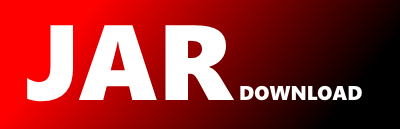
reactor.bus.registry.CachingRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reactor-bus Show documentation
Show all versions of reactor-bus Show documentation
Reactor Event Bus components
/*
* Copyright (c) 2011-2014 Pivotal Software, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package reactor.bus.registry;
import com.gs.collections.api.block.procedure.Procedure;
import com.gs.collections.api.list.MutableList;
import com.gs.collections.impl.list.mutable.FastList;
import com.gs.collections.impl.list.mutable.MultiReaderFastList;
import com.gs.collections.impl.map.mutable.UnifiedMap;
import reactor.bus.selector.Selector;
import reactor.fn.Consumer;
import reactor.jarjar.jsr166e.ConcurrentHashMapV8;
import java.util.Iterator;
import java.util.List;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* Implementation of {@link Registry} that uses a partitioned cache that partitions on thread
* id.
*
* @author Jon Brisbin
* @author Stephane Maldini
*/
public class CachingRegistry implements Registry {
private final NewThreadLocalRegsFn newThreadLocalRegsFn = new NewThreadLocalRegsFn();
private final boolean useCache;
private final boolean cacheNotFound;
private final Consumer onNotFound;
private final MultiReaderFastList> registrations;
private final ConcurrentHashMapV8>>> threadLocalCache;
CachingRegistry(boolean useCache, boolean cacheNotFound, Consumer onNotFound) {
this.useCache = useCache;
this.cacheNotFound = cacheNotFound;
this.onNotFound = onNotFound;
this.registrations = MultiReaderFastList.newList();
this.threadLocalCache = new ConcurrentHashMapV8>>>();
}
@Override
public Registration register(Selector sel, V obj) {
RemoveRegistration removeFn = new RemoveRegistration();
final Registration reg = new CachableRegistration<>(sel, obj, removeFn);
removeFn.reg = reg;
registrations.withWriteLockAndDelegate(new Procedure>>() {
@Override
public void value(MutableList> regs) {
regs.add(reg);
}
});
if (useCache) {
threadLocalCache.clear();
}
return reg;
}
@Override
@SuppressWarnings("unchecked")
public boolean unregister(final K key) {
final AtomicBoolean modified = new AtomicBoolean(false);
registrations.withWriteLockAndDelegate(new Procedure>>() {
@Override
public void value(final MutableList> regs) {
Iterator> registrationIterator = regs.iterator();
Registration reg;
while (registrationIterator.hasNext()) {
reg = registrationIterator.next();
if (reg.getSelector().matches(key)) {
registrationIterator.remove();
modified.compareAndSet(false, true);
}
}
if (useCache && modified.get()) {
threadLocalCache.clear();
}
}
});
return modified.get();
}
@Override
public List> select(K key) {
// use a thread-local cache
UnifiedMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy