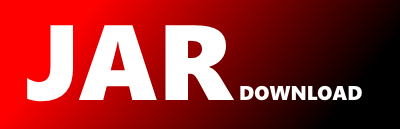
reactor.bus.selector.UriSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reactor-bus Show documentation
Show all versions of reactor-bus Show documentation
Reactor Event Bus components
The newest version!
/*
* Copyright (c) 2011-2014 Pivotal Software, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package reactor.bus.selector;
import javax.annotation.Nullable;
import java.io.UnsupportedEncodingException;
import java.net.URI;
import java.net.URLDecoder;
import java.util.HashMap;
import java.util.Map;
/**
* A {@link Selector} implementation that matches on various components of a full URI.
*
* The {@code UriSelector} will explode a URI into its component parts. If you use the following as a selector:
*
* reactor.on(U("scheme://host/path"), ...);
*
* Then any {@link java.net.URI} or String that matches those elements will be matched.
*
*
* It's not an exact string match but a match on components because you can pass parameters in the form of a query
* string. Consider the following example:
*
* reactor.on(U("tcp://*:3000/topic"), consumer);
*
* This means "match any URIs using scheme 'tcp' to port '3000' for the path '/topic' irregardless of host". When a URI
* is sent as the key to a notify like this:
*
* reactor.notify("tcp://user:[email protected]:3000/topic?param=value"), event);
*
* The match will succeed and the headers will be set as follows:
*
* - {@code scheme}: "tcp"
* - {@code authorization}: "user:[email protected]:3000"
* - {@code userInfo}: "user:ENCODEDPWD"
* - {@code host}: "192.168.0.10"
* - {@code port}: "3000"
* - {@code path}: "/topic"
* - {@code fragment}: {@code null}
* - {@code query}: "param=value"
* - {@code param}: "value"
*
*
*
* Wildcards can be used in place of {@code host}, and {@code path}. The former by replacing the host with '*' and the
* latter by replacing the path with '/*'.
*
*
* @author Jon Brisbin
*/
public class UriSelector extends ObjectSelector
© 2015 - 2025 Weber Informatics LLC | Privacy Policy