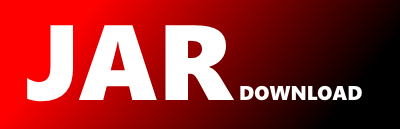
io.prestosql.execution.SqlTaskExecution Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.prestosql.execution;
import com.google.common.collect.AbstractIterator;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Iterables;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
import io.airlift.concurrent.SetThreadName;
import io.airlift.units.Duration;
import io.prestosql.event.SplitMonitor;
import io.prestosql.execution.StateMachine.StateChangeListener;
import io.prestosql.execution.buffer.BufferState;
import io.prestosql.execution.buffer.OutputBuffer;
import io.prestosql.execution.executor.TaskExecutor;
import io.prestosql.execution.executor.TaskHandle;
import io.prestosql.operator.Driver;
import io.prestosql.operator.DriverContext;
import io.prestosql.operator.DriverFactory;
import io.prestosql.operator.DriverStats;
import io.prestosql.operator.PipelineContext;
import io.prestosql.operator.PipelineExecutionStrategy;
import io.prestosql.operator.StageExecutionDescriptor;
import io.prestosql.operator.TaskContext;
import io.prestosql.sql.planner.LocalExecutionPlanner.LocalExecutionPlan;
import io.prestosql.sql.planner.plan.PlanNodeId;
import javax.annotation.Nullable;
import javax.annotation.concurrent.GuardedBy;
import javax.annotation.concurrent.NotThreadSafe;
import javax.annotation.concurrent.ThreadSafe;
import java.lang.ref.WeakReference;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.OptionalInt;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.concurrent.Executor;
import java.util.stream.Collectors;
import static com.google.common.base.MoreObjects.toStringHelper;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkState;
import static com.google.common.base.Verify.verify;
import static com.google.common.collect.ImmutableMap.toImmutableMap;
import static io.prestosql.SystemSessionProperties.getInitialSplitsPerNode;
import static io.prestosql.SystemSessionProperties.getMaxDriversPerTask;
import static io.prestosql.SystemSessionProperties.getSplitConcurrencyAdjustmentInterval;
import static io.prestosql.execution.SqlTaskExecution.SplitsState.ADDING_SPLITS;
import static io.prestosql.execution.SqlTaskExecution.SplitsState.FINISHED;
import static io.prestosql.execution.SqlTaskExecution.SplitsState.NO_MORE_SPLITS;
import static io.prestosql.operator.PipelineExecutionStrategy.UNGROUPED_EXECUTION;
import static java.lang.String.format;
import static java.util.Objects.requireNonNull;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.toList;
public class SqlTaskExecution
{
// For each driver in a task, it belong to a pipeline and a driver life cycle.
// Pipeline and driver life cycle are two perpendicular organizations of tasks.
//
// * All drivers in the same pipeline has the same shape.
// (i.e. operators are constructed from the same set of operator factories)
// * All drivers in the same driver life cycle are responsible for processing a group of data.
// (e.g. all rows that fall in bucket 42)
//
// Take a task with the following set of pipelines for example:
//
// pipeline 0 pipeline 1 pipeline 2 pipeline 3 ... pipeline id
// grouped grouped grouped ungrouped ... execution strategy
//
// PartitionedOutput
// |
// LookupJoin .................................. HashBuild
// | |
// LookupJoin ... HashBuild ExchangeSrc
// | |
// TableScan LocalExSrc ... LocalExSink
// |
// TableScan
//
// In this case,
// * a driver could belong to pipeline 1 and driver life cycle 42.
// * another driver could belong to pipeline 3 and task-wide driver life cycle.
private final TaskId taskId;
private final TaskStateMachine taskStateMachine;
private final TaskContext taskContext;
private final OutputBuffer outputBuffer;
private final TaskHandle taskHandle;
private final TaskExecutor taskExecutor;
private final Executor notificationExecutor;
private final SplitMonitor splitMonitor;
private final List> drivers = new CopyOnWriteArrayList<>();
private final Map driverRunnerFactoriesWithSplitLifeCycle;
private final List driverRunnerFactoriesWithDriverGroupLifeCycle;
private final List driverRunnerFactoriesWithTaskLifeCycle;
// guarded for update only
@GuardedBy("this")
private final ConcurrentMap unpartitionedSources = new ConcurrentHashMap<>();
@GuardedBy("this")
private long maxAcknowledgedSplit = Long.MIN_VALUE;
@GuardedBy("this")
private final SchedulingLifespanManager schedulingLifespanManager;
@GuardedBy("this")
private final Map pendingSplitsByPlanNode;
private final Status status;
static SqlTaskExecution createSqlTaskExecution(
TaskStateMachine taskStateMachine,
TaskContext taskContext,
OutputBuffer outputBuffer,
List sources,
LocalExecutionPlan localExecutionPlan,
TaskExecutor taskExecutor,
Executor notificationExecutor,
SplitMonitor queryMonitor)
{
SqlTaskExecution task = new SqlTaskExecution(
taskStateMachine,
taskContext,
outputBuffer,
localExecutionPlan,
taskExecutor,
queryMonitor,
notificationExecutor);
try (SetThreadName ignored = new SetThreadName("Task-%s", task.getTaskId())) {
// The scheduleDriversForTaskLifeCycle method calls enqueueDriverSplitRunner, which registers a callback with access to this object.
// The call back is accessed from another thread, so this code cannot be placed in the constructor.
task.scheduleDriversForTaskLifeCycle();
task.addSources(sources);
return task;
}
}
private SqlTaskExecution(
TaskStateMachine taskStateMachine,
TaskContext taskContext,
OutputBuffer outputBuffer,
LocalExecutionPlan localExecutionPlan,
TaskExecutor taskExecutor,
SplitMonitor splitMonitor,
Executor notificationExecutor)
{
this.taskStateMachine = requireNonNull(taskStateMachine, "taskStateMachine is null");
this.taskId = taskStateMachine.getTaskId();
this.taskContext = requireNonNull(taskContext, "taskContext is null");
this.outputBuffer = requireNonNull(outputBuffer, "outputBuffer is null");
this.taskExecutor = requireNonNull(taskExecutor, "driverExecutor is null");
this.notificationExecutor = requireNonNull(notificationExecutor, "notificationExecutor is null");
this.splitMonitor = requireNonNull(splitMonitor, "splitMonitor is null");
try (SetThreadName ignored = new SetThreadName("Task-%s", taskId)) {
// index driver factories
Set partitionedSources = ImmutableSet.copyOf(localExecutionPlan.getPartitionedSourceOrder());
ImmutableMap.Builder driverRunnerFactoriesWithSplitLifeCycle = ImmutableMap.builder();
ImmutableList.Builder driverRunnerFactoriesWithTaskLifeCycle = ImmutableList.builder();
ImmutableList.Builder driverRunnerFactoriesWithDriverGroupLifeCycle = ImmutableList.builder();
for (DriverFactory driverFactory : localExecutionPlan.getDriverFactories()) {
Optional sourceId = driverFactory.getSourceId();
if (sourceId.isPresent() && partitionedSources.contains(sourceId.get())) {
driverRunnerFactoriesWithSplitLifeCycle.put(sourceId.get(), new DriverSplitRunnerFactory(driverFactory, true));
}
else {
switch (driverFactory.getPipelineExecutionStrategy()) {
case GROUPED_EXECUTION:
driverRunnerFactoriesWithDriverGroupLifeCycle.add(new DriverSplitRunnerFactory(driverFactory, false));
break;
case UNGROUPED_EXECUTION:
driverRunnerFactoriesWithTaskLifeCycle.add(new DriverSplitRunnerFactory(driverFactory, false));
break;
default:
throw new UnsupportedOperationException();
}
}
}
this.driverRunnerFactoriesWithSplitLifeCycle = driverRunnerFactoriesWithSplitLifeCycle.build();
this.driverRunnerFactoriesWithDriverGroupLifeCycle = driverRunnerFactoriesWithDriverGroupLifeCycle.build();
this.driverRunnerFactoriesWithTaskLifeCycle = driverRunnerFactoriesWithTaskLifeCycle.build();
this.pendingSplitsByPlanNode = this.driverRunnerFactoriesWithSplitLifeCycle.keySet().stream()
.collect(toImmutableMap(identity(), ignore -> new PendingSplitsForPlanNode()));
this.status = new Status(
taskContext,
localExecutionPlan.getDriverFactories().stream()
.collect(toImmutableMap(DriverFactory::getPipelineId, DriverFactory::getPipelineExecutionStrategy)));
this.schedulingLifespanManager = new SchedulingLifespanManager(localExecutionPlan.getPartitionedSourceOrder(), localExecutionPlan.getStageExecutionDescriptor(), this.status);
checkArgument(this.driverRunnerFactoriesWithSplitLifeCycle.keySet().equals(partitionedSources),
"Fragment is partitioned, but not all partitioned drivers were found");
// Pre-register Lifespans for ungrouped partitioned drivers in case they end up get no splits.
for (Entry entry : this.driverRunnerFactoriesWithSplitLifeCycle.entrySet()) {
PlanNodeId planNodeId = entry.getKey();
DriverSplitRunnerFactory driverSplitRunnerFactory = entry.getValue();
if (driverSplitRunnerFactory.getPipelineExecutionStrategy() == UNGROUPED_EXECUTION) {
this.schedulingLifespanManager.addLifespanIfAbsent(Lifespan.taskWide());
this.pendingSplitsByPlanNode.get(planNodeId).getLifespan(Lifespan.taskWide());
}
}
// don't register the task if it is already completed (most likely failed during planning above)
if (!taskStateMachine.getState().isDone()) {
taskHandle = createTaskHandle(taskStateMachine, taskContext, outputBuffer, localExecutionPlan, taskExecutor);
}
else {
taskHandle = null;
}
outputBuffer.addStateChangeListener(new CheckTaskCompletionOnBufferFinish(SqlTaskExecution.this));
}
}
// this is a separate method to ensure that the `this` reference is not leaked during construction
private static TaskHandle createTaskHandle(
TaskStateMachine taskStateMachine,
TaskContext taskContext,
OutputBuffer outputBuffer,
LocalExecutionPlan localExecutionPlan,
TaskExecutor taskExecutor)
{
TaskHandle taskHandle = taskExecutor.addTask(
taskStateMachine.getTaskId(),
outputBuffer::getUtilization,
getInitialSplitsPerNode(taskContext.getSession()),
getSplitConcurrencyAdjustmentInterval(taskContext.getSession()),
getMaxDriversPerTask(taskContext.getSession()));
taskStateMachine.addStateChangeListener(state -> {
if (state.isDone()) {
taskExecutor.removeTask(taskHandle);
for (DriverFactory factory : localExecutionPlan.getDriverFactories()) {
factory.noMoreDrivers();
}
}
});
return taskHandle;
}
public TaskId getTaskId()
{
return taskId;
}
public TaskContext getTaskContext()
{
return taskContext;
}
public void addSources(List sources)
{
requireNonNull(sources, "sources is null");
checkState(!Thread.holdsLock(this), "Cannot add sources while holding a lock on the %s", getClass().getSimpleName());
try (SetThreadName ignored = new SetThreadName("Task-%s", taskId)) {
// update our record of sources and schedule drivers for new partitioned splits
Map updatedUnpartitionedSources = updateSources(sources);
// tell existing drivers about the new splits; it is safe to update drivers
// multiple times and out of order because sources contain full record of
// the unpartitioned splits
for (WeakReference driverReference : drivers) {
Driver driver = driverReference.get();
// the driver can be GCed due to a failure or a limit
if (driver == null) {
// remove the weak reference from the list to avoid a memory leak
// NOTE: this is a concurrent safe operation on a CopyOnWriteArrayList
drivers.remove(driverReference);
continue;
}
Optional sourceId = driver.getSourceId();
if (sourceId.isEmpty()) {
continue;
}
TaskSource sourceUpdate = updatedUnpartitionedSources.get(sourceId.get());
if (sourceUpdate == null) {
continue;
}
driver.updateSource(sourceUpdate);
}
// we may have transitioned to no more splits, so check for completion
checkTaskCompletion();
}
}
private synchronized Map updateSources(List sources)
{
Map updatedUnpartitionedSources = new HashMap<>();
// first remove any split that was already acknowledged
long currentMaxAcknowledgedSplit = this.maxAcknowledgedSplit;
sources = sources.stream()
.map(source -> new TaskSource(
source.getPlanNodeId(),
source.getSplits().stream()
.filter(scheduledSplit -> scheduledSplit.getSequenceId() > currentMaxAcknowledgedSplit)
.collect(Collectors.toSet()),
// Like splits, noMoreSplitsForLifespan could be pruned so that only new items will be processed.
// This is not happening here because correctness won't be compromised due to duplicate events for noMoreSplitsForLifespan.
source.getNoMoreSplitsForLifespan(),
source.isNoMoreSplits()))
.collect(toList());
// update task with new sources
for (TaskSource source : sources) {
if (driverRunnerFactoriesWithSplitLifeCycle.containsKey(source.getPlanNodeId())) {
schedulePartitionedSource(source);
}
else {
scheduleUnpartitionedSource(source, updatedUnpartitionedSources);
}
}
for (DriverSplitRunnerFactory driverSplitRunnerFactory :
Iterables.concat(driverRunnerFactoriesWithSplitLifeCycle.values(), driverRunnerFactoriesWithTaskLifeCycle, driverRunnerFactoriesWithDriverGroupLifeCycle)) {
driverSplitRunnerFactory.closeDriverFactoryIfFullyCreated();
}
// update maxAcknowledgedSplit
maxAcknowledgedSplit = sources.stream()
.flatMap(source -> source.getSplits().stream())
.mapToLong(ScheduledSplit::getSequenceId)
.max()
.orElse(maxAcknowledgedSplit);
return updatedUnpartitionedSources;
}
@GuardedBy("this")
private void mergeIntoPendingSplits(PlanNodeId planNodeId, Set scheduledSplits, Set noMoreSplitsForLifespan, boolean noMoreSplits)
{
checkHoldsLock();
DriverSplitRunnerFactory partitionedDriverFactory = driverRunnerFactoriesWithSplitLifeCycle.get(planNodeId);
PendingSplitsForPlanNode pendingSplitsForPlanNode = pendingSplitsByPlanNode.get(planNodeId);
partitionedDriverFactory.splitsAdded(scheduledSplits.size());
for (ScheduledSplit scheduledSplit : scheduledSplits) {
Lifespan lifespan = scheduledSplit.getSplit().getLifespan();
checkLifespan(partitionedDriverFactory.getPipelineExecutionStrategy(), lifespan);
pendingSplitsForPlanNode.getLifespan(lifespan).addSplit(scheduledSplit);
schedulingLifespanManager.addLifespanIfAbsent(lifespan);
}
for (Lifespan lifespanWithNoMoreSplits : noMoreSplitsForLifespan) {
checkLifespan(partitionedDriverFactory.getPipelineExecutionStrategy(), lifespanWithNoMoreSplits);
pendingSplitsForPlanNode.getLifespan(lifespanWithNoMoreSplits).noMoreSplits();
schedulingLifespanManager.addLifespanIfAbsent(lifespanWithNoMoreSplits);
}
if (noMoreSplits) {
pendingSplitsForPlanNode.setNoMoreSplits();
}
}
private synchronized void schedulePartitionedSource(TaskSource sourceUpdate)
{
mergeIntoPendingSplits(sourceUpdate.getPlanNodeId(), sourceUpdate.getSplits(), sourceUpdate.getNoMoreSplitsForLifespan(), sourceUpdate.isNoMoreSplits());
while (true) {
// SchedulingLifespanManager tracks how far each Lifespan has been scheduled. Here is an example.
// Let's say there are 4 source pipelines/nodes: A, B, C, and D, in scheduling order.
// And we're processing 3 concurrent lifespans at a time. In this case, we could have
//
// * Lifespan 10: A B [C] D; i.e. Pipeline A and B has finished scheduling (but not necessarily finished running).
// * Lifespan 20: [A] B C D
// * Lifespan 30: A [B] C D
//
// To recap, SchedulingLifespanManager records the next scheduling source node for each lifespan.
Iterator activeLifespans = schedulingLifespanManager.getActiveLifespans();
boolean madeProgress = false;
while (activeLifespans.hasNext()) {
SchedulingLifespan schedulingLifespan = activeLifespans.next();
Lifespan lifespan = schedulingLifespan.getLifespan();
// Continue using the example from above. Let's say the sourceUpdate adds some new splits for source node B.
//
// For lifespan 30, it could start new drivers and assign a pending split to each.
// Pending splits could include both pre-existing pending splits, and the new ones from sourceUpdate.
// If there is enough driver slots to deplete pending splits, one of the below would happen.
// * If it is marked that all splits for node B in lifespan 30 has been received, SchedulingLifespanManager
// will be updated so that lifespan 30 now processes source node C. It will immediately start processing them.
// * Otherwise, processing of lifespan 30 will be shelved for now.
//
// It is possible that the following loop would be a no-op for a particular lifespan.
// It is also possible that a single lifespan can proceed through multiple source nodes in one run.
//
// When different drivers in the task has different pipelineExecutionStrategy, it adds additional complexity.
// For example, when driver B is ungrouped and driver A, C, D is grouped, you could have something like this:
// TaskWide : [B]
// Lifespan 10: A [ ] C D
// Lifespan 20: [A] C D
// Lifespan 30: A [ ] C D
// In this example, Lifespan 30 cannot start executing drivers in pipeline C because pipeline B
// hasn't finished scheduling yet (albeit in a different lifespan).
// Similarly, it wouldn't make sense for TaskWide to start executing drivers in pipeline B until at least
// one lifespan has finished scheduling pipeline A.
// This is why getSchedulingPlanNode returns an Optional.
while (true) {
Optional optionalSchedulingPlanNode = schedulingLifespan.getSchedulingPlanNode();
if (optionalSchedulingPlanNode.isEmpty()) {
break;
}
PlanNodeId schedulingPlanNode = optionalSchedulingPlanNode.get();
DriverSplitRunnerFactory partitionedDriverRunnerFactory = driverRunnerFactoriesWithSplitLifeCycle.get(schedulingPlanNode);
PendingSplits pendingSplits = pendingSplitsByPlanNode.get(schedulingPlanNode).getLifespan(lifespan);
// Enqueue driver runners with driver group lifecycle for this driver life cycle, if not already enqueued.
if (!lifespan.isTaskWide() && !schedulingLifespan.getAndSetDriversForDriverGroupLifeCycleScheduled()) {
scheduleDriversForDriverGroupLifeCycle(lifespan);
}
// Enqueue driver runners with split lifecycle for this plan node and driver life cycle combination.
ImmutableList.Builder runners = ImmutableList.builder();
for (ScheduledSplit scheduledSplit : pendingSplits.removeAllSplits()) {
// create a new driver for the split
runners.add(partitionedDriverRunnerFactory.createDriverRunner(scheduledSplit, lifespan));
}
enqueueDriverSplitRunner(false, runners.build());
// If all driver runners have been enqueued for this plan node and driver life cycle combination,
// move on to the next plan node.
if (pendingSplits.getState() != NO_MORE_SPLITS) {
break;
}
partitionedDriverRunnerFactory.noMoreDriverRunner(ImmutableList.of(lifespan));
pendingSplits.markAsCleanedUp();
schedulingLifespan.nextPlanNode();
madeProgress = true;
if (schedulingLifespan.isDone()) {
break;
}
}
}
if (!madeProgress) {
break;
}
}
if (sourceUpdate.isNoMoreSplits()) {
schedulingLifespanManager.noMoreSplits(sourceUpdate.getPlanNodeId());
}
}
private synchronized void scheduleUnpartitionedSource(TaskSource sourceUpdate, Map updatedUnpartitionedSources)
{
// create new source
TaskSource newSource;
TaskSource currentSource = unpartitionedSources.get(sourceUpdate.getPlanNodeId());
if (currentSource == null) {
newSource = sourceUpdate;
}
else {
newSource = currentSource.update(sourceUpdate);
}
// only record new source if something changed
if (newSource != currentSource) {
unpartitionedSources.put(sourceUpdate.getPlanNodeId(), newSource);
updatedUnpartitionedSources.put(sourceUpdate.getPlanNodeId(), newSource);
}
}
// scheduleDriversForTaskLifeCycle and scheduleDriversForDriverGroupLifeCycle are similar.
// They are invoked under different circumstances, and schedules a disjoint set of drivers, as suggested by their names.
// They also have a few differences, making it more convenient to keep the two methods separate.
private void scheduleDriversForTaskLifeCycle()
{
// This method is called at the beginning of the task.
// It schedules drivers for all the pipelines that have task life cycle.
List runners = new ArrayList<>();
for (DriverSplitRunnerFactory driverRunnerFactory : driverRunnerFactoriesWithTaskLifeCycle) {
for (int i = 0; i < driverRunnerFactory.getDriverInstances().orElse(1); i++) {
runners.add(driverRunnerFactory.createDriverRunner(null, Lifespan.taskWide()));
}
}
enqueueDriverSplitRunner(true, runners);
for (DriverSplitRunnerFactory driverRunnerFactory : driverRunnerFactoriesWithTaskLifeCycle) {
driverRunnerFactory.noMoreDriverRunner(ImmutableList.of(Lifespan.taskWide()));
verify(driverRunnerFactory.isNoMoreDriverRunner());
}
}
private void scheduleDriversForDriverGroupLifeCycle(Lifespan lifespan)
{
// This method is called when a split that belongs to a previously unseen driver group is scheduled.
// It schedules drivers for all the pipelines that have driver group life cycle.
if (lifespan.isTaskWide()) {
checkArgument(driverRunnerFactoriesWithDriverGroupLifeCycle.isEmpty(), "Instantiating pipeline of driver group lifecycle at task level is not allowed");
return;
}
List runners = new ArrayList<>();
for (DriverSplitRunnerFactory driverSplitRunnerFactory : driverRunnerFactoriesWithDriverGroupLifeCycle) {
for (int i = 0; i < driverSplitRunnerFactory.getDriverInstances().orElse(1); i++) {
runners.add(driverSplitRunnerFactory.createDriverRunner(null, lifespan));
}
}
enqueueDriverSplitRunner(true, runners);
for (DriverSplitRunnerFactory driverRunnerFactory : driverRunnerFactoriesWithDriverGroupLifeCycle) {
driverRunnerFactory.noMoreDriverRunner(ImmutableList.of(lifespan));
}
}
private synchronized void enqueueDriverSplitRunner(boolean forceRunSplit, List runners)
{
// schedule driver to be executed
List> finishedFutures = taskExecutor.enqueueSplits(taskHandle, forceRunSplit, runners);
checkState(finishedFutures.size() == runners.size(), "Expected %s futures but got %s", runners.size(), finishedFutures.size());
// when driver completes, update state and fire events
for (int i = 0; i < finishedFutures.size(); i++) {
ListenableFuture> finishedFuture = finishedFutures.get(i);
DriverSplitRunner splitRunner = runners.get(i);
// record new driver
status.incrementRemainingDriver(splitRunner.getLifespan());
Futures.addCallback(finishedFuture, new FutureCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy