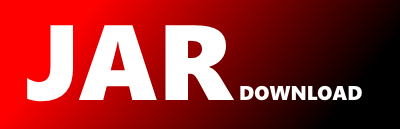
cosmos.distribution.v1beta1.Distribution Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: cosmos/distribution/v1beta1/distribution.proto
package cosmos.distribution.v1beta1;
public final class Distribution {
private Distribution() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ParamsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.Params)
com.google.protobuf.MessageOrBuilder {
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
java.lang.String getCommunityTax();
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
com.google.protobuf.ByteString
getCommunityTaxBytes();
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
java.lang.String getBaseProposerReward();
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
com.google.protobuf.ByteString
getBaseProposerRewardBytes();
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
java.lang.String getBonusProposerReward();
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
com.google.protobuf.ByteString
getBonusProposerRewardBytes();
/**
* bool withdraw_addr_enabled = 4 [(.gogoproto.moretags) = "yaml:\"withdraw_addr_enabled\""];
*/
boolean getWithdrawAddrEnabled();
}
/**
*
* Params defines the set of params for the distribution module.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.Params}
*/
public static final class Params extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.Params)
ParamsOrBuilder {
private static final long serialVersionUID = 0L;
// Use Params.newBuilder() to construct.
private Params(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Params() {
communityTax_ = "";
baseProposerReward_ = "";
bonusProposerReward_ = "";
withdrawAddrEnabled_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Params(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
communityTax_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
baseProposerReward_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
bonusProposerReward_ = s;
break;
}
case 32: {
withdrawAddrEnabled_ = input.readBool();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_Params_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_Params_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.Params.class, cosmos.distribution.v1beta1.Distribution.Params.Builder.class);
}
public static final int COMMUNITY_TAX_FIELD_NUMBER = 1;
private volatile java.lang.Object communityTax_;
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public java.lang.String getCommunityTax() {
java.lang.Object ref = communityTax_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
communityTax_ = s;
return s;
}
}
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public com.google.protobuf.ByteString
getCommunityTaxBytes() {
java.lang.Object ref = communityTax_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
communityTax_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BASE_PROPOSER_REWARD_FIELD_NUMBER = 2;
private volatile java.lang.Object baseProposerReward_;
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public java.lang.String getBaseProposerReward() {
java.lang.Object ref = baseProposerReward_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
baseProposerReward_ = s;
return s;
}
}
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public com.google.protobuf.ByteString
getBaseProposerRewardBytes() {
java.lang.Object ref = baseProposerReward_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
baseProposerReward_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BONUS_PROPOSER_REWARD_FIELD_NUMBER = 3;
private volatile java.lang.Object bonusProposerReward_;
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public java.lang.String getBonusProposerReward() {
java.lang.Object ref = bonusProposerReward_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bonusProposerReward_ = s;
return s;
}
}
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public com.google.protobuf.ByteString
getBonusProposerRewardBytes() {
java.lang.Object ref = bonusProposerReward_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bonusProposerReward_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WITHDRAW_ADDR_ENABLED_FIELD_NUMBER = 4;
private boolean withdrawAddrEnabled_;
/**
* bool withdraw_addr_enabled = 4 [(.gogoproto.moretags) = "yaml:\"withdraw_addr_enabled\""];
*/
public boolean getWithdrawAddrEnabled() {
return withdrawAddrEnabled_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCommunityTaxBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, communityTax_);
}
if (!getBaseProposerRewardBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, baseProposerReward_);
}
if (!getBonusProposerRewardBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, bonusProposerReward_);
}
if (withdrawAddrEnabled_ != false) {
output.writeBool(4, withdrawAddrEnabled_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCommunityTaxBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, communityTax_);
}
if (!getBaseProposerRewardBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, baseProposerReward_);
}
if (!getBonusProposerRewardBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, bonusProposerReward_);
}
if (withdrawAddrEnabled_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, withdrawAddrEnabled_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.Params)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.Params other = (cosmos.distribution.v1beta1.Distribution.Params) obj;
boolean result = true;
result = result && getCommunityTax()
.equals(other.getCommunityTax());
result = result && getBaseProposerReward()
.equals(other.getBaseProposerReward());
result = result && getBonusProposerReward()
.equals(other.getBonusProposerReward());
result = result && (getWithdrawAddrEnabled()
== other.getWithdrawAddrEnabled());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COMMUNITY_TAX_FIELD_NUMBER;
hash = (53 * hash) + getCommunityTax().hashCode();
hash = (37 * hash) + BASE_PROPOSER_REWARD_FIELD_NUMBER;
hash = (53 * hash) + getBaseProposerReward().hashCode();
hash = (37 * hash) + BONUS_PROPOSER_REWARD_FIELD_NUMBER;
hash = (53 * hash) + getBonusProposerReward().hashCode();
hash = (37 * hash) + WITHDRAW_ADDR_ENABLED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWithdrawAddrEnabled());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.Params parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.Params prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Params defines the set of params for the distribution module.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.Params}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.Params)
cosmos.distribution.v1beta1.Distribution.ParamsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_Params_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_Params_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.Params.class, cosmos.distribution.v1beta1.Distribution.Params.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.Params.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
communityTax_ = "";
baseProposerReward_ = "";
bonusProposerReward_ = "";
withdrawAddrEnabled_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_Params_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.Params getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.Params.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.Params build() {
cosmos.distribution.v1beta1.Distribution.Params result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.Params buildPartial() {
cosmos.distribution.v1beta1.Distribution.Params result = new cosmos.distribution.v1beta1.Distribution.Params(this);
result.communityTax_ = communityTax_;
result.baseProposerReward_ = baseProposerReward_;
result.bonusProposerReward_ = bonusProposerReward_;
result.withdrawAddrEnabled_ = withdrawAddrEnabled_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.Params) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.Params)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.Params other) {
if (other == cosmos.distribution.v1beta1.Distribution.Params.getDefaultInstance()) return this;
if (!other.getCommunityTax().isEmpty()) {
communityTax_ = other.communityTax_;
onChanged();
}
if (!other.getBaseProposerReward().isEmpty()) {
baseProposerReward_ = other.baseProposerReward_;
onChanged();
}
if (!other.getBonusProposerReward().isEmpty()) {
bonusProposerReward_ = other.bonusProposerReward_;
onChanged();
}
if (other.getWithdrawAddrEnabled() != false) {
setWithdrawAddrEnabled(other.getWithdrawAddrEnabled());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.Params parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.Params) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object communityTax_ = "";
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public java.lang.String getCommunityTax() {
java.lang.Object ref = communityTax_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
communityTax_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public com.google.protobuf.ByteString
getCommunityTaxBytes() {
java.lang.Object ref = communityTax_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
communityTax_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public Builder setCommunityTax(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
communityTax_ = value;
onChanged();
return this;
}
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public Builder clearCommunityTax() {
communityTax_ = getDefaultInstance().getCommunityTax();
onChanged();
return this;
}
/**
* string community_tax = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"community_tax\""];
*/
public Builder setCommunityTaxBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
communityTax_ = value;
onChanged();
return this;
}
private java.lang.Object baseProposerReward_ = "";
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public java.lang.String getBaseProposerReward() {
java.lang.Object ref = baseProposerReward_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
baseProposerReward_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public com.google.protobuf.ByteString
getBaseProposerRewardBytes() {
java.lang.Object ref = baseProposerReward_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
baseProposerReward_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public Builder setBaseProposerReward(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
baseProposerReward_ = value;
onChanged();
return this;
}
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public Builder clearBaseProposerReward() {
baseProposerReward_ = getDefaultInstance().getBaseProposerReward();
onChanged();
return this;
}
/**
* string base_proposer_reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"base_proposer_reward\""];
*/
public Builder setBaseProposerRewardBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
baseProposerReward_ = value;
onChanged();
return this;
}
private java.lang.Object bonusProposerReward_ = "";
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public java.lang.String getBonusProposerReward() {
java.lang.Object ref = bonusProposerReward_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bonusProposerReward_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public com.google.protobuf.ByteString
getBonusProposerRewardBytes() {
java.lang.Object ref = bonusProposerReward_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bonusProposerReward_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public Builder setBonusProposerReward(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bonusProposerReward_ = value;
onChanged();
return this;
}
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public Builder clearBonusProposerReward() {
bonusProposerReward_ = getDefaultInstance().getBonusProposerReward();
onChanged();
return this;
}
/**
* string bonus_proposer_reward = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"bonus_proposer_reward\""];
*/
public Builder setBonusProposerRewardBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bonusProposerReward_ = value;
onChanged();
return this;
}
private boolean withdrawAddrEnabled_ ;
/**
* bool withdraw_addr_enabled = 4 [(.gogoproto.moretags) = "yaml:\"withdraw_addr_enabled\""];
*/
public boolean getWithdrawAddrEnabled() {
return withdrawAddrEnabled_;
}
/**
* bool withdraw_addr_enabled = 4 [(.gogoproto.moretags) = "yaml:\"withdraw_addr_enabled\""];
*/
public Builder setWithdrawAddrEnabled(boolean value) {
withdrawAddrEnabled_ = value;
onChanged();
return this;
}
/**
* bool withdraw_addr_enabled = 4 [(.gogoproto.moretags) = "yaml:\"withdraw_addr_enabled\""];
*/
public Builder clearWithdrawAddrEnabled() {
withdrawAddrEnabled_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.Params)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.Params)
private static final cosmos.distribution.v1beta1.Distribution.Params DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.Params();
}
public static cosmos.distribution.v1beta1.Distribution.Params getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Params parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Params(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.Params getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorHistoricalRewardsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.ValidatorHistoricalRewards)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List
getCumulativeRewardRatioList();
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoin getCumulativeRewardRatio(int index);
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
int getCumulativeRewardRatioCount();
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCumulativeRewardRatioOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCumulativeRewardRatioOrBuilder(
int index);
/**
* uint32 reference_count = 2 [(.gogoproto.moretags) = "yaml:\"reference_count\""];
*/
int getReferenceCount();
}
/**
*
* ValidatorHistoricalRewards represents historical rewards for a validator.
* Height is implicit within the store key.
* Cumulative reward ratio is the sum from the zeroeth period
* until this period of rewards / tokens, per the spec.
* The reference count indicates the number of objects
* which might need to reference this historical entry at any point.
* ReferenceCount =
* number of outstanding delegations which ended the associated period (and
* might need to read that record)
* + number of slashes which ended the associated period (and might need to
* read that record)
* + one per validator for the zeroeth period, set on initialization
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorHistoricalRewards}
*/
public static final class ValidatorHistoricalRewards extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.ValidatorHistoricalRewards)
ValidatorHistoricalRewardsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorHistoricalRewards.newBuilder() to construct.
private ValidatorHistoricalRewards(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorHistoricalRewards() {
cumulativeRewardRatio_ = java.util.Collections.emptyList();
referenceCount_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorHistoricalRewards(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cumulativeRewardRatio_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
cumulativeRewardRatio_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.DecCoin.parser(), extensionRegistry));
break;
}
case 16: {
referenceCount_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
cumulativeRewardRatio_ = java.util.Collections.unmodifiableList(cumulativeRewardRatio_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.class, cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.Builder.class);
}
private int bitField0_;
public static final int CUMULATIVE_REWARD_RATIO_FIELD_NUMBER = 1;
private java.util.List cumulativeRewardRatio_;
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getCumulativeRewardRatioList() {
return cumulativeRewardRatio_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCumulativeRewardRatioOrBuilderList() {
return cumulativeRewardRatio_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getCumulativeRewardRatioCount() {
return cumulativeRewardRatio_.size();
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getCumulativeRewardRatio(int index) {
return cumulativeRewardRatio_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCumulativeRewardRatioOrBuilder(
int index) {
return cumulativeRewardRatio_.get(index);
}
public static final int REFERENCE_COUNT_FIELD_NUMBER = 2;
private int referenceCount_;
/**
* uint32 reference_count = 2 [(.gogoproto.moretags) = "yaml:\"reference_count\""];
*/
public int getReferenceCount() {
return referenceCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < cumulativeRewardRatio_.size(); i++) {
output.writeMessage(1, cumulativeRewardRatio_.get(i));
}
if (referenceCount_ != 0) {
output.writeUInt32(2, referenceCount_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < cumulativeRewardRatio_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, cumulativeRewardRatio_.get(i));
}
if (referenceCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, referenceCount_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards other = (cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards) obj;
boolean result = true;
result = result && getCumulativeRewardRatioList()
.equals(other.getCumulativeRewardRatioList());
result = result && (getReferenceCount()
== other.getReferenceCount());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCumulativeRewardRatioCount() > 0) {
hash = (37 * hash) + CUMULATIVE_REWARD_RATIO_FIELD_NUMBER;
hash = (53 * hash) + getCumulativeRewardRatioList().hashCode();
}
hash = (37 * hash) + REFERENCE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getReferenceCount();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorHistoricalRewards represents historical rewards for a validator.
* Height is implicit within the store key.
* Cumulative reward ratio is the sum from the zeroeth period
* until this period of rewards / tokens, per the spec.
* The reference count indicates the number of objects
* which might need to reference this historical entry at any point.
* ReferenceCount =
* number of outstanding delegations which ended the associated period (and
* might need to read that record)
* + number of slashes which ended the associated period (and might need to
* read that record)
* + one per validator for the zeroeth period, set on initialization
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorHistoricalRewards}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.ValidatorHistoricalRewards)
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewardsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.class, cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCumulativeRewardRatioFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (cumulativeRewardRatioBuilder_ == null) {
cumulativeRewardRatio_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
cumulativeRewardRatioBuilder_.clear();
}
referenceCount_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards build() {
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards buildPartial() {
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards result = new cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (cumulativeRewardRatioBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
cumulativeRewardRatio_ = java.util.Collections.unmodifiableList(cumulativeRewardRatio_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.cumulativeRewardRatio_ = cumulativeRewardRatio_;
} else {
result.cumulativeRewardRatio_ = cumulativeRewardRatioBuilder_.build();
}
result.referenceCount_ = referenceCount_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards other) {
if (other == cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards.getDefaultInstance()) return this;
if (cumulativeRewardRatioBuilder_ == null) {
if (!other.cumulativeRewardRatio_.isEmpty()) {
if (cumulativeRewardRatio_.isEmpty()) {
cumulativeRewardRatio_ = other.cumulativeRewardRatio_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.addAll(other.cumulativeRewardRatio_);
}
onChanged();
}
} else {
if (!other.cumulativeRewardRatio_.isEmpty()) {
if (cumulativeRewardRatioBuilder_.isEmpty()) {
cumulativeRewardRatioBuilder_.dispose();
cumulativeRewardRatioBuilder_ = null;
cumulativeRewardRatio_ = other.cumulativeRewardRatio_;
bitField0_ = (bitField0_ & ~0x00000001);
cumulativeRewardRatioBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCumulativeRewardRatioFieldBuilder() : null;
} else {
cumulativeRewardRatioBuilder_.addAllMessages(other.cumulativeRewardRatio_);
}
}
}
if (other.getReferenceCount() != 0) {
setReferenceCount(other.getReferenceCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List cumulativeRewardRatio_ =
java.util.Collections.emptyList();
private void ensureCumulativeRewardRatioIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
cumulativeRewardRatio_ = new java.util.ArrayList(cumulativeRewardRatio_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder> cumulativeRewardRatioBuilder_;
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getCumulativeRewardRatioList() {
if (cumulativeRewardRatioBuilder_ == null) {
return java.util.Collections.unmodifiableList(cumulativeRewardRatio_);
} else {
return cumulativeRewardRatioBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getCumulativeRewardRatioCount() {
if (cumulativeRewardRatioBuilder_ == null) {
return cumulativeRewardRatio_.size();
} else {
return cumulativeRewardRatioBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getCumulativeRewardRatio(int index) {
if (cumulativeRewardRatioBuilder_ == null) {
return cumulativeRewardRatio_.get(index);
} else {
return cumulativeRewardRatioBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setCumulativeRewardRatio(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (cumulativeRewardRatioBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.set(index, value);
onChanged();
} else {
cumulativeRewardRatioBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setCumulativeRewardRatio(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (cumulativeRewardRatioBuilder_ == null) {
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.set(index, builderForValue.build());
onChanged();
} else {
cumulativeRewardRatioBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCumulativeRewardRatio(cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (cumulativeRewardRatioBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.add(value);
onChanged();
} else {
cumulativeRewardRatioBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCumulativeRewardRatio(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (cumulativeRewardRatioBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.add(index, value);
onChanged();
} else {
cumulativeRewardRatioBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCumulativeRewardRatio(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (cumulativeRewardRatioBuilder_ == null) {
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.add(builderForValue.build());
onChanged();
} else {
cumulativeRewardRatioBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCumulativeRewardRatio(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (cumulativeRewardRatioBuilder_ == null) {
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.add(index, builderForValue.build());
onChanged();
} else {
cumulativeRewardRatioBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addAllCumulativeRewardRatio(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.DecCoin> values) {
if (cumulativeRewardRatioBuilder_ == null) {
ensureCumulativeRewardRatioIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cumulativeRewardRatio_);
onChanged();
} else {
cumulativeRewardRatioBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder clearCumulativeRewardRatio() {
if (cumulativeRewardRatioBuilder_ == null) {
cumulativeRewardRatio_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
cumulativeRewardRatioBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder removeCumulativeRewardRatio(int index) {
if (cumulativeRewardRatioBuilder_ == null) {
ensureCumulativeRewardRatioIsMutable();
cumulativeRewardRatio_.remove(index);
onChanged();
} else {
cumulativeRewardRatioBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder getCumulativeRewardRatioBuilder(
int index) {
return getCumulativeRewardRatioFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCumulativeRewardRatioOrBuilder(
int index) {
if (cumulativeRewardRatioBuilder_ == null) {
return cumulativeRewardRatio_.get(index); } else {
return cumulativeRewardRatioBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCumulativeRewardRatioOrBuilderList() {
if (cumulativeRewardRatioBuilder_ != null) {
return cumulativeRewardRatioBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(cumulativeRewardRatio_);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addCumulativeRewardRatioBuilder() {
return getCumulativeRewardRatioFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addCumulativeRewardRatioBuilder(
int index) {
return getCumulativeRewardRatioFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin cumulative_reward_ratio = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"cumulative_reward_ratio\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List
getCumulativeRewardRatioBuilderList() {
return getCumulativeRewardRatioFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCumulativeRewardRatioFieldBuilder() {
if (cumulativeRewardRatioBuilder_ == null) {
cumulativeRewardRatioBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>(
cumulativeRewardRatio_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
cumulativeRewardRatio_ = null;
}
return cumulativeRewardRatioBuilder_;
}
private int referenceCount_ ;
/**
* uint32 reference_count = 2 [(.gogoproto.moretags) = "yaml:\"reference_count\""];
*/
public int getReferenceCount() {
return referenceCount_;
}
/**
* uint32 reference_count = 2 [(.gogoproto.moretags) = "yaml:\"reference_count\""];
*/
public Builder setReferenceCount(int value) {
referenceCount_ = value;
onChanged();
return this;
}
/**
* uint32 reference_count = 2 [(.gogoproto.moretags) = "yaml:\"reference_count\""];
*/
public Builder clearReferenceCount() {
referenceCount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.ValidatorHistoricalRewards)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.ValidatorHistoricalRewards)
private static final cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards();
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorHistoricalRewards parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorHistoricalRewards(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorHistoricalRewards getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorCurrentRewardsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.ValidatorCurrentRewards)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List
getRewardsList();
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoin getRewards(int index);
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
int getRewardsCount();
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardsOrBuilder(
int index);
/**
* uint64 period = 2;
*/
long getPeriod();
}
/**
*
* ValidatorCurrentRewards represents current rewards and current
* period for a validator kept as a running counter and incremented
* each block as long as the validator's tokens remain constant.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorCurrentRewards}
*/
public static final class ValidatorCurrentRewards extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.ValidatorCurrentRewards)
ValidatorCurrentRewardsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorCurrentRewards.newBuilder() to construct.
private ValidatorCurrentRewards(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorCurrentRewards() {
rewards_ = java.util.Collections.emptyList();
period_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorCurrentRewards(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
rewards_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.DecCoin.parser(), extensionRegistry));
break;
}
case 16: {
period_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = java.util.Collections.unmodifiableList(rewards_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.class, cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.Builder.class);
}
private int bitField0_;
public static final int REWARDS_FIELD_NUMBER = 1;
private java.util.List rewards_;
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getRewardsList() {
return rewards_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsOrBuilderList() {
return rewards_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getRewardsCount() {
return rewards_.size();
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getRewards(int index) {
return rewards_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardsOrBuilder(
int index) {
return rewards_.get(index);
}
public static final int PERIOD_FIELD_NUMBER = 2;
private long period_;
/**
* uint64 period = 2;
*/
public long getPeriod() {
return period_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < rewards_.size(); i++) {
output.writeMessage(1, rewards_.get(i));
}
if (period_ != 0L) {
output.writeUInt64(2, period_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < rewards_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, rewards_.get(i));
}
if (period_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, period_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards other = (cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards) obj;
boolean result = true;
result = result && getRewardsList()
.equals(other.getRewardsList());
result = result && (getPeriod()
== other.getPeriod());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getRewardsCount() > 0) {
hash = (37 * hash) + REWARDS_FIELD_NUMBER;
hash = (53 * hash) + getRewardsList().hashCode();
}
hash = (37 * hash) + PERIOD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPeriod());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorCurrentRewards represents current rewards and current
* period for a validator kept as a running counter and incremented
* each block as long as the validator's tokens remain constant.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorCurrentRewards}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.ValidatorCurrentRewards)
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewardsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.class, cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRewardsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (rewardsBuilder_ == null) {
rewards_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
rewardsBuilder_.clear();
}
period_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards build() {
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards buildPartial() {
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards result = new cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (rewardsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = java.util.Collections.unmodifiableList(rewards_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.rewards_ = rewards_;
} else {
result.rewards_ = rewardsBuilder_.build();
}
result.period_ = period_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards other) {
if (other == cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards.getDefaultInstance()) return this;
if (rewardsBuilder_ == null) {
if (!other.rewards_.isEmpty()) {
if (rewards_.isEmpty()) {
rewards_ = other.rewards_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRewardsIsMutable();
rewards_.addAll(other.rewards_);
}
onChanged();
}
} else {
if (!other.rewards_.isEmpty()) {
if (rewardsBuilder_.isEmpty()) {
rewardsBuilder_.dispose();
rewardsBuilder_ = null;
rewards_ = other.rewards_;
bitField0_ = (bitField0_ & ~0x00000001);
rewardsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRewardsFieldBuilder() : null;
} else {
rewardsBuilder_.addAllMessages(other.rewards_);
}
}
}
if (other.getPeriod() != 0L) {
setPeriod(other.getPeriod());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List rewards_ =
java.util.Collections.emptyList();
private void ensureRewardsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = new java.util.ArrayList(rewards_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder> rewardsBuilder_;
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getRewardsList() {
if (rewardsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rewards_);
} else {
return rewardsBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getRewardsCount() {
if (rewardsBuilder_ == null) {
return rewards_.size();
} else {
return rewardsBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getRewards(int index) {
if (rewardsBuilder_ == null) {
return rewards_.get(index);
} else {
return rewardsBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardsIsMutable();
rewards_.set(index, value);
onChanged();
} else {
rewardsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.set(index, builderForValue.build());
onChanged();
} else {
rewardsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardsIsMutable();
rewards_.add(value);
onChanged();
} else {
rewardsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardsIsMutable();
rewards_.add(index, value);
onChanged();
} else {
rewardsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.add(builderForValue.build());
onChanged();
} else {
rewardsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.add(index, builderForValue.build());
onChanged();
} else {
rewardsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addAllRewards(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.DecCoin> values) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rewards_);
onChanged();
} else {
rewardsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder clearRewards() {
if (rewardsBuilder_ == null) {
rewards_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
rewardsBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder removeRewards(int index) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.remove(index);
onChanged();
} else {
rewardsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder getRewardsBuilder(
int index) {
return getRewardsFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardsOrBuilder(
int index) {
if (rewardsBuilder_ == null) {
return rewards_.get(index); } else {
return rewardsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsOrBuilderList() {
if (rewardsBuilder_ != null) {
return rewardsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rewards_);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addRewardsBuilder() {
return getRewardsFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addRewardsBuilder(
int index) {
return getRewardsFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List
getRewardsBuilderList() {
return getRewardsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsFieldBuilder() {
if (rewardsBuilder_ == null) {
rewardsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>(
rewards_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
rewards_ = null;
}
return rewardsBuilder_;
}
private long period_ ;
/**
* uint64 period = 2;
*/
public long getPeriod() {
return period_;
}
/**
* uint64 period = 2;
*/
public Builder setPeriod(long value) {
period_ = value;
onChanged();
return this;
}
/**
* uint64 period = 2;
*/
public Builder clearPeriod() {
period_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.ValidatorCurrentRewards)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.ValidatorCurrentRewards)
private static final cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards();
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorCurrentRewards parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorCurrentRewards(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorCurrentRewards getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorAccumulatedCommissionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.ValidatorAccumulatedCommission)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List
getCommissionList();
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoin getCommission(int index);
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
int getCommissionCount();
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommissionOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCommissionOrBuilder(
int index);
}
/**
*
* ValidatorAccumulatedCommission represents accumulated commission
* for a validator kept as a running counter, can be withdrawn at any time.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorAccumulatedCommission}
*/
public static final class ValidatorAccumulatedCommission extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.ValidatorAccumulatedCommission)
ValidatorAccumulatedCommissionOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorAccumulatedCommission.newBuilder() to construct.
private ValidatorAccumulatedCommission(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorAccumulatedCommission() {
commission_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorAccumulatedCommission(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
commission_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
commission_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.DecCoin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
commission_ = java.util.Collections.unmodifiableList(commission_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.class, cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.Builder.class);
}
public static final int COMMISSION_FIELD_NUMBER = 1;
private java.util.List commission_;
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getCommissionList() {
return commission_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommissionOrBuilderList() {
return commission_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getCommissionCount() {
return commission_.size();
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getCommission(int index) {
return commission_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCommissionOrBuilder(
int index) {
return commission_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < commission_.size(); i++) {
output.writeMessage(1, commission_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < commission_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, commission_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission other = (cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission) obj;
boolean result = true;
result = result && getCommissionList()
.equals(other.getCommissionList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCommissionCount() > 0) {
hash = (37 * hash) + COMMISSION_FIELD_NUMBER;
hash = (53 * hash) + getCommissionList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorAccumulatedCommission represents accumulated commission
* for a validator kept as a running counter, can be withdrawn at any time.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorAccumulatedCommission}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.ValidatorAccumulatedCommission)
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommissionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.class, cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommissionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (commissionBuilder_ == null) {
commission_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
commissionBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission build() {
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission buildPartial() {
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission result = new cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission(this);
int from_bitField0_ = bitField0_;
if (commissionBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
commission_ = java.util.Collections.unmodifiableList(commission_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.commission_ = commission_;
} else {
result.commission_ = commissionBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission other) {
if (other == cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission.getDefaultInstance()) return this;
if (commissionBuilder_ == null) {
if (!other.commission_.isEmpty()) {
if (commission_.isEmpty()) {
commission_ = other.commission_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCommissionIsMutable();
commission_.addAll(other.commission_);
}
onChanged();
}
} else {
if (!other.commission_.isEmpty()) {
if (commissionBuilder_.isEmpty()) {
commissionBuilder_.dispose();
commissionBuilder_ = null;
commission_ = other.commission_;
bitField0_ = (bitField0_ & ~0x00000001);
commissionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCommissionFieldBuilder() : null;
} else {
commissionBuilder_.addAllMessages(other.commission_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List commission_ =
java.util.Collections.emptyList();
private void ensureCommissionIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
commission_ = new java.util.ArrayList(commission_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder> commissionBuilder_;
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getCommissionList() {
if (commissionBuilder_ == null) {
return java.util.Collections.unmodifiableList(commission_);
} else {
return commissionBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getCommissionCount() {
if (commissionBuilder_ == null) {
return commission_.size();
} else {
return commissionBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getCommission(int index) {
if (commissionBuilder_ == null) {
return commission_.get(index);
} else {
return commissionBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setCommission(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (commissionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommissionIsMutable();
commission_.set(index, value);
onChanged();
} else {
commissionBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setCommission(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (commissionBuilder_ == null) {
ensureCommissionIsMutable();
commission_.set(index, builderForValue.build());
onChanged();
} else {
commissionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommission(cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (commissionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommissionIsMutable();
commission_.add(value);
onChanged();
} else {
commissionBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommission(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (commissionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommissionIsMutable();
commission_.add(index, value);
onChanged();
} else {
commissionBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommission(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (commissionBuilder_ == null) {
ensureCommissionIsMutable();
commission_.add(builderForValue.build());
onChanged();
} else {
commissionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommission(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (commissionBuilder_ == null) {
ensureCommissionIsMutable();
commission_.add(index, builderForValue.build());
onChanged();
} else {
commissionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addAllCommission(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.DecCoin> values) {
if (commissionBuilder_ == null) {
ensureCommissionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, commission_);
onChanged();
} else {
commissionBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder clearCommission() {
if (commissionBuilder_ == null) {
commission_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
commissionBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder removeCommission(int index) {
if (commissionBuilder_ == null) {
ensureCommissionIsMutable();
commission_.remove(index);
onChanged();
} else {
commissionBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder getCommissionBuilder(
int index) {
return getCommissionFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCommissionOrBuilder(
int index) {
if (commissionBuilder_ == null) {
return commission_.get(index); } else {
return commissionBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommissionOrBuilderList() {
if (commissionBuilder_ != null) {
return commissionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(commission_);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addCommissionBuilder() {
return getCommissionFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addCommissionBuilder(
int index) {
return getCommissionFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin commission = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List
getCommissionBuilderList() {
return getCommissionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommissionFieldBuilder() {
if (commissionBuilder_ == null) {
commissionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>(
commission_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
commission_ = null;
}
return commissionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.ValidatorAccumulatedCommission)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.ValidatorAccumulatedCommission)
private static final cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission();
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorAccumulatedCommission parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorAccumulatedCommission(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorAccumulatedCommission getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorOutstandingRewardsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.ValidatorOutstandingRewards)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List
getRewardsList();
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoin getRewards(int index);
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
int getRewardsCount();
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardsOrBuilder(
int index);
}
/**
*
* ValidatorOutstandingRewards represents outstanding (un-withdrawn) rewards
* for a validator inexpensive to track, allows simple sanity checks.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorOutstandingRewards}
*/
public static final class ValidatorOutstandingRewards extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.ValidatorOutstandingRewards)
ValidatorOutstandingRewardsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorOutstandingRewards.newBuilder() to construct.
private ValidatorOutstandingRewards(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorOutstandingRewards() {
rewards_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorOutstandingRewards(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
rewards_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.DecCoin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = java.util.Collections.unmodifiableList(rewards_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.class, cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.Builder.class);
}
public static final int REWARDS_FIELD_NUMBER = 1;
private java.util.List rewards_;
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getRewardsList() {
return rewards_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsOrBuilderList() {
return rewards_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getRewardsCount() {
return rewards_.size();
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getRewards(int index) {
return rewards_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardsOrBuilder(
int index) {
return rewards_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < rewards_.size(); i++) {
output.writeMessage(1, rewards_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < rewards_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, rewards_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards other = (cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards) obj;
boolean result = true;
result = result && getRewardsList()
.equals(other.getRewardsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getRewardsCount() > 0) {
hash = (37 * hash) + REWARDS_FIELD_NUMBER;
hash = (53 * hash) + getRewardsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorOutstandingRewards represents outstanding (un-withdrawn) rewards
* for a validator inexpensive to track, allows simple sanity checks.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorOutstandingRewards}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.ValidatorOutstandingRewards)
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewardsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.class, cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRewardsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (rewardsBuilder_ == null) {
rewards_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
rewardsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards build() {
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards buildPartial() {
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards result = new cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards(this);
int from_bitField0_ = bitField0_;
if (rewardsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = java.util.Collections.unmodifiableList(rewards_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.rewards_ = rewards_;
} else {
result.rewards_ = rewardsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards other) {
if (other == cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards.getDefaultInstance()) return this;
if (rewardsBuilder_ == null) {
if (!other.rewards_.isEmpty()) {
if (rewards_.isEmpty()) {
rewards_ = other.rewards_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRewardsIsMutable();
rewards_.addAll(other.rewards_);
}
onChanged();
}
} else {
if (!other.rewards_.isEmpty()) {
if (rewardsBuilder_.isEmpty()) {
rewardsBuilder_.dispose();
rewardsBuilder_ = null;
rewards_ = other.rewards_;
bitField0_ = (bitField0_ & ~0x00000001);
rewardsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRewardsFieldBuilder() : null;
} else {
rewardsBuilder_.addAllMessages(other.rewards_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List rewards_ =
java.util.Collections.emptyList();
private void ensureRewardsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
rewards_ = new java.util.ArrayList(rewards_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder> rewardsBuilder_;
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getRewardsList() {
if (rewardsBuilder_ == null) {
return java.util.Collections.unmodifiableList(rewards_);
} else {
return rewardsBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getRewardsCount() {
if (rewardsBuilder_ == null) {
return rewards_.size();
} else {
return rewardsBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getRewards(int index) {
if (rewardsBuilder_ == null) {
return rewards_.get(index);
} else {
return rewardsBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardsIsMutable();
rewards_.set(index, value);
onChanged();
} else {
rewardsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.set(index, builderForValue.build());
onChanged();
} else {
rewardsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardsIsMutable();
rewards_.add(value);
onChanged();
} else {
rewardsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardsIsMutable();
rewards_.add(index, value);
onChanged();
} else {
rewardsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.add(builderForValue.build());
onChanged();
} else {
rewardsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addRewards(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.add(index, builderForValue.build());
onChanged();
} else {
rewardsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addAllRewards(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.DecCoin> values) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, rewards_);
onChanged();
} else {
rewardsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder clearRewards() {
if (rewardsBuilder_ == null) {
rewards_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
rewardsBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder removeRewards(int index) {
if (rewardsBuilder_ == null) {
ensureRewardsIsMutable();
rewards_.remove(index);
onChanged();
} else {
rewardsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder getRewardsBuilder(
int index) {
return getRewardsFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardsOrBuilder(
int index) {
if (rewardsBuilder_ == null) {
return rewards_.get(index); } else {
return rewardsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsOrBuilderList() {
if (rewardsBuilder_ != null) {
return rewardsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(rewards_);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addRewardsBuilder() {
return getRewardsFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addRewardsBuilder(
int index) {
return getRewardsFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin rewards = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"rewards\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List
getRewardsBuilderList() {
return getRewardsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardsFieldBuilder() {
if (rewardsBuilder_ == null) {
rewardsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>(
rewards_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
rewards_ = null;
}
return rewardsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.ValidatorOutstandingRewards)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.ValidatorOutstandingRewards)
private static final cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards();
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorOutstandingRewards parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorOutstandingRewards(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorOutstandingRewards getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorSlashEventOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.ValidatorSlashEvent)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 validator_period = 1 [(.gogoproto.moretags) = "yaml:\"validator_period\""];
*/
long getValidatorPeriod();
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
java.lang.String getFraction();
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
com.google.protobuf.ByteString
getFractionBytes();
}
/**
*
* ValidatorSlashEvent represents a validator slash event.
* Height is implicit within the store key.
* This is needed to calculate appropriate amount of staking tokens
* for delegations which are withdrawn after a slash has occurred.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorSlashEvent}
*/
public static final class ValidatorSlashEvent extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.ValidatorSlashEvent)
ValidatorSlashEventOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorSlashEvent.newBuilder() to construct.
private ValidatorSlashEvent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorSlashEvent() {
validatorPeriod_ = 0L;
fraction_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorSlashEvent(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
validatorPeriod_ = input.readUInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
fraction_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.class, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder.class);
}
public static final int VALIDATOR_PERIOD_FIELD_NUMBER = 1;
private long validatorPeriod_;
/**
* uint64 validator_period = 1 [(.gogoproto.moretags) = "yaml:\"validator_period\""];
*/
public long getValidatorPeriod() {
return validatorPeriod_;
}
public static final int FRACTION_FIELD_NUMBER = 2;
private volatile java.lang.Object fraction_;
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getFraction() {
java.lang.Object ref = fraction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fraction_ = s;
return s;
}
}
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getFractionBytes() {
java.lang.Object ref = fraction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fraction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (validatorPeriod_ != 0L) {
output.writeUInt64(1, validatorPeriod_);
}
if (!getFractionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, fraction_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (validatorPeriod_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, validatorPeriod_);
}
if (!getFractionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, fraction_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent other = (cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent) obj;
boolean result = true;
result = result && (getValidatorPeriod()
== other.getValidatorPeriod());
result = result && getFraction()
.equals(other.getFraction());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALIDATOR_PERIOD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getValidatorPeriod());
hash = (37 * hash) + FRACTION_FIELD_NUMBER;
hash = (53 * hash) + getFraction().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorSlashEvent represents a validator slash event.
* Height is implicit within the store key.
* This is needed to calculate appropriate amount of staking tokens
* for delegations which are withdrawn after a slash has occurred.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorSlashEvent}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.ValidatorSlashEvent)
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.class, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
validatorPeriod_ = 0L;
fraction_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent build() {
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent buildPartial() {
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent result = new cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent(this);
result.validatorPeriod_ = validatorPeriod_;
result.fraction_ = fraction_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent other) {
if (other == cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.getDefaultInstance()) return this;
if (other.getValidatorPeriod() != 0L) {
setValidatorPeriod(other.getValidatorPeriod());
}
if (!other.getFraction().isEmpty()) {
fraction_ = other.fraction_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long validatorPeriod_ ;
/**
* uint64 validator_period = 1 [(.gogoproto.moretags) = "yaml:\"validator_period\""];
*/
public long getValidatorPeriod() {
return validatorPeriod_;
}
/**
* uint64 validator_period = 1 [(.gogoproto.moretags) = "yaml:\"validator_period\""];
*/
public Builder setValidatorPeriod(long value) {
validatorPeriod_ = value;
onChanged();
return this;
}
/**
* uint64 validator_period = 1 [(.gogoproto.moretags) = "yaml:\"validator_period\""];
*/
public Builder clearValidatorPeriod() {
validatorPeriod_ = 0L;
onChanged();
return this;
}
private java.lang.Object fraction_ = "";
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getFraction() {
java.lang.Object ref = fraction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fraction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getFractionBytes() {
java.lang.Object ref = fraction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fraction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setFraction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fraction_ = value;
onChanged();
return this;
}
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder clearFraction() {
fraction_ = getDefaultInstance().getFraction();
onChanged();
return this;
}
/**
* string fraction = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setFractionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fraction_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.ValidatorSlashEvent)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.ValidatorSlashEvent)
private static final cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent();
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorSlashEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorSlashEvent(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorSlashEventsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.ValidatorSlashEvents)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
java.util.List
getValidatorSlashEventsList();
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent getValidatorSlashEvents(int index);
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
int getValidatorSlashEventsCount();
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
java.util.List extends cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder>
getValidatorSlashEventsOrBuilderList();
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder getValidatorSlashEventsOrBuilder(
int index);
}
/**
*
* ValidatorSlashEvents is a collection of ValidatorSlashEvent messages.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorSlashEvents}
*/
public static final class ValidatorSlashEvents extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.ValidatorSlashEvents)
ValidatorSlashEventsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorSlashEvents.newBuilder() to construct.
private ValidatorSlashEvents(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorSlashEvents() {
validatorSlashEvents_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorSlashEvents(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
validatorSlashEvents_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
validatorSlashEvents_.add(
input.readMessage(cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
validatorSlashEvents_ = java.util.Collections.unmodifiableList(validatorSlashEvents_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.class, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.Builder.class);
}
public static final int VALIDATOR_SLASH_EVENTS_FIELD_NUMBER = 1;
private java.util.List validatorSlashEvents_;
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public java.util.List getValidatorSlashEventsList() {
return validatorSlashEvents_;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public java.util.List extends cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder>
getValidatorSlashEventsOrBuilderList() {
return validatorSlashEvents_;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public int getValidatorSlashEventsCount() {
return validatorSlashEvents_.size();
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent getValidatorSlashEvents(int index) {
return validatorSlashEvents_.get(index);
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder getValidatorSlashEventsOrBuilder(
int index) {
return validatorSlashEvents_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < validatorSlashEvents_.size(); i++) {
output.writeMessage(1, validatorSlashEvents_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < validatorSlashEvents_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, validatorSlashEvents_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents other = (cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents) obj;
boolean result = true;
result = result && getValidatorSlashEventsList()
.equals(other.getValidatorSlashEventsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getValidatorSlashEventsCount() > 0) {
hash = (37 * hash) + VALIDATOR_SLASH_EVENTS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorSlashEventsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorSlashEvents is a collection of ValidatorSlashEvent messages.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.ValidatorSlashEvents}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.ValidatorSlashEvents)
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.class, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValidatorSlashEventsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (validatorSlashEventsBuilder_ == null) {
validatorSlashEvents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
validatorSlashEventsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents build() {
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents buildPartial() {
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents result = new cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents(this);
int from_bitField0_ = bitField0_;
if (validatorSlashEventsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
validatorSlashEvents_ = java.util.Collections.unmodifiableList(validatorSlashEvents_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.validatorSlashEvents_ = validatorSlashEvents_;
} else {
result.validatorSlashEvents_ = validatorSlashEventsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents other) {
if (other == cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents.getDefaultInstance()) return this;
if (validatorSlashEventsBuilder_ == null) {
if (!other.validatorSlashEvents_.isEmpty()) {
if (validatorSlashEvents_.isEmpty()) {
validatorSlashEvents_ = other.validatorSlashEvents_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.addAll(other.validatorSlashEvents_);
}
onChanged();
}
} else {
if (!other.validatorSlashEvents_.isEmpty()) {
if (validatorSlashEventsBuilder_.isEmpty()) {
validatorSlashEventsBuilder_.dispose();
validatorSlashEventsBuilder_ = null;
validatorSlashEvents_ = other.validatorSlashEvents_;
bitField0_ = (bitField0_ & ~0x00000001);
validatorSlashEventsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValidatorSlashEventsFieldBuilder() : null;
} else {
validatorSlashEventsBuilder_.addAllMessages(other.validatorSlashEvents_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List validatorSlashEvents_ =
java.util.Collections.emptyList();
private void ensureValidatorSlashEventsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
validatorSlashEvents_ = new java.util.ArrayList(validatorSlashEvents_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder> validatorSlashEventsBuilder_;
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public java.util.List getValidatorSlashEventsList() {
if (validatorSlashEventsBuilder_ == null) {
return java.util.Collections.unmodifiableList(validatorSlashEvents_);
} else {
return validatorSlashEventsBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public int getValidatorSlashEventsCount() {
if (validatorSlashEventsBuilder_ == null) {
return validatorSlashEvents_.size();
} else {
return validatorSlashEventsBuilder_.getCount();
}
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent getValidatorSlashEvents(int index) {
if (validatorSlashEventsBuilder_ == null) {
return validatorSlashEvents_.get(index);
} else {
return validatorSlashEventsBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder setValidatorSlashEvents(
int index, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent value) {
if (validatorSlashEventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.set(index, value);
onChanged();
} else {
validatorSlashEventsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder setValidatorSlashEvents(
int index, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder builderForValue) {
if (validatorSlashEventsBuilder_ == null) {
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.set(index, builderForValue.build());
onChanged();
} else {
validatorSlashEventsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder addValidatorSlashEvents(cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent value) {
if (validatorSlashEventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.add(value);
onChanged();
} else {
validatorSlashEventsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder addValidatorSlashEvents(
int index, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent value) {
if (validatorSlashEventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.add(index, value);
onChanged();
} else {
validatorSlashEventsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder addValidatorSlashEvents(
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder builderForValue) {
if (validatorSlashEventsBuilder_ == null) {
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.add(builderForValue.build());
onChanged();
} else {
validatorSlashEventsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder addValidatorSlashEvents(
int index, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder builderForValue) {
if (validatorSlashEventsBuilder_ == null) {
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.add(index, builderForValue.build());
onChanged();
} else {
validatorSlashEventsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder addAllValidatorSlashEvents(
java.lang.Iterable extends cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent> values) {
if (validatorSlashEventsBuilder_ == null) {
ensureValidatorSlashEventsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, validatorSlashEvents_);
onChanged();
} else {
validatorSlashEventsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder clearValidatorSlashEvents() {
if (validatorSlashEventsBuilder_ == null) {
validatorSlashEvents_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
validatorSlashEventsBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public Builder removeValidatorSlashEvents(int index) {
if (validatorSlashEventsBuilder_ == null) {
ensureValidatorSlashEventsIsMutable();
validatorSlashEvents_.remove(index);
onChanged();
} else {
validatorSlashEventsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder getValidatorSlashEventsBuilder(
int index) {
return getValidatorSlashEventsFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder getValidatorSlashEventsOrBuilder(
int index) {
if (validatorSlashEventsBuilder_ == null) {
return validatorSlashEvents_.get(index); } else {
return validatorSlashEventsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public java.util.List extends cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder>
getValidatorSlashEventsOrBuilderList() {
if (validatorSlashEventsBuilder_ != null) {
return validatorSlashEventsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(validatorSlashEvents_);
}
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder addValidatorSlashEventsBuilder() {
return getValidatorSlashEventsFieldBuilder().addBuilder(
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.getDefaultInstance());
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder addValidatorSlashEventsBuilder(
int index) {
return getValidatorSlashEventsFieldBuilder().addBuilder(
index, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.getDefaultInstance());
}
/**
* repeated .cosmos.distribution.v1beta1.ValidatorSlashEvent validator_slash_events = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"validator_slash_events\""];
*/
public java.util.List
getValidatorSlashEventsBuilderList() {
return getValidatorSlashEventsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder>
getValidatorSlashEventsFieldBuilder() {
if (validatorSlashEventsBuilder_ == null) {
validatorSlashEventsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvent.Builder, cosmos.distribution.v1beta1.Distribution.ValidatorSlashEventOrBuilder>(
validatorSlashEvents_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
validatorSlashEvents_ = null;
}
return validatorSlashEventsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.ValidatorSlashEvents)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.ValidatorSlashEvents)
private static final cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents();
}
public static cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorSlashEvents parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorSlashEvents(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.ValidatorSlashEvents getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FeePoolOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.FeePool)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List
getCommunityPoolList();
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoin getCommunityPool(int index);
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
int getCommunityPoolCount();
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommunityPoolOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCommunityPoolOrBuilder(
int index);
}
/**
*
* FeePool is the global fee pool for distribution.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.FeePool}
*/
public static final class FeePool extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.FeePool)
FeePoolOrBuilder {
private static final long serialVersionUID = 0L;
// Use FeePool.newBuilder() to construct.
private FeePool(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FeePool() {
communityPool_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FeePool(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
communityPool_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
communityPool_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.DecCoin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
communityPool_ = java.util.Collections.unmodifiableList(communityPool_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_FeePool_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_FeePool_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.FeePool.class, cosmos.distribution.v1beta1.Distribution.FeePool.Builder.class);
}
public static final int COMMUNITY_POOL_FIELD_NUMBER = 1;
private java.util.List communityPool_;
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getCommunityPoolList() {
return communityPool_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommunityPoolOrBuilderList() {
return communityPool_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getCommunityPoolCount() {
return communityPool_.size();
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getCommunityPool(int index) {
return communityPool_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCommunityPoolOrBuilder(
int index) {
return communityPool_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < communityPool_.size(); i++) {
output.writeMessage(1, communityPool_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < communityPool_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, communityPool_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.FeePool)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.FeePool other = (cosmos.distribution.v1beta1.Distribution.FeePool) obj;
boolean result = true;
result = result && getCommunityPoolList()
.equals(other.getCommunityPoolList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCommunityPoolCount() > 0) {
hash = (37 * hash) + COMMUNITY_POOL_FIELD_NUMBER;
hash = (53 * hash) + getCommunityPoolList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.FeePool parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.FeePool prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* FeePool is the global fee pool for distribution.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.FeePool}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.FeePool)
cosmos.distribution.v1beta1.Distribution.FeePoolOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_FeePool_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_FeePool_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.FeePool.class, cosmos.distribution.v1beta1.Distribution.FeePool.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.FeePool.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommunityPoolFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (communityPoolBuilder_ == null) {
communityPool_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
communityPoolBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_FeePool_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.FeePool getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.FeePool.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.FeePool build() {
cosmos.distribution.v1beta1.Distribution.FeePool result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.FeePool buildPartial() {
cosmos.distribution.v1beta1.Distribution.FeePool result = new cosmos.distribution.v1beta1.Distribution.FeePool(this);
int from_bitField0_ = bitField0_;
if (communityPoolBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
communityPool_ = java.util.Collections.unmodifiableList(communityPool_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.communityPool_ = communityPool_;
} else {
result.communityPool_ = communityPoolBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.FeePool) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.FeePool)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.FeePool other) {
if (other == cosmos.distribution.v1beta1.Distribution.FeePool.getDefaultInstance()) return this;
if (communityPoolBuilder_ == null) {
if (!other.communityPool_.isEmpty()) {
if (communityPool_.isEmpty()) {
communityPool_ = other.communityPool_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCommunityPoolIsMutable();
communityPool_.addAll(other.communityPool_);
}
onChanged();
}
} else {
if (!other.communityPool_.isEmpty()) {
if (communityPoolBuilder_.isEmpty()) {
communityPoolBuilder_.dispose();
communityPoolBuilder_ = null;
communityPool_ = other.communityPool_;
bitField0_ = (bitField0_ & ~0x00000001);
communityPoolBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCommunityPoolFieldBuilder() : null;
} else {
communityPoolBuilder_.addAllMessages(other.communityPool_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.FeePool parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.FeePool) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List communityPool_ =
java.util.Collections.emptyList();
private void ensureCommunityPoolIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
communityPool_ = new java.util.ArrayList(communityPool_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder> communityPoolBuilder_;
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getCommunityPoolList() {
if (communityPoolBuilder_ == null) {
return java.util.Collections.unmodifiableList(communityPool_);
} else {
return communityPoolBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getCommunityPoolCount() {
if (communityPoolBuilder_ == null) {
return communityPool_.size();
} else {
return communityPoolBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getCommunityPool(int index) {
if (communityPoolBuilder_ == null) {
return communityPool_.get(index);
} else {
return communityPoolBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setCommunityPool(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (communityPoolBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommunityPoolIsMutable();
communityPool_.set(index, value);
onChanged();
} else {
communityPoolBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setCommunityPool(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (communityPoolBuilder_ == null) {
ensureCommunityPoolIsMutable();
communityPool_.set(index, builderForValue.build());
onChanged();
} else {
communityPoolBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommunityPool(cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (communityPoolBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommunityPoolIsMutable();
communityPool_.add(value);
onChanged();
} else {
communityPoolBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommunityPool(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (communityPoolBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommunityPoolIsMutable();
communityPool_.add(index, value);
onChanged();
} else {
communityPoolBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommunityPool(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (communityPoolBuilder_ == null) {
ensureCommunityPoolIsMutable();
communityPool_.add(builderForValue.build());
onChanged();
} else {
communityPoolBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addCommunityPool(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (communityPoolBuilder_ == null) {
ensureCommunityPoolIsMutable();
communityPool_.add(index, builderForValue.build());
onChanged();
} else {
communityPoolBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addAllCommunityPool(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.DecCoin> values) {
if (communityPoolBuilder_ == null) {
ensureCommunityPoolIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, communityPool_);
onChanged();
} else {
communityPoolBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder clearCommunityPool() {
if (communityPoolBuilder_ == null) {
communityPool_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
communityPoolBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder removeCommunityPool(int index) {
if (communityPoolBuilder_ == null) {
ensureCommunityPoolIsMutable();
communityPool_.remove(index);
onChanged();
} else {
communityPoolBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder getCommunityPoolBuilder(
int index) {
return getCommunityPoolFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getCommunityPoolOrBuilder(
int index) {
if (communityPoolBuilder_ == null) {
return communityPool_.get(index); } else {
return communityPoolBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommunityPoolOrBuilderList() {
if (communityPoolBuilder_ != null) {
return communityPoolBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(communityPool_);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addCommunityPoolBuilder() {
return getCommunityPoolFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addCommunityPoolBuilder(
int index) {
return getCommunityPoolFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin community_pool = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"community_pool\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List
getCommunityPoolBuilderList() {
return getCommunityPoolFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getCommunityPoolFieldBuilder() {
if (communityPoolBuilder_ == null) {
communityPoolBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>(
communityPool_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
communityPool_ = null;
}
return communityPoolBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.FeePool)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.FeePool)
private static final cosmos.distribution.v1beta1.Distribution.FeePool DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.FeePool();
}
public static cosmos.distribution.v1beta1.Distribution.FeePool getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FeePool parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FeePool(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.FeePool getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommunityPoolSpendProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.CommunityPoolSpendProposal)
com.google.protobuf.MessageOrBuilder {
/**
* string title = 1;
*/
java.lang.String getTitle();
/**
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
* string description = 2;
*/
java.lang.String getDescription();
/**
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
* string recipient = 3;
*/
java.lang.String getRecipient();
/**
* string recipient = 3;
*/
com.google.protobuf.ByteString
getRecipientBytes();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List
getAmountList();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index);
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
int getAmountCount();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index);
}
/**
*
* CommunityPoolSpendProposal details a proposal for use of community funds,
* together with how many coins are proposed to be spent, and to which
* recipient account.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.CommunityPoolSpendProposal}
*/
public static final class CommunityPoolSpendProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.CommunityPoolSpendProposal)
CommunityPoolSpendProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommunityPoolSpendProposal.newBuilder() to construct.
private CommunityPoolSpendProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommunityPoolSpendProposal() {
title_ = "";
description_ = "";
recipient_ = "";
amount_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommunityPoolSpendProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
recipient_ = s;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
amount_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
amount_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
amount_ = java.util.Collections.unmodifiableList(amount_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.class, cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.Builder.class);
}
private int bitField0_;
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RECIPIENT_FIELD_NUMBER = 3;
private volatile java.lang.Object recipient_;
/**
* string recipient = 3;
*/
public java.lang.String getRecipient() {
java.lang.Object ref = recipient_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
recipient_ = s;
return s;
}
}
/**
* string recipient = 3;
*/
public com.google.protobuf.ByteString
getRecipientBytes() {
java.lang.Object ref = recipient_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
recipient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AMOUNT_FIELD_NUMBER = 4;
private java.util.List amount_;
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getAmountList() {
return amount_;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList() {
return amount_;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getAmountCount() {
return amount_.size();
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index) {
return amount_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index) {
return amount_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getRecipientBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, recipient_);
}
for (int i = 0; i < amount_.size(); i++) {
output.writeMessage(4, amount_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getRecipientBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, recipient_);
}
for (int i = 0; i < amount_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, amount_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal other = (cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getRecipient()
.equals(other.getRecipient());
result = result && getAmountList()
.equals(other.getAmountList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + RECIPIENT_FIELD_NUMBER;
hash = (53 * hash) + getRecipient().hashCode();
if (getAmountCount() > 0) {
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmountList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* CommunityPoolSpendProposal details a proposal for use of community funds,
* together with how many coins are proposed to be spent, and to which
* recipient account.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.CommunityPoolSpendProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.CommunityPoolSpendProposal)
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.class, cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAmountFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
recipient_ = "";
if (amountBuilder_ == null) {
amount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
amountBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal build() {
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal buildPartial() {
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal result = new cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.title_ = title_;
result.description_ = description_;
result.recipient_ = recipient_;
if (amountBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
amount_ = java.util.Collections.unmodifiableList(amount_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.amount_ = amount_;
} else {
result.amount_ = amountBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal other) {
if (other == cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getRecipient().isEmpty()) {
recipient_ = other.recipient_;
onChanged();
}
if (amountBuilder_ == null) {
if (!other.amount_.isEmpty()) {
if (amount_.isEmpty()) {
amount_ = other.amount_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureAmountIsMutable();
amount_.addAll(other.amount_);
}
onChanged();
}
} else {
if (!other.amount_.isEmpty()) {
if (amountBuilder_.isEmpty()) {
amountBuilder_.dispose();
amountBuilder_ = null;
amount_ = other.amount_;
bitField0_ = (bitField0_ & ~0x00000008);
amountBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAmountFieldBuilder() : null;
} else {
amountBuilder_.addAllMessages(other.amount_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object title_ = "";
/**
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object recipient_ = "";
/**
* string recipient = 3;
*/
public java.lang.String getRecipient() {
java.lang.Object ref = recipient_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
recipient_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string recipient = 3;
*/
public com.google.protobuf.ByteString
getRecipientBytes() {
java.lang.Object ref = recipient_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
recipient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string recipient = 3;
*/
public Builder setRecipient(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
recipient_ = value;
onChanged();
return this;
}
/**
* string recipient = 3;
*/
public Builder clearRecipient() {
recipient_ = getDefaultInstance().getRecipient();
onChanged();
return this;
}
/**
* string recipient = 3;
*/
public Builder setRecipientBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
recipient_ = value;
onChanged();
return this;
}
private java.util.List amount_ =
java.util.Collections.emptyList();
private void ensureAmountIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
amount_ = new java.util.ArrayList(amount_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> amountBuilder_;
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getAmountList() {
if (amountBuilder_ == null) {
return java.util.Collections.unmodifiableList(amount_);
} else {
return amountBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getAmountCount() {
if (amountBuilder_ == null) {
return amount_.size();
} else {
return amountBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index) {
if (amountBuilder_ == null) {
return amount_.get(index);
} else {
return amountBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.set(index, value);
onChanged();
} else {
amountBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.set(index, builderForValue.build());
onChanged();
} else {
amountBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.add(value);
onChanged();
} else {
amountBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.add(index, value);
onChanged();
} else {
amountBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.add(builderForValue.build());
onChanged();
} else {
amountBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.add(index, builderForValue.build());
onChanged();
} else {
amountBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAllAmount(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.Coin> values) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, amount_);
onChanged();
} else {
amountBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder clearAmount() {
if (amountBuilder_ == null) {
amount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
amountBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder removeAmount(int index) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.remove(index);
onChanged();
} else {
amountBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getAmountBuilder(
int index) {
return getAmountFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index) {
if (amountBuilder_ == null) {
return amount_.get(index); } else {
return amountBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList() {
if (amountBuilder_ != null) {
return amountBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(amount_);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addAmountBuilder() {
return getAmountFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addAmountBuilder(
int index) {
return getAmountFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 4 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List
getAmountBuilderList() {
return getAmountFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountFieldBuilder() {
if (amountBuilder_ == null) {
amountBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
amount_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
amount_ = null;
}
return amountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.CommunityPoolSpendProposal)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.CommunityPoolSpendProposal)
private static final cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal();
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommunityPoolSpendProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommunityPoolSpendProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DelegatorStartingInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.DelegatorStartingInfo)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 previous_period = 1 [(.gogoproto.moretags) = "yaml:\"previous_period\""];
*/
long getPreviousPeriod();
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
java.lang.String getStake();
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
com.google.protobuf.ByteString
getStakeBytes();
/**
* uint64 height = 3 [(.gogoproto.jsontag) = "creation_height", (.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
long getHeight();
}
/**
*
* DelegatorStartingInfo represents the starting info for a delegator reward
* period. It tracks the previous validator period, the delegation's amount of
* staking token, and the creation height (to check later on if any slashes have
* occurred). NOTE: Even though validators are slashed to whole staking tokens,
* the delegators within the validator may be left with less than a full token,
* thus sdk.Dec is used.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.DelegatorStartingInfo}
*/
public static final class DelegatorStartingInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.DelegatorStartingInfo)
DelegatorStartingInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use DelegatorStartingInfo.newBuilder() to construct.
private DelegatorStartingInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DelegatorStartingInfo() {
previousPeriod_ = 0L;
stake_ = "";
height_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DelegatorStartingInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
previousPeriod_ = input.readUInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
stake_ = s;
break;
}
case 24: {
height_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.class, cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.Builder.class);
}
public static final int PREVIOUS_PERIOD_FIELD_NUMBER = 1;
private long previousPeriod_;
/**
* uint64 previous_period = 1 [(.gogoproto.moretags) = "yaml:\"previous_period\""];
*/
public long getPreviousPeriod() {
return previousPeriod_;
}
public static final int STAKE_FIELD_NUMBER = 2;
private volatile java.lang.Object stake_;
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public java.lang.String getStake() {
java.lang.Object ref = stake_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stake_ = s;
return s;
}
}
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public com.google.protobuf.ByteString
getStakeBytes() {
java.lang.Object ref = stake_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stake_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private long height_;
/**
* uint64 height = 3 [(.gogoproto.jsontag) = "creation_height", (.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public long getHeight() {
return height_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (previousPeriod_ != 0L) {
output.writeUInt64(1, previousPeriod_);
}
if (!getStakeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, stake_);
}
if (height_ != 0L) {
output.writeUInt64(3, height_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (previousPeriod_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, previousPeriod_);
}
if (!getStakeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, stake_);
}
if (height_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, height_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo other = (cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo) obj;
boolean result = true;
result = result && (getPreviousPeriod()
== other.getPreviousPeriod());
result = result && getStake()
.equals(other.getStake());
result = result && (getHeight()
== other.getHeight());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PREVIOUS_PERIOD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPreviousPeriod());
hash = (37 * hash) + STAKE_FIELD_NUMBER;
hash = (53 * hash) + getStake().hashCode();
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getHeight());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DelegatorStartingInfo represents the starting info for a delegator reward
* period. It tracks the previous validator period, the delegation's amount of
* staking token, and the creation height (to check later on if any slashes have
* occurred). NOTE: Even though validators are slashed to whole staking tokens,
* the delegators within the validator may be left with less than a full token,
* thus sdk.Dec is used.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.DelegatorStartingInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.DelegatorStartingInfo)
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.class, cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
previousPeriod_ = 0L;
stake_ = "";
height_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo build() {
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo buildPartial() {
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo result = new cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo(this);
result.previousPeriod_ = previousPeriod_;
result.stake_ = stake_;
result.height_ = height_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo other) {
if (other == cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo.getDefaultInstance()) return this;
if (other.getPreviousPeriod() != 0L) {
setPreviousPeriod(other.getPreviousPeriod());
}
if (!other.getStake().isEmpty()) {
stake_ = other.stake_;
onChanged();
}
if (other.getHeight() != 0L) {
setHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long previousPeriod_ ;
/**
* uint64 previous_period = 1 [(.gogoproto.moretags) = "yaml:\"previous_period\""];
*/
public long getPreviousPeriod() {
return previousPeriod_;
}
/**
* uint64 previous_period = 1 [(.gogoproto.moretags) = "yaml:\"previous_period\""];
*/
public Builder setPreviousPeriod(long value) {
previousPeriod_ = value;
onChanged();
return this;
}
/**
* uint64 previous_period = 1 [(.gogoproto.moretags) = "yaml:\"previous_period\""];
*/
public Builder clearPreviousPeriod() {
previousPeriod_ = 0L;
onChanged();
return this;
}
private java.lang.Object stake_ = "";
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public java.lang.String getStake() {
java.lang.Object ref = stake_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stake_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public com.google.protobuf.ByteString
getStakeBytes() {
java.lang.Object ref = stake_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stake_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public Builder setStake(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stake_ = value;
onChanged();
return this;
}
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public Builder clearStake() {
stake_ = getDefaultInstance().getStake();
onChanged();
return this;
}
/**
* string stake = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"stake\""];
*/
public Builder setStakeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stake_ = value;
onChanged();
return this;
}
private long height_ ;
/**
* uint64 height = 3 [(.gogoproto.jsontag) = "creation_height", (.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public long getHeight() {
return height_;
}
/**
* uint64 height = 3 [(.gogoproto.jsontag) = "creation_height", (.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public Builder setHeight(long value) {
height_ = value;
onChanged();
return this;
}
/**
* uint64 height = 3 [(.gogoproto.jsontag) = "creation_height", (.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public Builder clearHeight() {
height_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.DelegatorStartingInfo)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.DelegatorStartingInfo)
private static final cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo();
}
public static cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DelegatorStartingInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DelegatorStartingInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegatorStartingInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DelegationDelegatorRewardOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.DelegationDelegatorReward)
com.google.protobuf.MessageOrBuilder {
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
java.lang.String getValidatorAddress();
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
com.google.protobuf.ByteString
getValidatorAddressBytes();
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List
getRewardList();
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoin getReward(int index);
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
int getRewardCount();
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardOrBuilder(
int index);
}
/**
*
* DelegationDelegatorReward represents the properties
* of a delegator's delegation reward.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.DelegationDelegatorReward}
*/
public static final class DelegationDelegatorReward extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.DelegationDelegatorReward)
DelegationDelegatorRewardOrBuilder {
private static final long serialVersionUID = 0L;
// Use DelegationDelegatorReward.newBuilder() to construct.
private DelegationDelegatorReward(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DelegationDelegatorReward() {
validatorAddress_ = "";
reward_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DelegationDelegatorReward(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
validatorAddress_ = s;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
reward_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
reward_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.DecCoin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
reward_ = java.util.Collections.unmodifiableList(reward_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.class, cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.Builder.class);
}
private int bitField0_;
public static final int VALIDATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object validatorAddress_;
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
}
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REWARD_FIELD_NUMBER = 2;
private java.util.List reward_;
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getRewardList() {
return reward_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardOrBuilderList() {
return reward_;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getRewardCount() {
return reward_.size();
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getReward(int index) {
return reward_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardOrBuilder(
int index) {
return reward_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getValidatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, validatorAddress_);
}
for (int i = 0; i < reward_.size(); i++) {
output.writeMessage(2, reward_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getValidatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, validatorAddress_);
}
for (int i = 0; i < reward_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, reward_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward other = (cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward) obj;
boolean result = true;
result = result && getValidatorAddress()
.equals(other.getValidatorAddress());
result = result && getRewardList()
.equals(other.getRewardList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALIDATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorAddress().hashCode();
if (getRewardCount() > 0) {
hash = (37 * hash) + REWARD_FIELD_NUMBER;
hash = (53 * hash) + getRewardList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DelegationDelegatorReward represents the properties
* of a delegator's delegation reward.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.DelegationDelegatorReward}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.DelegationDelegatorReward)
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorRewardOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.class, cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRewardFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
validatorAddress_ = "";
if (rewardBuilder_ == null) {
reward_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
rewardBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward build() {
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward buildPartial() {
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward result = new cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.validatorAddress_ = validatorAddress_;
if (rewardBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
reward_ = java.util.Collections.unmodifiableList(reward_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.reward_ = reward_;
} else {
result.reward_ = rewardBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward other) {
if (other == cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward.getDefaultInstance()) return this;
if (!other.getValidatorAddress().isEmpty()) {
validatorAddress_ = other.validatorAddress_;
onChanged();
}
if (rewardBuilder_ == null) {
if (!other.reward_.isEmpty()) {
if (reward_.isEmpty()) {
reward_ = other.reward_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureRewardIsMutable();
reward_.addAll(other.reward_);
}
onChanged();
}
} else {
if (!other.reward_.isEmpty()) {
if (rewardBuilder_.isEmpty()) {
rewardBuilder_.dispose();
rewardBuilder_ = null;
reward_ = other.reward_;
bitField0_ = (bitField0_ & ~0x00000002);
rewardBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getRewardFieldBuilder() : null;
} else {
rewardBuilder_.addAllMessages(other.reward_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object validatorAddress_ = "";
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorAddress_ = value;
onChanged();
return this;
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder clearValidatorAddress() {
validatorAddress_ = getDefaultInstance().getValidatorAddress();
onChanged();
return this;
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorAddress_ = value;
onChanged();
return this;
}
private java.util.List reward_ =
java.util.Collections.emptyList();
private void ensureRewardIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
reward_ = new java.util.ArrayList(reward_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder> rewardBuilder_;
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List getRewardList() {
if (rewardBuilder_ == null) {
return java.util.Collections.unmodifiableList(reward_);
} else {
return rewardBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public int getRewardCount() {
if (rewardBuilder_ == null) {
return reward_.size();
} else {
return rewardBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin getReward(int index) {
if (rewardBuilder_ == null) {
return reward_.get(index);
} else {
return rewardBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setReward(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardIsMutable();
reward_.set(index, value);
onChanged();
} else {
rewardBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder setReward(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardBuilder_ == null) {
ensureRewardIsMutable();
reward_.set(index, builderForValue.build());
onChanged();
} else {
rewardBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addReward(cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardIsMutable();
reward_.add(value);
onChanged();
} else {
rewardBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addReward(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin value) {
if (rewardBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRewardIsMutable();
reward_.add(index, value);
onChanged();
} else {
rewardBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addReward(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardBuilder_ == null) {
ensureRewardIsMutable();
reward_.add(builderForValue.build());
onChanged();
} else {
rewardBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addReward(
int index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder builderForValue) {
if (rewardBuilder_ == null) {
ensureRewardIsMutable();
reward_.add(index, builderForValue.build());
onChanged();
} else {
rewardBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder addAllReward(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.DecCoin> values) {
if (rewardBuilder_ == null) {
ensureRewardIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, reward_);
onChanged();
} else {
rewardBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder clearReward() {
if (rewardBuilder_ == null) {
reward_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
rewardBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public Builder removeReward(int index) {
if (rewardBuilder_ == null) {
ensureRewardIsMutable();
reward_.remove(index);
onChanged();
} else {
rewardBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder getRewardBuilder(
int index) {
return getRewardFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder getRewardOrBuilder(
int index) {
if (rewardBuilder_ == null) {
return reward_.get(index); } else {
return rewardBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardOrBuilderList() {
if (rewardBuilder_ != null) {
return rewardBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(reward_);
}
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addRewardBuilder() {
return getRewardFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder addRewardBuilder(
int index) {
return getRewardFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.DecCoin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.DecCoin reward = 2 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.DecCoins"];
*/
public java.util.List
getRewardBuilderList() {
return getRewardFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>
getRewardFieldBuilder() {
if (rewardBuilder_ == null) {
rewardBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.DecCoin, cosmos.base.v1beta1.CoinOuterClass.DecCoin.Builder, cosmos.base.v1beta1.CoinOuterClass.DecCoinOrBuilder>(
reward_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
reward_ = null;
}
return rewardBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.DelegationDelegatorReward)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.DelegationDelegatorReward)
private static final cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward();
}
public static cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DelegationDelegatorReward parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DelegationDelegatorReward(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.DelegationDelegatorReward getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommunityPoolSpendProposalWithDepositOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit)
com.google.protobuf.MessageOrBuilder {
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
java.lang.String getTitle();
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
java.lang.String getDescription();
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
java.lang.String getRecipient();
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
com.google.protobuf.ByteString
getRecipientBytes();
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
java.lang.String getAmount();
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
com.google.protobuf.ByteString
getAmountBytes();
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
java.lang.String getDeposit();
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
com.google.protobuf.ByteString
getDepositBytes();
}
/**
*
* CommunityPoolSpendProposalWithDeposit defines a CommunityPoolSpendProposal
* with a deposit
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit}
*/
public static final class CommunityPoolSpendProposalWithDeposit extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit)
CommunityPoolSpendProposalWithDepositOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommunityPoolSpendProposalWithDeposit.newBuilder() to construct.
private CommunityPoolSpendProposalWithDeposit(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommunityPoolSpendProposalWithDeposit() {
title_ = "";
description_ = "";
recipient_ = "";
amount_ = "";
deposit_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommunityPoolSpendProposalWithDeposit(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
recipient_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
amount_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
deposit_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.class, cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.Builder.class);
}
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RECIPIENT_FIELD_NUMBER = 3;
private volatile java.lang.Object recipient_;
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public java.lang.String getRecipient() {
java.lang.Object ref = recipient_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
recipient_ = s;
return s;
}
}
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public com.google.protobuf.ByteString
getRecipientBytes() {
java.lang.Object ref = recipient_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
recipient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AMOUNT_FIELD_NUMBER = 4;
private volatile java.lang.Object amount_;
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public java.lang.String getAmount() {
java.lang.Object ref = amount_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
amount_ = s;
return s;
}
}
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public com.google.protobuf.ByteString
getAmountBytes() {
java.lang.Object ref = amount_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
amount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEPOSIT_FIELD_NUMBER = 5;
private volatile java.lang.Object deposit_;
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public java.lang.String getDeposit() {
java.lang.Object ref = deposit_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
deposit_ = s;
return s;
}
}
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public com.google.protobuf.ByteString
getDepositBytes() {
java.lang.Object ref = deposit_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deposit_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getRecipientBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, recipient_);
}
if (!getAmountBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, amount_);
}
if (!getDepositBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, deposit_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getRecipientBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, recipient_);
}
if (!getAmountBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, amount_);
}
if (!getDepositBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, deposit_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit other = (cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getRecipient()
.equals(other.getRecipient());
result = result && getAmount()
.equals(other.getAmount());
result = result && getDeposit()
.equals(other.getDeposit());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + RECIPIENT_FIELD_NUMBER;
hash = (53 * hash) + getRecipient().hashCode();
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmount().hashCode();
hash = (37 * hash) + DEPOSIT_FIELD_NUMBER;
hash = (53 * hash) + getDeposit().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* CommunityPoolSpendProposalWithDeposit defines a CommunityPoolSpendProposal
* with a deposit
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit)
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDepositOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.class, cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
recipient_ = "";
amount_ = "";
deposit_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Distribution.internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit build() {
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit buildPartial() {
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit result = new cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit(this);
result.title_ = title_;
result.description_ = description_;
result.recipient_ = recipient_;
result.amount_ = amount_;
result.deposit_ = deposit_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit) {
return mergeFrom((cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit other) {
if (other == cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getRecipient().isEmpty()) {
recipient_ = other.recipient_;
onChanged();
}
if (!other.getAmount().isEmpty()) {
amount_ = other.amount_;
onChanged();
}
if (!other.getDeposit().isEmpty()) {
deposit_ = other.deposit_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object title_ = "";
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object recipient_ = "";
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public java.lang.String getRecipient() {
java.lang.Object ref = recipient_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
recipient_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public com.google.protobuf.ByteString
getRecipientBytes() {
java.lang.Object ref = recipient_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
recipient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public Builder setRecipient(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
recipient_ = value;
onChanged();
return this;
}
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public Builder clearRecipient() {
recipient_ = getDefaultInstance().getRecipient();
onChanged();
return this;
}
/**
* string recipient = 3 [(.gogoproto.moretags) = "yaml:\"recipient\""];
*/
public Builder setRecipientBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
recipient_ = value;
onChanged();
return this;
}
private java.lang.Object amount_ = "";
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public java.lang.String getAmount() {
java.lang.Object ref = amount_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
amount_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public com.google.protobuf.ByteString
getAmountBytes() {
java.lang.Object ref = amount_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
amount_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public Builder setAmount(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
amount_ = value;
onChanged();
return this;
}
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public Builder clearAmount() {
amount_ = getDefaultInstance().getAmount();
onChanged();
return this;
}
/**
* string amount = 4 [(.gogoproto.moretags) = "yaml:\"amount\""];
*/
public Builder setAmountBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
amount_ = value;
onChanged();
return this;
}
private java.lang.Object deposit_ = "";
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public java.lang.String getDeposit() {
java.lang.Object ref = deposit_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
deposit_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public com.google.protobuf.ByteString
getDepositBytes() {
java.lang.Object ref = deposit_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deposit_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public Builder setDeposit(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
deposit_ = value;
onChanged();
return this;
}
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public Builder clearDeposit() {
deposit_ = getDefaultInstance().getDeposit();
onChanged();
return this;
}
/**
* string deposit = 5 [(.gogoproto.moretags) = "yaml:\"deposit\""];
*/
public Builder setDepositBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
deposit_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.CommunityPoolSpendProposalWithDeposit)
private static final cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit();
}
public static cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommunityPoolSpendProposalWithDeposit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommunityPoolSpendProposalWithDeposit(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Distribution.CommunityPoolSpendProposalWithDeposit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_Params_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_Params_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_FeePool_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_FeePool_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n.cosmos/distribution/v1beta1/distributi" +
"on.proto\022\033cosmos.distribution.v1beta1\032\024g" +
"ogoproto/gogo.proto\032\036cosmos/base/v1beta1" +
"/coin.proto\"\212\003\n\006Params\022]\n\rcommunity_tax\030" +
"\001 \001(\tBF\362\336\037\024yaml:\"community_tax\"\332\336\037&githu" +
"b.com/cosmos/cosmos-sdk/types.Dec\310\336\037\000\022k\n" +
"\024base_proposer_reward\030\002 \001(\tBM\362\336\037\033yaml:\"b" +
"ase_proposer_reward\"\332\336\037&github.com/cosmo" +
"s/cosmos-sdk/types.Dec\310\336\037\000\022m\n\025bonus_prop" +
"oser_reward\030\003 \001(\tBN\362\336\037\034yaml:\"bonus_propo" +
"ser_reward\"\332\336\037&github.com/cosmos/cosmos-" +
"sdk/types.Dec\310\336\037\000\022?\n\025withdraw_addr_enabl" +
"ed\030\004 \001(\010B \362\336\037\034yaml:\"withdraw_addr_enable" +
"d\":\004\230\240\037\000\"\350\001\n\032ValidatorHistoricalRewards\022" +
"\224\001\n\027cumulative_reward_ratio\030\001 \003(\0132\034.cosm" +
"os.base.v1beta1.DecCoinBU\362\336\037\036yaml:\"cumul" +
"ative_reward_ratio\"\252\337\037+github.com/cosmos" +
"/cosmos-sdk/types.DecCoins\310\336\037\000\0223\n\017refere" +
"nce_count\030\002 \001(\rB\032\362\336\037\026yaml:\"reference_cou" +
"nt\"\"\215\001\n\027ValidatorCurrentRewards\022b\n\007rewar" +
"ds\030\001 \003(\0132\034.cosmos.base.v1beta1.DecCoinB3" +
"\252\337\037+github.com/cosmos/cosmos-sdk/types.D" +
"ecCoins\310\336\037\000\022\016\n\006period\030\002 \001(\004\"\207\001\n\036Validato" +
"rAccumulatedCommission\022e\n\ncommission\030\001 \003" +
"(\0132\034.cosmos.base.v1beta1.DecCoinB3\252\337\037+gi" +
"thub.com/cosmos/cosmos-sdk/types.DecCoin" +
"s\310\336\037\000\"\223\001\n\033ValidatorOutstandingRewards\022t\n" +
"\007rewards\030\001 \003(\0132\034.cosmos.base.v1beta1.Dec" +
"CoinBE\362\336\037\016yaml:\"rewards\"\252\337\037+github.com/c" +
"osmos/cosmos-sdk/types.DecCoins\310\336\037\000\"\216\001\n\023" +
"ValidatorSlashEvent\0225\n\020validator_period\030" +
"\001 \001(\004B\033\362\336\037\027yaml:\"validator_period\"\022@\n\010fr" +
"action\030\002 \001(\tB.\332\336\037&github.com/cosmos/cosm" +
"os-sdk/types.Dec\310\336\037\000\"\225\001\n\024ValidatorSlashE" +
"vents\022w\n\026validator_slash_events\030\001 \003(\01320." +
"cosmos.distribution.v1beta1.ValidatorSla" +
"shEventB%\362\336\037\035yaml:\"validator_slash_event" +
"s\"\310\336\037\000:\004\230\240\037\000\"\216\001\n\007FeePool\022\202\001\n\016community_p" +
"ool\030\001 \003(\0132\034.cosmos.base.v1beta1.DecCoinB" +
"L\310\336\037\000\252\337\037+github.com/cosmos/cosmos-sdk/ty" +
"pes.DecCoins\362\336\037\025yaml:\"community_pool\"\"\276\001" +
"\n\032CommunityPoolSpendProposal\022\r\n\005title\030\001 " +
"\001(\t\022\023\n\013description\030\002 \001(\t\022\021\n\trecipient\030\003 " +
"\001(\t\022[\n\006amount\030\004 \003(\0132\031.cosmos.base.v1beta" +
"1.CoinB0\310\336\037\000\252\337\037(github.com/cosmos/cosmos" +
"-sdk/types.Coins:\014\350\240\037\000\210\240\037\000\230\240\037\000\"\332\001\n\025Deleg" +
"atorStartingInfo\0223\n\017previous_period\030\001 \001(" +
"\004B\032\362\336\037\026yaml:\"previous_period\"\022M\n\005stake\030\002" +
" \001(\tB>\362\336\037\014yaml:\"stake\"\332\336\037&github.com/cos" +
"mos/cosmos-sdk/types.Dec\310\336\037\000\022=\n\006height\030\003" +
" \001(\004B-\362\336\037\026yaml:\"creation_height\"\352\336\037\017crea" +
"tion_height\"\301\001\n\031DelegationDelegatorRewar" +
"d\0227\n\021validator_address\030\001 \001(\tB\034\362\336\037\030yaml:\"" +
"validator_address\"\022a\n\006reward\030\002 \003(\0132\034.cos" +
"mos.base.v1beta1.DecCoinB3\252\337\037+github.com" +
"/cosmos/cosmos-sdk/types.DecCoins\310\336\037\000:\010\210" +
"\240\037\000\230\240\037\001\"\360\001\n%CommunityPoolSpendProposalWi" +
"thDeposit\022\037\n\005title\030\001 \001(\tB\020\362\336\037\014yaml:\"titl" +
"e\"\022+\n\013description\030\002 \001(\tB\026\362\336\037\022yaml:\"descr" +
"iption\"\022\'\n\trecipient\030\003 \001(\tB\024\362\336\037\020yaml:\"re" +
"cipient\"\022!\n\006amount\030\004 \001(\tB\021\362\336\037\ryaml:\"amou" +
"nt\"\022#\n\007deposit\030\005 \001(\tB\022\362\336\037\016yaml:\"deposit\"" +
":\010\210\240\037\000\230\240\037\001B7Z1github.com/cosmos/cosmos-s" +
"dk/x/distribution/types\250\342\036\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
cosmos.base.v1beta1.CoinOuterClass.getDescriptor(),
}, assigner);
internal_static_cosmos_distribution_v1beta1_Params_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cosmos_distribution_v1beta1_Params_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_Params_descriptor,
new java.lang.String[] { "CommunityTax", "BaseProposerReward", "BonusProposerReward", "WithdrawAddrEnabled", });
internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_ValidatorHistoricalRewards_descriptor,
new java.lang.String[] { "CumulativeRewardRatio", "ReferenceCount", });
internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_ValidatorCurrentRewards_descriptor,
new java.lang.String[] { "Rewards", "Period", });
internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_ValidatorAccumulatedCommission_descriptor,
new java.lang.String[] { "Commission", });
internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_ValidatorOutstandingRewards_descriptor,
new java.lang.String[] { "Rewards", });
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvent_descriptor,
new java.lang.String[] { "ValidatorPeriod", "Fraction", });
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_ValidatorSlashEvents_descriptor,
new java.lang.String[] { "ValidatorSlashEvents", });
internal_static_cosmos_distribution_v1beta1_FeePool_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_cosmos_distribution_v1beta1_FeePool_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_FeePool_descriptor,
new java.lang.String[] { "CommunityPool", });
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposal_descriptor,
new java.lang.String[] { "Title", "Description", "Recipient", "Amount", });
internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_DelegatorStartingInfo_descriptor,
new java.lang.String[] { "PreviousPeriod", "Stake", "Height", });
internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_DelegationDelegatorReward_descriptor,
new java.lang.String[] { "ValidatorAddress", "Reward", });
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_CommunityPoolSpendProposalWithDeposit_descriptor,
new java.lang.String[] { "Title", "Description", "Recipient", "Amount", "Deposit", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.castrepeated);
registry.add(com.google.protobuf.GoGoProtos.customtype);
registry.add(com.google.protobuf.GoGoProtos.equal);
registry.add(com.google.protobuf.GoGoProtos.equalAll);
registry.add(com.google.protobuf.GoGoProtos.goprotoGetters);
registry.add(com.google.protobuf.GoGoProtos.goprotoStringer);
registry.add(com.google.protobuf.GoGoProtos.jsontag);
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
cosmos.base.v1beta1.CoinOuterClass.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy