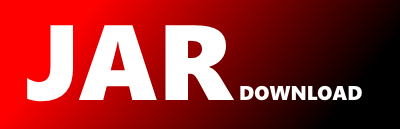
cosmos.gov.v1beta1.Gov Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: cosmos/gov/v1beta1/gov.proto
package cosmos.gov.v1beta1;
public final class Gov {
private Gov() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* VoteOption enumerates the valid vote options for a given governance proposal.
*
*
* Protobuf enum {@code cosmos.gov.v1beta1.VoteOption}
*/
public enum VoteOption
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* VOTE_OPTION_UNSPECIFIED defines a no-op vote option.
*
*
* VOTE_OPTION_UNSPECIFIED = 0 [(.gogoproto.enumvalue_customname) = "OptionEmpty"];
*/
VOTE_OPTION_UNSPECIFIED(0),
/**
*
* VOTE_OPTION_YES defines a yes vote option.
*
*
* VOTE_OPTION_YES = 1 [(.gogoproto.enumvalue_customname) = "OptionYes"];
*/
VOTE_OPTION_YES(1),
/**
*
* VOTE_OPTION_ABSTAIN defines an abstain vote option.
*
*
* VOTE_OPTION_ABSTAIN = 2 [(.gogoproto.enumvalue_customname) = "OptionAbstain"];
*/
VOTE_OPTION_ABSTAIN(2),
/**
*
* VOTE_OPTION_NO defines a no vote option.
*
*
* VOTE_OPTION_NO = 3 [(.gogoproto.enumvalue_customname) = "OptionNo"];
*/
VOTE_OPTION_NO(3),
/**
*
* VOTE_OPTION_NO_WITH_VETO defines a no with veto vote option.
*
*
* VOTE_OPTION_NO_WITH_VETO = 4 [(.gogoproto.enumvalue_customname) = "OptionNoWithVeto"];
*/
VOTE_OPTION_NO_WITH_VETO(4),
UNRECOGNIZED(-1),
;
/**
*
* VOTE_OPTION_UNSPECIFIED defines a no-op vote option.
*
*
* VOTE_OPTION_UNSPECIFIED = 0 [(.gogoproto.enumvalue_customname) = "OptionEmpty"];
*/
public static final int VOTE_OPTION_UNSPECIFIED_VALUE = 0;
/**
*
* VOTE_OPTION_YES defines a yes vote option.
*
*
* VOTE_OPTION_YES = 1 [(.gogoproto.enumvalue_customname) = "OptionYes"];
*/
public static final int VOTE_OPTION_YES_VALUE = 1;
/**
*
* VOTE_OPTION_ABSTAIN defines an abstain vote option.
*
*
* VOTE_OPTION_ABSTAIN = 2 [(.gogoproto.enumvalue_customname) = "OptionAbstain"];
*/
public static final int VOTE_OPTION_ABSTAIN_VALUE = 2;
/**
*
* VOTE_OPTION_NO defines a no vote option.
*
*
* VOTE_OPTION_NO = 3 [(.gogoproto.enumvalue_customname) = "OptionNo"];
*/
public static final int VOTE_OPTION_NO_VALUE = 3;
/**
*
* VOTE_OPTION_NO_WITH_VETO defines a no with veto vote option.
*
*
* VOTE_OPTION_NO_WITH_VETO = 4 [(.gogoproto.enumvalue_customname) = "OptionNoWithVeto"];
*/
public static final int VOTE_OPTION_NO_WITH_VETO_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static VoteOption valueOf(int value) {
return forNumber(value);
}
public static VoteOption forNumber(int value) {
switch (value) {
case 0: return VOTE_OPTION_UNSPECIFIED;
case 1: return VOTE_OPTION_YES;
case 2: return VOTE_OPTION_ABSTAIN;
case 3: return VOTE_OPTION_NO;
case 4: return VOTE_OPTION_NO_WITH_VETO;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
VoteOption> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public VoteOption findValueByNumber(int number) {
return VoteOption.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.getDescriptor().getEnumTypes().get(0);
}
private static final VoteOption[] VALUES = values();
public static VoteOption valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private VoteOption(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cosmos.gov.v1beta1.VoteOption)
}
/**
*
* ProposalStatus enumerates the valid statuses of a proposal.
*
*
* Protobuf enum {@code cosmos.gov.v1beta1.ProposalStatus}
*/
public enum ProposalStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* PROPOSAL_STATUS_UNSPECIFIED defines the default propopsal status.
*
*
* PROPOSAL_STATUS_UNSPECIFIED = 0 [(.gogoproto.enumvalue_customname) = "StatusNil"];
*/
PROPOSAL_STATUS_UNSPECIFIED(0),
/**
*
* PROPOSAL_STATUS_DEPOSIT_PERIOD defines a proposal status during the deposit
* period.
*
*
* PROPOSAL_STATUS_DEPOSIT_PERIOD = 1 [(.gogoproto.enumvalue_customname) = "StatusDepositPeriod"];
*/
PROPOSAL_STATUS_DEPOSIT_PERIOD(1),
/**
*
* PROPOSAL_STATUS_VOTING_PERIOD defines a proposal status during the voting
* period.
*
*
* PROPOSAL_STATUS_VOTING_PERIOD = 2 [(.gogoproto.enumvalue_customname) = "StatusVotingPeriod"];
*/
PROPOSAL_STATUS_VOTING_PERIOD(2),
/**
*
* PROPOSAL_STATUS_PASSED defines a proposal status of a proposal that has
* passed.
*
*
* PROPOSAL_STATUS_PASSED = 3 [(.gogoproto.enumvalue_customname) = "StatusPassed"];
*/
PROPOSAL_STATUS_PASSED(3),
/**
*
* PROPOSAL_STATUS_REJECTED defines a proposal status of a proposal that has
* been rejected.
*
*
* PROPOSAL_STATUS_REJECTED = 4 [(.gogoproto.enumvalue_customname) = "StatusRejected"];
*/
PROPOSAL_STATUS_REJECTED(4),
/**
*
* PROPOSAL_STATUS_FAILED defines a proposal status of a proposal that has
* failed.
*
*
* PROPOSAL_STATUS_FAILED = 5 [(.gogoproto.enumvalue_customname) = "StatusFailed"];
*/
PROPOSAL_STATUS_FAILED(5),
UNRECOGNIZED(-1),
;
/**
*
* PROPOSAL_STATUS_UNSPECIFIED defines the default propopsal status.
*
*
* PROPOSAL_STATUS_UNSPECIFIED = 0 [(.gogoproto.enumvalue_customname) = "StatusNil"];
*/
public static final int PROPOSAL_STATUS_UNSPECIFIED_VALUE = 0;
/**
*
* PROPOSAL_STATUS_DEPOSIT_PERIOD defines a proposal status during the deposit
* period.
*
*
* PROPOSAL_STATUS_DEPOSIT_PERIOD = 1 [(.gogoproto.enumvalue_customname) = "StatusDepositPeriod"];
*/
public static final int PROPOSAL_STATUS_DEPOSIT_PERIOD_VALUE = 1;
/**
*
* PROPOSAL_STATUS_VOTING_PERIOD defines a proposal status during the voting
* period.
*
*
* PROPOSAL_STATUS_VOTING_PERIOD = 2 [(.gogoproto.enumvalue_customname) = "StatusVotingPeriod"];
*/
public static final int PROPOSAL_STATUS_VOTING_PERIOD_VALUE = 2;
/**
*
* PROPOSAL_STATUS_PASSED defines a proposal status of a proposal that has
* passed.
*
*
* PROPOSAL_STATUS_PASSED = 3 [(.gogoproto.enumvalue_customname) = "StatusPassed"];
*/
public static final int PROPOSAL_STATUS_PASSED_VALUE = 3;
/**
*
* PROPOSAL_STATUS_REJECTED defines a proposal status of a proposal that has
* been rejected.
*
*
* PROPOSAL_STATUS_REJECTED = 4 [(.gogoproto.enumvalue_customname) = "StatusRejected"];
*/
public static final int PROPOSAL_STATUS_REJECTED_VALUE = 4;
/**
*
* PROPOSAL_STATUS_FAILED defines a proposal status of a proposal that has
* failed.
*
*
* PROPOSAL_STATUS_FAILED = 5 [(.gogoproto.enumvalue_customname) = "StatusFailed"];
*/
public static final int PROPOSAL_STATUS_FAILED_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProposalStatus valueOf(int value) {
return forNumber(value);
}
public static ProposalStatus forNumber(int value) {
switch (value) {
case 0: return PROPOSAL_STATUS_UNSPECIFIED;
case 1: return PROPOSAL_STATUS_DEPOSIT_PERIOD;
case 2: return PROPOSAL_STATUS_VOTING_PERIOD;
case 3: return PROPOSAL_STATUS_PASSED;
case 4: return PROPOSAL_STATUS_REJECTED;
case 5: return PROPOSAL_STATUS_FAILED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ProposalStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ProposalStatus findValueByNumber(int number) {
return ProposalStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.getDescriptor().getEnumTypes().get(1);
}
private static final ProposalStatus[] VALUES = values();
public static ProposalStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ProposalStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cosmos.gov.v1beta1.ProposalStatus)
}
public interface TextProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.TextProposal)
com.google.protobuf.MessageOrBuilder {
/**
* string title = 1;
*/
java.lang.String getTitle();
/**
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
* string description = 2;
*/
java.lang.String getDescription();
/**
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
*
* TextProposal defines a standard text proposal whose changes need to be
* manually updated in case of approval.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.TextProposal}
*/
public static final class TextProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.TextProposal)
TextProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use TextProposal.newBuilder() to construct.
private TextProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TextProposal() {
title_ = "";
description_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TextProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TextProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TextProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.TextProposal.class, cosmos.gov.v1beta1.Gov.TextProposal.Builder.class);
}
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.TextProposal)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.TextProposal other = (cosmos.gov.v1beta1.Gov.TextProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TextProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.TextProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TextProposal defines a standard text proposal whose changes need to be
* manually updated in case of approval.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.TextProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.TextProposal)
cosmos.gov.v1beta1.Gov.TextProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TextProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TextProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.TextProposal.class, cosmos.gov.v1beta1.Gov.TextProposal.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.TextProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TextProposal_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TextProposal getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.TextProposal.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TextProposal build() {
cosmos.gov.v1beta1.Gov.TextProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TextProposal buildPartial() {
cosmos.gov.v1beta1.Gov.TextProposal result = new cosmos.gov.v1beta1.Gov.TextProposal(this);
result.title_ = title_;
result.description_ = description_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.TextProposal) {
return mergeFrom((cosmos.gov.v1beta1.Gov.TextProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.TextProposal other) {
if (other == cosmos.gov.v1beta1.Gov.TextProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.TextProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.TextProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object title_ = "";
/**
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.TextProposal)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.TextProposal)
private static final cosmos.gov.v1beta1.Gov.TextProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.TextProposal();
}
public static cosmos.gov.v1beta1.Gov.TextProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TextProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TextProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TextProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DepositOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.Deposit)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
long getProposalId();
/**
* string depositor = 2;
*/
java.lang.String getDepositor();
/**
* string depositor = 2;
*/
com.google.protobuf.ByteString
getDepositorBytes();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List
getAmountList();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index);
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
int getAmountCount();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index);
}
/**
*
* Deposit defines an amount deposited by an account address to an active
* proposal.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.Deposit}
*/
public static final class Deposit extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.Deposit)
DepositOrBuilder {
private static final long serialVersionUID = 0L;
// Use Deposit.newBuilder() to construct.
private Deposit(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Deposit() {
proposalId_ = 0L;
depositor_ = "";
amount_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Deposit(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
proposalId_ = input.readUInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
depositor_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
amount_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
amount_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
amount_ = java.util.Collections.unmodifiableList(amount_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Deposit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Deposit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.Deposit.class, cosmos.gov.v1beta1.Gov.Deposit.Builder.class);
}
private int bitField0_;
public static final int PROPOSAL_ID_FIELD_NUMBER = 1;
private long proposalId_;
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public long getProposalId() {
return proposalId_;
}
public static final int DEPOSITOR_FIELD_NUMBER = 2;
private volatile java.lang.Object depositor_;
/**
* string depositor = 2;
*/
public java.lang.String getDepositor() {
java.lang.Object ref = depositor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
depositor_ = s;
return s;
}
}
/**
* string depositor = 2;
*/
public com.google.protobuf.ByteString
getDepositorBytes() {
java.lang.Object ref = depositor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
depositor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AMOUNT_FIELD_NUMBER = 3;
private java.util.List amount_;
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getAmountList() {
return amount_;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList() {
return amount_;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getAmountCount() {
return amount_.size();
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index) {
return amount_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index) {
return amount_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (proposalId_ != 0L) {
output.writeUInt64(1, proposalId_);
}
if (!getDepositorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, depositor_);
}
for (int i = 0; i < amount_.size(); i++) {
output.writeMessage(3, amount_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (proposalId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, proposalId_);
}
if (!getDepositorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, depositor_);
}
for (int i = 0; i < amount_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, amount_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.Deposit)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.Deposit other = (cosmos.gov.v1beta1.Gov.Deposit) obj;
boolean result = true;
result = result && (getProposalId()
== other.getProposalId());
result = result && getDepositor()
.equals(other.getDepositor());
result = result && getAmountList()
.equals(other.getAmountList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROPOSAL_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getProposalId());
hash = (37 * hash) + DEPOSITOR_FIELD_NUMBER;
hash = (53 * hash) + getDepositor().hashCode();
if (getAmountCount() > 0) {
hash = (37 * hash) + AMOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAmountList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Deposit parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.Deposit prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Deposit defines an amount deposited by an account address to an active
* proposal.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.Deposit}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.Deposit)
cosmos.gov.v1beta1.Gov.DepositOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Deposit_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Deposit_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.Deposit.class, cosmos.gov.v1beta1.Gov.Deposit.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.Deposit.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAmountFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
proposalId_ = 0L;
depositor_ = "";
if (amountBuilder_ == null) {
amount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
amountBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Deposit_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Deposit getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.Deposit.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Deposit build() {
cosmos.gov.v1beta1.Gov.Deposit result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Deposit buildPartial() {
cosmos.gov.v1beta1.Gov.Deposit result = new cosmos.gov.v1beta1.Gov.Deposit(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.proposalId_ = proposalId_;
result.depositor_ = depositor_;
if (amountBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
amount_ = java.util.Collections.unmodifiableList(amount_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.amount_ = amount_;
} else {
result.amount_ = amountBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.Deposit) {
return mergeFrom((cosmos.gov.v1beta1.Gov.Deposit)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.Deposit other) {
if (other == cosmos.gov.v1beta1.Gov.Deposit.getDefaultInstance()) return this;
if (other.getProposalId() != 0L) {
setProposalId(other.getProposalId());
}
if (!other.getDepositor().isEmpty()) {
depositor_ = other.depositor_;
onChanged();
}
if (amountBuilder_ == null) {
if (!other.amount_.isEmpty()) {
if (amount_.isEmpty()) {
amount_ = other.amount_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureAmountIsMutable();
amount_.addAll(other.amount_);
}
onChanged();
}
} else {
if (!other.amount_.isEmpty()) {
if (amountBuilder_.isEmpty()) {
amountBuilder_.dispose();
amountBuilder_ = null;
amount_ = other.amount_;
bitField0_ = (bitField0_ & ~0x00000004);
amountBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAmountFieldBuilder() : null;
} else {
amountBuilder_.addAllMessages(other.amount_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.Deposit parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.Deposit) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long proposalId_ ;
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public long getProposalId() {
return proposalId_;
}
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public Builder setProposalId(long value) {
proposalId_ = value;
onChanged();
return this;
}
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public Builder clearProposalId() {
proposalId_ = 0L;
onChanged();
return this;
}
private java.lang.Object depositor_ = "";
/**
* string depositor = 2;
*/
public java.lang.String getDepositor() {
java.lang.Object ref = depositor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
depositor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string depositor = 2;
*/
public com.google.protobuf.ByteString
getDepositorBytes() {
java.lang.Object ref = depositor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
depositor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string depositor = 2;
*/
public Builder setDepositor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
depositor_ = value;
onChanged();
return this;
}
/**
* string depositor = 2;
*/
public Builder clearDepositor() {
depositor_ = getDefaultInstance().getDepositor();
onChanged();
return this;
}
/**
* string depositor = 2;
*/
public Builder setDepositorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
depositor_ = value;
onChanged();
return this;
}
private java.util.List amount_ =
java.util.Collections.emptyList();
private void ensureAmountIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
amount_ = new java.util.ArrayList(amount_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> amountBuilder_;
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getAmountList() {
if (amountBuilder_ == null) {
return java.util.Collections.unmodifiableList(amount_);
} else {
return amountBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getAmountCount() {
if (amountBuilder_ == null) {
return amount_.size();
} else {
return amountBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getAmount(int index) {
if (amountBuilder_ == null) {
return amount_.get(index);
} else {
return amountBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.set(index, value);
onChanged();
} else {
amountBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.set(index, builderForValue.build());
onChanged();
} else {
amountBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.add(value);
onChanged();
} else {
amountBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (amountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAmountIsMutable();
amount_.add(index, value);
onChanged();
} else {
amountBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.add(builderForValue.build());
onChanged();
} else {
amountBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAmount(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.add(index, builderForValue.build());
onChanged();
} else {
amountBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAllAmount(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.Coin> values) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, amount_);
onChanged();
} else {
amountBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder clearAmount() {
if (amountBuilder_ == null) {
amount_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
amountBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder removeAmount(int index) {
if (amountBuilder_ == null) {
ensureAmountIsMutable();
amount_.remove(index);
onChanged();
} else {
amountBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getAmountBuilder(
int index) {
return getAmountFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getAmountOrBuilder(
int index) {
if (amountBuilder_ == null) {
return amount_.get(index); } else {
return amountBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountOrBuilderList() {
if (amountBuilder_ != null) {
return amountBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(amount_);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addAmountBuilder() {
return getAmountFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addAmountBuilder(
int index) {
return getAmountFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin amount = 3 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List
getAmountBuilderList() {
return getAmountFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getAmountFieldBuilder() {
if (amountBuilder_ == null) {
amountBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
amount_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
amount_ = null;
}
return amountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.Deposit)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.Deposit)
private static final cosmos.gov.v1beta1.Gov.Deposit DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.Deposit();
}
public static cosmos.gov.v1beta1.Gov.Deposit getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Deposit parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Deposit(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Deposit getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.Proposal)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 proposal_id = 1 [(.gogoproto.jsontag) = "id", (.gogoproto.moretags) = "yaml:\"id\""];
*/
long getProposalId();
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
boolean hasContent();
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
com.google.protobuf.Any getContent();
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
com.google.protobuf.AnyOrBuilder getContentOrBuilder();
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
int getStatusValue();
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
cosmos.gov.v1beta1.Gov.ProposalStatus getStatus();
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
boolean hasFinalTallyResult();
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
cosmos.gov.v1beta1.Gov.TallyResult getFinalTallyResult();
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
cosmos.gov.v1beta1.Gov.TallyResultOrBuilder getFinalTallyResultOrBuilder();
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasSubmitTime();
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getSubmitTime();
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getSubmitTimeOrBuilder();
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasDepositEndTime();
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getDepositEndTime();
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getDepositEndTimeOrBuilder();
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List
getTotalDepositList();
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getTotalDeposit(int index);
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
int getTotalDepositCount();
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getTotalDepositOrBuilderList();
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getTotalDepositOrBuilder(
int index);
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasVotingStartTime();
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getVotingStartTime();
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getVotingStartTimeOrBuilder();
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasVotingEndTime();
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getVotingEndTime();
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getVotingEndTimeOrBuilder();
}
/**
*
* Proposal defines the core field members of a governance proposal.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.Proposal}
*/
public static final class Proposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.Proposal)
ProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use Proposal.newBuilder() to construct.
private Proposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Proposal() {
proposalId_ = 0L;
status_ = 0;
totalDeposit_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Proposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
proposalId_ = input.readUInt64();
break;
}
case 18: {
com.google.protobuf.Any.Builder subBuilder = null;
if (content_ != null) {
subBuilder = content_.toBuilder();
}
content_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(content_);
content_ = subBuilder.buildPartial();
}
break;
}
case 24: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 34: {
cosmos.gov.v1beta1.Gov.TallyResult.Builder subBuilder = null;
if (finalTallyResult_ != null) {
subBuilder = finalTallyResult_.toBuilder();
}
finalTallyResult_ = input.readMessage(cosmos.gov.v1beta1.Gov.TallyResult.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(finalTallyResult_);
finalTallyResult_ = subBuilder.buildPartial();
}
break;
}
case 42: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (submitTime_ != null) {
subBuilder = submitTime_.toBuilder();
}
submitTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(submitTime_);
submitTime_ = subBuilder.buildPartial();
}
break;
}
case 50: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (depositEndTime_ != null) {
subBuilder = depositEndTime_.toBuilder();
}
depositEndTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(depositEndTime_);
depositEndTime_ = subBuilder.buildPartial();
}
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
totalDeposit_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
totalDeposit_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry));
break;
}
case 66: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (votingStartTime_ != null) {
subBuilder = votingStartTime_.toBuilder();
}
votingStartTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(votingStartTime_);
votingStartTime_ = subBuilder.buildPartial();
}
break;
}
case 74: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (votingEndTime_ != null) {
subBuilder = votingEndTime_.toBuilder();
}
votingEndTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(votingEndTime_);
votingEndTime_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
totalDeposit_ = java.util.Collections.unmodifiableList(totalDeposit_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Proposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Proposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.Proposal.class, cosmos.gov.v1beta1.Gov.Proposal.Builder.class);
}
private int bitField0_;
public static final int PROPOSAL_ID_FIELD_NUMBER = 1;
private long proposalId_;
/**
* uint64 proposal_id = 1 [(.gogoproto.jsontag) = "id", (.gogoproto.moretags) = "yaml:\"id\""];
*/
public long getProposalId() {
return proposalId_;
}
public static final int CONTENT_FIELD_NUMBER = 2;
private com.google.protobuf.Any content_;
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public boolean hasContent() {
return content_ != null;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public com.google.protobuf.Any getContent() {
return content_ == null ? com.google.protobuf.Any.getDefaultInstance() : content_;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public com.google.protobuf.AnyOrBuilder getContentOrBuilder() {
return getContent();
}
public static final int STATUS_FIELD_NUMBER = 3;
private int status_;
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public int getStatusValue() {
return status_;
}
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public cosmos.gov.v1beta1.Gov.ProposalStatus getStatus() {
@SuppressWarnings("deprecation")
cosmos.gov.v1beta1.Gov.ProposalStatus result = cosmos.gov.v1beta1.Gov.ProposalStatus.valueOf(status_);
return result == null ? cosmos.gov.v1beta1.Gov.ProposalStatus.UNRECOGNIZED : result;
}
public static final int FINAL_TALLY_RESULT_FIELD_NUMBER = 4;
private cosmos.gov.v1beta1.Gov.TallyResult finalTallyResult_;
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public boolean hasFinalTallyResult() {
return finalTallyResult_ != null;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public cosmos.gov.v1beta1.Gov.TallyResult getFinalTallyResult() {
return finalTallyResult_ == null ? cosmos.gov.v1beta1.Gov.TallyResult.getDefaultInstance() : finalTallyResult_;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public cosmos.gov.v1beta1.Gov.TallyResultOrBuilder getFinalTallyResultOrBuilder() {
return getFinalTallyResult();
}
public static final int SUBMIT_TIME_FIELD_NUMBER = 5;
private com.google.protobuf.Timestamp submitTime_;
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasSubmitTime() {
return submitTime_ != null;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getSubmitTime() {
return submitTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : submitTime_;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getSubmitTimeOrBuilder() {
return getSubmitTime();
}
public static final int DEPOSIT_END_TIME_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp depositEndTime_;
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasDepositEndTime() {
return depositEndTime_ != null;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getDepositEndTime() {
return depositEndTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : depositEndTime_;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getDepositEndTimeOrBuilder() {
return getDepositEndTime();
}
public static final int TOTAL_DEPOSIT_FIELD_NUMBER = 7;
private java.util.List totalDeposit_;
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getTotalDepositList() {
return totalDeposit_;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getTotalDepositOrBuilderList() {
return totalDeposit_;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getTotalDepositCount() {
return totalDeposit_.size();
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getTotalDeposit(int index) {
return totalDeposit_.get(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getTotalDepositOrBuilder(
int index) {
return totalDeposit_.get(index);
}
public static final int VOTING_START_TIME_FIELD_NUMBER = 8;
private com.google.protobuf.Timestamp votingStartTime_;
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasVotingStartTime() {
return votingStartTime_ != null;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getVotingStartTime() {
return votingStartTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : votingStartTime_;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getVotingStartTimeOrBuilder() {
return getVotingStartTime();
}
public static final int VOTING_END_TIME_FIELD_NUMBER = 9;
private com.google.protobuf.Timestamp votingEndTime_;
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasVotingEndTime() {
return votingEndTime_ != null;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getVotingEndTime() {
return votingEndTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : votingEndTime_;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getVotingEndTimeOrBuilder() {
return getVotingEndTime();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (proposalId_ != 0L) {
output.writeUInt64(1, proposalId_);
}
if (content_ != null) {
output.writeMessage(2, getContent());
}
if (status_ != cosmos.gov.v1beta1.Gov.ProposalStatus.PROPOSAL_STATUS_UNSPECIFIED.getNumber()) {
output.writeEnum(3, status_);
}
if (finalTallyResult_ != null) {
output.writeMessage(4, getFinalTallyResult());
}
if (submitTime_ != null) {
output.writeMessage(5, getSubmitTime());
}
if (depositEndTime_ != null) {
output.writeMessage(6, getDepositEndTime());
}
for (int i = 0; i < totalDeposit_.size(); i++) {
output.writeMessage(7, totalDeposit_.get(i));
}
if (votingStartTime_ != null) {
output.writeMessage(8, getVotingStartTime());
}
if (votingEndTime_ != null) {
output.writeMessage(9, getVotingEndTime());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (proposalId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, proposalId_);
}
if (content_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getContent());
}
if (status_ != cosmos.gov.v1beta1.Gov.ProposalStatus.PROPOSAL_STATUS_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, status_);
}
if (finalTallyResult_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getFinalTallyResult());
}
if (submitTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getSubmitTime());
}
if (depositEndTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getDepositEndTime());
}
for (int i = 0; i < totalDeposit_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, totalDeposit_.get(i));
}
if (votingStartTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getVotingStartTime());
}
if (votingEndTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getVotingEndTime());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.Proposal)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.Proposal other = (cosmos.gov.v1beta1.Gov.Proposal) obj;
boolean result = true;
result = result && (getProposalId()
== other.getProposalId());
result = result && (hasContent() == other.hasContent());
if (hasContent()) {
result = result && getContent()
.equals(other.getContent());
}
result = result && status_ == other.status_;
result = result && (hasFinalTallyResult() == other.hasFinalTallyResult());
if (hasFinalTallyResult()) {
result = result && getFinalTallyResult()
.equals(other.getFinalTallyResult());
}
result = result && (hasSubmitTime() == other.hasSubmitTime());
if (hasSubmitTime()) {
result = result && getSubmitTime()
.equals(other.getSubmitTime());
}
result = result && (hasDepositEndTime() == other.hasDepositEndTime());
if (hasDepositEndTime()) {
result = result && getDepositEndTime()
.equals(other.getDepositEndTime());
}
result = result && getTotalDepositList()
.equals(other.getTotalDepositList());
result = result && (hasVotingStartTime() == other.hasVotingStartTime());
if (hasVotingStartTime()) {
result = result && getVotingStartTime()
.equals(other.getVotingStartTime());
}
result = result && (hasVotingEndTime() == other.hasVotingEndTime());
if (hasVotingEndTime()) {
result = result && getVotingEndTime()
.equals(other.getVotingEndTime());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROPOSAL_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getProposalId());
if (hasContent()) {
hash = (37 * hash) + CONTENT_FIELD_NUMBER;
hash = (53 * hash) + getContent().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasFinalTallyResult()) {
hash = (37 * hash) + FINAL_TALLY_RESULT_FIELD_NUMBER;
hash = (53 * hash) + getFinalTallyResult().hashCode();
}
if (hasSubmitTime()) {
hash = (37 * hash) + SUBMIT_TIME_FIELD_NUMBER;
hash = (53 * hash) + getSubmitTime().hashCode();
}
if (hasDepositEndTime()) {
hash = (37 * hash) + DEPOSIT_END_TIME_FIELD_NUMBER;
hash = (53 * hash) + getDepositEndTime().hashCode();
}
if (getTotalDepositCount() > 0) {
hash = (37 * hash) + TOTAL_DEPOSIT_FIELD_NUMBER;
hash = (53 * hash) + getTotalDepositList().hashCode();
}
if (hasVotingStartTime()) {
hash = (37 * hash) + VOTING_START_TIME_FIELD_NUMBER;
hash = (53 * hash) + getVotingStartTime().hashCode();
}
if (hasVotingEndTime()) {
hash = (37 * hash) + VOTING_END_TIME_FIELD_NUMBER;
hash = (53 * hash) + getVotingEndTime().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Proposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.Proposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Proposal defines the core field members of a governance proposal.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.Proposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.Proposal)
cosmos.gov.v1beta1.Gov.ProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Proposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Proposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.Proposal.class, cosmos.gov.v1beta1.Gov.Proposal.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.Proposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTotalDepositFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
proposalId_ = 0L;
if (contentBuilder_ == null) {
content_ = null;
} else {
content_ = null;
contentBuilder_ = null;
}
status_ = 0;
if (finalTallyResultBuilder_ == null) {
finalTallyResult_ = null;
} else {
finalTallyResult_ = null;
finalTallyResultBuilder_ = null;
}
if (submitTimeBuilder_ == null) {
submitTime_ = null;
} else {
submitTime_ = null;
submitTimeBuilder_ = null;
}
if (depositEndTimeBuilder_ == null) {
depositEndTime_ = null;
} else {
depositEndTime_ = null;
depositEndTimeBuilder_ = null;
}
if (totalDepositBuilder_ == null) {
totalDeposit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
totalDepositBuilder_.clear();
}
if (votingStartTimeBuilder_ == null) {
votingStartTime_ = null;
} else {
votingStartTime_ = null;
votingStartTimeBuilder_ = null;
}
if (votingEndTimeBuilder_ == null) {
votingEndTime_ = null;
} else {
votingEndTime_ = null;
votingEndTimeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Proposal_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Proposal getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.Proposal.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Proposal build() {
cosmos.gov.v1beta1.Gov.Proposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Proposal buildPartial() {
cosmos.gov.v1beta1.Gov.Proposal result = new cosmos.gov.v1beta1.Gov.Proposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.proposalId_ = proposalId_;
if (contentBuilder_ == null) {
result.content_ = content_;
} else {
result.content_ = contentBuilder_.build();
}
result.status_ = status_;
if (finalTallyResultBuilder_ == null) {
result.finalTallyResult_ = finalTallyResult_;
} else {
result.finalTallyResult_ = finalTallyResultBuilder_.build();
}
if (submitTimeBuilder_ == null) {
result.submitTime_ = submitTime_;
} else {
result.submitTime_ = submitTimeBuilder_.build();
}
if (depositEndTimeBuilder_ == null) {
result.depositEndTime_ = depositEndTime_;
} else {
result.depositEndTime_ = depositEndTimeBuilder_.build();
}
if (totalDepositBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
totalDeposit_ = java.util.Collections.unmodifiableList(totalDeposit_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.totalDeposit_ = totalDeposit_;
} else {
result.totalDeposit_ = totalDepositBuilder_.build();
}
if (votingStartTimeBuilder_ == null) {
result.votingStartTime_ = votingStartTime_;
} else {
result.votingStartTime_ = votingStartTimeBuilder_.build();
}
if (votingEndTimeBuilder_ == null) {
result.votingEndTime_ = votingEndTime_;
} else {
result.votingEndTime_ = votingEndTimeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.Proposal) {
return mergeFrom((cosmos.gov.v1beta1.Gov.Proposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.Proposal other) {
if (other == cosmos.gov.v1beta1.Gov.Proposal.getDefaultInstance()) return this;
if (other.getProposalId() != 0L) {
setProposalId(other.getProposalId());
}
if (other.hasContent()) {
mergeContent(other.getContent());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasFinalTallyResult()) {
mergeFinalTallyResult(other.getFinalTallyResult());
}
if (other.hasSubmitTime()) {
mergeSubmitTime(other.getSubmitTime());
}
if (other.hasDepositEndTime()) {
mergeDepositEndTime(other.getDepositEndTime());
}
if (totalDepositBuilder_ == null) {
if (!other.totalDeposit_.isEmpty()) {
if (totalDeposit_.isEmpty()) {
totalDeposit_ = other.totalDeposit_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureTotalDepositIsMutable();
totalDeposit_.addAll(other.totalDeposit_);
}
onChanged();
}
} else {
if (!other.totalDeposit_.isEmpty()) {
if (totalDepositBuilder_.isEmpty()) {
totalDepositBuilder_.dispose();
totalDepositBuilder_ = null;
totalDeposit_ = other.totalDeposit_;
bitField0_ = (bitField0_ & ~0x00000040);
totalDepositBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTotalDepositFieldBuilder() : null;
} else {
totalDepositBuilder_.addAllMessages(other.totalDeposit_);
}
}
}
if (other.hasVotingStartTime()) {
mergeVotingStartTime(other.getVotingStartTime());
}
if (other.hasVotingEndTime()) {
mergeVotingEndTime(other.getVotingEndTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.Proposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.Proposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long proposalId_ ;
/**
* uint64 proposal_id = 1 [(.gogoproto.jsontag) = "id", (.gogoproto.moretags) = "yaml:\"id\""];
*/
public long getProposalId() {
return proposalId_;
}
/**
* uint64 proposal_id = 1 [(.gogoproto.jsontag) = "id", (.gogoproto.moretags) = "yaml:\"id\""];
*/
public Builder setProposalId(long value) {
proposalId_ = value;
onChanged();
return this;
}
/**
* uint64 proposal_id = 1 [(.gogoproto.jsontag) = "id", (.gogoproto.moretags) = "yaml:\"id\""];
*/
public Builder clearProposalId() {
proposalId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Any content_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> contentBuilder_;
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public boolean hasContent() {
return contentBuilder_ != null || content_ != null;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public com.google.protobuf.Any getContent() {
if (contentBuilder_ == null) {
return content_ == null ? com.google.protobuf.Any.getDefaultInstance() : content_;
} else {
return contentBuilder_.getMessage();
}
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public Builder setContent(com.google.protobuf.Any value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
content_ = value;
onChanged();
} else {
contentBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public Builder setContent(
com.google.protobuf.Any.Builder builderForValue) {
if (contentBuilder_ == null) {
content_ = builderForValue.build();
onChanged();
} else {
contentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public Builder mergeContent(com.google.protobuf.Any value) {
if (contentBuilder_ == null) {
if (content_ != null) {
content_ =
com.google.protobuf.Any.newBuilder(content_).mergeFrom(value).buildPartial();
} else {
content_ = value;
}
onChanged();
} else {
contentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public Builder clearContent() {
if (contentBuilder_ == null) {
content_ = null;
onChanged();
} else {
content_ = null;
contentBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public com.google.protobuf.Any.Builder getContentBuilder() {
onChanged();
return getContentFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
public com.google.protobuf.AnyOrBuilder getContentOrBuilder() {
if (contentBuilder_ != null) {
return contentBuilder_.getMessageOrBuilder();
} else {
return content_ == null ?
com.google.protobuf.Any.getDefaultInstance() : content_;
}
}
/**
* .google.protobuf.Any content = 2 [(.cosmos_proto.accepts_interface) = "Content"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getContentFieldBuilder() {
if (contentBuilder_ == null) {
contentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getContent(),
getParentForChildren(),
isClean());
content_ = null;
}
return contentBuilder_;
}
private int status_ = 0;
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public int getStatusValue() {
return status_;
}
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public cosmos.gov.v1beta1.Gov.ProposalStatus getStatus() {
@SuppressWarnings("deprecation")
cosmos.gov.v1beta1.Gov.ProposalStatus result = cosmos.gov.v1beta1.Gov.ProposalStatus.valueOf(status_);
return result == null ? cosmos.gov.v1beta1.Gov.ProposalStatus.UNRECOGNIZED : result;
}
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public Builder setStatus(cosmos.gov.v1beta1.Gov.ProposalStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
* .cosmos.gov.v1beta1.ProposalStatus status = 3 [(.gogoproto.moretags) = "yaml:\"proposal_status\""];
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private cosmos.gov.v1beta1.Gov.TallyResult finalTallyResult_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.gov.v1beta1.Gov.TallyResult, cosmos.gov.v1beta1.Gov.TallyResult.Builder, cosmos.gov.v1beta1.Gov.TallyResultOrBuilder> finalTallyResultBuilder_;
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public boolean hasFinalTallyResult() {
return finalTallyResultBuilder_ != null || finalTallyResult_ != null;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public cosmos.gov.v1beta1.Gov.TallyResult getFinalTallyResult() {
if (finalTallyResultBuilder_ == null) {
return finalTallyResult_ == null ? cosmos.gov.v1beta1.Gov.TallyResult.getDefaultInstance() : finalTallyResult_;
} else {
return finalTallyResultBuilder_.getMessage();
}
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public Builder setFinalTallyResult(cosmos.gov.v1beta1.Gov.TallyResult value) {
if (finalTallyResultBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
finalTallyResult_ = value;
onChanged();
} else {
finalTallyResultBuilder_.setMessage(value);
}
return this;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public Builder setFinalTallyResult(
cosmos.gov.v1beta1.Gov.TallyResult.Builder builderForValue) {
if (finalTallyResultBuilder_ == null) {
finalTallyResult_ = builderForValue.build();
onChanged();
} else {
finalTallyResultBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public Builder mergeFinalTallyResult(cosmos.gov.v1beta1.Gov.TallyResult value) {
if (finalTallyResultBuilder_ == null) {
if (finalTallyResult_ != null) {
finalTallyResult_ =
cosmos.gov.v1beta1.Gov.TallyResult.newBuilder(finalTallyResult_).mergeFrom(value).buildPartial();
} else {
finalTallyResult_ = value;
}
onChanged();
} else {
finalTallyResultBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public Builder clearFinalTallyResult() {
if (finalTallyResultBuilder_ == null) {
finalTallyResult_ = null;
onChanged();
} else {
finalTallyResult_ = null;
finalTallyResultBuilder_ = null;
}
return this;
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public cosmos.gov.v1beta1.Gov.TallyResult.Builder getFinalTallyResultBuilder() {
onChanged();
return getFinalTallyResultFieldBuilder().getBuilder();
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
public cosmos.gov.v1beta1.Gov.TallyResultOrBuilder getFinalTallyResultOrBuilder() {
if (finalTallyResultBuilder_ != null) {
return finalTallyResultBuilder_.getMessageOrBuilder();
} else {
return finalTallyResult_ == null ?
cosmos.gov.v1beta1.Gov.TallyResult.getDefaultInstance() : finalTallyResult_;
}
}
/**
* .cosmos.gov.v1beta1.TallyResult final_tally_result = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"final_tally_result\""];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.gov.v1beta1.Gov.TallyResult, cosmos.gov.v1beta1.Gov.TallyResult.Builder, cosmos.gov.v1beta1.Gov.TallyResultOrBuilder>
getFinalTallyResultFieldBuilder() {
if (finalTallyResultBuilder_ == null) {
finalTallyResultBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.gov.v1beta1.Gov.TallyResult, cosmos.gov.v1beta1.Gov.TallyResult.Builder, cosmos.gov.v1beta1.Gov.TallyResultOrBuilder>(
getFinalTallyResult(),
getParentForChildren(),
isClean());
finalTallyResult_ = null;
}
return finalTallyResultBuilder_;
}
private com.google.protobuf.Timestamp submitTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> submitTimeBuilder_;
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasSubmitTime() {
return submitTimeBuilder_ != null || submitTime_ != null;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getSubmitTime() {
if (submitTimeBuilder_ == null) {
return submitTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : submitTime_;
} else {
return submitTimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setSubmitTime(com.google.protobuf.Timestamp value) {
if (submitTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
submitTime_ = value;
onChanged();
} else {
submitTimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setSubmitTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (submitTimeBuilder_ == null) {
submitTime_ = builderForValue.build();
onChanged();
} else {
submitTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeSubmitTime(com.google.protobuf.Timestamp value) {
if (submitTimeBuilder_ == null) {
if (submitTime_ != null) {
submitTime_ =
com.google.protobuf.Timestamp.newBuilder(submitTime_).mergeFrom(value).buildPartial();
} else {
submitTime_ = value;
}
onChanged();
} else {
submitTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearSubmitTime() {
if (submitTimeBuilder_ == null) {
submitTime_ = null;
onChanged();
} else {
submitTime_ = null;
submitTimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getSubmitTimeBuilder() {
onChanged();
return getSubmitTimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getSubmitTimeOrBuilder() {
if (submitTimeBuilder_ != null) {
return submitTimeBuilder_.getMessageOrBuilder();
} else {
return submitTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : submitTime_;
}
}
/**
* .google.protobuf.Timestamp submit_time = 5 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"submit_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getSubmitTimeFieldBuilder() {
if (submitTimeBuilder_ == null) {
submitTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getSubmitTime(),
getParentForChildren(),
isClean());
submitTime_ = null;
}
return submitTimeBuilder_;
}
private com.google.protobuf.Timestamp depositEndTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> depositEndTimeBuilder_;
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasDepositEndTime() {
return depositEndTimeBuilder_ != null || depositEndTime_ != null;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getDepositEndTime() {
if (depositEndTimeBuilder_ == null) {
return depositEndTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : depositEndTime_;
} else {
return depositEndTimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setDepositEndTime(com.google.protobuf.Timestamp value) {
if (depositEndTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
depositEndTime_ = value;
onChanged();
} else {
depositEndTimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setDepositEndTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (depositEndTimeBuilder_ == null) {
depositEndTime_ = builderForValue.build();
onChanged();
} else {
depositEndTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeDepositEndTime(com.google.protobuf.Timestamp value) {
if (depositEndTimeBuilder_ == null) {
if (depositEndTime_ != null) {
depositEndTime_ =
com.google.protobuf.Timestamp.newBuilder(depositEndTime_).mergeFrom(value).buildPartial();
} else {
depositEndTime_ = value;
}
onChanged();
} else {
depositEndTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearDepositEndTime() {
if (depositEndTimeBuilder_ == null) {
depositEndTime_ = null;
onChanged();
} else {
depositEndTime_ = null;
depositEndTimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getDepositEndTimeBuilder() {
onChanged();
return getDepositEndTimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getDepositEndTimeOrBuilder() {
if (depositEndTimeBuilder_ != null) {
return depositEndTimeBuilder_.getMessageOrBuilder();
} else {
return depositEndTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : depositEndTime_;
}
}
/**
* .google.protobuf.Timestamp deposit_end_time = 6 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"deposit_end_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getDepositEndTimeFieldBuilder() {
if (depositEndTimeBuilder_ == null) {
depositEndTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getDepositEndTime(),
getParentForChildren(),
isClean());
depositEndTime_ = null;
}
return depositEndTimeBuilder_;
}
private java.util.List totalDeposit_ =
java.util.Collections.emptyList();
private void ensureTotalDepositIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
totalDeposit_ = new java.util.ArrayList(totalDeposit_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> totalDepositBuilder_;
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getTotalDepositList() {
if (totalDepositBuilder_ == null) {
return java.util.Collections.unmodifiableList(totalDeposit_);
} else {
return totalDepositBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getTotalDepositCount() {
if (totalDepositBuilder_ == null) {
return totalDeposit_.size();
} else {
return totalDepositBuilder_.getCount();
}
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getTotalDeposit(int index) {
if (totalDepositBuilder_ == null) {
return totalDeposit_.get(index);
} else {
return totalDepositBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setTotalDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (totalDepositBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTotalDepositIsMutable();
totalDeposit_.set(index, value);
onChanged();
} else {
totalDepositBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setTotalDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (totalDepositBuilder_ == null) {
ensureTotalDepositIsMutable();
totalDeposit_.set(index, builderForValue.build());
onChanged();
} else {
totalDepositBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addTotalDeposit(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (totalDepositBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTotalDepositIsMutable();
totalDeposit_.add(value);
onChanged();
} else {
totalDepositBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addTotalDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (totalDepositBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTotalDepositIsMutable();
totalDeposit_.add(index, value);
onChanged();
} else {
totalDepositBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addTotalDeposit(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (totalDepositBuilder_ == null) {
ensureTotalDepositIsMutable();
totalDeposit_.add(builderForValue.build());
onChanged();
} else {
totalDepositBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addTotalDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (totalDepositBuilder_ == null) {
ensureTotalDepositIsMutable();
totalDeposit_.add(index, builderForValue.build());
onChanged();
} else {
totalDepositBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAllTotalDeposit(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.Coin> values) {
if (totalDepositBuilder_ == null) {
ensureTotalDepositIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, totalDeposit_);
onChanged();
} else {
totalDepositBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder clearTotalDeposit() {
if (totalDepositBuilder_ == null) {
totalDeposit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
totalDepositBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder removeTotalDeposit(int index) {
if (totalDepositBuilder_ == null) {
ensureTotalDepositIsMutable();
totalDeposit_.remove(index);
onChanged();
} else {
totalDepositBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getTotalDepositBuilder(
int index) {
return getTotalDepositFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getTotalDepositOrBuilder(
int index) {
if (totalDepositBuilder_ == null) {
return totalDeposit_.get(index); } else {
return totalDepositBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getTotalDepositOrBuilderList() {
if (totalDepositBuilder_ != null) {
return totalDepositBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(totalDeposit_);
}
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addTotalDepositBuilder() {
return getTotalDepositFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addTotalDepositBuilder(
int index) {
return getTotalDepositFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
* repeated .cosmos.base.v1beta1.Coin total_deposit = 7 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"total_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List
getTotalDepositBuilderList() {
return getTotalDepositFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getTotalDepositFieldBuilder() {
if (totalDepositBuilder_ == null) {
totalDepositBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
totalDeposit_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
totalDeposit_ = null;
}
return totalDepositBuilder_;
}
private com.google.protobuf.Timestamp votingStartTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> votingStartTimeBuilder_;
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasVotingStartTime() {
return votingStartTimeBuilder_ != null || votingStartTime_ != null;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getVotingStartTime() {
if (votingStartTimeBuilder_ == null) {
return votingStartTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : votingStartTime_;
} else {
return votingStartTimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setVotingStartTime(com.google.protobuf.Timestamp value) {
if (votingStartTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
votingStartTime_ = value;
onChanged();
} else {
votingStartTimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setVotingStartTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (votingStartTimeBuilder_ == null) {
votingStartTime_ = builderForValue.build();
onChanged();
} else {
votingStartTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeVotingStartTime(com.google.protobuf.Timestamp value) {
if (votingStartTimeBuilder_ == null) {
if (votingStartTime_ != null) {
votingStartTime_ =
com.google.protobuf.Timestamp.newBuilder(votingStartTime_).mergeFrom(value).buildPartial();
} else {
votingStartTime_ = value;
}
onChanged();
} else {
votingStartTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearVotingStartTime() {
if (votingStartTimeBuilder_ == null) {
votingStartTime_ = null;
onChanged();
} else {
votingStartTime_ = null;
votingStartTimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getVotingStartTimeBuilder() {
onChanged();
return getVotingStartTimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getVotingStartTimeOrBuilder() {
if (votingStartTimeBuilder_ != null) {
return votingStartTimeBuilder_.getMessageOrBuilder();
} else {
return votingStartTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : votingStartTime_;
}
}
/**
* .google.protobuf.Timestamp voting_start_time = 8 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_start_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getVotingStartTimeFieldBuilder() {
if (votingStartTimeBuilder_ == null) {
votingStartTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getVotingStartTime(),
getParentForChildren(),
isClean());
votingStartTime_ = null;
}
return votingStartTimeBuilder_;
}
private com.google.protobuf.Timestamp votingEndTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> votingEndTimeBuilder_;
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasVotingEndTime() {
return votingEndTimeBuilder_ != null || votingEndTime_ != null;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getVotingEndTime() {
if (votingEndTimeBuilder_ == null) {
return votingEndTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : votingEndTime_;
} else {
return votingEndTimeBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setVotingEndTime(com.google.protobuf.Timestamp value) {
if (votingEndTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
votingEndTime_ = value;
onChanged();
} else {
votingEndTimeBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setVotingEndTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (votingEndTimeBuilder_ == null) {
votingEndTime_ = builderForValue.build();
onChanged();
} else {
votingEndTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeVotingEndTime(com.google.protobuf.Timestamp value) {
if (votingEndTimeBuilder_ == null) {
if (votingEndTime_ != null) {
votingEndTime_ =
com.google.protobuf.Timestamp.newBuilder(votingEndTime_).mergeFrom(value).buildPartial();
} else {
votingEndTime_ = value;
}
onChanged();
} else {
votingEndTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearVotingEndTime() {
if (votingEndTimeBuilder_ == null) {
votingEndTime_ = null;
onChanged();
} else {
votingEndTime_ = null;
votingEndTimeBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getVotingEndTimeBuilder() {
onChanged();
return getVotingEndTimeFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getVotingEndTimeOrBuilder() {
if (votingEndTimeBuilder_ != null) {
return votingEndTimeBuilder_.getMessageOrBuilder();
} else {
return votingEndTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : votingEndTime_;
}
}
/**
* .google.protobuf.Timestamp voting_end_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"voting_end_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getVotingEndTimeFieldBuilder() {
if (votingEndTimeBuilder_ == null) {
votingEndTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getVotingEndTime(),
getParentForChildren(),
isClean());
votingEndTime_ = null;
}
return votingEndTimeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.Proposal)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.Proposal)
private static final cosmos.gov.v1beta1.Gov.Proposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.Proposal();
}
public static cosmos.gov.v1beta1.Gov.Proposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Proposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Proposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Proposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TallyResultOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.TallyResult)
com.google.protobuf.MessageOrBuilder {
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
java.lang.String getYes();
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
com.google.protobuf.ByteString
getYesBytes();
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
java.lang.String getAbstain();
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
com.google.protobuf.ByteString
getAbstainBytes();
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
java.lang.String getNo();
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
com.google.protobuf.ByteString
getNoBytes();
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
java.lang.String getNoWithVeto();
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
com.google.protobuf.ByteString
getNoWithVetoBytes();
}
/**
*
* TallyResult defines a standard tally for a governance proposal.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.TallyResult}
*/
public static final class TallyResult extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.TallyResult)
TallyResultOrBuilder {
private static final long serialVersionUID = 0L;
// Use TallyResult.newBuilder() to construct.
private TallyResult(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TallyResult() {
yes_ = "";
abstain_ = "";
no_ = "";
noWithVeto_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TallyResult(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
yes_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
abstain_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
no_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
noWithVeto_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyResult_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyResult_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.TallyResult.class, cosmos.gov.v1beta1.Gov.TallyResult.Builder.class);
}
public static final int YES_FIELD_NUMBER = 1;
private volatile java.lang.Object yes_;
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getYes() {
java.lang.Object ref = yes_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
yes_ = s;
return s;
}
}
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getYesBytes() {
java.lang.Object ref = yes_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
yes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ABSTAIN_FIELD_NUMBER = 2;
private volatile java.lang.Object abstain_;
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getAbstain() {
java.lang.Object ref = abstain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
abstain_ = s;
return s;
}
}
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getAbstainBytes() {
java.lang.Object ref = abstain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
abstain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NO_FIELD_NUMBER = 3;
private volatile java.lang.Object no_;
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getNo() {
java.lang.Object ref = no_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
no_ = s;
return s;
}
}
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getNoBytes() {
java.lang.Object ref = no_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
no_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NO_WITH_VETO_FIELD_NUMBER = 4;
private volatile java.lang.Object noWithVeto_;
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public java.lang.String getNoWithVeto() {
java.lang.Object ref = noWithVeto_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
noWithVeto_ = s;
return s;
}
}
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public com.google.protobuf.ByteString
getNoWithVetoBytes() {
java.lang.Object ref = noWithVeto_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
noWithVeto_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getYesBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, yes_);
}
if (!getAbstainBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, abstain_);
}
if (!getNoBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, no_);
}
if (!getNoWithVetoBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, noWithVeto_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getYesBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, yes_);
}
if (!getAbstainBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, abstain_);
}
if (!getNoBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, no_);
}
if (!getNoWithVetoBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, noWithVeto_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.TallyResult)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.TallyResult other = (cosmos.gov.v1beta1.Gov.TallyResult) obj;
boolean result = true;
result = result && getYes()
.equals(other.getYes());
result = result && getAbstain()
.equals(other.getAbstain());
result = result && getNo()
.equals(other.getNo());
result = result && getNoWithVeto()
.equals(other.getNoWithVeto());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + YES_FIELD_NUMBER;
hash = (53 * hash) + getYes().hashCode();
hash = (37 * hash) + ABSTAIN_FIELD_NUMBER;
hash = (53 * hash) + getAbstain().hashCode();
hash = (37 * hash) + NO_FIELD_NUMBER;
hash = (53 * hash) + getNo().hashCode();
hash = (37 * hash) + NO_WITH_VETO_FIELD_NUMBER;
hash = (53 * hash) + getNoWithVeto().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TallyResult parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.TallyResult prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TallyResult defines a standard tally for a governance proposal.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.TallyResult}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.TallyResult)
cosmos.gov.v1beta1.Gov.TallyResultOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyResult_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyResult_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.TallyResult.class, cosmos.gov.v1beta1.Gov.TallyResult.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.TallyResult.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
yes_ = "";
abstain_ = "";
no_ = "";
noWithVeto_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyResult_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyResult getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.TallyResult.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyResult build() {
cosmos.gov.v1beta1.Gov.TallyResult result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyResult buildPartial() {
cosmos.gov.v1beta1.Gov.TallyResult result = new cosmos.gov.v1beta1.Gov.TallyResult(this);
result.yes_ = yes_;
result.abstain_ = abstain_;
result.no_ = no_;
result.noWithVeto_ = noWithVeto_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.TallyResult) {
return mergeFrom((cosmos.gov.v1beta1.Gov.TallyResult)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.TallyResult other) {
if (other == cosmos.gov.v1beta1.Gov.TallyResult.getDefaultInstance()) return this;
if (!other.getYes().isEmpty()) {
yes_ = other.yes_;
onChanged();
}
if (!other.getAbstain().isEmpty()) {
abstain_ = other.abstain_;
onChanged();
}
if (!other.getNo().isEmpty()) {
no_ = other.no_;
onChanged();
}
if (!other.getNoWithVeto().isEmpty()) {
noWithVeto_ = other.noWithVeto_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.TallyResult parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.TallyResult) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object yes_ = "";
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getYes() {
java.lang.Object ref = yes_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
yes_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getYesBytes() {
java.lang.Object ref = yes_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
yes_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setYes(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
yes_ = value;
onChanged();
return this;
}
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder clearYes() {
yes_ = getDefaultInstance().getYes();
onChanged();
return this;
}
/**
* string yes = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setYesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
yes_ = value;
onChanged();
return this;
}
private java.lang.Object abstain_ = "";
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getAbstain() {
java.lang.Object ref = abstain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
abstain_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getAbstainBytes() {
java.lang.Object ref = abstain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
abstain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setAbstain(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
abstain_ = value;
onChanged();
return this;
}
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder clearAbstain() {
abstain_ = getDefaultInstance().getAbstain();
onChanged();
return this;
}
/**
* string abstain = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setAbstainBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
abstain_ = value;
onChanged();
return this;
}
private java.lang.Object no_ = "";
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getNo() {
java.lang.Object ref = no_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
no_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getNoBytes() {
java.lang.Object ref = no_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
no_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setNo(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
no_ = value;
onChanged();
return this;
}
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder clearNo() {
no_ = getDefaultInstance().getNo();
onChanged();
return this;
}
/**
* string no = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setNoBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
no_ = value;
onChanged();
return this;
}
private java.lang.Object noWithVeto_ = "";
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public java.lang.String getNoWithVeto() {
java.lang.Object ref = noWithVeto_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
noWithVeto_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public com.google.protobuf.ByteString
getNoWithVetoBytes() {
java.lang.Object ref = noWithVeto_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
noWithVeto_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public Builder setNoWithVeto(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
noWithVeto_ = value;
onChanged();
return this;
}
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public Builder clearNoWithVeto() {
noWithVeto_ = getDefaultInstance().getNoWithVeto();
onChanged();
return this;
}
/**
* string no_with_veto = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"no_with_veto\""];
*/
public Builder setNoWithVetoBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
noWithVeto_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.TallyResult)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.TallyResult)
private static final cosmos.gov.v1beta1.Gov.TallyResult DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.TallyResult();
}
public static cosmos.gov.v1beta1.Gov.TallyResult getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TallyResult parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TallyResult(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyResult getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VoteOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.Vote)
com.google.protobuf.MessageOrBuilder {
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
long getProposalId();
/**
* string voter = 2;
*/
java.lang.String getVoter();
/**
* string voter = 2;
*/
com.google.protobuf.ByteString
getVoterBytes();
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
int getOptionValue();
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
cosmos.gov.v1beta1.Gov.VoteOption getOption();
}
/**
*
* Vote defines a vote on a governance proposal.
* A Vote consists of a proposal ID, the voter, and the vote option.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.Vote}
*/
public static final class Vote extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.Vote)
VoteOrBuilder {
private static final long serialVersionUID = 0L;
// Use Vote.newBuilder() to construct.
private Vote(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Vote() {
proposalId_ = 0L;
voter_ = "";
option_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Vote(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
proposalId_ = input.readUInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
voter_ = s;
break;
}
case 24: {
int rawValue = input.readEnum();
option_ = rawValue;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Vote_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Vote_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.Vote.class, cosmos.gov.v1beta1.Gov.Vote.Builder.class);
}
public static final int PROPOSAL_ID_FIELD_NUMBER = 1;
private long proposalId_;
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public long getProposalId() {
return proposalId_;
}
public static final int VOTER_FIELD_NUMBER = 2;
private volatile java.lang.Object voter_;
/**
* string voter = 2;
*/
public java.lang.String getVoter() {
java.lang.Object ref = voter_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
voter_ = s;
return s;
}
}
/**
* string voter = 2;
*/
public com.google.protobuf.ByteString
getVoterBytes() {
java.lang.Object ref = voter_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
voter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OPTION_FIELD_NUMBER = 3;
private int option_;
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public int getOptionValue() {
return option_;
}
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public cosmos.gov.v1beta1.Gov.VoteOption getOption() {
@SuppressWarnings("deprecation")
cosmos.gov.v1beta1.Gov.VoteOption result = cosmos.gov.v1beta1.Gov.VoteOption.valueOf(option_);
return result == null ? cosmos.gov.v1beta1.Gov.VoteOption.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (proposalId_ != 0L) {
output.writeUInt64(1, proposalId_);
}
if (!getVoterBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, voter_);
}
if (option_ != cosmos.gov.v1beta1.Gov.VoteOption.VOTE_OPTION_UNSPECIFIED.getNumber()) {
output.writeEnum(3, option_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (proposalId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, proposalId_);
}
if (!getVoterBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, voter_);
}
if (option_ != cosmos.gov.v1beta1.Gov.VoteOption.VOTE_OPTION_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, option_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.Vote)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.Vote other = (cosmos.gov.v1beta1.Gov.Vote) obj;
boolean result = true;
result = result && (getProposalId()
== other.getProposalId());
result = result && getVoter()
.equals(other.getVoter());
result = result && option_ == other.option_;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROPOSAL_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getProposalId());
hash = (37 * hash) + VOTER_FIELD_NUMBER;
hash = (53 * hash) + getVoter().hashCode();
hash = (37 * hash) + OPTION_FIELD_NUMBER;
hash = (53 * hash) + option_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Vote parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Vote parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.Vote parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.Vote prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Vote defines a vote on a governance proposal.
* A Vote consists of a proposal ID, the voter, and the vote option.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.Vote}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.Vote)
cosmos.gov.v1beta1.Gov.VoteOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Vote_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Vote_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.Vote.class, cosmos.gov.v1beta1.Gov.Vote.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.Vote.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
proposalId_ = 0L;
voter_ = "";
option_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_Vote_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Vote getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.Vote.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Vote build() {
cosmos.gov.v1beta1.Gov.Vote result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Vote buildPartial() {
cosmos.gov.v1beta1.Gov.Vote result = new cosmos.gov.v1beta1.Gov.Vote(this);
result.proposalId_ = proposalId_;
result.voter_ = voter_;
result.option_ = option_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.Vote) {
return mergeFrom((cosmos.gov.v1beta1.Gov.Vote)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.Vote other) {
if (other == cosmos.gov.v1beta1.Gov.Vote.getDefaultInstance()) return this;
if (other.getProposalId() != 0L) {
setProposalId(other.getProposalId());
}
if (!other.getVoter().isEmpty()) {
voter_ = other.voter_;
onChanged();
}
if (other.option_ != 0) {
setOptionValue(other.getOptionValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.Vote parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.Vote) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long proposalId_ ;
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public long getProposalId() {
return proposalId_;
}
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public Builder setProposalId(long value) {
proposalId_ = value;
onChanged();
return this;
}
/**
* uint64 proposal_id = 1 [(.gogoproto.moretags) = "yaml:\"proposal_id\""];
*/
public Builder clearProposalId() {
proposalId_ = 0L;
onChanged();
return this;
}
private java.lang.Object voter_ = "";
/**
* string voter = 2;
*/
public java.lang.String getVoter() {
java.lang.Object ref = voter_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
voter_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string voter = 2;
*/
public com.google.protobuf.ByteString
getVoterBytes() {
java.lang.Object ref = voter_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
voter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string voter = 2;
*/
public Builder setVoter(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
voter_ = value;
onChanged();
return this;
}
/**
* string voter = 2;
*/
public Builder clearVoter() {
voter_ = getDefaultInstance().getVoter();
onChanged();
return this;
}
/**
* string voter = 2;
*/
public Builder setVoterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
voter_ = value;
onChanged();
return this;
}
private int option_ = 0;
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public int getOptionValue() {
return option_;
}
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public Builder setOptionValue(int value) {
option_ = value;
onChanged();
return this;
}
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public cosmos.gov.v1beta1.Gov.VoteOption getOption() {
@SuppressWarnings("deprecation")
cosmos.gov.v1beta1.Gov.VoteOption result = cosmos.gov.v1beta1.Gov.VoteOption.valueOf(option_);
return result == null ? cosmos.gov.v1beta1.Gov.VoteOption.UNRECOGNIZED : result;
}
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public Builder setOption(cosmos.gov.v1beta1.Gov.VoteOption value) {
if (value == null) {
throw new NullPointerException();
}
option_ = value.getNumber();
onChanged();
return this;
}
/**
* .cosmos.gov.v1beta1.VoteOption option = 3;
*/
public Builder clearOption() {
option_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.Vote)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.Vote)
private static final cosmos.gov.v1beta1.Gov.Vote DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.Vote();
}
public static cosmos.gov.v1beta1.Gov.Vote getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Vote parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Vote(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.Vote getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DepositParamsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.DepositParams)
com.google.protobuf.MessageOrBuilder {
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List
getMinDepositList();
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getMinDeposit(int index);
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
int getMinDepositCount();
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getMinDepositOrBuilderList();
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getMinDepositOrBuilder(
int index);
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
boolean hasMaxDepositPeriod();
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.Duration getMaxDepositPeriod();
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getMaxDepositPeriodOrBuilder();
}
/**
*
* DepositParams defines the params for deposits on governance proposals.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.DepositParams}
*/
public static final class DepositParams extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.DepositParams)
DepositParamsOrBuilder {
private static final long serialVersionUID = 0L;
// Use DepositParams.newBuilder() to construct.
private DepositParams(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DepositParams() {
minDeposit_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DepositParams(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
minDeposit_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
minDeposit_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry));
break;
}
case 18: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (maxDepositPeriod_ != null) {
subBuilder = maxDepositPeriod_.toBuilder();
}
maxDepositPeriod_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(maxDepositPeriod_);
maxDepositPeriod_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
minDeposit_ = java.util.Collections.unmodifiableList(minDeposit_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_DepositParams_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_DepositParams_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.DepositParams.class, cosmos.gov.v1beta1.Gov.DepositParams.Builder.class);
}
private int bitField0_;
public static final int MIN_DEPOSIT_FIELD_NUMBER = 1;
private java.util.List minDeposit_;
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getMinDepositList() {
return minDeposit_;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getMinDepositOrBuilderList() {
return minDeposit_;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getMinDepositCount() {
return minDeposit_.size();
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getMinDeposit(int index) {
return minDeposit_.get(index);
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getMinDepositOrBuilder(
int index) {
return minDeposit_.get(index);
}
public static final int MAX_DEPOSIT_PERIOD_FIELD_NUMBER = 2;
private com.google.protobuf.Duration maxDepositPeriod_;
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public boolean hasMaxDepositPeriod() {
return maxDepositPeriod_ != null;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getMaxDepositPeriod() {
return maxDepositPeriod_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxDepositPeriod_;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getMaxDepositPeriodOrBuilder() {
return getMaxDepositPeriod();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < minDeposit_.size(); i++) {
output.writeMessage(1, minDeposit_.get(i));
}
if (maxDepositPeriod_ != null) {
output.writeMessage(2, getMaxDepositPeriod());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < minDeposit_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, minDeposit_.get(i));
}
if (maxDepositPeriod_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getMaxDepositPeriod());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.DepositParams)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.DepositParams other = (cosmos.gov.v1beta1.Gov.DepositParams) obj;
boolean result = true;
result = result && getMinDepositList()
.equals(other.getMinDepositList());
result = result && (hasMaxDepositPeriod() == other.hasMaxDepositPeriod());
if (hasMaxDepositPeriod()) {
result = result && getMaxDepositPeriod()
.equals(other.getMaxDepositPeriod());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMinDepositCount() > 0) {
hash = (37 * hash) + MIN_DEPOSIT_FIELD_NUMBER;
hash = (53 * hash) + getMinDepositList().hashCode();
}
if (hasMaxDepositPeriod()) {
hash = (37 * hash) + MAX_DEPOSIT_PERIOD_FIELD_NUMBER;
hash = (53 * hash) + getMaxDepositPeriod().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.DepositParams parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.DepositParams prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DepositParams defines the params for deposits on governance proposals.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.DepositParams}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.DepositParams)
cosmos.gov.v1beta1.Gov.DepositParamsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_DepositParams_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_DepositParams_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.DepositParams.class, cosmos.gov.v1beta1.Gov.DepositParams.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.DepositParams.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMinDepositFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (minDepositBuilder_ == null) {
minDeposit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
minDepositBuilder_.clear();
}
if (maxDepositPeriodBuilder_ == null) {
maxDepositPeriod_ = null;
} else {
maxDepositPeriod_ = null;
maxDepositPeriodBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_DepositParams_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.DepositParams getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.DepositParams.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.DepositParams build() {
cosmos.gov.v1beta1.Gov.DepositParams result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.DepositParams buildPartial() {
cosmos.gov.v1beta1.Gov.DepositParams result = new cosmos.gov.v1beta1.Gov.DepositParams(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (minDepositBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
minDeposit_ = java.util.Collections.unmodifiableList(minDeposit_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.minDeposit_ = minDeposit_;
} else {
result.minDeposit_ = minDepositBuilder_.build();
}
if (maxDepositPeriodBuilder_ == null) {
result.maxDepositPeriod_ = maxDepositPeriod_;
} else {
result.maxDepositPeriod_ = maxDepositPeriodBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.DepositParams) {
return mergeFrom((cosmos.gov.v1beta1.Gov.DepositParams)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.DepositParams other) {
if (other == cosmos.gov.v1beta1.Gov.DepositParams.getDefaultInstance()) return this;
if (minDepositBuilder_ == null) {
if (!other.minDeposit_.isEmpty()) {
if (minDeposit_.isEmpty()) {
minDeposit_ = other.minDeposit_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMinDepositIsMutable();
minDeposit_.addAll(other.minDeposit_);
}
onChanged();
}
} else {
if (!other.minDeposit_.isEmpty()) {
if (minDepositBuilder_.isEmpty()) {
minDepositBuilder_.dispose();
minDepositBuilder_ = null;
minDeposit_ = other.minDeposit_;
bitField0_ = (bitField0_ & ~0x00000001);
minDepositBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMinDepositFieldBuilder() : null;
} else {
minDepositBuilder_.addAllMessages(other.minDeposit_);
}
}
}
if (other.hasMaxDepositPeriod()) {
mergeMaxDepositPeriod(other.getMaxDepositPeriod());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.DepositParams parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.DepositParams) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List minDeposit_ =
java.util.Collections.emptyList();
private void ensureMinDepositIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
minDeposit_ = new java.util.ArrayList(minDeposit_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> minDepositBuilder_;
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getMinDepositList() {
if (minDepositBuilder_ == null) {
return java.util.Collections.unmodifiableList(minDeposit_);
} else {
return minDepositBuilder_.getMessageList();
}
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getMinDepositCount() {
if (minDepositBuilder_ == null) {
return minDeposit_.size();
} else {
return minDepositBuilder_.getCount();
}
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getMinDeposit(int index) {
if (minDepositBuilder_ == null) {
return minDeposit_.get(index);
} else {
return minDepositBuilder_.getMessage(index);
}
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setMinDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (minDepositBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMinDepositIsMutable();
minDeposit_.set(index, value);
onChanged();
} else {
minDepositBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setMinDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (minDepositBuilder_ == null) {
ensureMinDepositIsMutable();
minDeposit_.set(index, builderForValue.build());
onChanged();
} else {
minDepositBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addMinDeposit(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (minDepositBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMinDepositIsMutable();
minDeposit_.add(value);
onChanged();
} else {
minDepositBuilder_.addMessage(value);
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addMinDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (minDepositBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMinDepositIsMutable();
minDeposit_.add(index, value);
onChanged();
} else {
minDepositBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addMinDeposit(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (minDepositBuilder_ == null) {
ensureMinDepositIsMutable();
minDeposit_.add(builderForValue.build());
onChanged();
} else {
minDepositBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addMinDeposit(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (minDepositBuilder_ == null) {
ensureMinDepositIsMutable();
minDeposit_.add(index, builderForValue.build());
onChanged();
} else {
minDepositBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAllMinDeposit(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.Coin> values) {
if (minDepositBuilder_ == null) {
ensureMinDepositIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, minDeposit_);
onChanged();
} else {
minDepositBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder clearMinDeposit() {
if (minDepositBuilder_ == null) {
minDeposit_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
minDepositBuilder_.clear();
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder removeMinDeposit(int index) {
if (minDepositBuilder_ == null) {
ensureMinDepositIsMutable();
minDeposit_.remove(index);
onChanged();
} else {
minDepositBuilder_.remove(index);
}
return this;
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getMinDepositBuilder(
int index) {
return getMinDepositFieldBuilder().getBuilder(index);
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getMinDepositOrBuilder(
int index) {
if (minDepositBuilder_ == null) {
return minDeposit_.get(index); } else {
return minDepositBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getMinDepositOrBuilderList() {
if (minDepositBuilder_ != null) {
return minDepositBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(minDeposit_);
}
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addMinDepositBuilder() {
return getMinDepositFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addMinDepositBuilder(
int index) {
return getMinDepositFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
*
* Minimum deposit for a proposal to enter voting period.
*
*
* repeated .cosmos.base.v1beta1.Coin min_deposit = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "min_deposit,omitempty", (.gogoproto.moretags) = "yaml:\"min_deposit\"", (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List
getMinDepositBuilderList() {
return getMinDepositFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getMinDepositFieldBuilder() {
if (minDepositBuilder_ == null) {
minDepositBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
minDeposit_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
minDeposit_ = null;
}
return minDepositBuilder_;
}
private com.google.protobuf.Duration maxDepositPeriod_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> maxDepositPeriodBuilder_;
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public boolean hasMaxDepositPeriod() {
return maxDepositPeriodBuilder_ != null || maxDepositPeriod_ != null;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getMaxDepositPeriod() {
if (maxDepositPeriodBuilder_ == null) {
return maxDepositPeriod_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxDepositPeriod_;
} else {
return maxDepositPeriodBuilder_.getMessage();
}
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public Builder setMaxDepositPeriod(com.google.protobuf.Duration value) {
if (maxDepositPeriodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxDepositPeriod_ = value;
onChanged();
} else {
maxDepositPeriodBuilder_.setMessage(value);
}
return this;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public Builder setMaxDepositPeriod(
com.google.protobuf.Duration.Builder builderForValue) {
if (maxDepositPeriodBuilder_ == null) {
maxDepositPeriod_ = builderForValue.build();
onChanged();
} else {
maxDepositPeriodBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public Builder mergeMaxDepositPeriod(com.google.protobuf.Duration value) {
if (maxDepositPeriodBuilder_ == null) {
if (maxDepositPeriod_ != null) {
maxDepositPeriod_ =
com.google.protobuf.Duration.newBuilder(maxDepositPeriod_).mergeFrom(value).buildPartial();
} else {
maxDepositPeriod_ = value;
}
onChanged();
} else {
maxDepositPeriodBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public Builder clearMaxDepositPeriod() {
if (maxDepositPeriodBuilder_ == null) {
maxDepositPeriod_ = null;
onChanged();
} else {
maxDepositPeriod_ = null;
maxDepositPeriodBuilder_ = null;
}
return this;
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration.Builder getMaxDepositPeriodBuilder() {
onChanged();
return getMaxDepositPeriodFieldBuilder().getBuilder();
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getMaxDepositPeriodOrBuilder() {
if (maxDepositPeriodBuilder_ != null) {
return maxDepositPeriodBuilder_.getMessageOrBuilder();
} else {
return maxDepositPeriod_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : maxDepositPeriod_;
}
}
/**
*
* Maximum period for Atom holders to deposit on a proposal. Initial value: 2
* months.
*
*
* .google.protobuf.Duration max_deposit_period = 2 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "max_deposit_period,omitempty", (.gogoproto.moretags) = "yaml:\"max_deposit_period\"", (.gogoproto.stdduration) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getMaxDepositPeriodFieldBuilder() {
if (maxDepositPeriodBuilder_ == null) {
maxDepositPeriodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getMaxDepositPeriod(),
getParentForChildren(),
isClean());
maxDepositPeriod_ = null;
}
return maxDepositPeriodBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.DepositParams)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.DepositParams)
private static final cosmos.gov.v1beta1.Gov.DepositParams DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.DepositParams();
}
public static cosmos.gov.v1beta1.Gov.DepositParams getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DepositParams parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DepositParams(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.DepositParams getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface VotingParamsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.VotingParams)
com.google.protobuf.MessageOrBuilder {
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
boolean hasVotingPeriod();
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.Duration getVotingPeriod();
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getVotingPeriodOrBuilder();
}
/**
*
* VotingParams defines the params for voting on governance proposals.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.VotingParams}
*/
public static final class VotingParams extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.VotingParams)
VotingParamsOrBuilder {
private static final long serialVersionUID = 0L;
// Use VotingParams.newBuilder() to construct.
private VotingParams(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VotingParams() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private VotingParams(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (votingPeriod_ != null) {
subBuilder = votingPeriod_.toBuilder();
}
votingPeriod_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(votingPeriod_);
votingPeriod_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_VotingParams_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_VotingParams_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.VotingParams.class, cosmos.gov.v1beta1.Gov.VotingParams.Builder.class);
}
public static final int VOTING_PERIOD_FIELD_NUMBER = 1;
private com.google.protobuf.Duration votingPeriod_;
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public boolean hasVotingPeriod() {
return votingPeriod_ != null;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getVotingPeriod() {
return votingPeriod_ == null ? com.google.protobuf.Duration.getDefaultInstance() : votingPeriod_;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getVotingPeriodOrBuilder() {
return getVotingPeriod();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (votingPeriod_ != null) {
output.writeMessage(1, getVotingPeriod());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (votingPeriod_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getVotingPeriod());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.VotingParams)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.VotingParams other = (cosmos.gov.v1beta1.Gov.VotingParams) obj;
boolean result = true;
result = result && (hasVotingPeriod() == other.hasVotingPeriod());
if (hasVotingPeriod()) {
result = result && getVotingPeriod()
.equals(other.getVotingPeriod());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasVotingPeriod()) {
hash = (37 * hash) + VOTING_PERIOD_FIELD_NUMBER;
hash = (53 * hash) + getVotingPeriod().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.VotingParams parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.VotingParams prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* VotingParams defines the params for voting on governance proposals.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.VotingParams}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.VotingParams)
cosmos.gov.v1beta1.Gov.VotingParamsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_VotingParams_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_VotingParams_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.VotingParams.class, cosmos.gov.v1beta1.Gov.VotingParams.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.VotingParams.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (votingPeriodBuilder_ == null) {
votingPeriod_ = null;
} else {
votingPeriod_ = null;
votingPeriodBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_VotingParams_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.VotingParams getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.VotingParams.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.VotingParams build() {
cosmos.gov.v1beta1.Gov.VotingParams result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.VotingParams buildPartial() {
cosmos.gov.v1beta1.Gov.VotingParams result = new cosmos.gov.v1beta1.Gov.VotingParams(this);
if (votingPeriodBuilder_ == null) {
result.votingPeriod_ = votingPeriod_;
} else {
result.votingPeriod_ = votingPeriodBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.VotingParams) {
return mergeFrom((cosmos.gov.v1beta1.Gov.VotingParams)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.VotingParams other) {
if (other == cosmos.gov.v1beta1.Gov.VotingParams.getDefaultInstance()) return this;
if (other.hasVotingPeriod()) {
mergeVotingPeriod(other.getVotingPeriod());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.VotingParams parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.VotingParams) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.Duration votingPeriod_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> votingPeriodBuilder_;
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public boolean hasVotingPeriod() {
return votingPeriodBuilder_ != null || votingPeriod_ != null;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getVotingPeriod() {
if (votingPeriodBuilder_ == null) {
return votingPeriod_ == null ? com.google.protobuf.Duration.getDefaultInstance() : votingPeriod_;
} else {
return votingPeriodBuilder_.getMessage();
}
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public Builder setVotingPeriod(com.google.protobuf.Duration value) {
if (votingPeriodBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
votingPeriod_ = value;
onChanged();
} else {
votingPeriodBuilder_.setMessage(value);
}
return this;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public Builder setVotingPeriod(
com.google.protobuf.Duration.Builder builderForValue) {
if (votingPeriodBuilder_ == null) {
votingPeriod_ = builderForValue.build();
onChanged();
} else {
votingPeriodBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public Builder mergeVotingPeriod(com.google.protobuf.Duration value) {
if (votingPeriodBuilder_ == null) {
if (votingPeriod_ != null) {
votingPeriod_ =
com.google.protobuf.Duration.newBuilder(votingPeriod_).mergeFrom(value).buildPartial();
} else {
votingPeriod_ = value;
}
onChanged();
} else {
votingPeriodBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public Builder clearVotingPeriod() {
if (votingPeriodBuilder_ == null) {
votingPeriod_ = null;
onChanged();
} else {
votingPeriod_ = null;
votingPeriodBuilder_ = null;
}
return this;
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration.Builder getVotingPeriodBuilder() {
onChanged();
return getVotingPeriodFieldBuilder().getBuilder();
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getVotingPeriodOrBuilder() {
if (votingPeriodBuilder_ != null) {
return votingPeriodBuilder_.getMessageOrBuilder();
} else {
return votingPeriod_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : votingPeriod_;
}
}
/**
*
* Length of the voting period.
*
*
* .google.protobuf.Duration voting_period = 1 [(.gogoproto.nullable) = false, (.gogoproto.jsontag) = "voting_period,omitempty", (.gogoproto.moretags) = "yaml:\"voting_period\"", (.gogoproto.stdduration) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getVotingPeriodFieldBuilder() {
if (votingPeriodBuilder_ == null) {
votingPeriodBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getVotingPeriod(),
getParentForChildren(),
isClean());
votingPeriod_ = null;
}
return votingPeriodBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.VotingParams)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.VotingParams)
private static final cosmos.gov.v1beta1.Gov.VotingParams DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.VotingParams();
}
public static cosmos.gov.v1beta1.Gov.VotingParams getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VotingParams parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new VotingParams(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.VotingParams getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TallyParamsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.gov.v1beta1.TallyParams)
com.google.protobuf.MessageOrBuilder {
/**
*
* Minimum percentage of total stake needed to vote for a result to be
* considered valid.
*
*
* bytes quorum = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "quorum,omitempty"];
*/
com.google.protobuf.ByteString getQuorum();
/**
*
* Minimum proportion of Yes votes for proposal to pass. Default value: 0.5.
*
*
* bytes threshold = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "threshold,omitempty"];
*/
com.google.protobuf.ByteString getThreshold();
/**
*
* Minimum value of Veto votes to Total votes ratio for proposal to be
* vetoed. Default value: 1/3.
*
*
* bytes veto_threshold = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "veto_threshold,omitempty", (.gogoproto.moretags) = "yaml:\"veto_threshold\""];
*/
com.google.protobuf.ByteString getVetoThreshold();
}
/**
*
* TallyParams defines the params for tallying votes on governance proposals.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.TallyParams}
*/
public static final class TallyParams extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.gov.v1beta1.TallyParams)
TallyParamsOrBuilder {
private static final long serialVersionUID = 0L;
// Use TallyParams.newBuilder() to construct.
private TallyParams(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TallyParams() {
quorum_ = com.google.protobuf.ByteString.EMPTY;
threshold_ = com.google.protobuf.ByteString.EMPTY;
vetoThreshold_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TallyParams(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
quorum_ = input.readBytes();
break;
}
case 18: {
threshold_ = input.readBytes();
break;
}
case 26: {
vetoThreshold_ = input.readBytes();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyParams_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyParams_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.TallyParams.class, cosmos.gov.v1beta1.Gov.TallyParams.Builder.class);
}
public static final int QUORUM_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString quorum_;
/**
*
* Minimum percentage of total stake needed to vote for a result to be
* considered valid.
*
*
* bytes quorum = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "quorum,omitempty"];
*/
public com.google.protobuf.ByteString getQuorum() {
return quorum_;
}
public static final int THRESHOLD_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString threshold_;
/**
*
* Minimum proportion of Yes votes for proposal to pass. Default value: 0.5.
*
*
* bytes threshold = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "threshold,omitempty"];
*/
public com.google.protobuf.ByteString getThreshold() {
return threshold_;
}
public static final int VETO_THRESHOLD_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString vetoThreshold_;
/**
*
* Minimum value of Veto votes to Total votes ratio for proposal to be
* vetoed. Default value: 1/3.
*
*
* bytes veto_threshold = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "veto_threshold,omitempty", (.gogoproto.moretags) = "yaml:\"veto_threshold\""];
*/
public com.google.protobuf.ByteString getVetoThreshold() {
return vetoThreshold_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!quorum_.isEmpty()) {
output.writeBytes(1, quorum_);
}
if (!threshold_.isEmpty()) {
output.writeBytes(2, threshold_);
}
if (!vetoThreshold_.isEmpty()) {
output.writeBytes(3, vetoThreshold_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!quorum_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, quorum_);
}
if (!threshold_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, threshold_);
}
if (!vetoThreshold_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, vetoThreshold_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.gov.v1beta1.Gov.TallyParams)) {
return super.equals(obj);
}
cosmos.gov.v1beta1.Gov.TallyParams other = (cosmos.gov.v1beta1.Gov.TallyParams) obj;
boolean result = true;
result = result && getQuorum()
.equals(other.getQuorum());
result = result && getThreshold()
.equals(other.getThreshold());
result = result && getVetoThreshold()
.equals(other.getVetoThreshold());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + QUORUM_FIELD_NUMBER;
hash = (53 * hash) + getQuorum().hashCode();
hash = (37 * hash) + THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + getThreshold().hashCode();
hash = (37 * hash) + VETO_THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + getVetoThreshold().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.gov.v1beta1.Gov.TallyParams parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.gov.v1beta1.Gov.TallyParams prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TallyParams defines the params for tallying votes on governance proposals.
*
*
* Protobuf type {@code cosmos.gov.v1beta1.TallyParams}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.gov.v1beta1.TallyParams)
cosmos.gov.v1beta1.Gov.TallyParamsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyParams_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyParams_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.gov.v1beta1.Gov.TallyParams.class, cosmos.gov.v1beta1.Gov.TallyParams.Builder.class);
}
// Construct using cosmos.gov.v1beta1.Gov.TallyParams.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
quorum_ = com.google.protobuf.ByteString.EMPTY;
threshold_ = com.google.protobuf.ByteString.EMPTY;
vetoThreshold_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.gov.v1beta1.Gov.internal_static_cosmos_gov_v1beta1_TallyParams_descriptor;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyParams getDefaultInstanceForType() {
return cosmos.gov.v1beta1.Gov.TallyParams.getDefaultInstance();
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyParams build() {
cosmos.gov.v1beta1.Gov.TallyParams result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyParams buildPartial() {
cosmos.gov.v1beta1.Gov.TallyParams result = new cosmos.gov.v1beta1.Gov.TallyParams(this);
result.quorum_ = quorum_;
result.threshold_ = threshold_;
result.vetoThreshold_ = vetoThreshold_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.gov.v1beta1.Gov.TallyParams) {
return mergeFrom((cosmos.gov.v1beta1.Gov.TallyParams)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.gov.v1beta1.Gov.TallyParams other) {
if (other == cosmos.gov.v1beta1.Gov.TallyParams.getDefaultInstance()) return this;
if (other.getQuorum() != com.google.protobuf.ByteString.EMPTY) {
setQuorum(other.getQuorum());
}
if (other.getThreshold() != com.google.protobuf.ByteString.EMPTY) {
setThreshold(other.getThreshold());
}
if (other.getVetoThreshold() != com.google.protobuf.ByteString.EMPTY) {
setVetoThreshold(other.getVetoThreshold());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.gov.v1beta1.Gov.TallyParams parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.gov.v1beta1.Gov.TallyParams) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString quorum_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Minimum percentage of total stake needed to vote for a result to be
* considered valid.
*
*
* bytes quorum = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "quorum,omitempty"];
*/
public com.google.protobuf.ByteString getQuorum() {
return quorum_;
}
/**
*
* Minimum percentage of total stake needed to vote for a result to be
* considered valid.
*
*
* bytes quorum = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "quorum,omitempty"];
*/
public Builder setQuorum(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
quorum_ = value;
onChanged();
return this;
}
/**
*
* Minimum percentage of total stake needed to vote for a result to be
* considered valid.
*
*
* bytes quorum = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "quorum,omitempty"];
*/
public Builder clearQuorum() {
quorum_ = getDefaultInstance().getQuorum();
onChanged();
return this;
}
private com.google.protobuf.ByteString threshold_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Minimum proportion of Yes votes for proposal to pass. Default value: 0.5.
*
*
* bytes threshold = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "threshold,omitempty"];
*/
public com.google.protobuf.ByteString getThreshold() {
return threshold_;
}
/**
*
* Minimum proportion of Yes votes for proposal to pass. Default value: 0.5.
*
*
* bytes threshold = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "threshold,omitempty"];
*/
public Builder setThreshold(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
threshold_ = value;
onChanged();
return this;
}
/**
*
* Minimum proportion of Yes votes for proposal to pass. Default value: 0.5.
*
*
* bytes threshold = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "threshold,omitempty"];
*/
public Builder clearThreshold() {
threshold_ = getDefaultInstance().getThreshold();
onChanged();
return this;
}
private com.google.protobuf.ByteString vetoThreshold_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Minimum value of Veto votes to Total votes ratio for proposal to be
* vetoed. Default value: 1/3.
*
*
* bytes veto_threshold = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "veto_threshold,omitempty", (.gogoproto.moretags) = "yaml:\"veto_threshold\""];
*/
public com.google.protobuf.ByteString getVetoThreshold() {
return vetoThreshold_;
}
/**
*
* Minimum value of Veto votes to Total votes ratio for proposal to be
* vetoed. Default value: 1/3.
*
*
* bytes veto_threshold = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "veto_threshold,omitempty", (.gogoproto.moretags) = "yaml:\"veto_threshold\""];
*/
public Builder setVetoThreshold(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
vetoThreshold_ = value;
onChanged();
return this;
}
/**
*
* Minimum value of Veto votes to Total votes ratio for proposal to be
* vetoed. Default value: 1/3.
*
*
* bytes veto_threshold = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.jsontag) = "veto_threshold,omitempty", (.gogoproto.moretags) = "yaml:\"veto_threshold\""];
*/
public Builder clearVetoThreshold() {
vetoThreshold_ = getDefaultInstance().getVetoThreshold();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.gov.v1beta1.TallyParams)
}
// @@protoc_insertion_point(class_scope:cosmos.gov.v1beta1.TallyParams)
private static final cosmos.gov.v1beta1.Gov.TallyParams DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.gov.v1beta1.Gov.TallyParams();
}
public static cosmos.gov.v1beta1.Gov.TallyParams getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TallyParams parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TallyParams(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.gov.v1beta1.Gov.TallyParams getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_TextProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_TextProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_Deposit_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_Deposit_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_Proposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_Proposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_TallyResult_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_TallyResult_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_Vote_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_Vote_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_DepositParams_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_DepositParams_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_VotingParams_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_VotingParams_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_gov_v1beta1_TallyParams_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_gov_v1beta1_TallyParams_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\034cosmos/gov/v1beta1/gov.proto\022\022cosmos.g" +
"ov.v1beta1\032\036cosmos/base/v1beta1/coin.pro" +
"to\032\024gogoproto/gogo.proto\032\031cosmos_proto/c" +
"osmos.proto\032\037google/protobuf/timestamp.p" +
"roto\032\031google/protobuf/any.proto\032\036google/" +
"protobuf/duration.proto\"C\n\014TextProposal\022" +
"\r\n\005title\030\001 \001(\t\022\023\n\013description\030\002 \001(\t:\017\322\264-" +
"\007Content\350\240\037\001\"\260\001\n\007Deposit\022+\n\013proposal_id\030" +
"\001 \001(\004B\026\362\336\037\022yaml:\"proposal_id\"\022\021\n\tdeposit" +
"or\030\002 \001(\t\022[\n\006amount\030\003 \003(\0132\031.cosmos.base.v" +
"1beta1.CoinB0\310\336\037\000\252\337\037(github.com/cosmos/c" +
"osmos-sdk/types.Coins:\010\210\240\037\000\350\240\037\000\"\374\005\n\010Prop" +
"osal\022(\n\013proposal_id\030\001 \001(\004B\023\352\336\037\002id\362\336\037\tyam" +
"l:\"id\"\0222\n\007content\030\002 \001(\0132\024.google.protobu" +
"f.AnyB\013\312\264-\007Content\022N\n\006status\030\003 \001(\0162\".cos" +
"mos.gov.v1beta1.ProposalStatusB\032\362\336\037\026yaml" +
":\"proposal_status\"\022^\n\022final_tally_result" +
"\030\004 \001(\0132\037.cosmos.gov.v1beta1.TallyResultB" +
"!\310\336\037\000\362\336\037\031yaml:\"final_tally_result\"\022O\n\013su" +
"bmit_time\030\005 \001(\0132\032.google.protobuf.Timest" +
"ampB\036\220\337\037\001\310\336\037\000\362\336\037\022yaml:\"submit_time\"\022Y\n\020d" +
"eposit_end_time\030\006 \001(\0132\032.google.protobuf." +
"TimestampB#\220\337\037\001\310\336\037\000\362\336\037\027yaml:\"deposit_end" +
"_time\"\022z\n\rtotal_deposit\030\007 \003(\0132\031.cosmos.b" +
"ase.v1beta1.CoinBH\310\336\037\000\252\337\037(github.com/cos" +
"mos/cosmos-sdk/types.Coins\362\336\037\024yaml:\"tota" +
"l_deposit\"\022[\n\021voting_start_time\030\010 \001(\0132\032." +
"google.protobuf.TimestampB$\220\337\037\001\310\336\037\000\362\336\037\030y" +
"aml:\"voting_start_time\"\022W\n\017voting_end_ti" +
"me\030\t \001(\0132\032.google.protobuf.TimestampB\"\220\337" +
"\037\001\310\336\037\000\362\336\037\026yaml:\"voting_end_time\":\004\350\240\037\001\"\252" +
"\002\n\013TallyResult\022;\n\003yes\030\001 \001(\tB.\332\336\037&github." +
"com/cosmos/cosmos-sdk/types.Int\310\336\037\000\022?\n\007a" +
"bstain\030\002 \001(\tB.\332\336\037&github.com/cosmos/cosm" +
"os-sdk/types.Int\310\336\037\000\022:\n\002no\030\003 \001(\tB.\332\336\037&gi" +
"thub.com/cosmos/cosmos-sdk/types.Int\310\336\037\000" +
"\022[\n\014no_with_veto\030\004 \001(\tBE\332\336\037&github.com/c" +
"osmos/cosmos-sdk/types.Int\310\336\037\000\362\336\037\023yaml:\"" +
"no_with_veto\":\004\350\240\037\001\"|\n\004Vote\022+\n\013proposal_" +
"id\030\001 \001(\004B\026\362\336\037\022yaml:\"proposal_id\"\022\r\n\005vote" +
"r\030\002 \001(\t\022.\n\006option\030\003 \001(\0162\036.cosmos.gov.v1b" +
"eta1.VoteOption:\010\230\240\037\000\350\240\037\000\"\237\002\n\rDepositPar" +
"ams\022\217\001\n\013min_deposit\030\001 \003(\0132\031.cosmos.base." +
"v1beta1.CoinB_\310\336\037\000\252\337\037(github.com/cosmos/" +
"cosmos-sdk/types.Coins\362\336\037\022yaml:\"min_depo" +
"sit\"\352\336\037\025min_deposit,omitempty\022|\n\022max_dep" +
"osit_period\030\002 \001(\0132\031.google.protobuf.Dura" +
"tionBE\310\336\037\000\230\337\037\001\352\336\037\034max_deposit_period,omi" +
"tempty\362\336\037\031yaml:\"max_deposit_period\"\"}\n\014V" +
"otingParams\022m\n\rvoting_period\030\001 \001(\0132\031.goo" +
"gle.protobuf.DurationB;\310\336\037\000\230\337\037\001\352\336\037\027votin" +
"g_period,omitempty\362\336\037\024yaml:\"voting_perio" +
"d\"\"\270\002\n\013TallyParams\022R\n\006quorum\030\001 \001(\014BB\332\336\037&" +
"github.com/cosmos/cosmos-sdk/types.Dec\310\336" +
"\037\000\352\336\037\020quorum,omitempty\022X\n\tthreshold\030\002 \001(" +
"\014BE\332\336\037&github.com/cosmos/cosmos-sdk/type" +
"s.Dec\310\336\037\000\352\336\037\023threshold,omitempty\022{\n\016veto" +
"_threshold\030\003 \001(\014Bc\332\336\037&github.com/cosmos/" +
"cosmos-sdk/types.Dec\310\336\037\000\352\336\037\030veto_thresho" +
"ld,omitempty\362\336\037\025yaml:\"veto_threshold\"*\346\001" +
"\n\nVoteOption\022,\n\027VOTE_OPTION_UNSPECIFIED\020" +
"\000\032\017\212\235 \013OptionEmpty\022\"\n\017VOTE_OPTION_YES\020\001\032" +
"\r\212\235 \tOptionYes\022*\n\023VOTE_OPTION_ABSTAIN\020\002\032" +
"\021\212\235 \rOptionAbstain\022 \n\016VOTE_OPTION_NO\020\003\032\014" +
"\212\235 \010OptionNo\0222\n\030VOTE_OPTION_NO_WITH_VETO" +
"\020\004\032\024\212\235 \020OptionNoWithVeto\032\004\210\243\036\000*\314\002\n\016Propo" +
"salStatus\022.\n\033PROPOSAL_STATUS_UNSPECIFIED" +
"\020\000\032\r\212\235 \tStatusNil\022;\n\036PROPOSAL_STATUS_DEP" +
"OSIT_PERIOD\020\001\032\027\212\235 \023StatusDepositPeriod\0229" +
"\n\035PROPOSAL_STATUS_VOTING_PERIOD\020\002\032\026\212\235 \022S" +
"tatusVotingPeriod\022,\n\026PROPOSAL_STATUS_PAS" +
"SED\020\003\032\020\212\235 \014StatusPassed\0220\n\030PROPOSAL_STAT" +
"US_REJECTED\020\004\032\022\212\235 \016StatusRejected\022,\n\026PRO" +
"POSAL_STATUS_FAILED\020\005\032\020\212\235 \014StatusFailed\032" +
"\004\210\243\036\000B6Z(github.com/cosmos/cosmos-sdk/x/" +
"gov/types\330\341\036\000\200\342\036\000\310\341\036\000b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
cosmos.base.v1beta1.CoinOuterClass.getDescriptor(),
com.google.protobuf.GoGoProtos.getDescriptor(),
cosmos_proto.Cosmos.getDescriptor(),
com.google.protobuf.TimestampProto.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
com.google.protobuf.DurationProto.getDescriptor(),
}, assigner);
internal_static_cosmos_gov_v1beta1_TextProposal_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cosmos_gov_v1beta1_TextProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_TextProposal_descriptor,
new java.lang.String[] { "Title", "Description", });
internal_static_cosmos_gov_v1beta1_Deposit_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cosmos_gov_v1beta1_Deposit_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_Deposit_descriptor,
new java.lang.String[] { "ProposalId", "Depositor", "Amount", });
internal_static_cosmos_gov_v1beta1_Proposal_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_cosmos_gov_v1beta1_Proposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_Proposal_descriptor,
new java.lang.String[] { "ProposalId", "Content", "Status", "FinalTallyResult", "SubmitTime", "DepositEndTime", "TotalDeposit", "VotingStartTime", "VotingEndTime", });
internal_static_cosmos_gov_v1beta1_TallyResult_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_cosmos_gov_v1beta1_TallyResult_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_TallyResult_descriptor,
new java.lang.String[] { "Yes", "Abstain", "No", "NoWithVeto", });
internal_static_cosmos_gov_v1beta1_Vote_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_cosmos_gov_v1beta1_Vote_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_Vote_descriptor,
new java.lang.String[] { "ProposalId", "Voter", "Option", });
internal_static_cosmos_gov_v1beta1_DepositParams_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_cosmos_gov_v1beta1_DepositParams_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_DepositParams_descriptor,
new java.lang.String[] { "MinDeposit", "MaxDepositPeriod", });
internal_static_cosmos_gov_v1beta1_VotingParams_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_cosmos_gov_v1beta1_VotingParams_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_VotingParams_descriptor,
new java.lang.String[] { "VotingPeriod", });
internal_static_cosmos_gov_v1beta1_TallyParams_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_cosmos_gov_v1beta1_TallyParams_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_gov_v1beta1_TallyParams_descriptor,
new java.lang.String[] { "Quorum", "Threshold", "VetoThreshold", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(cosmos_proto.Cosmos.acceptsInterface);
registry.add(cosmos_proto.Cosmos.implementsInterface);
registry.add(com.google.protobuf.GoGoProtos.castrepeated);
registry.add(com.google.protobuf.GoGoProtos.customtype);
registry.add(com.google.protobuf.GoGoProtos.enumvalueCustomname);
registry.add(com.google.protobuf.GoGoProtos.equal);
registry.add(com.google.protobuf.GoGoProtos.goprotoEnumPrefix);
registry.add(com.google.protobuf.GoGoProtos.goprotoGetters);
registry.add(com.google.protobuf.GoGoProtos.goprotoGettersAll);
registry.add(com.google.protobuf.GoGoProtos.goprotoStringer);
registry.add(com.google.protobuf.GoGoProtos.goprotoStringerAll);
registry.add(com.google.protobuf.GoGoProtos.jsontag);
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
registry.add(com.google.protobuf.GoGoProtos.stdduration);
registry.add(com.google.protobuf.GoGoProtos.stdtime);
registry.add(com.google.protobuf.GoGoProtos.stringerAll);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
cosmos.base.v1beta1.CoinOuterClass.getDescriptor();
com.google.protobuf.GoGoProtos.getDescriptor();
cosmos_proto.Cosmos.getDescriptor();
com.google.protobuf.TimestampProto.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
com.google.protobuf.DurationProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy