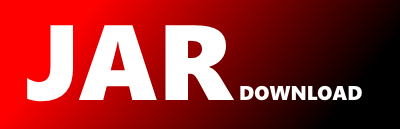
io.polyglotted.eswrapper.indexing.IndexMutations Maven / Gradle / Ivy
package io.polyglotted.eswrapper.indexing;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import lombok.AccessLevel;
import lombok.RequiredArgsConstructor;
import lombok.ToString;
import java.util.*;
import java.util.Map.Entry;
import static com.google.common.collect.Iterables.transform;
import static io.polyglotted.eswrapper.indexing.Indexable.indexableBuilder;
@RequiredArgsConstructor
@ToString(includeFieldNames = false, doNotUseGetters = true)
public final class IndexMutations {
public final ImmutableList creates;
public final ImmutableMap updates;
public final ImmutableList deletes;
public Indexable toIndexable(String index, long timestamp, Function
creator, Function, IndexRecord> updator) {
return indexableBuilder().index(index).timestamp(timestamp)
.records(transform(creates, creator))
.records(transform(updates.entrySet(), updator))
.records(transform(deletes, IndexRecord::deleteRecord))
.build();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
IndexMutations that = (IndexMutations) o;
return creates.equals(that.creates) && updates.equals(that.updates) && deletes.equals(that.deletes);
}
@Override
public int hashCode() {
return Objects.hash(creates, updates, deletes);
}
public static Builder mutationsBuilder() {
return new Builder<>();
}
@RequiredArgsConstructor(access = AccessLevel.PRIVATE)
public static class Builder {
private final List creates = new LinkedList<>();
private final Map updates = new LinkedHashMap<>();
private final List deletes = new LinkedList<>();
public Builder creates(List objects) {
this.creates.addAll(objects);
return this;
}
public Builder updates(Map updates) {
this.updates.putAll(updates);
return this;
}
public Builder deletes(List keys) {
this.deletes.addAll(keys);
return this;
}
public IndexMutations build() {
return new IndexMutations<>(ImmutableList.copyOf(creates), ImmutableMap.copyOf(updates),
ImmutableList.copyOf(deletes));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy