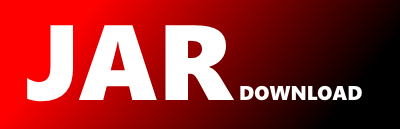
cosmos.distribution.v1beta1.Tx Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: cosmos/distribution/v1beta1/tx.proto
package cosmos.distribution.v1beta1;
public final class Tx {
private Tx() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface MsgSetWithdrawAddressOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgSetWithdrawAddress)
com.google.protobuf.MessageOrBuilder {
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
java.lang.String getWithdrawAddress();
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
com.google.protobuf.ByteString
getWithdrawAddressBytes();
}
/**
*
* MsgSetWithdrawAddress sets the withdraw address for
* a delegator (or validator self-delegation).
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgSetWithdrawAddress}
*/
public static final class MsgSetWithdrawAddress extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgSetWithdrawAddress)
MsgSetWithdrawAddressOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgSetWithdrawAddress.newBuilder() to construct.
private MsgSetWithdrawAddress(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgSetWithdrawAddress() {
delegatorAddress_ = "";
withdrawAddress_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgSetWithdrawAddress(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
withdrawAddress_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.class, cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.Builder.class);
}
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WITHDRAW_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object withdrawAddress_;
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public java.lang.String getWithdrawAddress() {
java.lang.Object ref = withdrawAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
withdrawAddress_ = s;
return s;
}
}
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public com.google.protobuf.ByteString
getWithdrawAddressBytes() {
java.lang.Object ref = withdrawAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
withdrawAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getWithdrawAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, withdrawAddress_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getWithdrawAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, withdrawAddress_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress other = (cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getWithdrawAddress()
.equals(other.getWithdrawAddress());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + WITHDRAW_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getWithdrawAddress().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgSetWithdrawAddress sets the withdraw address for
* a delegator (or validator self-delegation).
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgSetWithdrawAddress}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgSetWithdrawAddress)
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.class, cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
withdrawAddress_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress build() {
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress result = new cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress(this);
result.delegatorAddress_ = delegatorAddress_;
result.withdrawAddress_ = withdrawAddress_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getWithdrawAddress().isEmpty()) {
withdrawAddress_ = other.withdrawAddress_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object delegatorAddress_ = "";
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object withdrawAddress_ = "";
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public java.lang.String getWithdrawAddress() {
java.lang.Object ref = withdrawAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
withdrawAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public com.google.protobuf.ByteString
getWithdrawAddressBytes() {
java.lang.Object ref = withdrawAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
withdrawAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public Builder setWithdrawAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
withdrawAddress_ = value;
onChanged();
return this;
}
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public Builder clearWithdrawAddress() {
withdrawAddress_ = getDefaultInstance().getWithdrawAddress();
onChanged();
return this;
}
/**
* string withdraw_address = 2 [(.gogoproto.moretags) = "yaml:\"withdraw_address\""];
*/
public Builder setWithdrawAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
withdrawAddress_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgSetWithdrawAddress)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgSetWithdrawAddress)
private static final cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress();
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgSetWithdrawAddress parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgSetWithdrawAddress(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddress getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgSetWithdrawAddressResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgSetWithdrawAddressResponse defines the Msg/SetWithdrawAddress response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse}
*/
public static final class MsgSetWithdrawAddressResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse)
MsgSetWithdrawAddressResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgSetWithdrawAddressResponse.newBuilder() to construct.
private MsgSetWithdrawAddressResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgSetWithdrawAddressResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgSetWithdrawAddressResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.class, cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse other = (cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgSetWithdrawAddressResponse defines the Msg/SetWithdrawAddress response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse)
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.class, cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse build() {
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse result = new cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgSetWithdrawAddressResponse)
private static final cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse();
}
public static cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgSetWithdrawAddressResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgSetWithdrawAddressResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgSetWithdrawAddressResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgWithdrawDelegatorRewardOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward)
com.google.protobuf.MessageOrBuilder {
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
java.lang.String getValidatorAddress();
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
com.google.protobuf.ByteString
getValidatorAddressBytes();
}
/**
*
* MsgWithdrawDelegatorReward represents delegation withdrawal to a delegator
* from a single validator.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward}
*/
public static final class MsgWithdrawDelegatorReward extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward)
MsgWithdrawDelegatorRewardOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgWithdrawDelegatorReward.newBuilder() to construct.
private MsgWithdrawDelegatorReward(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgWithdrawDelegatorReward() {
delegatorAddress_ = "";
validatorAddress_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgWithdrawDelegatorReward(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
validatorAddress_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.Builder.class);
}
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object validatorAddress_;
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
}
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, validatorAddress_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, validatorAddress_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward other = (cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getValidatorAddress()
.equals(other.getValidatorAddress());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + VALIDATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorAddress().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgWithdrawDelegatorReward represents delegation withdrawal to a delegator
* from a single validator.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward)
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
validatorAddress_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward build() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward result = new cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward(this);
result.delegatorAddress_ = delegatorAddress_;
result.validatorAddress_ = validatorAddress_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getValidatorAddress().isEmpty()) {
validatorAddress_ = other.validatorAddress_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object delegatorAddress_ = "";
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorAddress_ = "";
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorAddress_ = value;
onChanged();
return this;
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder clearValidatorAddress() {
validatorAddress_ = getDefaultInstance().getValidatorAddress();
onChanged();
return this;
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorAddress_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgWithdrawDelegatorReward)
private static final cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward();
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgWithdrawDelegatorReward parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgWithdrawDelegatorReward(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorReward getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgWithdrawDelegatorRewardResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgWithdrawDelegatorRewardResponse defines the Msg/WithdrawDelegatorReward response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse}
*/
public static final class MsgWithdrawDelegatorRewardResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse)
MsgWithdrawDelegatorRewardResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgWithdrawDelegatorRewardResponse.newBuilder() to construct.
private MsgWithdrawDelegatorRewardResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgWithdrawDelegatorRewardResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgWithdrawDelegatorRewardResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse other = (cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgWithdrawDelegatorRewardResponse defines the Msg/WithdrawDelegatorReward response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse)
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse build() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse result = new cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgWithdrawDelegatorRewardResponse)
private static final cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse();
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgWithdrawDelegatorRewardResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgWithdrawDelegatorRewardResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawDelegatorRewardResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgWithdrawValidatorCommissionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission)
com.google.protobuf.MessageOrBuilder {
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
java.lang.String getValidatorAddress();
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
com.google.protobuf.ByteString
getValidatorAddressBytes();
}
/**
*
* MsgWithdrawValidatorCommission withdraws the full commission to the validator
* address.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission}
*/
public static final class MsgWithdrawValidatorCommission extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission)
MsgWithdrawValidatorCommissionOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgWithdrawValidatorCommission.newBuilder() to construct.
private MsgWithdrawValidatorCommission(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgWithdrawValidatorCommission() {
validatorAddress_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgWithdrawValidatorCommission(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
validatorAddress_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.Builder.class);
}
public static final int VALIDATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object validatorAddress_;
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
}
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getValidatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, validatorAddress_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getValidatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, validatorAddress_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission other = (cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission) obj;
boolean result = true;
result = result && getValidatorAddress()
.equals(other.getValidatorAddress());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VALIDATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorAddress().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgWithdrawValidatorCommission withdraws the full commission to the validator
* address.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission)
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
validatorAddress_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission build() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission result = new cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission(this);
result.validatorAddress_ = validatorAddress_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission.getDefaultInstance()) return this;
if (!other.getValidatorAddress().isEmpty()) {
validatorAddress_ = other.validatorAddress_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object validatorAddress_ = "";
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorAddress_ = value;
onChanged();
return this;
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder clearValidatorAddress() {
validatorAddress_ = getDefaultInstance().getValidatorAddress();
onChanged();
return this;
}
/**
* string validator_address = 1 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorAddress_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommission)
private static final cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission();
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgWithdrawValidatorCommission parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgWithdrawValidatorCommission(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommission getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgWithdrawValidatorCommissionResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgWithdrawValidatorCommissionResponse defines the Msg/WithdrawValidatorCommission response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse}
*/
public static final class MsgWithdrawValidatorCommissionResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse)
MsgWithdrawValidatorCommissionResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgWithdrawValidatorCommissionResponse.newBuilder() to construct.
private MsgWithdrawValidatorCommissionResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgWithdrawValidatorCommissionResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgWithdrawValidatorCommissionResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse other = (cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgWithdrawValidatorCommissionResponse defines the Msg/WithdrawValidatorCommission response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse)
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.class, cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse build() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse result = new cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgWithdrawValidatorCommissionResponse)
private static final cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse();
}
public static cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgWithdrawValidatorCommissionResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgWithdrawValidatorCommissionResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgWithdrawValidatorCommissionResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgFundCommunityPoolOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgFundCommunityPool)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List
getAmountList();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index);
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
int getAmountCount();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index);
/**
* string depositor = 2;
*/
java.lang.String getDepositor();
/**
* string depositor = 2;
*/
com.google.protobuf.ByteString
getDepositorBytes();
}
/**
*
* MsgFundCommunityPool allows an account to directly
* fund the community pool.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgFundCommunityPool}
*/
public static final class MsgFundCommunityPool extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgFundCommunityPool)
MsgFundCommunityPoolOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgFundCommunityPool.newBuilder() to construct.
private MsgFundCommunityPool(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgFundCommunityPool() {
amount_ = java.util.Collections.emptyList();
depositor_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgFundCommunityPool(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
amount_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
amount_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry));
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
depositor_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
amount_ = java.util.Collections.unmodifiableList(amount_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.class, cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.Builder.class);
}
private int bitField0_;
public static final int AMOUNT_FIELD_NUMBER = 1;
private java.util.List amount_;
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getAmountList() {
return amount_;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList() {
return amount_;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getAmountCount() {
return amount_.size();
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index) {
return amount_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index) {
return amount_.get(index);
}
public static final int DEPOSITOR_FIELD_NUMBER = 2;
private volatile java.lang.Object depositor_;
/**
* string depositor = 2;
*/
public java.lang.String getDepositor() {
java.lang.Object ref = depositor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
depositor_ = s;
return s;
}
}
/**
* string depositor = 2;
*/
public com.google.protobuf.ByteString
getDepositorBytes() {
java.lang.Object ref = depositor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
depositor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < amount_.size(); i++) {
output.writeMessage(1, amount_.get(i));
}
if (!getDepositorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, depositor_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < amount_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, amount_.get(i));
}
if (!getDepositorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, depositor_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool other = (cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool) obj;
boolean result = true;
result = result && getAmountList()
.equals(other.getAmountList());
result = result && getDepositor()
.equals(other.getDepositor());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAmountCount() > 0) {
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmountList().hashCode();
}
hash = (37 * hash) + DEPOSITOR_FIELD_NUMBER;
hash = (53 * hash) + getDepositor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgFundCommunityPool allows an account to directly
* fund the community pool.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgFundCommunityPool}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgFundCommunityPool)
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.class, cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAmountFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (amountBuilder_ == null) {
amount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
amountBuilder_.clear();
}
depositor_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool build() {
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool result = new cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (amountBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
amount_ = java.util.Collections.unmodifiableList(amount_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.amount_ = amount_;
} else {
result.amount_ = amountBuilder_.build();
}
result.depositor_ = depositor_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool.getDefaultInstance()) return this;
if (amountBuilder_ == null) {
if (!other.amount_.isEmpty()) {
if (amount_.isEmpty()) {
amount_ = other.amount_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAmountIsMutable();
amount_.addAll(other.amount_);
}
onChanged();
}
} else {
if (!other.amount_.isEmpty()) {
if (amountBuilder_.isEmpty()) {
amountBuilder_.dispose();
amountBuilder_ = null;
amount_ = other.amount_;
bitField0_ = (bitField0_ & ~0x00000001);
amountBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAmountFieldBuilder() : null;
} else {
amountBuilder_.addAllMessages(other.amount_);
}
}
}
if (!other.getDepositor().isEmpty()) {
depositor_ = other.depositor_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List amount_ =
java.util.Collections.emptyList();
private void ensureAmountIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
amount_ = new java.util.ArrayList(amount_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> amountBuilder_;
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getAmountList() {
if (amountBuilder_ == null) {
return java.util.Collections.unmodifiableList(amount_);
} else {
return amountBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getAmountCount() {
if (amountBuilder_ == null) {
return amount_.size();
} else {
return amountBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index) {
if (amountBuilder_ == null) {
return amount_.get(index);
} else {
return amountBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.set(index, value);
onChanged();
} else {
amountBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.set(index, builderForValue.build());
onChanged();
} else {
amountBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.add(value);
onChanged();
} else {
amountBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.add(index, value);
onChanged();
} else {
amountBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.add(builderForValue.build());
onChanged();
} else {
amountBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.add(index, builderForValue.build());
onChanged();
} else {
amountBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAllAmount(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.Coin> values) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, amount_);
onChanged();
} else {
amountBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder clearAmount() {
if (amountBuilder_ == null) {
amount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
amountBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder removeAmount(int index) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.remove(index);
onChanged();
} else {
amountBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getAmountBuilder(
int index) {
return getAmountFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index) {
if (amountBuilder_ == null) {
return amount_.get(index); } else {
return amountBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList() {
if (amountBuilder_ != null) {
return amountBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(amount_);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addAmountBuilder() {
return getAmountFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addAmountBuilder(
int index) {
return getAmountFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 1 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List
getAmountBuilderList() {
return getAmountFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountFieldBuilder() {
if (amountBuilder_ == null) {
amountBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
amount_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
amount_ = null;
}
return amountBuilder_;
}
private java.lang.Object depositor_ = "";
/**
* string depositor = 2;
*/
public java.lang.String getDepositor() {
java.lang.Object ref = depositor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
depositor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string depositor = 2;
*/
public com.google.protobuf.ByteString
getDepositorBytes() {
java.lang.Object ref = depositor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
depositor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string depositor = 2;
*/
public Builder setDepositor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
depositor_ = value;
onChanged();
return this;
}
/**
* string depositor = 2;
*/
public Builder clearDepositor() {
depositor_ = getDefaultInstance().getDepositor();
onChanged();
return this;
}
/**
* string depositor = 2;
*/
public Builder setDepositorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
depositor_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgFundCommunityPool)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgFundCommunityPool)
private static final cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool();
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgFundCommunityPool parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgFundCommunityPool(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPool getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgFundCommunityPoolResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgFundCommunityPoolResponse defines the Msg/FundCommunityPool response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse}
*/
public static final class MsgFundCommunityPoolResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse)
MsgFundCommunityPoolResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgFundCommunityPoolResponse.newBuilder() to construct.
private MsgFundCommunityPoolResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgFundCommunityPoolResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgFundCommunityPoolResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.class, cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse)) {
return super.equals(obj);
}
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse other = (cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgFundCommunityPoolResponse defines the Msg/FundCommunityPool response type.
*
*
* Protobuf type {@code cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse)
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.class, cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.Builder.class);
}
// Construct using cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.distribution.v1beta1.Tx.internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_descriptor;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse getDefaultInstanceForType() {
return cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse build() {
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse buildPartial() {
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse result = new cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse) {
return mergeFrom((cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse other) {
if (other == cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.distribution.v1beta1.MsgFundCommunityPoolResponse)
private static final cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse();
}
public static cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgFundCommunityPoolResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgFundCommunityPoolResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.distribution.v1beta1.Tx.MsgFundCommunityPoolResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n$cosmos/distribution/v1beta1/tx.proto\022\033" +
"cosmos.distribution.v1beta1\032\024gogoproto/g" +
"ogo.proto\032\036cosmos/base/v1beta1/coin.prot" +
"o\"\221\001\n\025MsgSetWithdrawAddress\0227\n\021delegator" +
"_address\030\001 \001(\tB\034\362\336\037\030yaml:\"delegator_addr" +
"ess\"\0225\n\020withdraw_address\030\002 \001(\tB\033\362\336\037\027yaml" +
":\"withdraw_address\":\010\350\240\037\000\210\240\037\000\"\037\n\035MsgSetW" +
"ithdrawAddressResponse\"\230\001\n\032MsgWithdrawDe" +
"legatorReward\0227\n\021delegator_address\030\001 \001(\t" +
"B\034\362\336\037\030yaml:\"delegator_address\"\0227\n\021valida" +
"tor_address\030\002 \001(\tB\034\362\336\037\030yaml:\"validator_a" +
"ddress\":\010\350\240\037\000\210\240\037\000\"$\n\"MsgWithdrawDelegato" +
"rRewardResponse\"c\n\036MsgWithdrawValidatorC" +
"ommission\0227\n\021validator_address\030\001 \001(\tB\034\362\336" +
"\037\030yaml:\"validator_address\":\010\350\240\037\000\210\240\037\000\"(\n&" +
"MsgWithdrawValidatorCommissionResponse\"\220" +
"\001\n\024MsgFundCommunityPool\022[\n\006amount\030\001 \003(\0132" +
"\031.cosmos.base.v1beta1.CoinB0\310\336\037\000\252\337\037(gith" +
"ub.com/cosmos/cosmos-sdk/types.Coins\022\021\n\t" +
"depositor\030\002 \001(\t:\010\350\240\037\000\210\240\037\000\"\036\n\034MsgFundComm" +
"unityPoolResponse2\310\004\n\003Msg\022\204\001\n\022SetWithdra" +
"wAddress\0222.cosmos.distribution.v1beta1.M" +
"sgSetWithdrawAddress\032:.cosmos.distributi" +
"on.v1beta1.MsgSetWithdrawAddressResponse" +
"\022\223\001\n\027WithdrawDelegatorReward\0227.cosmos.di" +
"stribution.v1beta1.MsgWithdrawDelegatorR" +
"eward\032?.cosmos.distribution.v1beta1.MsgW" +
"ithdrawDelegatorRewardResponse\022\237\001\n\033Withd" +
"rawValidatorCommission\022;.cosmos.distribu" +
"tion.v1beta1.MsgWithdrawValidatorCommiss" +
"ion\032C.cosmos.distribution.v1beta1.MsgWit" +
"hdrawValidatorCommissionResponse\022\201\001\n\021Fun" +
"dCommunityPool\0221.cosmos.distribution.v1b" +
"eta1.MsgFundCommunityPool\0329.cosmos.distr" +
"ibution.v1beta1.MsgFundCommunityPoolResp" +
"onseB7Z1github.com/cosmos/cosmos-sdk/x/d" +
"istribution/types\250\342\036\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
cosmos.base.v1beta1.CoinOuterClass.getDescriptor(),
}, assigner);
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddress_descriptor,
new java.lang.String[] { "DelegatorAddress", "WithdrawAddress", });
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgSetWithdrawAddressResponse_descriptor,
new java.lang.String[] { });
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorReward_descriptor,
new java.lang.String[] { "DelegatorAddress", "ValidatorAddress", });
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgWithdrawDelegatorRewardResponse_descriptor,
new java.lang.String[] { });
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommission_descriptor,
new java.lang.String[] { "ValidatorAddress", });
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgWithdrawValidatorCommissionResponse_descriptor,
new java.lang.String[] { });
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPool_descriptor,
new java.lang.String[] { "Amount", "Depositor", });
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_distribution_v1beta1_MsgFundCommunityPoolResponse_descriptor,
new java.lang.String[] { });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.castrepeated);
registry.add(com.google.protobuf.GoGoProtos.equal);
registry.add(com.google.protobuf.GoGoProtos.equalAll);
registry.add(com.google.protobuf.GoGoProtos.goprotoGetters);
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
cosmos.base.v1beta1.CoinOuterClass.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy