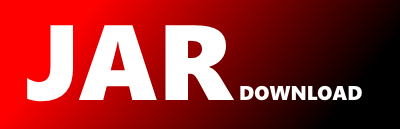
io.prismic.Document Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-kit Show documentation
Show all versions of java-kit Show documentation
The developer kit to access Prismic.io repositories using the Java language.
The newest version!
package io.prismic;
import com.fasterxml.jackson.databind.JsonNode;
import io.prismic.Fragment.Link;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.util.*;
public class Document extends WithFragments {
private static final DateTimeFormatter PUBLICATION_DATE_FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd'T'HH:mm:ssZ");
private final String id;
private final String uid;
private final String href;
private final Set tags;
private final List slugs;
private final String type;
private final String lang;
private final List alternateLanguages;
private final ZonedDateTime firstPublicationDate;
private final ZonedDateTime lastPublicationDate;
private final Map fragments;
public Document(String id, String uid, String type, String href, Set tags, List slugs, String lang, List alternateLanguages, ZonedDateTime firstPublicationDate, ZonedDateTime lastPublicationDate, Map fragments) {
this.id = id;
this.uid = uid;
this.type = type;
this.href = href;
this.tags = Collections.unmodifiableSet(tags);
this.slugs = Collections.unmodifiableList(slugs);
this.lang = lang;
this.alternateLanguages = Collections.unmodifiableList(alternateLanguages);
this.firstPublicationDate = firstPublicationDate;
this.lastPublicationDate = lastPublicationDate;
this.fragments = Collections.unmodifiableMap(fragments);
}
public String getId() {
return id;
}
public String getUid() {
return uid;
}
public String getType() {
return type;
}
public String getHref() {
return href;
}
public Set getTags() {
return tags;
}
public List getSlugs() {
return slugs;
}
public String getSlug() {
if(slugs.size() > 0) {
return slugs.get(0);
}
return null;
}
public String getLang() {
return lang;
}
public List getAlternateLanguages() {
return alternateLanguages;
}
public ZonedDateTime getFirstPublicationDate() {
return firstPublicationDate;
}
public ZonedDateTime getLastPublicationDate() {
return lastPublicationDate;
}
@Override
public Map getFragments() {
return fragments;
}
public Fragment.Group getGroup(String field) {
Fragment fragment = get(field);
if(fragment != null && fragment instanceof Fragment.Group) {
return (Fragment.Group)fragment;
}
return null;
}
public String toString() {
return "Document#" + id + " [" + type + "]";
}
public Fragment.DocumentLink asDocumentLink() {
return new Fragment.DocumentLink(id, uid, type, tags, getSlug(), lang, fragments, false);
}
static Fragment parseFragment(String type, JsonNode json) {
switch (type) {
case "StructuredText":
return Fragment.StructuredText.parse(json);
case "Image":
return Fragment.Image.parse(json);
case "Link.web":
return Link.WebLink.parse(json);
case "Link.document":
return Link.DocumentLink.parse(json);
case "Link.file":
return Link.FileLink.parse(json);
case "Link.image":
return Link.ImageLink.parse(json);
case "Text":
return Fragment.Text.parse(json);
case "Select":
return Fragment.Text.parse(json);
case "Date":
return Fragment.Date.parse(json);
case "Timestamp":
return Fragment.Timestamp.parse(json);
case "Number":
return Fragment.Number.parse(json);
case "Color":
return Fragment.Color.parse(json);
case "Embed":
return Fragment.Embed.parse(json);
case "GeoPoint":
return Fragment.GeoPoint.parse(json);
case "Group":
return Fragment.Group.parse(json);
case "SliceZone":
return Fragment.SliceZone.parse(json);
default:
return Fragment.Raw.parse(json);
}
}
static Map parseFragments(JsonNode json, String type) {
Iterator dataJson = json.fieldNames();
Map fragments = new LinkedHashMap<>();
while(dataJson.hasNext()) {
String field = dataJson.next();
JsonNode fieldJson = json.path(field);
if(fieldJson.isArray()) {
for(int i=0; i alternateLanguagesJson = json.withArray("alternate_languages").elements();
List alternateLanguages = new ArrayList<>();
while(alternateLanguagesJson.hasNext()) {
JsonNode altLangJson = alternateLanguagesJson.next();
String altLangId = altLangJson.path("id").asText();
String altLangUid = altLangJson.has("uid") ? altLangJson.path("uid").asText() : null;
String altLangType = altLangJson.path("type").asText();
String altLangCode = altLangJson.path("lang").asText();
alternateLanguages.add(new AlternateLanguage(altLangId, altLangUid, altLangType, altLangCode));
}
Iterator tagsJson = json.withArray("tags").elements();
Set tags = new HashSet<>();
while(tagsJson.hasNext()) {
tags.add(tagsJson.next().asText());
}
Iterator slugsJson = json.withArray("slugs").elements();
List slugs = new ArrayList<>();
while(slugsJson.hasNext()) {
try {
slugs.add(URLDecoder.decode(slugsJson.next().asText(), "UTF-8"));
} catch (UnsupportedEncodingException e) {
// Never happens, UTF-8 is supported everywhere!
throw new RuntimeException(e);
}
}
Map fragments = parseFragments(json.with("data").with(type), type);
return new Document(id, uid, type, href, tags, slugs, lang, alternateLanguages, firstPublicationDate, lastPublicationDate, fragments);
}
private static ZonedDateTime parseDateTime(JsonNode json) {
return "null".equals(json.asText())
? null
: ZonedDateTime.parse(json.asText(), PUBLICATION_DATE_FORMATTER);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy