.proto.Core.ScoredResults.Builder builderForValue) {
if (matchesBuilder_ == null) {
ensureMatchesIsMutable();
matches_.add(index, builderForValue.build());
onChanged();
} else {
matchesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder addAllMatches(
java.lang.Iterable extends io.pinecone.proto.Core.ScoredResults> values) {
if (matchesBuilder_ == null) {
ensureMatchesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, matches_);
onChanged();
} else {
matchesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder clearMatches() {
if (matchesBuilder_ == null) {
matches_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
matchesBuilder_.clear();
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public Builder removeMatches(int index) {
if (matchesBuilder_ == null) {
ensureMatchesIsMutable();
matches_.remove(index);
onChanged();
} else {
matchesBuilder_.remove(index);
}
return this;
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public io.pinecone.proto.Core.ScoredResults.Builder getMatchesBuilder(
int index) {
return getMatchesFieldBuilder().getBuilder(index);
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public io.pinecone.proto.Core.ScoredResultsOrBuilder getMatchesOrBuilder(
int index) {
if (matchesBuilder_ == null) {
return matches_.get(index); } else {
return matchesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public java.util.List extends io.pinecone.proto.Core.ScoredResultsOrBuilder>
getMatchesOrBuilderList() {
if (matchesBuilder_ != null) {
return matchesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(matches_);
}
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public io.pinecone.proto.Core.ScoredResults.Builder addMatchesBuilder() {
return getMatchesFieldBuilder().addBuilder(
io.pinecone.proto.Core.ScoredResults.getDefaultInstance());
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public io.pinecone.proto.Core.ScoredResults.Builder addMatchesBuilder(
int index) {
return getMatchesFieldBuilder().addBuilder(
index, io.pinecone.proto.Core.ScoredResults.getDefaultInstance());
}
/**
*
* The corresponding matches returned for each query in the batch
*
*
* repeated .core.ScoredResults matches = 4;
*/
public java.util.List
getMatchesBuilderList() {
return getMatchesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.ScoredResults, io.pinecone.proto.Core.ScoredResults.Builder, io.pinecone.proto.Core.ScoredResultsOrBuilder>
getMatchesFieldBuilder() {
if (matchesBuilder_ == null) {
matchesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.ScoredResults, io.pinecone.proto.Core.ScoredResults.Builder, io.pinecone.proto.Core.ScoredResultsOrBuilder>(
matches_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
matches_ = null;
}
return matchesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.QueryRequest)
}
// @@protoc_insertion_point(class_scope:core.QueryRequest)
private static final io.pinecone.proto.Core.QueryRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.QueryRequest();
}
public static io.pinecone.proto.Core.QueryRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.QueryRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DeleteRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.DeleteRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
java.util.List
getIdsList();
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
int getIdsCount();
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
java.lang.String getIds(int index);
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
com.google.protobuf.ByteString
getIdsBytes(int index);
}
/**
*
**
* Represents a delete request
*
*
* Protobuf type {@code core.DeleteRequest}
*/
public static final class DeleteRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.DeleteRequest)
DeleteRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeleteRequest.newBuilder() to construct.
private DeleteRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeleteRequest() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DeleteRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DeleteRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
ids_.add(s);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_DeleteRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_DeleteRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.DeleteRequest.class, io.pinecone.proto.Core.DeleteRequest.Builder.class);
}
public static final int IDS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList ids_;
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_;
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < ids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, ids_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ids_.size(); i++) {
dataSize += computeStringSizeNoTag(ids_.getRaw(i));
}
size += dataSize;
size += 1 * getIdsList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.DeleteRequest)) {
return super.equals(obj);
}
io.pinecone.proto.Core.DeleteRequest other = (io.pinecone.proto.Core.DeleteRequest) obj;
if (!getIdsList()
.equals(other.getIdsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getIdsCount() > 0) {
hash = (37 * hash) + IDS_FIELD_NUMBER;
hash = (53 * hash) + getIdsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.DeleteRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.DeleteRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.DeleteRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.DeleteRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents a delete request
*
*
* Protobuf type {@code core.DeleteRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.DeleteRequest)
io.pinecone.proto.Core.DeleteRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_DeleteRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_DeleteRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.DeleteRequest.class, io.pinecone.proto.Core.DeleteRequest.Builder.class);
}
// Construct using io.pinecone.proto.Core.DeleteRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_DeleteRequest_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequest getDefaultInstanceForType() {
return io.pinecone.proto.Core.DeleteRequest.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequest build() {
io.pinecone.proto.Core.DeleteRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequest buildPartial() {
io.pinecone.proto.Core.DeleteRequest result = new io.pinecone.proto.Core.DeleteRequest(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ids_ = ids_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.DeleteRequest) {
return mergeFrom((io.pinecone.proto.Core.DeleteRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.DeleteRequest other) {
if (other == io.pinecone.proto.Core.DeleteRequest.getDefaultInstance()) return this;
if (!other.ids_.isEmpty()) {
if (ids_.isEmpty()) {
ids_ = other.ids_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIdsIsMutable();
ids_.addAll(other.ids_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.DeleteRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.DeleteRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList(ids_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_.getUnmodifiableView();
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param index The index to set the value at.
* @param value The ids to set.
* @return This builder for chaining.
*/
public Builder setIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.set(index, value);
onChanged();
return this;
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param value The ids to add.
* @return This builder for chaining.
*/
public Builder addIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param values The ids to add.
* @return This builder for chaining.
*/
public Builder addAllIds(
java.lang.Iterable values) {
ensureIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ids_);
onChanged();
return this;
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @return This builder for chaining.
*/
public Builder clearIds() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Ids of items to delete
*
*
* repeated string ids = 1;
* @param value The bytes of the ids to add.
* @return This builder for chaining.
*/
public Builder addIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.DeleteRequest)
}
// @@protoc_insertion_point(class_scope:core.DeleteRequest)
private static final io.pinecone.proto.Core.DeleteRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.DeleteRequest();
}
public static io.pinecone.proto.Core.DeleteRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeleteRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DeleteRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.DeleteRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InfoRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.InfoRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Size of a stateful function (Index)
*
*
* uint64 index_size = 1;
* @return The indexSize.
*/
long getIndexSize();
}
/**
*
**
* Represents an info request
*
*
* Protobuf type {@code core.InfoRequest}
*/
public static final class InfoRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.InfoRequest)
InfoRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use InfoRequest.newBuilder() to construct.
private InfoRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InfoRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new InfoRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InfoRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
indexSize_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_InfoRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_InfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.InfoRequest.class, io.pinecone.proto.Core.InfoRequest.Builder.class);
}
public static final int INDEX_SIZE_FIELD_NUMBER = 1;
private long indexSize_;
/**
*
* Size of a stateful function (Index)
*
*
* uint64 index_size = 1;
* @return The indexSize.
*/
@java.lang.Override
public long getIndexSize() {
return indexSize_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (indexSize_ != 0L) {
output.writeUInt64(1, indexSize_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (indexSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, indexSize_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.InfoRequest)) {
return super.equals(obj);
}
io.pinecone.proto.Core.InfoRequest other = (io.pinecone.proto.Core.InfoRequest) obj;
if (getIndexSize()
!= other.getIndexSize()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INDEX_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndexSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.InfoRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.InfoRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.InfoRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.InfoRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents an info request
*
*
* Protobuf type {@code core.InfoRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.InfoRequest)
io.pinecone.proto.Core.InfoRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_InfoRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_InfoRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.InfoRequest.class, io.pinecone.proto.Core.InfoRequest.Builder.class);
}
// Construct using io.pinecone.proto.Core.InfoRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
indexSize_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_InfoRequest_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.InfoRequest getDefaultInstanceForType() {
return io.pinecone.proto.Core.InfoRequest.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.InfoRequest build() {
io.pinecone.proto.Core.InfoRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.InfoRequest buildPartial() {
io.pinecone.proto.Core.InfoRequest result = new io.pinecone.proto.Core.InfoRequest(this);
result.indexSize_ = indexSize_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.InfoRequest) {
return mergeFrom((io.pinecone.proto.Core.InfoRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.InfoRequest other) {
if (other == io.pinecone.proto.Core.InfoRequest.getDefaultInstance()) return this;
if (other.getIndexSize() != 0L) {
setIndexSize(other.getIndexSize());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.InfoRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.InfoRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long indexSize_ ;
/**
*
* Size of a stateful function (Index)
*
*
* uint64 index_size = 1;
* @return The indexSize.
*/
@java.lang.Override
public long getIndexSize() {
return indexSize_;
}
/**
*
* Size of a stateful function (Index)
*
*
* uint64 index_size = 1;
* @param value The indexSize to set.
* @return This builder for chaining.
*/
public Builder setIndexSize(long value) {
indexSize_ = value;
onChanged();
return this;
}
/**
*
* Size of a stateful function (Index)
*
*
* uint64 index_size = 1;
* @return This builder for chaining.
*/
public Builder clearIndexSize() {
indexSize_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.InfoRequest)
}
// @@protoc_insertion_point(class_scope:core.InfoRequest)
private static final io.pinecone.proto.Core.InfoRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.InfoRequest();
}
public static io.pinecone.proto.Core.InfoRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InfoRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InfoRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.InfoRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FetchRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.FetchRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
java.util.List
getIdsList();
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
int getIdsCount();
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
java.lang.String getIds(int index);
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
com.google.protobuf.ByteString
getIdsBytes(int index);
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
java.util.List
getVectorsList();
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
io.pinecone.proto.Core.NdArray getVectors(int index);
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
int getVectorsCount();
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
java.util.List extends io.pinecone.proto.Core.NdArrayOrBuilder>
getVectorsOrBuilderList();
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
io.pinecone.proto.Core.NdArrayOrBuilder getVectorsOrBuilder(
int index);
}
/**
*
**
* Represents a fetch request
*
*
* Protobuf type {@code core.FetchRequest}
*/
public static final class FetchRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.FetchRequest)
FetchRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use FetchRequest.newBuilder() to construct.
private FetchRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FetchRequest() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
vectors_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FetchRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FetchRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
ids_.add(s);
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
vectors_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
vectors_.add(
input.readMessage(io.pinecone.proto.Core.NdArray.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
vectors_ = java.util.Collections.unmodifiableList(vectors_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_FetchRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_FetchRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.FetchRequest.class, io.pinecone.proto.Core.FetchRequest.Builder.class);
}
public static final int IDS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList ids_;
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_;
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
public static final int VECTORS_FIELD_NUMBER = 2;
private java.util.List vectors_;
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
@java.lang.Override
public java.util.List getVectorsList() {
return vectors_;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
@java.lang.Override
public java.util.List extends io.pinecone.proto.Core.NdArrayOrBuilder>
getVectorsOrBuilderList() {
return vectors_;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
@java.lang.Override
public int getVectorsCount() {
return vectors_.size();
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArray getVectors(int index) {
return vectors_.get(index);
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
@java.lang.Override
public io.pinecone.proto.Core.NdArrayOrBuilder getVectorsOrBuilder(
int index) {
return vectors_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < ids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, ids_.getRaw(i));
}
for (int i = 0; i < vectors_.size(); i++) {
output.writeMessage(2, vectors_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ids_.size(); i++) {
dataSize += computeStringSizeNoTag(ids_.getRaw(i));
}
size += dataSize;
size += 1 * getIdsList().size();
}
for (int i = 0; i < vectors_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, vectors_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.FetchRequest)) {
return super.equals(obj);
}
io.pinecone.proto.Core.FetchRequest other = (io.pinecone.proto.Core.FetchRequest) obj;
if (!getIdsList()
.equals(other.getIdsList())) return false;
if (!getVectorsList()
.equals(other.getVectorsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getIdsCount() > 0) {
hash = (37 * hash) + IDS_FIELD_NUMBER;
hash = (53 * hash) + getIdsList().hashCode();
}
if (getVectorsCount() > 0) {
hash = (37 * hash) + VECTORS_FIELD_NUMBER;
hash = (53 * hash) + getVectorsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.FetchRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.FetchRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.FetchRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.FetchRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Represents a fetch request
*
*
* Protobuf type {@code core.FetchRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.FetchRequest)
io.pinecone.proto.Core.FetchRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_FetchRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_FetchRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.FetchRequest.class, io.pinecone.proto.Core.FetchRequest.Builder.class);
}
// Construct using io.pinecone.proto.Core.FetchRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getVectorsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (vectorsBuilder_ == null) {
vectors_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
vectorsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_FetchRequest_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.FetchRequest getDefaultInstanceForType() {
return io.pinecone.proto.Core.FetchRequest.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.FetchRequest build() {
io.pinecone.proto.Core.FetchRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.FetchRequest buildPartial() {
io.pinecone.proto.Core.FetchRequest result = new io.pinecone.proto.Core.FetchRequest(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
ids_ = ids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ids_ = ids_;
if (vectorsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
vectors_ = java.util.Collections.unmodifiableList(vectors_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.vectors_ = vectors_;
} else {
result.vectors_ = vectorsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.FetchRequest) {
return mergeFrom((io.pinecone.proto.Core.FetchRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.FetchRequest other) {
if (other == io.pinecone.proto.Core.FetchRequest.getDefaultInstance()) return this;
if (!other.ids_.isEmpty()) {
if (ids_.isEmpty()) {
ids_ = other.ids_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIdsIsMutable();
ids_.addAll(other.ids_);
}
onChanged();
}
if (vectorsBuilder_ == null) {
if (!other.vectors_.isEmpty()) {
if (vectors_.isEmpty()) {
vectors_ = other.vectors_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureVectorsIsMutable();
vectors_.addAll(other.vectors_);
}
onChanged();
}
} else {
if (!other.vectors_.isEmpty()) {
if (vectorsBuilder_.isEmpty()) {
vectorsBuilder_.dispose();
vectorsBuilder_ = null;
vectors_ = other.vectors_;
bitField0_ = (bitField0_ & ~0x00000002);
vectorsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getVectorsFieldBuilder() : null;
} else {
vectorsBuilder_.addAllMessages(other.vectors_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.FetchRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.FetchRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
ids_ = new com.google.protobuf.LazyStringArrayList(ids_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return A list containing the ids.
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_.getUnmodifiableView();
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return The count of ids.
*/
public int getIdsCount() {
return ids_.size();
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index of the element to return.
* @return The ids at the given index.
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index of the value to return.
* @return The bytes of the ids at the given index.
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param index The index to set the value at.
* @param value The ids to set.
* @return This builder for chaining.
*/
public Builder setIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.set(index, value);
onChanged();
return this;
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param value The ids to add.
* @return This builder for chaining.
*/
public Builder addIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param values The ids to add.
* @return This builder for chaining.
*/
public Builder addAllIds(
java.lang.Iterable values) {
ensureIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ids_);
onChanged();
return this;
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @return This builder for chaining.
*/
public Builder clearIds() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Ids of vectors to fetch
*
*
* repeated string ids = 1;
* @param value The bytes of the ids to add.
* @return This builder for chaining.
*/
public Builder addIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
private java.util.List vectors_ =
java.util.Collections.emptyList();
private void ensureVectorsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
vectors_ = new java.util.ArrayList(vectors_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder> vectorsBuilder_;
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public java.util.List getVectorsList() {
if (vectorsBuilder_ == null) {
return java.util.Collections.unmodifiableList(vectors_);
} else {
return vectorsBuilder_.getMessageList();
}
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public int getVectorsCount() {
if (vectorsBuilder_ == null) {
return vectors_.size();
} else {
return vectorsBuilder_.getCount();
}
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public io.pinecone.proto.Core.NdArray getVectors(int index) {
if (vectorsBuilder_ == null) {
return vectors_.get(index);
} else {
return vectorsBuilder_.getMessage(index);
}
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder setVectors(
int index, io.pinecone.proto.Core.NdArray value) {
if (vectorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVectorsIsMutable();
vectors_.set(index, value);
onChanged();
} else {
vectorsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder setVectors(
int index, io.pinecone.proto.Core.NdArray.Builder builderForValue) {
if (vectorsBuilder_ == null) {
ensureVectorsIsMutable();
vectors_.set(index, builderForValue.build());
onChanged();
} else {
vectorsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder addVectors(io.pinecone.proto.Core.NdArray value) {
if (vectorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVectorsIsMutable();
vectors_.add(value);
onChanged();
} else {
vectorsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder addVectors(
int index, io.pinecone.proto.Core.NdArray value) {
if (vectorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVectorsIsMutable();
vectors_.add(index, value);
onChanged();
} else {
vectorsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder addVectors(
io.pinecone.proto.Core.NdArray.Builder builderForValue) {
if (vectorsBuilder_ == null) {
ensureVectorsIsMutable();
vectors_.add(builderForValue.build());
onChanged();
} else {
vectorsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder addVectors(
int index, io.pinecone.proto.Core.NdArray.Builder builderForValue) {
if (vectorsBuilder_ == null) {
ensureVectorsIsMutable();
vectors_.add(index, builderForValue.build());
onChanged();
} else {
vectorsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder addAllVectors(
java.lang.Iterable extends io.pinecone.proto.Core.NdArray> values) {
if (vectorsBuilder_ == null) {
ensureVectorsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, vectors_);
onChanged();
} else {
vectorsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder clearVectors() {
if (vectorsBuilder_ == null) {
vectors_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
vectorsBuilder_.clear();
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public Builder removeVectors(int index) {
if (vectorsBuilder_ == null) {
ensureVectorsIsMutable();
vectors_.remove(index);
onChanged();
} else {
vectorsBuilder_.remove(index);
}
return this;
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public io.pinecone.proto.Core.NdArray.Builder getVectorsBuilder(
int index) {
return getVectorsFieldBuilder().getBuilder(index);
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public io.pinecone.proto.Core.NdArrayOrBuilder getVectorsOrBuilder(
int index) {
if (vectorsBuilder_ == null) {
return vectors_.get(index); } else {
return vectorsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public java.util.List extends io.pinecone.proto.Core.NdArrayOrBuilder>
getVectorsOrBuilderList() {
if (vectorsBuilder_ != null) {
return vectorsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(vectors_);
}
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public io.pinecone.proto.Core.NdArray.Builder addVectorsBuilder() {
return getVectorsFieldBuilder().addBuilder(
io.pinecone.proto.Core.NdArray.getDefaultInstance());
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public io.pinecone.proto.Core.NdArray.Builder addVectorsBuilder(
int index) {
return getVectorsFieldBuilder().addBuilder(
index, io.pinecone.proto.Core.NdArray.getDefaultInstance());
}
/**
*
* Result vectors corresponding to ids
*
*
* repeated .core.NdArray vectors = 2;
*/
public java.util.List
getVectorsBuilderList() {
return getVectorsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>
getVectorsFieldBuilder() {
if (vectorsBuilder_ == null) {
vectorsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.NdArray, io.pinecone.proto.Core.NdArray.Builder, io.pinecone.proto.Core.NdArrayOrBuilder>(
vectors_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
vectors_ = null;
}
return vectorsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.FetchRequest)
}
// @@protoc_insertion_point(class_scope:core.FetchRequest)
private static final io.pinecone.proto.Core.FetchRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.FetchRequest();
}
public static io.pinecone.proto.Core.FetchRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FetchRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FetchRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.FetchRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AckOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.Ack)
com.google.protobuf.MessageOrBuilder {
/**
*
* Ordinal of replica the request comes from
*
*
* uint32 replica = 1;
* @return The replica.
*/
int getReplica();
/**
*
* Whether the replica is requesting a replay starting from acked offset
*
*
* bool replay = 2;
* @return The replay.
*/
boolean getReplay();
}
/**
*
**
* An acknowledgement from a replica to the leader that it has caught up to that point in the log.
*
*
* Protobuf type {@code core.Ack}
*/
public static final class Ack extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.Ack)
AckOrBuilder {
private static final long serialVersionUID = 0L;
// Use Ack.newBuilder() to construct.
private Ack(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Ack() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Ack();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Ack(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
replica_ = input.readUInt32();
break;
}
case 16: {
replay_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Ack_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Ack_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Ack.class, io.pinecone.proto.Core.Ack.Builder.class);
}
public static final int REPLICA_FIELD_NUMBER = 1;
private int replica_;
/**
*
* Ordinal of replica the request comes from
*
*
* uint32 replica = 1;
* @return The replica.
*/
@java.lang.Override
public int getReplica() {
return replica_;
}
public static final int REPLAY_FIELD_NUMBER = 2;
private boolean replay_;
/**
*
* Whether the replica is requesting a replay starting from acked offset
*
*
* bool replay = 2;
* @return The replay.
*/
@java.lang.Override
public boolean getReplay() {
return replay_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (replica_ != 0) {
output.writeUInt32(1, replica_);
}
if (replay_ != false) {
output.writeBool(2, replay_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (replica_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, replica_);
}
if (replay_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, replay_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.Ack)) {
return super.equals(obj);
}
io.pinecone.proto.Core.Ack other = (io.pinecone.proto.Core.Ack) obj;
if (getReplica()
!= other.getReplica()) return false;
if (getReplay()
!= other.getReplay()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REPLICA_FIELD_NUMBER;
hash = (53 * hash) + getReplica();
hash = (37 * hash) + REPLAY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReplay());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.Ack parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Ack parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Ack parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Ack parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Ack parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.Ack parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.Ack parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Ack parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Ack parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Ack parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.Ack parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.Ack parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.Ack prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* An acknowledgement from a replica to the leader that it has caught up to that point in the log.
*
*
* Protobuf type {@code core.Ack}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.Ack)
io.pinecone.proto.Core.AckOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_Ack_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_Ack_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.Ack.class, io.pinecone.proto.Core.Ack.Builder.class);
}
// Construct using io.pinecone.proto.Core.Ack.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
replica_ = 0;
replay_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_Ack_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.Ack getDefaultInstanceForType() {
return io.pinecone.proto.Core.Ack.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.Ack build() {
io.pinecone.proto.Core.Ack result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.Ack buildPartial() {
io.pinecone.proto.Core.Ack result = new io.pinecone.proto.Core.Ack(this);
result.replica_ = replica_;
result.replay_ = replay_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.Ack) {
return mergeFrom((io.pinecone.proto.Core.Ack)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.Ack other) {
if (other == io.pinecone.proto.Core.Ack.getDefaultInstance()) return this;
if (other.getReplica() != 0) {
setReplica(other.getReplica());
}
if (other.getReplay() != false) {
setReplay(other.getReplay());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.Ack parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.Ack) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int replica_ ;
/**
*
* Ordinal of replica the request comes from
*
*
* uint32 replica = 1;
* @return The replica.
*/
@java.lang.Override
public int getReplica() {
return replica_;
}
/**
*
* Ordinal of replica the request comes from
*
*
* uint32 replica = 1;
* @param value The replica to set.
* @return This builder for chaining.
*/
public Builder setReplica(int value) {
replica_ = value;
onChanged();
return this;
}
/**
*
* Ordinal of replica the request comes from
*
*
* uint32 replica = 1;
* @return This builder for chaining.
*/
public Builder clearReplica() {
replica_ = 0;
onChanged();
return this;
}
private boolean replay_ ;
/**
*
* Whether the replica is requesting a replay starting from acked offset
*
*
* bool replay = 2;
* @return The replay.
*/
@java.lang.Override
public boolean getReplay() {
return replay_;
}
/**
*
* Whether the replica is requesting a replay starting from acked offset
*
*
* bool replay = 2;
* @param value The replay to set.
* @return This builder for chaining.
*/
public Builder setReplay(boolean value) {
replay_ = value;
onChanged();
return this;
}
/**
*
* Whether the replica is requesting a replay starting from acked offset
*
*
* bool replay = 2;
* @return This builder for chaining.
*/
public Builder clearReplay() {
replay_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.Ack)
}
// @@protoc_insertion_point(class_scope:core.Ack)
private static final io.pinecone.proto.Core.Ack DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.Ack();
}
public static io.pinecone.proto.Core.Ack getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Ack parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Ack(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.Ack getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.LogEntry)
com.google.protobuf.MessageOrBuilder {
/**
*
* WAL offset of this entry
*
*
* uint64 offset = 1;
* @return The offset.
*/
long getOffset();
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
* @return Whether the entry field is set.
*/
boolean hasEntry();
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
* @return The entry.
*/
io.pinecone.proto.Core.Request getEntry();
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
io.pinecone.proto.Core.RequestOrBuilder getEntryOrBuilder();
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
* @return Whether the ack field is set.
*/
boolean hasAck();
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
* @return The ack.
*/
io.pinecone.proto.Core.Ack getAck();
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
io.pinecone.proto.Core.AckOrBuilder getAckOrBuilder();
public io.pinecone.proto.Core.LogEntry.DataCase getDataCase();
}
/**
* Protobuf type {@code core.LogEntry}
*/
public static final class LogEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.LogEntry)
LogEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogEntry.newBuilder() to construct.
private LogEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogEntry() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LogEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
offset_ = input.readUInt64();
break;
}
case 18: {
io.pinecone.proto.Core.Request.Builder subBuilder = null;
if (dataCase_ == 2) {
subBuilder = ((io.pinecone.proto.Core.Request) data_).toBuilder();
}
data_ =
input.readMessage(io.pinecone.proto.Core.Request.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.Request) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 2;
break;
}
case 26: {
io.pinecone.proto.Core.Ack.Builder subBuilder = null;
if (dataCase_ == 3) {
subBuilder = ((io.pinecone.proto.Core.Ack) data_).toBuilder();
}
data_ =
input.readMessage(io.pinecone.proto.Core.Ack.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((io.pinecone.proto.Core.Ack) data_);
data_ = subBuilder.buildPartial();
}
dataCase_ = 3;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_LogEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_LogEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.LogEntry.class, io.pinecone.proto.Core.LogEntry.Builder.class);
}
private int dataCase_ = 0;
private java.lang.Object data_;
public enum DataCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
ENTRY(2),
ACK(3),
DATA_NOT_SET(0);
private final int value;
private DataCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DataCase valueOf(int value) {
return forNumber(value);
}
public static DataCase forNumber(int value) {
switch (value) {
case 2: return ENTRY;
case 3: return ACK;
case 0: return DATA_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
public static final int OFFSET_FIELD_NUMBER = 1;
private long offset_;
/**
*
* WAL offset of this entry
*
*
* uint64 offset = 1;
* @return The offset.
*/
@java.lang.Override
public long getOffset() {
return offset_;
}
public static final int ENTRY_FIELD_NUMBER = 2;
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
* @return Whether the entry field is set.
*/
@java.lang.Override
public boolean hasEntry() {
return dataCase_ == 2;
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
* @return The entry.
*/
@java.lang.Override
public io.pinecone.proto.Core.Request getEntry() {
if (dataCase_ == 2) {
return (io.pinecone.proto.Core.Request) data_;
}
return io.pinecone.proto.Core.Request.getDefaultInstance();
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
@java.lang.Override
public io.pinecone.proto.Core.RequestOrBuilder getEntryOrBuilder() {
if (dataCase_ == 2) {
return (io.pinecone.proto.Core.Request) data_;
}
return io.pinecone.proto.Core.Request.getDefaultInstance();
}
public static final int ACK_FIELD_NUMBER = 3;
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
* @return Whether the ack field is set.
*/
@java.lang.Override
public boolean hasAck() {
return dataCase_ == 3;
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
* @return The ack.
*/
@java.lang.Override
public io.pinecone.proto.Core.Ack getAck() {
if (dataCase_ == 3) {
return (io.pinecone.proto.Core.Ack) data_;
}
return io.pinecone.proto.Core.Ack.getDefaultInstance();
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.AckOrBuilder getAckOrBuilder() {
if (dataCase_ == 3) {
return (io.pinecone.proto.Core.Ack) data_;
}
return io.pinecone.proto.Core.Ack.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (offset_ != 0L) {
output.writeUInt64(1, offset_);
}
if (dataCase_ == 2) {
output.writeMessage(2, (io.pinecone.proto.Core.Request) data_);
}
if (dataCase_ == 3) {
output.writeMessage(3, (io.pinecone.proto.Core.Ack) data_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (offset_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, offset_);
}
if (dataCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (io.pinecone.proto.Core.Request) data_);
}
if (dataCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (io.pinecone.proto.Core.Ack) data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.LogEntry)) {
return super.equals(obj);
}
io.pinecone.proto.Core.LogEntry other = (io.pinecone.proto.Core.LogEntry) obj;
if (getOffset()
!= other.getOffset()) return false;
if (!getDataCase().equals(other.getDataCase())) return false;
switch (dataCase_) {
case 2:
if (!getEntry()
.equals(other.getEntry())) return false;
break;
case 3:
if (!getAck()
.equals(other.getAck())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOffset());
switch (dataCase_) {
case 2:
hash = (37 * hash) + ENTRY_FIELD_NUMBER;
hash = (53 * hash) + getEntry().hashCode();
break;
case 3:
hash = (37 * hash) + ACK_FIELD_NUMBER;
hash = (53 * hash) + getAck().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.LogEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.LogEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.LogEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.LogEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code core.LogEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.LogEntry)
io.pinecone.proto.Core.LogEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_LogEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_LogEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.LogEntry.class, io.pinecone.proto.Core.LogEntry.Builder.class);
}
// Construct using io.pinecone.proto.Core.LogEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
offset_ = 0L;
dataCase_ = 0;
data_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_LogEntry_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.LogEntry getDefaultInstanceForType() {
return io.pinecone.proto.Core.LogEntry.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.LogEntry build() {
io.pinecone.proto.Core.LogEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.LogEntry buildPartial() {
io.pinecone.proto.Core.LogEntry result = new io.pinecone.proto.Core.LogEntry(this);
result.offset_ = offset_;
if (dataCase_ == 2) {
if (entryBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = entryBuilder_.build();
}
}
if (dataCase_ == 3) {
if (ackBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = ackBuilder_.build();
}
}
result.dataCase_ = dataCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.LogEntry) {
return mergeFrom((io.pinecone.proto.Core.LogEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.LogEntry other) {
if (other == io.pinecone.proto.Core.LogEntry.getDefaultInstance()) return this;
if (other.getOffset() != 0L) {
setOffset(other.getOffset());
}
switch (other.getDataCase()) {
case ENTRY: {
mergeEntry(other.getEntry());
break;
}
case ACK: {
mergeAck(other.getAck());
break;
}
case DATA_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.LogEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.LogEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int dataCase_ = 0;
private java.lang.Object data_;
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
public Builder clearData() {
dataCase_ = 0;
data_ = null;
onChanged();
return this;
}
private long offset_ ;
/**
*
* WAL offset of this entry
*
*
* uint64 offset = 1;
* @return The offset.
*/
@java.lang.Override
public long getOffset() {
return offset_;
}
/**
*
* WAL offset of this entry
*
*
* uint64 offset = 1;
* @param value The offset to set.
* @return This builder for chaining.
*/
public Builder setOffset(long value) {
offset_ = value;
onChanged();
return this;
}
/**
*
* WAL offset of this entry
*
*
* uint64 offset = 1;
* @return This builder for chaining.
*/
public Builder clearOffset() {
offset_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Request, io.pinecone.proto.Core.Request.Builder, io.pinecone.proto.Core.RequestOrBuilder> entryBuilder_;
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
* @return Whether the entry field is set.
*/
@java.lang.Override
public boolean hasEntry() {
return dataCase_ == 2;
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
* @return The entry.
*/
@java.lang.Override
public io.pinecone.proto.Core.Request getEntry() {
if (entryBuilder_ == null) {
if (dataCase_ == 2) {
return (io.pinecone.proto.Core.Request) data_;
}
return io.pinecone.proto.Core.Request.getDefaultInstance();
} else {
if (dataCase_ == 2) {
return entryBuilder_.getMessage();
}
return io.pinecone.proto.Core.Request.getDefaultInstance();
}
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
public Builder setEntry(io.pinecone.proto.Core.Request value) {
if (entryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
entryBuilder_.setMessage(value);
}
dataCase_ = 2;
return this;
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
public Builder setEntry(
io.pinecone.proto.Core.Request.Builder builderForValue) {
if (entryBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
entryBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 2;
return this;
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
public Builder mergeEntry(io.pinecone.proto.Core.Request value) {
if (entryBuilder_ == null) {
if (dataCase_ == 2 &&
data_ != io.pinecone.proto.Core.Request.getDefaultInstance()) {
data_ = io.pinecone.proto.Core.Request.newBuilder((io.pinecone.proto.Core.Request) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 2) {
entryBuilder_.mergeFrom(value);
}
entryBuilder_.setMessage(value);
}
dataCase_ = 2;
return this;
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
public Builder clearEntry() {
if (entryBuilder_ == null) {
if (dataCase_ == 2) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 2) {
dataCase_ = 0;
data_ = null;
}
entryBuilder_.clear();
}
return this;
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
public io.pinecone.proto.Core.Request.Builder getEntryBuilder() {
return getEntryFieldBuilder().getBuilder();
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
@java.lang.Override
public io.pinecone.proto.Core.RequestOrBuilder getEntryOrBuilder() {
if ((dataCase_ == 2) && (entryBuilder_ != null)) {
return entryBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 2) {
return (io.pinecone.proto.Core.Request) data_;
}
return io.pinecone.proto.Core.Request.getDefaultInstance();
}
}
/**
*
* The request itself as it was received
*
*
* .core.Request entry = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Request, io.pinecone.proto.Core.Request.Builder, io.pinecone.proto.Core.RequestOrBuilder>
getEntryFieldBuilder() {
if (entryBuilder_ == null) {
if (!(dataCase_ == 2)) {
data_ = io.pinecone.proto.Core.Request.getDefaultInstance();
}
entryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Request, io.pinecone.proto.Core.Request.Builder, io.pinecone.proto.Core.RequestOrBuilder>(
(io.pinecone.proto.Core.Request) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 2;
onChanged();;
return entryBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Ack, io.pinecone.proto.Core.Ack.Builder, io.pinecone.proto.Core.AckOrBuilder> ackBuilder_;
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
* @return Whether the ack field is set.
*/
@java.lang.Override
public boolean hasAck() {
return dataCase_ == 3;
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
* @return The ack.
*/
@java.lang.Override
public io.pinecone.proto.Core.Ack getAck() {
if (ackBuilder_ == null) {
if (dataCase_ == 3) {
return (io.pinecone.proto.Core.Ack) data_;
}
return io.pinecone.proto.Core.Ack.getDefaultInstance();
} else {
if (dataCase_ == 3) {
return ackBuilder_.getMessage();
}
return io.pinecone.proto.Core.Ack.getDefaultInstance();
}
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
public Builder setAck(io.pinecone.proto.Core.Ack value) {
if (ackBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
ackBuilder_.setMessage(value);
}
dataCase_ = 3;
return this;
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
public Builder setAck(
io.pinecone.proto.Core.Ack.Builder builderForValue) {
if (ackBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
ackBuilder_.setMessage(builderForValue.build());
}
dataCase_ = 3;
return this;
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
public Builder mergeAck(io.pinecone.proto.Core.Ack value) {
if (ackBuilder_ == null) {
if (dataCase_ == 3 &&
data_ != io.pinecone.proto.Core.Ack.getDefaultInstance()) {
data_ = io.pinecone.proto.Core.Ack.newBuilder((io.pinecone.proto.Core.Ack) data_)
.mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
if (dataCase_ == 3) {
ackBuilder_.mergeFrom(value);
}
ackBuilder_.setMessage(value);
}
dataCase_ = 3;
return this;
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
public Builder clearAck() {
if (ackBuilder_ == null) {
if (dataCase_ == 3) {
dataCase_ = 0;
data_ = null;
onChanged();
}
} else {
if (dataCase_ == 3) {
dataCase_ = 0;
data_ = null;
}
ackBuilder_.clear();
}
return this;
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
public io.pinecone.proto.Core.Ack.Builder getAckBuilder() {
return getAckFieldBuilder().getBuilder();
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
@java.lang.Override
public io.pinecone.proto.Core.AckOrBuilder getAckOrBuilder() {
if ((dataCase_ == 3) && (ackBuilder_ != null)) {
return ackBuilder_.getMessageOrBuilder();
} else {
if (dataCase_ == 3) {
return (io.pinecone.proto.Core.Ack) data_;
}
return io.pinecone.proto.Core.Ack.getDefaultInstance();
}
}
/**
*
* Or an acknowledgement from a follower of receiving this entry
*
*
* .core.Ack ack = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Ack, io.pinecone.proto.Core.Ack.Builder, io.pinecone.proto.Core.AckOrBuilder>
getAckFieldBuilder() {
if (ackBuilder_ == null) {
if (!(dataCase_ == 3)) {
data_ = io.pinecone.proto.Core.Ack.getDefaultInstance();
}
ackBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.pinecone.proto.Core.Ack, io.pinecone.proto.Core.Ack.Builder, io.pinecone.proto.Core.AckOrBuilder>(
(io.pinecone.proto.Core.Ack) data_,
getParentForChildren(),
isClean());
data_ = null;
}
dataCase_ = 3;
onChanged();;
return ackBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.LogEntry)
}
// @@protoc_insertion_point(class_scope:core.LogEntry)
private static final io.pinecone.proto.Core.LogEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.LogEntry();
}
public static io.pinecone.proto.Core.LogEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LogEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.LogEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TraceRouteOrBuilder extends
// @@protoc_insertion_point(interface_extends:core.TraceRoute)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 request_id = 1;
* @return The requestId.
*/
long getRequestId();
/**
* uint32 client_id = 2;
* @return The clientId.
*/
int getClientId();
/**
* uint32 client_offset = 3;
* @return The clientOffset.
*/
int getClientOffset();
/**
* repeated .core.Route routes = 4;
*/
java.util.List
getRoutesList();
/**
* repeated .core.Route routes = 4;
*/
io.pinecone.proto.Core.Route getRoutes(int index);
/**
* repeated .core.Route routes = 4;
*/
int getRoutesCount();
/**
* repeated .core.Route routes = 4;
*/
java.util.List extends io.pinecone.proto.Core.RouteOrBuilder>
getRoutesOrBuilderList();
/**
* repeated .core.Route routes = 4;
*/
io.pinecone.proto.Core.RouteOrBuilder getRoutesOrBuilder(
int index);
}
/**
*
**
* Receipts sent back to gateway from each function if "traceroute" flag is set
*
*
* Protobuf type {@code core.TraceRoute}
*/
public static final class TraceRoute extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:core.TraceRoute)
TraceRouteOrBuilder {
private static final long serialVersionUID = 0L;
// Use TraceRoute.newBuilder() to construct.
private TraceRoute(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TraceRoute() {
routes_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TraceRoute();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TraceRoute(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
requestId_ = input.readUInt64();
break;
}
case 16: {
clientId_ = input.readUInt32();
break;
}
case 24: {
clientOffset_ = input.readUInt32();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
routes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
routes_.add(
input.readMessage(io.pinecone.proto.Core.Route.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
routes_ = java.util.Collections.unmodifiableList(routes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_TraceRoute_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_TraceRoute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.TraceRoute.class, io.pinecone.proto.Core.TraceRoute.Builder.class);
}
public static final int REQUEST_ID_FIELD_NUMBER = 1;
private long requestId_;
/**
* uint64 request_id = 1;
* @return The requestId.
*/
@java.lang.Override
public long getRequestId() {
return requestId_;
}
public static final int CLIENT_ID_FIELD_NUMBER = 2;
private int clientId_;
/**
* uint32 client_id = 2;
* @return The clientId.
*/
@java.lang.Override
public int getClientId() {
return clientId_;
}
public static final int CLIENT_OFFSET_FIELD_NUMBER = 3;
private int clientOffset_;
/**
* uint32 client_offset = 3;
* @return The clientOffset.
*/
@java.lang.Override
public int getClientOffset() {
return clientOffset_;
}
public static final int ROUTES_FIELD_NUMBER = 4;
private java.util.List routes_;
/**
* repeated .core.Route routes = 4;
*/
@java.lang.Override
public java.util.List getRoutesList() {
return routes_;
}
/**
* repeated .core.Route routes = 4;
*/
@java.lang.Override
public java.util.List extends io.pinecone.proto.Core.RouteOrBuilder>
getRoutesOrBuilderList() {
return routes_;
}
/**
* repeated .core.Route routes = 4;
*/
@java.lang.Override
public int getRoutesCount() {
return routes_.size();
}
/**
* repeated .core.Route routes = 4;
*/
@java.lang.Override
public io.pinecone.proto.Core.Route getRoutes(int index) {
return routes_.get(index);
}
/**
* repeated .core.Route routes = 4;
*/
@java.lang.Override
public io.pinecone.proto.Core.RouteOrBuilder getRoutesOrBuilder(
int index) {
return routes_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (requestId_ != 0L) {
output.writeUInt64(1, requestId_);
}
if (clientId_ != 0) {
output.writeUInt32(2, clientId_);
}
if (clientOffset_ != 0) {
output.writeUInt32(3, clientOffset_);
}
for (int i = 0; i < routes_.size(); i++) {
output.writeMessage(4, routes_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (requestId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, requestId_);
}
if (clientId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, clientId_);
}
if (clientOffset_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, clientOffset_);
}
for (int i = 0; i < routes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, routes_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.pinecone.proto.Core.TraceRoute)) {
return super.equals(obj);
}
io.pinecone.proto.Core.TraceRoute other = (io.pinecone.proto.Core.TraceRoute) obj;
if (getRequestId()
!= other.getRequestId()) return false;
if (getClientId()
!= other.getClientId()) return false;
if (getClientOffset()
!= other.getClientOffset()) return false;
if (!getRoutesList()
.equals(other.getRoutesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRequestId());
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId();
hash = (37 * hash) + CLIENT_OFFSET_FIELD_NUMBER;
hash = (53 * hash) + getClientOffset();
if (getRoutesCount() > 0) {
hash = (37 * hash) + ROUTES_FIELD_NUMBER;
hash = (53 * hash) + getRoutesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.TraceRoute parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.TraceRoute parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.pinecone.proto.Core.TraceRoute parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.pinecone.proto.Core.TraceRoute prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Receipts sent back to gateway from each function if "traceroute" flag is set
*
*
* Protobuf type {@code core.TraceRoute}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:core.TraceRoute)
io.pinecone.proto.Core.TraceRouteOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.pinecone.proto.Core.internal_static_core_TraceRoute_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.pinecone.proto.Core.internal_static_core_TraceRoute_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.pinecone.proto.Core.TraceRoute.class, io.pinecone.proto.Core.TraceRoute.Builder.class);
}
// Construct using io.pinecone.proto.Core.TraceRoute.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRoutesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
requestId_ = 0L;
clientId_ = 0;
clientOffset_ = 0;
if (routesBuilder_ == null) {
routes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
routesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.pinecone.proto.Core.internal_static_core_TraceRoute_descriptor;
}
@java.lang.Override
public io.pinecone.proto.Core.TraceRoute getDefaultInstanceForType() {
return io.pinecone.proto.Core.TraceRoute.getDefaultInstance();
}
@java.lang.Override
public io.pinecone.proto.Core.TraceRoute build() {
io.pinecone.proto.Core.TraceRoute result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.pinecone.proto.Core.TraceRoute buildPartial() {
io.pinecone.proto.Core.TraceRoute result = new io.pinecone.proto.Core.TraceRoute(this);
int from_bitField0_ = bitField0_;
result.requestId_ = requestId_;
result.clientId_ = clientId_;
result.clientOffset_ = clientOffset_;
if (routesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
routes_ = java.util.Collections.unmodifiableList(routes_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.routes_ = routes_;
} else {
result.routes_ = routesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.pinecone.proto.Core.TraceRoute) {
return mergeFrom((io.pinecone.proto.Core.TraceRoute)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.pinecone.proto.Core.TraceRoute other) {
if (other == io.pinecone.proto.Core.TraceRoute.getDefaultInstance()) return this;
if (other.getRequestId() != 0L) {
setRequestId(other.getRequestId());
}
if (other.getClientId() != 0) {
setClientId(other.getClientId());
}
if (other.getClientOffset() != 0) {
setClientOffset(other.getClientOffset());
}
if (routesBuilder_ == null) {
if (!other.routes_.isEmpty()) {
if (routes_.isEmpty()) {
routes_ = other.routes_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRoutesIsMutable();
routes_.addAll(other.routes_);
}
onChanged();
}
} else {
if (!other.routes_.isEmpty()) {
if (routesBuilder_.isEmpty()) {
routesBuilder_.dispose();
routesBuilder_ = null;
routes_ = other.routes_;
bitField0_ = (bitField0_ & ~0x00000001);
routesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRoutesFieldBuilder() : null;
} else {
routesBuilder_.addAllMessages(other.routes_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
io.pinecone.proto.Core.TraceRoute parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (io.pinecone.proto.Core.TraceRoute) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long requestId_ ;
/**
* uint64 request_id = 1;
* @return The requestId.
*/
@java.lang.Override
public long getRequestId() {
return requestId_;
}
/**
* uint64 request_id = 1;
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(long value) {
requestId_ = value;
onChanged();
return this;
}
/**
* uint64 request_id = 1;
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = 0L;
onChanged();
return this;
}
private int clientId_ ;
/**
* uint32 client_id = 2;
* @return The clientId.
*/
@java.lang.Override
public int getClientId() {
return clientId_;
}
/**
* uint32 client_id = 2;
* @param value The clientId to set.
* @return This builder for chaining.
*/
public Builder setClientId(int value) {
clientId_ = value;
onChanged();
return this;
}
/**
* uint32 client_id = 2;
* @return This builder for chaining.
*/
public Builder clearClientId() {
clientId_ = 0;
onChanged();
return this;
}
private int clientOffset_ ;
/**
* uint32 client_offset = 3;
* @return The clientOffset.
*/
@java.lang.Override
public int getClientOffset() {
return clientOffset_;
}
/**
* uint32 client_offset = 3;
* @param value The clientOffset to set.
* @return This builder for chaining.
*/
public Builder setClientOffset(int value) {
clientOffset_ = value;
onChanged();
return this;
}
/**
* uint32 client_offset = 3;
* @return This builder for chaining.
*/
public Builder clearClientOffset() {
clientOffset_ = 0;
onChanged();
return this;
}
private java.util.List routes_ =
java.util.Collections.emptyList();
private void ensureRoutesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
routes_ = new java.util.ArrayList(routes_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Route, io.pinecone.proto.Core.Route.Builder, io.pinecone.proto.Core.RouteOrBuilder> routesBuilder_;
/**
* repeated .core.Route routes = 4;
*/
public java.util.List getRoutesList() {
if (routesBuilder_ == null) {
return java.util.Collections.unmodifiableList(routes_);
} else {
return routesBuilder_.getMessageList();
}
}
/**
* repeated .core.Route routes = 4;
*/
public int getRoutesCount() {
if (routesBuilder_ == null) {
return routes_.size();
} else {
return routesBuilder_.getCount();
}
}
/**
* repeated .core.Route routes = 4;
*/
public io.pinecone.proto.Core.Route getRoutes(int index) {
if (routesBuilder_ == null) {
return routes_.get(index);
} else {
return routesBuilder_.getMessage(index);
}
}
/**
* repeated .core.Route routes = 4;
*/
public Builder setRoutes(
int index, io.pinecone.proto.Core.Route value) {
if (routesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRoutesIsMutable();
routes_.set(index, value);
onChanged();
} else {
routesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder setRoutes(
int index, io.pinecone.proto.Core.Route.Builder builderForValue) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.set(index, builderForValue.build());
onChanged();
} else {
routesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder addRoutes(io.pinecone.proto.Core.Route value) {
if (routesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRoutesIsMutable();
routes_.add(value);
onChanged();
} else {
routesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder addRoutes(
int index, io.pinecone.proto.Core.Route value) {
if (routesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRoutesIsMutable();
routes_.add(index, value);
onChanged();
} else {
routesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder addRoutes(
io.pinecone.proto.Core.Route.Builder builderForValue) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.add(builderForValue.build());
onChanged();
} else {
routesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder addRoutes(
int index, io.pinecone.proto.Core.Route.Builder builderForValue) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.add(index, builderForValue.build());
onChanged();
} else {
routesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder addAllRoutes(
java.lang.Iterable extends io.pinecone.proto.Core.Route> values) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, routes_);
onChanged();
} else {
routesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder clearRoutes() {
if (routesBuilder_ == null) {
routes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
routesBuilder_.clear();
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public Builder removeRoutes(int index) {
if (routesBuilder_ == null) {
ensureRoutesIsMutable();
routes_.remove(index);
onChanged();
} else {
routesBuilder_.remove(index);
}
return this;
}
/**
* repeated .core.Route routes = 4;
*/
public io.pinecone.proto.Core.Route.Builder getRoutesBuilder(
int index) {
return getRoutesFieldBuilder().getBuilder(index);
}
/**
* repeated .core.Route routes = 4;
*/
public io.pinecone.proto.Core.RouteOrBuilder getRoutesOrBuilder(
int index) {
if (routesBuilder_ == null) {
return routes_.get(index); } else {
return routesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .core.Route routes = 4;
*/
public java.util.List extends io.pinecone.proto.Core.RouteOrBuilder>
getRoutesOrBuilderList() {
if (routesBuilder_ != null) {
return routesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(routes_);
}
}
/**
* repeated .core.Route routes = 4;
*/
public io.pinecone.proto.Core.Route.Builder addRoutesBuilder() {
return getRoutesFieldBuilder().addBuilder(
io.pinecone.proto.Core.Route.getDefaultInstance());
}
/**
* repeated .core.Route routes = 4;
*/
public io.pinecone.proto.Core.Route.Builder addRoutesBuilder(
int index) {
return getRoutesFieldBuilder().addBuilder(
index, io.pinecone.proto.Core.Route.getDefaultInstance());
}
/**
* repeated .core.Route routes = 4;
*/
public java.util.List
getRoutesBuilderList() {
return getRoutesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Route, io.pinecone.proto.Core.Route.Builder, io.pinecone.proto.Core.RouteOrBuilder>
getRoutesFieldBuilder() {
if (routesBuilder_ == null) {
routesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.pinecone.proto.Core.Route, io.pinecone.proto.Core.Route.Builder, io.pinecone.proto.Core.RouteOrBuilder>(
routes_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
routes_ = null;
}
return routesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:core.TraceRoute)
}
// @@protoc_insertion_point(class_scope:core.TraceRoute)
private static final io.pinecone.proto.Core.TraceRoute DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.pinecone.proto.Core.TraceRoute();
}
public static io.pinecone.proto.Core.TraceRoute getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TraceRoute parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TraceRoute(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.pinecone.proto.Core.TraceRoute getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_ServiceControlRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_ServiceControlRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_NdArray_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_NdArray_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_Route_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_Route_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_Request_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_Request_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_Status_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_Status_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_Status_Details_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_Status_Details_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_Status_AvgTimeEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_Status_AvgTimeEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_ScoredResults_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_ScoredResults_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_IndexRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_IndexRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_QueryRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_QueryRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_DeleteRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_DeleteRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_InfoRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_InfoRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_FetchRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_FetchRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_Ack_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_Ack_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_LogEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_LogEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_core_TraceRoute_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_core_TraceRoute_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\ncore.proto\022\004core\032\037google/protobuf/time" +
"stamp.proto\"m\n\025ServiceControlRequest\022\020\n\010" +
"function\030\001 \001(\t\022\023\n\013function_id\030\002 \001(\004\022\034\n\006s" +
"tatus\030\003 \001(\0132\014.core.Status\022\017\n\007service\030\004 \001" +
"(\t\"K\n\007NdArray\022\016\n\006buffer\030\001 \001(\014\022\r\n\005shape\030\002" +
" \003(\r\022\r\n\005dtype\030\003 \001(\t\022\022\n\ncompressed\030\004 \001(\010\"" +
"\214\001\n\005Route\022\020\n\010function\030\001 \001(\t\022\023\n\013function_" +
"id\030\002 \001(\005\022.\n\nstart_time\030\003 \001(\0132\032.google.pr" +
"otobuf.Timestamp\022,\n\010end_time\030\004 \001(\0132\032.goo" +
"gle.protobuf.Timestamp\"\221\004\n\007Request\022\022\n\nre" +
"quest_id\030\001 \001(\004\022\017\n\007timeout\030\002 \001(\r\022\014\n\004path\030" +
"\003 \001(\t\022\017\n\007version\030\004 \001(\t\022\033\n\006routes\030\005 \003(\0132\013" +
".core.Route\022\034\n\006status\030\006 \001(\0132\014.core.Statu" +
"s\022#\n\005query\030\007 \001(\0132\022.core.QueryRequestH\000\022#" +
"\n\005index\030\010 \001(\0132\022.core.IndexRequestH\000\022%\n\006d" +
"elete\030\t \001(\0132\023.core.DeleteRequestH\000\022!\n\004in" +
"fo\030\n \001(\0132\021.core.InfoRequestH\000\022#\n\005fetch\030\013" +
" \001(\0132\022.core.FetchRequestH\000\022\021\n\tnamespace\030" +
"\014 \001(\t\022\021\n\tclient_id\030\r \001(\r\022\025\n\rclient_offse" +
"t\030\016 \001(\r\022\021\n\tshard_num\030\017 \001(\r\022\023\n\013gateway_nu" +
"m\030\020 \001(\r\022\032\n\022telemetry_trace_id\030\021 \001(\004\022\033\n\023t" +
"elemetry_parent_id\030\022 \001(\004\022\024\n\014service_name" +
"\030\023 \001(\t\022\022\n\ntraceroute\030\024 \001(\010B\006\n\004body\"\303\003\n\006S" +
"tatus\022%\n\004code\030\001 \001(\0162\027.core.Status.Status" +
"Code\022\023\n\013description\030\002 \001(\t\022%\n\007details\030\003 \003" +
"(\0132\024.core.Status.Details\022\020\n\010msg_sent\030\004 \001" +
"(\004\022\020\n\010msg_recv\030\005 \001(\004\022+\n\010avg_time\030\006 \003(\0132\031" +
".core.Status.AvgTimeEntry\022\014\n\004size\030\007 \001(\004\032" +
"\200\001\n\007Details\022\020\n\010function\030\001 \001(\t\022\023\n\013functio" +
"n_id\030\002 \001(\t\022\021\n\texception\030\003 \001(\t\022\021\n\ttraceba" +
"ck\030\004 \001(\t\022(\n\004time\030\005 \001(\0132\032.google.protobuf" +
".Timestamp\032.\n\014AvgTimeEntry\022\013\n\003key\030\001 \001(\t\022" +
"\r\n\005value\030\002 \001(\r:\0028\001\"D\n\nStatusCode\022\013\n\007SUCC" +
"ESS\020\000\022\t\n\005READY\020\001\022\t\n\005ERROR\020\002\022\023\n\017ERROR_DUP" +
"LICATE\020\003\"I\n\rScoredResults\022\013\n\003ids\030\001 \003(\t\022\016" +
"\n\006scores\030\002 \003(\002\022\033\n\004data\030\003 \001(\0132\r.core.NdAr" +
"ray\"8\n\014IndexRequest\022\013\n\003ids\030\001 \003(\t\022\033\n\004data" +
"\030\002 \001(\0132\r.core.NdArray\"v\n\014QueryRequest\022\r\n" +
"\005top_k\030\001 \001(\r\022\024\n\014include_data\030\002 \001(\010\022\033\n\004da" +
"ta\030\003 \001(\0132\r.core.NdArray\022$\n\007matches\030\004 \003(\013" +
"2\023.core.ScoredResults\"\034\n\rDeleteRequest\022\013" +
"\n\003ids\030\001 \003(\t\"!\n\013InfoRequest\022\022\n\nindex_size" +
"\030\001 \001(\004\";\n\014FetchRequest\022\013\n\003ids\030\001 \003(\t\022\036\n\007v" +
"ectors\030\002 \003(\0132\r.core.NdArray\"&\n\003Ack\022\017\n\007re" +
"plica\030\001 \001(\r\022\016\n\006replay\030\002 \001(\010\"\\\n\010LogEntry\022" +
"\016\n\006offset\030\001 \001(\004\022\036\n\005entry\030\002 \001(\0132\r.core.Re" +
"questH\000\022\030\n\003ack\030\003 \001(\0132\t.core.AckH\000B\006\n\004dat" +
"a\"g\n\nTraceRoute\022\022\n\nrequest_id\030\001 \001(\004\022\021\n\tc" +
"lient_id\030\002 \001(\r\022\025\n\rclient_offset\030\003 \001(\r\022\033\n" +
"\006routes\030\004 \003(\0132\013.core.Route2d\n\tRPCClient\022" +
"*\n\004Call\022\r.core.Request\032\r.core.Request\"\000(" +
"\0010\001\022+\n\tCallUnary\022\r.core.Request\032\r.core.R" +
"equest\"\000B\023\n\021io.pinecone.protob\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.TimestampProto.getDescriptor(),
});
internal_static_core_ServiceControlRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_core_ServiceControlRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_ServiceControlRequest_descriptor,
new java.lang.String[] { "Function", "FunctionId", "Status", "Service", });
internal_static_core_NdArray_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_core_NdArray_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_NdArray_descriptor,
new java.lang.String[] { "Buffer", "Shape", "Dtype", "Compressed", });
internal_static_core_Route_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_core_Route_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_Route_descriptor,
new java.lang.String[] { "Function", "FunctionId", "StartTime", "EndTime", });
internal_static_core_Request_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_core_Request_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_Request_descriptor,
new java.lang.String[] { "RequestId", "Timeout", "Path", "Version", "Routes", "Status", "Query", "Index", "Delete", "Info", "Fetch", "Namespace", "ClientId", "ClientOffset", "ShardNum", "GatewayNum", "TelemetryTraceId", "TelemetryParentId", "ServiceName", "Traceroute", "Body", });
internal_static_core_Status_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_core_Status_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_Status_descriptor,
new java.lang.String[] { "Code", "Description", "Details", "MsgSent", "MsgRecv", "AvgTime", "Size", });
internal_static_core_Status_Details_descriptor =
internal_static_core_Status_descriptor.getNestedTypes().get(0);
internal_static_core_Status_Details_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_Status_Details_descriptor,
new java.lang.String[] { "Function", "FunctionId", "Exception", "Traceback", "Time", });
internal_static_core_Status_AvgTimeEntry_descriptor =
internal_static_core_Status_descriptor.getNestedTypes().get(1);
internal_static_core_Status_AvgTimeEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_Status_AvgTimeEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_core_ScoredResults_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_core_ScoredResults_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_ScoredResults_descriptor,
new java.lang.String[] { "Ids", "Scores", "Data", });
internal_static_core_IndexRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_core_IndexRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_IndexRequest_descriptor,
new java.lang.String[] { "Ids", "Data", });
internal_static_core_QueryRequest_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_core_QueryRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_QueryRequest_descriptor,
new java.lang.String[] { "TopK", "IncludeData", "Data", "Matches", });
internal_static_core_DeleteRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_core_DeleteRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_DeleteRequest_descriptor,
new java.lang.String[] { "Ids", });
internal_static_core_InfoRequest_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_core_InfoRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_InfoRequest_descriptor,
new java.lang.String[] { "IndexSize", });
internal_static_core_FetchRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_core_FetchRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_FetchRequest_descriptor,
new java.lang.String[] { "Ids", "Vectors", });
internal_static_core_Ack_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_core_Ack_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_Ack_descriptor,
new java.lang.String[] { "Replica", "Replay", });
internal_static_core_LogEntry_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_core_LogEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_LogEntry_descriptor,
new java.lang.String[] { "Offset", "Entry", "Ack", "Data", });
internal_static_core_TraceRoute_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_core_TraceRoute_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_core_TraceRoute_descriptor,
new java.lang.String[] { "RequestId", "ClientId", "ClientOffset", "Routes", });
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy