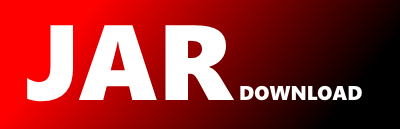
cosmwasm.wasm.v1beta1.Proposal Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: x/wasm/internal/types/proposal.proto
package cosmwasm.wasm.v1beta1;
public final class Proposal {
private Proposal() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface StoreCodeProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.StoreCodeProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
java.lang.String getRunAs();
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
com.google.protobuf.ByteString
getRunAsBytes();
/**
*
* WASMByteCode can be raw or gzip compressed
*
*
* bytes wasm_byte_code = 4 [(.gogoproto.customname) = "WASMByteCode"];
*/
com.google.protobuf.ByteString getWasmByteCode();
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
java.lang.String getSource();
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
com.google.protobuf.ByteString
getSourceBytes();
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
java.lang.String getBuilder();
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
com.google.protobuf.ByteString
getBuilderBytes();
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
boolean hasInstantiatePermission();
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
cosmwasm.wasm.v1beta1.Types.AccessConfig getInstantiatePermission();
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
cosmwasm.wasm.v1beta1.Types.AccessConfigOrBuilder getInstantiatePermissionOrBuilder();
}
/**
*
* StoreCodeProposal gov proposal content type to submit WASM code to the system
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.StoreCodeProposal}
*/
public static final class StoreCodeProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.StoreCodeProposal)
StoreCodeProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use StoreCodeProposal.newBuilder() to construct.
private StoreCodeProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StoreCodeProposal() {
title_ = "";
description_ = "";
runAs_ = "";
wasmByteCode_ = com.google.protobuf.ByteString.EMPTY;
source_ = "";
builder_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StoreCodeProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
runAs_ = s;
break;
}
case 34: {
wasmByteCode_ = input.readBytes();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
source_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
builder_ = s;
break;
}
case 58: {
cosmwasm.wasm.v1beta1.Types.AccessConfig.Builder subBuilder = null;
if (instantiatePermission_ != null) {
subBuilder = instantiatePermission_.toBuilder();
}
instantiatePermission_ = input.readMessage(cosmwasm.wasm.v1beta1.Types.AccessConfig.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(instantiatePermission_);
instantiatePermission_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.class, cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.Builder.class);
}
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RUN_AS_FIELD_NUMBER = 3;
private volatile java.lang.Object runAs_;
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public java.lang.String getRunAs() {
java.lang.Object ref = runAs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runAs_ = s;
return s;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public com.google.protobuf.ByteString
getRunAsBytes() {
java.lang.Object ref = runAs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runAs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WASM_BYTE_CODE_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString wasmByteCode_;
/**
*
* WASMByteCode can be raw or gzip compressed
*
*
* bytes wasm_byte_code = 4 [(.gogoproto.customname) = "WASMByteCode"];
*/
public com.google.protobuf.ByteString getWasmByteCode() {
return wasmByteCode_;
}
public static final int SOURCE_FIELD_NUMBER = 5;
private volatile java.lang.Object source_;
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
}
}
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BUILDER_FIELD_NUMBER = 6;
private volatile java.lang.Object builder_;
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public java.lang.String getBuilder() {
java.lang.Object ref = builder_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
builder_ = s;
return s;
}
}
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public com.google.protobuf.ByteString
getBuilderBytes() {
java.lang.Object ref = builder_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
builder_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANTIATE_PERMISSION_FIELD_NUMBER = 7;
private cosmwasm.wasm.v1beta1.Types.AccessConfig instantiatePermission_;
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public boolean hasInstantiatePermission() {
return instantiatePermission_ != null;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public cosmwasm.wasm.v1beta1.Types.AccessConfig getInstantiatePermission() {
return instantiatePermission_ == null ? cosmwasm.wasm.v1beta1.Types.AccessConfig.getDefaultInstance() : instantiatePermission_;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public cosmwasm.wasm.v1beta1.Types.AccessConfigOrBuilder getInstantiatePermissionOrBuilder() {
return getInstantiatePermission();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getRunAsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, runAs_);
}
if (!wasmByteCode_.isEmpty()) {
output.writeBytes(4, wasmByteCode_);
}
if (!getSourceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, source_);
}
if (!getBuilderBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, builder_);
}
if (instantiatePermission_ != null) {
output.writeMessage(7, getInstantiatePermission());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getRunAsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, runAs_);
}
if (!wasmByteCode_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, wasmByteCode_);
}
if (!getSourceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, source_);
}
if (!getBuilderBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, builder_);
}
if (instantiatePermission_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getInstantiatePermission());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal other = (cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getRunAs()
.equals(other.getRunAs());
result = result && getWasmByteCode()
.equals(other.getWasmByteCode());
result = result && getSource()
.equals(other.getSource());
result = result && getBuilder()
.equals(other.getBuilder());
result = result && (hasInstantiatePermission() == other.hasInstantiatePermission());
if (hasInstantiatePermission()) {
result = result && getInstantiatePermission()
.equals(other.getInstantiatePermission());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + RUN_AS_FIELD_NUMBER;
hash = (53 * hash) + getRunAs().hashCode();
hash = (37 * hash) + WASM_BYTE_CODE_FIELD_NUMBER;
hash = (53 * hash) + getWasmByteCode().hashCode();
hash = (37 * hash) + SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSource().hashCode();
hash = (37 * hash) + BUILDER_FIELD_NUMBER;
hash = (53 * hash) + getBuilder().hashCode();
if (hasInstantiatePermission()) {
hash = (37 * hash) + INSTANTIATE_PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + getInstantiatePermission().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* StoreCodeProposal gov proposal content type to submit WASM code to the system
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.StoreCodeProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.StoreCodeProposal)
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.class, cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
runAs_ = "";
wasmByteCode_ = com.google.protobuf.ByteString.EMPTY;
source_ = "";
builder_ = "";
if (instantiatePermissionBuilder_ == null) {
instantiatePermission_ = null;
} else {
instantiatePermission_ = null;
instantiatePermissionBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal build() {
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal result = new cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal(this);
result.title_ = title_;
result.description_ = description_;
result.runAs_ = runAs_;
result.wasmByteCode_ = wasmByteCode_;
result.source_ = source_;
result.builder_ = builder_;
if (instantiatePermissionBuilder_ == null) {
result.instantiatePermission_ = instantiatePermission_;
} else {
result.instantiatePermission_ = instantiatePermissionBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getRunAs().isEmpty()) {
runAs_ = other.runAs_;
onChanged();
}
if (other.getWasmByteCode() != com.google.protobuf.ByteString.EMPTY) {
setWasmByteCode(other.getWasmByteCode());
}
if (!other.getSource().isEmpty()) {
source_ = other.source_;
onChanged();
}
if (!other.getBuilder().isEmpty()) {
builder_ = other.builder_;
onChanged();
}
if (other.hasInstantiatePermission()) {
mergeInstantiatePermission(other.getInstantiatePermission());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object runAs_ = "";
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public java.lang.String getRunAs() {
java.lang.Object ref = runAs_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runAs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public com.google.protobuf.ByteString
getRunAsBytes() {
java.lang.Object ref = runAs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runAs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder setRunAs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runAs_ = value;
onChanged();
return this;
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder clearRunAs() {
runAs_ = getDefaultInstance().getRunAs();
onChanged();
return this;
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder setRunAsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runAs_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString wasmByteCode_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* WASMByteCode can be raw or gzip compressed
*
*
* bytes wasm_byte_code = 4 [(.gogoproto.customname) = "WASMByteCode"];
*/
public com.google.protobuf.ByteString getWasmByteCode() {
return wasmByteCode_;
}
/**
*
* WASMByteCode can be raw or gzip compressed
*
*
* bytes wasm_byte_code = 4 [(.gogoproto.customname) = "WASMByteCode"];
*/
public Builder setWasmByteCode(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
wasmByteCode_ = value;
onChanged();
return this;
}
/**
*
* WASMByteCode can be raw or gzip compressed
*
*
* bytes wasm_byte_code = 4 [(.gogoproto.customname) = "WASMByteCode"];
*/
public Builder clearWasmByteCode() {
wasmByteCode_ = getDefaultInstance().getWasmByteCode();
onChanged();
return this;
}
private java.lang.Object source_ = "";
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public com.google.protobuf.ByteString
getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public Builder setSource(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
source_ = value;
onChanged();
return this;
}
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public Builder clearSource() {
source_ = getDefaultInstance().getSource();
onChanged();
return this;
}
/**
*
* Source is a valid absolute HTTPS URI to the contract's source code, optional
*
*
* string source = 5;
*/
public Builder setSourceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
source_ = value;
onChanged();
return this;
}
private java.lang.Object builder_ = "";
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public java.lang.String getBuilder() {
java.lang.Object ref = builder_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
builder_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public com.google.protobuf.ByteString
getBuilderBytes() {
java.lang.Object ref = builder_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
builder_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public Builder setBuilder(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
builder_ = value;
onChanged();
return this;
}
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public Builder clearBuilder() {
builder_ = getDefaultInstance().getBuilder();
onChanged();
return this;
}
/**
*
* Builder is a valid docker image name with tag, optional
*
*
* string builder = 6;
*/
public Builder setBuilderBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
builder_ = value;
onChanged();
return this;
}
private cosmwasm.wasm.v1beta1.Types.AccessConfig instantiatePermission_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmwasm.wasm.v1beta1.Types.AccessConfig, cosmwasm.wasm.v1beta1.Types.AccessConfig.Builder, cosmwasm.wasm.v1beta1.Types.AccessConfigOrBuilder> instantiatePermissionBuilder_;
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public boolean hasInstantiatePermission() {
return instantiatePermissionBuilder_ != null || instantiatePermission_ != null;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public cosmwasm.wasm.v1beta1.Types.AccessConfig getInstantiatePermission() {
if (instantiatePermissionBuilder_ == null) {
return instantiatePermission_ == null ? cosmwasm.wasm.v1beta1.Types.AccessConfig.getDefaultInstance() : instantiatePermission_;
} else {
return instantiatePermissionBuilder_.getMessage();
}
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public Builder setInstantiatePermission(cosmwasm.wasm.v1beta1.Types.AccessConfig value) {
if (instantiatePermissionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
instantiatePermission_ = value;
onChanged();
} else {
instantiatePermissionBuilder_.setMessage(value);
}
return this;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public Builder setInstantiatePermission(
cosmwasm.wasm.v1beta1.Types.AccessConfig.Builder builderForValue) {
if (instantiatePermissionBuilder_ == null) {
instantiatePermission_ = builderForValue.build();
onChanged();
} else {
instantiatePermissionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public Builder mergeInstantiatePermission(cosmwasm.wasm.v1beta1.Types.AccessConfig value) {
if (instantiatePermissionBuilder_ == null) {
if (instantiatePermission_ != null) {
instantiatePermission_ =
cosmwasm.wasm.v1beta1.Types.AccessConfig.newBuilder(instantiatePermission_).mergeFrom(value).buildPartial();
} else {
instantiatePermission_ = value;
}
onChanged();
} else {
instantiatePermissionBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public Builder clearInstantiatePermission() {
if (instantiatePermissionBuilder_ == null) {
instantiatePermission_ = null;
onChanged();
} else {
instantiatePermission_ = null;
instantiatePermissionBuilder_ = null;
}
return this;
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public cosmwasm.wasm.v1beta1.Types.AccessConfig.Builder getInstantiatePermissionBuilder() {
onChanged();
return getInstantiatePermissionFieldBuilder().getBuilder();
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
public cosmwasm.wasm.v1beta1.Types.AccessConfigOrBuilder getInstantiatePermissionOrBuilder() {
if (instantiatePermissionBuilder_ != null) {
return instantiatePermissionBuilder_.getMessageOrBuilder();
} else {
return instantiatePermission_ == null ?
cosmwasm.wasm.v1beta1.Types.AccessConfig.getDefaultInstance() : instantiatePermission_;
}
}
/**
*
* InstantiatePermission to apply on contract creation, optional
*
*
* .cosmwasm.wasm.v1beta1.AccessConfig instantiate_permission = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmwasm.wasm.v1beta1.Types.AccessConfig, cosmwasm.wasm.v1beta1.Types.AccessConfig.Builder, cosmwasm.wasm.v1beta1.Types.AccessConfigOrBuilder>
getInstantiatePermissionFieldBuilder() {
if (instantiatePermissionBuilder_ == null) {
instantiatePermissionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmwasm.wasm.v1beta1.Types.AccessConfig, cosmwasm.wasm.v1beta1.Types.AccessConfig.Builder, cosmwasm.wasm.v1beta1.Types.AccessConfigOrBuilder>(
getInstantiatePermission(),
getParentForChildren(),
isClean());
instantiatePermission_ = null;
}
return instantiatePermissionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.StoreCodeProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.StoreCodeProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StoreCodeProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StoreCodeProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.StoreCodeProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InstantiateContractProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.InstantiateContractProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
java.lang.String getRunAs();
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
com.google.protobuf.ByteString
getRunAsBytes();
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
java.lang.String getAdmin();
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
com.google.protobuf.ByteString
getAdminBytes();
/**
*
* CodeID is the reference to the stored WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
long getCodeId();
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
java.lang.String getLabel();
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
com.google.protobuf.ByteString
getLabelBytes();
/**
*
* InitMsg json encoded message to be passed to the contract on instantiation
*
*
* bytes init_msg = 7 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
com.google.protobuf.ByteString getInitMsg();
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List
getFundsList();
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.Coin getFunds(int index);
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
int getFundsCount();
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getFundsOrBuilderList();
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getFundsOrBuilder(
int index);
}
/**
*
* InstantiateContractProposal gov proposal content type to instantiate a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.InstantiateContractProposal}
*/
public static final class InstantiateContractProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.InstantiateContractProposal)
InstantiateContractProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use InstantiateContractProposal.newBuilder() to construct.
private InstantiateContractProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InstantiateContractProposal() {
title_ = "";
description_ = "";
runAs_ = "";
admin_ = "";
codeId_ = 0L;
label_ = "";
initMsg_ = com.google.protobuf.ByteString.EMPTY;
funds_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InstantiateContractProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
runAs_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
admin_ = s;
break;
}
case 40: {
codeId_ = input.readUInt64();
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
label_ = s;
break;
}
case 58: {
initMsg_ = input.readBytes();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
funds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
funds_.add(
input.readMessage(cosmos.base.v1beta1.CoinOuterClass.Coin.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
funds_ = java.util.Collections.unmodifiableList(funds_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.class, cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.Builder.class);
}
private int bitField0_;
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RUN_AS_FIELD_NUMBER = 3;
private volatile java.lang.Object runAs_;
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public java.lang.String getRunAs() {
java.lang.Object ref = runAs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runAs_ = s;
return s;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public com.google.protobuf.ByteString
getRunAsBytes() {
java.lang.Object ref = runAs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runAs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADMIN_FIELD_NUMBER = 4;
private volatile java.lang.Object admin_;
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public java.lang.String getAdmin() {
java.lang.Object ref = admin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
admin_ = s;
return s;
}
}
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public com.google.protobuf.ByteString
getAdminBytes() {
java.lang.Object ref = admin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
admin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CODE_ID_FIELD_NUMBER = 5;
private long codeId_;
/**
*
* CodeID is the reference to the stored WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public long getCodeId() {
return codeId_;
}
public static final int LABEL_FIELD_NUMBER = 6;
private volatile java.lang.Object label_;
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
label_ = s;
return s;
}
}
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INIT_MSG_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString initMsg_;
/**
*
* InitMsg json encoded message to be passed to the contract on instantiation
*
*
* bytes init_msg = 7 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public com.google.protobuf.ByteString getInitMsg() {
return initMsg_;
}
public static final int FUNDS_FIELD_NUMBER = 8;
private java.util.List funds_;
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getFundsList() {
return funds_;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getFundsOrBuilderList() {
return funds_;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getFundsCount() {
return funds_.size();
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getFunds(int index) {
return funds_.get(index);
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getFundsOrBuilder(
int index) {
return funds_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getRunAsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, runAs_);
}
if (!getAdminBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, admin_);
}
if (codeId_ != 0L) {
output.writeUInt64(5, codeId_);
}
if (!getLabelBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, label_);
}
if (!initMsg_.isEmpty()) {
output.writeBytes(7, initMsg_);
}
for (int i = 0; i < funds_.size(); i++) {
output.writeMessage(8, funds_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getRunAsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, runAs_);
}
if (!getAdminBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, admin_);
}
if (codeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, codeId_);
}
if (!getLabelBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, label_);
}
if (!initMsg_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, initMsg_);
}
for (int i = 0; i < funds_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, funds_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal other = (cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getRunAs()
.equals(other.getRunAs());
result = result && getAdmin()
.equals(other.getAdmin());
result = result && (getCodeId()
== other.getCodeId());
result = result && getLabel()
.equals(other.getLabel());
result = result && getInitMsg()
.equals(other.getInitMsg());
result = result && getFundsList()
.equals(other.getFundsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + RUN_AS_FIELD_NUMBER;
hash = (53 * hash) + getRunAs().hashCode();
hash = (37 * hash) + ADMIN_FIELD_NUMBER;
hash = (53 * hash) + getAdmin().hashCode();
hash = (37 * hash) + CODE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCodeId());
hash = (37 * hash) + LABEL_FIELD_NUMBER;
hash = (53 * hash) + getLabel().hashCode();
hash = (37 * hash) + INIT_MSG_FIELD_NUMBER;
hash = (53 * hash) + getInitMsg().hashCode();
if (getFundsCount() > 0) {
hash = (37 * hash) + FUNDS_FIELD_NUMBER;
hash = (53 * hash) + getFundsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* InstantiateContractProposal gov proposal content type to instantiate a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.InstantiateContractProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.InstantiateContractProposal)
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.class, cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFundsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
runAs_ = "";
admin_ = "";
codeId_ = 0L;
label_ = "";
initMsg_ = com.google.protobuf.ByteString.EMPTY;
if (fundsBuilder_ == null) {
funds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
fundsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal build() {
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal result = new cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.title_ = title_;
result.description_ = description_;
result.runAs_ = runAs_;
result.admin_ = admin_;
result.codeId_ = codeId_;
result.label_ = label_;
result.initMsg_ = initMsg_;
if (fundsBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
funds_ = java.util.Collections.unmodifiableList(funds_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.funds_ = funds_;
} else {
result.funds_ = fundsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getRunAs().isEmpty()) {
runAs_ = other.runAs_;
onChanged();
}
if (!other.getAdmin().isEmpty()) {
admin_ = other.admin_;
onChanged();
}
if (other.getCodeId() != 0L) {
setCodeId(other.getCodeId());
}
if (!other.getLabel().isEmpty()) {
label_ = other.label_;
onChanged();
}
if (other.getInitMsg() != com.google.protobuf.ByteString.EMPTY) {
setInitMsg(other.getInitMsg());
}
if (fundsBuilder_ == null) {
if (!other.funds_.isEmpty()) {
if (funds_.isEmpty()) {
funds_ = other.funds_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureFundsIsMutable();
funds_.addAll(other.funds_);
}
onChanged();
}
} else {
if (!other.funds_.isEmpty()) {
if (fundsBuilder_.isEmpty()) {
fundsBuilder_.dispose();
fundsBuilder_ = null;
funds_ = other.funds_;
bitField0_ = (bitField0_ & ~0x00000080);
fundsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFundsFieldBuilder() : null;
} else {
fundsBuilder_.addAllMessages(other.funds_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object runAs_ = "";
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public java.lang.String getRunAs() {
java.lang.Object ref = runAs_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runAs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public com.google.protobuf.ByteString
getRunAsBytes() {
java.lang.Object ref = runAs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runAs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder setRunAs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runAs_ = value;
onChanged();
return this;
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder clearRunAs() {
runAs_ = getDefaultInstance().getRunAs();
onChanged();
return this;
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder setRunAsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runAs_ = value;
onChanged();
return this;
}
private java.lang.Object admin_ = "";
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public java.lang.String getAdmin() {
java.lang.Object ref = admin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
admin_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public com.google.protobuf.ByteString
getAdminBytes() {
java.lang.Object ref = admin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
admin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public Builder setAdmin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
admin_ = value;
onChanged();
return this;
}
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public Builder clearAdmin() {
admin_ = getDefaultInstance().getAdmin();
onChanged();
return this;
}
/**
*
* Admin is an optional address that can execute migrations
*
*
* string admin = 4;
*/
public Builder setAdminBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
admin_ = value;
onChanged();
return this;
}
private long codeId_ ;
/**
*
* CodeID is the reference to the stored WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public long getCodeId() {
return codeId_;
}
/**
*
* CodeID is the reference to the stored WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public Builder setCodeId(long value) {
codeId_ = value;
onChanged();
return this;
}
/**
*
* CodeID is the reference to the stored WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public Builder clearCodeId() {
codeId_ = 0L;
onChanged();
return this;
}
private java.lang.Object label_ = "";
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
label_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public Builder setLabel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
label_ = value;
onChanged();
return this;
}
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public Builder clearLabel() {
label_ = getDefaultInstance().getLabel();
onChanged();
return this;
}
/**
*
* Label is optional metadata to be stored with a constract instance.
*
*
* string label = 6;
*/
public Builder setLabelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
label_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString initMsg_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* InitMsg json encoded message to be passed to the contract on instantiation
*
*
* bytes init_msg = 7 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public com.google.protobuf.ByteString getInitMsg() {
return initMsg_;
}
/**
*
* InitMsg json encoded message to be passed to the contract on instantiation
*
*
* bytes init_msg = 7 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public Builder setInitMsg(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
initMsg_ = value;
onChanged();
return this;
}
/**
*
* InitMsg json encoded message to be passed to the contract on instantiation
*
*
* bytes init_msg = 7 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public Builder clearInitMsg() {
initMsg_ = getDefaultInstance().getInitMsg();
onChanged();
return this;
}
private java.util.List funds_ =
java.util.Collections.emptyList();
private void ensureFundsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
funds_ = new java.util.ArrayList(funds_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder> fundsBuilder_;
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List getFundsList() {
if (fundsBuilder_ == null) {
return java.util.Collections.unmodifiableList(funds_);
} else {
return fundsBuilder_.getMessageList();
}
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public int getFundsCount() {
if (fundsBuilder_ == null) {
return funds_.size();
} else {
return fundsBuilder_.getCount();
}
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin getFunds(int index) {
if (fundsBuilder_ == null) {
return funds_.get(index);
} else {
return fundsBuilder_.getMessage(index);
}
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setFunds(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (fundsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFundsIsMutable();
funds_.set(index, value);
onChanged();
} else {
fundsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder setFunds(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (fundsBuilder_ == null) {
ensureFundsIsMutable();
funds_.set(index, builderForValue.build());
onChanged();
} else {
fundsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addFunds(cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (fundsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFundsIsMutable();
funds_.add(value);
onChanged();
} else {
fundsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addFunds(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin value) {
if (fundsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFundsIsMutable();
funds_.add(index, value);
onChanged();
} else {
fundsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addFunds(
cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (fundsBuilder_ == null) {
ensureFundsIsMutable();
funds_.add(builderForValue.build());
onChanged();
} else {
fundsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addFunds(
int index, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder builderForValue) {
if (fundsBuilder_ == null) {
ensureFundsIsMutable();
funds_.add(index, builderForValue.build());
onChanged();
} else {
fundsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder addAllFunds(
java.lang.Iterable extends cosmos.base.v1beta1.CoinOuterClass.Coin> values) {
if (fundsBuilder_ == null) {
ensureFundsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, funds_);
onChanged();
} else {
fundsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder clearFunds() {
if (fundsBuilder_ == null) {
funds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
fundsBuilder_.clear();
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public Builder removeFunds(int index) {
if (fundsBuilder_ == null) {
ensureFundsIsMutable();
funds_.remove(index);
onChanged();
} else {
fundsBuilder_.remove(index);
}
return this;
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder getFundsBuilder(
int index) {
return getFundsFieldBuilder().getBuilder(index);
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder getFundsOrBuilder(
int index) {
if (fundsBuilder_ == null) {
return funds_.get(index); } else {
return fundsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List extends cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getFundsOrBuilderList() {
if (fundsBuilder_ != null) {
return fundsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(funds_);
}
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addFundsBuilder() {
return getFundsFieldBuilder().addBuilder(
cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public cosmos.base.v1beta1.CoinOuterClass.Coin.Builder addFundsBuilder(
int index) {
return getFundsFieldBuilder().addBuilder(
index, cosmos.base.v1beta1.CoinOuterClass.Coin.getDefaultInstance());
}
/**
*
* Funds coins that are transferred to the contract on instantiation
*
*
* repeated .cosmos.base.v1beta1.Coin funds = 8 [(.gogoproto.nullable) = false, (.gogoproto.castrepeated) = "github.com/cosmos/cosmos-sdk/types.Coins"];
*/
public java.util.List
getFundsBuilderList() {
return getFundsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>
getFundsFieldBuilder() {
if (fundsBuilder_ == null) {
fundsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cosmos.base.v1beta1.CoinOuterClass.Coin, cosmos.base.v1beta1.CoinOuterClass.Coin.Builder, cosmos.base.v1beta1.CoinOuterClass.CoinOrBuilder>(
funds_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
funds_ = null;
}
return fundsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.InstantiateContractProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.InstantiateContractProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InstantiateContractProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InstantiateContractProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.InstantiateContractProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MigrateContractProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.MigrateContractProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
java.lang.String getRunAs();
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
com.google.protobuf.ByteString
getRunAsBytes();
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
java.lang.String getContract();
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
com.google.protobuf.ByteString
getContractBytes();
/**
*
* CodeID references the new WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
long getCodeId();
/**
*
* MigrateMsg json encoded message to be passed to the contract on migration
*
*
* bytes migrate_msg = 6 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
com.google.protobuf.ByteString getMigrateMsg();
}
/**
*
* MigrateContractProposal gov proposal content type to migrate a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.MigrateContractProposal}
*/
public static final class MigrateContractProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.MigrateContractProposal)
MigrateContractProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use MigrateContractProposal.newBuilder() to construct.
private MigrateContractProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MigrateContractProposal() {
title_ = "";
description_ = "";
runAs_ = "";
contract_ = "";
codeId_ = 0L;
migrateMsg_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MigrateContractProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
runAs_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
contract_ = s;
break;
}
case 40: {
codeId_ = input.readUInt64();
break;
}
case 50: {
migrateMsg_ = input.readBytes();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.class, cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.Builder.class);
}
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RUN_AS_FIELD_NUMBER = 3;
private volatile java.lang.Object runAs_;
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public java.lang.String getRunAs() {
java.lang.Object ref = runAs_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runAs_ = s;
return s;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public com.google.protobuf.ByteString
getRunAsBytes() {
java.lang.Object ref = runAs_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runAs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTRACT_FIELD_NUMBER = 4;
private volatile java.lang.Object contract_;
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public java.lang.String getContract() {
java.lang.Object ref = contract_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contract_ = s;
return s;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public com.google.protobuf.ByteString
getContractBytes() {
java.lang.Object ref = contract_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contract_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CODE_ID_FIELD_NUMBER = 5;
private long codeId_;
/**
*
* CodeID references the new WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public long getCodeId() {
return codeId_;
}
public static final int MIGRATE_MSG_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString migrateMsg_;
/**
*
* MigrateMsg json encoded message to be passed to the contract on migration
*
*
* bytes migrate_msg = 6 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public com.google.protobuf.ByteString getMigrateMsg() {
return migrateMsg_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getRunAsBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, runAs_);
}
if (!getContractBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, contract_);
}
if (codeId_ != 0L) {
output.writeUInt64(5, codeId_);
}
if (!migrateMsg_.isEmpty()) {
output.writeBytes(6, migrateMsg_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getRunAsBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, runAs_);
}
if (!getContractBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, contract_);
}
if (codeId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, codeId_);
}
if (!migrateMsg_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, migrateMsg_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal other = (cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getRunAs()
.equals(other.getRunAs());
result = result && getContract()
.equals(other.getContract());
result = result && (getCodeId()
== other.getCodeId());
result = result && getMigrateMsg()
.equals(other.getMigrateMsg());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + RUN_AS_FIELD_NUMBER;
hash = (53 * hash) + getRunAs().hashCode();
hash = (37 * hash) + CONTRACT_FIELD_NUMBER;
hash = (53 * hash) + getContract().hashCode();
hash = (37 * hash) + CODE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCodeId());
hash = (37 * hash) + MIGRATE_MSG_FIELD_NUMBER;
hash = (53 * hash) + getMigrateMsg().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MigrateContractProposal gov proposal content type to migrate a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.MigrateContractProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.MigrateContractProposal)
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.class, cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
runAs_ = "";
contract_ = "";
codeId_ = 0L;
migrateMsg_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal build() {
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal result = new cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal(this);
result.title_ = title_;
result.description_ = description_;
result.runAs_ = runAs_;
result.contract_ = contract_;
result.codeId_ = codeId_;
result.migrateMsg_ = migrateMsg_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getRunAs().isEmpty()) {
runAs_ = other.runAs_;
onChanged();
}
if (!other.getContract().isEmpty()) {
contract_ = other.contract_;
onChanged();
}
if (other.getCodeId() != 0L) {
setCodeId(other.getCodeId());
}
if (other.getMigrateMsg() != com.google.protobuf.ByteString.EMPTY) {
setMigrateMsg(other.getMigrateMsg());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object runAs_ = "";
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public java.lang.String getRunAs() {
java.lang.Object ref = runAs_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
runAs_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public com.google.protobuf.ByteString
getRunAsBytes() {
java.lang.Object ref = runAs_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runAs_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder setRunAs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
runAs_ = value;
onChanged();
return this;
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder clearRunAs() {
runAs_ = getDefaultInstance().getRunAs();
onChanged();
return this;
}
/**
*
* RunAs is the address that is passed to the contract's environment as sender
*
*
* string run_as = 3;
*/
public Builder setRunAsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
runAs_ = value;
onChanged();
return this;
}
private java.lang.Object contract_ = "";
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public java.lang.String getContract() {
java.lang.Object ref = contract_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contract_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public com.google.protobuf.ByteString
getContractBytes() {
java.lang.Object ref = contract_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contract_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public Builder setContract(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contract_ = value;
onChanged();
return this;
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public Builder clearContract() {
contract_ = getDefaultInstance().getContract();
onChanged();
return this;
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public Builder setContractBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contract_ = value;
onChanged();
return this;
}
private long codeId_ ;
/**
*
* CodeID references the new WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public long getCodeId() {
return codeId_;
}
/**
*
* CodeID references the new WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public Builder setCodeId(long value) {
codeId_ = value;
onChanged();
return this;
}
/**
*
* CodeID references the new WASM code
*
*
* uint64 code_id = 5 [(.gogoproto.customname) = "CodeID"];
*/
public Builder clearCodeId() {
codeId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString migrateMsg_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* MigrateMsg json encoded message to be passed to the contract on migration
*
*
* bytes migrate_msg = 6 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public com.google.protobuf.ByteString getMigrateMsg() {
return migrateMsg_;
}
/**
*
* MigrateMsg json encoded message to be passed to the contract on migration
*
*
* bytes migrate_msg = 6 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public Builder setMigrateMsg(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
migrateMsg_ = value;
onChanged();
return this;
}
/**
*
* MigrateMsg json encoded message to be passed to the contract on migration
*
*
* bytes migrate_msg = 6 [(.gogoproto.casttype) = "encoding/json.RawMessage"];
*/
public Builder clearMigrateMsg() {
migrateMsg_ = getDefaultInstance().getMigrateMsg();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.MigrateContractProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.MigrateContractProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MigrateContractProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MigrateContractProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.MigrateContractProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UpdateAdminProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.UpdateAdminProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
java.lang.String getNewAdmin();
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
com.google.protobuf.ByteString
getNewAdminBytes();
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
java.lang.String getContract();
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
com.google.protobuf.ByteString
getContractBytes();
}
/**
*
* UpdateAdminProposal gov proposal content type to set an admin for a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.UpdateAdminProposal}
*/
public static final class UpdateAdminProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.UpdateAdminProposal)
UpdateAdminProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use UpdateAdminProposal.newBuilder() to construct.
private UpdateAdminProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UpdateAdminProposal() {
title_ = "";
description_ = "";
newAdmin_ = "";
contract_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UpdateAdminProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
newAdmin_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
contract_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.class, cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.Builder.class);
}
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NEW_ADMIN_FIELD_NUMBER = 3;
private volatile java.lang.Object newAdmin_;
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public java.lang.String getNewAdmin() {
java.lang.Object ref = newAdmin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
newAdmin_ = s;
return s;
}
}
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public com.google.protobuf.ByteString
getNewAdminBytes() {
java.lang.Object ref = newAdmin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
newAdmin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTRACT_FIELD_NUMBER = 4;
private volatile java.lang.Object contract_;
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public java.lang.String getContract() {
java.lang.Object ref = contract_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contract_ = s;
return s;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public com.google.protobuf.ByteString
getContractBytes() {
java.lang.Object ref = contract_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contract_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getNewAdminBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, newAdmin_);
}
if (!getContractBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, contract_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getNewAdminBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, newAdmin_);
}
if (!getContractBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, contract_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal other = (cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getNewAdmin()
.equals(other.getNewAdmin());
result = result && getContract()
.equals(other.getContract());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + NEW_ADMIN_FIELD_NUMBER;
hash = (53 * hash) + getNewAdmin().hashCode();
hash = (37 * hash) + CONTRACT_FIELD_NUMBER;
hash = (53 * hash) + getContract().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* UpdateAdminProposal gov proposal content type to set an admin for a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.UpdateAdminProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.UpdateAdminProposal)
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.class, cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
newAdmin_ = "";
contract_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal build() {
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal result = new cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal(this);
result.title_ = title_;
result.description_ = description_;
result.newAdmin_ = newAdmin_;
result.contract_ = contract_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getNewAdmin().isEmpty()) {
newAdmin_ = other.newAdmin_;
onChanged();
}
if (!other.getContract().isEmpty()) {
contract_ = other.contract_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object newAdmin_ = "";
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public java.lang.String getNewAdmin() {
java.lang.Object ref = newAdmin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
newAdmin_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public com.google.protobuf.ByteString
getNewAdminBytes() {
java.lang.Object ref = newAdmin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
newAdmin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public Builder setNewAdmin(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
newAdmin_ = value;
onChanged();
return this;
}
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public Builder clearNewAdmin() {
newAdmin_ = getDefaultInstance().getNewAdmin();
onChanged();
return this;
}
/**
*
* NewAdmin address to be set
*
*
* string new_admin = 3 [(.gogoproto.moretags) = "yaml:\"new_admin\""];
*/
public Builder setNewAdminBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
newAdmin_ = value;
onChanged();
return this;
}
private java.lang.Object contract_ = "";
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public java.lang.String getContract() {
java.lang.Object ref = contract_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contract_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public com.google.protobuf.ByteString
getContractBytes() {
java.lang.Object ref = contract_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contract_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public Builder setContract(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contract_ = value;
onChanged();
return this;
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public Builder clearContract() {
contract_ = getDefaultInstance().getContract();
onChanged();
return this;
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 4;
*/
public Builder setContractBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contract_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.UpdateAdminProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.UpdateAdminProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UpdateAdminProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UpdateAdminProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UpdateAdminProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ClearAdminProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.ClearAdminProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
java.lang.String getContract();
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
com.google.protobuf.ByteString
getContractBytes();
}
/**
*
* ClearAdminProposal gov proposal content type to clear the admin of a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.ClearAdminProposal}
*/
public static final class ClearAdminProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.ClearAdminProposal)
ClearAdminProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use ClearAdminProposal.newBuilder() to construct.
private ClearAdminProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ClearAdminProposal() {
title_ = "";
description_ = "";
contract_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ClearAdminProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
contract_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.class, cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.Builder.class);
}
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTRACT_FIELD_NUMBER = 3;
private volatile java.lang.Object contract_;
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public java.lang.String getContract() {
java.lang.Object ref = contract_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contract_ = s;
return s;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public com.google.protobuf.ByteString
getContractBytes() {
java.lang.Object ref = contract_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contract_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (!getContractBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, contract_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
if (!getContractBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, contract_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal other = (cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getContract()
.equals(other.getContract());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + CONTRACT_FIELD_NUMBER;
hash = (53 * hash) + getContract().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* ClearAdminProposal gov proposal content type to clear the admin of a contract.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.ClearAdminProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.ClearAdminProposal)
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.class, cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
contract_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal build() {
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal result = new cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal(this);
result.title_ = title_;
result.description_ = description_;
result.contract_ = contract_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.getContract().isEmpty()) {
contract_ = other.contract_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1;
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.lang.Object contract_ = "";
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public java.lang.String getContract() {
java.lang.Object ref = contract_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contract_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public com.google.protobuf.ByteString
getContractBytes() {
java.lang.Object ref = contract_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contract_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public Builder setContract(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contract_ = value;
onChanged();
return this;
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public Builder clearContract() {
contract_ = getDefaultInstance().getContract();
onChanged();
return this;
}
/**
*
* Contract is the address of the smart contract
*
*
* string contract = 3;
*/
public Builder setContractBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contract_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.ClearAdminProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.ClearAdminProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ClearAdminProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ClearAdminProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.ClearAdminProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PinCodesProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.PinCodesProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
java.util.List getCodeIdsList();
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
int getCodeIdsCount();
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
long getCodeIds(int index);
}
/**
*
* PinCodesProposal gov proposal content type to pin a set of code ids in the wasmvm cache.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.PinCodesProposal}
*/
public static final class PinCodesProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.PinCodesProposal)
PinCodesProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use PinCodesProposal.newBuilder() to construct.
private PinCodesProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PinCodesProposal() {
title_ = "";
description_ = "";
codeIds_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PinCodesProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
codeIds_.add(input.readUInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
codeIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
codeIds_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = java.util.Collections.unmodifiableList(codeIds_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.class, cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.Builder.class);
}
private int bitField0_;
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CODE_IDS_FIELD_NUMBER = 3;
private java.util.List codeIds_;
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public java.util.List
getCodeIdsList() {
return codeIds_;
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public int getCodeIdsCount() {
return codeIds_.size();
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public long getCodeIds(int index) {
return codeIds_.get(index);
}
private int codeIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (getCodeIdsList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(codeIdsMemoizedSerializedSize);
}
for (int i = 0; i < codeIds_.size(); i++) {
output.writeUInt64NoTag(codeIds_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
{
int dataSize = 0;
for (int i = 0; i < codeIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(codeIds_.get(i));
}
size += dataSize;
if (!getCodeIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
codeIdsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal other = (cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getCodeIdsList()
.equals(other.getCodeIdsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
if (getCodeIdsCount() > 0) {
hash = (37 * hash) + CODE_IDS_FIELD_NUMBER;
hash = (53 * hash) + getCodeIdsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* PinCodesProposal gov proposal content type to pin a set of code ids in the wasmvm cache.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.PinCodesProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.PinCodesProposal)
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.class, cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
codeIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal build() {
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal result = new cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.title_ = title_;
result.description_ = description_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = java.util.Collections.unmodifiableList(codeIds_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.codeIds_ = codeIds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.codeIds_.isEmpty()) {
if (codeIds_.isEmpty()) {
codeIds_ = other.codeIds_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureCodeIdsIsMutable();
codeIds_.addAll(other.codeIds_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.util.List codeIds_ = java.util.Collections.emptyList();
private void ensureCodeIdsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = new java.util.ArrayList(codeIds_);
bitField0_ |= 0x00000004;
}
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public java.util.List
getCodeIdsList() {
return java.util.Collections.unmodifiableList(codeIds_);
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public int getCodeIdsCount() {
return codeIds_.size();
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public long getCodeIds(int index) {
return codeIds_.get(index);
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder setCodeIds(
int index, long value) {
ensureCodeIdsIsMutable();
codeIds_.set(index, value);
onChanged();
return this;
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder addCodeIds(long value) {
ensureCodeIdsIsMutable();
codeIds_.add(value);
onChanged();
return this;
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder addAllCodeIds(
java.lang.Iterable extends java.lang.Long> values) {
ensureCodeIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, codeIds_);
onChanged();
return this;
}
/**
*
* CodeIDs references the new WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder clearCodeIds() {
codeIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.PinCodesProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.PinCodesProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PinCodesProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PinCodesProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.PinCodesProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnpinCodesProposalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cosmwasm.wasm.v1beta1.UnpinCodesProposal)
com.google.protobuf.MessageOrBuilder {
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
java.lang.String getTitle();
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
java.lang.String getDescription();
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
java.util.List getCodeIdsList();
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
int getCodeIdsCount();
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
long getCodeIds(int index);
}
/**
*
* UnpinCodesProposal gov proposal content type to unpin a set of code ids in the wasmvm cache.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.UnpinCodesProposal}
*/
public static final class UnpinCodesProposal extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cosmwasm.wasm.v1beta1.UnpinCodesProposal)
UnpinCodesProposalOrBuilder {
private static final long serialVersionUID = 0L;
// Use UnpinCodesProposal.newBuilder() to construct.
private UnpinCodesProposal(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UnpinCodesProposal() {
title_ = "";
description_ = "";
codeIds_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UnpinCodesProposal(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
codeIds_.add(input.readUInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
codeIds_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
codeIds_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = java.util.Collections.unmodifiableList(codeIds_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.class, cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.Builder.class);
}
private int bitField0_;
public static final int TITLE_FIELD_NUMBER = 1;
private volatile java.lang.Object title_;
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
private volatile java.lang.Object description_;
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CODE_IDS_FIELD_NUMBER = 3;
private java.util.List codeIds_;
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public java.util.List
getCodeIdsList() {
return codeIds_;
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public int getCodeIdsCount() {
return codeIds_.size();
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public long getCodeIds(int index) {
return codeIds_.get(index);
}
private int codeIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getTitleBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
if (getCodeIdsList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(codeIdsMemoizedSerializedSize);
}
for (int i = 0; i < codeIds_.size(); i++) {
output.writeUInt64NoTag(codeIds_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTitleBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, title_);
}
if (!getDescriptionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
{
int dataSize = 0;
for (int i = 0; i < codeIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(codeIds_.get(i));
}
size += dataSize;
if (!getCodeIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
codeIdsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal)) {
return super.equals(obj);
}
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal other = (cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal) obj;
boolean result = true;
result = result && getTitle()
.equals(other.getTitle());
result = result && getDescription()
.equals(other.getDescription());
result = result && getCodeIdsList()
.equals(other.getCodeIdsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
if (getCodeIdsCount() > 0) {
hash = (37 * hash) + CODE_IDS_FIELD_NUMBER;
hash = (53 * hash) + getCodeIdsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* UnpinCodesProposal gov proposal content type to unpin a set of code ids in the wasmvm cache.
*
*
* Protobuf type {@code cosmwasm.wasm.v1beta1.UnpinCodesProposal}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cosmwasm.wasm.v1beta1.UnpinCodesProposal)
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.class, cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.Builder.class);
}
// Construct using cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
title_ = "";
description_ = "";
codeIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cosmwasm.wasm.v1beta1.Proposal.internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_descriptor;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal getDefaultInstanceForType() {
return cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.getDefaultInstance();
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal build() {
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal buildPartial() {
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal result = new cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.title_ = title_;
result.description_ = description_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = java.util.Collections.unmodifiableList(codeIds_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.codeIds_ = codeIds_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal) {
return mergeFrom((cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal other) {
if (other == cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal.getDefaultInstance()) return this;
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (!other.codeIds_.isEmpty()) {
if (codeIds_.isEmpty()) {
codeIds_ = other.codeIds_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureCodeIdsIsMutable();
codeIds_.addAll(other.codeIds_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object title_ = "";
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* Title is a short summary
*
*
* string title = 1 [(.gogoproto.moretags) = "yaml:\"title\""];
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Description is a human readable text
*
*
* string description = 2 [(.gogoproto.moretags) = "yaml:\"description\""];
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private java.util.List codeIds_ = java.util.Collections.emptyList();
private void ensureCodeIdsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
codeIds_ = new java.util.ArrayList(codeIds_);
bitField0_ |= 0x00000004;
}
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public java.util.List
getCodeIdsList() {
return java.util.Collections.unmodifiableList(codeIds_);
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public int getCodeIdsCount() {
return codeIds_.size();
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public long getCodeIds(int index) {
return codeIds_.get(index);
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder setCodeIds(
int index, long value) {
ensureCodeIdsIsMutable();
codeIds_.set(index, value);
onChanged();
return this;
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder addCodeIds(long value) {
ensureCodeIdsIsMutable();
codeIds_.add(value);
onChanged();
return this;
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder addAllCodeIds(
java.lang.Iterable extends java.lang.Long> values) {
ensureCodeIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, codeIds_);
onChanged();
return this;
}
/**
*
* CodeIDs references the WASM codes
*
*
* repeated uint64 code_ids = 3 [(.gogoproto.customname) = "CodeIDs", (.gogoproto.moretags) = "yaml:\"code_ids\""];
*/
public Builder clearCodeIds() {
codeIds_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cosmwasm.wasm.v1beta1.UnpinCodesProposal)
}
// @@protoc_insertion_point(class_scope:cosmwasm.wasm.v1beta1.UnpinCodesProposal)
private static final cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal();
}
public static cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UnpinCodesProposal parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UnpinCodesProposal(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cosmwasm.wasm.v1beta1.Proposal.UnpinCodesProposal getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n$x/wasm/internal/types/proposal.proto\022\025" +
"cosmwasm.wasm.v1beta1\032\024gogoproto/gogo.pr" +
"oto\032\036cosmos/base/v1beta1/coin.proto\032!x/w" +
"asm/internal/types/types.proto\"\327\001\n\021Store" +
"CodeProposal\022\r\n\005title\030\001 \001(\t\022\023\n\013descripti" +
"on\030\002 \001(\t\022\016\n\006run_as\030\003 \001(\t\022(\n\016wasm_byte_co" +
"de\030\004 \001(\014B\020\342\336\037\014WASMByteCode\022\016\n\006source\030\005 \001" +
"(\t\022\017\n\007builder\030\006 \001(\t\022C\n\026instantiate_permi" +
"ssion\030\007 \001(\0132#.cosmwasm.wasm.v1beta1.Acce" +
"ssConfig\"\230\002\n\033InstantiateContractProposal" +
"\022\r\n\005title\030\001 \001(\t\022\023\n\013description\030\002 \001(\t\022\016\n\006" +
"run_as\030\003 \001(\t\022\r\n\005admin\030\004 \001(\t\022\033\n\007code_id\030\005" +
" \001(\004B\n\342\336\037\006CodeID\022\r\n\005label\030\006 \001(\t\022.\n\010init_" +
"msg\030\007 \001(\014B\034\372\336\037\030encoding/json.RawMessage\022" +
"Z\n\005funds\030\010 \003(\0132\031.cosmos.base.v1beta1.Coi" +
"nB0\310\336\037\000\252\337\037(github.com/cosmos/cosmos-sdk/" +
"types.Coins\"\257\001\n\027MigrateContractProposal\022" +
"\r\n\005title\030\001 \001(\t\022\023\n\013description\030\002 \001(\t\022\016\n\006r" +
"un_as\030\003 \001(\t\022\020\n\010contract\030\004 \001(\t\022\033\n\007code_id" +
"\030\005 \001(\004B\n\342\336\037\006CodeID\0221\n\013migrate_msg\030\006 \001(\014B" +
"\034\372\336\037\030encoding/json.RawMessage\"t\n\023UpdateA" +
"dminProposal\022\r\n\005title\030\001 \001(\t\022\023\n\013descripti" +
"on\030\002 \001(\t\022\'\n\tnew_admin\030\003 \001(\tB\024\362\336\037\020yaml:\"n" +
"ew_admin\"\022\020\n\010contract\030\004 \001(\t\"J\n\022ClearAdmi" +
"nProposal\022\r\n\005title\030\001 \001(\t\022\023\n\013description\030" +
"\002 \001(\t\022\020\n\010contract\030\003 \001(\t\"\222\001\n\020PinCodesProp" +
"osal\022\037\n\005title\030\001 \001(\tB\020\362\336\037\014yaml:\"title\"\022+\n" +
"\013description\030\002 \001(\tB\026\362\336\037\022yaml:\"descriptio" +
"n\"\0220\n\010code_ids\030\003 \003(\004B\036\342\336\037\007CodeIDs\362\336\037\017yam" +
"l:\"code_ids\"\"\224\001\n\022UnpinCodesProposal\022\037\n\005t" +
"itle\030\001 \001(\tB\020\362\336\037\014yaml:\"title\"\022+\n\013descript" +
"ion\030\002 \001(\tB\026\362\336\037\022yaml:\"description\"\0220\n\010cod" +
"e_ids\030\003 \003(\004B\036\342\336\037\007CodeIDs\362\336\037\017yaml:\"code_i" +
"ds\"B>Z0github.com/CosmWasm/wasmd/x/wasmd" +
"/internal/types\330\341\036\000\310\341\036\000\250\342\036\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
cosmos.base.v1beta1.CoinOuterClass.getDescriptor(),
cosmwasm.wasm.v1beta1.Types.getDescriptor(),
}, assigner);
internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_StoreCodeProposal_descriptor,
new java.lang.String[] { "Title", "Description", "RunAs", "WasmByteCode", "Source", "Builder", "InstantiatePermission", });
internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_InstantiateContractProposal_descriptor,
new java.lang.String[] { "Title", "Description", "RunAs", "Admin", "CodeId", "Label", "InitMsg", "Funds", });
internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_MigrateContractProposal_descriptor,
new java.lang.String[] { "Title", "Description", "RunAs", "Contract", "CodeId", "MigrateMsg", });
internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_UpdateAdminProposal_descriptor,
new java.lang.String[] { "Title", "Description", "NewAdmin", "Contract", });
internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_ClearAdminProposal_descriptor,
new java.lang.String[] { "Title", "Description", "Contract", });
internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_PinCodesProposal_descriptor,
new java.lang.String[] { "Title", "Description", "CodeIds", });
internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_cosmwasm_wasm_v1beta1_UnpinCodesProposal_descriptor,
new java.lang.String[] { "Title", "Description", "CodeIds", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.castrepeated);
registry.add(com.google.protobuf.GoGoProtos.casttype);
registry.add(com.google.protobuf.GoGoProtos.customname);
registry.add(com.google.protobuf.GoGoProtos.equalAll);
registry.add(com.google.protobuf.GoGoProtos.goprotoGettersAll);
registry.add(com.google.protobuf.GoGoProtos.goprotoStringerAll);
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
cosmos.base.v1beta1.CoinOuterClass.getDescriptor();
cosmwasm.wasm.v1beta1.Types.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy