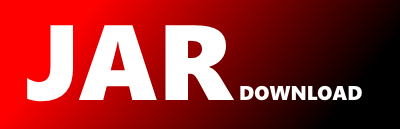
reactor.rx.stream.BarrierStream Maven / Gradle / Ivy
package reactor.rx.stream;
import org.reactivestreams.Subscriber;
import reactor.Environment;
import reactor.core.Dispatcher;
import reactor.core.support.Exceptions;
import reactor.fn.Consumer;
import reactor.fn.Function;
import reactor.rx.Stream;
import reactor.rx.subscription.PushSubscription;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
/**
* A {@code BarrierStream} provides a type of {@code Stream} into which you can bind {@link reactor.fn.Consumer
* Consumers} and {@link reactor.fn.Function Functions} from arbitrary components. This allows you to create a {@code
* Stream} that will be triggered when all bound callbacks have been invoked. The {@code List<Object>} passed
* downstream will be the accumulation of all the values either passed into a {@code Consumer} or the return value from
* a bound {@code Function}. This provides a way for the user to cleanly create a dependency chain of arbitrary
* callbacks and aggregate the results into a single value.
*
* BarrierStream barrierStream = new BarrierStream();
*
* EventBus bus = EventBus.create(Environment.get());
* bus.on($("hello"), barrierStream.wrap((Event ev) -> {
* System.out.println("got in bus: " + ev.getData());
* }));
*
* Streams.just("Hello World!")
* .map(barrierStream.wrap((Function) String::toUpperCase))
* .consume(s -> {
* System.out.println("got in stream: " + s);
* });
*
* barrierStream.consume(vals -> {
* System.out.println("got vals: " + vals);
* });
*
* bus.notify("hello", Event.wrap("Hello World!"));
*
*
* NOTE: To get blocking semantics for the calling thread, you only need to call {@link reactor.rx.Stream#next()} to return a
* {@code Promise}.
*
*/
public class BarrierStream extends Stream> {
private final AtomicInteger wrappedCnt = new AtomicInteger(0);
private final AtomicInteger resultCnt = new AtomicInteger(0);
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy