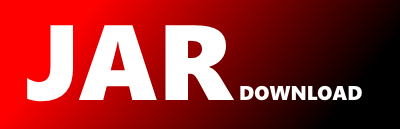
io.prometheus.metrics.instrumentation.jvm.JvmGarbageCollectorMetrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmx_prometheus_httpserver Show documentation
Show all versions of jmx_prometheus_httpserver Show documentation
See https://github.com/prometheus/jmx_exporter/blob/master/README.md
package io.prometheus.metrics.instrumentation.jvm;
import io.prometheus.metrics.config.PrometheusProperties;
import io.prometheus.metrics.core.metrics.SummaryWithCallback;
import io.prometheus.metrics.model.registry.PrometheusRegistry;
import io.prometheus.metrics.model.snapshots.Quantiles;
import io.prometheus.metrics.model.snapshots.Unit;
import java.lang.management.GarbageCollectorMXBean;
import java.lang.management.ManagementFactory;
import java.util.List;
/**
* JVM Garbage Collector metrics. The {@link JvmGarbageCollectorMetrics} are registered as part of the {@link JvmMetrics} like this:
* {@code
* JvmMetrics.builder().register();
* }
* However, if you want only the {@link JvmGarbageCollectorMetrics} you can also register them directly:
* {@code
* JvmGarbageCollectorMetrics.builder().register();
* }
* Example metrics being exported:
*
* # HELP jvm_gc_collection_seconds Time spent in a given JVM garbage collector in seconds.
* # TYPE jvm_gc_collection_seconds summary
* jvm_gc_collection_seconds_count{gc="PS MarkSweep"} 0
* jvm_gc_collection_seconds_sum{gc="PS MarkSweep"} 0.0
* jvm_gc_collection_seconds_count{gc="PS Scavenge"} 0
* jvm_gc_collection_seconds_sum{gc="PS Scavenge"} 0.0
*
*/
public class JvmGarbageCollectorMetrics {
private static final String JVM_GC_COLLECTION_SECONDS = "jvm_gc_collection_seconds";
private final PrometheusProperties config;
private final List garbageCollectorBeans;
private JvmGarbageCollectorMetrics(List garbageCollectorBeans, PrometheusProperties config) {
this.config = config;
this.garbageCollectorBeans = garbageCollectorBeans;
}
private void register(PrometheusRegistry registry) {
SummaryWithCallback.builder(config)
.name(JVM_GC_COLLECTION_SECONDS)
.help("Time spent in a given JVM garbage collector in seconds.")
.unit(Unit.SECONDS)
.labelNames("gc")
.callback(callback -> {
for (GarbageCollectorMXBean gc : garbageCollectorBeans) {
callback.call(gc.getCollectionCount(), Unit.millisToSeconds(gc.getCollectionTime()), Quantiles.EMPTY, gc.getName());
}
})
.register(registry);
}
public static Builder builder() {
return new Builder(PrometheusProperties.get());
}
public static Builder builder(PrometheusProperties config) {
return new Builder(config);
}
public static class Builder {
private final PrometheusProperties config;
private List garbageCollectorBeans;
private Builder(PrometheusProperties config) {
this.config = config;
}
/**
* Package private. For testing only.
*/
Builder garbageCollectorBeans(List garbageCollectorBeans) {
this.garbageCollectorBeans = garbageCollectorBeans;
return this;
}
public void register() {
register(PrometheusRegistry.defaultRegistry);
}
public void register(PrometheusRegistry registry) {
List garbageCollectorBeans = this.garbageCollectorBeans;
if (garbageCollectorBeans == null) {
garbageCollectorBeans = ManagementFactory.getGarbageCollectorMXBeans();
}
new JvmGarbageCollectorMetrics(garbageCollectorBeans, config).register(registry);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy