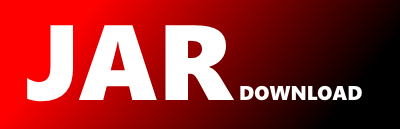
io.prestosql.plugin.jdbc.JdbcColumnHandle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of presto-base-jdbc Show documentation
Show all versions of presto-base-jdbc Show documentation
Presto - Base JDBC Connector
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.prestosql.plugin.jdbc;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.Joiner;
import io.prestosql.spi.connector.ColumnHandle;
import io.prestosql.spi.connector.ColumnMetadata;
import io.prestosql.spi.type.Type;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
import static java.util.Objects.requireNonNull;
public final class JdbcColumnHandle
implements ColumnHandle
{
private final Optional expression;
private final String columnName;
private final JdbcTypeHandle jdbcTypeHandle;
private final Type columnType;
private final boolean nullable;
private final Optional comment;
// All and only required fields
public JdbcColumnHandle(String columnName, JdbcTypeHandle jdbcTypeHandle, Type columnType)
{
this(Optional.empty(), columnName, jdbcTypeHandle, columnType, true, Optional.empty());
}
/**
* @deprecated Use {@link #builder()} instead.
*/
@Deprecated
public JdbcColumnHandle(String columnName, JdbcTypeHandle jdbcTypeHandle, Type columnType, boolean nullable)
{
this(Optional.empty(), columnName, jdbcTypeHandle, columnType, nullable, Optional.empty());
}
/**
* @deprecated This constructor is intended to be used by JSON deserialization only. Use {@link #builder()} instead.
*/
@Deprecated
@JsonCreator
public JdbcColumnHandle(
@JsonProperty("expression") Optional expression,
@JsonProperty("columnName") String columnName,
@JsonProperty("jdbcTypeHandle") JdbcTypeHandle jdbcTypeHandle,
@JsonProperty("columnType") Type columnType,
@JsonProperty("nullable") boolean nullable,
@JsonProperty("comment") Optional comment)
{
this.expression = requireNonNull(expression, "expression is null");
this.columnName = requireNonNull(columnName, "columnName is null");
this.jdbcTypeHandle = requireNonNull(jdbcTypeHandle, "jdbcTypeHandle is null");
this.columnType = requireNonNull(columnType, "columnType is null");
this.nullable = nullable;
this.comment = requireNonNull(comment, "comment is null");
}
@JsonProperty
public Optional getExpression()
{
return expression;
}
@JsonProperty
public String getColumnName()
{
return columnName;
}
@JsonProperty
public JdbcTypeHandle getJdbcTypeHandle()
{
return jdbcTypeHandle;
}
@JsonProperty
public Type getColumnType()
{
return columnType;
}
@JsonProperty
public boolean isNullable()
{
return nullable;
}
@JsonProperty
public Optional getComment()
{
return comment;
}
@JsonIgnore
public boolean isSynthetic()
{
return expression.isPresent();
}
public ColumnMetadata getColumnMetadata()
{
return ColumnMetadata.builder()
.setName(columnName)
.setType(columnType)
.setNullable(nullable)
.setComment(comment)
.build();
}
@Override
public boolean equals(Object obj)
{
if (this == obj) {
return true;
}
if ((obj == null) || (getClass() != obj.getClass())) {
return false;
}
JdbcColumnHandle o = (JdbcColumnHandle) obj;
return Objects.equals(this.columnName, o.columnName);
}
@Override
public int hashCode()
{
return Objects.hash(columnName);
}
@Override
public String toString()
{
return Joiner.on(":").skipNulls().join(
expression.orElse(null),
columnName,
columnType.getDisplayName(),
jdbcTypeHandle.getJdbcTypeName().orElse(null));
}
public String toSqlExpression(Function identifierQuote)
{
requireNonNull(identifierQuote, "identifierQuote is null");
return expression
.orElseGet(() -> identifierQuote.apply(columnName));
}
public static Builder builder()
{
return new Builder();
}
public static Builder builderFrom(JdbcColumnHandle handle)
{
return new Builder(handle);
}
public static final class Builder
{
private Optional expression = Optional.empty();
private String columnName;
private JdbcTypeHandle jdbcTypeHandle;
private Type columnType;
private boolean nullable = true;
private Optional comment = Optional.empty();
public Builder() {}
private Builder(JdbcColumnHandle handle)
{
this.expression = handle.getExpression();
this.columnName = handle.getColumnName();
this.jdbcTypeHandle = handle.getJdbcTypeHandle();
this.columnType = handle.getColumnType();
this.nullable = handle.isNullable();
this.comment = handle.getComment();
}
public Builder setExpression(Optional expression)
{
this.expression = expression;
return this;
}
public Builder setColumnName(String columnName)
{
this.columnName = columnName;
return this;
}
public Builder setJdbcTypeHandle(JdbcTypeHandle jdbcTypeHandle)
{
this.jdbcTypeHandle = jdbcTypeHandle;
return this;
}
public Builder setColumnType(Type columnType)
{
this.columnType = columnType;
return this;
}
public Builder setNullable(boolean nullable)
{
this.nullable = nullable;
return this;
}
public Builder setComment(Optional comment)
{
this.comment = comment;
return this;
}
public JdbcColumnHandle build()
{
return new JdbcColumnHandle(
expression,
columnName,
jdbcTypeHandle,
columnType,
nullable,
comment);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy