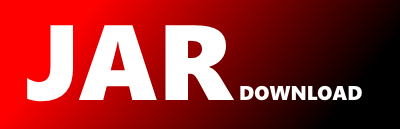
cosmos.staking.v1beta1.Staking Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: cosmos/staking/v1beta1/staking.proto
package cosmos.staking.v1beta1;
public final class Staking {
private Staking() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* BondStatus is the status of a validator.
*
*
* Protobuf enum {@code cosmos.staking.v1beta1.BondStatus}
*/
public enum BondStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* UNSPECIFIED defines an invalid validator status.
*
*
* BOND_STATUS_UNSPECIFIED = 0 [(.gogoproto.enumvalue_customname) = "Unspecified"];
*/
BOND_STATUS_UNSPECIFIED(0),
/**
*
* UNBONDED defines a validator that is not bonded.
*
*
* BOND_STATUS_UNBONDED = 1 [(.gogoproto.enumvalue_customname) = "Unbonded"];
*/
BOND_STATUS_UNBONDED(1),
/**
*
* UNBONDING defines a validator that is unbonding.
*
*
* BOND_STATUS_UNBONDING = 2 [(.gogoproto.enumvalue_customname) = "Unbonding"];
*/
BOND_STATUS_UNBONDING(2),
/**
*
* BONDED defines a validator that is bonded.
*
*
* BOND_STATUS_BONDED = 3 [(.gogoproto.enumvalue_customname) = "Bonded"];
*/
BOND_STATUS_BONDED(3),
UNRECOGNIZED(-1),
;
/**
*
* UNSPECIFIED defines an invalid validator status.
*
*
* BOND_STATUS_UNSPECIFIED = 0 [(.gogoproto.enumvalue_customname) = "Unspecified"];
*/
public static final int BOND_STATUS_UNSPECIFIED_VALUE = 0;
/**
*
* UNBONDED defines a validator that is not bonded.
*
*
* BOND_STATUS_UNBONDED = 1 [(.gogoproto.enumvalue_customname) = "Unbonded"];
*/
public static final int BOND_STATUS_UNBONDED_VALUE = 1;
/**
*
* UNBONDING defines a validator that is unbonding.
*
*
* BOND_STATUS_UNBONDING = 2 [(.gogoproto.enumvalue_customname) = "Unbonding"];
*/
public static final int BOND_STATUS_UNBONDING_VALUE = 2;
/**
*
* BONDED defines a validator that is bonded.
*
*
* BOND_STATUS_BONDED = 3 [(.gogoproto.enumvalue_customname) = "Bonded"];
*/
public static final int BOND_STATUS_BONDED_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BondStatus valueOf(int value) {
return forNumber(value);
}
public static BondStatus forNumber(int value) {
switch (value) {
case 0: return BOND_STATUS_UNSPECIFIED;
case 1: return BOND_STATUS_UNBONDED;
case 2: return BOND_STATUS_UNBONDING;
case 3: return BOND_STATUS_BONDED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BondStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BondStatus findValueByNumber(int number) {
return BondStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.getDescriptor().getEnumTypes().get(0);
}
private static final BondStatus[] VALUES = values();
public static BondStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private BondStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cosmos.staking.v1beta1.BondStatus)
}
public interface HistoricalInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.HistoricalInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
boolean hasHeader();
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
tendermint.types.Types.Header getHeader();
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
tendermint.types.Types.HeaderOrBuilder getHeaderOrBuilder();
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
java.util.List
getValsetList();
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.Validator getValset(int index);
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
int getValsetCount();
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
java.util.List extends cosmos.staking.v1beta1.Staking.ValidatorOrBuilder>
getValsetOrBuilderList();
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.ValidatorOrBuilder getValsetOrBuilder(
int index);
}
/**
*
* HistoricalInfo contains header and validator information for a given block.
* It is stored as part of staking module's state, which persists the `n` most
* recent HistoricalInfo
* (`n` is set by the staking module's `historical_entries` parameter).
*
*
* Protobuf type {@code cosmos.staking.v1beta1.HistoricalInfo}
*/
public static final class HistoricalInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.HistoricalInfo)
HistoricalInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use HistoricalInfo.newBuilder() to construct.
private HistoricalInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HistoricalInfo() {
valset_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HistoricalInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tendermint.types.Types.Header.Builder subBuilder = null;
if (header_ != null) {
subBuilder = header_.toBuilder();
}
header_ = input.readMessage(tendermint.types.Types.Header.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(header_);
header_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
valset_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
valset_.add(
input.readMessage(cosmos.staking.v1beta1.Staking.Validator.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
valset_ = java.util.Collections.unmodifiableList(valset_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_HistoricalInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_HistoricalInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.HistoricalInfo.class, cosmos.staking.v1beta1.Staking.HistoricalInfo.Builder.class);
}
private int bitField0_;
public static final int HEADER_FIELD_NUMBER = 1;
private tendermint.types.Types.Header header_;
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasHeader() {
return header_ != null;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public tendermint.types.Types.Header getHeader() {
return header_ == null ? tendermint.types.Types.Header.getDefaultInstance() : header_;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public tendermint.types.Types.HeaderOrBuilder getHeaderOrBuilder() {
return getHeader();
}
public static final int VALSET_FIELD_NUMBER = 2;
private java.util.List valset_;
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List getValsetList() {
return valset_;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.ValidatorOrBuilder>
getValsetOrBuilderList() {
return valset_;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public int getValsetCount() {
return valset_.size();
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Validator getValset(int index) {
return valset_.get(index);
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.ValidatorOrBuilder getValsetOrBuilder(
int index) {
return valset_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (header_ != null) {
output.writeMessage(1, getHeader());
}
for (int i = 0; i < valset_.size(); i++) {
output.writeMessage(2, valset_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (header_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getHeader());
}
for (int i = 0; i < valset_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, valset_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.HistoricalInfo)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.HistoricalInfo other = (cosmos.staking.v1beta1.Staking.HistoricalInfo) obj;
boolean result = true;
result = result && (hasHeader() == other.hasHeader());
if (hasHeader()) {
result = result && getHeader()
.equals(other.getHeader());
}
result = result && getValsetList()
.equals(other.getValsetList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHeader()) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeader().hashCode();
}
if (getValsetCount() > 0) {
hash = (37 * hash) + VALSET_FIELD_NUMBER;
hash = (53 * hash) + getValsetList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.HistoricalInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* HistoricalInfo contains header and validator information for a given block.
* It is stored as part of staking module's state, which persists the `n` most
* recent HistoricalInfo
* (`n` is set by the staking module's `historical_entries` parameter).
*
*
* Protobuf type {@code cosmos.staking.v1beta1.HistoricalInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.HistoricalInfo)
cosmos.staking.v1beta1.Staking.HistoricalInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_HistoricalInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_HistoricalInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.HistoricalInfo.class, cosmos.staking.v1beta1.Staking.HistoricalInfo.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.HistoricalInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValsetFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (headerBuilder_ == null) {
header_ = null;
} else {
header_ = null;
headerBuilder_ = null;
}
if (valsetBuilder_ == null) {
valset_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
valsetBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_HistoricalInfo_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.HistoricalInfo getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.HistoricalInfo.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.HistoricalInfo build() {
cosmos.staking.v1beta1.Staking.HistoricalInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.HistoricalInfo buildPartial() {
cosmos.staking.v1beta1.Staking.HistoricalInfo result = new cosmos.staking.v1beta1.Staking.HistoricalInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (headerBuilder_ == null) {
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
if (valsetBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
valset_ = java.util.Collections.unmodifiableList(valset_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.valset_ = valset_;
} else {
result.valset_ = valsetBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.HistoricalInfo) {
return mergeFrom((cosmos.staking.v1beta1.Staking.HistoricalInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.HistoricalInfo other) {
if (other == cosmos.staking.v1beta1.Staking.HistoricalInfo.getDefaultInstance()) return this;
if (other.hasHeader()) {
mergeHeader(other.getHeader());
}
if (valsetBuilder_ == null) {
if (!other.valset_.isEmpty()) {
if (valset_.isEmpty()) {
valset_ = other.valset_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureValsetIsMutable();
valset_.addAll(other.valset_);
}
onChanged();
}
} else {
if (!other.valset_.isEmpty()) {
if (valsetBuilder_.isEmpty()) {
valsetBuilder_.dispose();
valsetBuilder_ = null;
valset_ = other.valset_;
bitField0_ = (bitField0_ & ~0x00000002);
valsetBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getValsetFieldBuilder() : null;
} else {
valsetBuilder_.addAllMessages(other.valset_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.HistoricalInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.HistoricalInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private tendermint.types.Types.Header header_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tendermint.types.Types.Header, tendermint.types.Types.Header.Builder, tendermint.types.Types.HeaderOrBuilder> headerBuilder_;
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasHeader() {
return headerBuilder_ != null || header_ != null;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public tendermint.types.Types.Header getHeader() {
if (headerBuilder_ == null) {
return header_ == null ? tendermint.types.Types.Header.getDefaultInstance() : header_;
} else {
return headerBuilder_.getMessage();
}
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public Builder setHeader(tendermint.types.Types.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
header_ = value;
onChanged();
} else {
headerBuilder_.setMessage(value);
}
return this;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public Builder setHeader(
tendermint.types.Types.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
header_ = builderForValue.build();
onChanged();
} else {
headerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeader(tendermint.types.Types.Header value) {
if (headerBuilder_ == null) {
if (header_ != null) {
header_ =
tendermint.types.Types.Header.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
onChanged();
} else {
headerBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = null;
onChanged();
} else {
header_ = null;
headerBuilder_ = null;
}
return this;
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public tendermint.types.Types.Header.Builder getHeaderBuilder() {
onChanged();
return getHeaderFieldBuilder().getBuilder();
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
public tendermint.types.Types.HeaderOrBuilder getHeaderOrBuilder() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilder();
} else {
return header_ == null ?
tendermint.types.Types.Header.getDefaultInstance() : header_;
}
}
/**
* .tendermint.types.Header header = 1 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
tendermint.types.Types.Header, tendermint.types.Types.Header.Builder, tendermint.types.Types.HeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tendermint.types.Types.Header, tendermint.types.Types.Header.Builder, tendermint.types.Types.HeaderOrBuilder>(
getHeader(),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private java.util.List valset_ =
java.util.Collections.emptyList();
private void ensureValsetIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
valset_ = new java.util.ArrayList(valset_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Validator, cosmos.staking.v1beta1.Staking.Validator.Builder, cosmos.staking.v1beta1.Staking.ValidatorOrBuilder> valsetBuilder_;
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List getValsetList() {
if (valsetBuilder_ == null) {
return java.util.Collections.unmodifiableList(valset_);
} else {
return valsetBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public int getValsetCount() {
if (valsetBuilder_ == null) {
return valset_.size();
} else {
return valsetBuilder_.getCount();
}
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Validator getValset(int index) {
if (valsetBuilder_ == null) {
return valset_.get(index);
} else {
return valsetBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder setValset(
int index, cosmos.staking.v1beta1.Staking.Validator value) {
if (valsetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValsetIsMutable();
valset_.set(index, value);
onChanged();
} else {
valsetBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder setValset(
int index, cosmos.staking.v1beta1.Staking.Validator.Builder builderForValue) {
if (valsetBuilder_ == null) {
ensureValsetIsMutable();
valset_.set(index, builderForValue.build());
onChanged();
} else {
valsetBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder addValset(cosmos.staking.v1beta1.Staking.Validator value) {
if (valsetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValsetIsMutable();
valset_.add(value);
onChanged();
} else {
valsetBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder addValset(
int index, cosmos.staking.v1beta1.Staking.Validator value) {
if (valsetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureValsetIsMutable();
valset_.add(index, value);
onChanged();
} else {
valsetBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder addValset(
cosmos.staking.v1beta1.Staking.Validator.Builder builderForValue) {
if (valsetBuilder_ == null) {
ensureValsetIsMutable();
valset_.add(builderForValue.build());
onChanged();
} else {
valsetBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder addValset(
int index, cosmos.staking.v1beta1.Staking.Validator.Builder builderForValue) {
if (valsetBuilder_ == null) {
ensureValsetIsMutable();
valset_.add(index, builderForValue.build());
onChanged();
} else {
valsetBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder addAllValset(
java.lang.Iterable extends cosmos.staking.v1beta1.Staking.Validator> values) {
if (valsetBuilder_ == null) {
ensureValsetIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, valset_);
onChanged();
} else {
valsetBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearValset() {
if (valsetBuilder_ == null) {
valset_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
valsetBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public Builder removeValset(int index) {
if (valsetBuilder_ == null) {
ensureValsetIsMutable();
valset_.remove(index);
onChanged();
} else {
valsetBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Validator.Builder getValsetBuilder(
int index) {
return getValsetFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.ValidatorOrBuilder getValsetOrBuilder(
int index) {
if (valsetBuilder_ == null) {
return valset_.get(index); } else {
return valsetBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.ValidatorOrBuilder>
getValsetOrBuilderList() {
if (valsetBuilder_ != null) {
return valsetBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(valset_);
}
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Validator.Builder addValsetBuilder() {
return getValsetFieldBuilder().addBuilder(
cosmos.staking.v1beta1.Staking.Validator.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Validator.Builder addValsetBuilder(
int index) {
return getValsetFieldBuilder().addBuilder(
index, cosmos.staking.v1beta1.Staking.Validator.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.Validator valset = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List
getValsetBuilderList() {
return getValsetFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Validator, cosmos.staking.v1beta1.Staking.Validator.Builder, cosmos.staking.v1beta1.Staking.ValidatorOrBuilder>
getValsetFieldBuilder() {
if (valsetBuilder_ == null) {
valsetBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Validator, cosmos.staking.v1beta1.Staking.Validator.Builder, cosmos.staking.v1beta1.Staking.ValidatorOrBuilder>(
valset_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
valset_ = null;
}
return valsetBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.HistoricalInfo)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.HistoricalInfo)
private static final cosmos.staking.v1beta1.Staking.HistoricalInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.HistoricalInfo();
}
public static cosmos.staking.v1beta1.Staking.HistoricalInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HistoricalInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HistoricalInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.HistoricalInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommissionRatesOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.CommissionRates)
com.google.protobuf.MessageOrBuilder {
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
java.lang.String getRate();
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
com.google.protobuf.ByteString
getRateBytes();
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
java.lang.String getMaxRate();
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
com.google.protobuf.ByteString
getMaxRateBytes();
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
java.lang.String getMaxChangeRate();
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
com.google.protobuf.ByteString
getMaxChangeRateBytes();
}
/**
*
* CommissionRates defines the initial commission rates to be used for creating
* a validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.CommissionRates}
*/
public static final class CommissionRates extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.CommissionRates)
CommissionRatesOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommissionRates.newBuilder() to construct.
private CommissionRates(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommissionRates() {
rate_ = "";
maxRate_ = "";
maxChangeRate_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommissionRates(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
rate_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
maxRate_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
maxChangeRate_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_CommissionRates_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_CommissionRates_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.CommissionRates.class, cosmos.staking.v1beta1.Staking.CommissionRates.Builder.class);
}
public static final int RATE_FIELD_NUMBER = 1;
private volatile java.lang.Object rate_;
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getRate() {
java.lang.Object ref = rate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rate_ = s;
return s;
}
}
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getRateBytes() {
java.lang.Object ref = rate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAX_RATE_FIELD_NUMBER = 2;
private volatile java.lang.Object maxRate_;
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public java.lang.String getMaxRate() {
java.lang.Object ref = maxRate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
maxRate_ = s;
return s;
}
}
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public com.google.protobuf.ByteString
getMaxRateBytes() {
java.lang.Object ref = maxRate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
maxRate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAX_CHANGE_RATE_FIELD_NUMBER = 3;
private volatile java.lang.Object maxChangeRate_;
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public java.lang.String getMaxChangeRate() {
java.lang.Object ref = maxChangeRate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
maxChangeRate_ = s;
return s;
}
}
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public com.google.protobuf.ByteString
getMaxChangeRateBytes() {
java.lang.Object ref = maxChangeRate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
maxChangeRate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getRateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, rate_);
}
if (!getMaxRateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, maxRate_);
}
if (!getMaxChangeRateBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, maxChangeRate_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getRateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, rate_);
}
if (!getMaxRateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, maxRate_);
}
if (!getMaxChangeRateBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, maxChangeRate_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.CommissionRates)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.CommissionRates other = (cosmos.staking.v1beta1.Staking.CommissionRates) obj;
boolean result = true;
result = result && getRate()
.equals(other.getRate());
result = result && getMaxRate()
.equals(other.getMaxRate());
result = result && getMaxChangeRate()
.equals(other.getMaxChangeRate());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RATE_FIELD_NUMBER;
hash = (53 * hash) + getRate().hashCode();
hash = (37 * hash) + MAX_RATE_FIELD_NUMBER;
hash = (53 * hash) + getMaxRate().hashCode();
hash = (37 * hash) + MAX_CHANGE_RATE_FIELD_NUMBER;
hash = (53 * hash) + getMaxChangeRate().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.CommissionRates parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.CommissionRates prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* CommissionRates defines the initial commission rates to be used for creating
* a validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.CommissionRates}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.CommissionRates)
cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_CommissionRates_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_CommissionRates_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.CommissionRates.class, cosmos.staking.v1beta1.Staking.CommissionRates.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.CommissionRates.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
rate_ = "";
maxRate_ = "";
maxChangeRate_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_CommissionRates_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.CommissionRates getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.CommissionRates.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.CommissionRates build() {
cosmos.staking.v1beta1.Staking.CommissionRates result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.CommissionRates buildPartial() {
cosmos.staking.v1beta1.Staking.CommissionRates result = new cosmos.staking.v1beta1.Staking.CommissionRates(this);
result.rate_ = rate_;
result.maxRate_ = maxRate_;
result.maxChangeRate_ = maxChangeRate_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.CommissionRates) {
return mergeFrom((cosmos.staking.v1beta1.Staking.CommissionRates)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.CommissionRates other) {
if (other == cosmos.staking.v1beta1.Staking.CommissionRates.getDefaultInstance()) return this;
if (!other.getRate().isEmpty()) {
rate_ = other.rate_;
onChanged();
}
if (!other.getMaxRate().isEmpty()) {
maxRate_ = other.maxRate_;
onChanged();
}
if (!other.getMaxChangeRate().isEmpty()) {
maxChangeRate_ = other.maxChangeRate_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.CommissionRates parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.CommissionRates) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object rate_ = "";
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getRate() {
java.lang.Object ref = rate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
rate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getRateBytes() {
java.lang.Object ref = rate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
rate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setRate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
rate_ = value;
onChanged();
return this;
}
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder clearRate() {
rate_ = getDefaultInstance().getRate();
onChanged();
return this;
}
/**
*
* rate is the commission rate charged to delegators, as a fraction.
*
*
* string rate = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setRateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
rate_ = value;
onChanged();
return this;
}
private java.lang.Object maxRate_ = "";
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public java.lang.String getMaxRate() {
java.lang.Object ref = maxRate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
maxRate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public com.google.protobuf.ByteString
getMaxRateBytes() {
java.lang.Object ref = maxRate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
maxRate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public Builder setMaxRate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
maxRate_ = value;
onChanged();
return this;
}
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public Builder clearMaxRate() {
maxRate_ = getDefaultInstance().getMaxRate();
onChanged();
return this;
}
/**
*
* max_rate defines the maximum commission rate which validator can ever charge, as a fraction.
*
*
* string max_rate = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_rate\""];
*/
public Builder setMaxRateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
maxRate_ = value;
onChanged();
return this;
}
private java.lang.Object maxChangeRate_ = "";
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public java.lang.String getMaxChangeRate() {
java.lang.Object ref = maxChangeRate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
maxChangeRate_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public com.google.protobuf.ByteString
getMaxChangeRateBytes() {
java.lang.Object ref = maxChangeRate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
maxChangeRate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public Builder setMaxChangeRate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
maxChangeRate_ = value;
onChanged();
return this;
}
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public Builder clearMaxChangeRate() {
maxChangeRate_ = getDefaultInstance().getMaxChangeRate();
onChanged();
return this;
}
/**
*
* max_change_rate defines the maximum daily increase of the validator commission, as a fraction.
*
*
* string max_change_rate = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"max_change_rate\""];
*/
public Builder setMaxChangeRateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
maxChangeRate_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.CommissionRates)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.CommissionRates)
private static final cosmos.staking.v1beta1.Staking.CommissionRates DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.CommissionRates();
}
public static cosmos.staking.v1beta1.Staking.CommissionRates getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommissionRates parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommissionRates(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.CommissionRates getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommissionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Commission)
com.google.protobuf.MessageOrBuilder {
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
boolean hasCommissionRates();
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
cosmos.staking.v1beta1.Staking.CommissionRates getCommissionRates();
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder getCommissionRatesOrBuilder();
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasUpdateTime();
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getUpdateTime();
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder();
}
/**
*
* Commission defines commission parameters for a given validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Commission}
*/
public static final class Commission extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Commission)
CommissionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Commission.newBuilder() to construct.
private Commission(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Commission() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Commission(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cosmos.staking.v1beta1.Staking.CommissionRates.Builder subBuilder = null;
if (commissionRates_ != null) {
subBuilder = commissionRates_.toBuilder();
}
commissionRates_ = input.readMessage(cosmos.staking.v1beta1.Staking.CommissionRates.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(commissionRates_);
commissionRates_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (updateTime_ != null) {
subBuilder = updateTime_.toBuilder();
}
updateTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(updateTime_);
updateTime_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Commission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Commission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Commission.class, cosmos.staking.v1beta1.Staking.Commission.Builder.class);
}
public static final int COMMISSION_RATES_FIELD_NUMBER = 1;
private cosmos.staking.v1beta1.Staking.CommissionRates commissionRates_;
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public boolean hasCommissionRates() {
return commissionRates_ != null;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public cosmos.staking.v1beta1.Staking.CommissionRates getCommissionRates() {
return commissionRates_ == null ? cosmos.staking.v1beta1.Staking.CommissionRates.getDefaultInstance() : commissionRates_;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder getCommissionRatesOrBuilder() {
return getCommissionRates();
}
public static final int UPDATE_TIME_FIELD_NUMBER = 2;
private com.google.protobuf.Timestamp updateTime_;
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasUpdateTime() {
return updateTime_ != null;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getUpdateTime() {
return updateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder() {
return getUpdateTime();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (commissionRates_ != null) {
output.writeMessage(1, getCommissionRates());
}
if (updateTime_ != null) {
output.writeMessage(2, getUpdateTime());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (commissionRates_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getCommissionRates());
}
if (updateTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getUpdateTime());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Commission)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Commission other = (cosmos.staking.v1beta1.Staking.Commission) obj;
boolean result = true;
result = result && (hasCommissionRates() == other.hasCommissionRates());
if (hasCommissionRates()) {
result = result && getCommissionRates()
.equals(other.getCommissionRates());
}
result = result && (hasUpdateTime() == other.hasUpdateTime());
if (hasUpdateTime()) {
result = result && getUpdateTime()
.equals(other.getUpdateTime());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCommissionRates()) {
hash = (37 * hash) + COMMISSION_RATES_FIELD_NUMBER;
hash = (53 * hash) + getCommissionRates().hashCode();
}
if (hasUpdateTime()) {
hash = (37 * hash) + UPDATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getUpdateTime().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Commission parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Commission parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Commission parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Commission prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Commission defines commission parameters for a given validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Commission}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Commission)
cosmos.staking.v1beta1.Staking.CommissionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Commission_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Commission_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Commission.class, cosmos.staking.v1beta1.Staking.Commission.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Commission.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (commissionRatesBuilder_ == null) {
commissionRates_ = null;
} else {
commissionRates_ = null;
commissionRatesBuilder_ = null;
}
if (updateTimeBuilder_ == null) {
updateTime_ = null;
} else {
updateTime_ = null;
updateTimeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Commission_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Commission getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Commission.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Commission build() {
cosmos.staking.v1beta1.Staking.Commission result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Commission buildPartial() {
cosmos.staking.v1beta1.Staking.Commission result = new cosmos.staking.v1beta1.Staking.Commission(this);
if (commissionRatesBuilder_ == null) {
result.commissionRates_ = commissionRates_;
} else {
result.commissionRates_ = commissionRatesBuilder_.build();
}
if (updateTimeBuilder_ == null) {
result.updateTime_ = updateTime_;
} else {
result.updateTime_ = updateTimeBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Commission) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Commission)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Commission other) {
if (other == cosmos.staking.v1beta1.Staking.Commission.getDefaultInstance()) return this;
if (other.hasCommissionRates()) {
mergeCommissionRates(other.getCommissionRates());
}
if (other.hasUpdateTime()) {
mergeUpdateTime(other.getUpdateTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Commission parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Commission) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cosmos.staking.v1beta1.Staking.CommissionRates commissionRates_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.CommissionRates, cosmos.staking.v1beta1.Staking.CommissionRates.Builder, cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder> commissionRatesBuilder_;
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public boolean hasCommissionRates() {
return commissionRatesBuilder_ != null || commissionRates_ != null;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public cosmos.staking.v1beta1.Staking.CommissionRates getCommissionRates() {
if (commissionRatesBuilder_ == null) {
return commissionRates_ == null ? cosmos.staking.v1beta1.Staking.CommissionRates.getDefaultInstance() : commissionRates_;
} else {
return commissionRatesBuilder_.getMessage();
}
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public Builder setCommissionRates(cosmos.staking.v1beta1.Staking.CommissionRates value) {
if (commissionRatesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
commissionRates_ = value;
onChanged();
} else {
commissionRatesBuilder_.setMessage(value);
}
return this;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public Builder setCommissionRates(
cosmos.staking.v1beta1.Staking.CommissionRates.Builder builderForValue) {
if (commissionRatesBuilder_ == null) {
commissionRates_ = builderForValue.build();
onChanged();
} else {
commissionRatesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public Builder mergeCommissionRates(cosmos.staking.v1beta1.Staking.CommissionRates value) {
if (commissionRatesBuilder_ == null) {
if (commissionRates_ != null) {
commissionRates_ =
cosmos.staking.v1beta1.Staking.CommissionRates.newBuilder(commissionRates_).mergeFrom(value).buildPartial();
} else {
commissionRates_ = value;
}
onChanged();
} else {
commissionRatesBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public Builder clearCommissionRates() {
if (commissionRatesBuilder_ == null) {
commissionRates_ = null;
onChanged();
} else {
commissionRates_ = null;
commissionRatesBuilder_ = null;
}
return this;
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public cosmos.staking.v1beta1.Staking.CommissionRates.Builder getCommissionRatesBuilder() {
onChanged();
return getCommissionRatesFieldBuilder().getBuilder();
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
public cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder getCommissionRatesOrBuilder() {
if (commissionRatesBuilder_ != null) {
return commissionRatesBuilder_.getMessageOrBuilder();
} else {
return commissionRates_ == null ?
cosmos.staking.v1beta1.Staking.CommissionRates.getDefaultInstance() : commissionRates_;
}
}
/**
*
* commission_rates defines the initial commission rates to be used for creating a validator.
*
*
* .cosmos.staking.v1beta1.CommissionRates commission_rates = 1 [(.gogoproto.nullable) = false, (.gogoproto.embed) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.CommissionRates, cosmos.staking.v1beta1.Staking.CommissionRates.Builder, cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder>
getCommissionRatesFieldBuilder() {
if (commissionRatesBuilder_ == null) {
commissionRatesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.CommissionRates, cosmos.staking.v1beta1.Staking.CommissionRates.Builder, cosmos.staking.v1beta1.Staking.CommissionRatesOrBuilder>(
getCommissionRates(),
getParentForChildren(),
isClean());
commissionRates_ = null;
}
return commissionRatesBuilder_;
}
private com.google.protobuf.Timestamp updateTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> updateTimeBuilder_;
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasUpdateTime() {
return updateTimeBuilder_ != null || updateTime_ != null;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getUpdateTime() {
if (updateTimeBuilder_ == null) {
return updateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
} else {
return updateTimeBuilder_.getMessage();
}
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setUpdateTime(com.google.protobuf.Timestamp value) {
if (updateTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updateTime_ = value;
onChanged();
} else {
updateTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setUpdateTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (updateTimeBuilder_ == null) {
updateTime_ = builderForValue.build();
onChanged();
} else {
updateTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeUpdateTime(com.google.protobuf.Timestamp value) {
if (updateTimeBuilder_ == null) {
if (updateTime_ != null) {
updateTime_ =
com.google.protobuf.Timestamp.newBuilder(updateTime_).mergeFrom(value).buildPartial();
} else {
updateTime_ = value;
}
onChanged();
} else {
updateTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearUpdateTime() {
if (updateTimeBuilder_ == null) {
updateTime_ = null;
onChanged();
} else {
updateTime_ = null;
updateTimeBuilder_ = null;
}
return this;
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getUpdateTimeBuilder() {
onChanged();
return getUpdateTimeFieldBuilder().getBuilder();
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder() {
if (updateTimeBuilder_ != null) {
return updateTimeBuilder_.getMessageOrBuilder();
} else {
return updateTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
}
}
/**
*
* update_time is the last time the commission rate was changed.
*
*
* .google.protobuf.Timestamp update_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"update_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getUpdateTimeFieldBuilder() {
if (updateTimeBuilder_ == null) {
updateTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getUpdateTime(),
getParentForChildren(),
isClean());
updateTime_ = null;
}
return updateTimeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Commission)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Commission)
private static final cosmos.staking.v1beta1.Staking.Commission DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Commission();
}
public static cosmos.staking.v1beta1.Staking.Commission getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Commission parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Commission(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Commission getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DescriptionOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Description)
com.google.protobuf.MessageOrBuilder {
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
java.lang.String getMoniker();
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
com.google.protobuf.ByteString
getMonikerBytes();
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
java.lang.String getIdentity();
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
com.google.protobuf.ByteString
getIdentityBytes();
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
java.lang.String getWebsite();
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
com.google.protobuf.ByteString
getWebsiteBytes();
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
java.lang.String getSecurityContact();
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
com.google.protobuf.ByteString
getSecurityContactBytes();
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
java.lang.String getDetails();
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
com.google.protobuf.ByteString
getDetailsBytes();
}
/**
*
* Description defines a validator description.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Description}
*/
public static final class Description extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Description)
DescriptionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Description.newBuilder() to construct.
private Description(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Description() {
moniker_ = "";
identity_ = "";
website_ = "";
securityContact_ = "";
details_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Description(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
moniker_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
identity_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
website_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
securityContact_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
details_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Description_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Description_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Description.class, cosmos.staking.v1beta1.Staking.Description.Builder.class);
}
public static final int MONIKER_FIELD_NUMBER = 1;
private volatile java.lang.Object moniker_;
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public java.lang.String getMoniker() {
java.lang.Object ref = moniker_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
moniker_ = s;
return s;
}
}
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public com.google.protobuf.ByteString
getMonikerBytes() {
java.lang.Object ref = moniker_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
moniker_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IDENTITY_FIELD_NUMBER = 2;
private volatile java.lang.Object identity_;
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public java.lang.String getIdentity() {
java.lang.Object ref = identity_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identity_ = s;
return s;
}
}
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public com.google.protobuf.ByteString
getIdentityBytes() {
java.lang.Object ref = identity_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WEBSITE_FIELD_NUMBER = 3;
private volatile java.lang.Object website_;
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public java.lang.String getWebsite() {
java.lang.Object ref = website_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
website_ = s;
return s;
}
}
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public com.google.protobuf.ByteString
getWebsiteBytes() {
java.lang.Object ref = website_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
website_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SECURITY_CONTACT_FIELD_NUMBER = 4;
private volatile java.lang.Object securityContact_;
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public java.lang.String getSecurityContact() {
java.lang.Object ref = securityContact_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
securityContact_ = s;
return s;
}
}
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public com.google.protobuf.ByteString
getSecurityContactBytes() {
java.lang.Object ref = securityContact_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
securityContact_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DETAILS_FIELD_NUMBER = 5;
private volatile java.lang.Object details_;
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public java.lang.String getDetails() {
java.lang.Object ref = details_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
details_ = s;
return s;
}
}
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public com.google.protobuf.ByteString
getDetailsBytes() {
java.lang.Object ref = details_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
details_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getMonikerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, moniker_);
}
if (!getIdentityBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, identity_);
}
if (!getWebsiteBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, website_);
}
if (!getSecurityContactBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, securityContact_);
}
if (!getDetailsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, details_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getMonikerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, moniker_);
}
if (!getIdentityBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, identity_);
}
if (!getWebsiteBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, website_);
}
if (!getSecurityContactBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, securityContact_);
}
if (!getDetailsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, details_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Description)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Description other = (cosmos.staking.v1beta1.Staking.Description) obj;
boolean result = true;
result = result && getMoniker()
.equals(other.getMoniker());
result = result && getIdentity()
.equals(other.getIdentity());
result = result && getWebsite()
.equals(other.getWebsite());
result = result && getSecurityContact()
.equals(other.getSecurityContact());
result = result && getDetails()
.equals(other.getDetails());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MONIKER_FIELD_NUMBER;
hash = (53 * hash) + getMoniker().hashCode();
hash = (37 * hash) + IDENTITY_FIELD_NUMBER;
hash = (53 * hash) + getIdentity().hashCode();
hash = (37 * hash) + WEBSITE_FIELD_NUMBER;
hash = (53 * hash) + getWebsite().hashCode();
hash = (37 * hash) + SECURITY_CONTACT_FIELD_NUMBER;
hash = (53 * hash) + getSecurityContact().hashCode();
hash = (37 * hash) + DETAILS_FIELD_NUMBER;
hash = (53 * hash) + getDetails().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Description parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Description parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Description parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Description prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Description defines a validator description.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Description}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Description)
cosmos.staking.v1beta1.Staking.DescriptionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Description_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Description_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Description.class, cosmos.staking.v1beta1.Staking.Description.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Description.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
moniker_ = "";
identity_ = "";
website_ = "";
securityContact_ = "";
details_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Description_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Description getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Description.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Description build() {
cosmos.staking.v1beta1.Staking.Description result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Description buildPartial() {
cosmos.staking.v1beta1.Staking.Description result = new cosmos.staking.v1beta1.Staking.Description(this);
result.moniker_ = moniker_;
result.identity_ = identity_;
result.website_ = website_;
result.securityContact_ = securityContact_;
result.details_ = details_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Description) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Description)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Description other) {
if (other == cosmos.staking.v1beta1.Staking.Description.getDefaultInstance()) return this;
if (!other.getMoniker().isEmpty()) {
moniker_ = other.moniker_;
onChanged();
}
if (!other.getIdentity().isEmpty()) {
identity_ = other.identity_;
onChanged();
}
if (!other.getWebsite().isEmpty()) {
website_ = other.website_;
onChanged();
}
if (!other.getSecurityContact().isEmpty()) {
securityContact_ = other.securityContact_;
onChanged();
}
if (!other.getDetails().isEmpty()) {
details_ = other.details_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Description parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Description) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object moniker_ = "";
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public java.lang.String getMoniker() {
java.lang.Object ref = moniker_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
moniker_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public com.google.protobuf.ByteString
getMonikerBytes() {
java.lang.Object ref = moniker_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
moniker_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public Builder setMoniker(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
moniker_ = value;
onChanged();
return this;
}
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public Builder clearMoniker() {
moniker_ = getDefaultInstance().getMoniker();
onChanged();
return this;
}
/**
*
* moniker defines a human-readable name for the validator.
*
*
* string moniker = 1;
*/
public Builder setMonikerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
moniker_ = value;
onChanged();
return this;
}
private java.lang.Object identity_ = "";
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public java.lang.String getIdentity() {
java.lang.Object ref = identity_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identity_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public com.google.protobuf.ByteString
getIdentityBytes() {
java.lang.Object ref = identity_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public Builder setIdentity(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
identity_ = value;
onChanged();
return this;
}
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public Builder clearIdentity() {
identity_ = getDefaultInstance().getIdentity();
onChanged();
return this;
}
/**
*
* identity defines an optional identity signature (ex. UPort or Keybase).
*
*
* string identity = 2;
*/
public Builder setIdentityBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
identity_ = value;
onChanged();
return this;
}
private java.lang.Object website_ = "";
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public java.lang.String getWebsite() {
java.lang.Object ref = website_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
website_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public com.google.protobuf.ByteString
getWebsiteBytes() {
java.lang.Object ref = website_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
website_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public Builder setWebsite(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
website_ = value;
onChanged();
return this;
}
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public Builder clearWebsite() {
website_ = getDefaultInstance().getWebsite();
onChanged();
return this;
}
/**
*
* website defines an optional website link.
*
*
* string website = 3;
*/
public Builder setWebsiteBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
website_ = value;
onChanged();
return this;
}
private java.lang.Object securityContact_ = "";
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public java.lang.String getSecurityContact() {
java.lang.Object ref = securityContact_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
securityContact_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public com.google.protobuf.ByteString
getSecurityContactBytes() {
java.lang.Object ref = securityContact_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
securityContact_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public Builder setSecurityContact(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
securityContact_ = value;
onChanged();
return this;
}
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public Builder clearSecurityContact() {
securityContact_ = getDefaultInstance().getSecurityContact();
onChanged();
return this;
}
/**
*
* security_contact defines an optional email for security contact.
*
*
* string security_contact = 4 [(.gogoproto.moretags) = "yaml:\"security_contact\""];
*/
public Builder setSecurityContactBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
securityContact_ = value;
onChanged();
return this;
}
private java.lang.Object details_ = "";
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public java.lang.String getDetails() {
java.lang.Object ref = details_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
details_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public com.google.protobuf.ByteString
getDetailsBytes() {
java.lang.Object ref = details_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
details_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public Builder setDetails(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
return this;
}
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public Builder clearDetails() {
details_ = getDefaultInstance().getDetails();
onChanged();
return this;
}
/**
*
* details define other optional details.
*
*
* string details = 5;
*/
public Builder setDetailsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
details_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Description)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Description)
private static final cosmos.staking.v1beta1.Staking.Description DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Description();
}
public static cosmos.staking.v1beta1.Staking.Description getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Description parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Description(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Description getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValidatorOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Validator)
com.google.protobuf.MessageOrBuilder {
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
java.lang.String getOperatorAddress();
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
com.google.protobuf.ByteString
getOperatorAddressBytes();
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
boolean hasConsensusPubkey();
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
com.google.protobuf.Any getConsensusPubkey();
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
com.google.protobuf.AnyOrBuilder getConsensusPubkeyOrBuilder();
/**
*
* jailed defined whether the validator has been jailed from bonded status or not.
*
*
* bool jailed = 3;
*/
boolean getJailed();
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
int getStatusValue();
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
cosmos.staking.v1beta1.Staking.BondStatus getStatus();
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
java.lang.String getTokens();
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
com.google.protobuf.ByteString
getTokensBytes();
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
java.lang.String getDelegatorShares();
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
com.google.protobuf.ByteString
getDelegatorSharesBytes();
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
boolean hasDescription();
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.Description getDescription();
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.DescriptionOrBuilder getDescriptionOrBuilder();
/**
*
* unbonding_height defines, if unbonding, the height at which this validator has begun unbonding.
*
*
* int64 unbonding_height = 8 [(.gogoproto.moretags) = "yaml:\"unbonding_height\""];
*/
long getUnbondingHeight();
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasUnbondingTime();
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getUnbondingTime();
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getUnbondingTimeOrBuilder();
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
boolean hasCommission();
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.Commission getCommission();
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.CommissionOrBuilder getCommissionOrBuilder();
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
java.lang.String getMinSelfDelegation();
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
com.google.protobuf.ByteString
getMinSelfDelegationBytes();
}
/**
*
* Validator defines a validator, together with the total amount of the
* Validator's bond shares and their exchange rate to coins. Slashing results in
* a decrease in the exchange rate, allowing correct calculation of future
* undelegations without iterating over delegators. When coins are delegated to
* this validator, the validator is credited with a delegation whose number of
* bond shares is based on the amount of coins delegated divided by the current
* exchange rate. Voting power can be calculated as total bonded shares
* multiplied by exchange rate.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Validator}
*/
public static final class Validator extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Validator)
ValidatorOrBuilder {
private static final long serialVersionUID = 0L;
// Use Validator.newBuilder() to construct.
private Validator(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Validator() {
operatorAddress_ = "";
jailed_ = false;
status_ = 0;
tokens_ = "";
delegatorShares_ = "";
unbondingHeight_ = 0L;
minSelfDelegation_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Validator(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
operatorAddress_ = s;
break;
}
case 18: {
com.google.protobuf.Any.Builder subBuilder = null;
if (consensusPubkey_ != null) {
subBuilder = consensusPubkey_.toBuilder();
}
consensusPubkey_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(consensusPubkey_);
consensusPubkey_ = subBuilder.buildPartial();
}
break;
}
case 24: {
jailed_ = input.readBool();
break;
}
case 32: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
tokens_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
delegatorShares_ = s;
break;
}
case 58: {
cosmos.staking.v1beta1.Staking.Description.Builder subBuilder = null;
if (description_ != null) {
subBuilder = description_.toBuilder();
}
description_ = input.readMessage(cosmos.staking.v1beta1.Staking.Description.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(description_);
description_ = subBuilder.buildPartial();
}
break;
}
case 64: {
unbondingHeight_ = input.readInt64();
break;
}
case 74: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (unbondingTime_ != null) {
subBuilder = unbondingTime_.toBuilder();
}
unbondingTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(unbondingTime_);
unbondingTime_ = subBuilder.buildPartial();
}
break;
}
case 82: {
cosmos.staking.v1beta1.Staking.Commission.Builder subBuilder = null;
if (commission_ != null) {
subBuilder = commission_.toBuilder();
}
commission_ = input.readMessage(cosmos.staking.v1beta1.Staking.Commission.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(commission_);
commission_ = subBuilder.buildPartial();
}
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
minSelfDelegation_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Validator_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Validator_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Validator.class, cosmos.staking.v1beta1.Staking.Validator.Builder.class);
}
public static final int OPERATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object operatorAddress_;
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public java.lang.String getOperatorAddress() {
java.lang.Object ref = operatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
operatorAddress_ = s;
return s;
}
}
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public com.google.protobuf.ByteString
getOperatorAddressBytes() {
java.lang.Object ref = operatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
operatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONSENSUS_PUBKEY_FIELD_NUMBER = 2;
private com.google.protobuf.Any consensusPubkey_;
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public boolean hasConsensusPubkey() {
return consensusPubkey_ != null;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public com.google.protobuf.Any getConsensusPubkey() {
return consensusPubkey_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusPubkey_;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public com.google.protobuf.AnyOrBuilder getConsensusPubkeyOrBuilder() {
return getConsensusPubkey();
}
public static final int JAILED_FIELD_NUMBER = 3;
private boolean jailed_;
/**
*
* jailed defined whether the validator has been jailed from bonded status or not.
*
*
* bool jailed = 3;
*/
public boolean getJailed() {
return jailed_;
}
public static final int STATUS_FIELD_NUMBER = 4;
private int status_;
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public int getStatusValue() {
return status_;
}
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public cosmos.staking.v1beta1.Staking.BondStatus getStatus() {
@SuppressWarnings("deprecation")
cosmos.staking.v1beta1.Staking.BondStatus result = cosmos.staking.v1beta1.Staking.BondStatus.valueOf(status_);
return result == null ? cosmos.staking.v1beta1.Staking.BondStatus.UNRECOGNIZED : result;
}
public static final int TOKENS_FIELD_NUMBER = 5;
private volatile java.lang.Object tokens_;
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getTokens() {
java.lang.Object ref = tokens_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tokens_ = s;
return s;
}
}
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getTokensBytes() {
java.lang.Object ref = tokens_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tokens_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DELEGATOR_SHARES_FIELD_NUMBER = 6;
private volatile java.lang.Object delegatorShares_;
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public java.lang.String getDelegatorShares() {
java.lang.Object ref = delegatorShares_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorShares_ = s;
return s;
}
}
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public com.google.protobuf.ByteString
getDelegatorSharesBytes() {
java.lang.Object ref = delegatorShares_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorShares_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 7;
private cosmos.staking.v1beta1.Staking.Description description_;
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public boolean hasDescription() {
return description_ != null;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Description getDescription() {
return description_ == null ? cosmos.staking.v1beta1.Staking.Description.getDefaultInstance() : description_;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DescriptionOrBuilder getDescriptionOrBuilder() {
return getDescription();
}
public static final int UNBONDING_HEIGHT_FIELD_NUMBER = 8;
private long unbondingHeight_;
/**
*
* unbonding_height defines, if unbonding, the height at which this validator has begun unbonding.
*
*
* int64 unbonding_height = 8 [(.gogoproto.moretags) = "yaml:\"unbonding_height\""];
*/
public long getUnbondingHeight() {
return unbondingHeight_;
}
public static final int UNBONDING_TIME_FIELD_NUMBER = 9;
private com.google.protobuf.Timestamp unbondingTime_;
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasUnbondingTime() {
return unbondingTime_ != null;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getUnbondingTime() {
return unbondingTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : unbondingTime_;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getUnbondingTimeOrBuilder() {
return getUnbondingTime();
}
public static final int COMMISSION_FIELD_NUMBER = 10;
private cosmos.staking.v1beta1.Staking.Commission commission_;
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public boolean hasCommission() {
return commission_ != null;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Commission getCommission() {
return commission_ == null ? cosmos.staking.v1beta1.Staking.Commission.getDefaultInstance() : commission_;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.CommissionOrBuilder getCommissionOrBuilder() {
return getCommission();
}
public static final int MIN_SELF_DELEGATION_FIELD_NUMBER = 11;
private volatile java.lang.Object minSelfDelegation_;
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public java.lang.String getMinSelfDelegation() {
java.lang.Object ref = minSelfDelegation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
minSelfDelegation_ = s;
return s;
}
}
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public com.google.protobuf.ByteString
getMinSelfDelegationBytes() {
java.lang.Object ref = minSelfDelegation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
minSelfDelegation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOperatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, operatorAddress_);
}
if (consensusPubkey_ != null) {
output.writeMessage(2, getConsensusPubkey());
}
if (jailed_ != false) {
output.writeBool(3, jailed_);
}
if (status_ != cosmos.staking.v1beta1.Staking.BondStatus.BOND_STATUS_UNSPECIFIED.getNumber()) {
output.writeEnum(4, status_);
}
if (!getTokensBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, tokens_);
}
if (!getDelegatorSharesBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, delegatorShares_);
}
if (description_ != null) {
output.writeMessage(7, getDescription());
}
if (unbondingHeight_ != 0L) {
output.writeInt64(8, unbondingHeight_);
}
if (unbondingTime_ != null) {
output.writeMessage(9, getUnbondingTime());
}
if (commission_ != null) {
output.writeMessage(10, getCommission());
}
if (!getMinSelfDelegationBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, minSelfDelegation_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOperatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, operatorAddress_);
}
if (consensusPubkey_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getConsensusPubkey());
}
if (jailed_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, jailed_);
}
if (status_ != cosmos.staking.v1beta1.Staking.BondStatus.BOND_STATUS_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, status_);
}
if (!getTokensBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, tokens_);
}
if (!getDelegatorSharesBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, delegatorShares_);
}
if (description_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getDescription());
}
if (unbondingHeight_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, unbondingHeight_);
}
if (unbondingTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getUnbondingTime());
}
if (commission_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getCommission());
}
if (!getMinSelfDelegationBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, minSelfDelegation_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Validator)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Validator other = (cosmos.staking.v1beta1.Staking.Validator) obj;
boolean result = true;
result = result && getOperatorAddress()
.equals(other.getOperatorAddress());
result = result && (hasConsensusPubkey() == other.hasConsensusPubkey());
if (hasConsensusPubkey()) {
result = result && getConsensusPubkey()
.equals(other.getConsensusPubkey());
}
result = result && (getJailed()
== other.getJailed());
result = result && status_ == other.status_;
result = result && getTokens()
.equals(other.getTokens());
result = result && getDelegatorShares()
.equals(other.getDelegatorShares());
result = result && (hasDescription() == other.hasDescription());
if (hasDescription()) {
result = result && getDescription()
.equals(other.getDescription());
}
result = result && (getUnbondingHeight()
== other.getUnbondingHeight());
result = result && (hasUnbondingTime() == other.hasUnbondingTime());
if (hasUnbondingTime()) {
result = result && getUnbondingTime()
.equals(other.getUnbondingTime());
}
result = result && (hasCommission() == other.hasCommission());
if (hasCommission()) {
result = result && getCommission()
.equals(other.getCommission());
}
result = result && getMinSelfDelegation()
.equals(other.getMinSelfDelegation());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPERATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getOperatorAddress().hashCode();
if (hasConsensusPubkey()) {
hash = (37 * hash) + CONSENSUS_PUBKEY_FIELD_NUMBER;
hash = (53 * hash) + getConsensusPubkey().hashCode();
}
hash = (37 * hash) + JAILED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getJailed());
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + TOKENS_FIELD_NUMBER;
hash = (53 * hash) + getTokens().hashCode();
hash = (37 * hash) + DELEGATOR_SHARES_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorShares().hashCode();
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
hash = (37 * hash) + UNBONDING_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUnbondingHeight());
if (hasUnbondingTime()) {
hash = (37 * hash) + UNBONDING_TIME_FIELD_NUMBER;
hash = (53 * hash) + getUnbondingTime().hashCode();
}
if (hasCommission()) {
hash = (37 * hash) + COMMISSION_FIELD_NUMBER;
hash = (53 * hash) + getCommission().hashCode();
}
hash = (37 * hash) + MIN_SELF_DELEGATION_FIELD_NUMBER;
hash = (53 * hash) + getMinSelfDelegation().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Validator parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Validator parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Validator parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Validator prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Validator defines a validator, together with the total amount of the
* Validator's bond shares and their exchange rate to coins. Slashing results in
* a decrease in the exchange rate, allowing correct calculation of future
* undelegations without iterating over delegators. When coins are delegated to
* this validator, the validator is credited with a delegation whose number of
* bond shares is based on the amount of coins delegated divided by the current
* exchange rate. Voting power can be calculated as total bonded shares
* multiplied by exchange rate.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Validator}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Validator)
cosmos.staking.v1beta1.Staking.ValidatorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Validator_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Validator_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Validator.class, cosmos.staking.v1beta1.Staking.Validator.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Validator.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
operatorAddress_ = "";
if (consensusPubkeyBuilder_ == null) {
consensusPubkey_ = null;
} else {
consensusPubkey_ = null;
consensusPubkeyBuilder_ = null;
}
jailed_ = false;
status_ = 0;
tokens_ = "";
delegatorShares_ = "";
if (descriptionBuilder_ == null) {
description_ = null;
} else {
description_ = null;
descriptionBuilder_ = null;
}
unbondingHeight_ = 0L;
if (unbondingTimeBuilder_ == null) {
unbondingTime_ = null;
} else {
unbondingTime_ = null;
unbondingTimeBuilder_ = null;
}
if (commissionBuilder_ == null) {
commission_ = null;
} else {
commission_ = null;
commissionBuilder_ = null;
}
minSelfDelegation_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Validator_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Validator getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Validator.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Validator build() {
cosmos.staking.v1beta1.Staking.Validator result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Validator buildPartial() {
cosmos.staking.v1beta1.Staking.Validator result = new cosmos.staking.v1beta1.Staking.Validator(this);
result.operatorAddress_ = operatorAddress_;
if (consensusPubkeyBuilder_ == null) {
result.consensusPubkey_ = consensusPubkey_;
} else {
result.consensusPubkey_ = consensusPubkeyBuilder_.build();
}
result.jailed_ = jailed_;
result.status_ = status_;
result.tokens_ = tokens_;
result.delegatorShares_ = delegatorShares_;
if (descriptionBuilder_ == null) {
result.description_ = description_;
} else {
result.description_ = descriptionBuilder_.build();
}
result.unbondingHeight_ = unbondingHeight_;
if (unbondingTimeBuilder_ == null) {
result.unbondingTime_ = unbondingTime_;
} else {
result.unbondingTime_ = unbondingTimeBuilder_.build();
}
if (commissionBuilder_ == null) {
result.commission_ = commission_;
} else {
result.commission_ = commissionBuilder_.build();
}
result.minSelfDelegation_ = minSelfDelegation_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Validator) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Validator)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Validator other) {
if (other == cosmos.staking.v1beta1.Staking.Validator.getDefaultInstance()) return this;
if (!other.getOperatorAddress().isEmpty()) {
operatorAddress_ = other.operatorAddress_;
onChanged();
}
if (other.hasConsensusPubkey()) {
mergeConsensusPubkey(other.getConsensusPubkey());
}
if (other.getJailed() != false) {
setJailed(other.getJailed());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.getTokens().isEmpty()) {
tokens_ = other.tokens_;
onChanged();
}
if (!other.getDelegatorShares().isEmpty()) {
delegatorShares_ = other.delegatorShares_;
onChanged();
}
if (other.hasDescription()) {
mergeDescription(other.getDescription());
}
if (other.getUnbondingHeight() != 0L) {
setUnbondingHeight(other.getUnbondingHeight());
}
if (other.hasUnbondingTime()) {
mergeUnbondingTime(other.getUnbondingTime());
}
if (other.hasCommission()) {
mergeCommission(other.getCommission());
}
if (!other.getMinSelfDelegation().isEmpty()) {
minSelfDelegation_ = other.minSelfDelegation_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Validator parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Validator) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object operatorAddress_ = "";
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public java.lang.String getOperatorAddress() {
java.lang.Object ref = operatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
operatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public com.google.protobuf.ByteString
getOperatorAddressBytes() {
java.lang.Object ref = operatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
operatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public Builder setOperatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
operatorAddress_ = value;
onChanged();
return this;
}
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public Builder clearOperatorAddress() {
operatorAddress_ = getDefaultInstance().getOperatorAddress();
onChanged();
return this;
}
/**
*
* operator_address defines the address of the validator's operator; bech encoded in JSON.
*
*
* string operator_address = 1 [(.gogoproto.moretags) = "yaml:\"operator_address\""];
*/
public Builder setOperatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
operatorAddress_ = value;
onChanged();
return this;
}
private com.google.protobuf.Any consensusPubkey_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> consensusPubkeyBuilder_;
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public boolean hasConsensusPubkey() {
return consensusPubkeyBuilder_ != null || consensusPubkey_ != null;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public com.google.protobuf.Any getConsensusPubkey() {
if (consensusPubkeyBuilder_ == null) {
return consensusPubkey_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusPubkey_;
} else {
return consensusPubkeyBuilder_.getMessage();
}
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public Builder setConsensusPubkey(com.google.protobuf.Any value) {
if (consensusPubkeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consensusPubkey_ = value;
onChanged();
} else {
consensusPubkeyBuilder_.setMessage(value);
}
return this;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public Builder setConsensusPubkey(
com.google.protobuf.Any.Builder builderForValue) {
if (consensusPubkeyBuilder_ == null) {
consensusPubkey_ = builderForValue.build();
onChanged();
} else {
consensusPubkeyBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public Builder mergeConsensusPubkey(com.google.protobuf.Any value) {
if (consensusPubkeyBuilder_ == null) {
if (consensusPubkey_ != null) {
consensusPubkey_ =
com.google.protobuf.Any.newBuilder(consensusPubkey_).mergeFrom(value).buildPartial();
} else {
consensusPubkey_ = value;
}
onChanged();
} else {
consensusPubkeyBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public Builder clearConsensusPubkey() {
if (consensusPubkeyBuilder_ == null) {
consensusPubkey_ = null;
onChanged();
} else {
consensusPubkey_ = null;
consensusPubkeyBuilder_ = null;
}
return this;
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public com.google.protobuf.Any.Builder getConsensusPubkeyBuilder() {
onChanged();
return getConsensusPubkeyFieldBuilder().getBuilder();
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
public com.google.protobuf.AnyOrBuilder getConsensusPubkeyOrBuilder() {
if (consensusPubkeyBuilder_ != null) {
return consensusPubkeyBuilder_.getMessageOrBuilder();
} else {
return consensusPubkey_ == null ?
com.google.protobuf.Any.getDefaultInstance() : consensusPubkey_;
}
}
/**
*
* consensus_pubkey is the consensus public key of the validator, as a Protobuf Any.
*
*
* .google.protobuf.Any consensus_pubkey = 2 [(.gogoproto.moretags) = "yaml:\"consensus_pubkey\"", (.cosmos_proto.accepts_interface) = "cosmos.crypto.PubKey"];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getConsensusPubkeyFieldBuilder() {
if (consensusPubkeyBuilder_ == null) {
consensusPubkeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getConsensusPubkey(),
getParentForChildren(),
isClean());
consensusPubkey_ = null;
}
return consensusPubkeyBuilder_;
}
private boolean jailed_ ;
/**
*
* jailed defined whether the validator has been jailed from bonded status or not.
*
*
* bool jailed = 3;
*/
public boolean getJailed() {
return jailed_;
}
/**
*
* jailed defined whether the validator has been jailed from bonded status or not.
*
*
* bool jailed = 3;
*/
public Builder setJailed(boolean value) {
jailed_ = value;
onChanged();
return this;
}
/**
*
* jailed defined whether the validator has been jailed from bonded status or not.
*
*
* bool jailed = 3;
*/
public Builder clearJailed() {
jailed_ = false;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public int getStatusValue() {
return status_;
}
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public cosmos.staking.v1beta1.Staking.BondStatus getStatus() {
@SuppressWarnings("deprecation")
cosmos.staking.v1beta1.Staking.BondStatus result = cosmos.staking.v1beta1.Staking.BondStatus.valueOf(status_);
return result == null ? cosmos.staking.v1beta1.Staking.BondStatus.UNRECOGNIZED : result;
}
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public Builder setStatus(cosmos.staking.v1beta1.Staking.BondStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* status is the validator status (bonded/unbonding/unbonded).
*
*
* .cosmos.staking.v1beta1.BondStatus status = 4;
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private java.lang.Object tokens_ = "";
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getTokens() {
java.lang.Object ref = tokens_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tokens_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getTokensBytes() {
java.lang.Object ref = tokens_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tokens_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setTokens(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tokens_ = value;
onChanged();
return this;
}
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder clearTokens() {
tokens_ = getDefaultInstance().getTokens();
onChanged();
return this;
}
/**
*
* tokens define the delegated tokens (incl. self-delegation).
*
*
* string tokens = 5 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setTokensBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tokens_ = value;
onChanged();
return this;
}
private java.lang.Object delegatorShares_ = "";
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public java.lang.String getDelegatorShares() {
java.lang.Object ref = delegatorShares_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorShares_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public com.google.protobuf.ByteString
getDelegatorSharesBytes() {
java.lang.Object ref = delegatorShares_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorShares_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public Builder setDelegatorShares(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorShares_ = value;
onChanged();
return this;
}
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public Builder clearDelegatorShares() {
delegatorShares_ = getDefaultInstance().getDelegatorShares();
onChanged();
return this;
}
/**
*
* delegator_shares defines total shares issued to a validator's delegators.
*
*
* string delegator_shares = 6 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec", (.gogoproto.moretags) = "yaml:\"delegator_shares\""];
*/
public Builder setDelegatorSharesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorShares_ = value;
onChanged();
return this;
}
private cosmos.staking.v1beta1.Staking.Description description_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Description, cosmos.staking.v1beta1.Staking.Description.Builder, cosmos.staking.v1beta1.Staking.DescriptionOrBuilder> descriptionBuilder_;
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public boolean hasDescription() {
return descriptionBuilder_ != null || description_ != null;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Description getDescription() {
if (descriptionBuilder_ == null) {
return description_ == null ? cosmos.staking.v1beta1.Staking.Description.getDefaultInstance() : description_;
} else {
return descriptionBuilder_.getMessage();
}
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public Builder setDescription(cosmos.staking.v1beta1.Staking.Description value) {
if (descriptionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
} else {
descriptionBuilder_.setMessage(value);
}
return this;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public Builder setDescription(
cosmos.staking.v1beta1.Staking.Description.Builder builderForValue) {
if (descriptionBuilder_ == null) {
description_ = builderForValue.build();
onChanged();
} else {
descriptionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public Builder mergeDescription(cosmos.staking.v1beta1.Staking.Description value) {
if (descriptionBuilder_ == null) {
if (description_ != null) {
description_ =
cosmos.staking.v1beta1.Staking.Description.newBuilder(description_).mergeFrom(value).buildPartial();
} else {
description_ = value;
}
onChanged();
} else {
descriptionBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public Builder clearDescription() {
if (descriptionBuilder_ == null) {
description_ = null;
onChanged();
} else {
description_ = null;
descriptionBuilder_ = null;
}
return this;
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Description.Builder getDescriptionBuilder() {
onChanged();
return getDescriptionFieldBuilder().getBuilder();
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DescriptionOrBuilder getDescriptionOrBuilder() {
if (descriptionBuilder_ != null) {
return descriptionBuilder_.getMessageOrBuilder();
} else {
return description_ == null ?
cosmos.staking.v1beta1.Staking.Description.getDefaultInstance() : description_;
}
}
/**
*
* description defines the description terms for the validator.
*
*
* .cosmos.staking.v1beta1.Description description = 7 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Description, cosmos.staking.v1beta1.Staking.Description.Builder, cosmos.staking.v1beta1.Staking.DescriptionOrBuilder>
getDescriptionFieldBuilder() {
if (descriptionBuilder_ == null) {
descriptionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Description, cosmos.staking.v1beta1.Staking.Description.Builder, cosmos.staking.v1beta1.Staking.DescriptionOrBuilder>(
getDescription(),
getParentForChildren(),
isClean());
description_ = null;
}
return descriptionBuilder_;
}
private long unbondingHeight_ ;
/**
*
* unbonding_height defines, if unbonding, the height at which this validator has begun unbonding.
*
*
* int64 unbonding_height = 8 [(.gogoproto.moretags) = "yaml:\"unbonding_height\""];
*/
public long getUnbondingHeight() {
return unbondingHeight_;
}
/**
*
* unbonding_height defines, if unbonding, the height at which this validator has begun unbonding.
*
*
* int64 unbonding_height = 8 [(.gogoproto.moretags) = "yaml:\"unbonding_height\""];
*/
public Builder setUnbondingHeight(long value) {
unbondingHeight_ = value;
onChanged();
return this;
}
/**
*
* unbonding_height defines, if unbonding, the height at which this validator has begun unbonding.
*
*
* int64 unbonding_height = 8 [(.gogoproto.moretags) = "yaml:\"unbonding_height\""];
*/
public Builder clearUnbondingHeight() {
unbondingHeight_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Timestamp unbondingTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> unbondingTimeBuilder_;
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasUnbondingTime() {
return unbondingTimeBuilder_ != null || unbondingTime_ != null;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getUnbondingTime() {
if (unbondingTimeBuilder_ == null) {
return unbondingTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : unbondingTime_;
} else {
return unbondingTimeBuilder_.getMessage();
}
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setUnbondingTime(com.google.protobuf.Timestamp value) {
if (unbondingTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
unbondingTime_ = value;
onChanged();
} else {
unbondingTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setUnbondingTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (unbondingTimeBuilder_ == null) {
unbondingTime_ = builderForValue.build();
onChanged();
} else {
unbondingTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeUnbondingTime(com.google.protobuf.Timestamp value) {
if (unbondingTimeBuilder_ == null) {
if (unbondingTime_ != null) {
unbondingTime_ =
com.google.protobuf.Timestamp.newBuilder(unbondingTime_).mergeFrom(value).buildPartial();
} else {
unbondingTime_ = value;
}
onChanged();
} else {
unbondingTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearUnbondingTime() {
if (unbondingTimeBuilder_ == null) {
unbondingTime_ = null;
onChanged();
} else {
unbondingTime_ = null;
unbondingTimeBuilder_ = null;
}
return this;
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getUnbondingTimeBuilder() {
onChanged();
return getUnbondingTimeFieldBuilder().getBuilder();
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getUnbondingTimeOrBuilder() {
if (unbondingTimeBuilder_ != null) {
return unbondingTimeBuilder_.getMessageOrBuilder();
} else {
return unbondingTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : unbondingTime_;
}
}
/**
*
* unbonding_time defines, if unbonding, the min time for the validator to complete unbonding.
*
*
* .google.protobuf.Timestamp unbonding_time = 9 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getUnbondingTimeFieldBuilder() {
if (unbondingTimeBuilder_ == null) {
unbondingTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getUnbondingTime(),
getParentForChildren(),
isClean());
unbondingTime_ = null;
}
return unbondingTimeBuilder_;
}
private cosmos.staking.v1beta1.Staking.Commission commission_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Commission, cosmos.staking.v1beta1.Staking.Commission.Builder, cosmos.staking.v1beta1.Staking.CommissionOrBuilder> commissionBuilder_;
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public boolean hasCommission() {
return commissionBuilder_ != null || commission_ != null;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Commission getCommission() {
if (commissionBuilder_ == null) {
return commission_ == null ? cosmos.staking.v1beta1.Staking.Commission.getDefaultInstance() : commission_;
} else {
return commissionBuilder_.getMessage();
}
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public Builder setCommission(cosmos.staking.v1beta1.Staking.Commission value) {
if (commissionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
commission_ = value;
onChanged();
} else {
commissionBuilder_.setMessage(value);
}
return this;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public Builder setCommission(
cosmos.staking.v1beta1.Staking.Commission.Builder builderForValue) {
if (commissionBuilder_ == null) {
commission_ = builderForValue.build();
onChanged();
} else {
commissionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public Builder mergeCommission(cosmos.staking.v1beta1.Staking.Commission value) {
if (commissionBuilder_ == null) {
if (commission_ != null) {
commission_ =
cosmos.staking.v1beta1.Staking.Commission.newBuilder(commission_).mergeFrom(value).buildPartial();
} else {
commission_ = value;
}
onChanged();
} else {
commissionBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public Builder clearCommission() {
if (commissionBuilder_ == null) {
commission_ = null;
onChanged();
} else {
commission_ = null;
commissionBuilder_ = null;
}
return this;
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Commission.Builder getCommissionBuilder() {
onChanged();
return getCommissionFieldBuilder().getBuilder();
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.CommissionOrBuilder getCommissionOrBuilder() {
if (commissionBuilder_ != null) {
return commissionBuilder_.getMessageOrBuilder();
} else {
return commission_ == null ?
cosmos.staking.v1beta1.Staking.Commission.getDefaultInstance() : commission_;
}
}
/**
*
* commission defines the commission parameters.
*
*
* .cosmos.staking.v1beta1.Commission commission = 10 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Commission, cosmos.staking.v1beta1.Staking.Commission.Builder, cosmos.staking.v1beta1.Staking.CommissionOrBuilder>
getCommissionFieldBuilder() {
if (commissionBuilder_ == null) {
commissionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Commission, cosmos.staking.v1beta1.Staking.Commission.Builder, cosmos.staking.v1beta1.Staking.CommissionOrBuilder>(
getCommission(),
getParentForChildren(),
isClean());
commission_ = null;
}
return commissionBuilder_;
}
private java.lang.Object minSelfDelegation_ = "";
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public java.lang.String getMinSelfDelegation() {
java.lang.Object ref = minSelfDelegation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
minSelfDelegation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public com.google.protobuf.ByteString
getMinSelfDelegationBytes() {
java.lang.Object ref = minSelfDelegation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
minSelfDelegation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public Builder setMinSelfDelegation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
minSelfDelegation_ = value;
onChanged();
return this;
}
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public Builder clearMinSelfDelegation() {
minSelfDelegation_ = getDefaultInstance().getMinSelfDelegation();
onChanged();
return this;
}
/**
*
* min_self_delegation is the validator's self declared minimum self delegation.
*
*
* string min_self_delegation = 11 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"min_self_delegation\""];
*/
public Builder setMinSelfDelegationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
minSelfDelegation_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Validator)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Validator)
private static final cosmos.staking.v1beta1.Staking.Validator DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Validator();
}
public static cosmos.staking.v1beta1.Staking.Validator getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Validator parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Validator(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Validator getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValAddressesOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.ValAddresses)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string addresses = 1;
*/
java.util.List
getAddressesList();
/**
* repeated string addresses = 1;
*/
int getAddressesCount();
/**
* repeated string addresses = 1;
*/
java.lang.String getAddresses(int index);
/**
* repeated string addresses = 1;
*/
com.google.protobuf.ByteString
getAddressesBytes(int index);
}
/**
*
* ValAddresses defines a repeated set of validator addresses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.ValAddresses}
*/
public static final class ValAddresses extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.ValAddresses)
ValAddressesOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValAddresses.newBuilder() to construct.
private ValAddresses(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValAddresses() {
addresses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ValAddresses(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
addresses_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
addresses_.add(s);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
addresses_ = addresses_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_ValAddresses_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_ValAddresses_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.ValAddresses.class, cosmos.staking.v1beta1.Staking.ValAddresses.Builder.class);
}
public static final int ADDRESSES_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList addresses_;
/**
* repeated string addresses = 1;
*/
public com.google.protobuf.ProtocolStringList
getAddressesList() {
return addresses_;
}
/**
* repeated string addresses = 1;
*/
public int getAddressesCount() {
return addresses_.size();
}
/**
* repeated string addresses = 1;
*/
public java.lang.String getAddresses(int index) {
return addresses_.get(index);
}
/**
* repeated string addresses = 1;
*/
public com.google.protobuf.ByteString
getAddressesBytes(int index) {
return addresses_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < addresses_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, addresses_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < addresses_.size(); i++) {
dataSize += computeStringSizeNoTag(addresses_.getRaw(i));
}
size += dataSize;
size += 1 * getAddressesList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.ValAddresses)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.ValAddresses other = (cosmos.staking.v1beta1.Staking.ValAddresses) obj;
boolean result = true;
result = result && getAddressesList()
.equals(other.getAddressesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAddressesCount() > 0) {
hash = (37 * hash) + ADDRESSES_FIELD_NUMBER;
hash = (53 * hash) + getAddressesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.ValAddresses parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.ValAddresses prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ValAddresses defines a repeated set of validator addresses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.ValAddresses}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.ValAddresses)
cosmos.staking.v1beta1.Staking.ValAddressesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_ValAddresses_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_ValAddresses_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.ValAddresses.class, cosmos.staking.v1beta1.Staking.ValAddresses.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.ValAddresses.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
addresses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_ValAddresses_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.ValAddresses getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.ValAddresses.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.ValAddresses build() {
cosmos.staking.v1beta1.Staking.ValAddresses result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.ValAddresses buildPartial() {
cosmos.staking.v1beta1.Staking.ValAddresses result = new cosmos.staking.v1beta1.Staking.ValAddresses(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
addresses_ = addresses_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.addresses_ = addresses_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.ValAddresses) {
return mergeFrom((cosmos.staking.v1beta1.Staking.ValAddresses)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.ValAddresses other) {
if (other == cosmos.staking.v1beta1.Staking.ValAddresses.getDefaultInstance()) return this;
if (!other.addresses_.isEmpty()) {
if (addresses_.isEmpty()) {
addresses_ = other.addresses_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAddressesIsMutable();
addresses_.addAll(other.addresses_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.ValAddresses parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.ValAddresses) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList addresses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAddressesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
addresses_ = new com.google.protobuf.LazyStringArrayList(addresses_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string addresses = 1;
*/
public com.google.protobuf.ProtocolStringList
getAddressesList() {
return addresses_.getUnmodifiableView();
}
/**
* repeated string addresses = 1;
*/
public int getAddressesCount() {
return addresses_.size();
}
/**
* repeated string addresses = 1;
*/
public java.lang.String getAddresses(int index) {
return addresses_.get(index);
}
/**
* repeated string addresses = 1;
*/
public com.google.protobuf.ByteString
getAddressesBytes(int index) {
return addresses_.getByteString(index);
}
/**
* repeated string addresses = 1;
*/
public Builder setAddresses(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddressesIsMutable();
addresses_.set(index, value);
onChanged();
return this;
}
/**
* repeated string addresses = 1;
*/
public Builder addAddresses(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAddressesIsMutable();
addresses_.add(value);
onChanged();
return this;
}
/**
* repeated string addresses = 1;
*/
public Builder addAllAddresses(
java.lang.Iterable values) {
ensureAddressesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, addresses_);
onChanged();
return this;
}
/**
* repeated string addresses = 1;
*/
public Builder clearAddresses() {
addresses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string addresses = 1;
*/
public Builder addAddressesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAddressesIsMutable();
addresses_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.ValAddresses)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.ValAddresses)
private static final cosmos.staking.v1beta1.Staking.ValAddresses DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.ValAddresses();
}
public static cosmos.staking.v1beta1.Staking.ValAddresses getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValAddresses parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ValAddresses(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.ValAddresses getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DVPairOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.DVPair)
com.google.protobuf.MessageOrBuilder {
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
java.lang.String getValidatorAddress();
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
com.google.protobuf.ByteString
getValidatorAddressBytes();
}
/**
*
* DVPair is struct that just has a delegator-validator pair with no other data.
* It is intended to be used as a marshalable pointer. For example, a DVPair can
* be used to construct the key to getting an UnbondingDelegation from state.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVPair}
*/
public static final class DVPair extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.DVPair)
DVPairOrBuilder {
private static final long serialVersionUID = 0L;
// Use DVPair.newBuilder() to construct.
private DVPair(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DVPair() {
delegatorAddress_ = "";
validatorAddress_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DVPair(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
validatorAddress_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPair_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPair_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVPair.class, cosmos.staking.v1beta1.Staking.DVPair.Builder.class);
}
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object validatorAddress_;
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
}
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, validatorAddress_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, validatorAddress_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.DVPair)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.DVPair other = (cosmos.staking.v1beta1.Staking.DVPair) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getValidatorAddress()
.equals(other.getValidatorAddress());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + VALIDATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorAddress().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVPair parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.DVPair prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DVPair is struct that just has a delegator-validator pair with no other data.
* It is intended to be used as a marshalable pointer. For example, a DVPair can
* be used to construct the key to getting an UnbondingDelegation from state.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVPair}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.DVPair)
cosmos.staking.v1beta1.Staking.DVPairOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPair_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPair_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVPair.class, cosmos.staking.v1beta1.Staking.DVPair.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.DVPair.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
validatorAddress_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPair_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPair getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.DVPair.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPair build() {
cosmos.staking.v1beta1.Staking.DVPair result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPair buildPartial() {
cosmos.staking.v1beta1.Staking.DVPair result = new cosmos.staking.v1beta1.Staking.DVPair(this);
result.delegatorAddress_ = delegatorAddress_;
result.validatorAddress_ = validatorAddress_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.DVPair) {
return mergeFrom((cosmos.staking.v1beta1.Staking.DVPair)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.DVPair other) {
if (other == cosmos.staking.v1beta1.Staking.DVPair.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getValidatorAddress().isEmpty()) {
validatorAddress_ = other.validatorAddress_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.DVPair parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.DVPair) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object delegatorAddress_ = "";
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorAddress_ = "";
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorAddress_ = value;
onChanged();
return this;
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder clearValidatorAddress() {
validatorAddress_ = getDefaultInstance().getValidatorAddress();
onChanged();
return this;
}
/**
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorAddress_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.DVPair)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.DVPair)
private static final cosmos.staking.v1beta1.Staking.DVPair DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.DVPair();
}
public static cosmos.staking.v1beta1.Staking.DVPair getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DVPair parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DVPair(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPair getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DVPairsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.DVPairs)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
java.util.List
getPairsList();
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.DVPair getPairs(int index);
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
int getPairsCount();
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
java.util.List extends cosmos.staking.v1beta1.Staking.DVPairOrBuilder>
getPairsOrBuilderList();
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.DVPairOrBuilder getPairsOrBuilder(
int index);
}
/**
*
* DVPairs defines an array of DVPair objects.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVPairs}
*/
public static final class DVPairs extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.DVPairs)
DVPairsOrBuilder {
private static final long serialVersionUID = 0L;
// Use DVPairs.newBuilder() to construct.
private DVPairs(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DVPairs() {
pairs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DVPairs(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
pairs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
pairs_.add(
input.readMessage(cosmos.staking.v1beta1.Staking.DVPair.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
pairs_ = java.util.Collections.unmodifiableList(pairs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPairs_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPairs_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVPairs.class, cosmos.staking.v1beta1.Staking.DVPairs.Builder.class);
}
public static final int PAIRS_FIELD_NUMBER = 1;
private java.util.List pairs_;
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List getPairsList() {
return pairs_;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.DVPairOrBuilder>
getPairsOrBuilderList() {
return pairs_;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public int getPairsCount() {
return pairs_.size();
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPair getPairs(int index) {
return pairs_.get(index);
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPairOrBuilder getPairsOrBuilder(
int index) {
return pairs_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < pairs_.size(); i++) {
output.writeMessage(1, pairs_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < pairs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, pairs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.DVPairs)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.DVPairs other = (cosmos.staking.v1beta1.Staking.DVPairs) obj;
boolean result = true;
result = result && getPairsList()
.equals(other.getPairsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getPairsCount() > 0) {
hash = (37 * hash) + PAIRS_FIELD_NUMBER;
hash = (53 * hash) + getPairsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVPairs parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.DVPairs prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DVPairs defines an array of DVPair objects.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVPairs}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.DVPairs)
cosmos.staking.v1beta1.Staking.DVPairsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPairs_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPairs_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVPairs.class, cosmos.staking.v1beta1.Staking.DVPairs.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.DVPairs.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPairsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (pairsBuilder_ == null) {
pairs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
pairsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVPairs_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPairs getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.DVPairs.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPairs build() {
cosmos.staking.v1beta1.Staking.DVPairs result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPairs buildPartial() {
cosmos.staking.v1beta1.Staking.DVPairs result = new cosmos.staking.v1beta1.Staking.DVPairs(this);
int from_bitField0_ = bitField0_;
if (pairsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
pairs_ = java.util.Collections.unmodifiableList(pairs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.pairs_ = pairs_;
} else {
result.pairs_ = pairsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.DVPairs) {
return mergeFrom((cosmos.staking.v1beta1.Staking.DVPairs)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.DVPairs other) {
if (other == cosmos.staking.v1beta1.Staking.DVPairs.getDefaultInstance()) return this;
if (pairsBuilder_ == null) {
if (!other.pairs_.isEmpty()) {
if (pairs_.isEmpty()) {
pairs_ = other.pairs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensurePairsIsMutable();
pairs_.addAll(other.pairs_);
}
onChanged();
}
} else {
if (!other.pairs_.isEmpty()) {
if (pairsBuilder_.isEmpty()) {
pairsBuilder_.dispose();
pairsBuilder_ = null;
pairs_ = other.pairs_;
bitField0_ = (bitField0_ & ~0x00000001);
pairsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPairsFieldBuilder() : null;
} else {
pairsBuilder_.addAllMessages(other.pairs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.DVPairs parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.DVPairs) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List pairs_ =
java.util.Collections.emptyList();
private void ensurePairsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
pairs_ = new java.util.ArrayList(pairs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.DVPair, cosmos.staking.v1beta1.Staking.DVPair.Builder, cosmos.staking.v1beta1.Staking.DVPairOrBuilder> pairsBuilder_;
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List getPairsList() {
if (pairsBuilder_ == null) {
return java.util.Collections.unmodifiableList(pairs_);
} else {
return pairsBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public int getPairsCount() {
if (pairsBuilder_ == null) {
return pairs_.size();
} else {
return pairsBuilder_.getCount();
}
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPair getPairs(int index) {
if (pairsBuilder_ == null) {
return pairs_.get(index);
} else {
return pairsBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder setPairs(
int index, cosmos.staking.v1beta1.Staking.DVPair value) {
if (pairsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePairsIsMutable();
pairs_.set(index, value);
onChanged();
} else {
pairsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder setPairs(
int index, cosmos.staking.v1beta1.Staking.DVPair.Builder builderForValue) {
if (pairsBuilder_ == null) {
ensurePairsIsMutable();
pairs_.set(index, builderForValue.build());
onChanged();
} else {
pairsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder addPairs(cosmos.staking.v1beta1.Staking.DVPair value) {
if (pairsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePairsIsMutable();
pairs_.add(value);
onChanged();
} else {
pairsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder addPairs(
int index, cosmos.staking.v1beta1.Staking.DVPair value) {
if (pairsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePairsIsMutable();
pairs_.add(index, value);
onChanged();
} else {
pairsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder addPairs(
cosmos.staking.v1beta1.Staking.DVPair.Builder builderForValue) {
if (pairsBuilder_ == null) {
ensurePairsIsMutable();
pairs_.add(builderForValue.build());
onChanged();
} else {
pairsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder addPairs(
int index, cosmos.staking.v1beta1.Staking.DVPair.Builder builderForValue) {
if (pairsBuilder_ == null) {
ensurePairsIsMutable();
pairs_.add(index, builderForValue.build());
onChanged();
} else {
pairsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder addAllPairs(
java.lang.Iterable extends cosmos.staking.v1beta1.Staking.DVPair> values) {
if (pairsBuilder_ == null) {
ensurePairsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, pairs_);
onChanged();
} else {
pairsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearPairs() {
if (pairsBuilder_ == null) {
pairs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
pairsBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public Builder removePairs(int index) {
if (pairsBuilder_ == null) {
ensurePairsIsMutable();
pairs_.remove(index);
onChanged();
} else {
pairsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPair.Builder getPairsBuilder(
int index) {
return getPairsFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPairOrBuilder getPairsOrBuilder(
int index) {
if (pairsBuilder_ == null) {
return pairs_.get(index); } else {
return pairsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.DVPairOrBuilder>
getPairsOrBuilderList() {
if (pairsBuilder_ != null) {
return pairsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(pairs_);
}
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPair.Builder addPairsBuilder() {
return getPairsFieldBuilder().addBuilder(
cosmos.staking.v1beta1.Staking.DVPair.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVPair.Builder addPairsBuilder(
int index) {
return getPairsFieldBuilder().addBuilder(
index, cosmos.staking.v1beta1.Staking.DVPair.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.DVPair pairs = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List
getPairsBuilderList() {
return getPairsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.DVPair, cosmos.staking.v1beta1.Staking.DVPair.Builder, cosmos.staking.v1beta1.Staking.DVPairOrBuilder>
getPairsFieldBuilder() {
if (pairsBuilder_ == null) {
pairsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.DVPair, cosmos.staking.v1beta1.Staking.DVPair.Builder, cosmos.staking.v1beta1.Staking.DVPairOrBuilder>(
pairs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
pairs_ = null;
}
return pairsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.DVPairs)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.DVPairs)
private static final cosmos.staking.v1beta1.Staking.DVPairs DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.DVPairs();
}
public static cosmos.staking.v1beta1.Staking.DVPairs getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DVPairs parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DVPairs(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVPairs getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DVVTripletOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.DVVTriplet)
com.google.protobuf.MessageOrBuilder {
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
java.lang.String getValidatorSrcAddress();
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
com.google.protobuf.ByteString
getValidatorSrcAddressBytes();
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
java.lang.String getValidatorDstAddress();
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
com.google.protobuf.ByteString
getValidatorDstAddressBytes();
}
/**
*
* DVVTriplet is struct that just has a delegator-validator-validator triplet
* with no other data. It is intended to be used as a marshalable pointer. For
* example, a DVVTriplet can be used to construct the key to getting a
* Redelegation from state.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVVTriplet}
*/
public static final class DVVTriplet extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.DVVTriplet)
DVVTripletOrBuilder {
private static final long serialVersionUID = 0L;
// Use DVVTriplet.newBuilder() to construct.
private DVVTriplet(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DVVTriplet() {
delegatorAddress_ = "";
validatorSrcAddress_ = "";
validatorDstAddress_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DVVTriplet(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
validatorSrcAddress_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
validatorDstAddress_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVVTriplet.class, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder.class);
}
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_SRC_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object validatorSrcAddress_;
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public java.lang.String getValidatorSrcAddress() {
java.lang.Object ref = validatorSrcAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorSrcAddress_ = s;
return s;
}
}
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public com.google.protobuf.ByteString
getValidatorSrcAddressBytes() {
java.lang.Object ref = validatorSrcAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorSrcAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_DST_ADDRESS_FIELD_NUMBER = 3;
private volatile java.lang.Object validatorDstAddress_;
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public java.lang.String getValidatorDstAddress() {
java.lang.Object ref = validatorDstAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorDstAddress_ = s;
return s;
}
}
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public com.google.protobuf.ByteString
getValidatorDstAddressBytes() {
java.lang.Object ref = validatorDstAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorDstAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getValidatorSrcAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, validatorSrcAddress_);
}
if (!getValidatorDstAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, validatorDstAddress_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getValidatorSrcAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, validatorSrcAddress_);
}
if (!getValidatorDstAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, validatorDstAddress_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.DVVTriplet)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.DVVTriplet other = (cosmos.staking.v1beta1.Staking.DVVTriplet) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getValidatorSrcAddress()
.equals(other.getValidatorSrcAddress());
result = result && getValidatorDstAddress()
.equals(other.getValidatorDstAddress());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + VALIDATOR_SRC_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorSrcAddress().hashCode();
hash = (37 * hash) + VALIDATOR_DST_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorDstAddress().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.DVVTriplet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DVVTriplet is struct that just has a delegator-validator-validator triplet
* with no other data. It is intended to be used as a marshalable pointer. For
* example, a DVVTriplet can be used to construct the key to getting a
* Redelegation from state.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVVTriplet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.DVVTriplet)
cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVVTriplet.class, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.DVVTriplet.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
validatorSrcAddress_ = "";
validatorDstAddress_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplet_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplet getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.DVVTriplet.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplet build() {
cosmos.staking.v1beta1.Staking.DVVTriplet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplet buildPartial() {
cosmos.staking.v1beta1.Staking.DVVTriplet result = new cosmos.staking.v1beta1.Staking.DVVTriplet(this);
result.delegatorAddress_ = delegatorAddress_;
result.validatorSrcAddress_ = validatorSrcAddress_;
result.validatorDstAddress_ = validatorDstAddress_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.DVVTriplet) {
return mergeFrom((cosmos.staking.v1beta1.Staking.DVVTriplet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.DVVTriplet other) {
if (other == cosmos.staking.v1beta1.Staking.DVVTriplet.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getValidatorSrcAddress().isEmpty()) {
validatorSrcAddress_ = other.validatorSrcAddress_;
onChanged();
}
if (!other.getValidatorDstAddress().isEmpty()) {
validatorDstAddress_ = other.validatorDstAddress_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.DVVTriplet parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.DVVTriplet) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object delegatorAddress_ = "";
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorSrcAddress_ = "";
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public java.lang.String getValidatorSrcAddress() {
java.lang.Object ref = validatorSrcAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorSrcAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public com.google.protobuf.ByteString
getValidatorSrcAddressBytes() {
java.lang.Object ref = validatorSrcAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorSrcAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public Builder setValidatorSrcAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorSrcAddress_ = value;
onChanged();
return this;
}
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public Builder clearValidatorSrcAddress() {
validatorSrcAddress_ = getDefaultInstance().getValidatorSrcAddress();
onChanged();
return this;
}
/**
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public Builder setValidatorSrcAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorSrcAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorDstAddress_ = "";
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public java.lang.String getValidatorDstAddress() {
java.lang.Object ref = validatorDstAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorDstAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public com.google.protobuf.ByteString
getValidatorDstAddressBytes() {
java.lang.Object ref = validatorDstAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorDstAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public Builder setValidatorDstAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorDstAddress_ = value;
onChanged();
return this;
}
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public Builder clearValidatorDstAddress() {
validatorDstAddress_ = getDefaultInstance().getValidatorDstAddress();
onChanged();
return this;
}
/**
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public Builder setValidatorDstAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorDstAddress_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.DVVTriplet)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.DVVTriplet)
private static final cosmos.staking.v1beta1.Staking.DVVTriplet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.DVVTriplet();
}
public static cosmos.staking.v1beta1.Staking.DVVTriplet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DVVTriplet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DVVTriplet(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DVVTripletsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.DVVTriplets)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
java.util.List
getTripletsList();
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.DVVTriplet getTriplets(int index);
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
int getTripletsCount();
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
java.util.List extends cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder>
getTripletsOrBuilderList();
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder getTripletsOrBuilder(
int index);
}
/**
*
* DVVTriplets defines an array of DVVTriplet objects.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVVTriplets}
*/
public static final class DVVTriplets extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.DVVTriplets)
DVVTripletsOrBuilder {
private static final long serialVersionUID = 0L;
// Use DVVTriplets.newBuilder() to construct.
private DVVTriplets(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DVVTriplets() {
triplets_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DVVTriplets(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
triplets_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
triplets_.add(
input.readMessage(cosmos.staking.v1beta1.Staking.DVVTriplet.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
triplets_ = java.util.Collections.unmodifiableList(triplets_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplets_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplets_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVVTriplets.class, cosmos.staking.v1beta1.Staking.DVVTriplets.Builder.class);
}
public static final int TRIPLETS_FIELD_NUMBER = 1;
private java.util.List triplets_;
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List getTripletsList() {
return triplets_;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder>
getTripletsOrBuilderList() {
return triplets_;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public int getTripletsCount() {
return triplets_.size();
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTriplet getTriplets(int index) {
return triplets_.get(index);
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder getTripletsOrBuilder(
int index) {
return triplets_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < triplets_.size(); i++) {
output.writeMessage(1, triplets_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < triplets_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, triplets_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.DVVTriplets)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.DVVTriplets other = (cosmos.staking.v1beta1.Staking.DVVTriplets) obj;
boolean result = true;
result = result && getTripletsList()
.equals(other.getTripletsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTripletsCount() > 0) {
hash = (37 * hash) + TRIPLETS_FIELD_NUMBER;
hash = (53 * hash) + getTripletsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.DVVTriplets prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DVVTriplets defines an array of DVVTriplet objects.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DVVTriplets}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.DVVTriplets)
cosmos.staking.v1beta1.Staking.DVVTripletsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplets_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplets_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DVVTriplets.class, cosmos.staking.v1beta1.Staking.DVVTriplets.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.DVVTriplets.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTripletsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tripletsBuilder_ == null) {
triplets_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
tripletsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DVVTriplets_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplets getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.DVVTriplets.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplets build() {
cosmos.staking.v1beta1.Staking.DVVTriplets result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplets buildPartial() {
cosmos.staking.v1beta1.Staking.DVVTriplets result = new cosmos.staking.v1beta1.Staking.DVVTriplets(this);
int from_bitField0_ = bitField0_;
if (tripletsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
triplets_ = java.util.Collections.unmodifiableList(triplets_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.triplets_ = triplets_;
} else {
result.triplets_ = tripletsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.DVVTriplets) {
return mergeFrom((cosmos.staking.v1beta1.Staking.DVVTriplets)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.DVVTriplets other) {
if (other == cosmos.staking.v1beta1.Staking.DVVTriplets.getDefaultInstance()) return this;
if (tripletsBuilder_ == null) {
if (!other.triplets_.isEmpty()) {
if (triplets_.isEmpty()) {
triplets_ = other.triplets_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTripletsIsMutable();
triplets_.addAll(other.triplets_);
}
onChanged();
}
} else {
if (!other.triplets_.isEmpty()) {
if (tripletsBuilder_.isEmpty()) {
tripletsBuilder_.dispose();
tripletsBuilder_ = null;
triplets_ = other.triplets_;
bitField0_ = (bitField0_ & ~0x00000001);
tripletsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTripletsFieldBuilder() : null;
} else {
tripletsBuilder_.addAllMessages(other.triplets_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.DVVTriplets parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.DVVTriplets) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List triplets_ =
java.util.Collections.emptyList();
private void ensureTripletsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
triplets_ = new java.util.ArrayList(triplets_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.DVVTriplet, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder, cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder> tripletsBuilder_;
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List getTripletsList() {
if (tripletsBuilder_ == null) {
return java.util.Collections.unmodifiableList(triplets_);
} else {
return tripletsBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public int getTripletsCount() {
if (tripletsBuilder_ == null) {
return triplets_.size();
} else {
return tripletsBuilder_.getCount();
}
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTriplet getTriplets(int index) {
if (tripletsBuilder_ == null) {
return triplets_.get(index);
} else {
return tripletsBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder setTriplets(
int index, cosmos.staking.v1beta1.Staking.DVVTriplet value) {
if (tripletsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTripletsIsMutable();
triplets_.set(index, value);
onChanged();
} else {
tripletsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder setTriplets(
int index, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder builderForValue) {
if (tripletsBuilder_ == null) {
ensureTripletsIsMutable();
triplets_.set(index, builderForValue.build());
onChanged();
} else {
tripletsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder addTriplets(cosmos.staking.v1beta1.Staking.DVVTriplet value) {
if (tripletsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTripletsIsMutable();
triplets_.add(value);
onChanged();
} else {
tripletsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder addTriplets(
int index, cosmos.staking.v1beta1.Staking.DVVTriplet value) {
if (tripletsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTripletsIsMutable();
triplets_.add(index, value);
onChanged();
} else {
tripletsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder addTriplets(
cosmos.staking.v1beta1.Staking.DVVTriplet.Builder builderForValue) {
if (tripletsBuilder_ == null) {
ensureTripletsIsMutable();
triplets_.add(builderForValue.build());
onChanged();
} else {
tripletsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder addTriplets(
int index, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder builderForValue) {
if (tripletsBuilder_ == null) {
ensureTripletsIsMutable();
triplets_.add(index, builderForValue.build());
onChanged();
} else {
tripletsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder addAllTriplets(
java.lang.Iterable extends cosmos.staking.v1beta1.Staking.DVVTriplet> values) {
if (tripletsBuilder_ == null) {
ensureTripletsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, triplets_);
onChanged();
} else {
tripletsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearTriplets() {
if (tripletsBuilder_ == null) {
triplets_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
tripletsBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public Builder removeTriplets(int index) {
if (tripletsBuilder_ == null) {
ensureTripletsIsMutable();
triplets_.remove(index);
onChanged();
} else {
tripletsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTriplet.Builder getTripletsBuilder(
int index) {
return getTripletsFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder getTripletsOrBuilder(
int index) {
if (tripletsBuilder_ == null) {
return triplets_.get(index); } else {
return tripletsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder>
getTripletsOrBuilderList() {
if (tripletsBuilder_ != null) {
return tripletsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(triplets_);
}
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTriplet.Builder addTripletsBuilder() {
return getTripletsFieldBuilder().addBuilder(
cosmos.staking.v1beta1.Staking.DVVTriplet.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DVVTriplet.Builder addTripletsBuilder(
int index) {
return getTripletsFieldBuilder().addBuilder(
index, cosmos.staking.v1beta1.Staking.DVVTriplet.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.DVVTriplet triplets = 1 [(.gogoproto.nullable) = false];
*/
public java.util.List
getTripletsBuilderList() {
return getTripletsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.DVVTriplet, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder, cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder>
getTripletsFieldBuilder() {
if (tripletsBuilder_ == null) {
tripletsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.DVVTriplet, cosmos.staking.v1beta1.Staking.DVVTriplet.Builder, cosmos.staking.v1beta1.Staking.DVVTripletOrBuilder>(
triplets_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
triplets_ = null;
}
return tripletsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.DVVTriplets)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.DVVTriplets)
private static final cosmos.staking.v1beta1.Staking.DVVTriplets DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.DVVTriplets();
}
public static cosmos.staking.v1beta1.Staking.DVVTriplets getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DVVTriplets parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DVVTriplets(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DVVTriplets getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DelegationOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Delegation)
com.google.protobuf.MessageOrBuilder {
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
java.lang.String getValidatorAddress();
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
com.google.protobuf.ByteString
getValidatorAddressBytes();
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
java.lang.String getShares();
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
com.google.protobuf.ByteString
getSharesBytes();
}
/**
*
* Delegation represents the bond with tokens held by an account. It is
* owned by one delegator, and is associated with the voting power of one
* validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Delegation}
*/
public static final class Delegation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Delegation)
DelegationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Delegation.newBuilder() to construct.
private Delegation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Delegation() {
delegatorAddress_ = "";
validatorAddress_ = "";
shares_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Delegation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
validatorAddress_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
shares_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Delegation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Delegation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Delegation.class, cosmos.staking.v1beta1.Staking.Delegation.Builder.class);
}
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object validatorAddress_;
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
}
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHARES_FIELD_NUMBER = 3;
private volatile java.lang.Object shares_;
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getShares() {
java.lang.Object ref = shares_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
shares_ = s;
return s;
}
}
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getSharesBytes() {
java.lang.Object ref = shares_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shares_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, validatorAddress_);
}
if (!getSharesBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, shares_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, validatorAddress_);
}
if (!getSharesBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, shares_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Delegation)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Delegation other = (cosmos.staking.v1beta1.Staking.Delegation) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getValidatorAddress()
.equals(other.getValidatorAddress());
result = result && getShares()
.equals(other.getShares());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + VALIDATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorAddress().hashCode();
hash = (37 * hash) + SHARES_FIELD_NUMBER;
hash = (53 * hash) + getShares().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Delegation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Delegation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Delegation represents the bond with tokens held by an account. It is
* owned by one delegator, and is associated with the voting power of one
* validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Delegation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Delegation)
cosmos.staking.v1beta1.Staking.DelegationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Delegation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Delegation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Delegation.class, cosmos.staking.v1beta1.Staking.Delegation.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Delegation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
validatorAddress_ = "";
shares_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Delegation_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Delegation getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Delegation.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Delegation build() {
cosmos.staking.v1beta1.Staking.Delegation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Delegation buildPartial() {
cosmos.staking.v1beta1.Staking.Delegation result = new cosmos.staking.v1beta1.Staking.Delegation(this);
result.delegatorAddress_ = delegatorAddress_;
result.validatorAddress_ = validatorAddress_;
result.shares_ = shares_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Delegation) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Delegation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Delegation other) {
if (other == cosmos.staking.v1beta1.Staking.Delegation.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getValidatorAddress().isEmpty()) {
validatorAddress_ = other.validatorAddress_;
onChanged();
}
if (!other.getShares().isEmpty()) {
shares_ = other.shares_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Delegation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Delegation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object delegatorAddress_ = "";
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorAddress_ = "";
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorAddress_ = value;
onChanged();
return this;
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder clearValidatorAddress() {
validatorAddress_ = getDefaultInstance().getValidatorAddress();
onChanged();
return this;
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object shares_ = "";
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getShares() {
java.lang.Object ref = shares_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
shares_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getSharesBytes() {
java.lang.Object ref = shares_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shares_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setShares(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
shares_ = value;
onChanged();
return this;
}
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder clearShares() {
shares_ = getDefaultInstance().getShares();
onChanged();
return this;
}
/**
*
* shares define the delegation shares received.
*
*
* string shares = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setSharesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
shares_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Delegation)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Delegation)
private static final cosmos.staking.v1beta1.Staking.Delegation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Delegation();
}
public static cosmos.staking.v1beta1.Staking.Delegation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Delegation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Delegation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Delegation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnbondingDelegationOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.UnbondingDelegation)
com.google.protobuf.MessageOrBuilder {
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
java.lang.String getValidatorAddress();
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
com.google.protobuf.ByteString
getValidatorAddressBytes();
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
java.util.List
getEntriesList();
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry getEntries(int index);
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
int getEntriesCount();
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
java.util.List extends cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder>
getEntriesOrBuilderList();
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder getEntriesOrBuilder(
int index);
}
/**
*
* UnbondingDelegation stores all of a single delegator's unbonding bonds
* for a single validator in an time-ordered list.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.UnbondingDelegation}
*/
public static final class UnbondingDelegation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.UnbondingDelegation)
UnbondingDelegationOrBuilder {
private static final long serialVersionUID = 0L;
// Use UnbondingDelegation.newBuilder() to construct.
private UnbondingDelegation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UnbondingDelegation() {
delegatorAddress_ = "";
validatorAddress_ = "";
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UnbondingDelegation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
validatorAddress_ = s;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
entries_.add(
input.readMessage(cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.UnbondingDelegation.class, cosmos.staking.v1beta1.Staking.UnbondingDelegation.Builder.class);
}
private int bitField0_;
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object validatorAddress_;
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
}
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENTRIES_FIELD_NUMBER = 3;
private java.util.List entries_;
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public int getEntriesCount() {
return entries_.size();
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry getEntries(int index) {
return entries_.get(index);
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, validatorAddress_);
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(3, entries_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getValidatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, validatorAddress_);
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, entries_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.UnbondingDelegation)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.UnbondingDelegation other = (cosmos.staking.v1beta1.Staking.UnbondingDelegation) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getValidatorAddress()
.equals(other.getValidatorAddress());
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + VALIDATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorAddress().hashCode();
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.UnbondingDelegation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* UnbondingDelegation stores all of a single delegator's unbonding bonds
* for a single validator in an time-ordered list.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.UnbondingDelegation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.UnbondingDelegation)
cosmos.staking.v1beta1.Staking.UnbondingDelegationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.UnbondingDelegation.class, cosmos.staking.v1beta1.Staking.UnbondingDelegation.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.UnbondingDelegation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
validatorAddress_ = "";
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
entriesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegation_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegation getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.UnbondingDelegation.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegation build() {
cosmos.staking.v1beta1.Staking.UnbondingDelegation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegation buildPartial() {
cosmos.staking.v1beta1.Staking.UnbondingDelegation result = new cosmos.staking.v1beta1.Staking.UnbondingDelegation(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.delegatorAddress_ = delegatorAddress_;
result.validatorAddress_ = validatorAddress_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.UnbondingDelegation) {
return mergeFrom((cosmos.staking.v1beta1.Staking.UnbondingDelegation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.UnbondingDelegation other) {
if (other == cosmos.staking.v1beta1.Staking.UnbondingDelegation.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getValidatorAddress().isEmpty()) {
validatorAddress_ = other.validatorAddress_;
onChanged();
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000004);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.UnbondingDelegation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.UnbondingDelegation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object delegatorAddress_ = "";
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorAddress_ = "";
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public java.lang.String getValidatorAddress() {
java.lang.Object ref = validatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public com.google.protobuf.ByteString
getValidatorAddressBytes() {
java.lang.Object ref = validatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorAddress_ = value;
onChanged();
return this;
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder clearValidatorAddress() {
validatorAddress_ = getDefaultInstance().getValidatorAddress();
onChanged();
return this;
}
/**
*
* validator_address is the bech32-encoded address of the validator.
*
*
* string validator_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_address\""];
*/
public Builder setValidatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorAddress_ = value;
onChanged();
return this;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder> entriesBuilder_;
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder setEntries(
int index, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder setEntries(
int index, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
int index, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
int index, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder addAllEntries(
java.lang.Iterable extends cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.getDefaultInstance());
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.getDefaultInstance());
}
/**
*
* entries are the unbonding delegation entries.
*
*
* repeated .cosmos.staking.v1beta1.UnbondingDelegationEntry entries = 3 [(.gogoproto.nullable) = false];
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder>(
entries_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.UnbondingDelegation)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.UnbondingDelegation)
private static final cosmos.staking.v1beta1.Staking.UnbondingDelegation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.UnbondingDelegation();
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UnbondingDelegation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UnbondingDelegation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnbondingDelegationEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.UnbondingDelegationEntry)
com.google.protobuf.MessageOrBuilder {
/**
*
* creation_height is the height which the unbonding took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
long getCreationHeight();
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasCompletionTime();
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getCompletionTime();
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getCompletionTimeOrBuilder();
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
java.lang.String getInitialBalance();
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
com.google.protobuf.ByteString
getInitialBalanceBytes();
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
java.lang.String getBalance();
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
com.google.protobuf.ByteString
getBalanceBytes();
}
/**
*
* UnbondingDelegationEntry defines an unbonding object with relevant metadata.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.UnbondingDelegationEntry}
*/
public static final class UnbondingDelegationEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.UnbondingDelegationEntry)
UnbondingDelegationEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use UnbondingDelegationEntry.newBuilder() to construct.
private UnbondingDelegationEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UnbondingDelegationEntry() {
creationHeight_ = 0L;
initialBalance_ = "";
balance_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UnbondingDelegationEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
creationHeight_ = input.readInt64();
break;
}
case 18: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (completionTime_ != null) {
subBuilder = completionTime_.toBuilder();
}
completionTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(completionTime_);
completionTime_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
initialBalance_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
balance_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.class, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder.class);
}
public static final int CREATION_HEIGHT_FIELD_NUMBER = 1;
private long creationHeight_;
/**
*
* creation_height is the height which the unbonding took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public long getCreationHeight() {
return creationHeight_;
}
public static final int COMPLETION_TIME_FIELD_NUMBER = 2;
private com.google.protobuf.Timestamp completionTime_;
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasCompletionTime() {
return completionTime_ != null;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getCompletionTime() {
return completionTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : completionTime_;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getCompletionTimeOrBuilder() {
return getCompletionTime();
}
public static final int INITIAL_BALANCE_FIELD_NUMBER = 3;
private volatile java.lang.Object initialBalance_;
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public java.lang.String getInitialBalance() {
java.lang.Object ref = initialBalance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
initialBalance_ = s;
return s;
}
}
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public com.google.protobuf.ByteString
getInitialBalanceBytes() {
java.lang.Object ref = initialBalance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
initialBalance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BALANCE_FIELD_NUMBER = 4;
private volatile java.lang.Object balance_;
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getBalance() {
java.lang.Object ref = balance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
balance_ = s;
return s;
}
}
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getBalanceBytes() {
java.lang.Object ref = balance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
balance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (creationHeight_ != 0L) {
output.writeInt64(1, creationHeight_);
}
if (completionTime_ != null) {
output.writeMessage(2, getCompletionTime());
}
if (!getInitialBalanceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, initialBalance_);
}
if (!getBalanceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, balance_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (creationHeight_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, creationHeight_);
}
if (completionTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCompletionTime());
}
if (!getInitialBalanceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, initialBalance_);
}
if (!getBalanceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, balance_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry other = (cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry) obj;
boolean result = true;
result = result && (getCreationHeight()
== other.getCreationHeight());
result = result && (hasCompletionTime() == other.hasCompletionTime());
if (hasCompletionTime()) {
result = result && getCompletionTime()
.equals(other.getCompletionTime());
}
result = result && getInitialBalance()
.equals(other.getInitialBalance());
result = result && getBalance()
.equals(other.getBalance());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CREATION_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreationHeight());
if (hasCompletionTime()) {
hash = (37 * hash) + COMPLETION_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCompletionTime().hashCode();
}
hash = (37 * hash) + INITIAL_BALANCE_FIELD_NUMBER;
hash = (53 * hash) + getInitialBalance().hashCode();
hash = (37 * hash) + BALANCE_FIELD_NUMBER;
hash = (53 * hash) + getBalance().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* UnbondingDelegationEntry defines an unbonding object with relevant metadata.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.UnbondingDelegationEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.UnbondingDelegationEntry)
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.class, cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
creationHeight_ = 0L;
if (completionTimeBuilder_ == null) {
completionTime_ = null;
} else {
completionTime_ = null;
completionTimeBuilder_ = null;
}
initialBalance_ = "";
balance_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry build() {
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry buildPartial() {
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry result = new cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry(this);
result.creationHeight_ = creationHeight_;
if (completionTimeBuilder_ == null) {
result.completionTime_ = completionTime_;
} else {
result.completionTime_ = completionTimeBuilder_.build();
}
result.initialBalance_ = initialBalance_;
result.balance_ = balance_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry) {
return mergeFrom((cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry other) {
if (other == cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry.getDefaultInstance()) return this;
if (other.getCreationHeight() != 0L) {
setCreationHeight(other.getCreationHeight());
}
if (other.hasCompletionTime()) {
mergeCompletionTime(other.getCompletionTime());
}
if (!other.getInitialBalance().isEmpty()) {
initialBalance_ = other.initialBalance_;
onChanged();
}
if (!other.getBalance().isEmpty()) {
balance_ = other.balance_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long creationHeight_ ;
/**
*
* creation_height is the height which the unbonding took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public long getCreationHeight() {
return creationHeight_;
}
/**
*
* creation_height is the height which the unbonding took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public Builder setCreationHeight(long value) {
creationHeight_ = value;
onChanged();
return this;
}
/**
*
* creation_height is the height which the unbonding took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public Builder clearCreationHeight() {
creationHeight_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Timestamp completionTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> completionTimeBuilder_;
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasCompletionTime() {
return completionTimeBuilder_ != null || completionTime_ != null;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getCompletionTime() {
if (completionTimeBuilder_ == null) {
return completionTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : completionTime_;
} else {
return completionTimeBuilder_.getMessage();
}
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setCompletionTime(com.google.protobuf.Timestamp value) {
if (completionTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
completionTime_ = value;
onChanged();
} else {
completionTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setCompletionTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (completionTimeBuilder_ == null) {
completionTime_ = builderForValue.build();
onChanged();
} else {
completionTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeCompletionTime(com.google.protobuf.Timestamp value) {
if (completionTimeBuilder_ == null) {
if (completionTime_ != null) {
completionTime_ =
com.google.protobuf.Timestamp.newBuilder(completionTime_).mergeFrom(value).buildPartial();
} else {
completionTime_ = value;
}
onChanged();
} else {
completionTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearCompletionTime() {
if (completionTimeBuilder_ == null) {
completionTime_ = null;
onChanged();
} else {
completionTime_ = null;
completionTimeBuilder_ = null;
}
return this;
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getCompletionTimeBuilder() {
onChanged();
return getCompletionTimeFieldBuilder().getBuilder();
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getCompletionTimeOrBuilder() {
if (completionTimeBuilder_ != null) {
return completionTimeBuilder_.getMessageOrBuilder();
} else {
return completionTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : completionTime_;
}
}
/**
*
* completion_time is the unix time for unbonding completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getCompletionTimeFieldBuilder() {
if (completionTimeBuilder_ == null) {
completionTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getCompletionTime(),
getParentForChildren(),
isClean());
completionTime_ = null;
}
return completionTimeBuilder_;
}
private java.lang.Object initialBalance_ = "";
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public java.lang.String getInitialBalance() {
java.lang.Object ref = initialBalance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
initialBalance_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public com.google.protobuf.ByteString
getInitialBalanceBytes() {
java.lang.Object ref = initialBalance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
initialBalance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public Builder setInitialBalance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
initialBalance_ = value;
onChanged();
return this;
}
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public Builder clearInitialBalance() {
initialBalance_ = getDefaultInstance().getInitialBalance();
onChanged();
return this;
}
/**
*
* initial_balance defines the tokens initially scheduled to receive at completion.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public Builder setInitialBalanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
initialBalance_ = value;
onChanged();
return this;
}
private java.lang.Object balance_ = "";
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getBalance() {
java.lang.Object ref = balance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
balance_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getBalanceBytes() {
java.lang.Object ref = balance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
balance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setBalance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
balance_ = value;
onChanged();
return this;
}
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder clearBalance() {
balance_ = getDefaultInstance().getBalance();
onChanged();
return this;
}
/**
*
* balance defines the tokens to receive at completion.
*
*
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setBalanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
balance_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.UnbondingDelegationEntry)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.UnbondingDelegationEntry)
private static final cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry();
}
public static cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UnbondingDelegationEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UnbondingDelegationEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.UnbondingDelegationEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RedelegationEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.RedelegationEntry)
com.google.protobuf.MessageOrBuilder {
/**
*
* creation_height defines the height which the redelegation took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
long getCreationHeight();
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
boolean hasCompletionTime();
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.Timestamp getCompletionTime();
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
com.google.protobuf.TimestampOrBuilder getCompletionTimeOrBuilder();
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
java.lang.String getInitialBalance();
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
com.google.protobuf.ByteString
getInitialBalanceBytes();
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
java.lang.String getSharesDst();
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
com.google.protobuf.ByteString
getSharesDstBytes();
}
/**
*
* RedelegationEntry defines a redelegation object with relevant metadata.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.RedelegationEntry}
*/
public static final class RedelegationEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.RedelegationEntry)
RedelegationEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use RedelegationEntry.newBuilder() to construct.
private RedelegationEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RedelegationEntry() {
creationHeight_ = 0L;
initialBalance_ = "";
sharesDst_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RedelegationEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
creationHeight_ = input.readInt64();
break;
}
case 18: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (completionTime_ != null) {
subBuilder = completionTime_.toBuilder();
}
completionTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(completionTime_);
completionTime_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
initialBalance_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
sharesDst_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.RedelegationEntry.class, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder.class);
}
public static final int CREATION_HEIGHT_FIELD_NUMBER = 1;
private long creationHeight_;
/**
*
* creation_height defines the height which the redelegation took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public long getCreationHeight() {
return creationHeight_;
}
public static final int COMPLETION_TIME_FIELD_NUMBER = 2;
private com.google.protobuf.Timestamp completionTime_;
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasCompletionTime() {
return completionTime_ != null;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getCompletionTime() {
return completionTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : completionTime_;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getCompletionTimeOrBuilder() {
return getCompletionTime();
}
public static final int INITIAL_BALANCE_FIELD_NUMBER = 3;
private volatile java.lang.Object initialBalance_;
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public java.lang.String getInitialBalance() {
java.lang.Object ref = initialBalance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
initialBalance_ = s;
return s;
}
}
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public com.google.protobuf.ByteString
getInitialBalanceBytes() {
java.lang.Object ref = initialBalance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
initialBalance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHARES_DST_FIELD_NUMBER = 4;
private volatile java.lang.Object sharesDst_;
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getSharesDst() {
java.lang.Object ref = sharesDst_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sharesDst_ = s;
return s;
}
}
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getSharesDstBytes() {
java.lang.Object ref = sharesDst_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sharesDst_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (creationHeight_ != 0L) {
output.writeInt64(1, creationHeight_);
}
if (completionTime_ != null) {
output.writeMessage(2, getCompletionTime());
}
if (!getInitialBalanceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, initialBalance_);
}
if (!getSharesDstBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, sharesDst_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (creationHeight_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, creationHeight_);
}
if (completionTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCompletionTime());
}
if (!getInitialBalanceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, initialBalance_);
}
if (!getSharesDstBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, sharesDst_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.RedelegationEntry)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.RedelegationEntry other = (cosmos.staking.v1beta1.Staking.RedelegationEntry) obj;
boolean result = true;
result = result && (getCreationHeight()
== other.getCreationHeight());
result = result && (hasCompletionTime() == other.hasCompletionTime());
if (hasCompletionTime()) {
result = result && getCompletionTime()
.equals(other.getCompletionTime());
}
result = result && getInitialBalance()
.equals(other.getInitialBalance());
result = result && getSharesDst()
.equals(other.getSharesDst());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CREATION_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreationHeight());
if (hasCompletionTime()) {
hash = (37 * hash) + COMPLETION_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCompletionTime().hashCode();
}
hash = (37 * hash) + INITIAL_BALANCE_FIELD_NUMBER;
hash = (53 * hash) + getInitialBalance().hashCode();
hash = (37 * hash) + SHARES_DST_FIELD_NUMBER;
hash = (53 * hash) + getSharesDst().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.RedelegationEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* RedelegationEntry defines a redelegation object with relevant metadata.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.RedelegationEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.RedelegationEntry)
cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.RedelegationEntry.class, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.RedelegationEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
creationHeight_ = 0L;
if (completionTimeBuilder_ == null) {
completionTime_ = null;
} else {
completionTime_ = null;
completionTimeBuilder_ = null;
}
initialBalance_ = "";
sharesDst_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntry_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntry getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntry build() {
cosmos.staking.v1beta1.Staking.RedelegationEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntry buildPartial() {
cosmos.staking.v1beta1.Staking.RedelegationEntry result = new cosmos.staking.v1beta1.Staking.RedelegationEntry(this);
result.creationHeight_ = creationHeight_;
if (completionTimeBuilder_ == null) {
result.completionTime_ = completionTime_;
} else {
result.completionTime_ = completionTimeBuilder_.build();
}
result.initialBalance_ = initialBalance_;
result.sharesDst_ = sharesDst_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.RedelegationEntry) {
return mergeFrom((cosmos.staking.v1beta1.Staking.RedelegationEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.RedelegationEntry other) {
if (other == cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance()) return this;
if (other.getCreationHeight() != 0L) {
setCreationHeight(other.getCreationHeight());
}
if (other.hasCompletionTime()) {
mergeCompletionTime(other.getCompletionTime());
}
if (!other.getInitialBalance().isEmpty()) {
initialBalance_ = other.initialBalance_;
onChanged();
}
if (!other.getSharesDst().isEmpty()) {
sharesDst_ = other.sharesDst_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.RedelegationEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.RedelegationEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long creationHeight_ ;
/**
*
* creation_height defines the height which the redelegation took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public long getCreationHeight() {
return creationHeight_;
}
/**
*
* creation_height defines the height which the redelegation took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public Builder setCreationHeight(long value) {
creationHeight_ = value;
onChanged();
return this;
}
/**
*
* creation_height defines the height which the redelegation took place.
*
*
* int64 creation_height = 1 [(.gogoproto.moretags) = "yaml:\"creation_height\""];
*/
public Builder clearCreationHeight() {
creationHeight_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Timestamp completionTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> completionTimeBuilder_;
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public boolean hasCompletionTime() {
return completionTimeBuilder_ != null || completionTime_ != null;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp getCompletionTime() {
if (completionTimeBuilder_ == null) {
return completionTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : completionTime_;
} else {
return completionTimeBuilder_.getMessage();
}
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setCompletionTime(com.google.protobuf.Timestamp value) {
if (completionTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
completionTime_ = value;
onChanged();
} else {
completionTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder setCompletionTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (completionTimeBuilder_ == null) {
completionTime_ = builderForValue.build();
onChanged();
} else {
completionTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder mergeCompletionTime(com.google.protobuf.Timestamp value) {
if (completionTimeBuilder_ == null) {
if (completionTime_ != null) {
completionTime_ =
com.google.protobuf.Timestamp.newBuilder(completionTime_).mergeFrom(value).buildPartial();
} else {
completionTime_ = value;
}
onChanged();
} else {
completionTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public Builder clearCompletionTime() {
if (completionTimeBuilder_ == null) {
completionTime_ = null;
onChanged();
} else {
completionTime_ = null;
completionTimeBuilder_ = null;
}
return this;
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.Timestamp.Builder getCompletionTimeBuilder() {
onChanged();
return getCompletionTimeFieldBuilder().getBuilder();
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
public com.google.protobuf.TimestampOrBuilder getCompletionTimeOrBuilder() {
if (completionTimeBuilder_ != null) {
return completionTimeBuilder_.getMessageOrBuilder();
} else {
return completionTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : completionTime_;
}
}
/**
*
* completion_time defines the unix time for redelegation completion.
*
*
* .google.protobuf.Timestamp completion_time = 2 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"completion_time\"", (.gogoproto.stdtime) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getCompletionTimeFieldBuilder() {
if (completionTimeBuilder_ == null) {
completionTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getCompletionTime(),
getParentForChildren(),
isClean());
completionTime_ = null;
}
return completionTimeBuilder_;
}
private java.lang.Object initialBalance_ = "";
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public java.lang.String getInitialBalance() {
java.lang.Object ref = initialBalance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
initialBalance_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public com.google.protobuf.ByteString
getInitialBalanceBytes() {
java.lang.Object ref = initialBalance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
initialBalance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public Builder setInitialBalance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
initialBalance_ = value;
onChanged();
return this;
}
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public Builder clearInitialBalance() {
initialBalance_ = getDefaultInstance().getInitialBalance();
onChanged();
return this;
}
/**
*
* initial_balance defines the initial balance when redelegation started.
*
*
* string initial_balance = 3 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.moretags) = "yaml:\"initial_balance\""];
*/
public Builder setInitialBalanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
initialBalance_ = value;
onChanged();
return this;
}
private java.lang.Object sharesDst_ = "";
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public java.lang.String getSharesDst() {
java.lang.Object ref = sharesDst_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sharesDst_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public com.google.protobuf.ByteString
getSharesDstBytes() {
java.lang.Object ref = sharesDst_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sharesDst_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setSharesDst(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sharesDst_ = value;
onChanged();
return this;
}
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder clearSharesDst() {
sharesDst_ = getDefaultInstance().getSharesDst();
onChanged();
return this;
}
/**
*
* shares_dst is the amount of destination-validator shares created by redelegation.
*
*
* string shares_dst = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Dec"];
*/
public Builder setSharesDstBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sharesDst_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.RedelegationEntry)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.RedelegationEntry)
private static final cosmos.staking.v1beta1.Staking.RedelegationEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.RedelegationEntry();
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RedelegationEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RedelegationEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RedelegationOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Redelegation)
com.google.protobuf.MessageOrBuilder {
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
java.lang.String getDelegatorAddress();
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
com.google.protobuf.ByteString
getDelegatorAddressBytes();
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
java.lang.String getValidatorSrcAddress();
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
com.google.protobuf.ByteString
getValidatorSrcAddressBytes();
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
java.lang.String getValidatorDstAddress();
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
com.google.protobuf.ByteString
getValidatorDstAddressBytes();
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
java.util.List
getEntriesList();
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationEntry getEntries(int index);
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
int getEntriesCount();
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
java.util.List extends cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>
getEntriesOrBuilderList();
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder getEntriesOrBuilder(
int index);
}
/**
*
* Redelegation contains the list of a particular delegator's redelegating bonds
* from a particular source validator to a particular destination validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Redelegation}
*/
public static final class Redelegation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Redelegation)
RedelegationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Redelegation.newBuilder() to construct.
private Redelegation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Redelegation() {
delegatorAddress_ = "";
validatorSrcAddress_ = "";
validatorDstAddress_ = "";
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Redelegation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
delegatorAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
validatorSrcAddress_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
validatorDstAddress_ = s;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
entries_.add(
input.readMessage(cosmos.staking.v1beta1.Staking.RedelegationEntry.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Redelegation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Redelegation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Redelegation.class, cosmos.staking.v1beta1.Staking.Redelegation.Builder.class);
}
private int bitField0_;
public static final int DELEGATOR_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object delegatorAddress_;
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_SRC_ADDRESS_FIELD_NUMBER = 2;
private volatile java.lang.Object validatorSrcAddress_;
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public java.lang.String getValidatorSrcAddress() {
java.lang.Object ref = validatorSrcAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorSrcAddress_ = s;
return s;
}
}
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public com.google.protobuf.ByteString
getValidatorSrcAddressBytes() {
java.lang.Object ref = validatorSrcAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorSrcAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATOR_DST_ADDRESS_FIELD_NUMBER = 3;
private volatile java.lang.Object validatorDstAddress_;
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public java.lang.String getValidatorDstAddress() {
java.lang.Object ref = validatorDstAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorDstAddress_ = s;
return s;
}
}
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public com.google.protobuf.ByteString
getValidatorDstAddressBytes() {
java.lang.Object ref = validatorDstAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorDstAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENTRIES_FIELD_NUMBER = 4;
private java.util.List entries_;
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public int getEntriesCount() {
return entries_.size();
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry getEntries(int index) {
return entries_.get(index);
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDelegatorAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, delegatorAddress_);
}
if (!getValidatorSrcAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, validatorSrcAddress_);
}
if (!getValidatorDstAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, validatorDstAddress_);
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(4, entries_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDelegatorAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, delegatorAddress_);
}
if (!getValidatorSrcAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, validatorSrcAddress_);
}
if (!getValidatorDstAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, validatorDstAddress_);
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, entries_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Redelegation)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Redelegation other = (cosmos.staking.v1beta1.Staking.Redelegation) obj;
boolean result = true;
result = result && getDelegatorAddress()
.equals(other.getDelegatorAddress());
result = result && getValidatorSrcAddress()
.equals(other.getValidatorSrcAddress());
result = result && getValidatorDstAddress()
.equals(other.getValidatorDstAddress());
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DELEGATOR_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getDelegatorAddress().hashCode();
hash = (37 * hash) + VALIDATOR_SRC_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorSrcAddress().hashCode();
hash = (37 * hash) + VALIDATOR_DST_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getValidatorDstAddress().hashCode();
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Redelegation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Redelegation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Redelegation contains the list of a particular delegator's redelegating bonds
* from a particular source validator to a particular destination validator.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Redelegation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Redelegation)
cosmos.staking.v1beta1.Staking.RedelegationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Redelegation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Redelegation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Redelegation.class, cosmos.staking.v1beta1.Staking.Redelegation.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Redelegation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
delegatorAddress_ = "";
validatorSrcAddress_ = "";
validatorDstAddress_ = "";
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
entriesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Redelegation_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Redelegation getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Redelegation.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Redelegation build() {
cosmos.staking.v1beta1.Staking.Redelegation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Redelegation buildPartial() {
cosmos.staking.v1beta1.Staking.Redelegation result = new cosmos.staking.v1beta1.Staking.Redelegation(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.delegatorAddress_ = delegatorAddress_;
result.validatorSrcAddress_ = validatorSrcAddress_;
result.validatorDstAddress_ = validatorDstAddress_;
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Redelegation) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Redelegation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Redelegation other) {
if (other == cosmos.staking.v1beta1.Staking.Redelegation.getDefaultInstance()) return this;
if (!other.getDelegatorAddress().isEmpty()) {
delegatorAddress_ = other.delegatorAddress_;
onChanged();
}
if (!other.getValidatorSrcAddress().isEmpty()) {
validatorSrcAddress_ = other.validatorSrcAddress_;
onChanged();
}
if (!other.getValidatorDstAddress().isEmpty()) {
validatorDstAddress_ = other.validatorDstAddress_;
onChanged();
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000008);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Redelegation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Redelegation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object delegatorAddress_ = "";
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public java.lang.String getDelegatorAddress() {
java.lang.Object ref = delegatorAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
delegatorAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public com.google.protobuf.ByteString
getDelegatorAddressBytes() {
java.lang.Object ref = delegatorAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
delegatorAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
delegatorAddress_ = value;
onChanged();
return this;
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder clearDelegatorAddress() {
delegatorAddress_ = getDefaultInstance().getDelegatorAddress();
onChanged();
return this;
}
/**
*
* delegator_address is the bech32-encoded address of the delegator.
*
*
* string delegator_address = 1 [(.gogoproto.moretags) = "yaml:\"delegator_address\""];
*/
public Builder setDelegatorAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
delegatorAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorSrcAddress_ = "";
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public java.lang.String getValidatorSrcAddress() {
java.lang.Object ref = validatorSrcAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorSrcAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public com.google.protobuf.ByteString
getValidatorSrcAddressBytes() {
java.lang.Object ref = validatorSrcAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorSrcAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public Builder setValidatorSrcAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorSrcAddress_ = value;
onChanged();
return this;
}
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public Builder clearValidatorSrcAddress() {
validatorSrcAddress_ = getDefaultInstance().getValidatorSrcAddress();
onChanged();
return this;
}
/**
*
* validator_src_address is the validator redelegation source operator address.
*
*
* string validator_src_address = 2 [(.gogoproto.moretags) = "yaml:\"validator_src_address\""];
*/
public Builder setValidatorSrcAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorSrcAddress_ = value;
onChanged();
return this;
}
private java.lang.Object validatorDstAddress_ = "";
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public java.lang.String getValidatorDstAddress() {
java.lang.Object ref = validatorDstAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
validatorDstAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public com.google.protobuf.ByteString
getValidatorDstAddressBytes() {
java.lang.Object ref = validatorDstAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
validatorDstAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public Builder setValidatorDstAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
validatorDstAddress_ = value;
onChanged();
return this;
}
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public Builder clearValidatorDstAddress() {
validatorDstAddress_ = getDefaultInstance().getValidatorDstAddress();
onChanged();
return this;
}
/**
*
* validator_dst_address is the validator redelegation destination operator address.
*
*
* string validator_dst_address = 3 [(.gogoproto.moretags) = "yaml:\"validator_dst_address\""];
*/
public Builder setValidatorDstAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
validatorDstAddress_ = value;
onChanged();
return this;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntry, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder> entriesBuilder_;
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder setEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder setEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(cosmos.staking.v1beta1.Staking.RedelegationEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntry value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder addAllEntries(
java.lang.Iterable extends cosmos.staking.v1beta1.Staking.RedelegationEntry> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance());
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance());
}
/**
*
* entries are the redelegation entries.
*
*
* repeated .cosmos.staking.v1beta1.RedelegationEntry entries = 4 [(.gogoproto.nullable) = false];
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntry, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntry, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>(
entries_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Redelegation)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Redelegation)
private static final cosmos.staking.v1beta1.Staking.Redelegation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Redelegation();
}
public static cosmos.staking.v1beta1.Staking.Redelegation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Redelegation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Redelegation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Redelegation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ParamsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Params)
com.google.protobuf.MessageOrBuilder {
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
boolean hasUnbondingTime();
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.Duration getUnbondingTime();
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
com.google.protobuf.DurationOrBuilder getUnbondingTimeOrBuilder();
/**
*
* max_validators is the maximum number of validators.
*
*
* uint32 max_validators = 2 [(.gogoproto.moretags) = "yaml:\"max_validators\""];
*/
int getMaxValidators();
/**
*
* max_entries is the max entries for either unbonding delegation or redelegation (per pair/trio).
*
*
* uint32 max_entries = 3 [(.gogoproto.moretags) = "yaml:\"max_entries\""];
*/
int getMaxEntries();
/**
*
* historical_entries is the number of historical entries to persist.
*
*
* uint32 historical_entries = 4 [(.gogoproto.moretags) = "yaml:\"historical_entries\""];
*/
int getHistoricalEntries();
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
java.lang.String getBondDenom();
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
com.google.protobuf.ByteString
getBondDenomBytes();
}
/**
*
* Params defines the parameters for the staking module.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Params}
*/
public static final class Params extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Params)
ParamsOrBuilder {
private static final long serialVersionUID = 0L;
// Use Params.newBuilder() to construct.
private Params(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Params() {
maxValidators_ = 0;
maxEntries_ = 0;
historicalEntries_ = 0;
bondDenom_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Params(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (unbondingTime_ != null) {
subBuilder = unbondingTime_.toBuilder();
}
unbondingTime_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(unbondingTime_);
unbondingTime_ = subBuilder.buildPartial();
}
break;
}
case 16: {
maxValidators_ = input.readUInt32();
break;
}
case 24: {
maxEntries_ = input.readUInt32();
break;
}
case 32: {
historicalEntries_ = input.readUInt32();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
bondDenom_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Params_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Params_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Params.class, cosmos.staking.v1beta1.Staking.Params.Builder.class);
}
public static final int UNBONDING_TIME_FIELD_NUMBER = 1;
private com.google.protobuf.Duration unbondingTime_;
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public boolean hasUnbondingTime() {
return unbondingTime_ != null;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getUnbondingTime() {
return unbondingTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : unbondingTime_;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getUnbondingTimeOrBuilder() {
return getUnbondingTime();
}
public static final int MAX_VALIDATORS_FIELD_NUMBER = 2;
private int maxValidators_;
/**
*
* max_validators is the maximum number of validators.
*
*
* uint32 max_validators = 2 [(.gogoproto.moretags) = "yaml:\"max_validators\""];
*/
public int getMaxValidators() {
return maxValidators_;
}
public static final int MAX_ENTRIES_FIELD_NUMBER = 3;
private int maxEntries_;
/**
*
* max_entries is the max entries for either unbonding delegation or redelegation (per pair/trio).
*
*
* uint32 max_entries = 3 [(.gogoproto.moretags) = "yaml:\"max_entries\""];
*/
public int getMaxEntries() {
return maxEntries_;
}
public static final int HISTORICAL_ENTRIES_FIELD_NUMBER = 4;
private int historicalEntries_;
/**
*
* historical_entries is the number of historical entries to persist.
*
*
* uint32 historical_entries = 4 [(.gogoproto.moretags) = "yaml:\"historical_entries\""];
*/
public int getHistoricalEntries() {
return historicalEntries_;
}
public static final int BOND_DENOM_FIELD_NUMBER = 5;
private volatile java.lang.Object bondDenom_;
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public java.lang.String getBondDenom() {
java.lang.Object ref = bondDenom_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bondDenom_ = s;
return s;
}
}
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public com.google.protobuf.ByteString
getBondDenomBytes() {
java.lang.Object ref = bondDenom_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bondDenom_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (unbondingTime_ != null) {
output.writeMessage(1, getUnbondingTime());
}
if (maxValidators_ != 0) {
output.writeUInt32(2, maxValidators_);
}
if (maxEntries_ != 0) {
output.writeUInt32(3, maxEntries_);
}
if (historicalEntries_ != 0) {
output.writeUInt32(4, historicalEntries_);
}
if (!getBondDenomBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, bondDenom_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (unbondingTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getUnbondingTime());
}
if (maxValidators_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, maxValidators_);
}
if (maxEntries_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, maxEntries_);
}
if (historicalEntries_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, historicalEntries_);
}
if (!getBondDenomBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, bondDenom_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Params)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Params other = (cosmos.staking.v1beta1.Staking.Params) obj;
boolean result = true;
result = result && (hasUnbondingTime() == other.hasUnbondingTime());
if (hasUnbondingTime()) {
result = result && getUnbondingTime()
.equals(other.getUnbondingTime());
}
result = result && (getMaxValidators()
== other.getMaxValidators());
result = result && (getMaxEntries()
== other.getMaxEntries());
result = result && (getHistoricalEntries()
== other.getHistoricalEntries());
result = result && getBondDenom()
.equals(other.getBondDenom());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUnbondingTime()) {
hash = (37 * hash) + UNBONDING_TIME_FIELD_NUMBER;
hash = (53 * hash) + getUnbondingTime().hashCode();
}
hash = (37 * hash) + MAX_VALIDATORS_FIELD_NUMBER;
hash = (53 * hash) + getMaxValidators();
hash = (37 * hash) + MAX_ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getMaxEntries();
hash = (37 * hash) + HISTORICAL_ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getHistoricalEntries();
hash = (37 * hash) + BOND_DENOM_FIELD_NUMBER;
hash = (53 * hash) + getBondDenom().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Params parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Params parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Params parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Params prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Params defines the parameters for the staking module.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Params}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Params)
cosmos.staking.v1beta1.Staking.ParamsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Params_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Params_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Params.class, cosmos.staking.v1beta1.Staking.Params.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Params.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (unbondingTimeBuilder_ == null) {
unbondingTime_ = null;
} else {
unbondingTime_ = null;
unbondingTimeBuilder_ = null;
}
maxValidators_ = 0;
maxEntries_ = 0;
historicalEntries_ = 0;
bondDenom_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Params_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Params getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Params.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Params build() {
cosmos.staking.v1beta1.Staking.Params result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Params buildPartial() {
cosmos.staking.v1beta1.Staking.Params result = new cosmos.staking.v1beta1.Staking.Params(this);
if (unbondingTimeBuilder_ == null) {
result.unbondingTime_ = unbondingTime_;
} else {
result.unbondingTime_ = unbondingTimeBuilder_.build();
}
result.maxValidators_ = maxValidators_;
result.maxEntries_ = maxEntries_;
result.historicalEntries_ = historicalEntries_;
result.bondDenom_ = bondDenom_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Params) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Params)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Params other) {
if (other == cosmos.staking.v1beta1.Staking.Params.getDefaultInstance()) return this;
if (other.hasUnbondingTime()) {
mergeUnbondingTime(other.getUnbondingTime());
}
if (other.getMaxValidators() != 0) {
setMaxValidators(other.getMaxValidators());
}
if (other.getMaxEntries() != 0) {
setMaxEntries(other.getMaxEntries());
}
if (other.getHistoricalEntries() != 0) {
setHistoricalEntries(other.getHistoricalEntries());
}
if (!other.getBondDenom().isEmpty()) {
bondDenom_ = other.bondDenom_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Params parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Params) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.Duration unbondingTime_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> unbondingTimeBuilder_;
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public boolean hasUnbondingTime() {
return unbondingTimeBuilder_ != null || unbondingTime_ != null;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration getUnbondingTime() {
if (unbondingTimeBuilder_ == null) {
return unbondingTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : unbondingTime_;
} else {
return unbondingTimeBuilder_.getMessage();
}
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public Builder setUnbondingTime(com.google.protobuf.Duration value) {
if (unbondingTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
unbondingTime_ = value;
onChanged();
} else {
unbondingTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public Builder setUnbondingTime(
com.google.protobuf.Duration.Builder builderForValue) {
if (unbondingTimeBuilder_ == null) {
unbondingTime_ = builderForValue.build();
onChanged();
} else {
unbondingTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public Builder mergeUnbondingTime(com.google.protobuf.Duration value) {
if (unbondingTimeBuilder_ == null) {
if (unbondingTime_ != null) {
unbondingTime_ =
com.google.protobuf.Duration.newBuilder(unbondingTime_).mergeFrom(value).buildPartial();
} else {
unbondingTime_ = value;
}
onChanged();
} else {
unbondingTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public Builder clearUnbondingTime() {
if (unbondingTimeBuilder_ == null) {
unbondingTime_ = null;
onChanged();
} else {
unbondingTime_ = null;
unbondingTimeBuilder_ = null;
}
return this;
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.Duration.Builder getUnbondingTimeBuilder() {
onChanged();
return getUnbondingTimeFieldBuilder().getBuilder();
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
public com.google.protobuf.DurationOrBuilder getUnbondingTimeOrBuilder() {
if (unbondingTimeBuilder_ != null) {
return unbondingTimeBuilder_.getMessageOrBuilder();
} else {
return unbondingTime_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : unbondingTime_;
}
}
/**
*
* unbonding_time is the time duration of unbonding.
*
*
* .google.protobuf.Duration unbonding_time = 1 [(.gogoproto.nullable) = false, (.gogoproto.moretags) = "yaml:\"unbonding_time\"", (.gogoproto.stdduration) = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getUnbondingTimeFieldBuilder() {
if (unbondingTimeBuilder_ == null) {
unbondingTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getUnbondingTime(),
getParentForChildren(),
isClean());
unbondingTime_ = null;
}
return unbondingTimeBuilder_;
}
private int maxValidators_ ;
/**
*
* max_validators is the maximum number of validators.
*
*
* uint32 max_validators = 2 [(.gogoproto.moretags) = "yaml:\"max_validators\""];
*/
public int getMaxValidators() {
return maxValidators_;
}
/**
*
* max_validators is the maximum number of validators.
*
*
* uint32 max_validators = 2 [(.gogoproto.moretags) = "yaml:\"max_validators\""];
*/
public Builder setMaxValidators(int value) {
maxValidators_ = value;
onChanged();
return this;
}
/**
*
* max_validators is the maximum number of validators.
*
*
* uint32 max_validators = 2 [(.gogoproto.moretags) = "yaml:\"max_validators\""];
*/
public Builder clearMaxValidators() {
maxValidators_ = 0;
onChanged();
return this;
}
private int maxEntries_ ;
/**
*
* max_entries is the max entries for either unbonding delegation or redelegation (per pair/trio).
*
*
* uint32 max_entries = 3 [(.gogoproto.moretags) = "yaml:\"max_entries\""];
*/
public int getMaxEntries() {
return maxEntries_;
}
/**
*
* max_entries is the max entries for either unbonding delegation or redelegation (per pair/trio).
*
*
* uint32 max_entries = 3 [(.gogoproto.moretags) = "yaml:\"max_entries\""];
*/
public Builder setMaxEntries(int value) {
maxEntries_ = value;
onChanged();
return this;
}
/**
*
* max_entries is the max entries for either unbonding delegation or redelegation (per pair/trio).
*
*
* uint32 max_entries = 3 [(.gogoproto.moretags) = "yaml:\"max_entries\""];
*/
public Builder clearMaxEntries() {
maxEntries_ = 0;
onChanged();
return this;
}
private int historicalEntries_ ;
/**
*
* historical_entries is the number of historical entries to persist.
*
*
* uint32 historical_entries = 4 [(.gogoproto.moretags) = "yaml:\"historical_entries\""];
*/
public int getHistoricalEntries() {
return historicalEntries_;
}
/**
*
* historical_entries is the number of historical entries to persist.
*
*
* uint32 historical_entries = 4 [(.gogoproto.moretags) = "yaml:\"historical_entries\""];
*/
public Builder setHistoricalEntries(int value) {
historicalEntries_ = value;
onChanged();
return this;
}
/**
*
* historical_entries is the number of historical entries to persist.
*
*
* uint32 historical_entries = 4 [(.gogoproto.moretags) = "yaml:\"historical_entries\""];
*/
public Builder clearHistoricalEntries() {
historicalEntries_ = 0;
onChanged();
return this;
}
private java.lang.Object bondDenom_ = "";
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public java.lang.String getBondDenom() {
java.lang.Object ref = bondDenom_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bondDenom_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public com.google.protobuf.ByteString
getBondDenomBytes() {
java.lang.Object ref = bondDenom_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bondDenom_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public Builder setBondDenom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bondDenom_ = value;
onChanged();
return this;
}
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public Builder clearBondDenom() {
bondDenom_ = getDefaultInstance().getBondDenom();
onChanged();
return this;
}
/**
*
* bond_denom defines the bondable coin denomination.
*
*
* string bond_denom = 5 [(.gogoproto.moretags) = "yaml:\"bond_denom\""];
*/
public Builder setBondDenomBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bondDenom_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Params)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Params)
private static final cosmos.staking.v1beta1.Staking.Params DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Params();
}
public static cosmos.staking.v1beta1.Staking.Params getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Params parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Params(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Params getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DelegationResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.DelegationResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
boolean hasDelegation();
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.Delegation getDelegation();
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.DelegationOrBuilder getDelegationOrBuilder();
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
boolean hasBalance();
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getBalance();
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getBalanceOrBuilder();
}
/**
*
* DelegationResponse is equivalent to Delegation except that it contains a
* balance in addition to shares which is more suitable for client responses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DelegationResponse}
*/
public static final class DelegationResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.DelegationResponse)
DelegationResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use DelegationResponse.newBuilder() to construct.
private DelegationResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DelegationResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DelegationResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cosmos.staking.v1beta1.Staking.Delegation.Builder subBuilder = null;
if (delegation_ != null) {
subBuilder = delegation_.toBuilder();
}
delegation_ = input.readMessage(cosmos.staking.v1beta1.Staking.Delegation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(delegation_);
delegation_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder subBuilder = null;
if (balance_ != null) {
subBuilder = balance_.toBuilder();
}
balance_ = input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(balance_);
balance_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DelegationResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DelegationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DelegationResponse.class, cosmos.staking.v1beta1.Staking.DelegationResponse.Builder.class);
}
public static final int DELEGATION_FIELD_NUMBER = 1;
private cosmos.staking.v1beta1.Staking.Delegation delegation_;
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasDelegation() {
return delegation_ != null;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Delegation getDelegation() {
return delegation_ == null ? cosmos.staking.v1beta1.Staking.Delegation.getDefaultInstance() : delegation_;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DelegationOrBuilder getDelegationOrBuilder() {
return getDelegation();
}
public static final int BALANCE_FIELD_NUMBER = 2;
private cosmos.base.v1beta1.CoinOuterClass.Coin balance_;
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasBalance() {
return balance_ != null;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getBalance() {
return balance_ == null ? cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance() : balance_;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getBalanceOrBuilder() {
return getBalance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (delegation_ != null) {
output.writeMessage(1, getDelegation());
}
if (balance_ != null) {
output.writeMessage(2, getBalance());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (delegation_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getDelegation());
}
if (balance_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getBalance());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.DelegationResponse)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.DelegationResponse other = (cosmos.staking.v1beta1.Staking.DelegationResponse) obj;
boolean result = true;
result = result && (hasDelegation() == other.hasDelegation());
if (hasDelegation()) {
result = result && getDelegation()
.equals(other.getDelegation());
}
result = result && (hasBalance() == other.hasBalance());
if (hasBalance()) {
result = result && getBalance()
.equals(other.getBalance());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDelegation()) {
hash = (37 * hash) + DELEGATION_FIELD_NUMBER;
hash = (53 * hash) + getDelegation().hashCode();
}
if (hasBalance()) {
hash = (37 * hash) + BALANCE_FIELD_NUMBER;
hash = (53 * hash) + getBalance().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.DelegationResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* DelegationResponse is equivalent to Delegation except that it contains a
* balance in addition to shares which is more suitable for client responses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.DelegationResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.DelegationResponse)
cosmos.staking.v1beta1.Staking.DelegationResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DelegationResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DelegationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.DelegationResponse.class, cosmos.staking.v1beta1.Staking.DelegationResponse.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.DelegationResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (delegationBuilder_ == null) {
delegation_ = null;
} else {
delegation_ = null;
delegationBuilder_ = null;
}
if (balanceBuilder_ == null) {
balance_ = null;
} else {
balance_ = null;
balanceBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_DelegationResponse_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DelegationResponse getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.DelegationResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DelegationResponse build() {
cosmos.staking.v1beta1.Staking.DelegationResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DelegationResponse buildPartial() {
cosmos.staking.v1beta1.Staking.DelegationResponse result = new cosmos.staking.v1beta1.Staking.DelegationResponse(this);
if (delegationBuilder_ == null) {
result.delegation_ = delegation_;
} else {
result.delegation_ = delegationBuilder_.build();
}
if (balanceBuilder_ == null) {
result.balance_ = balance_;
} else {
result.balance_ = balanceBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.DelegationResponse) {
return mergeFrom((cosmos.staking.v1beta1.Staking.DelegationResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.DelegationResponse other) {
if (other == cosmos.staking.v1beta1.Staking.DelegationResponse.getDefaultInstance()) return this;
if (other.hasDelegation()) {
mergeDelegation(other.getDelegation());
}
if (other.hasBalance()) {
mergeBalance(other.getBalance());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.DelegationResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.DelegationResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cosmos.staking.v1beta1.Staking.Delegation delegation_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Delegation, cosmos.staking.v1beta1.Staking.Delegation.Builder, cosmos.staking.v1beta1.Staking.DelegationOrBuilder> delegationBuilder_;
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasDelegation() {
return delegationBuilder_ != null || delegation_ != null;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Delegation getDelegation() {
if (delegationBuilder_ == null) {
return delegation_ == null ? cosmos.staking.v1beta1.Staking.Delegation.getDefaultInstance() : delegation_;
} else {
return delegationBuilder_.getMessage();
}
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder setDelegation(cosmos.staking.v1beta1.Staking.Delegation value) {
if (delegationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
delegation_ = value;
onChanged();
} else {
delegationBuilder_.setMessage(value);
}
return this;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder setDelegation(
cosmos.staking.v1beta1.Staking.Delegation.Builder builderForValue) {
if (delegationBuilder_ == null) {
delegation_ = builderForValue.build();
onChanged();
} else {
delegationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder mergeDelegation(cosmos.staking.v1beta1.Staking.Delegation value) {
if (delegationBuilder_ == null) {
if (delegation_ != null) {
delegation_ =
cosmos.staking.v1beta1.Staking.Delegation.newBuilder(delegation_).mergeFrom(value).buildPartial();
} else {
delegation_ = value;
}
onChanged();
} else {
delegationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearDelegation() {
if (delegationBuilder_ == null) {
delegation_ = null;
onChanged();
} else {
delegation_ = null;
delegationBuilder_ = null;
}
return this;
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Delegation.Builder getDelegationBuilder() {
onChanged();
return getDelegationFieldBuilder().getBuilder();
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.DelegationOrBuilder getDelegationOrBuilder() {
if (delegationBuilder_ != null) {
return delegationBuilder_.getMessageOrBuilder();
} else {
return delegation_ == null ?
cosmos.staking.v1beta1.Staking.Delegation.getDefaultInstance() : delegation_;
}
}
/**
* .cosmos.staking.v1beta1.Delegation delegation = 1 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Delegation, cosmos.staking.v1beta1.Staking.Delegation.Builder, cosmos.staking.v1beta1.Staking.DelegationOrBuilder>
getDelegationFieldBuilder() {
if (delegationBuilder_ == null) {
delegationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Delegation, cosmos.staking.v1beta1.Staking.Delegation.Builder, cosmos.staking.v1beta1.Staking.DelegationOrBuilder>(
getDelegation(),
getParentForChildren(),
isClean());
delegation_ = null;
}
return delegationBuilder_;
}
private cosmos.base.v1beta1.CoinOuterClass.Coin balance_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> balanceBuilder_;
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasBalance() {
return balanceBuilder_ != null || balance_ != null;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getBalance() {
if (balanceBuilder_ == null) {
return balance_ == null ? cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance() : balance_;
} else {
return balanceBuilder_.getMessage();
}
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public Builder setBalance(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (balanceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
balance_ = value;
onChanged();
} else {
balanceBuilder_.setMessage(value);
}
return this;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public Builder setBalance(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (balanceBuilder_ == null) {
balance_ = builderForValue.build();
onChanged();
} else {
balanceBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public Builder mergeBalance(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (balanceBuilder_ == null) {
if (balance_ != null) {
balance_ =
cosmos.base.v1beta1.CoinOuterClass.Coin.newBuilder(balance_).mergeFrom(value).buildPartial();
} else {
balance_ = value;
}
onChanged();
} else {
balanceBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearBalance() {
if (balanceBuilder_ == null) {
balance_ = null;
onChanged();
} else {
balance_ = null;
balanceBuilder_ = null;
}
return this;
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getBalanceBuilder() {
onChanged();
return getBalanceFieldBuilder().getBuilder();
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getBalanceOrBuilder() {
if (balanceBuilder_ != null) {
return balanceBuilder_.getMessageOrBuilder();
} else {
return balance_ == null ?
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance() : balance_;
}
}
/**
* .cosmos.base.v1beta1.Coin balance = 2 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getBalanceFieldBuilder() {
if (balanceBuilder_ == null) {
balanceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
getBalance(),
getParentForChildren(),
isClean());
balance_ = null;
}
return balanceBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.DelegationResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.DelegationResponse)
private static final cosmos.staking.v1beta1.Staking.DelegationResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.DelegationResponse();
}
public static cosmos.staking.v1beta1.Staking.DelegationResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DelegationResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DelegationResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.DelegationResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RedelegationEntryResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.RedelegationEntryResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
boolean hasRedelegationEntry();
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationEntry getRedelegationEntry();
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder getRedelegationEntryOrBuilder();
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
java.lang.String getBalance();
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
com.google.protobuf.ByteString
getBalanceBytes();
}
/**
*
* RedelegationEntryResponse is equivalent to a RedelegationEntry except that it
* contains a balance in addition to shares which is more suitable for client
* responses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.RedelegationEntryResponse}
*/
public static final class RedelegationEntryResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.RedelegationEntryResponse)
RedelegationEntryResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RedelegationEntryResponse.newBuilder() to construct.
private RedelegationEntryResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RedelegationEntryResponse() {
balance_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RedelegationEntryResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder subBuilder = null;
if (redelegationEntry_ != null) {
subBuilder = redelegationEntry_.toBuilder();
}
redelegationEntry_ = input.readMessage(cosmos.staking.v1beta1.Staking.RedelegationEntry.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(redelegationEntry_);
redelegationEntry_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
balance_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.class, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder.class);
}
public static final int REDELEGATION_ENTRY_FIELD_NUMBER = 1;
private cosmos.staking.v1beta1.Staking.RedelegationEntry redelegationEntry_;
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasRedelegationEntry() {
return redelegationEntry_ != null;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry getRedelegationEntry() {
return redelegationEntry_ == null ? cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance() : redelegationEntry_;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder getRedelegationEntryOrBuilder() {
return getRedelegationEntry();
}
public static final int BALANCE_FIELD_NUMBER = 4;
private volatile java.lang.Object balance_;
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getBalance() {
java.lang.Object ref = balance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
balance_ = s;
return s;
}
}
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getBalanceBytes() {
java.lang.Object ref = balance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
balance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (redelegationEntry_ != null) {
output.writeMessage(1, getRedelegationEntry());
}
if (!getBalanceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, balance_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (redelegationEntry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRedelegationEntry());
}
if (!getBalanceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, balance_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.RedelegationEntryResponse)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse other = (cosmos.staking.v1beta1.Staking.RedelegationEntryResponse) obj;
boolean result = true;
result = result && (hasRedelegationEntry() == other.hasRedelegationEntry());
if (hasRedelegationEntry()) {
result = result && getRedelegationEntry()
.equals(other.getRedelegationEntry());
}
result = result && getBalance()
.equals(other.getBalance());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRedelegationEntry()) {
hash = (37 * hash) + REDELEGATION_ENTRY_FIELD_NUMBER;
hash = (53 * hash) + getRedelegationEntry().hashCode();
}
hash = (37 * hash) + BALANCE_FIELD_NUMBER;
hash = (53 * hash) + getBalance().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.RedelegationEntryResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* RedelegationEntryResponse is equivalent to a RedelegationEntry except that it
* contains a balance in addition to shares which is more suitable for client
* responses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.RedelegationEntryResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.RedelegationEntryResponse)
cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.class, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (redelegationEntryBuilder_ == null) {
redelegationEntry_ = null;
} else {
redelegationEntry_ = null;
redelegationEntryBuilder_ = null;
}
balance_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse build() {
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse buildPartial() {
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse result = new cosmos.staking.v1beta1.Staking.RedelegationEntryResponse(this);
if (redelegationEntryBuilder_ == null) {
result.redelegationEntry_ = redelegationEntry_;
} else {
result.redelegationEntry_ = redelegationEntryBuilder_.build();
}
result.balance_ = balance_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.RedelegationEntryResponse) {
return mergeFrom((cosmos.staking.v1beta1.Staking.RedelegationEntryResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.RedelegationEntryResponse other) {
if (other == cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.getDefaultInstance()) return this;
if (other.hasRedelegationEntry()) {
mergeRedelegationEntry(other.getRedelegationEntry());
}
if (!other.getBalance().isEmpty()) {
balance_ = other.balance_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.RedelegationEntryResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cosmos.staking.v1beta1.Staking.RedelegationEntry redelegationEntry_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntry, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder> redelegationEntryBuilder_;
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasRedelegationEntry() {
return redelegationEntryBuilder_ != null || redelegationEntry_ != null;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry getRedelegationEntry() {
if (redelegationEntryBuilder_ == null) {
return redelegationEntry_ == null ? cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance() : redelegationEntry_;
} else {
return redelegationEntryBuilder_.getMessage();
}
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public Builder setRedelegationEntry(cosmos.staking.v1beta1.Staking.RedelegationEntry value) {
if (redelegationEntryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
redelegationEntry_ = value;
onChanged();
} else {
redelegationEntryBuilder_.setMessage(value);
}
return this;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public Builder setRedelegationEntry(
cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder builderForValue) {
if (redelegationEntryBuilder_ == null) {
redelegationEntry_ = builderForValue.build();
onChanged();
} else {
redelegationEntryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public Builder mergeRedelegationEntry(cosmos.staking.v1beta1.Staking.RedelegationEntry value) {
if (redelegationEntryBuilder_ == null) {
if (redelegationEntry_ != null) {
redelegationEntry_ =
cosmos.staking.v1beta1.Staking.RedelegationEntry.newBuilder(redelegationEntry_).mergeFrom(value).buildPartial();
} else {
redelegationEntry_ = value;
}
onChanged();
} else {
redelegationEntryBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearRedelegationEntry() {
if (redelegationEntryBuilder_ == null) {
redelegationEntry_ = null;
onChanged();
} else {
redelegationEntry_ = null;
redelegationEntryBuilder_ = null;
}
return this;
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder getRedelegationEntryBuilder() {
onChanged();
return getRedelegationEntryFieldBuilder().getBuilder();
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder getRedelegationEntryOrBuilder() {
if (redelegationEntryBuilder_ != null) {
return redelegationEntryBuilder_.getMessageOrBuilder();
} else {
return redelegationEntry_ == null ?
cosmos.staking.v1beta1.Staking.RedelegationEntry.getDefaultInstance() : redelegationEntry_;
}
}
/**
* .cosmos.staking.v1beta1.RedelegationEntry redelegation_entry = 1 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntry, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>
getRedelegationEntryFieldBuilder() {
if (redelegationEntryBuilder_ == null) {
redelegationEntryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntry, cosmos.staking.v1beta1.Staking.RedelegationEntry.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryOrBuilder>(
getRedelegationEntry(),
getParentForChildren(),
isClean());
redelegationEntry_ = null;
}
return redelegationEntryBuilder_;
}
private java.lang.Object balance_ = "";
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public java.lang.String getBalance() {
java.lang.Object ref = balance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
balance_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public com.google.protobuf.ByteString
getBalanceBytes() {
java.lang.Object ref = balance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
balance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setBalance(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
balance_ = value;
onChanged();
return this;
}
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder clearBalance() {
balance_ = getDefaultInstance().getBalance();
onChanged();
return this;
}
/**
* string balance = 4 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int"];
*/
public Builder setBalanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
balance_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.RedelegationEntryResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.RedelegationEntryResponse)
private static final cosmos.staking.v1beta1.Staking.RedelegationEntryResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.RedelegationEntryResponse();
}
public static cosmos.staking.v1beta1.Staking.RedelegationEntryResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RedelegationEntryResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RedelegationEntryResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RedelegationResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.RedelegationResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
boolean hasRedelegation();
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.Redelegation getRedelegation();
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationOrBuilder getRedelegationOrBuilder();
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
java.util.List
getEntriesList();
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse getEntries(int index);
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
int getEntriesCount();
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
java.util.List extends cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder>
getEntriesOrBuilderList();
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder getEntriesOrBuilder(
int index);
}
/**
*
* RedelegationResponse is equivalent to a Redelegation except that its entries
* contain a balance in addition to shares which is more suitable for client
* responses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.RedelegationResponse}
*/
public static final class RedelegationResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.RedelegationResponse)
RedelegationResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RedelegationResponse.newBuilder() to construct.
private RedelegationResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RedelegationResponse() {
entries_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RedelegationResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cosmos.staking.v1beta1.Staking.Redelegation.Builder subBuilder = null;
if (redelegation_ != null) {
subBuilder = redelegation_.toBuilder();
}
redelegation_ = input.readMessage(cosmos.staking.v1beta1.Staking.Redelegation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(redelegation_);
redelegation_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
entries_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
entries_.add(
input.readMessage(cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.RedelegationResponse.class, cosmos.staking.v1beta1.Staking.RedelegationResponse.Builder.class);
}
private int bitField0_;
public static final int REDELEGATION_FIELD_NUMBER = 1;
private cosmos.staking.v1beta1.Staking.Redelegation redelegation_;
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasRedelegation() {
return redelegation_ != null;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Redelegation getRedelegation() {
return redelegation_ == null ? cosmos.staking.v1beta1.Staking.Redelegation.getDefaultInstance() : redelegation_;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationOrBuilder getRedelegationOrBuilder() {
return getRedelegation();
}
public static final int ENTRIES_FIELD_NUMBER = 2;
private java.util.List entries_;
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List getEntriesList() {
return entries_;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder>
getEntriesOrBuilderList() {
return entries_;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public int getEntriesCount() {
return entries_.size();
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse getEntries(int index) {
return entries_.get(index);
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder getEntriesOrBuilder(
int index) {
return entries_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (redelegation_ != null) {
output.writeMessage(1, getRedelegation());
}
for (int i = 0; i < entries_.size(); i++) {
output.writeMessage(2, entries_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (redelegation_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRedelegation());
}
for (int i = 0; i < entries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, entries_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.RedelegationResponse)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.RedelegationResponse other = (cosmos.staking.v1beta1.Staking.RedelegationResponse) obj;
boolean result = true;
result = result && (hasRedelegation() == other.hasRedelegation());
if (hasRedelegation()) {
result = result && getRedelegation()
.equals(other.getRedelegation());
}
result = result && getEntriesList()
.equals(other.getEntriesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRedelegation()) {
hash = (37 * hash) + REDELEGATION_FIELD_NUMBER;
hash = (53 * hash) + getRedelegation().hashCode();
}
if (getEntriesCount() > 0) {
hash = (37 * hash) + ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getEntriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.RedelegationResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* RedelegationResponse is equivalent to a Redelegation except that its entries
* contain a balance in addition to shares which is more suitable for client
* responses.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.RedelegationResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.RedelegationResponse)
cosmos.staking.v1beta1.Staking.RedelegationResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.RedelegationResponse.class, cosmos.staking.v1beta1.Staking.RedelegationResponse.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.RedelegationResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEntriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (redelegationBuilder_ == null) {
redelegation_ = null;
} else {
redelegation_ = null;
redelegationBuilder_ = null;
}
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
entriesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_RedelegationResponse_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationResponse getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.RedelegationResponse.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationResponse build() {
cosmos.staking.v1beta1.Staking.RedelegationResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationResponse buildPartial() {
cosmos.staking.v1beta1.Staking.RedelegationResponse result = new cosmos.staking.v1beta1.Staking.RedelegationResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (redelegationBuilder_ == null) {
result.redelegation_ = redelegation_;
} else {
result.redelegation_ = redelegationBuilder_.build();
}
if (entriesBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
entries_ = java.util.Collections.unmodifiableList(entries_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.entries_ = entries_;
} else {
result.entries_ = entriesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.RedelegationResponse) {
return mergeFrom((cosmos.staking.v1beta1.Staking.RedelegationResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.RedelegationResponse other) {
if (other == cosmos.staking.v1beta1.Staking.RedelegationResponse.getDefaultInstance()) return this;
if (other.hasRedelegation()) {
mergeRedelegation(other.getRedelegation());
}
if (entriesBuilder_ == null) {
if (!other.entries_.isEmpty()) {
if (entries_.isEmpty()) {
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureEntriesIsMutable();
entries_.addAll(other.entries_);
}
onChanged();
}
} else {
if (!other.entries_.isEmpty()) {
if (entriesBuilder_.isEmpty()) {
entriesBuilder_.dispose();
entriesBuilder_ = null;
entries_ = other.entries_;
bitField0_ = (bitField0_ & ~0x00000002);
entriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEntriesFieldBuilder() : null;
} else {
entriesBuilder_.addAllMessages(other.entries_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.RedelegationResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.RedelegationResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cosmos.staking.v1beta1.Staking.Redelegation redelegation_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Redelegation, cosmos.staking.v1beta1.Staking.Redelegation.Builder, cosmos.staking.v1beta1.Staking.RedelegationOrBuilder> redelegationBuilder_;
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public boolean hasRedelegation() {
return redelegationBuilder_ != null || redelegation_ != null;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Redelegation getRedelegation() {
if (redelegationBuilder_ == null) {
return redelegation_ == null ? cosmos.staking.v1beta1.Staking.Redelegation.getDefaultInstance() : redelegation_;
} else {
return redelegationBuilder_.getMessage();
}
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder setRedelegation(cosmos.staking.v1beta1.Staking.Redelegation value) {
if (redelegationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
redelegation_ = value;
onChanged();
} else {
redelegationBuilder_.setMessage(value);
}
return this;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder setRedelegation(
cosmos.staking.v1beta1.Staking.Redelegation.Builder builderForValue) {
if (redelegationBuilder_ == null) {
redelegation_ = builderForValue.build();
onChanged();
} else {
redelegationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder mergeRedelegation(cosmos.staking.v1beta1.Staking.Redelegation value) {
if (redelegationBuilder_ == null) {
if (redelegation_ != null) {
redelegation_ =
cosmos.staking.v1beta1.Staking.Redelegation.newBuilder(redelegation_).mergeFrom(value).buildPartial();
} else {
redelegation_ = value;
}
onChanged();
} else {
redelegationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public Builder clearRedelegation() {
if (redelegationBuilder_ == null) {
redelegation_ = null;
onChanged();
} else {
redelegation_ = null;
redelegationBuilder_ = null;
}
return this;
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.Redelegation.Builder getRedelegationBuilder() {
onChanged();
return getRedelegationFieldBuilder().getBuilder();
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationOrBuilder getRedelegationOrBuilder() {
if (redelegationBuilder_ != null) {
return redelegationBuilder_.getMessageOrBuilder();
} else {
return redelegation_ == null ?
cosmos.staking.v1beta1.Staking.Redelegation.getDefaultInstance() : redelegation_;
}
}
/**
* .cosmos.staking.v1beta1.Redelegation redelegation = 1 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Redelegation, cosmos.staking.v1beta1.Staking.Redelegation.Builder, cosmos.staking.v1beta1.Staking.RedelegationOrBuilder>
getRedelegationFieldBuilder() {
if (redelegationBuilder_ == null) {
redelegationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.staking.v1beta1.Staking.Redelegation, cosmos.staking.v1beta1.Staking.Redelegation.Builder, cosmos.staking.v1beta1.Staking.RedelegationOrBuilder>(
getRedelegation(),
getParentForChildren(),
isClean());
redelegation_ = null;
}
return redelegationBuilder_;
}
private java.util.List entries_ =
java.util.Collections.emptyList();
private void ensureEntriesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
entries_ = new java.util.ArrayList(entries_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder> entriesBuilder_;
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List getEntriesList() {
if (entriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(entries_);
} else {
return entriesBuilder_.getMessageList();
}
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public int getEntriesCount() {
if (entriesBuilder_ == null) {
return entries_.size();
} else {
return entriesBuilder_.getCount();
}
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse getEntries(int index) {
if (entriesBuilder_ == null) {
return entries_.get(index);
} else {
return entriesBuilder_.getMessage(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder setEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.set(index, value);
onChanged();
} else {
entriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder setEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.set(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(cosmos.staking.v1beta1.Staking.RedelegationEntryResponse value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(value);
onChanged();
} else {
entriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse value) {
if (entriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEntriesIsMutable();
entries_.add(index, value);
onChanged();
} else {
entriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder addEntries(
int index, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder builderForValue) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.add(index, builderForValue.build());
onChanged();
} else {
entriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder addAllEntries(
java.lang.Iterable extends cosmos.staking.v1beta1.Staking.RedelegationEntryResponse> values) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, entries_);
onChanged();
} else {
entriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearEntries() {
if (entriesBuilder_ == null) {
entries_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
entriesBuilder_.clear();
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public Builder removeEntries(int index) {
if (entriesBuilder_ == null) {
ensureEntriesIsMutable();
entries_.remove(index);
onChanged();
} else {
entriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder getEntriesBuilder(
int index) {
return getEntriesFieldBuilder().getBuilder(index);
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder getEntriesOrBuilder(
int index) {
if (entriesBuilder_ == null) {
return entries_.get(index); } else {
return entriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List extends cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder>
getEntriesOrBuilderList() {
if (entriesBuilder_ != null) {
return entriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(entries_);
}
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder addEntriesBuilder() {
return getEntriesFieldBuilder().addBuilder(
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder addEntriesBuilder(
int index) {
return getEntriesFieldBuilder().addBuilder(
index, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.getDefaultInstance());
}
/**
* repeated .cosmos.staking.v1beta1.RedelegationEntryResponse entries = 2 [(.gogoproto.nullable) = false];
*/
public java.util.List
getEntriesBuilderList() {
return getEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder>
getEntriesFieldBuilder() {
if (entriesBuilder_ == null) {
entriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.staking.v1beta1.Staking.RedelegationEntryResponse, cosmos.staking.v1beta1.Staking.RedelegationEntryResponse.Builder, cosmos.staking.v1beta1.Staking.RedelegationEntryResponseOrBuilder>(
entries_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
entries_ = null;
}
return entriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.RedelegationResponse)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.RedelegationResponse)
private static final cosmos.staking.v1beta1.Staking.RedelegationResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.RedelegationResponse();
}
public static cosmos.staking.v1beta1.Staking.RedelegationResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RedelegationResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RedelegationResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.RedelegationResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PoolOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmos.staking.v1beta1.Pool)
com.google.protobuf.MessageOrBuilder {
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
java.lang.String getNotBondedTokens();
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
com.google.protobuf.ByteString
getNotBondedTokensBytes();
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
java.lang.String getBondedTokens();
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
com.google.protobuf.ByteString
getBondedTokensBytes();
}
/**
*
* Pool is used for tracking bonded and not-bonded token supply of the bond
* denomination.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Pool}
*/
public static final class Pool extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmos.staking.v1beta1.Pool)
PoolOrBuilder {
private static final long serialVersionUID = 0L;
// Use Pool.newBuilder() to construct.
private Pool(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Pool() {
notBondedTokens_ = "";
bondedTokens_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Pool(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
notBondedTokens_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
bondedTokens_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Pool_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Pool_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Pool.class, cosmos.staking.v1beta1.Staking.Pool.Builder.class);
}
public static final int NOT_BONDED_TOKENS_FIELD_NUMBER = 1;
private volatile java.lang.Object notBondedTokens_;
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public java.lang.String getNotBondedTokens() {
java.lang.Object ref = notBondedTokens_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notBondedTokens_ = s;
return s;
}
}
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public com.google.protobuf.ByteString
getNotBondedTokensBytes() {
java.lang.Object ref = notBondedTokens_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notBondedTokens_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BONDED_TOKENS_FIELD_NUMBER = 2;
private volatile java.lang.Object bondedTokens_;
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public java.lang.String getBondedTokens() {
java.lang.Object ref = bondedTokens_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bondedTokens_ = s;
return s;
}
}
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public com.google.protobuf.ByteString
getBondedTokensBytes() {
java.lang.Object ref = bondedTokens_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bondedTokens_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNotBondedTokensBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, notBondedTokens_);
}
if (!getBondedTokensBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, bondedTokens_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNotBondedTokensBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, notBondedTokens_);
}
if (!getBondedTokensBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, bondedTokens_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmos.staking.v1beta1.Staking.Pool)) {
return super.equals(obj);
}
cosmos.staking.v1beta1.Staking.Pool other = (cosmos.staking.v1beta1.Staking.Pool) obj;
boolean result = true;
result = result && getNotBondedTokens()
.equals(other.getNotBondedTokens());
result = result && getBondedTokens()
.equals(other.getBondedTokens());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NOT_BONDED_TOKENS_FIELD_NUMBER;
hash = (53 * hash) + getNotBondedTokens().hashCode();
hash = (37 * hash) + BONDED_TOKENS_FIELD_NUMBER;
hash = (53 * hash) + getBondedTokens().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Pool parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Pool parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmos.staking.v1beta1.Staking.Pool parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmos.staking.v1beta1.Staking.Pool prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Pool is used for tracking bonded and not-bonded token supply of the bond
* denomination.
*
*
* Protobuf type {@code cosmos.staking.v1beta1.Pool}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmos.staking.v1beta1.Pool)
cosmos.staking.v1beta1.Staking.PoolOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Pool_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Pool_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmos.staking.v1beta1.Staking.Pool.class, cosmos.staking.v1beta1.Staking.Pool.Builder.class);
}
// Construct using cosmos.staking.v1beta1.Staking.Pool.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
notBondedTokens_ = "";
bondedTokens_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmos.staking.v1beta1.Staking.internal_static_cosmos_staking_v1beta1_Pool_descriptor;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Pool getDefaultInstanceForType() {
return cosmos.staking.v1beta1.Staking.Pool.getDefaultInstance();
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Pool build() {
cosmos.staking.v1beta1.Staking.Pool result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Pool buildPartial() {
cosmos.staking.v1beta1.Staking.Pool result = new cosmos.staking.v1beta1.Staking.Pool(this);
result.notBondedTokens_ = notBondedTokens_;
result.bondedTokens_ = bondedTokens_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmos.staking.v1beta1.Staking.Pool) {
return mergeFrom((cosmos.staking.v1beta1.Staking.Pool)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmos.staking.v1beta1.Staking.Pool other) {
if (other == cosmos.staking.v1beta1.Staking.Pool.getDefaultInstance()) return this;
if (!other.getNotBondedTokens().isEmpty()) {
notBondedTokens_ = other.notBondedTokens_;
onChanged();
}
if (!other.getBondedTokens().isEmpty()) {
bondedTokens_ = other.bondedTokens_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmos.staking.v1beta1.Staking.Pool parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmos.staking.v1beta1.Staking.Pool) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object notBondedTokens_ = "";
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public java.lang.String getNotBondedTokens() {
java.lang.Object ref = notBondedTokens_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
notBondedTokens_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public com.google.protobuf.ByteString
getNotBondedTokensBytes() {
java.lang.Object ref = notBondedTokens_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
notBondedTokens_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public Builder setNotBondedTokens(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
notBondedTokens_ = value;
onChanged();
return this;
}
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public Builder clearNotBondedTokens() {
notBondedTokens_ = getDefaultInstance().getNotBondedTokens();
onChanged();
return this;
}
/**
* string not_bonded_tokens = 1 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "not_bonded_tokens"];
*/
public Builder setNotBondedTokensBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
notBondedTokens_ = value;
onChanged();
return this;
}
private java.lang.Object bondedTokens_ = "";
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public java.lang.String getBondedTokens() {
java.lang.Object ref = bondedTokens_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
bondedTokens_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public com.google.protobuf.ByteString
getBondedTokensBytes() {
java.lang.Object ref = bondedTokens_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
bondedTokens_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public Builder setBondedTokens(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bondedTokens_ = value;
onChanged();
return this;
}
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public Builder clearBondedTokens() {
bondedTokens_ = getDefaultInstance().getBondedTokens();
onChanged();
return this;
}
/**
* string bonded_tokens = 2 [(.gogoproto.nullable) = false, (.gogoproto.customtype) = "github.com/cosmos/cosmos-sdk/types.Int", (.gogoproto.jsontag) = "bonded_tokens", (.gogoproto.moretags) = "yaml:\"bonded_tokens\""];
*/
public Builder setBondedTokensBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bondedTokens_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmos.staking.v1beta1.Pool)
}
// @@protoc_insertion_point(class_scope:cosmos.staking.v1beta1.Pool)
private static final cosmos.staking.v1beta1.Staking.Pool DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmos.staking.v1beta1.Staking.Pool();
}
public static cosmos.staking.v1beta1.Staking.Pool getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Pool parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Pool(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmos.staking.v1beta1.Staking.Pool getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_HistoricalInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_HistoricalInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_CommissionRates_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_CommissionRates_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Commission_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Commission_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Description_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Description_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Validator_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Validator_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_ValAddresses_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_ValAddresses_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_DVPair_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_DVPair_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_DVPairs_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_DVPairs_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_DVVTriplet_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_DVVTriplet_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_DVVTriplets_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_DVVTriplets_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Delegation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Delegation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_UnbondingDelegation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_UnbondingDelegation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_RedelegationEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_RedelegationEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Redelegation_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Redelegation_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Params_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Params_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_DelegationResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_DelegationResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_RedelegationResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_RedelegationResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmos_staking_v1beta1_Pool_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmos_staking_v1beta1_Pool_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n$cosmos/staking/v1beta1/staking.proto\022\026" +
"cosmos.staking.v1beta1\032\024gogoproto/gogo.p" +
"roto\032\031google/protobuf/any.proto\032\036google/" +
"protobuf/duration.proto\032\037google/protobuf" +
"/timestamp.proto\032\031cosmos_proto/cosmos.pr" +
"oto\032\036cosmos/base/v1beta1/coin.proto\032\034ten" +
"dermint/types/types.proto\"y\n\016HistoricalI" +
"nfo\022.\n\006header\030\001 \001(\0132\030.tendermint.types.H" +
"eaderB\004\310\336\037\000\0227\n\006valset\030\002 \003(\0132!.cosmos.sta" +
"king.v1beta1.ValidatorB\004\310\336\037\000\"\221\002\n\017Commiss" +
"ionRates\022<\n\004rate\030\001 \001(\tB.\332\336\037&github.com/c" +
"osmos/cosmos-sdk/types.Dec\310\336\037\000\022S\n\010max_ra" +
"te\030\002 \001(\tBA\362\336\037\017yaml:\"max_rate\"\332\336\037&github." +
"com/cosmos/cosmos-sdk/types.Dec\310\336\037\000\022a\n\017m" +
"ax_change_rate\030\003 \001(\tBH\362\336\037\026yaml:\"max_chan" +
"ge_rate\"\332\336\037&github.com/cosmos/cosmos-sdk" +
"/types.Dec\310\336\037\000:\010\350\240\037\001\230\240\037\000\"\264\001\n\nCommission\022" +
"K\n\020commission_rates\030\001 \001(\0132\'.cosmos.staki" +
"ng.v1beta1.CommissionRatesB\010\320\336\037\001\310\336\037\000\022O\n\013" +
"update_time\030\002 \001(\0132\032.google.protobuf.Time" +
"stampB\036\310\336\037\000\220\337\037\001\362\336\037\022yaml:\"update_time\":\010\350" +
"\240\037\001\230\240\037\000\"\223\001\n\013Description\022\017\n\007moniker\030\001 \001(\t" +
"\022\020\n\010identity\030\002 \001(\t\022\017\n\007website\030\003 \001(\t\0225\n\020s" +
"ecurity_contact\030\004 \001(\tB\033\362\336\037\027yaml:\"securit" +
"y_contact\"\022\017\n\007details\030\005 \001(\t:\010\350\240\037\001\230\240\037\000\"\225\006" +
"\n\tValidator\0225\n\020operator_address\030\001 \001(\tB\033\362" +
"\336\037\027yaml:\"operator_address\"\022c\n\020consensus_" +
"pubkey\030\002 \001(\0132\024.google.protobuf.AnyB3\312\264-\024" +
"cosmos.crypto.PubKey\362\336\037\027yaml:\"consensus_" +
"pubkey\"\022\016\n\006jailed\030\003 \001(\010\0222\n\006status\030\004 \001(\0162" +
"\".cosmos.staking.v1beta1.BondStatus\022>\n\006t" +
"okens\030\005 \001(\tB.\332\336\037&github.com/cosmos/cosmo" +
"s-sdk/types.Int\310\336\037\000\022c\n\020delegator_shares\030" +
"\006 \001(\tBI\362\336\037\027yaml:\"delegator_shares\"\332\336\037&gi" +
"thub.com/cosmos/cosmos-sdk/types.Dec\310\336\037\000" +
"\022>\n\013description\030\007 \001(\0132#.cosmos.staking.v" +
"1beta1.DescriptionB\004\310\336\037\000\0225\n\020unbonding_he" +
"ight\030\010 \001(\003B\033\362\336\037\027yaml:\"unbonding_height\"\022" +
"U\n\016unbonding_time\030\t \001(\0132\032.google.protobu" +
"f.TimestampB!\310\336\037\000\220\337\037\001\362\336\037\025yaml:\"unbonding" +
"_time\"\022<\n\ncommission\030\n \001(\0132\".cosmos.stak" +
"ing.v1beta1.CommissionB\004\310\336\037\000\022i\n\023min_self" +
"_delegation\030\013 \001(\tBL\362\336\037\032yaml:\"min_self_de" +
"legation\"\332\336\037&github.com/cosmos/cosmos-sd" +
"k/types.Int\310\336\037\000:\014\350\240\037\000\230\240\037\000\210\240\037\000\"+\n\014ValAddr" +
"esses\022\021\n\taddresses\030\001 \003(\t:\010\230\240\037\000\200\334 \001\"\210\001\n\006D" +
"VPair\0227\n\021delegator_address\030\001 \001(\tB\034\362\336\037\030ya" +
"ml:\"delegator_address\"\0227\n\021validator_addr" +
"ess\030\002 \001(\tB\034\362\336\037\030yaml:\"validator_address\":" +
"\014\350\240\037\000\210\240\037\000\230\240\037\000\">\n\007DVPairs\0223\n\005pairs\030\001 \003(\0132" +
"\036.cosmos.staking.v1beta1.DVPairB\004\310\336\037\000\"\325\001" +
"\n\nDVVTriplet\0227\n\021delegator_address\030\001 \001(\tB" +
"\034\362\336\037\030yaml:\"delegator_address\"\022?\n\025validat" +
"or_src_address\030\002 \001(\tB \362\336\037\034yaml:\"validato" +
"r_src_address\"\022?\n\025validator_dst_address\030" +
"\003 \001(\tB \362\336\037\034yaml:\"validator_dst_address\":" +
"\014\350\240\037\000\210\240\037\000\230\240\037\000\"I\n\013DVVTriplets\022:\n\010triplets" +
"\030\001 \003(\0132\".cosmos.staking.v1beta1.DVVTripl" +
"etB\004\310\336\037\000\"\314\001\n\nDelegation\0227\n\021delegator_add" +
"ress\030\001 \001(\tB\034\362\336\037\030yaml:\"delegator_address\"" +
"\0227\n\021validator_address\030\002 \001(\tB\034\362\336\037\030yaml:\"v" +
"alidator_address\"\022>\n\006shares\030\003 \001(\tB.\332\336\037&g" +
"ithub.com/cosmos/cosmos-sdk/types.Dec\310\336\037" +
"\000:\014\350\240\037\000\210\240\037\000\230\240\037\000\"\336\001\n\023UnbondingDelegation\022" +
"7\n\021delegator_address\030\001 \001(\tB\034\362\336\037\030yaml:\"de" +
"legator_address\"\0227\n\021validator_address\030\002 " +
"\001(\tB\034\362\336\037\030yaml:\"validator_address\"\022G\n\007ent" +
"ries\030\003 \003(\01320.cosmos.staking.v1beta1.Unbo" +
"ndingDelegationEntryB\004\310\336\037\000:\014\350\240\037\000\210\240\037\000\230\240\037\000" +
"\"\326\002\n\030UnbondingDelegationEntry\0223\n\017creatio" +
"n_height\030\001 \001(\003B\032\362\336\037\026yaml:\"creation_heigh" +
"t\"\022W\n\017completion_time\030\002 \001(\0132\032.google.pro" +
"tobuf.TimestampB\"\310\336\037\000\220\337\037\001\362\336\037\026yaml:\"compl" +
"etion_time\"\022a\n\017initial_balance\030\003 \001(\tBH\332\336" +
"\037&github.com/cosmos/cosmos-sdk/types.Int" +
"\310\336\037\000\362\336\037\026yaml:\"initial_balance\"\022?\n\007balanc" +
"e\030\004 \001(\tB.\332\336\037&github.com/cosmos/cosmos-sd" +
"k/types.Int\310\336\037\000:\010\350\240\037\001\230\240\037\000\"\322\002\n\021Redelegati" +
"onEntry\0223\n\017creation_height\030\001 \001(\003B\032\362\336\037\026ya" +
"ml:\"creation_height\"\022W\n\017completion_time\030" +
"\002 \001(\0132\032.google.protobuf.TimestampB\"\310\336\037\000\220" +
"\337\037\001\362\336\037\026yaml:\"completion_time\"\022a\n\017initial" +
"_balance\030\003 \001(\tBH\332\336\037&github.com/cosmos/co" +
"smos-sdk/types.Int\310\336\037\000\362\336\037\026yaml:\"initial_" +
"balance\"\022B\n\nshares_dst\030\004 \001(\tB.\332\336\037&github" +
".com/cosmos/cosmos-sdk/types.Dec\310\336\037\000:\010\350\240" +
"\037\001\230\240\037\000\"\231\002\n\014Redelegation\0227\n\021delegator_add" +
"ress\030\001 \001(\tB\034\362\336\037\030yaml:\"delegator_address\"" +
"\022?\n\025validator_src_address\030\002 \001(\tB \362\336\037\034yam" +
"l:\"validator_src_address\"\022?\n\025validator_d" +
"st_address\030\003 \001(\tB \362\336\037\034yaml:\"validator_ds" +
"t_address\"\022@\n\007entries\030\004 \003(\0132).cosmos.sta" +
"king.v1beta1.RedelegationEntryB\004\310\336\037\000:\014\350\240" +
"\037\000\210\240\037\000\230\240\037\000\"\256\002\n\006Params\022T\n\016unbonding_time\030" +
"\001 \001(\0132\031.google.protobuf.DurationB!\310\336\037\000\230\337" +
"\037\001\362\336\037\025yaml:\"unbonding_time\"\0221\n\016max_valid" +
"ators\030\002 \001(\rB\031\362\336\037\025yaml:\"max_validators\"\022+" +
"\n\013max_entries\030\003 \001(\rB\026\362\336\037\022yaml:\"max_entri" +
"es\"\0229\n\022historical_entries\030\004 \001(\rB\035\362\336\037\031yam" +
"l:\"historical_entries\"\022)\n\nbond_denom\030\005 \001" +
"(\tB\025\362\336\037\021yaml:\"bond_denom\":\010\350\240\037\001\230\240\037\000\"\216\001\n\022" +
"DelegationResponse\022<\n\ndelegation\030\001 \001(\0132\"" +
".cosmos.staking.v1beta1.DelegationB\004\310\336\037\000" +
"\0220\n\007balance\030\002 \001(\0132\031.cosmos.base.v1beta1." +
"CoinB\004\310\336\037\000:\010\350\240\037\000\230\240\037\000\"\257\001\n\031RedelegationEnt" +
"ryResponse\022K\n\022redelegation_entry\030\001 \001(\0132)" +
".cosmos.staking.v1beta1.RedelegationEntr" +
"yB\004\310\336\037\000\022?\n\007balance\030\004 \001(\tB.\332\336\037&github.com" +
"/cosmos/cosmos-sdk/types.Int\310\336\037\000:\004\350\240\037\001\"\250" +
"\001\n\024RedelegationResponse\022@\n\014redelegation\030" +
"\001 \001(\0132$.cosmos.staking.v1beta1.Redelegat" +
"ionB\004\310\336\037\000\022H\n\007entries\030\002 \003(\01321.cosmos.stak" +
"ing.v1beta1.RedelegationEntryResponseB\004\310" +
"\336\037\000:\004\350\240\037\000\"\340\001\n\004Pool\022^\n\021not_bonded_tokens\030" +
"\001 \001(\tBC\332\336\037&github.com/cosmos/cosmos-sdk/" +
"types.Int\352\336\037\021not_bonded_tokens\310\336\037\000\022n\n\rbo" +
"nded_tokens\030\002 \001(\tBW\352\336\037\rbonded_tokens\332\336\037&" +
"github.com/cosmos/cosmos-sdk/types.Int\310\336" +
"\037\000\362\336\037\024yaml:\"bonded_tokens\":\010\360\240\037\001\350\240\037\001*\266\001\n" +
"\nBondStatus\022,\n\027BOND_STATUS_UNSPECIFIED\020\000" +
"\032\017\212\235 \013Unspecified\022&\n\024BOND_STATUS_UNBONDE" +
"D\020\001\032\014\212\235 \010Unbonded\022(\n\025BOND_STATUS_UNBONDI" +
"NG\020\002\032\r\212\235 \tUnbonding\022\"\n\022BOND_STATUS_BONDE" +
"D\020\003\032\n\212\235 \006Bonded\032\004\210\243\036\000B.Z,github.com/cosm" +
"os/cosmos-sdk/x/staking/typesb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
com.google.protobuf.DurationProto.getDescriptor(),
com.google.protobuf.TimestampProto.getDescriptor(),
cosmos_proto.Cosmos.getDescriptor(),
cosmos.base.v1beta1.CoinOuterClass.getDescriptor(),
tendermint.types.Types.getDescriptor(),
}, assigner);
internal_static_cosmos_staking_v1beta1_HistoricalInfo_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cosmos_staking_v1beta1_HistoricalInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_HistoricalInfo_descriptor,
new java.lang.String[] { "Header", "Valset", });
internal_static_cosmos_staking_v1beta1_CommissionRates_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cosmos_staking_v1beta1_CommissionRates_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_CommissionRates_descriptor,
new java.lang.String[] { "Rate", "MaxRate", "MaxChangeRate", });
internal_static_cosmos_staking_v1beta1_Commission_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_cosmos_staking_v1beta1_Commission_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Commission_descriptor,
new java.lang.String[] { "CommissionRates", "UpdateTime", });
internal_static_cosmos_staking_v1beta1_Description_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_cosmos_staking_v1beta1_Description_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Description_descriptor,
new java.lang.String[] { "Moniker", "Identity", "Website", "SecurityContact", "Details", });
internal_static_cosmos_staking_v1beta1_Validator_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_cosmos_staking_v1beta1_Validator_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Validator_descriptor,
new java.lang.String[] { "OperatorAddress", "ConsensusPubkey", "Jailed", "Status", "Tokens", "DelegatorShares", "Description", "UnbondingHeight", "UnbondingTime", "Commission", "MinSelfDelegation", });
internal_static_cosmos_staking_v1beta1_ValAddresses_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_cosmos_staking_v1beta1_ValAddresses_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_ValAddresses_descriptor,
new java.lang.String[] { "Addresses", });
internal_static_cosmos_staking_v1beta1_DVPair_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_cosmos_staking_v1beta1_DVPair_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_DVPair_descriptor,
new java.lang.String[] { "DelegatorAddress", "ValidatorAddress", });
internal_static_cosmos_staking_v1beta1_DVPairs_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_cosmos_staking_v1beta1_DVPairs_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_DVPairs_descriptor,
new java.lang.String[] { "Pairs", });
internal_static_cosmos_staking_v1beta1_DVVTriplet_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_cosmos_staking_v1beta1_DVVTriplet_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_DVVTriplet_descriptor,
new java.lang.String[] { "DelegatorAddress", "ValidatorSrcAddress", "ValidatorDstAddress", });
internal_static_cosmos_staking_v1beta1_DVVTriplets_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_cosmos_staking_v1beta1_DVVTriplets_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_DVVTriplets_descriptor,
new java.lang.String[] { "Triplets", });
internal_static_cosmos_staking_v1beta1_Delegation_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_cosmos_staking_v1beta1_Delegation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Delegation_descriptor,
new java.lang.String[] { "DelegatorAddress", "ValidatorAddress", "Shares", });
internal_static_cosmos_staking_v1beta1_UnbondingDelegation_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_cosmos_staking_v1beta1_UnbondingDelegation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_UnbondingDelegation_descriptor,
new java.lang.String[] { "DelegatorAddress", "ValidatorAddress", "Entries", });
internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_UnbondingDelegationEntry_descriptor,
new java.lang.String[] { "CreationHeight", "CompletionTime", "InitialBalance", "Balance", });
internal_static_cosmos_staking_v1beta1_RedelegationEntry_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_cosmos_staking_v1beta1_RedelegationEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_RedelegationEntry_descriptor,
new java.lang.String[] { "CreationHeight", "CompletionTime", "InitialBalance", "SharesDst", });
internal_static_cosmos_staking_v1beta1_Redelegation_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_cosmos_staking_v1beta1_Redelegation_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Redelegation_descriptor,
new java.lang.String[] { "DelegatorAddress", "ValidatorSrcAddress", "ValidatorDstAddress", "Entries", });
internal_static_cosmos_staking_v1beta1_Params_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_cosmos_staking_v1beta1_Params_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Params_descriptor,
new java.lang.String[] { "UnbondingTime", "MaxValidators", "MaxEntries", "HistoricalEntries", "BondDenom", });
internal_static_cosmos_staking_v1beta1_DelegationResponse_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_cosmos_staking_v1beta1_DelegationResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_DelegationResponse_descriptor,
new java.lang.String[] { "Delegation", "Balance", });
internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_RedelegationEntryResponse_descriptor,
new java.lang.String[] { "RedelegationEntry", "Balance", });
internal_static_cosmos_staking_v1beta1_RedelegationResponse_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_cosmos_staking_v1beta1_RedelegationResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_RedelegationResponse_descriptor,
new java.lang.String[] { "Redelegation", "Entries", });
internal_static_cosmos_staking_v1beta1_Pool_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_cosmos_staking_v1beta1_Pool_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmos_staking_v1beta1_Pool_descriptor,
new java.lang.String[] { "NotBondedTokens", "BondedTokens", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(cosmos_proto.Cosmos.acceptsInterface);
registry.add(com.google.protobuf.GoGoProtos.customtype);
registry.add(com.google.protobuf.GoGoProtos.description);
registry.add(com.google.protobuf.GoGoProtos.embed);
registry.add(com.google.protobuf.GoGoProtos.enumvalueCustomname);
registry.add(com.google.protobuf.GoGoProtos.equal);
registry.add(com.google.protobuf.GoGoProtos.goprotoEnumPrefix);
registry.add(com.google.protobuf.GoGoProtos.goprotoGetters);
registry.add(com.google.protobuf.GoGoProtos.goprotoStringer);
registry.add(com.google.protobuf.GoGoProtos.jsontag);
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
registry.add(com.google.protobuf.GoGoProtos.stdduration);
registry.add(com.google.protobuf.GoGoProtos.stdtime);
registry.add(com.google.protobuf.GoGoProtos.stringer);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
com.google.protobuf.DurationProto.getDescriptor();
com.google.protobuf.TimestampProto.getDescriptor();
cosmos_proto.Cosmos.getDescriptor();
cosmos.base.v1beta1.CoinOuterClass.getDescriptor();
tendermint.types.Types.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy