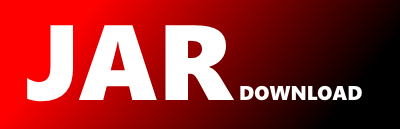
io.pravega.schemaregistry.serializer.avro.schemas.AvroSchema Maven / Gradle / Ivy
/**
* Copyright (c) Dell Inc., or its subsidiaries. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*/
package io.pravega.schemaregistry.serializer.avro.schemas;
import com.google.common.base.Charsets;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableMap;
import io.pravega.schemaregistry.contract.data.SchemaInfo;
import io.pravega.schemaregistry.contract.data.SerializationFormat;
import io.pravega.schemaregistry.serializer.shared.schemas.Schema;
import lombok.Getter;
import org.apache.avro.generic.GenericRecord;
import org.apache.avro.reflect.ReflectData;
import org.apache.avro.specific.SpecificData;
import org.apache.avro.specific.SpecificRecordBase;
import java.nio.ByteBuffer;
/**
* Container class for Avro Schema.
*
* @param Type of element.
*/
public class AvroSchema implements Schema {
@Getter
private final org.apache.avro.Schema schema;
private final SchemaInfo schemaInfo;
@Getter
private final Class tClass;
private AvroSchema(org.apache.avro.Schema schema, Class tClass) {
this.schema = schema;
this.schemaInfo = new SchemaInfo(schema.getFullName(),
SerializationFormat.Avro, getSchemaBytes(), ImmutableMap.of());
this.tClass = tClass;
}
private AvroSchema(SchemaInfo schemaInfo) {
String schemaString = new String(schemaInfo.getSchemaData().array(), Charsets.UTF_8);
this.schema = new org.apache.avro.Schema.Parser().parse(schemaString);
this.schemaInfo = schemaInfo;
this.tClass = null;
}
/**
* Method to create a typed AvroSchema for the given class. It extracts the avro schema from the class.
* For Avro generated classes, the schema is retrieved from the class.
* For POJOs the schema is extracted using avro's {@link ReflectData}.
*
* @param tClass Class whose object's schema is used.
* @param Type of the Java class.
* @return {@link AvroSchema} with generic type T that extracts and captures the avro schema.
*/
public static AvroSchema of(Class tClass) {
org.apache.avro.Schema schema;
if (SpecificRecordBase.class.isAssignableFrom(tClass)) {
schema = SpecificData.get().getSchema(tClass);
} else {
schema = ReflectData.get().getSchema(tClass);
}
return new AvroSchema<>(schema, tClass);
}
/**
* Method to create a typed AvroSchema of type {@link Object} from the given schema.
* This schema can be used to express any non record schema.
*
* @param schema Schema to use.
* @return Returns an AvroSchema with {@link Object} type.
*/
public static AvroSchema
© 2015 - 2025 Weber Informatics LLC | Privacy Policy