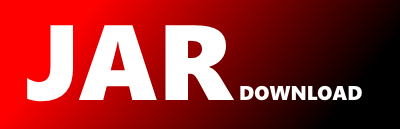
io.protostuff.compiler.model.ImmutableModuleConfiguration Maven / Gradle / Ivy
package io.protostuff.compiler.model;
import com.google.common.base.MoreObjects;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Lists;
import java.nio.file.Path;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link ModuleConfiguration}.
*
* Use builder to create immutable instances:
* {@code ImmutableModuleConfiguration.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "ModuleConfiguration"})
@Immutable
public final class ImmutableModuleConfiguration implements ModuleConfiguration {
private final String name;
private final ImmutableList includePaths;
private final ImmutableList protoFiles;
private final String generator;
private final String output;
private final ImmutableMap options;
private ImmutableModuleConfiguration(
String name,
ImmutableList includePaths,
ImmutableList protoFiles,
String generator,
String output,
ImmutableMap options) {
this.name = name;
this.includePaths = includePaths;
this.protoFiles = protoFiles;
this.generator = generator;
this.output = output;
this.options = options;
}
/**
* Module name.
*/
@Override
public String getName() {
return name;
}
/**
* List of "include" folders used to search for proto files.
*/
@Override
public ImmutableList getIncludePaths() {
return includePaths;
}
/**
* List of proto files to compile.
*/
@Override
public ImmutableList getProtoFiles() {
return protoFiles;
}
/**
* Generator used to compile proto files. Currently following generators
* are supported:
*
* java
- produces Java source code that uses protostuff runtime;
* html
- produces HTML documentation;
* st4
- generic generator, you should provide custom template
* (StringTemplate 4) using {@linkplain #getOptions()}.
*
*
*/
@Override
public String getGenerator() {
return generator;
}
/**
* Output directory.
*/
@Override
public String getOutput() {
return output;
}
/**
* Map of custom settings passed to the generator.
*/
@Override
public ImmutableMap getOptions() {
return options;
}
/**
* Copy current immutable object by setting value for {@link ModuleConfiguration#getName() name}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for name
* @return modified copy of the {@code this} object
*/
public final ImmutableModuleConfiguration withName(String value) {
if (this.name == value) return this;
String newValue = Preconditions.checkNotNull(value);
return new ImmutableModuleConfiguration(newValue, this.includePaths, this.protoFiles, this.generator, this.output, this.options);
}
/**
* Copy current immutable object with elements that replace content of {@link ModuleConfiguration#getIncludePaths() includePaths}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final ImmutableModuleConfiguration withIncludePaths(Path... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableModuleConfiguration(this.name, newValue, this.protoFiles, this.generator, this.output, this.options);
}
/**
* Copy current immutable object with elements that replace content of {@link ModuleConfiguration#getIncludePaths() includePaths}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of includePaths elements to set
* @return modified copy of {@code this} object
*/
public final ImmutableModuleConfiguration withIncludePaths(Iterable extends Path> elements) {
if (this.includePaths == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableModuleConfiguration(this.name, newValue, this.protoFiles, this.generator, this.output, this.options);
}
/**
* Copy current immutable object with elements that replace content of {@link ModuleConfiguration#getProtoFiles() protoFiles}.
* @param elements elements to set
* @return modified copy of {@code this} object
*/
public final ImmutableModuleConfiguration withProtoFiles(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableModuleConfiguration(this.name, this.includePaths, newValue, this.generator, this.output, this.options);
}
/**
* Copy current immutable object with elements that replace content of {@link ModuleConfiguration#getProtoFiles() protoFiles}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements iterable of protoFiles elements to set
* @return modified copy of {@code this} object
*/
public final ImmutableModuleConfiguration withProtoFiles(Iterable elements) {
if (this.protoFiles == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableModuleConfiguration(this.name, this.includePaths, newValue, this.generator, this.output, this.options);
}
/**
* Copy current immutable object by setting value for {@link ModuleConfiguration#getGenerator() generator}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for generator
* @return modified copy of the {@code this} object
*/
public final ImmutableModuleConfiguration withGenerator(String value) {
if (this.generator == value) return this;
String newValue = Preconditions.checkNotNull(value);
return new ImmutableModuleConfiguration(this.name, this.includePaths, this.protoFiles, newValue, this.output, this.options);
}
/**
* Copy current immutable object by setting value for {@link ModuleConfiguration#getOutput() output}.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value new value for output
* @return modified copy of the {@code this} object
*/
public final ImmutableModuleConfiguration withOutput(String value) {
if (this.output == value) return this;
String newValue = Preconditions.checkNotNull(value);
return new ImmutableModuleConfiguration(this.name, this.includePaths, this.protoFiles, this.generator, newValue, this.options);
}
/**
* Copy current immutable object by replacing {@link ModuleConfiguration#getOptions() options} map with specified map.
* Nulls are not permitted as keys or values.
* Shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries to be added to options map
* @return modified copy of {@code this} object
*/
public final ImmutableModuleConfiguration withOptions(Map entries) {
if (this.options == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableModuleConfiguration(this.name, this.includePaths, this.protoFiles, this.generator, this.output, newValue);
}
/**
* This instance is equal to instances of {@code ImmutableModuleConfiguration} with equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableModuleConfiguration
&& equalTo((ImmutableModuleConfiguration) another);
}
private boolean equalTo(ImmutableModuleConfiguration another) {
return name.equals(another.name)
&& includePaths.equals(another.includePaths)
&& protoFiles.equals(another.protoFiles)
&& generator.equals(another.generator)
&& output.equals(another.output)
&& options.equals(another.options);
}
/**
* Computes hash code from attributes: {@code name}, {@code includePaths}, {@code protoFiles}, {@code generator}, {@code output}, {@code options}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + name.hashCode();
h = h * 17 + includePaths.hashCode();
h = h * 17 + protoFiles.hashCode();
h = h * 17 + generator.hashCode();
h = h * 17 + output.hashCode();
h = h * 17 + options.hashCode();
return h;
}
/**
* Prints immutable value {@code ModuleConfiguration...} with attribute values,
* excluding any non-generated and auxiliary attributes.
* @return string representation of value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ModuleConfiguration")
.add("name", name)
.add("includePaths", includePaths)
.add("protoFiles", protoFiles)
.add("generator", generator)
.add("output", output)
.add("options", options)
.toString();
}
/**
* Creates immutable copy of {@link ModuleConfiguration}.
* Uses accessors to get values to initialize immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance instance to copy
* @return copied immutable ModuleConfiguration instance
*/
public static ImmutableModuleConfiguration copyOf(ModuleConfiguration instance) {
if (instance instanceof ImmutableModuleConfiguration) {
return (ImmutableModuleConfiguration) instance;
}
return ImmutableModuleConfiguration.builder()
.from(instance)
.build();
}
/**
* Creates builder for {@link io.protostuff.compiler.model.ImmutableModuleConfiguration ImmutableModuleConfiguration}.
* @return new ImmutableModuleConfiguration builder
*/
public static ImmutableModuleConfiguration.Builder builder() {
return new ImmutableModuleConfiguration.Builder();
}
/**
* Builds instances of {@link io.protostuff.compiler.model.ImmutableModuleConfiguration ImmutableModuleConfiguration}.
* Initialize attributes and then invoke {@link #build()} method to create
* immutable instance.
* {@code Builder} is not thread safe and generally should not be stored in field or collection,
* but used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_GENERATOR = 0x2L;
private static final long INIT_BIT_OUTPUT = 0x4L;
private long initBits = 0x7;
private @Nullable String name;
private ImmutableList.Builder includePathsBuilder = ImmutableList.builder();
private ImmutableList.Builder protoFilesBuilder = ImmutableList.builder();
private @Nullable String generator;
private @Nullable String output;
private ImmutableMap.Builder optionsBuilder = ImmutableMap.builder();
private Builder() {}
/**
* Fill builder with attribute values from provided {@link ModuleConfiguration} instance.
* Regular attribute values will be replaced with ones of an instance.
* Instance's absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance instance to copy values from
* @return {@code this} builder for chained invocation
*/
public final Builder from(ModuleConfiguration instance) {
Preconditions.checkNotNull(instance);
name(instance.getName());
addAllIncludePaths(instance.getIncludePaths());
addAllProtoFiles(instance.getProtoFiles());
generator(instance.getGenerator());
output(instance.getOutput());
putAllOptions(instance.getOptions());
return this;
}
/**
* Initializes value for {@link ModuleConfiguration#getName() name}.
* @param name value for name
* @return {@code this} builder for chained invocation
*/
public final Builder name(String name) {
this.name = Preconditions.checkNotNull(name);
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Adds one element to {@link ModuleConfiguration#getIncludePaths() includePaths} list.
* @param element includePaths element
* @return {@code this} builder for chained invocation
*/
public final Builder addIncludePaths(Path element) {
includePathsBuilder.add(element);
return this;
}
/**
* Adds elements to {@link ModuleConfiguration#getIncludePaths() includePaths} list.
* @param elements array of includePaths elements
* @return {@code this} builder for chained invocation
*/
public final Builder addIncludePaths(Path... elements) {
includePathsBuilder.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link ModuleConfiguration#getIncludePaths() includePaths} list.
* @param elements iterable of includePaths elements
* @return {@code this} builder for chained invocation
*/
public final Builder includePaths(Iterable extends Path> elements) {
includePathsBuilder = ImmutableList.builder();
return addAllIncludePaths(elements);
}
/**
* Adds elements to {@link ModuleConfiguration#getIncludePaths() includePaths} list.
* @param elements iterable of includePaths elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllIncludePaths(Iterable extends Path> elements) {
includePathsBuilder.addAll(elements);
return this;
}
/**
* Adds one element to {@link ModuleConfiguration#getProtoFiles() protoFiles} list.
* @param element protoFiles element
* @return {@code this} builder for chained invocation
*/
public final Builder addProtoFiles(String element) {
protoFilesBuilder.add(element);
return this;
}
/**
* Adds elements to {@link ModuleConfiguration#getProtoFiles() protoFiles} list.
* @param elements array of protoFiles elements
* @return {@code this} builder for chained invocation
*/
public final Builder addProtoFiles(String... elements) {
protoFilesBuilder.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link ModuleConfiguration#getProtoFiles() protoFiles} list.
* @param elements iterable of protoFiles elements
* @return {@code this} builder for chained invocation
*/
public final Builder protoFiles(Iterable elements) {
protoFilesBuilder = ImmutableList.builder();
return addAllProtoFiles(elements);
}
/**
* Adds elements to {@link ModuleConfiguration#getProtoFiles() protoFiles} list.
* @param elements iterable of protoFiles elements
* @return {@code this} builder for chained invocation
*/
public final Builder addAllProtoFiles(Iterable elements) {
protoFilesBuilder.addAll(elements);
return this;
}
/**
* Initializes value for {@link ModuleConfiguration#getGenerator() generator}.
* @param generator value for generator
* @return {@code this} builder for chained invocation
*/
public final Builder generator(String generator) {
this.generator = Preconditions.checkNotNull(generator);
initBits &= ~INIT_BIT_GENERATOR;
return this;
}
/**
* Initializes value for {@link ModuleConfiguration#getOutput() output}.
* @param output value for output
* @return {@code this} builder for chained invocation
*/
public final Builder output(String output) {
this.output = Preconditions.checkNotNull(output);
initBits &= ~INIT_BIT_OUTPUT;
return this;
}
/**
* Put one entry to {@link ModuleConfiguration#getOptions() options} map.
* @param key the key in options map
* @param value the associated value in options map
* @return {@code this} builder for chained invocation
*/
public final Builder putOptions(String key, java.lang.Object value) {
optionsBuilder.put(key, value);
return this;
}
/**
* Put one entry to {@link ModuleConfiguration#getOptions() options} map. Nulls are not permitted
* @param entry the key and value entry
* @return {@code this} builder for chained invocation
*/
public final Builder putOptions(Map.Entry entry) {
optionsBuilder.put(entry);
return this;
}
/**
* Sets or replaces all mappings from specified map as entries for {@link ModuleConfiguration#getOptions() options} map. Nulls are not permitted
* @param entries to be added to options map
* @return {@code this} builder for chained invocation
*/
public final Builder options(Map entries) {
optionsBuilder = ImmutableMap.builder();
return putAllOptions(entries);
}
/**
* Put all mappings from specified map as entries to {@link ModuleConfiguration#getOptions() options} map. Nulls are not permitted
* @param entries to be added to options map
* @return {@code this} builder for chained invocation
*/
public final Builder putAllOptions(Map entries) {
optionsBuilder.putAll(entries);
return this;
}
/**
* Builds new {@link io.protostuff.compiler.model.ImmutableModuleConfiguration ImmutableModuleConfiguration}.
* @return immutable instance of ModuleConfiguration
* @throws exception {@code java.lang.IllegalStateException} if any required attributes are missing
*/
public ImmutableModuleConfiguration build()
throws IllegalStateException {
checkRequiredAttributes();
return new ImmutableModuleConfiguration(
name,
includePathsBuilder.build(),
protoFilesBuilder.build(),
generator,
output,
optionsBuilder.build());
}
private boolean nameIsSet() {
return (initBits & INIT_BIT_NAME) == 0;
}
private boolean generatorIsSet() {
return (initBits & INIT_BIT_GENERATOR) == 0;
}
private boolean outputIsSet() {
return (initBits & INIT_BIT_OUTPUT) == 0;
}
private void checkRequiredAttributes() throws IllegalStateException {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if (!nameIsSet()) attributes.add("name");
if (!generatorIsSet()) attributes.add("generator");
if (!outputIsSet()) attributes.add("output");
return "Cannot build ModuleConfiguration, some of required attributes are not set " + attributes;
}
}
}