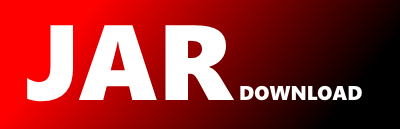
io.pivotal.services.dataTx.geode.office.ParNewCollectionsChartStatsVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dataTx-geode-office Show documentation
Show all versions of dataTx-geode-office Show documentation
APIs to convert
Apache Geode/GemFire data points to office documents such as CSVs or graphic reports
The newest version!
package io.pivotal.services.dataTx.geode.office;
import java.util.Date;
import java.util.Map;
import java.util.TreeMap;
import io.pivotal.services.dataTx.geode.operations.stats.ResourceInst;
import io.pivotal.services.dataTx.geode.operations.stats.ResourceType;
import io.pivotal.services.dataTx.geode.operations.stats.StatDescriptor;
import io.pivotal.services.dataTx.geode.operations.stats.StatValue;
import nyla.solutions.core.data.NumberedProperty;
import nyla.solutions.core.data.clock.Day;
import nyla.solutions.core.util.Text;
import nyla.solutions.office.chart.Chart;
import nyla.solutions.office.chart.JFreeChartFacade;
/**
*
* Generates a chart for Garbage collection Par New Collections per hour.
*
* @author Gregory Green
*
*/
public class ParNewCollectionsChartStatsVisitor extends AbstractChartVisitor
{
private Map countPerHour = new TreeMap<>();
private String resourceResourceNameFilter = "ParNew";
private String filterTypeName = "VMGCStats".toUpperCase();
private String filterStatName = "collections";
private String appName;
private int threshold = 1;
private final Day dayFilter;
public ParNewCollectionsChartStatsVisitor(Day dayFilter)
{
this.dayFilter = dayFilter;
String title = "Collections per second greater than "+threshold+" "+
Text.formatDate("MM/dd/yyyy",dayFilter.getDate());
this.chart.setTitle(title);
this.chart.setGraphType(Chart.BAR_GRAPH_TYPE);
}//------------------------------------------------
@Override
public void visitResourceInsts(ResourceInst[] resourceInsts)
{
this.appName = StatsUtil.getAppName(resourceInsts);
}
@Override
public void visitResourceInst(ResourceInst resourceInst)
{
String name = resourceInst.getName();
ResourceType resourceType= resourceInst.getType();
boolean skip = !this.resourceResourceNameFilter.equals(name) || resourceType == null || resourceType.getName() == null ||
(this.filterTypeName != null && !resourceType.getName().toUpperCase().contains(this.filterTypeName));
if(skip)
{
return;
}
StatValue[] statValues = resourceInst.getStatValues();
if(statValues == null)
return;
for (StatValue statValue : statValues)
{
String statName = statValue.getDescriptor().getName();
if(filterStatName != null && !filterStatName.equalsIgnoreCase(statName))
{
continue; //skip;
}
StatDescriptor statDescriptor = resourceInst.getType().getStat(statName);
long [] times = statValue.getRawAbsoluteTimeStamps();
//double [] values = statValue.getRawSnapshots();
double [] values = statValue.getSnapshots();
String timeFormat = "HH:mm:ss";
NumberedProperty current = null;
Date date = null;
Day day = null;
int newValue;
for (int i = 0; i < values.length; i++)
{
date = new Date(times[i]);
day = new Day(date);
if(!this.dayFilter.isSameDay(day))
continue;
String timeValue = Text.formatDate(timeFormat,date);
NumberedProperty value = this.countPerHour.get(timeValue);
current = new NumberedProperty(appName,Integer.valueOf(Double.valueOf(values[i]).intValue()));
if(value == null)
value = current;
else
{
newValue = Integer.valueOf(value.getNumber() + current.getNumber());
value.setNumber(newValue);
}
countPerHour.put(timeValue, value);
}
}
String entryName = null;
int intValue;
for(Map.Entry entry : this.countPerHour.entrySet())
{
entryName = entry.getValue().getName();
if(entryName == null)
entryName = "Unknown";
entryName = entryName.replace("amd64 ", "");
intValue = entry.getValue().getValueInteger();
if(intValue >= threshold)
{
this.chart.plotValue(intValue, entryName, entry.getKey());
}
}
}//------------------------------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy