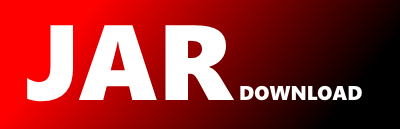
playn.java.JavaGraphics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of playn-java-base Show documentation
Show all versions of playn-java-base Show documentation
Code shared by all PlayN Java (JVM) backends
/**
* Copyright 2012 The PlayN Authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package playn.java;
import java.awt.Graphics2D;
import java.awt.RenderingHints;
import java.awt.font.FontRenderContext;
import java.awt.image.BufferedImage;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.HashMap;
import java.util.Map;
import playn.core.*;
public abstract class JavaGraphics extends Graphics {
private final Map fonts = new HashMap();
private ByteBuffer imgBuf;
// antialiased font context and aliased font context
private FontRenderContext aaFontContext, aFontContext;
protected JavaGraphics(Platform plat, GL20 gl20, Scale scale) {
super(plat, gl20, scale);
}
/** Sets the title of the window. */
abstract void setTitle (String title);
/** Uploads the image data in {@code img} into {@code tex}. */
abstract void upload (BufferedImage img, Texture tex);
// these are initialized lazily to avoid doing any AWT stuff during startup
FontRenderContext aaFontContext() {
if (aaFontContext == null) {
// set up the dummy font contexts
Graphics2D aaGfx = new BufferedImage(1, 1, BufferedImage.TYPE_INT_ARGB).createGraphics();
aaGfx.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
aaFontContext = aaGfx.getFontRenderContext();
}
return aaFontContext;
}
FontRenderContext aFontContext() {
if (aFontContext == null) {
Graphics2D aGfx = new BufferedImage(1, 1, BufferedImage.TYPE_INT_ARGB).createGraphics();
aGfx.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_OFF);
aFontContext = aGfx.getFontRenderContext();
}
return aFontContext;
}
/**
* Registers a font with the graphics system.
*
* @param name the name under which to register the font.
* @param font the Java font, which can be loaded from a path via {@link JavaAssets#getFont}.
*/
public void registerFont (String name, java.awt.Font font) {
if (font == null) throw new NullPointerException();
fonts.put(name, font);
}
/**
* Changes the size of the PlayN window. The supplied size is in display units, it will be
* converted to pixels based on the display scale factor.
*/
public abstract void setSize (int width, int height, boolean fullscreen);
@Override
public Path createPath() {
return new JavaPath();
}
@Override public Gradient createGradient(Gradient.Config cfg) {
if (cfg instanceof Gradient.Linear) return JavaGradient.create((Gradient.Linear)cfg);
else if (cfg instanceof Gradient.Radial) return JavaGradient.create((Gradient.Radial)cfg);
else throw new IllegalArgumentException("Unknown config: " + cfg);
}
@Override public TextLayout layoutText(String text, TextFormat format) {
return JavaTextLayout.layoutText(this, text, format);
}
@Override public TextLayout[] layoutText(String text, TextFormat format, TextWrap wrap) {
return JavaTextLayout.layoutText(this, text, format, wrap);
}
@Override protected Canvas createCanvasImpl (Scale scale, int pixelWidth, int pixelHeight) {
BufferedImage bitmap = new BufferedImage(
pixelWidth, pixelHeight, BufferedImage.TYPE_INT_ARGB_PRE);
return new JavaCanvas(this, new JavaImage(this, scale, bitmap, "
© 2015 - 2025 Weber Informatics LLC | Privacy Policy