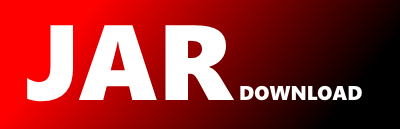
ibc.core.channel.v1.QueryOuterClass Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ibc/core/channel/v1/query.proto
package ibc.core.channel.v1;
public final class QueryOuterClass {
private QueryOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface QueryChannelRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
}
/**
*
* QueryChannelRequest is the request type for the Query/Channel RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelRequest}
*/
public static final class QueryChannelRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelRequest)
QueryChannelRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelRequest.newBuilder() to construct.
private QueryChannelRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelRequest() {
portId_ = "";
channelId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelRequest is the request type for the Query/Channel RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelRequest)
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
boolean hasChannel();
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.Channel getChannel();
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.ChannelOrBuilder getChannelOrBuilder();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryChannelResponse is the response type for the Query/Channel RPC method.
* Besides the Channel end, it includes a proof and the height from which the
* proof was retrieved.
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelResponse}
*/
public static final class QueryChannelResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelResponse)
QueryChannelResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelResponse.newBuilder() to construct.
private QueryChannelResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelResponse() {
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
ibc.core.channel.v1.ChannelOuterClass.Channel.Builder subBuilder = null;
if (channel_ != null) {
subBuilder = channel_.toBuilder();
}
channel_ = input.readMessage(ibc.core.channel.v1.ChannelOuterClass.Channel.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(channel_);
channel_ = subBuilder.buildPartial();
}
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.Builder.class);
}
public static final int CHANNEL_FIELD_NUMBER = 1;
private ibc.core.channel.v1.ChannelOuterClass.Channel channel_;
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public boolean hasChannel() {
return channel_ != null;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.Channel getChannel() {
return channel_ == null ? ibc.core.channel.v1.ChannelOuterClass.Channel.getDefaultInstance() : channel_;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.ChannelOrBuilder getChannelOrBuilder() {
return getChannel();
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (channel_ != null) {
output.writeMessage(1, getChannel());
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (channel_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getChannel());
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse) obj;
boolean result = true;
result = result && (hasChannel() == other.hasChannel());
if (hasChannel()) {
result = result && getChannel()
.equals(other.getChannel());
}
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasChannel()) {
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getChannel().hashCode();
}
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelResponse is the response type for the Query/Channel RPC method.
* Besides the Channel end, it includes a proof and the height from which the
* proof was retrieved.
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelResponse)
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (channelBuilder_ == null) {
channel_ = null;
} else {
channel_ = null;
channelBuilder_ = null;
}
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse(this);
if (channelBuilder_ == null) {
result.channel_ = channel_;
} else {
result.channel_ = channelBuilder_.build();
}
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse.getDefaultInstance()) return this;
if (other.hasChannel()) {
mergeChannel(other.getChannel());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private ibc.core.channel.v1.ChannelOuterClass.Channel channel_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.Channel, ibc.core.channel.v1.ChannelOuterClass.Channel.Builder, ibc.core.channel.v1.ChannelOuterClass.ChannelOrBuilder> channelBuilder_;
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public boolean hasChannel() {
return channelBuilder_ != null || channel_ != null;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.Channel getChannel() {
if (channelBuilder_ == null) {
return channel_ == null ? ibc.core.channel.v1.ChannelOuterClass.Channel.getDefaultInstance() : channel_;
} else {
return channelBuilder_.getMessage();
}
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public Builder setChannel(ibc.core.channel.v1.ChannelOuterClass.Channel value) {
if (channelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
channel_ = value;
onChanged();
} else {
channelBuilder_.setMessage(value);
}
return this;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public Builder setChannel(
ibc.core.channel.v1.ChannelOuterClass.Channel.Builder builderForValue) {
if (channelBuilder_ == null) {
channel_ = builderForValue.build();
onChanged();
} else {
channelBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public Builder mergeChannel(ibc.core.channel.v1.ChannelOuterClass.Channel value) {
if (channelBuilder_ == null) {
if (channel_ != null) {
channel_ =
ibc.core.channel.v1.ChannelOuterClass.Channel.newBuilder(channel_).mergeFrom(value).buildPartial();
} else {
channel_ = value;
}
onChanged();
} else {
channelBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public Builder clearChannel() {
if (channelBuilder_ == null) {
channel_ = null;
onChanged();
} else {
channel_ = null;
channelBuilder_ = null;
}
return this;
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.Channel.Builder getChannelBuilder() {
onChanged();
return getChannelFieldBuilder().getBuilder();
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.ChannelOrBuilder getChannelOrBuilder() {
if (channelBuilder_ != null) {
return channelBuilder_.getMessageOrBuilder();
} else {
return channel_ == null ?
ibc.core.channel.v1.ChannelOuterClass.Channel.getDefaultInstance() : channel_;
}
}
/**
*
* channel associated with the request identifiers
*
*
* .ibc.core.channel.v1.Channel channel = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.Channel, ibc.core.channel.v1.ChannelOuterClass.Channel.Builder, ibc.core.channel.v1.ChannelOuterClass.ChannelOrBuilder>
getChannelFieldBuilder() {
if (channelBuilder_ == null) {
channelBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.Channel, ibc.core.channel.v1.ChannelOuterClass.Channel.Builder, ibc.core.channel.v1.ChannelOuterClass.ChannelOrBuilder>(
getChannel(),
getParentForChildren(),
isClean());
channel_ = null;
}
return channelBuilder_;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
boolean hasPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
cosmos.base.query.v1beta1.Pagination.PageRequest getPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder();
}
/**
*
* QueryChannelsRequest is the request type for the Query/Channels RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelsRequest}
*/
public static final class QueryChannelsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelsRequest)
QueryChannelsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelsRequest.newBuilder() to construct.
private QueryChannelsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelsRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.Builder.class);
}
public static final int PAGINATION_FIELD_NUMBER = 1;
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
return getPagination();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (pagination_ != null) {
output.writeMessage(1, getPagination());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getPagination());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest) obj;
boolean result = true;
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelsRequest is the request type for the Query/Channels RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelsRequest)
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest(this);
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest.getDefaultInstance()) return this;
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder> paginationBuilder_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageRequest.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelsRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
java.util.List
getChannelsList();
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel getChannels(int index);
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
int getChannelsCount();
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
java.util.List extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsOrBuilderList();
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder getChannelsOrBuilder(
int index);
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
boolean hasPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponse getPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryChannelsResponse is the response type for the Query/Channels RPC method.
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelsResponse}
*/
public static final class QueryChannelsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelsResponse)
QueryChannelsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelsResponse.newBuilder() to construct.
private QueryChannelsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelsResponse() {
channels_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
channels_.add(
input.readMessage(ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.parser(), extensionRegistry));
break;
}
case 18: {
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = java.util.Collections.unmodifiableList(channels_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.Builder.class);
}
private int bitField0_;
public static final int CHANNELS_FIELD_NUMBER = 1;
private java.util.List channels_;
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List getChannelsList() {
return channels_;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsOrBuilderList() {
return channels_;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public int getChannelsCount() {
return channels_.size();
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel getChannels(int index) {
return channels_.get(index);
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder getChannelsOrBuilder(
int index) {
return channels_.get(index);
}
public static final int PAGINATION_FIELD_NUMBER = 2;
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
return getPagination();
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < channels_.size(); i++) {
output.writeMessage(1, channels_.get(i));
}
if (pagination_ != null) {
output.writeMessage(2, getPagination());
}
if (height_ != null) {
output.writeMessage(3, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < channels_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, channels_.get(i));
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagination());
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse) obj;
boolean result = true;
result = result && getChannelsList()
.equals(other.getChannelsList());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getChannelsCount() > 0) {
hash = (37 * hash) + CHANNELS_FIELD_NUMBER;
hash = (53 * hash) + getChannelsList().hashCode();
}
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelsResponse is the response type for the Query/Channels RPC method.
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelsResponse)
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChannelsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (channelsBuilder_ == null) {
channels_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
channelsBuilder_.clear();
}
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelsResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (channelsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = java.util.Collections.unmodifiableList(channels_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.channels_ = channels_;
} else {
result.channels_ = channelsBuilder_.build();
}
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse.getDefaultInstance()) return this;
if (channelsBuilder_ == null) {
if (!other.channels_.isEmpty()) {
if (channels_.isEmpty()) {
channels_ = other.channels_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureChannelsIsMutable();
channels_.addAll(other.channels_);
}
onChanged();
}
} else {
if (!other.channels_.isEmpty()) {
if (channelsBuilder_.isEmpty()) {
channelsBuilder_.dispose();
channelsBuilder_ = null;
channels_ = other.channels_;
bitField0_ = (bitField0_ & ~0x00000001);
channelsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getChannelsFieldBuilder() : null;
} else {
channelsBuilder_.addAllMessages(other.channels_);
}
}
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List channels_ =
java.util.Collections.emptyList();
private void ensureChannelsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = new java.util.ArrayList(channels_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder> channelsBuilder_;
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List getChannelsList() {
if (channelsBuilder_ == null) {
return java.util.Collections.unmodifiableList(channels_);
} else {
return channelsBuilder_.getMessageList();
}
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public int getChannelsCount() {
if (channelsBuilder_ == null) {
return channels_.size();
} else {
return channelsBuilder_.getCount();
}
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel getChannels(int index) {
if (channelsBuilder_ == null) {
return channels_.get(index);
} else {
return channelsBuilder_.getMessage(index);
}
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder setChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel value) {
if (channelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelsIsMutable();
channels_.set(index, value);
onChanged();
} else {
channelsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder setChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder builderForValue) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.set(index, builderForValue.build());
onChanged();
} else {
channelsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel value) {
if (channelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelsIsMutable();
channels_.add(value);
onChanged();
} else {
channelsBuilder_.addMessage(value);
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel value) {
if (channelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelsIsMutable();
channels_.add(index, value);
onChanged();
} else {
channelsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder builderForValue) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.add(builderForValue.build());
onChanged();
} else {
channelsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder builderForValue) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.add(index, builderForValue.build());
onChanged();
} else {
channelsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addAllChannels(
java.lang.Iterable extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel> values) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, channels_);
onChanged();
} else {
channelsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder clearChannels() {
if (channelsBuilder_ == null) {
channels_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
channelsBuilder_.clear();
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder removeChannels(int index) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.remove(index);
onChanged();
} else {
channelsBuilder_.remove(index);
}
return this;
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder getChannelsBuilder(
int index) {
return getChannelsFieldBuilder().getBuilder(index);
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder getChannelsOrBuilder(
int index) {
if (channelsBuilder_ == null) {
return channels_.get(index); } else {
return channelsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsOrBuilderList() {
if (channelsBuilder_ != null) {
return channelsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(channels_);
}
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder addChannelsBuilder() {
return getChannelsFieldBuilder().addBuilder(
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.getDefaultInstance());
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder addChannelsBuilder(
int index) {
return getChannelsFieldBuilder().addBuilder(
index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.getDefaultInstance());
}
/**
*
* list of stored channels of the chain.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List
getChannelsBuilderList() {
return getChannelsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsFieldBuilder() {
if (channelsBuilder_ == null) {
channelsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>(
channels_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
channels_ = null;
}
return channelsBuilder_;
}
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder> paginationBuilder_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageResponse.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelsResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionChannelsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryConnectionChannelsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
java.lang.String getConnection();
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
com.google.protobuf.ByteString
getConnectionBytes();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
boolean hasPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageRequest getPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder();
}
/**
*
* QueryConnectionChannelsRequest is the request type for the
* Query/QueryConnectionChannels RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryConnectionChannelsRequest}
*/
public static final class QueryConnectionChannelsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryConnectionChannelsRequest)
QueryConnectionChannelsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionChannelsRequest.newBuilder() to construct.
private QueryConnectionChannelsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionChannelsRequest() {
connection_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionChannelsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
connection_ = s;
break;
}
case 18: {
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.Builder.class);
}
public static final int CONNECTION_FIELD_NUMBER = 1;
private volatile java.lang.Object connection_;
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public java.lang.String getConnection() {
java.lang.Object ref = connection_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connection_ = s;
return s;
}
}
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public com.google.protobuf.ByteString
getConnectionBytes() {
java.lang.Object ref = connection_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connection_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGINATION_FIELD_NUMBER = 2;
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
return getPagination();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getConnectionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, connection_);
}
if (pagination_ != null) {
output.writeMessage(2, getPagination());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getConnectionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, connection_);
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagination());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest) obj;
boolean result = true;
result = result && getConnection()
.equals(other.getConnection());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONNECTION_FIELD_NUMBER;
hash = (53 * hash) + getConnection().hashCode();
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionChannelsRequest is the request type for the
* Query/QueryConnectionChannels RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryConnectionChannelsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryConnectionChannelsRequest)
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
connection_ = "";
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest(this);
result.connection_ = connection_;
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest.getDefaultInstance()) return this;
if (!other.getConnection().isEmpty()) {
connection_ = other.connection_;
onChanged();
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object connection_ = "";
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public java.lang.String getConnection() {
java.lang.Object ref = connection_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connection_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public com.google.protobuf.ByteString
getConnectionBytes() {
java.lang.Object ref = connection_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connection_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public Builder setConnection(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
connection_ = value;
onChanged();
return this;
}
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public Builder clearConnection() {
connection_ = getDefaultInstance().getConnection();
onChanged();
return this;
}
/**
*
* connection unique identifier
*
*
* string connection = 1;
*/
public Builder setConnectionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
connection_ = value;
onChanged();
return this;
}
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder> paginationBuilder_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageRequest.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryConnectionChannelsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryConnectionChannelsRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionChannelsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionChannelsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionChannelsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryConnectionChannelsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
java.util.List
getChannelsList();
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel getChannels(int index);
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
int getChannelsCount();
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
java.util.List extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsOrBuilderList();
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder getChannelsOrBuilder(
int index);
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
boolean hasPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponse getPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryConnectionChannelsResponse is the Response type for the
* Query/QueryConnectionChannels RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryConnectionChannelsResponse}
*/
public static final class QueryConnectionChannelsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryConnectionChannelsResponse)
QueryConnectionChannelsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionChannelsResponse.newBuilder() to construct.
private QueryConnectionChannelsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionChannelsResponse() {
channels_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionChannelsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
channels_.add(
input.readMessage(ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.parser(), extensionRegistry));
break;
}
case 18: {
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = java.util.Collections.unmodifiableList(channels_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.Builder.class);
}
private int bitField0_;
public static final int CHANNELS_FIELD_NUMBER = 1;
private java.util.List channels_;
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List getChannelsList() {
return channels_;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsOrBuilderList() {
return channels_;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public int getChannelsCount() {
return channels_.size();
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel getChannels(int index) {
return channels_.get(index);
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder getChannelsOrBuilder(
int index) {
return channels_.get(index);
}
public static final int PAGINATION_FIELD_NUMBER = 2;
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
return getPagination();
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < channels_.size(); i++) {
output.writeMessage(1, channels_.get(i));
}
if (pagination_ != null) {
output.writeMessage(2, getPagination());
}
if (height_ != null) {
output.writeMessage(3, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < channels_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, channels_.get(i));
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagination());
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse) obj;
boolean result = true;
result = result && getChannelsList()
.equals(other.getChannelsList());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getChannelsCount() > 0) {
hash = (37 * hash) + CHANNELS_FIELD_NUMBER;
hash = (53 * hash) + getChannelsList().hashCode();
}
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionChannelsResponse is the Response type for the
* Query/QueryConnectionChannels RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryConnectionChannelsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryConnectionChannelsResponse)
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChannelsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (channelsBuilder_ == null) {
channels_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
channelsBuilder_.clear();
}
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (channelsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = java.util.Collections.unmodifiableList(channels_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.channels_ = channels_;
} else {
result.channels_ = channelsBuilder_.build();
}
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse.getDefaultInstance()) return this;
if (channelsBuilder_ == null) {
if (!other.channels_.isEmpty()) {
if (channels_.isEmpty()) {
channels_ = other.channels_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureChannelsIsMutable();
channels_.addAll(other.channels_);
}
onChanged();
}
} else {
if (!other.channels_.isEmpty()) {
if (channelsBuilder_.isEmpty()) {
channelsBuilder_.dispose();
channelsBuilder_ = null;
channels_ = other.channels_;
bitField0_ = (bitField0_ & ~0x00000001);
channelsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getChannelsFieldBuilder() : null;
} else {
channelsBuilder_.addAllMessages(other.channels_);
}
}
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List channels_ =
java.util.Collections.emptyList();
private void ensureChannelsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
channels_ = new java.util.ArrayList(channels_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder> channelsBuilder_;
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List getChannelsList() {
if (channelsBuilder_ == null) {
return java.util.Collections.unmodifiableList(channels_);
} else {
return channelsBuilder_.getMessageList();
}
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public int getChannelsCount() {
if (channelsBuilder_ == null) {
return channels_.size();
} else {
return channelsBuilder_.getCount();
}
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel getChannels(int index) {
if (channelsBuilder_ == null) {
return channels_.get(index);
} else {
return channelsBuilder_.getMessage(index);
}
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder setChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel value) {
if (channelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelsIsMutable();
channels_.set(index, value);
onChanged();
} else {
channelsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder setChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder builderForValue) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.set(index, builderForValue.build());
onChanged();
} else {
channelsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel value) {
if (channelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelsIsMutable();
channels_.add(value);
onChanged();
} else {
channelsBuilder_.addMessage(value);
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel value) {
if (channelsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChannelsIsMutable();
channels_.add(index, value);
onChanged();
} else {
channelsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder builderForValue) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.add(builderForValue.build());
onChanged();
} else {
channelsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addChannels(
int index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder builderForValue) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.add(index, builderForValue.build());
onChanged();
} else {
channelsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder addAllChannels(
java.lang.Iterable extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel> values) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, channels_);
onChanged();
} else {
channelsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder clearChannels() {
if (channelsBuilder_ == null) {
channels_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
channelsBuilder_.clear();
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public Builder removeChannels(int index) {
if (channelsBuilder_ == null) {
ensureChannelsIsMutable();
channels_.remove(index);
onChanged();
} else {
channelsBuilder_.remove(index);
}
return this;
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder getChannelsBuilder(
int index) {
return getChannelsFieldBuilder().getBuilder(index);
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder getChannelsOrBuilder(
int index) {
if (channelsBuilder_ == null) {
return channels_.get(index); } else {
return channelsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsOrBuilderList() {
if (channelsBuilder_ != null) {
return channelsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(channels_);
}
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder addChannelsBuilder() {
return getChannelsFieldBuilder().addBuilder(
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.getDefaultInstance());
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder addChannelsBuilder(
int index) {
return getChannelsFieldBuilder().addBuilder(
index, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.getDefaultInstance());
}
/**
*
* list of channels associated with a connection.
*
*
* repeated .ibc.core.channel.v1.IdentifiedChannel channels = 1;
*/
public java.util.List
getChannelsBuilderList() {
return getChannelsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>
getChannelsFieldBuilder() {
if (channelsBuilder_ == null) {
channelsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannel.Builder, ibc.core.channel.v1.ChannelOuterClass.IdentifiedChannelOrBuilder>(
channels_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
channels_ = null;
}
return channelsBuilder_;
}
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder> paginationBuilder_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageResponse.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryConnectionChannelsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryConnectionChannelsResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionChannelsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionChannelsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryConnectionChannelsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelClientStateRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelClientStateRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
}
/**
*
* QueryChannelClientStateRequest is the request type for the Query/ClientState
* RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelClientStateRequest}
*/
public static final class QueryChannelClientStateRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelClientStateRequest)
QueryChannelClientStateRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelClientStateRequest.newBuilder() to construct.
private QueryChannelClientStateRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelClientStateRequest() {
portId_ = "";
channelId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelClientStateRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelClientStateRequest is the request type for the Query/ClientState
* RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelClientStateRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelClientStateRequest)
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelClientStateRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelClientStateRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelClientStateRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelClientStateRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelClientStateResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelClientStateResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
boolean hasIdentifiedClientState();
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
ibc.core.client.v1.Client.IdentifiedClientState getIdentifiedClientState();
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder getIdentifiedClientStateOrBuilder();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryChannelClientStateResponse is the Response type for the
* Query/QueryChannelClientState RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelClientStateResponse}
*/
public static final class QueryChannelClientStateResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelClientStateResponse)
QueryChannelClientStateResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelClientStateResponse.newBuilder() to construct.
private QueryChannelClientStateResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelClientStateResponse() {
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelClientStateResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
ibc.core.client.v1.Client.IdentifiedClientState.Builder subBuilder = null;
if (identifiedClientState_ != null) {
subBuilder = identifiedClientState_.toBuilder();
}
identifiedClientState_ = input.readMessage(ibc.core.client.v1.Client.IdentifiedClientState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifiedClientState_);
identifiedClientState_ = subBuilder.buildPartial();
}
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.Builder.class);
}
public static final int IDENTIFIED_CLIENT_STATE_FIELD_NUMBER = 1;
private ibc.core.client.v1.Client.IdentifiedClientState identifiedClientState_;
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public boolean hasIdentifiedClientState() {
return identifiedClientState_ != null;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientState getIdentifiedClientState() {
return identifiedClientState_ == null ? ibc.core.client.v1.Client.IdentifiedClientState.getDefaultInstance() : identifiedClientState_;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder getIdentifiedClientStateOrBuilder() {
return getIdentifiedClientState();
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (identifiedClientState_ != null) {
output.writeMessage(1, getIdentifiedClientState());
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (identifiedClientState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getIdentifiedClientState());
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse) obj;
boolean result = true;
result = result && (hasIdentifiedClientState() == other.hasIdentifiedClientState());
if (hasIdentifiedClientState()) {
result = result && getIdentifiedClientState()
.equals(other.getIdentifiedClientState());
}
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasIdentifiedClientState()) {
hash = (37 * hash) + IDENTIFIED_CLIENT_STATE_FIELD_NUMBER;
hash = (53 * hash) + getIdentifiedClientState().hashCode();
}
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelClientStateResponse is the Response type for the
* Query/QueryChannelClientState RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelClientStateResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelClientStateResponse)
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (identifiedClientStateBuilder_ == null) {
identifiedClientState_ = null;
} else {
identifiedClientState_ = null;
identifiedClientStateBuilder_ = null;
}
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse(this);
if (identifiedClientStateBuilder_ == null) {
result.identifiedClientState_ = identifiedClientState_;
} else {
result.identifiedClientState_ = identifiedClientStateBuilder_.build();
}
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse.getDefaultInstance()) return this;
if (other.hasIdentifiedClientState()) {
mergeIdentifiedClientState(other.getIdentifiedClientState());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private ibc.core.client.v1.Client.IdentifiedClientState identifiedClientState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.IdentifiedClientState, ibc.core.client.v1.Client.IdentifiedClientState.Builder, ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder> identifiedClientStateBuilder_;
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public boolean hasIdentifiedClientState() {
return identifiedClientStateBuilder_ != null || identifiedClientState_ != null;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientState getIdentifiedClientState() {
if (identifiedClientStateBuilder_ == null) {
return identifiedClientState_ == null ? ibc.core.client.v1.Client.IdentifiedClientState.getDefaultInstance() : identifiedClientState_;
} else {
return identifiedClientStateBuilder_.getMessage();
}
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder setIdentifiedClientState(ibc.core.client.v1.Client.IdentifiedClientState value) {
if (identifiedClientStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifiedClientState_ = value;
onChanged();
} else {
identifiedClientStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder setIdentifiedClientState(
ibc.core.client.v1.Client.IdentifiedClientState.Builder builderForValue) {
if (identifiedClientStateBuilder_ == null) {
identifiedClientState_ = builderForValue.build();
onChanged();
} else {
identifiedClientStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder mergeIdentifiedClientState(ibc.core.client.v1.Client.IdentifiedClientState value) {
if (identifiedClientStateBuilder_ == null) {
if (identifiedClientState_ != null) {
identifiedClientState_ =
ibc.core.client.v1.Client.IdentifiedClientState.newBuilder(identifiedClientState_).mergeFrom(value).buildPartial();
} else {
identifiedClientState_ = value;
}
onChanged();
} else {
identifiedClientStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder clearIdentifiedClientState() {
if (identifiedClientStateBuilder_ == null) {
identifiedClientState_ = null;
onChanged();
} else {
identifiedClientState_ = null;
identifiedClientStateBuilder_ = null;
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientState.Builder getIdentifiedClientStateBuilder() {
onChanged();
return getIdentifiedClientStateFieldBuilder().getBuilder();
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder getIdentifiedClientStateOrBuilder() {
if (identifiedClientStateBuilder_ != null) {
return identifiedClientStateBuilder_.getMessageOrBuilder();
} else {
return identifiedClientState_ == null ?
ibc.core.client.v1.Client.IdentifiedClientState.getDefaultInstance() : identifiedClientState_;
}
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.IdentifiedClientState, ibc.core.client.v1.Client.IdentifiedClientState.Builder, ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder>
getIdentifiedClientStateFieldBuilder() {
if (identifiedClientStateBuilder_ == null) {
identifiedClientStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.IdentifiedClientState, ibc.core.client.v1.Client.IdentifiedClientState.Builder, ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder>(
getIdentifiedClientState(),
getParentForChildren(),
isClean());
identifiedClientState_ = null;
}
return identifiedClientStateBuilder_;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelClientStateResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelClientStateResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelClientStateResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelClientStateResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelClientStateResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelConsensusStateRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelConsensusStateRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* revision number of the consensus state
*
*
* uint64 revision_number = 3;
*/
long getRevisionNumber();
/**
*
* revision height of the consensus state
*
*
* uint64 revision_height = 4;
*/
long getRevisionHeight();
}
/**
*
* QueryChannelConsensusStateRequest is the request type for the
* Query/ConsensusState RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelConsensusStateRequest}
*/
public static final class QueryChannelConsensusStateRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelConsensusStateRequest)
QueryChannelConsensusStateRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelConsensusStateRequest.newBuilder() to construct.
private QueryChannelConsensusStateRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelConsensusStateRequest() {
portId_ = "";
channelId_ = "";
revisionNumber_ = 0L;
revisionHeight_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelConsensusStateRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 24: {
revisionNumber_ = input.readUInt64();
break;
}
case 32: {
revisionHeight_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REVISION_NUMBER_FIELD_NUMBER = 3;
private long revisionNumber_;
/**
*
* revision number of the consensus state
*
*
* uint64 revision_number = 3;
*/
public long getRevisionNumber() {
return revisionNumber_;
}
public static final int REVISION_HEIGHT_FIELD_NUMBER = 4;
private long revisionHeight_;
/**
*
* revision height of the consensus state
*
*
* uint64 revision_height = 4;
*/
public long getRevisionHeight() {
return revisionHeight_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (revisionNumber_ != 0L) {
output.writeUInt64(3, revisionNumber_);
}
if (revisionHeight_ != 0L) {
output.writeUInt64(4, revisionHeight_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
if (revisionNumber_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, revisionNumber_);
}
if (revisionHeight_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, revisionHeight_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && (getRevisionNumber()
== other.getRevisionNumber());
result = result && (getRevisionHeight()
== other.getRevisionHeight());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (37 * hash) + REVISION_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRevisionNumber());
hash = (37 * hash) + REVISION_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRevisionHeight());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelConsensusStateRequest is the request type for the
* Query/ConsensusState RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelConsensusStateRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelConsensusStateRequest)
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
revisionNumber_ = 0L;
revisionHeight_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
result.revisionNumber_ = revisionNumber_;
result.revisionHeight_ = revisionHeight_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (other.getRevisionNumber() != 0L) {
setRevisionNumber(other.getRevisionNumber());
}
if (other.getRevisionHeight() != 0L) {
setRevisionHeight(other.getRevisionHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private long revisionNumber_ ;
/**
*
* revision number of the consensus state
*
*
* uint64 revision_number = 3;
*/
public long getRevisionNumber() {
return revisionNumber_;
}
/**
*
* revision number of the consensus state
*
*
* uint64 revision_number = 3;
*/
public Builder setRevisionNumber(long value) {
revisionNumber_ = value;
onChanged();
return this;
}
/**
*
* revision number of the consensus state
*
*
* uint64 revision_number = 3;
*/
public Builder clearRevisionNumber() {
revisionNumber_ = 0L;
onChanged();
return this;
}
private long revisionHeight_ ;
/**
*
* revision height of the consensus state
*
*
* uint64 revision_height = 4;
*/
public long getRevisionHeight() {
return revisionHeight_;
}
/**
*
* revision height of the consensus state
*
*
* uint64 revision_height = 4;
*/
public Builder setRevisionHeight(long value) {
revisionHeight_ = value;
onChanged();
return this;
}
/**
*
* revision height of the consensus state
*
*
* uint64 revision_height = 4;
*/
public Builder clearRevisionHeight() {
revisionHeight_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelConsensusStateRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelConsensusStateRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelConsensusStateRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelConsensusStateRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryChannelConsensusStateResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryChannelConsensusStateResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
boolean hasConsensusState();
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
com.google.protobuf.Any getConsensusState();
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder();
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
java.lang.String getClientId();
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryChannelClientStateResponse is the Response type for the
* Query/QueryChannelClientState RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelConsensusStateResponse}
*/
public static final class QueryChannelConsensusStateResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryChannelConsensusStateResponse)
QueryChannelConsensusStateResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryChannelConsensusStateResponse.newBuilder() to construct.
private QueryChannelConsensusStateResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryChannelConsensusStateResponse() {
clientId_ = "";
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryChannelConsensusStateResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Any.Builder subBuilder = null;
if (consensusState_ != null) {
subBuilder = consensusState_.toBuilder();
}
consensusState_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(consensusState_);
consensusState_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
case 26: {
proof_ = input.readBytes();
break;
}
case 34: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.Builder.class);
}
public static final int CONSENSUS_STATE_FIELD_NUMBER = 1;
private com.google.protobuf.Any consensusState_;
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public boolean hasConsensusState() {
return consensusState_ != null;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.Any getConsensusState() {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
return getConsensusState();
}
public static final int CLIENT_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object clientId_;
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROOF_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 4;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consensusState_ != null) {
output.writeMessage(1, getConsensusState());
}
if (!getClientIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, clientId_);
}
if (!proof_.isEmpty()) {
output.writeBytes(3, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(4, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consensusState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsensusState());
}
if (!getClientIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, clientId_);
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse) obj;
boolean result = true;
result = result && (hasConsensusState() == other.hasConsensusState());
if (hasConsensusState()) {
result = result && getConsensusState()
.equals(other.getConsensusState());
}
result = result && getClientId()
.equals(other.getClientId());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsensusState()) {
hash = (37 * hash) + CONSENSUS_STATE_FIELD_NUMBER;
hash = (53 * hash) + getConsensusState().hashCode();
}
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryChannelClientStateResponse is the Response type for the
* Query/QueryChannelClientState RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryChannelConsensusStateResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryChannelConsensusStateResponse)
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (consensusStateBuilder_ == null) {
consensusState_ = null;
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
clientId_ = "";
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse(this);
if (consensusStateBuilder_ == null) {
result.consensusState_ = consensusState_;
} else {
result.consensusState_ = consensusStateBuilder_.build();
}
result.clientId_ = clientId_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse.getDefaultInstance()) return this;
if (other.hasConsensusState()) {
mergeConsensusState(other.getConsensusState());
}
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.Any consensusState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> consensusStateBuilder_;
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public boolean hasConsensusState() {
return consensusStateBuilder_ != null || consensusState_ != null;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.Any getConsensusState() {
if (consensusStateBuilder_ == null) {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
} else {
return consensusStateBuilder_.getMessage();
}
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder setConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consensusState_ = value;
onChanged();
} else {
consensusStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder setConsensusState(
com.google.protobuf.Any.Builder builderForValue) {
if (consensusStateBuilder_ == null) {
consensusState_ = builderForValue.build();
onChanged();
} else {
consensusStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder mergeConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (consensusState_ != null) {
consensusState_ =
com.google.protobuf.Any.newBuilder(consensusState_).mergeFrom(value).buildPartial();
} else {
consensusState_ = value;
}
onChanged();
} else {
consensusStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder clearConsensusState() {
if (consensusStateBuilder_ == null) {
consensusState_ = null;
onChanged();
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.Any.Builder getConsensusStateBuilder() {
onChanged();
return getConsensusStateFieldBuilder().getBuilder();
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
if (consensusStateBuilder_ != null) {
return consensusStateBuilder_.getMessageOrBuilder();
} else {
return consensusState_ == null ?
com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getConsensusStateFieldBuilder() {
if (consensusStateBuilder_ == null) {
consensusStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getConsensusState(),
getParentForChildren(),
isClean());
consensusState_ = null;
}
return consensusStateBuilder_;
}
private java.lang.Object clientId_ = "";
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryChannelConsensusStateResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryChannelConsensusStateResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryChannelConsensusStateResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryChannelConsensusStateResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryChannelConsensusStateResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketCommitmentRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketCommitmentRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
long getSequence();
}
/**
*
* QueryPacketCommitmentRequest is the request type for the
* Query/PacketCommitment RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentRequest}
*/
public static final class QueryPacketCommitmentRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketCommitmentRequest)
QueryPacketCommitmentRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketCommitmentRequest.newBuilder() to construct.
private QueryPacketCommitmentRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketCommitmentRequest() {
portId_ = "";
channelId_ = "";
sequence_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketCommitmentRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 24: {
sequence_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEQUENCE_FIELD_NUMBER = 3;
private long sequence_;
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public long getSequence() {
return sequence_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (sequence_ != 0L) {
output.writeUInt64(3, sequence_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
if (sequence_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, sequence_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && (getSequence()
== other.getSequence());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (37 * hash) + SEQUENCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSequence());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketCommitmentRequest is the request type for the
* Query/PacketCommitment RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketCommitmentRequest)
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
sequence_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
result.sequence_ = sequence_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (other.getSequence() != 0L) {
setSequence(other.getSequence());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private long sequence_ ;
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public long getSequence() {
return sequence_;
}
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public Builder setSequence(long value) {
sequence_ = value;
onChanged();
return this;
}
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public Builder clearSequence() {
sequence_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketCommitmentRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketCommitmentRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketCommitmentRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketCommitmentRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketCommitmentResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketCommitmentResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* packet associated with the request fields
*
*
* bytes commitment = 1;
*/
com.google.protobuf.ByteString getCommitment();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryPacketCommitmentResponse defines the client query response for a packet
* which also includes a proof and the height from which the proof was
* retrieved
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentResponse}
*/
public static final class QueryPacketCommitmentResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketCommitmentResponse)
QueryPacketCommitmentResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketCommitmentResponse.newBuilder() to construct.
private QueryPacketCommitmentResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketCommitmentResponse() {
commitment_ = com.google.protobuf.ByteString.EMPTY;
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketCommitmentResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
commitment_ = input.readBytes();
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.Builder.class);
}
public static final int COMMITMENT_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString commitment_;
/**
*
* packet associated with the request fields
*
*
* bytes commitment = 1;
*/
public com.google.protobuf.ByteString getCommitment() {
return commitment_;
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!commitment_.isEmpty()) {
output.writeBytes(1, commitment_);
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!commitment_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, commitment_);
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse) obj;
boolean result = true;
result = result && getCommitment()
.equals(other.getCommitment());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COMMITMENT_FIELD_NUMBER;
hash = (53 * hash) + getCommitment().hashCode();
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketCommitmentResponse defines the client query response for a packet
* which also includes a proof and the height from which the proof was
* retrieved
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketCommitmentResponse)
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
commitment_ = com.google.protobuf.ByteString.EMPTY;
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse(this);
result.commitment_ = commitment_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse.getDefaultInstance()) return this;
if (other.getCommitment() != com.google.protobuf.ByteString.EMPTY) {
setCommitment(other.getCommitment());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString commitment_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* packet associated with the request fields
*
*
* bytes commitment = 1;
*/
public com.google.protobuf.ByteString getCommitment() {
return commitment_;
}
/**
*
* packet associated with the request fields
*
*
* bytes commitment = 1;
*/
public Builder setCommitment(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
commitment_ = value;
onChanged();
return this;
}
/**
*
* packet associated with the request fields
*
*
* bytes commitment = 1;
*/
public Builder clearCommitment() {
commitment_ = getDefaultInstance().getCommitment();
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketCommitmentResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketCommitmentResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketCommitmentResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketCommitmentResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketCommitmentsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketCommitmentsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
boolean hasPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
cosmos.base.query.v1beta1.Pagination.PageRequest getPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder();
}
/**
*
* QueryPacketCommitmentsRequest is the request type for the
* Query/QueryPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentsRequest}
*/
public static final class QueryPacketCommitmentsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketCommitmentsRequest)
QueryPacketCommitmentsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketCommitmentsRequest.newBuilder() to construct.
private QueryPacketCommitmentsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketCommitmentsRequest() {
portId_ = "";
channelId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketCommitmentsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 26: {
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGINATION_FIELD_NUMBER = 3;
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
return getPagination();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (pagination_ != null) {
output.writeMessage(3, getPagination());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPagination());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketCommitmentsRequest is the request type for the
* Query/QueryPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketCommitmentsRequest)
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder> paginationBuilder_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageRequest.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketCommitmentsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketCommitmentsRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketCommitmentsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketCommitmentsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketCommitmentsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketCommitmentsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
java.util.List
getCommitmentsList();
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.PacketState getCommitments(int index);
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
int getCommitmentsCount();
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
java.util.List extends ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getCommitmentsOrBuilderList();
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder getCommitmentsOrBuilder(
int index);
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
boolean hasPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponse getPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryPacketCommitmentsResponse is the request type for the
* Query/QueryPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentsResponse}
*/
public static final class QueryPacketCommitmentsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketCommitmentsResponse)
QueryPacketCommitmentsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketCommitmentsResponse.newBuilder() to construct.
private QueryPacketCommitmentsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketCommitmentsResponse() {
commitments_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketCommitmentsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
commitments_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
commitments_.add(
input.readMessage(ibc.core.channel.v1.ChannelOuterClass.PacketState.parser(), extensionRegistry));
break;
}
case 18: {
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
commitments_ = java.util.Collections.unmodifiableList(commitments_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.Builder.class);
}
private int bitField0_;
public static final int COMMITMENTS_FIELD_NUMBER = 1;
private java.util.List commitments_;
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public java.util.List getCommitmentsList() {
return commitments_;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getCommitmentsOrBuilderList() {
return commitments_;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public int getCommitmentsCount() {
return commitments_.size();
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState getCommitments(int index) {
return commitments_.get(index);
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder getCommitmentsOrBuilder(
int index) {
return commitments_.get(index);
}
public static final int PAGINATION_FIELD_NUMBER = 2;
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
return getPagination();
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < commitments_.size(); i++) {
output.writeMessage(1, commitments_.get(i));
}
if (pagination_ != null) {
output.writeMessage(2, getPagination());
}
if (height_ != null) {
output.writeMessage(3, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < commitments_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, commitments_.get(i));
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagination());
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse) obj;
boolean result = true;
result = result && getCommitmentsList()
.equals(other.getCommitmentsList());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getCommitmentsCount() > 0) {
hash = (37 * hash) + COMMITMENTS_FIELD_NUMBER;
hash = (53 * hash) + getCommitmentsList().hashCode();
}
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketCommitmentsResponse is the request type for the
* Query/QueryPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketCommitmentsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketCommitmentsResponse)
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getCommitmentsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (commitmentsBuilder_ == null) {
commitments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
commitmentsBuilder_.clear();
}
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (commitmentsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
commitments_ = java.util.Collections.unmodifiableList(commitments_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.commitments_ = commitments_;
} else {
result.commitments_ = commitmentsBuilder_.build();
}
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse.getDefaultInstance()) return this;
if (commitmentsBuilder_ == null) {
if (!other.commitments_.isEmpty()) {
if (commitments_.isEmpty()) {
commitments_ = other.commitments_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureCommitmentsIsMutable();
commitments_.addAll(other.commitments_);
}
onChanged();
}
} else {
if (!other.commitments_.isEmpty()) {
if (commitmentsBuilder_.isEmpty()) {
commitmentsBuilder_.dispose();
commitmentsBuilder_ = null;
commitments_ = other.commitments_;
bitField0_ = (bitField0_ & ~0x00000001);
commitmentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCommitmentsFieldBuilder() : null;
} else {
commitmentsBuilder_.addAllMessages(other.commitments_);
}
}
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List commitments_ =
java.util.Collections.emptyList();
private void ensureCommitmentsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
commitments_ = new java.util.ArrayList(commitments_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.PacketState, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder, ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder> commitmentsBuilder_;
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public java.util.List getCommitmentsList() {
if (commitmentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(commitments_);
} else {
return commitmentsBuilder_.getMessageList();
}
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public int getCommitmentsCount() {
if (commitmentsBuilder_ == null) {
return commitments_.size();
} else {
return commitmentsBuilder_.getCount();
}
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState getCommitments(int index) {
if (commitmentsBuilder_ == null) {
return commitments_.get(index);
} else {
return commitmentsBuilder_.getMessage(index);
}
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder setCommitments(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState value) {
if (commitmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommitmentsIsMutable();
commitments_.set(index, value);
onChanged();
} else {
commitmentsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder setCommitments(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder builderForValue) {
if (commitmentsBuilder_ == null) {
ensureCommitmentsIsMutable();
commitments_.set(index, builderForValue.build());
onChanged();
} else {
commitmentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder addCommitments(ibc.core.channel.v1.ChannelOuterClass.PacketState value) {
if (commitmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommitmentsIsMutable();
commitments_.add(value);
onChanged();
} else {
commitmentsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder addCommitments(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState value) {
if (commitmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCommitmentsIsMutable();
commitments_.add(index, value);
onChanged();
} else {
commitmentsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder addCommitments(
ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder builderForValue) {
if (commitmentsBuilder_ == null) {
ensureCommitmentsIsMutable();
commitments_.add(builderForValue.build());
onChanged();
} else {
commitmentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder addCommitments(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder builderForValue) {
if (commitmentsBuilder_ == null) {
ensureCommitmentsIsMutable();
commitments_.add(index, builderForValue.build());
onChanged();
} else {
commitmentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder addAllCommitments(
java.lang.Iterable extends ibc.core.channel.v1.ChannelOuterClass.PacketState> values) {
if (commitmentsBuilder_ == null) {
ensureCommitmentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, commitments_);
onChanged();
} else {
commitmentsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder clearCommitments() {
if (commitmentsBuilder_ == null) {
commitments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
commitmentsBuilder_.clear();
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public Builder removeCommitments(int index) {
if (commitmentsBuilder_ == null) {
ensureCommitmentsIsMutable();
commitments_.remove(index);
onChanged();
} else {
commitmentsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder getCommitmentsBuilder(
int index) {
return getCommitmentsFieldBuilder().getBuilder(index);
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder getCommitmentsOrBuilder(
int index) {
if (commitmentsBuilder_ == null) {
return commitments_.get(index); } else {
return commitmentsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getCommitmentsOrBuilderList() {
if (commitmentsBuilder_ != null) {
return commitmentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(commitments_);
}
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder addCommitmentsBuilder() {
return getCommitmentsFieldBuilder().addBuilder(
ibc.core.channel.v1.ChannelOuterClass.PacketState.getDefaultInstance());
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder addCommitmentsBuilder(
int index) {
return getCommitmentsFieldBuilder().addBuilder(
index, ibc.core.channel.v1.ChannelOuterClass.PacketState.getDefaultInstance());
}
/**
* repeated .ibc.core.channel.v1.PacketState commitments = 1;
*/
public java.util.List
getCommitmentsBuilderList() {
return getCommitmentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.PacketState, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder, ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getCommitmentsFieldBuilder() {
if (commitmentsBuilder_ == null) {
commitmentsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.PacketState, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder, ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>(
commitments_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
commitments_ = null;
}
return commitmentsBuilder_;
}
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder> paginationBuilder_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageResponse.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketCommitmentsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketCommitmentsResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketCommitmentsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketCommitmentsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketCommitmentsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketReceiptRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketReceiptRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
long getSequence();
}
/**
*
* QueryPacketReceiptRequest is the request type for the
* Query/PacketReceipt RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketReceiptRequest}
*/
public static final class QueryPacketReceiptRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketReceiptRequest)
QueryPacketReceiptRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketReceiptRequest.newBuilder() to construct.
private QueryPacketReceiptRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketReceiptRequest() {
portId_ = "";
channelId_ = "";
sequence_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketReceiptRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 24: {
sequence_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEQUENCE_FIELD_NUMBER = 3;
private long sequence_;
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public long getSequence() {
return sequence_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (sequence_ != 0L) {
output.writeUInt64(3, sequence_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
if (sequence_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, sequence_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && (getSequence()
== other.getSequence());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (37 * hash) + SEQUENCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSequence());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketReceiptRequest is the request type for the
* Query/PacketReceipt RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketReceiptRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketReceiptRequest)
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
sequence_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
result.sequence_ = sequence_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (other.getSequence() != 0L) {
setSequence(other.getSequence());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private long sequence_ ;
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public long getSequence() {
return sequence_;
}
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public Builder setSequence(long value) {
sequence_ = value;
onChanged();
return this;
}
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public Builder clearSequence() {
sequence_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketReceiptRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketReceiptRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketReceiptRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketReceiptRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketReceiptResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketReceiptResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* success flag for if receipt exists
*
*
* bool received = 2;
*/
boolean getReceived();
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryPacketReceiptResponse defines the client query response for a packet receipt
* which also includes a proof, and the height from which the proof was
* retrieved
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketReceiptResponse}
*/
public static final class QueryPacketReceiptResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketReceiptResponse)
QueryPacketReceiptResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketReceiptResponse.newBuilder() to construct.
private QueryPacketReceiptResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketReceiptResponse() {
received_ = false;
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketReceiptResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 16: {
received_ = input.readBool();
break;
}
case 26: {
proof_ = input.readBytes();
break;
}
case 34: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.Builder.class);
}
public static final int RECEIVED_FIELD_NUMBER = 2;
private boolean received_;
/**
*
* success flag for if receipt exists
*
*
* bool received = 2;
*/
public boolean getReceived() {
return received_;
}
public static final int PROOF_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 4;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (received_ != false) {
output.writeBool(2, received_);
}
if (!proof_.isEmpty()) {
output.writeBytes(3, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(4, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (received_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, received_);
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse) obj;
boolean result = true;
result = result && (getReceived()
== other.getReceived());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + RECEIVED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReceived());
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketReceiptResponse defines the client query response for a packet receipt
* which also includes a proof, and the height from which the proof was
* retrieved
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketReceiptResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketReceiptResponse)
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
received_ = false;
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse(this);
result.received_ = received_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse.getDefaultInstance()) return this;
if (other.getReceived() != false) {
setReceived(other.getReceived());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private boolean received_ ;
/**
*
* success flag for if receipt exists
*
*
* bool received = 2;
*/
public boolean getReceived() {
return received_;
}
/**
*
* success flag for if receipt exists
*
*
* bool received = 2;
*/
public Builder setReceived(boolean value) {
received_ = value;
onChanged();
return this;
}
/**
*
* success flag for if receipt exists
*
*
* bool received = 2;
*/
public Builder clearReceived() {
received_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketReceiptResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketReceiptResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketReceiptResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketReceiptResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketReceiptResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketAcknowledgementRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketAcknowledgementRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
long getSequence();
}
/**
*
* QueryPacketAcknowledgementRequest is the request type for the
* Query/PacketAcknowledgement RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementRequest}
*/
public static final class QueryPacketAcknowledgementRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketAcknowledgementRequest)
QueryPacketAcknowledgementRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketAcknowledgementRequest.newBuilder() to construct.
private QueryPacketAcknowledgementRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketAcknowledgementRequest() {
portId_ = "";
channelId_ = "";
sequence_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketAcknowledgementRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 24: {
sequence_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SEQUENCE_FIELD_NUMBER = 3;
private long sequence_;
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public long getSequence() {
return sequence_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (sequence_ != 0L) {
output.writeUInt64(3, sequence_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
if (sequence_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, sequence_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && (getSequence()
== other.getSequence());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (37 * hash) + SEQUENCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSequence());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketAcknowledgementRequest is the request type for the
* Query/PacketAcknowledgement RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketAcknowledgementRequest)
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
sequence_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
result.sequence_ = sequence_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (other.getSequence() != 0L) {
setSequence(other.getSequence());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private long sequence_ ;
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public long getSequence() {
return sequence_;
}
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public Builder setSequence(long value) {
sequence_ = value;
onChanged();
return this;
}
/**
*
* packet sequence
*
*
* uint64 sequence = 3;
*/
public Builder clearSequence() {
sequence_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketAcknowledgementRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketAcknowledgementRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketAcknowledgementRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketAcknowledgementRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketAcknowledgementResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketAcknowledgementResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* packet associated with the request fields
*
*
* bytes acknowledgement = 1;
*/
com.google.protobuf.ByteString getAcknowledgement();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryPacketAcknowledgementResponse defines the client query response for a
* packet which also includes a proof and the height from which the
* proof was retrieved
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementResponse}
*/
public static final class QueryPacketAcknowledgementResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketAcknowledgementResponse)
QueryPacketAcknowledgementResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketAcknowledgementResponse.newBuilder() to construct.
private QueryPacketAcknowledgementResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketAcknowledgementResponse() {
acknowledgement_ = com.google.protobuf.ByteString.EMPTY;
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketAcknowledgementResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
acknowledgement_ = input.readBytes();
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.Builder.class);
}
public static final int ACKNOWLEDGEMENT_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString acknowledgement_;
/**
*
* packet associated with the request fields
*
*
* bytes acknowledgement = 1;
*/
public com.google.protobuf.ByteString getAcknowledgement() {
return acknowledgement_;
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!acknowledgement_.isEmpty()) {
output.writeBytes(1, acknowledgement_);
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!acknowledgement_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, acknowledgement_);
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse) obj;
boolean result = true;
result = result && getAcknowledgement()
.equals(other.getAcknowledgement());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACKNOWLEDGEMENT_FIELD_NUMBER;
hash = (53 * hash) + getAcknowledgement().hashCode();
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketAcknowledgementResponse defines the client query response for a
* packet which also includes a proof and the height from which the
* proof was retrieved
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketAcknowledgementResponse)
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
acknowledgement_ = com.google.protobuf.ByteString.EMPTY;
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse(this);
result.acknowledgement_ = acknowledgement_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse.getDefaultInstance()) return this;
if (other.getAcknowledgement() != com.google.protobuf.ByteString.EMPTY) {
setAcknowledgement(other.getAcknowledgement());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.ByteString acknowledgement_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* packet associated with the request fields
*
*
* bytes acknowledgement = 1;
*/
public com.google.protobuf.ByteString getAcknowledgement() {
return acknowledgement_;
}
/**
*
* packet associated with the request fields
*
*
* bytes acknowledgement = 1;
*/
public Builder setAcknowledgement(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
acknowledgement_ = value;
onChanged();
return this;
}
/**
*
* packet associated with the request fields
*
*
* bytes acknowledgement = 1;
*/
public Builder clearAcknowledgement() {
acknowledgement_ = getDefaultInstance().getAcknowledgement();
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketAcknowledgementResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketAcknowledgementResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketAcknowledgementResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketAcknowledgementResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketAcknowledgementsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketAcknowledgementsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
boolean hasPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
cosmos.base.query.v1beta1.Pagination.PageRequest getPagination();
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder();
}
/**
*
* QueryPacketAcknowledgementsRequest is the request type for the
* Query/QueryPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementsRequest}
*/
public static final class QueryPacketAcknowledgementsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketAcknowledgementsRequest)
QueryPacketAcknowledgementsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketAcknowledgementsRequest.newBuilder() to construct.
private QueryPacketAcknowledgementsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketAcknowledgementsRequest() {
portId_ = "";
channelId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketAcknowledgementsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 26: {
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGINATION_FIELD_NUMBER = 3;
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
return getPagination();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (pagination_ != null) {
output.writeMessage(3, getPagination());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPagination());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketAcknowledgementsRequest is the request type for the
* Query/QueryPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketAcknowledgementsRequest)
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder> paginationBuilder_;
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageRequest.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination request
*
*
* .cosmos.base.query.v1beta1.PageRequest pagination = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketAcknowledgementsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketAcknowledgementsRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketAcknowledgementsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketAcknowledgementsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryPacketAcknowledgementsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryPacketAcknowledgementsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
java.util.List
getAcknowledgementsList();
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.PacketState getAcknowledgements(int index);
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
int getAcknowledgementsCount();
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
java.util.List extends ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getAcknowledgementsOrBuilderList();
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder getAcknowledgementsOrBuilder(
int index);
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
boolean hasPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponse getPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryPacketAcknowledgemetsResponse is the request type for the
* Query/QueryPacketAcknowledgements RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementsResponse}
*/
public static final class QueryPacketAcknowledgementsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryPacketAcknowledgementsResponse)
QueryPacketAcknowledgementsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryPacketAcknowledgementsResponse.newBuilder() to construct.
private QueryPacketAcknowledgementsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryPacketAcknowledgementsResponse() {
acknowledgements_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryPacketAcknowledgementsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
acknowledgements_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
acknowledgements_.add(
input.readMessage(ibc.core.channel.v1.ChannelOuterClass.PacketState.parser(), extensionRegistry));
break;
}
case 18: {
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
acknowledgements_ = java.util.Collections.unmodifiableList(acknowledgements_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.Builder.class);
}
private int bitField0_;
public static final int ACKNOWLEDGEMENTS_FIELD_NUMBER = 1;
private java.util.List acknowledgements_;
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public java.util.List getAcknowledgementsList() {
return acknowledgements_;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getAcknowledgementsOrBuilderList() {
return acknowledgements_;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public int getAcknowledgementsCount() {
return acknowledgements_.size();
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState getAcknowledgements(int index) {
return acknowledgements_.get(index);
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder getAcknowledgementsOrBuilder(
int index) {
return acknowledgements_.get(index);
}
public static final int PAGINATION_FIELD_NUMBER = 2;
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
return getPagination();
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < acknowledgements_.size(); i++) {
output.writeMessage(1, acknowledgements_.get(i));
}
if (pagination_ != null) {
output.writeMessage(2, getPagination());
}
if (height_ != null) {
output.writeMessage(3, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < acknowledgements_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, acknowledgements_.get(i));
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagination());
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse) obj;
boolean result = true;
result = result && getAcknowledgementsList()
.equals(other.getAcknowledgementsList());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAcknowledgementsCount() > 0) {
hash = (37 * hash) + ACKNOWLEDGEMENTS_FIELD_NUMBER;
hash = (53 * hash) + getAcknowledgementsList().hashCode();
}
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryPacketAcknowledgemetsResponse is the request type for the
* Query/QueryPacketAcknowledgements RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryPacketAcknowledgementsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryPacketAcknowledgementsResponse)
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getAcknowledgementsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (acknowledgementsBuilder_ == null) {
acknowledgements_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
acknowledgementsBuilder_.clear();
}
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (acknowledgementsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
acknowledgements_ = java.util.Collections.unmodifiableList(acknowledgements_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.acknowledgements_ = acknowledgements_;
} else {
result.acknowledgements_ = acknowledgementsBuilder_.build();
}
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse.getDefaultInstance()) return this;
if (acknowledgementsBuilder_ == null) {
if (!other.acknowledgements_.isEmpty()) {
if (acknowledgements_.isEmpty()) {
acknowledgements_ = other.acknowledgements_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureAcknowledgementsIsMutable();
acknowledgements_.addAll(other.acknowledgements_);
}
onChanged();
}
} else {
if (!other.acknowledgements_.isEmpty()) {
if (acknowledgementsBuilder_.isEmpty()) {
acknowledgementsBuilder_.dispose();
acknowledgementsBuilder_ = null;
acknowledgements_ = other.acknowledgements_;
bitField0_ = (bitField0_ & ~0x00000001);
acknowledgementsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getAcknowledgementsFieldBuilder() : null;
} else {
acknowledgementsBuilder_.addAllMessages(other.acknowledgements_);
}
}
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List acknowledgements_ =
java.util.Collections.emptyList();
private void ensureAcknowledgementsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
acknowledgements_ = new java.util.ArrayList(acknowledgements_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.PacketState, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder, ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder> acknowledgementsBuilder_;
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public java.util.List getAcknowledgementsList() {
if (acknowledgementsBuilder_ == null) {
return java.util.Collections.unmodifiableList(acknowledgements_);
} else {
return acknowledgementsBuilder_.getMessageList();
}
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public int getAcknowledgementsCount() {
if (acknowledgementsBuilder_ == null) {
return acknowledgements_.size();
} else {
return acknowledgementsBuilder_.getCount();
}
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState getAcknowledgements(int index) {
if (acknowledgementsBuilder_ == null) {
return acknowledgements_.get(index);
} else {
return acknowledgementsBuilder_.getMessage(index);
}
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder setAcknowledgements(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState value) {
if (acknowledgementsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAcknowledgementsIsMutable();
acknowledgements_.set(index, value);
onChanged();
} else {
acknowledgementsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder setAcknowledgements(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder builderForValue) {
if (acknowledgementsBuilder_ == null) {
ensureAcknowledgementsIsMutable();
acknowledgements_.set(index, builderForValue.build());
onChanged();
} else {
acknowledgementsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder addAcknowledgements(ibc.core.channel.v1.ChannelOuterClass.PacketState value) {
if (acknowledgementsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAcknowledgementsIsMutable();
acknowledgements_.add(value);
onChanged();
} else {
acknowledgementsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder addAcknowledgements(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState value) {
if (acknowledgementsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAcknowledgementsIsMutable();
acknowledgements_.add(index, value);
onChanged();
} else {
acknowledgementsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder addAcknowledgements(
ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder builderForValue) {
if (acknowledgementsBuilder_ == null) {
ensureAcknowledgementsIsMutable();
acknowledgements_.add(builderForValue.build());
onChanged();
} else {
acknowledgementsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder addAcknowledgements(
int index, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder builderForValue) {
if (acknowledgementsBuilder_ == null) {
ensureAcknowledgementsIsMutable();
acknowledgements_.add(index, builderForValue.build());
onChanged();
} else {
acknowledgementsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder addAllAcknowledgements(
java.lang.Iterable extends ibc.core.channel.v1.ChannelOuterClass.PacketState> values) {
if (acknowledgementsBuilder_ == null) {
ensureAcknowledgementsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, acknowledgements_);
onChanged();
} else {
acknowledgementsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder clearAcknowledgements() {
if (acknowledgementsBuilder_ == null) {
acknowledgements_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
acknowledgementsBuilder_.clear();
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public Builder removeAcknowledgements(int index) {
if (acknowledgementsBuilder_ == null) {
ensureAcknowledgementsIsMutable();
acknowledgements_.remove(index);
onChanged();
} else {
acknowledgementsBuilder_.remove(index);
}
return this;
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder getAcknowledgementsBuilder(
int index) {
return getAcknowledgementsFieldBuilder().getBuilder(index);
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder getAcknowledgementsOrBuilder(
int index) {
if (acknowledgementsBuilder_ == null) {
return acknowledgements_.get(index); } else {
return acknowledgementsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public java.util.List extends ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getAcknowledgementsOrBuilderList() {
if (acknowledgementsBuilder_ != null) {
return acknowledgementsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(acknowledgements_);
}
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder addAcknowledgementsBuilder() {
return getAcknowledgementsFieldBuilder().addBuilder(
ibc.core.channel.v1.ChannelOuterClass.PacketState.getDefaultInstance());
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder addAcknowledgementsBuilder(
int index) {
return getAcknowledgementsFieldBuilder().addBuilder(
index, ibc.core.channel.v1.ChannelOuterClass.PacketState.getDefaultInstance());
}
/**
* repeated .ibc.core.channel.v1.PacketState acknowledgements = 1;
*/
public java.util.List
getAcknowledgementsBuilderList() {
return getAcknowledgementsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.PacketState, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder, ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>
getAcknowledgementsFieldBuilder() {
if (acknowledgementsBuilder_ == null) {
acknowledgementsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.channel.v1.ChannelOuterClass.PacketState, ibc.core.channel.v1.ChannelOuterClass.PacketState.Builder, ibc.core.channel.v1.ChannelOuterClass.PacketStateOrBuilder>(
acknowledgements_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
acknowledgements_ = null;
}
return acknowledgementsBuilder_;
}
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder> paginationBuilder_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageResponse.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryPacketAcknowledgementsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryPacketAcknowledgementsResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryPacketAcknowledgementsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryPacketAcknowledgementsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryPacketAcknowledgementsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryUnreceivedPacketsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryUnreceivedPacketsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
java.util.List getPacketCommitmentSequencesList();
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
int getPacketCommitmentSequencesCount();
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
long getPacketCommitmentSequences(int index);
}
/**
*
* QueryUnreceivedPacketsRequest is the request type for the
* Query/UnreceivedPackets RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedPacketsRequest}
*/
public static final class QueryUnreceivedPacketsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryUnreceivedPacketsRequest)
QueryUnreceivedPacketsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryUnreceivedPacketsRequest.newBuilder() to construct.
private QueryUnreceivedPacketsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryUnreceivedPacketsRequest() {
portId_ = "";
channelId_ = "";
packetCommitmentSequences_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryUnreceivedPacketsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
packetCommitmentSequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
packetCommitmentSequences_.add(input.readUInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
packetCommitmentSequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
packetCommitmentSequences_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
packetCommitmentSequences_ = java.util.Collections.unmodifiableList(packetCommitmentSequences_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.Builder.class);
}
private int bitField0_;
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PACKET_COMMITMENT_SEQUENCES_FIELD_NUMBER = 3;
private java.util.List packetCommitmentSequences_;
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public java.util.List
getPacketCommitmentSequencesList() {
return packetCommitmentSequences_;
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public int getPacketCommitmentSequencesCount() {
return packetCommitmentSequences_.size();
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public long getPacketCommitmentSequences(int index) {
return packetCommitmentSequences_.get(index);
}
private int packetCommitmentSequencesMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (getPacketCommitmentSequencesList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(packetCommitmentSequencesMemoizedSerializedSize);
}
for (int i = 0; i < packetCommitmentSequences_.size(); i++) {
output.writeUInt64NoTag(packetCommitmentSequences_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
{
int dataSize = 0;
for (int i = 0; i < packetCommitmentSequences_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(packetCommitmentSequences_.get(i));
}
size += dataSize;
if (!getPacketCommitmentSequencesList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
packetCommitmentSequencesMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && getPacketCommitmentSequencesList()
.equals(other.getPacketCommitmentSequencesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
if (getPacketCommitmentSequencesCount() > 0) {
hash = (37 * hash) + PACKET_COMMITMENT_SEQUENCES_FIELD_NUMBER;
hash = (53 * hash) + getPacketCommitmentSequencesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryUnreceivedPacketsRequest is the request type for the
* Query/UnreceivedPackets RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedPacketsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryUnreceivedPacketsRequest)
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
packetCommitmentSequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.portId_ = portId_;
result.channelId_ = channelId_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
packetCommitmentSequences_ = java.util.Collections.unmodifiableList(packetCommitmentSequences_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.packetCommitmentSequences_ = packetCommitmentSequences_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (!other.packetCommitmentSequences_.isEmpty()) {
if (packetCommitmentSequences_.isEmpty()) {
packetCommitmentSequences_ = other.packetCommitmentSequences_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensurePacketCommitmentSequencesIsMutable();
packetCommitmentSequences_.addAll(other.packetCommitmentSequences_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private java.util.List packetCommitmentSequences_ = java.util.Collections.emptyList();
private void ensurePacketCommitmentSequencesIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
packetCommitmentSequences_ = new java.util.ArrayList(packetCommitmentSequences_);
bitField0_ |= 0x00000004;
}
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public java.util.List
getPacketCommitmentSequencesList() {
return java.util.Collections.unmodifiableList(packetCommitmentSequences_);
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public int getPacketCommitmentSequencesCount() {
return packetCommitmentSequences_.size();
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public long getPacketCommitmentSequences(int index) {
return packetCommitmentSequences_.get(index);
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public Builder setPacketCommitmentSequences(
int index, long value) {
ensurePacketCommitmentSequencesIsMutable();
packetCommitmentSequences_.set(index, value);
onChanged();
return this;
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public Builder addPacketCommitmentSequences(long value) {
ensurePacketCommitmentSequencesIsMutable();
packetCommitmentSequences_.add(value);
onChanged();
return this;
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public Builder addAllPacketCommitmentSequences(
java.lang.Iterable extends java.lang.Long> values) {
ensurePacketCommitmentSequencesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, packetCommitmentSequences_);
onChanged();
return this;
}
/**
*
* list of packet sequences
*
*
* repeated uint64 packet_commitment_sequences = 3;
*/
public Builder clearPacketCommitmentSequences() {
packetCommitmentSequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryUnreceivedPacketsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryUnreceivedPacketsRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryUnreceivedPacketsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryUnreceivedPacketsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryUnreceivedPacketsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryUnreceivedPacketsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
java.util.List getSequencesList();
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
int getSequencesCount();
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
long getSequences(int index);
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryUnreceivedPacketsResponse is the response type for the
* Query/UnreceivedPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedPacketsResponse}
*/
public static final class QueryUnreceivedPacketsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryUnreceivedPacketsResponse)
QueryUnreceivedPacketsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryUnreceivedPacketsResponse.newBuilder() to construct.
private QueryUnreceivedPacketsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryUnreceivedPacketsResponse() {
sequences_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryUnreceivedPacketsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
sequences_.add(input.readUInt64());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
sequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
sequences_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 18: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = java.util.Collections.unmodifiableList(sequences_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.Builder.class);
}
private int bitField0_;
public static final int SEQUENCES_FIELD_NUMBER = 1;
private java.util.List sequences_;
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public java.util.List
getSequencesList() {
return sequences_;
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public int getSequencesCount() {
return sequences_.size();
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public long getSequences(int index) {
return sequences_.get(index);
}
private int sequencesMemoizedSerializedSize = -1;
public static final int HEIGHT_FIELD_NUMBER = 2;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getSequencesList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(sequencesMemoizedSerializedSize);
}
for (int i = 0; i < sequences_.size(); i++) {
output.writeUInt64NoTag(sequences_.get(i));
}
if (height_ != null) {
output.writeMessage(2, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < sequences_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(sequences_.get(i));
}
size += dataSize;
if (!getSequencesList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
sequencesMemoizedSerializedSize = dataSize;
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse) obj;
boolean result = true;
result = result && getSequencesList()
.equals(other.getSequencesList());
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSequencesCount() > 0) {
hash = (37 * hash) + SEQUENCES_FIELD_NUMBER;
hash = (53 * hash) + getSequencesList().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryUnreceivedPacketsResponse is the response type for the
* Query/UnreceivedPacketCommitments RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedPacketsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryUnreceivedPacketsResponse)
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = java.util.Collections.unmodifiableList(sequences_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.sequences_ = sequences_;
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse.getDefaultInstance()) return this;
if (!other.sequences_.isEmpty()) {
if (sequences_.isEmpty()) {
sequences_ = other.sequences_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSequencesIsMutable();
sequences_.addAll(other.sequences_);
}
onChanged();
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List sequences_ = java.util.Collections.emptyList();
private void ensureSequencesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = new java.util.ArrayList(sequences_);
bitField0_ |= 0x00000001;
}
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public java.util.List
getSequencesList() {
return java.util.Collections.unmodifiableList(sequences_);
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public int getSequencesCount() {
return sequences_.size();
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public long getSequences(int index) {
return sequences_.get(index);
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder setSequences(
int index, long value) {
ensureSequencesIsMutable();
sequences_.set(index, value);
onChanged();
return this;
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder addSequences(long value) {
ensureSequencesIsMutable();
sequences_.add(value);
onChanged();
return this;
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder addAllSequences(
java.lang.Iterable extends java.lang.Long> values) {
ensureSequencesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sequences_);
onChanged();
return this;
}
/**
*
* list of unreceived packet sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder clearSequences() {
sequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryUnreceivedPacketsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryUnreceivedPacketsResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryUnreceivedPacketsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryUnreceivedPacketsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedPacketsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryUnreceivedAcksRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryUnreceivedAcksRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
java.util.List getPacketAckSequencesList();
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
int getPacketAckSequencesCount();
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
long getPacketAckSequences(int index);
}
/**
*
* QueryUnreceivedAcks is the request type for the
* Query/UnreceivedAcks RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedAcksRequest}
*/
public static final class QueryUnreceivedAcksRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryUnreceivedAcksRequest)
QueryUnreceivedAcksRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryUnreceivedAcksRequest.newBuilder() to construct.
private QueryUnreceivedAcksRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryUnreceivedAcksRequest() {
portId_ = "";
channelId_ = "";
packetAckSequences_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryUnreceivedAcksRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
packetAckSequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
packetAckSequences_.add(input.readUInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004) && input.getBytesUntilLimit() > 0) {
packetAckSequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
packetAckSequences_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
packetAckSequences_ = java.util.Collections.unmodifiableList(packetAckSequences_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.Builder.class);
}
private int bitField0_;
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PACKET_ACK_SEQUENCES_FIELD_NUMBER = 3;
private java.util.List packetAckSequences_;
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public java.util.List
getPacketAckSequencesList() {
return packetAckSequences_;
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public int getPacketAckSequencesCount() {
return packetAckSequences_.size();
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public long getPacketAckSequences(int index) {
return packetAckSequences_.get(index);
}
private int packetAckSequencesMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
if (getPacketAckSequencesList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(packetAckSequencesMemoizedSerializedSize);
}
for (int i = 0; i < packetAckSequences_.size(); i++) {
output.writeUInt64NoTag(packetAckSequences_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
{
int dataSize = 0;
for (int i = 0; i < packetAckSequences_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(packetAckSequences_.get(i));
}
size += dataSize;
if (!getPacketAckSequencesList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
packetAckSequencesMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && getPacketAckSequencesList()
.equals(other.getPacketAckSequencesList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
if (getPacketAckSequencesCount() > 0) {
hash = (37 * hash) + PACKET_ACK_SEQUENCES_FIELD_NUMBER;
hash = (53 * hash) + getPacketAckSequencesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryUnreceivedAcks is the request type for the
* Query/UnreceivedAcks RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedAcksRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryUnreceivedAcksRequest)
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
packetAckSequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.portId_ = portId_;
result.channelId_ = channelId_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
packetAckSequences_ = java.util.Collections.unmodifiableList(packetAckSequences_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.packetAckSequences_ = packetAckSequences_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
if (!other.packetAckSequences_.isEmpty()) {
if (packetAckSequences_.isEmpty()) {
packetAckSequences_ = other.packetAckSequences_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensurePacketAckSequencesIsMutable();
packetAckSequences_.addAll(other.packetAckSequences_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
private java.util.List packetAckSequences_ = java.util.Collections.emptyList();
private void ensurePacketAckSequencesIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
packetAckSequences_ = new java.util.ArrayList(packetAckSequences_);
bitField0_ |= 0x00000004;
}
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public java.util.List
getPacketAckSequencesList() {
return java.util.Collections.unmodifiableList(packetAckSequences_);
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public int getPacketAckSequencesCount() {
return packetAckSequences_.size();
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public long getPacketAckSequences(int index) {
return packetAckSequences_.get(index);
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public Builder setPacketAckSequences(
int index, long value) {
ensurePacketAckSequencesIsMutable();
packetAckSequences_.set(index, value);
onChanged();
return this;
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public Builder addPacketAckSequences(long value) {
ensurePacketAckSequencesIsMutable();
packetAckSequences_.add(value);
onChanged();
return this;
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public Builder addAllPacketAckSequences(
java.lang.Iterable extends java.lang.Long> values) {
ensurePacketAckSequencesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, packetAckSequences_);
onChanged();
return this;
}
/**
*
* list of acknowledgement sequences
*
*
* repeated uint64 packet_ack_sequences = 3;
*/
public Builder clearPacketAckSequences() {
packetAckSequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryUnreceivedAcksRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryUnreceivedAcksRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryUnreceivedAcksRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryUnreceivedAcksRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryUnreceivedAcksResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryUnreceivedAcksResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
java.util.List getSequencesList();
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
int getSequencesCount();
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
long getSequences(int index);
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryUnreceivedAcksResponse is the response type for the
* Query/UnreceivedAcks RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedAcksResponse}
*/
public static final class QueryUnreceivedAcksResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryUnreceivedAcksResponse)
QueryUnreceivedAcksResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryUnreceivedAcksResponse.newBuilder() to construct.
private QueryUnreceivedAcksResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryUnreceivedAcksResponse() {
sequences_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryUnreceivedAcksResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
sequences_.add(input.readUInt64());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
sequences_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
sequences_.add(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 18: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = java.util.Collections.unmodifiableList(sequences_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.Builder.class);
}
private int bitField0_;
public static final int SEQUENCES_FIELD_NUMBER = 1;
private java.util.List sequences_;
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public java.util.List
getSequencesList() {
return sequences_;
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public int getSequencesCount() {
return sequences_.size();
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public long getSequences(int index) {
return sequences_.get(index);
}
private int sequencesMemoizedSerializedSize = -1;
public static final int HEIGHT_FIELD_NUMBER = 2;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getSequencesList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(sequencesMemoizedSerializedSize);
}
for (int i = 0; i < sequences_.size(); i++) {
output.writeUInt64NoTag(sequences_.get(i));
}
if (height_ != null) {
output.writeMessage(2, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < sequences_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(sequences_.get(i));
}
size += dataSize;
if (!getSequencesList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
sequencesMemoizedSerializedSize = dataSize;
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse) obj;
boolean result = true;
result = result && getSequencesList()
.equals(other.getSequencesList());
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSequencesCount() > 0) {
hash = (37 * hash) + SEQUENCES_FIELD_NUMBER;
hash = (53 * hash) + getSequencesList().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryUnreceivedAcksResponse is the response type for the
* Query/UnreceivedAcks RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryUnreceivedAcksResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryUnreceivedAcksResponse)
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
sequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = java.util.Collections.unmodifiableList(sequences_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.sequences_ = sequences_;
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse.getDefaultInstance()) return this;
if (!other.sequences_.isEmpty()) {
if (sequences_.isEmpty()) {
sequences_ = other.sequences_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSequencesIsMutable();
sequences_.addAll(other.sequences_);
}
onChanged();
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List sequences_ = java.util.Collections.emptyList();
private void ensureSequencesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
sequences_ = new java.util.ArrayList(sequences_);
bitField0_ |= 0x00000001;
}
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public java.util.List
getSequencesList() {
return java.util.Collections.unmodifiableList(sequences_);
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public int getSequencesCount() {
return sequences_.size();
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public long getSequences(int index) {
return sequences_.get(index);
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder setSequences(
int index, long value) {
ensureSequencesIsMutable();
sequences_.set(index, value);
onChanged();
return this;
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder addSequences(long value) {
ensureSequencesIsMutable();
sequences_.add(value);
onChanged();
return this;
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder addAllSequences(
java.lang.Iterable extends java.lang.Long> values) {
ensureSequencesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sequences_);
onChanged();
return this;
}
/**
*
* list of unreceived acknowledgement sequences
*
*
* repeated uint64 sequences = 1;
*/
public Builder clearSequences() {
sequences_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 2 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryUnreceivedAcksResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryUnreceivedAcksResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryUnreceivedAcksResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryUnreceivedAcksResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryUnreceivedAcksResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryNextSequenceReceiveRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryNextSequenceReceiveRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
java.lang.String getPortId();
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
com.google.protobuf.ByteString
getPortIdBytes();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
java.lang.String getChannelId();
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
com.google.protobuf.ByteString
getChannelIdBytes();
}
/**
*
* QueryNextSequenceReceiveRequest is the request type for the
* Query/QueryNextSequenceReceiveRequest RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryNextSequenceReceiveRequest}
*/
public static final class QueryNextSequenceReceiveRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryNextSequenceReceiveRequest)
QueryNextSequenceReceiveRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryNextSequenceReceiveRequest.newBuilder() to construct.
private QueryNextSequenceReceiveRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryNextSequenceReceiveRequest() {
portId_ = "";
channelId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryNextSequenceReceiveRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
portId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
channelId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.Builder.class);
}
public static final int PORT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object portId_;
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNEL_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object channelId_;
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPortIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, channelId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPortIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, portId_);
}
if (!getChannelIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, channelId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest other = (ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest) obj;
boolean result = true;
result = result && getPortId()
.equals(other.getPortId());
result = result && getChannelId()
.equals(other.getChannelId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PORT_ID_FIELD_NUMBER;
hash = (53 * hash) + getPortId().hashCode();
hash = (37 * hash) + CHANNEL_ID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryNextSequenceReceiveRequest is the request type for the
* Query/QueryNextSequenceReceiveRequest RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryNextSequenceReceiveRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryNextSequenceReceiveRequest)
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.class, ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
portId_ = "";
channelId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest build() {
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest result = new ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest(this);
result.portId_ = portId_;
result.channelId_ = channelId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest.getDefaultInstance()) return this;
if (!other.getPortId().isEmpty()) {
portId_ = other.portId_;
onChanged();
}
if (!other.getChannelId().isEmpty()) {
channelId_ = other.channelId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object portId_ = "";
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public java.lang.String getPortId() {
java.lang.Object ref = portId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
portId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public com.google.protobuf.ByteString
getPortIdBytes() {
java.lang.Object ref = portId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
portId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
portId_ = value;
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder clearPortId() {
portId_ = getDefaultInstance().getPortId();
onChanged();
return this;
}
/**
*
* port unique identifier
*
*
* string port_id = 1;
*/
public Builder setPortIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
portId_ = value;
onChanged();
return this;
}
private java.lang.Object channelId_ = "";
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public java.lang.String getChannelId() {
java.lang.Object ref = channelId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
channelId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public com.google.protobuf.ByteString
getChannelIdBytes() {
java.lang.Object ref = channelId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
channelId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
channelId_ = value;
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder clearChannelId() {
channelId_ = getDefaultInstance().getChannelId();
onChanged();
return this;
}
/**
*
* channel unique identifier
*
*
* string channel_id = 2;
*/
public Builder setChannelIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
channelId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryNextSequenceReceiveRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryNextSequenceReceiveRequest)
private static final ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryNextSequenceReceiveRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryNextSequenceReceiveRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryNextSequenceReceiveResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.channel.v1.QueryNextSequenceReceiveResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* next sequence receive number
*
*
* uint64 next_sequence_receive = 1;
*/
long getNextSequenceReceive();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QuerySequenceResponse is the request type for the
* Query/QueryNextSequenceReceiveResponse RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryNextSequenceReceiveResponse}
*/
public static final class QueryNextSequenceReceiveResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.channel.v1.QueryNextSequenceReceiveResponse)
QueryNextSequenceReceiveResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryNextSequenceReceiveResponse.newBuilder() to construct.
private QueryNextSequenceReceiveResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryNextSequenceReceiveResponse() {
nextSequenceReceive_ = 0L;
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryNextSequenceReceiveResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
nextSequenceReceive_ = input.readUInt64();
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.Builder.class);
}
public static final int NEXT_SEQUENCE_RECEIVE_FIELD_NUMBER = 1;
private long nextSequenceReceive_;
/**
*
* next sequence receive number
*
*
* uint64 next_sequence_receive = 1;
*/
public long getNextSequenceReceive() {
return nextSequenceReceive_;
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (nextSequenceReceive_ != 0L) {
output.writeUInt64(1, nextSequenceReceive_);
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (nextSequenceReceive_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, nextSequenceReceive_);
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse)) {
return super.equals(obj);
}
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse other = (ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse) obj;
boolean result = true;
result = result && (getNextSequenceReceive()
== other.getNextSequenceReceive());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NEXT_SEQUENCE_RECEIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNextSequenceReceive());
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QuerySequenceResponse is the request type for the
* Query/QueryNextSequenceReceiveResponse RPC method
*
*
* Protobuf type {@code ibc.core.channel.v1.QueryNextSequenceReceiveResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.channel.v1.QueryNextSequenceReceiveResponse)
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.class, ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.Builder.class);
}
// Construct using ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
nextSequenceReceive_ = 0L;
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.channel.v1.QueryOuterClass.internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_descriptor;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse getDefaultInstanceForType() {
return ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse build() {
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse buildPartial() {
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse result = new ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse(this);
result.nextSequenceReceive_ = nextSequenceReceive_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse) {
return mergeFrom((ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse other) {
if (other == ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse.getDefaultInstance()) return this;
if (other.getNextSequenceReceive() != 0L) {
setNextSequenceReceive(other.getNextSequenceReceive());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long nextSequenceReceive_ ;
/**
*
* next sequence receive number
*
*
* uint64 next_sequence_receive = 1;
*/
public long getNextSequenceReceive() {
return nextSequenceReceive_;
}
/**
*
* next sequence receive number
*
*
* uint64 next_sequence_receive = 1;
*/
public Builder setNextSequenceReceive(long value) {
nextSequenceReceive_ = value;
onChanged();
return this;
}
/**
*
* next sequence receive number
*
*
* uint64 next_sequence_receive = 1;
*/
public Builder clearNextSequenceReceive() {
nextSequenceReceive_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.channel.v1.QueryNextSequenceReceiveResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.channel.v1.QueryNextSequenceReceiveResponse)
private static final ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse();
}
public static ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryNextSequenceReceiveResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryNextSequenceReceiveResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.channel.v1.QueryOuterClass.QueryNextSequenceReceiveResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\037ibc/core/channel/v1/query.proto\022\023ibc.c" +
"ore.channel.v1\032\037ibc/core/client/v1/clien" +
"t.proto\032*cosmos/base/query/v1beta1/pagin" +
"ation.proto\032!ibc/core/channel/v1/channel" +
".proto\032\034google/api/annotations.proto\032\031go" +
"ogle/protobuf/any.proto\032\024gogoproto/gogo." +
"proto\":\n\023QueryChannelRequest\022\017\n\007port_id\030" +
"\001 \001(\t\022\022\n\nchannel_id\030\002 \001(\t\"\214\001\n\024QueryChann" +
"elResponse\022-\n\007channel\030\001 \001(\0132\034.ibc.core.c" +
"hannel.v1.Channel\022\r\n\005proof\030\002 \001(\014\0226\n\014proo" +
"f_height\030\003 \001(\0132\032.ibc.core.client.v1.Heig" +
"htB\004\310\336\037\000\"R\n\024QueryChannelsRequest\022:\n\npagi" +
"nation\030\001 \001(\0132&.cosmos.base.query.v1beta1" +
".PageRequest\"\300\001\n\025QueryChannelsResponse\0228" +
"\n\010channels\030\001 \003(\0132&.ibc.core.channel.v1.I" +
"dentifiedChannel\022;\n\npagination\030\002 \001(\0132\'.c" +
"osmos.base.query.v1beta1.PageResponse\0220\n" +
"\006height\030\003 \001(\0132\032.ibc.core.client.v1.Heigh" +
"tB\004\310\336\037\000\"p\n\036QueryConnectionChannelsReques" +
"t\022\022\n\nconnection\030\001 \001(\t\022:\n\npagination\030\002 \001(" +
"\0132&.cosmos.base.query.v1beta1.PageReques" +
"t\"\312\001\n\037QueryConnectionChannelsResponse\0228\n" +
"\010channels\030\001 \003(\0132&.ibc.core.channel.v1.Id" +
"entifiedChannel\022;\n\npagination\030\002 \001(\0132\'.co" +
"smos.base.query.v1beta1.PageResponse\0220\n\006" +
"height\030\003 \001(\0132\032.ibc.core.client.v1.Height" +
"B\004\310\336\037\000\"E\n\036QueryChannelClientStateRequest" +
"\022\017\n\007port_id\030\001 \001(\t\022\022\n\nchannel_id\030\002 \001(\t\"\264\001" +
"\n\037QueryChannelClientStateResponse\022J\n\027ide" +
"ntified_client_state\030\001 \001(\0132).ibc.core.cl" +
"ient.v1.IdentifiedClientState\022\r\n\005proof\030\002" +
" \001(\014\0226\n\014proof_height\030\003 \001(\0132\032.ibc.core.cl" +
"ient.v1.HeightB\004\310\336\037\000\"z\n!QueryChannelCons" +
"ensusStateRequest\022\017\n\007port_id\030\001 \001(\t\022\022\n\nch" +
"annel_id\030\002 \001(\t\022\027\n\017revision_number\030\003 \001(\004\022" +
"\027\n\017revision_height\030\004 \001(\004\"\255\001\n\"QueryChanne" +
"lConsensusStateResponse\022-\n\017consensus_sta" +
"te\030\001 \001(\0132\024.google.protobuf.Any\022\021\n\tclient" +
"_id\030\002 \001(\t\022\r\n\005proof\030\003 \001(\014\0226\n\014proof_height" +
"\030\004 \001(\0132\032.ibc.core.client.v1.HeightB\004\310\336\037\000" +
"\"U\n\034QueryPacketCommitmentRequest\022\017\n\007port" +
"_id\030\001 \001(\t\022\022\n\nchannel_id\030\002 \001(\t\022\020\n\010sequenc" +
"e\030\003 \001(\004\"z\n\035QueryPacketCommitmentResponse" +
"\022\022\n\ncommitment\030\001 \001(\014\022\r\n\005proof\030\002 \001(\014\0226\n\014p" +
"roof_height\030\003 \001(\0132\032.ibc.core.client.v1.H" +
"eightB\004\310\336\037\000\"\200\001\n\035QueryPacketCommitmentsRe" +
"quest\022\017\n\007port_id\030\001 \001(\t\022\022\n\nchannel_id\030\002 \001" +
"(\t\022:\n\npagination\030\003 \001(\0132&.cosmos.base.que" +
"ry.v1beta1.PageRequest\"\306\001\n\036QueryPacketCo" +
"mmitmentsResponse\0225\n\013commitments\030\001 \003(\0132 " +
".ibc.core.channel.v1.PacketState\022;\n\npagi" +
"nation\030\002 \001(\0132\'.cosmos.base.query.v1beta1" +
".PageResponse\0220\n\006height\030\003 \001(\0132\032.ibc.core" +
".client.v1.HeightB\004\310\336\037\000\"R\n\031QueryPacketRe" +
"ceiptRequest\022\017\n\007port_id\030\001 \001(\t\022\022\n\nchannel" +
"_id\030\002 \001(\t\022\020\n\010sequence\030\003 \001(\004\"u\n\032QueryPack" +
"etReceiptResponse\022\020\n\010received\030\002 \001(\010\022\r\n\005p" +
"roof\030\003 \001(\014\0226\n\014proof_height\030\004 \001(\0132\032.ibc.c" +
"ore.client.v1.HeightB\004\310\336\037\000\"Z\n!QueryPacke" +
"tAcknowledgementRequest\022\017\n\007port_id\030\001 \001(\t" +
"\022\022\n\nchannel_id\030\002 \001(\t\022\020\n\010sequence\030\003 \001(\004\"\204" +
"\001\n\"QueryPacketAcknowledgementResponse\022\027\n" +
"\017acknowledgement\030\001 \001(\014\022\r\n\005proof\030\002 \001(\014\0226\n" +
"\014proof_height\030\003 \001(\0132\032.ibc.core.client.v1" +
".HeightB\004\310\336\037\000\"\205\001\n\"QueryPacketAcknowledge" +
"mentsRequest\022\017\n\007port_id\030\001 \001(\t\022\022\n\nchannel" +
"_id\030\002 \001(\t\022:\n\npagination\030\003 \001(\0132&.cosmos.b" +
"ase.query.v1beta1.PageRequest\"\320\001\n#QueryP" +
"acketAcknowledgementsResponse\022:\n\020acknowl" +
"edgements\030\001 \003(\0132 .ibc.core.channel.v1.Pa" +
"cketState\022;\n\npagination\030\002 \001(\0132\'.cosmos.b" +
"ase.query.v1beta1.PageResponse\0220\n\006height" +
"\030\003 \001(\0132\032.ibc.core.client.v1.HeightB\004\310\336\037\000" +
"\"i\n\035QueryUnreceivedPacketsRequest\022\017\n\007por" +
"t_id\030\001 \001(\t\022\022\n\nchannel_id\030\002 \001(\t\022#\n\033packet" +
"_commitment_sequences\030\003 \003(\004\"e\n\036QueryUnre" +
"ceivedPacketsResponse\022\021\n\tsequences\030\001 \003(\004" +
"\0220\n\006height\030\002 \001(\0132\032.ibc.core.client.v1.He" +
"ightB\004\310\336\037\000\"_\n\032QueryUnreceivedAcksRequest" +
"\022\017\n\007port_id\030\001 \001(\t\022\022\n\nchannel_id\030\002 \001(\t\022\034\n" +
"\024packet_ack_sequences\030\003 \003(\004\"b\n\033QueryUnre" +
"ceivedAcksResponse\022\021\n\tsequences\030\001 \003(\004\0220\n" +
"\006height\030\002 \001(\0132\032.ibc.core.client.v1.Heigh" +
"tB\004\310\336\037\000\"F\n\037QueryNextSequenceReceiveReque" +
"st\022\017\n\007port_id\030\001 \001(\t\022\022\n\nchannel_id\030\002 \001(\t\"" +
"\210\001\n QueryNextSequenceReceiveResponse\022\035\n\025" +
"next_sequence_receive\030\001 \001(\004\022\r\n\005proof\030\002 \001" +
"(\014\0226\n\014proof_height\030\003 \001(\0132\032.ibc.core.clie" +
"nt.v1.HeightB\004\310\336\037\0002\317\026\n\005Query\022\247\001\n\007Channel" +
"\022(.ibc.core.channel.v1.QueryChannelReque" +
"st\032).ibc.core.channel.v1.QueryChannelRes" +
"ponse\"G\202\323\344\223\002A\022?/ibc/core/channel/v1beta1" +
"/channels/{channel_id}/ports/{port_id}\022\215" +
"\001\n\010Channels\022).ibc.core.channel.v1.QueryC" +
"hannelsRequest\032*.ibc.core.channel.v1.Que" +
"ryChannelsResponse\"*\202\323\344\223\002$\022\"/ibc/core/ch" +
"annel/v1beta1/channels\022\304\001\n\022ConnectionCha" +
"nnels\0223.ibc.core.channel.v1.QueryConnect" +
"ionChannelsRequest\0324.ibc.core.channel.v1" +
".QueryConnectionChannelsResponse\"C\202\323\344\223\002=" +
"\022;/ibc/core/channel/v1beta1/connections/" +
"{connection}/channels\022\325\001\n\022ChannelClientS" +
"tate\0223.ibc.core.channel.v1.QueryChannelC" +
"lientStateRequest\0324.ibc.core.channel.v1." +
"QueryChannelClientStateResponse\"T\202\323\344\223\002N\022" +
"L/ibc/core/channel/v1beta1/channels/{cha" +
"nnel_id}/ports/{port_id}/client_state\022\230\002" +
"\n\025ChannelConsensusState\0226.ibc.core.chann" +
"el.v1.QueryChannelConsensusStateRequest\032" +
"7.ibc.core.channel.v1.QueryChannelConsen" +
"susStateResponse\"\215\001\202\323\344\223\002\206\001\022\203\001/ibc/core/c" +
"hannel/v1beta1/channels/{channel_id}/por" +
"ts/{port_id}/consensus_state/revision/{r" +
"evision_number}/height/{revision_height}" +
"\022\340\001\n\020PacketCommitment\0221.ibc.core.channel" +
".v1.QueryPacketCommitmentRequest\0322.ibc.c" +
"ore.channel.v1.QueryPacketCommitmentResp" +
"onse\"e\202\323\344\223\002_\022]/ibc/core/channel/v1beta1/" +
"channels/{channel_id}/ports/{port_id}/pa" +
"cket_commitments/{sequence}\022\330\001\n\021PacketCo" +
"mmitments\0222.ibc.core.channel.v1.QueryPac" +
"ketCommitmentsRequest\0323.ibc.core.channel" +
".v1.QueryPacketCommitmentsResponse\"Z\202\323\344\223" +
"\002T\022R/ibc/core/channel/v1beta1/channels/{" +
"channel_id}/ports/{port_id}/packet_commi" +
"tments\022\324\001\n\rPacketReceipt\022..ibc.core.chan" +
"nel.v1.QueryPacketReceiptRequest\032/.ibc.c" +
"ore.channel.v1.QueryPacketReceiptRespons" +
"e\"b\202\323\344\223\002\\\022Z/ibc/core/channel/v1beta1/cha" +
"nnels/{channel_id}/ports/{port_id}/packe" +
"t_receipts/{sequence}\022\350\001\n\025PacketAcknowle" +
"dgement\0226.ibc.core.channel.v1.QueryPacke" +
"tAcknowledgementRequest\0327.ibc.core.chann" +
"el.v1.QueryPacketAcknowledgementResponse" +
"\"^\202\323\344\223\002X\022V/ibc/core/channel/v1beta1/chan" +
"nels/{channel_id}/ports/{port_id}/packet" +
"_acks/{sequence}\022\354\001\n\026PacketAcknowledgeme" +
"nts\0227.ibc.core.channel.v1.QueryPacketAck" +
"nowledgementsRequest\0328.ibc.core.channel." +
"v1.QueryPacketAcknowledgementsResponse\"_" +
"\202\323\344\223\002Y\022W/ibc/core/channel/v1beta1/channe" +
"ls/{channel_id}/ports/{port_id}/packet_a" +
"cknowledgements\022\214\002\n\021UnreceivedPackets\0222." +
"ibc.core.channel.v1.QueryUnreceivedPacke" +
"tsRequest\0323.ibc.core.channel.v1.QueryUnr" +
"eceivedPacketsResponse\"\215\001\202\323\344\223\002\206\001\022\203\001/ibc/" +
"core/channel/v1beta1/channels/{channel_i" +
"d}/ports/{port_id}/packet_commitments/{p" +
"acket_commitment_sequences}/unreceived_p" +
"ackets\022\367\001\n\016UnreceivedAcks\022/.ibc.core.cha" +
"nnel.v1.QueryUnreceivedAcksRequest\0320.ibc" +
".core.channel.v1.QueryUnreceivedAcksResp" +
"onse\"\201\001\202\323\344\223\002{\022y/ibc/core/channel/v1beta1" +
"/channels/{channel_id}/ports/{port_id}/p" +
"acket_commitments/{packet_ack_sequences}" +
"/unreceived_acks\022\331\001\n\023NextSequenceReceive" +
"\0224.ibc.core.channel.v1.QueryNextSequence" +
"ReceiveRequest\0325.ibc.core.channel.v1.Que" +
"ryNextSequenceReceiveResponse\"U\202\323\344\223\002O\022M/" +
"ibc/core/channel/v1beta1/channels/{chann" +
"el_id}/ports/{port_id}/next_sequenceB:Z8" +
"github.com/cosmos/cosmos-sdk/x/ibc/core/" +
"04-channel/typesb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
ibc.core.client.v1.Client.getDescriptor(),
cosmos.base.query.v1beta1.Pagination.getDescriptor(),
ibc.core.channel.v1.ChannelOuterClass.getDescriptor(),
com.google.api.AnnotationsProto.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
com.google.protobuf.GoGoProtos.getDescriptor(),
}, assigner);
internal_static_ibc_core_channel_v1_QueryChannelRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ibc_core_channel_v1_QueryChannelRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", });
internal_static_ibc_core_channel_v1_QueryChannelResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ibc_core_channel_v1_QueryChannelResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelResponse_descriptor,
new java.lang.String[] { "Channel", "Proof", "ProofHeight", });
internal_static_ibc_core_channel_v1_QueryChannelsRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ibc_core_channel_v1_QueryChannelsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelsRequest_descriptor,
new java.lang.String[] { "Pagination", });
internal_static_ibc_core_channel_v1_QueryChannelsResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_ibc_core_channel_v1_QueryChannelsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelsResponse_descriptor,
new java.lang.String[] { "Channels", "Pagination", "Height", });
internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryConnectionChannelsRequest_descriptor,
new java.lang.String[] { "Connection", "Pagination", });
internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryConnectionChannelsResponse_descriptor,
new java.lang.String[] { "Channels", "Pagination", "Height", });
internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelClientStateRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", });
internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelClientStateResponse_descriptor,
new java.lang.String[] { "IdentifiedClientState", "Proof", "ProofHeight", });
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "RevisionNumber", "RevisionHeight", });
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryChannelConsensusStateResponse_descriptor,
new java.lang.String[] { "ConsensusState", "ClientId", "Proof", "ProofHeight", });
internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketCommitmentRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "Sequence", });
internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketCommitmentResponse_descriptor,
new java.lang.String[] { "Commitment", "Proof", "ProofHeight", });
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "Pagination", });
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketCommitmentsResponse_descriptor,
new java.lang.String[] { "Commitments", "Pagination", "Height", });
internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketReceiptRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "Sequence", });
internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketReceiptResponse_descriptor,
new java.lang.String[] { "Received", "Proof", "ProofHeight", });
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "Sequence", });
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementResponse_descriptor,
new java.lang.String[] { "Acknowledgement", "Proof", "ProofHeight", });
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "Pagination", });
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryPacketAcknowledgementsResponse_descriptor,
new java.lang.String[] { "Acknowledgements", "Pagination", "Height", });
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_descriptor =
getDescriptor().getMessageTypes().get(20);
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "PacketCommitmentSequences", });
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_descriptor =
getDescriptor().getMessageTypes().get(21);
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryUnreceivedPacketsResponse_descriptor,
new java.lang.String[] { "Sequences", "Height", });
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_descriptor =
getDescriptor().getMessageTypes().get(22);
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", "PacketAckSequences", });
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_descriptor =
getDescriptor().getMessageTypes().get(23);
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryUnreceivedAcksResponse_descriptor,
new java.lang.String[] { "Sequences", "Height", });
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_descriptor =
getDescriptor().getMessageTypes().get(24);
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveRequest_descriptor,
new java.lang.String[] { "PortId", "ChannelId", });
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_descriptor =
getDescriptor().getMessageTypes().get(25);
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_channel_v1_QueryNextSequenceReceiveResponse_descriptor,
new java.lang.String[] { "NextSequenceReceive", "Proof", "ProofHeight", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.nullable);
registry.add(com.google.api.AnnotationsProto.http);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
ibc.core.client.v1.Client.getDescriptor();
cosmos.base.query.v1beta1.Pagination.getDescriptor();
ibc.core.channel.v1.ChannelOuterClass.getDescriptor();
com.google.api.AnnotationsProto.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
com.google.protobuf.GoGoProtos.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy