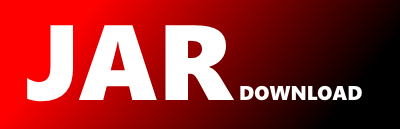
reactor.ipc.stream.StreamOperationsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reactor-ipc Show documentation
Show all versions of reactor-ipc Show documentation
IPC contracts for Reactive transport and protocol
The newest version!
/*
* Copyright (c) 2011-2016 Pivotal Software Inc, All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package reactor.ipc.stream;
import java.io.IOException;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicLong;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Subscription;
import reactor.core.publisher.Operators;
import reactor.ipc.connector.Inbound;
import reactor.util.Logger;
import reactor.util.Loggers;
/**
* Allows registering Subscribers and Subscriptions for incoming messages
* and allows sending messages.
*/
final class StreamOperationsImpl extends AtomicLong
implements StreamOperations {
static Logger log = Loggers.getLogger(StreamOperationsImpl.class);
final ConcurrentMap> subscribers;
final ConcurrentMap subscriptions;
final StreamOutbound remote;
final String name;
final OnStream onNew;
final Runnable onTerminate;
final AtomicBoolean terminateOnce;
final Inbound extends IN> channel;
StreamOperationsImpl(String name,
OnStream onNew,
StreamOutbound remote,
Inbound extends IN> channel,
Runnable onTerminate) {
super(1);
this.name = name;
this.channel = channel;
this.remote = remote;
this.onNew = onNew;
this.onTerminate = onTerminate;
this.terminateOnce = new AtomicBoolean();
this.subscribers = new ConcurrentHashMap<>();
this.subscriptions = new ConcurrentHashMap<>();
}
long newStreamId() {
return getAndIncrement();
}
void registerSubscription(long streamId, Subscription s) {
if (subscriptions.putIfAbsent(streamId, s) != null) {
throw new IllegalStateException("StreamID " + streamId + " already registered");
}
}
void registerSubscriber(long streamId, Subscriber s) {
if (subscribers.putIfAbsent(streamId, s) != null) {
throw new IllegalStateException("StreamID " + streamId + " already registered");
}
}
boolean deregister(long streamId) {
subscribers.remove(streamId);
return subscriptions.remove(streamId) != null;
}
@Override
public void onNew(long streamId, String function) {
if (log.isDebugEnabled()) {
log.debug("{}/onStream/{}/{}", name, streamId, function);
}
if (!onNew.onStream(streamId, function, this)) {
if (log.isDebugEnabled()) {
log.debug("{}/onStream/{} {}",
name,
streamId,
"New stream(" + function + ") rejected");
}
sendCancel(streamId, "New stream(" + function + ") rejected");
}
}
@Override
public void onNext(long streamId, Object o) {
if (log.isDebugEnabled()) {
log.debug("{}/onNext/{}/value={}", name, streamId, o);
}
@SuppressWarnings("unchecked") Subscriber
© 2015 - 2025 Weber Informatics LLC | Privacy Policy