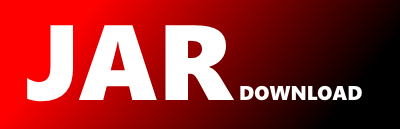
cosmos.slashing.v1beta1.Slashing Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: cosmos/slashing/v1beta1/slashing.proto
package cosmos.slashing.v1beta1;
public final class Slashing {
private Slashing() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ValidatorSigningInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.slashing.v1beta1.ValidatorSigningInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string address = 1;
*/
java.lang.String getAddress();
/**
* string address = 1;
*/
com.google.protobuf.ByteString
getAddressBytes();
/**
*
* height at which validator was first a candidate OR was unjailed
*
*
* int64 start_height = 2 [(.gogoproto.moretags) = "yaml:\"start_height\""];
*/
long getStartHeight();
/**
*
* index offset into signed block bit array
*
*
* int64 index_offset = 3 [(.gogoproto.moretags) = "yaml:\"index_offset\""];
*/
long getIndexOffset();
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
boolean hasJailedUntil();
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getJailedUntil();
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getJailedUntilOrBuilder();
/**
*
* whether or not a validator has been tombstoned (killed out of validator
* set)
*
*
* bool tombstoned = 5;
*/
boolean getTombstoned();
/**
*
* missed blocks counter (to avoid scanning the array every time)
*
*
* int64 missed_blocks_counter = 6 [(.gogoproto.moretags) = "yaml:\"missed_blocks_counter\""];
*/
long getMissedBlocksCounter();
}
/**
*
* ValidatorSigningInfo defines a validator's signing info for monitoring their
* liveness activity.
*
*
* Protobuf type {@code cosmos.slashing.v1beta1.ValidatorSigningInfo}
*/
public static final class ValidatorSigningInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.slashing.v1beta1.ValidatorSigningInfo)
ValidatorSigningInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValidatorSigningInfo.newBuilder() to construct.
private ValidatorSigningInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValidatorSigningInfo() {
address_ = "";
startHeight_ = 0L;
indexOffset_ = 0L;
tombstoned_ = false;
missedBlocksCounter_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValidatorSigningInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
address_ = s;
break;
}
case 16: {
startHeight_ = input.readInt64();
break;
}
case 24: {
indexOffset_ = input.readInt64();
break;
}
case 34: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (jailedUntil_ != null) {
subBuilder = jailedUntil_.toBuilder();
}
jailedUntil_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jailedUntil_);
jailedUntil_ = subBuilder.buildPartial();
}
break;
}
case 40: {
tombstoned_ = input.readBool();
break;
}
case 48: {
missedBlocksCounter_ = input.readInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.class, cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.Builder.class);
}
public static final int ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object address_;
/**
* string address = 1;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
}
}
/**
* string address = 1;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int START_HEIGHT_FIELD_NUMBER = 2;
private long startHeight_;
/**
*
* height at which validator was first a candidate OR was unjailed
*
*
* int64 start_height = 2 [(.gogoproto.moretags) = "yaml:\"start_height\""];
*/
public long getStartHeight() {
return startHeight_;
}
public static final int INDEX_OFFSET_FIELD_NUMBER = 3;
private long indexOffset_;
/**
*
* index offset into signed block bit array
*
*
* int64 index_offset = 3 [(.gogoproto.moretags) = "yaml:\"index_offset\""];
*/
public long getIndexOffset() {
return indexOffset_;
}
public static final int JAILED_UNTIL_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp jailedUntil_;
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public boolean hasJailedUntil() {
return jailedUntil_ != null;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getJailedUntil() {
return jailedUntil_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : jailedUntil_;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getJailedUntilOrBuilder() {
return getJailedUntil();
}
public static final int TOMBSTONED_FIELD_NUMBER = 5;
private boolean tombstoned_;
/**
*
* whether or not a validator has been tombstoned (killed out of validator
* set)
*
*
* bool tombstoned = 5;
*/
public boolean getTombstoned() {
return tombstoned_;
}
public static final int MISSED_BLOCKS_COUNTER_FIELD_NUMBER = 6;
private long missedBlocksCounter_;
/**
*
* missed blocks counter (to avoid scanning the array every time)
*
*
* int64 missed_blocks_counter = 6 [(.gogoproto.moretags) = "yaml:\"missed_blocks_counter\""];
*/
public long getMissedBlocksCounter() {
return missedBlocksCounter_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, address_);
}
if (startHeight_ != 0L) {
output.writeInt64(2, startHeight_);
}
if (indexOffset_ != 0L) {
output.writeInt64(3, indexOffset_);
}
if (jailedUntil_ != null) {
output.writeMessage(4, getJailedUntil());
}
if (tombstoned_ != false) {
output.writeBool(5, tombstoned_);
}
if (missedBlocksCounter_ != 0L) {
output.writeInt64(6, missedBlocksCounter_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, address_);
}
if (startHeight_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, startHeight_);
}
if (indexOffset_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, indexOffset_);
}
if (jailedUntil_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getJailedUntil());
}
if (tombstoned_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, tombstoned_);
}
if (missedBlocksCounter_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, missedBlocksCounter_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo)) {
return super.equals(obj);
}
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo other = (cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo) obj;
boolean result = true;
result = result && getAddress()
.equals(other.getAddress());
result = result && (getStartHeight()
== other.getStartHeight());
result = result && (getIndexOffset()
== other.getIndexOffset());
result = result && (hasJailedUntil() == other.hasJailedUntil());
if (hasJailedUntil()) {
result = result && getJailedUntil()
.equals(other.getJailedUntil());
}
result = result && (getTombstoned()
== other.getTombstoned());
result = result && (getMissedBlocksCounter()
== other.getMissedBlocksCounter());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getAddress().hashCode();
hash = (37 * hash) + START_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStartHeight());
hash = (37 * hash) + INDEX_OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndexOffset());
if (hasJailedUntil()) {
hash = (37 * hash) + JAILED_UNTIL_FIELD_NUMBER;
hash = (53 * hash) + getJailedUntil().hashCode();
}
hash = (37 * hash) + TOMBSTONED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTombstoned());
hash = (37 * hash) + MISSED_BLOCKS_COUNTER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMissedBlocksCounter());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValidatorSigningInfo defines a validator's signing info for monitoring their
* liveness activity.
*
*
* Protobuf type {@code cosmos.slashing.v1beta1.ValidatorSigningInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.slashing.v1beta1.ValidatorSigningInfo)
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.class, cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.Builder.class);
}
// Construct using cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
address_ = "";
startHeight_ = 0L;
indexOffset_ = 0L;
if (jailedUntilBuilder_ == null) {
jailedUntil_ = null;
} else {
jailedUntil_ = null;
jailedUntilBuilder_ = null;
}
tombstoned_ = false;
missedBlocksCounter_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_descriptor;
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo getDefaultInstanceForType() {
return cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.getDefaultInstance();
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo build() {
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo buildPartial() {
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo result = new cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo(this);
result.address_ = address_;
result.startHeight_ = startHeight_;
result.indexOffset_ = indexOffset_;
if (jailedUntilBuilder_ == null) {
result.jailedUntil_ = jailedUntil_;
} else {
result.jailedUntil_ = jailedUntilBuilder_.build();
}
result.tombstoned_ = tombstoned_;
result.missedBlocksCounter_ = missedBlocksCounter_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo) {
return mergeFrom((cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo other) {
if (other == cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo.getDefaultInstance()) return this;
if (!other.getAddress().isEmpty()) {
address_ = other.address_;
onChanged();
}
if (other.getStartHeight() != 0L) {
setStartHeight(other.getStartHeight());
}
if (other.getIndexOffset() != 0L) {
setIndexOffset(other.getIndexOffset());
}
if (other.hasJailedUntil()) {
mergeJailedUntil(other.getJailedUntil());
}
if (other.getTombstoned() != false) {
setTombstoned(other.getTombstoned());
}
if (other.getMissedBlocksCounter() != 0L) {
setMissedBlocksCounter(other.getMissedBlocksCounter());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object address_ = "";
/**
* string address = 1;
*/
public java.lang.String getAddress() {
java.lang.Object ref = address_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
address_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string address = 1;
*/
public com.google.protobuf.ByteString
getAddressBytes() {
java.lang.Object ref = address_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
address_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string address = 1;
*/
public Builder setAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
address_ = value;
onChanged();
return this;
}
/**
* string address = 1;
*/
public Builder clearAddress() {
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
/**
* string address = 1;
*/
public Builder setAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
address_ = value;
onChanged();
return this;
}
private long startHeight_ ;
/**
*
* height at which validator was first a candidate OR was unjailed
*
*
* int64 start_height = 2 [(.gogoproto.moretags) = "yaml:\"start_height\""];
*/
public long getStartHeight() {
return startHeight_;
}
/**
*
* height at which validator was first a candidate OR was unjailed
*
*
* int64 start_height = 2 [(.gogoproto.moretags) = "yaml:\"start_height\""];
*/
public Builder setStartHeight(long value) {
startHeight_ = value;
onChanged();
return this;
}
/**
*
* height at which validator was first a candidate OR was unjailed
*
*
* int64 start_height = 2 [(.gogoproto.moretags) = "yaml:\"start_height\""];
*/
public Builder clearStartHeight() {
startHeight_ = 0L;
onChanged();
return this;
}
private long indexOffset_ ;
/**
*
* index offset into signed block bit array
*
*
* int64 index_offset = 3 [(.gogoproto.moretags) = "yaml:\"index_offset\""];
*/
public long getIndexOffset() {
return indexOffset_;
}
/**
*
* index offset into signed block bit array
*
*
* int64 index_offset = 3 [(.gogoproto.moretags) = "yaml:\"index_offset\""];
*/
public Builder setIndexOffset(long value) {
indexOffset_ = value;
onChanged();
return this;
}
/**
*
* index offset into signed block bit array
*
*
* int64 index_offset = 3 [(.gogoproto.moretags) = "yaml:\"index_offset\""];
*/
public Builder clearIndexOffset() {
indexOffset_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Timestamp jailedUntil_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> jailedUntilBuilder_;
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public boolean hasJailedUntil() {
return jailedUntilBuilder_ != null || jailedUntil_ != null;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getJailedUntil() {
if (jailedUntilBuilder_ == null) {
return jailedUntil_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : jailedUntil_;
} else {
return jailedUntilBuilder_.getMessage();
}
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public Builder setJailedUntil(com.google.protobuf.Timestamp value) {
if (jailedUntilBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jailedUntil_ = value;
onChanged();
} else {
jailedUntilBuilder_.setMessage(value);
}
return this;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public Builder setJailedUntil(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (jailedUntilBuilder_ == null) {
jailedUntil_ = builderForValue.build();
onChanged();
} else {
jailedUntilBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeJailedUntil(com.google.protobuf.Timestamp value) {
if (jailedUntilBuilder_ == null) {
if (jailedUntil_ != null) {
jailedUntil_ =
com.google.protobuf.Timestamp.newBuilder(jailedUntil_).mergeFrom(value).buildPartial();
} else {
jailedUntil_ = value;
}
onChanged();
} else {
jailedUntilBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public Builder clearJailedUntil() {
if (jailedUntilBuilder_ == null) {
jailedUntil_ = null;
onChanged();
} else {
jailedUntil_ = null;
jailedUntilBuilder_ = null;
}
return this;
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getJailedUntilBuilder() {
onChanged();
return getJailedUntilFieldBuilder().getBuilder();
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getJailedUntilOrBuilder() {
if (jailedUntilBuilder_ != null) {
return jailedUntilBuilder_.getMessageOrBuilder();
} else {
return jailedUntil_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : jailedUntil_;
}
}
/**
*
* timestamp validator cannot be unjailed until
*
*
* .google.protobuf.Timestamp jailed_until = 4 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"jailed_until\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getJailedUntilFieldBuilder() {
if (jailedUntilBuilder_ == null) {
jailedUntilBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getJailedUntil(),
getParentForChildren(),
isClean());
jailedUntil_ = null;
}
return jailedUntilBuilder_;
}
private boolean tombstoned_ ;
/**
*
* whether or not a validator has been tombstoned (killed out of validator
* set)
*
*
* bool tombstoned = 5;
*/
public boolean getTombstoned() {
return tombstoned_;
}
/**
*
* whether or not a validator has been tombstoned (killed out of validator
* set)
*
*
* bool tombstoned = 5;
*/
public Builder setTombstoned(boolean value) {
tombstoned_ = value;
onChanged();
return this;
}
/**
*
* whether or not a validator has been tombstoned (killed out of validator
* set)
*
*
* bool tombstoned = 5;
*/
public Builder clearTombstoned() {
tombstoned_ = false;
onChanged();
return this;
}
private long missedBlocksCounter_ ;
/**
*
* missed blocks counter (to avoid scanning the array every time)
*
*
* int64 missed_blocks_counter = 6 [(.gogoproto.moretags) = "yaml:\"missed_blocks_counter\""];
*/
public long getMissedBlocksCounter() {
return missedBlocksCounter_;
}
/**
*
* missed blocks counter (to avoid scanning the array every time)
*
*
* int64 missed_blocks_counter = 6 [(.gogoproto.moretags) = "yaml:\"missed_blocks_counter\""];
*/
public Builder setMissedBlocksCounter(long value) {
missedBlocksCounter_ = value;
onChanged();
return this;
}
/**
*
* missed blocks counter (to avoid scanning the array every time)
*
*
* int64 missed_blocks_counter = 6 [(.gogoproto.moretags) = "yaml:\"missed_blocks_counter\""];
*/
public Builder clearMissedBlocksCounter() {
missedBlocksCounter_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.slashing.v1beta1.ValidatorSigningInfo)
}
// @@protoc_insertion_point(class_scope:cosmos.slashing.v1beta1.ValidatorSigningInfo)
private static final cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo();
}
public static cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValidatorSigningInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValidatorSigningInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.ValidatorSigningInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ParamsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.slashing.v1beta1.Params)
com.google.protobuf.MessageOrBuilder {
/**
* int64 signed_blocks_window = 1 [(.gogoproto.moretags) = "yaml:\"signed_blocks_window\""];
*/
long getSignedBlocksWindow();
/**
* bytes min_signed_per_window = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"min_signed_per_window\""];
*/
com.google.protobuf.ByteString getMinSignedPerWindow();
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
boolean hasDowntimeJailDuration();
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.Duration getDowntimeJailDuration();
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getDowntimeJailDurationOrBuilder();
/**
* bytes slash_fraction_double_sign = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_double_sign\""];
*/
com.google.protobuf.ByteString getSlashFractionDoubleSign();
/**
* bytes slash_fraction_downtime = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_downtime\""];
*/
com.google.protobuf.ByteString getSlashFractionDowntime();
}
/**
*
* Params represents the parameters used for by the slashing module.
*
*
* Protobuf type {@code cosmos.slashing.v1beta1.Params}
*/
public static final class Params extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.slashing.v1beta1.Params)
ParamsOrBuilder {
private static final long serialVersionUID = 0L;
// Use Params.newBuilder() to construct.
private Params(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Params() {
signedBlocksWindow_ = 0L;
minSignedPerWindow_ = com.google.protobuf.ByteString.EMPTY;
slashFractionDoubleSign_ = com.google.protobuf.ByteString.EMPTY;
slashFractionDowntime_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Params(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
signedBlocksWindow_ = input.readInt64();
break;
}
case 18: {
minSignedPerWindow_ = input.readBytes();
break;
}
case 26: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (downtimeJailDuration_ != null) {
subBuilder = downtimeJailDuration_.toBuilder();
}
downtimeJailDuration_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(downtimeJailDuration_);
downtimeJailDuration_ = subBuilder.buildPartial();
}
break;
}
case 34: {
slashFractionDoubleSign_ = input.readBytes();
break;
}
case 42: {
slashFractionDowntime_ = input.readBytes();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_Params_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_Params_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.slashing.v1beta1.Slashing.Params.class, cosmos.slashing.v1beta1.Slashing.Params.Builder.class);
}
public static final int SIGNED_BLOCKS_WINDOW_FIELD_NUMBER = 1;
private long signedBlocksWindow_;
/**
* int64 signed_blocks_window = 1 [(.gogoproto.moretags) = "yaml:\"signed_blocks_window\""];
*/
public long getSignedBlocksWindow() {
return signedBlocksWindow_;
}
public static final int MIN_SIGNED_PER_WINDOW_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString minSignedPerWindow_;
/**
* bytes min_signed_per_window = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"min_signed_per_window\""];
*/
public com.google.protobuf.ByteString getMinSignedPerWindow() {
return minSignedPerWindow_;
}
public static final int DOWNTIME_JAIL_DURATION_FIELD_NUMBER = 3;
private com.google.protobuf.Duration downtimeJailDuration_;
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public boolean hasDowntimeJailDuration() {
return downtimeJailDuration_ != null;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getDowntimeJailDuration() {
return downtimeJailDuration_ == null ? com.google.protobuf.Duration.getDefaultInstance() : downtimeJailDuration_;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getDowntimeJailDurationOrBuilder() {
return getDowntimeJailDuration();
}
public static final int SLASH_FRACTION_DOUBLE_SIGN_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString slashFractionDoubleSign_;
/**
* bytes slash_fraction_double_sign = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_double_sign\""];
*/
public com.google.protobuf.ByteString getSlashFractionDoubleSign() {
return slashFractionDoubleSign_;
}
public static final int SLASH_FRACTION_DOWNTIME_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString slashFractionDowntime_;
/**
* bytes slash_fraction_downtime = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_downtime\""];
*/
public com.google.protobuf.ByteString getSlashFractionDowntime() {
return slashFractionDowntime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (signedBlocksWindow_ != 0L) {
output.writeInt64(1, signedBlocksWindow_);
}
if (!minSignedPerWindow_.isEmpty()) {
output.writeBytes(2, minSignedPerWindow_);
}
if (downtimeJailDuration_ != null) {
output.writeMessage(3, getDowntimeJailDuration());
}
if (!slashFractionDoubleSign_.isEmpty()) {
output.writeBytes(4, slashFractionDoubleSign_);
}
if (!slashFractionDowntime_.isEmpty()) {
output.writeBytes(5, slashFractionDowntime_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (signedBlocksWindow_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, signedBlocksWindow_);
}
if (!minSignedPerWindow_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, minSignedPerWindow_);
}
if (downtimeJailDuration_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getDowntimeJailDuration());
}
if (!slashFractionDoubleSign_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, slashFractionDoubleSign_);
}
if (!slashFractionDowntime_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, slashFractionDowntime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.slashing.v1beta1.Slashing.Params)) {
return super.equals(obj);
}
cosmos.slashing.v1beta1.Slashing.Params other = (cosmos.slashing.v1beta1.Slashing.Params) obj;
boolean result = true;
result = result && (getSignedBlocksWindow()
== other.getSignedBlocksWindow());
result = result && getMinSignedPerWindow()
.equals(other.getMinSignedPerWindow());
result = result && (hasDowntimeJailDuration() == other.hasDowntimeJailDuration());
if (hasDowntimeJailDuration()) {
result = result && getDowntimeJailDuration()
.equals(other.getDowntimeJailDuration());
}
result = result && getSlashFractionDoubleSign()
.equals(other.getSlashFractionDoubleSign());
result = result && getSlashFractionDowntime()
.equals(other.getSlashFractionDowntime());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SIGNED_BLOCKS_WINDOW_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSignedBlocksWindow());
hash = (37 * hash) + MIN_SIGNED_PER_WINDOW_FIELD_NUMBER;
hash = (53 * hash) + getMinSignedPerWindow().hashCode();
if (hasDowntimeJailDuration()) {
hash = (37 * hash) + DOWNTIME_JAIL_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getDowntimeJailDuration().hashCode();
}
hash = (37 * hash) + SLASH_FRACTION_DOUBLE_SIGN_FIELD_NUMBER;
hash = (53 * hash) + getSlashFractionDoubleSign().hashCode();
hash = (37 * hash) + SLASH_FRACTION_DOWNTIME_FIELD_NUMBER;
hash = (53 * hash) + getSlashFractionDowntime().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.slashing.v1beta1.Slashing.Params parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.slashing.v1beta1.Slashing.Params prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Params represents the parameters used for by the slashing module.
*
*
* Protobuf type {@code cosmos.slashing.v1beta1.Params}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.slashing.v1beta1.Params)
cosmos.slashing.v1beta1.Slashing.ParamsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_Params_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_Params_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.slashing.v1beta1.Slashing.Params.class, cosmos.slashing.v1beta1.Slashing.Params.Builder.class);
}
// Construct using cosmos.slashing.v1beta1.Slashing.Params.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
signedBlocksWindow_ = 0L;
minSignedPerWindow_ = com.google.protobuf.ByteString.EMPTY;
if (downtimeJailDurationBuilder_ == null) {
downtimeJailDuration_ = null;
} else {
downtimeJailDuration_ = null;
downtimeJailDurationBuilder_ = null;
}
slashFractionDoubleSign_ = com.google.protobuf.ByteString.EMPTY;
slashFractionDowntime_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.slashing.v1beta1.Slashing.internal_static_cosmos_slashing_v1beta1_Params_descriptor;
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.Params getDefaultInstanceForType() {
return cosmos.slashing.v1beta1.Slashing.Params.getDefaultInstance();
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.Params build() {
cosmos.slashing.v1beta1.Slashing.Params result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.Params buildPartial() {
cosmos.slashing.v1beta1.Slashing.Params result = new cosmos.slashing.v1beta1.Slashing.Params(this);
result.signedBlocksWindow_ = signedBlocksWindow_;
result.minSignedPerWindow_ = minSignedPerWindow_;
if (downtimeJailDurationBuilder_ == null) {
result.downtimeJailDuration_ = downtimeJailDuration_;
} else {
result.downtimeJailDuration_ = downtimeJailDurationBuilder_.build();
}
result.slashFractionDoubleSign_ = slashFractionDoubleSign_;
result.slashFractionDowntime_ = slashFractionDowntime_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.slashing.v1beta1.Slashing.Params) {
return mergeFrom((cosmos.slashing.v1beta1.Slashing.Params)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.slashing.v1beta1.Slashing.Params other) {
if (other == cosmos.slashing.v1beta1.Slashing.Params.getDefaultInstance()) return this;
if (other.getSignedBlocksWindow() != 0L) {
setSignedBlocksWindow(other.getSignedBlocksWindow());
}
if (other.getMinSignedPerWindow() != com.google.protobuf.ByteString.EMPTY) {
setMinSignedPerWindow(other.getMinSignedPerWindow());
}
if (other.hasDowntimeJailDuration()) {
mergeDowntimeJailDuration(other.getDowntimeJailDuration());
}
if (other.getSlashFractionDoubleSign() != com.google.protobuf.ByteString.EMPTY) {
setSlashFractionDoubleSign(other.getSlashFractionDoubleSign());
}
if (other.getSlashFractionDowntime() != com.google.protobuf.ByteString.EMPTY) {
setSlashFractionDowntime(other.getSlashFractionDowntime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.slashing.v1beta1.Slashing.Params parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.slashing.v1beta1.Slashing.Params) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long signedBlocksWindow_ ;
/**
* int64 signed_blocks_window = 1 [(.gogoproto.moretags) = "yaml:\"signed_blocks_window\""];
*/
public long getSignedBlocksWindow() {
return signedBlocksWindow_;
}
/**
* int64 signed_blocks_window = 1 [(.gogoproto.moretags) = "yaml:\"signed_blocks_window\""];
*/
public Builder setSignedBlocksWindow(long value) {
signedBlocksWindow_ = value;
onChanged();
return this;
}
/**
* int64 signed_blocks_window = 1 [(.gogoproto.moretags) = "yaml:\"signed_blocks_window\""];
*/
public Builder clearSignedBlocksWindow() {
signedBlocksWindow_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString minSignedPerWindow_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes min_signed_per_window = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"min_signed_per_window\""];
*/
public com.google.protobuf.ByteString getMinSignedPerWindow() {
return minSignedPerWindow_;
}
/**
* bytes min_signed_per_window = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"min_signed_per_window\""];
*/
public Builder setMinSignedPerWindow(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
minSignedPerWindow_ = value;
onChanged();
return this;
}
/**
* bytes min_signed_per_window = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"min_signed_per_window\""];
*/
public Builder clearMinSignedPerWindow() {
minSignedPerWindow_ = getDefaultInstance().getMinSignedPerWindow();
onChanged();
return this;
}
private com.google.protobuf.Duration downtimeJailDuration_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> downtimeJailDurationBuilder_;
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public boolean hasDowntimeJailDuration() {
return downtimeJailDurationBuilder_ != null || downtimeJailDuration_ != null;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getDowntimeJailDuration() {
if (downtimeJailDurationBuilder_ == null) {
return downtimeJailDuration_ == null ? com.google.protobuf.Duration.getDefaultInstance() : downtimeJailDuration_;
} else {
return downtimeJailDurationBuilder_.getMessage();
}
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public Builder setDowntimeJailDuration(com.google.protobuf.Duration value) {
if (downtimeJailDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
downtimeJailDuration_ = value;
onChanged();
} else {
downtimeJailDurationBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public Builder setDowntimeJailDuration(
com.google.protobuf.Duration.Builder builderForValue) {
if (downtimeJailDurationBuilder_ == null) {
downtimeJailDuration_ = builderForValue.build();
onChanged();
} else {
downtimeJailDurationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public Builder mergeDowntimeJailDuration(com.google.protobuf.Duration value) {
if (downtimeJailDurationBuilder_ == null) {
if (downtimeJailDuration_ != null) {
downtimeJailDuration_ =
com.google.protobuf.Duration.newBuilder(downtimeJailDuration_).mergeFrom(value).buildPartial();
} else {
downtimeJailDuration_ = value;
}
onChanged();
} else {
downtimeJailDurationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public Builder clearDowntimeJailDuration() {
if (downtimeJailDurationBuilder_ == null) {
downtimeJailDuration_ = null;
onChanged();
} else {
downtimeJailDuration_ = null;
downtimeJailDurationBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration.Builder getDowntimeJailDurationBuilder() {
onChanged();
return getDowntimeJailDurationFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getDowntimeJailDurationOrBuilder() {
if (downtimeJailDurationBuilder_ != null) {
return downtimeJailDurationBuilder_.getMessageOrBuilder();
} else {
return downtimeJailDuration_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : downtimeJailDuration_;
}
}
/**
* .google.protobuf.Duration downtime_jail_duration = 3 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"downtime_jail_duration\"", (.gogoproto.stdduration) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getDowntimeJailDurationFieldBuilder() {
if (downtimeJailDurationBuilder_ == null) {
downtimeJailDurationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getDowntimeJailDuration(),
getParentForChildren(),
isClean());
downtimeJailDuration_ = null;
}
return downtimeJailDurationBuilder_;
}
private com.google.protobuf.ByteString slashFractionDoubleSign_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes slash_fraction_double_sign = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_double_sign\""];
*/
public com.google.protobuf.ByteString getSlashFractionDoubleSign() {
return slashFractionDoubleSign_;
}
/**
* bytes slash_fraction_double_sign = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_double_sign\""];
*/
public Builder setSlashFractionDoubleSign(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
slashFractionDoubleSign_ = value;
onChanged();
return this;
}
/**
* bytes slash_fraction_double_sign = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_double_sign\""];
*/
public Builder clearSlashFractionDoubleSign() {
slashFractionDoubleSign_ = getDefaultInstance().getSlashFractionDoubleSign();
onChanged();
return this;
}
private com.google.protobuf.ByteString slashFractionDowntime_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes slash_fraction_downtime = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_downtime\""];
*/
public com.google.protobuf.ByteString getSlashFractionDowntime() {
return slashFractionDowntime_;
}
/**
* bytes slash_fraction_downtime = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_downtime\""];
*/
public Builder setSlashFractionDowntime(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
slashFractionDowntime_ = value;
onChanged();
return this;
}
/**
* bytes slash_fraction_downtime = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"slash_fraction_downtime\""];
*/
public Builder clearSlashFractionDowntime() {
slashFractionDowntime_ = getDefaultInstance().getSlashFractionDowntime();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.slashing.v1beta1.Params)
}
// @@protoc_insertion_point(class_scope:cosmos.slashing.v1beta1.Params)
private static final cosmos.slashing.v1beta1.Slashing.Params DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.slashing.v1beta1.Slashing.Params();
}
public static cosmos.slashing.v1beta1.Slashing.Params getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Params parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Params(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.slashing.v1beta1.Slashing.Params getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_slashing_v1beta1_Params_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_slashing_v1beta1_Params_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n&cosmos/slashing/v1beta1/slashing.proto" +
"\022\027cosmos.slashing.v1beta1\032\024gogoproto/gog" +
"o.proto\032\036google/protobuf/duration.proto\032" +
"\037google/protobuf/timestamp.proto\"\267\002\n\024Val" +
"idatorSigningInfo\022\017\n\007address\030\001 \001(\t\022-\n\014st" +
"art_height\030\002 \001(\003B\027\362\336\037\023yaml:\"start_height" +
"\"\022-\n\014index_offset\030\003 \001(\003B\027\362\336\037\023yaml:\"index" +
"_offset\"\022Q\n\014jailed_until\030\004 \001(\0132\032.google." +
"protobuf.TimestampB\037\362\336\037\023yaml:\"jailed_unt" +
"il\"\220\337\037\001\310\336\037\000\022\022\n\ntombstoned\030\005 \001(\010\022?\n\025misse" +
"d_blocks_counter\030\006 \001(\003B \362\336\037\034yaml:\"missed" +
"_blocks_counter\":\010\350\240\037\001\230\240\037\000\"\210\004\n\006Params\022=\n" +
"\024signed_blocks_window\030\001 \001(\003B\037\362\336\037\033yaml:\"s" +
"igned_blocks_window\"\022m\n\025min_signed_per_w" +
"indow\030\002 \001(\014BN\362\336\037\034yaml:\"min_signed_per_wi" +
"ndow\"\332\336\037&github.com/cosmos/cosmos-sdk/ty" +
"pes.Dec\310\336\037\000\022d\n\026downtime_jail_duration\030\003 " +
"\001(\0132\031.google.protobuf.DurationB)\310\336\037\000\230\337\037\001" +
"\362\336\037\035yaml:\"downtime_jail_duration\"\022w\n\032sla" +
"sh_fraction_double_sign\030\004 \001(\014BS\362\336\037!yaml:" +
"\"slash_fraction_double_sign\"\332\336\037&github.c" +
"om/cosmos/cosmos-sdk/types.Dec\310\336\037\000\022q\n\027sl" +
"ash_fraction_downtime\030\005 \001(\014BP\362\336\037\036yaml:\"s" +
"lash_fraction_downtime\"\332\336\037&github.com/co" +
"smos/cosmos-sdk/types.Dec\310\336\037\000B3Z-github." +
"com/cosmos/cosmos-sdk/x/slashing/types\250\342" +
"\036\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
com.google.protobuf.DurationProto.getDescriptor(),
com.google.protobuf.TimestampProto.getDescriptor(),
}, assigner);
internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_slashing_v1beta1_ValidatorSigningInfo_descriptor,
new java.lang.String[] { "Address", "StartHeight", "IndexOffset", "JailedUntil", "Tombstoned", "MissedBlocksCounter", });
internal_static_cosmos_slashing_v1beta1_Params_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cosmos_slashing_v1beta1_Params_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_slashing_v1beta1_Params_descriptor,
new java.lang.String[] { "SignedBlocksWindow", "MinSignedPerWindow", "DowntimeJailDuration", "SlashFractionDoubleSign", "SlashFractionDowntime", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.customtype);
registry.add(com.google.protobuf.GoGoProtos.equal);
registry.add(com.google.protobuf.GoGoProtos.equalAll);
registry.add(com.google.protobuf.GoGoProtos.goprotoStringer);
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
registry.add(com.google.protobuf.GoGoProtos.stdduration);
registry.add(com.google.protobuf.GoGoProtos.stdtime);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
com.google.protobuf.DurationProto.getDescriptor();
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy