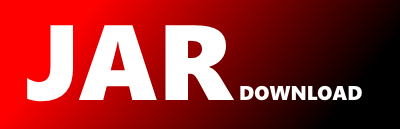
io.polygenesis.abstraction.thing.Activity Maven / Gradle / Ivy
The newest version!
/*-
* ==========================LICENSE_START=================================
* PolyGenesis Platform
* ========================================================================
* Copyright (C) 2015 - 2019 Christos Tsakostas, OREGOR LTD
* ========================================================================
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ===========================LICENSE_END==================================
*/
package io.polygenesis.abstraction.thing;
import io.polygenesis.abstraction.data.Data;
import io.polygenesis.commons.assertion.Assertion;
import io.polygenesis.commons.keyvalue.KeyValue;
import java.util.Arrays;
import java.util.LinkedHashSet;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
/**
* The type Activity.
*
* @author Christos Tsakostas
*/
public class Activity {
// ===============================================================================================
// STATE
// ===============================================================================================
private Set keyValues;
private Set externalData;
private Set externalFunctions;
private Set strings;
// ===============================================================================================
// STATIC
// ===============================================================================================
/**
* Empty activity.
*
* @return the activity
*/
public static Activity empty() {
return new Activity(
new LinkedHashSet<>(), new LinkedHashSet<>(), new LinkedHashSet<>(), new LinkedHashSet<>());
}
/**
* Key value activity.
*
* @param keyValue the key value
* @return the activity
*/
public static Activity keyValue(KeyValue keyValue) {
return new Activity(
new LinkedHashSet<>(Arrays.asList(keyValue)),
new LinkedHashSet<>(),
new LinkedHashSet<>(),
new LinkedHashSet<>());
}
/**
* Key values activity.
*
* @param keyValues the key values
* @return the activity
*/
public static Activity keyValues(Set keyValues) {
return new Activity(
keyValues, new LinkedHashSet<>(), new LinkedHashSet<>(), new LinkedHashSet<>());
}
/**
* Message handler activity.
*
* @param jsonData the json data
* @param handlerFunctions the handler functions
* @return the activity
*/
public static Activity messageHandler(Set jsonData, Set handlerFunctions) {
return new Activity(new LinkedHashSet<>(), jsonData, handlerFunctions, new LinkedHashSet<>());
}
/**
* Message handler supported messages activity.
*
* @param supportedMessages the supported messages
* @return the activity
*/
public static Activity messageHandlerSupportedMessages(Set supportedMessages) {
return new Activity(
new LinkedHashSet<>(), new LinkedHashSet<>(), new LinkedHashSet<>(), supportedMessages);
}
// ===============================================================================================
// CONSTRUCTOR(S)
// ===============================================================================================
/**
* Instantiates a new Activity.
*
* @param keyValues the key values
* @param externalData the external data
* @param externalFunctions the external functions
* @param strings the strings
*/
public Activity(
Set keyValues,
Set externalData,
Set externalFunctions,
Set strings) {
setKeyValues(keyValues);
setExternalData(externalData);
setExternalFunctions(externalFunctions);
setStrings(strings);
}
// ===============================================================================================
// GETTERS
// ===============================================================================================
/**
* Gets key values.
*
* @return the key values
*/
public Set getKeyValues() {
return keyValues;
}
/**
* Gets external data.
*
* @return the external data
*/
public Set getExternalData() {
return externalData;
}
/**
* Gets external functions.
*
* @return the external functions
*/
public Set getExternalFunctions() {
return externalFunctions;
}
/**
* Gets strings.
*
* @return the strings
*/
public Set getStrings() {
return strings;
}
// ===============================================================================================
// QUERIES
// ===============================================================================================
/**
* Has value boolean.
*
* @param key the key
* @return the boolean
*/
public Boolean hasValue(Object key) {
Optional optionalKeyValue =
getKeyValues().stream().filter(keyValue -> keyValue.getKey().equals(key)).findFirst();
return optionalKeyValue.isPresent();
}
/**
* Gets value.
*
* @param key the key
* @return the value
*/
public Object getValue(Object key) {
Assertion.isNotNull(key, "key is required");
return getKeyValues()
.stream()
.filter(keyValue -> keyValue.getKey().equals(key))
.map(keyValue -> keyValue.getValue())
.findFirst()
.orElseThrow(
() ->
new IllegalArgumentException(
String.format("Cannot find activity metadata for key=%s", key)));
}
// ===============================================================================================
// GUARDS
// ===============================================================================================
/**
* Sets key values.
*
* @param keyValues the key values
*/
private void setKeyValues(Set keyValues) {
Assertion.isNotNull(keyValues, "keyValues is required");
this.keyValues = keyValues;
}
/**
* Sets external data.
*
* @param externalData the external data
*/
public void setExternalData(Set externalData) {
Assertion.isNotNull(externalData, "externalData is required");
this.externalData = externalData;
}
/**
* Sets related functions.
*
* @param externalFunctions the related functions
*/
private void setExternalFunctions(Set externalFunctions) {
Assertion.isNotNull(externalFunctions, "externalFunctions is required");
this.externalFunctions = externalFunctions;
}
/**
* Sets strings.
*
* @param strings the strings
*/
private void setStrings(Set strings) {
Assertion.isNotNull(strings, "strings is required");
this.strings = strings;
}
// ===============================================================================================
// OVERRIDES
// ===============================================================================================
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Activity activity = (Activity) o;
return Objects.equals(externalData, activity.externalData)
&& Objects.equals(externalFunctions, activity.externalFunctions)
&& Objects.equals(strings, activity.strings);
}
@Override
public int hashCode() {
return Objects.hash(externalData, externalFunctions, strings);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy