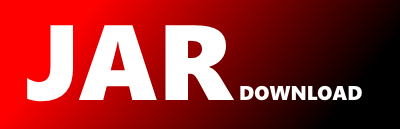
io.prometheus.client.dropwizard.DropwizardExports Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simpleclient_dropwizard Show documentation
Show all versions of simpleclient_dropwizard Show documentation
Collector of data from Dropwizard metrics library.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy