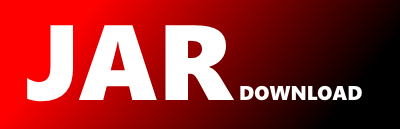
io.pivotal.arca.broadcaster.ArcaBroadcastManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of arca-broadcaster Show documentation
Show all versions of arca-broadcaster Show documentation
A comprehensive framework for caching and displaying data.
/*
* Copyright (C) 2014 Pivotal Software, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.pivotal.arca.broadcaster;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.os.Handler;
import android.os.Looper;
import android.os.Message;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class ArcaBroadcastManager {
private static final int MSG_SEND_BROADCAST = 100;
private static final Map> RECEIVERS = new HashMap>();
private static final Map> ACTIONS = new HashMap>();
private static final List BROADCASTS = new ArrayList();
private static Context sContext;
private static Handler sHandler;
public static void initialize(final Context context, final BroadcastHandler handler) {
sContext = context.getApplicationContext();
sHandler = handler;
}
private static void initializeDefaultHandler(final Context context) {
initialize(context, new BroadcastHandler(context.getMainLooper()));
}
// ====================================================
public static synchronized void registerReceiver(final BroadcastReceiver receiver, final String action) {
addToActions(receiver, action);
addToReceivers(receiver, action);
}
public static synchronized void unregisterReceiver(final BroadcastReceiver receiver) {
final Set actions = RECEIVERS.remove(receiver);
removeReceiverFromActions(receiver, actions);
}
// ====================================================
private static void addToReceivers(final BroadcastReceiver receiver, final String action) {
Set actions = RECEIVERS.get(receiver);
if (actions == null) {
actions = new HashSet(1);
RECEIVERS.put(receiver, actions);
}
actions.add(action);
}
private static void addToActions(final BroadcastReceiver receiver, final String action) {
Set receivers = ACTIONS.get(action);
if (receivers == null) {
receivers = new HashSet(1);
ACTIONS.put(action, receivers);
}
receivers.add(receiver);
}
private static void removeReceiverFromActions(final BroadcastReceiver receiver, final Set actions) {
for (final String action : actions) {
final Set receivers = ACTIONS.get(action);
if (receivers != null) {
receivers.remove(receiver);
if (receivers.size() <= 0) {
ACTIONS.remove(action);
}
}
}
}
// ====================================================
public static synchronized void sendBroadcast(final Context context, final Intent intent) {
final String action = intent.getAction();
final Set receivers = ACTIONS.get(action);
if (receivers != null) {
for (final BroadcastReceiver receiver : receivers) {
BROADCASTS.add(new Broadcast(receiver, intent));
}
notifyMessageHandler(context);
}
}
private static void notifyMessageHandler(final Context context) {
if (sHandler == null) {
initializeDefaultHandler(context);
}
if (!sHandler.hasMessages(MSG_SEND_BROADCAST)) {
sHandler.sendEmptyMessage(MSG_SEND_BROADCAST);
}
}
private static synchronized void sendAndClearBroadcasts() {
for (final Broadcast broadcast : BROADCASTS) {
broadcast.getReceiver().onReceive(sContext, broadcast.getIntent());
}
BROADCASTS.clear();
}
// ====================================================
public static class BroadcastHandler extends Handler {
public BroadcastHandler(final Looper looper) {
super(looper);
}
@Override
public void handleMessage(final Message msg) {
if (msg.what == MSG_SEND_BROADCAST) {
sendAndClearBroadcasts();
}
}
}
// ====================================================
private static final class Broadcast {
private final Intent mIntent;
private final BroadcastReceiver mReceiver;
public Broadcast(final BroadcastReceiver receiver, final Intent intent) {
mReceiver = receiver;
mIntent = intent;
}
public BroadcastReceiver getReceiver() {
return mReceiver;
}
public Intent getIntent() {
return mIntent;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy