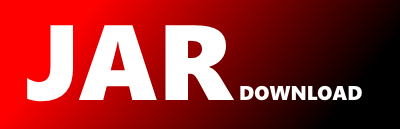
ibc.core.client.v1.Tx Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ibc/core/client/v1/tx.proto
package ibc.core.client.v1;
public final class Tx {
private Tx() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface MsgCreateClientOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgCreateClient)
com.google.protobuf.MessageOrBuilder {
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
boolean hasClientState();
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
com.google.protobuf.Any getClientState();
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
com.google.protobuf.AnyOrBuilder getClientStateOrBuilder();
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
boolean hasConsensusState();
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
com.google.protobuf.Any getConsensusState();
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder();
/**
*
* signer address
*
*
* string signer = 3;
*/
java.lang.String getSigner();
/**
*
* signer address
*
*
* string signer = 3;
*/
com.google.protobuf.ByteString
getSignerBytes();
}
/**
*
* MsgCreateClient defines a message to create an IBC client
*
*
* Protobuf type {@code ibc.core.client.v1.MsgCreateClient}
*/
public static final class MsgCreateClient extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgCreateClient)
MsgCreateClientOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgCreateClient.newBuilder() to construct.
private MsgCreateClient(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgCreateClient() {
signer_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgCreateClient(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Any.Builder subBuilder = null;
if (clientState_ != null) {
subBuilder = clientState_.toBuilder();
}
clientState_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientState_);
clientState_ = subBuilder.buildPartial();
}
break;
}
case 18: {
com.google.protobuf.Any.Builder subBuilder = null;
if (consensusState_ != null) {
subBuilder = consensusState_.toBuilder();
}
consensusState_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(consensusState_);
consensusState_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
signer_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgCreateClient.class, ibc.core.client.v1.Tx.MsgCreateClient.Builder.class);
}
public static final int CLIENT_STATE_FIELD_NUMBER = 1;
private com.google.protobuf.Any clientState_;
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public boolean hasClientState() {
return clientState_ != null;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.Any getClientState() {
return clientState_ == null ? com.google.protobuf.Any.getDefaultInstance() : clientState_;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.AnyOrBuilder getClientStateOrBuilder() {
return getClientState();
}
public static final int CONSENSUS_STATE_FIELD_NUMBER = 2;
private com.google.protobuf.Any consensusState_;
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public boolean hasConsensusState() {
return consensusState_ != null;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.Any getConsensusState() {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
return getConsensusState();
}
public static final int SIGNER_FIELD_NUMBER = 3;
private volatile java.lang.Object signer_;
/**
*
* signer address
*
*
* string signer = 3;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (clientState_ != null) {
output.writeMessage(1, getClientState());
}
if (consensusState_ != null) {
output.writeMessage(2, getConsensusState());
}
if (!getSignerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, signer_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (clientState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getClientState());
}
if (consensusState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getConsensusState());
}
if (!getSignerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, signer_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgCreateClient)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgCreateClient other = (ibc.core.client.v1.Tx.MsgCreateClient) obj;
boolean result = true;
result = result && (hasClientState() == other.hasClientState());
if (hasClientState()) {
result = result && getClientState()
.equals(other.getClientState());
}
result = result && (hasConsensusState() == other.hasConsensusState());
if (hasConsensusState()) {
result = result && getConsensusState()
.equals(other.getConsensusState());
}
result = result && getSigner()
.equals(other.getSigner());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasClientState()) {
hash = (37 * hash) + CLIENT_STATE_FIELD_NUMBER;
hash = (53 * hash) + getClientState().hashCode();
}
if (hasConsensusState()) {
hash = (37 * hash) + CONSENSUS_STATE_FIELD_NUMBER;
hash = (53 * hash) + getConsensusState().hashCode();
}
hash = (37 * hash) + SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getSigner().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgCreateClient parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgCreateClient prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgCreateClient defines a message to create an IBC client
*
*
* Protobuf type {@code ibc.core.client.v1.MsgCreateClient}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgCreateClient)
ibc.core.client.v1.Tx.MsgCreateClientOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgCreateClient.class, ibc.core.client.v1.Tx.MsgCreateClient.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgCreateClient.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (clientStateBuilder_ == null) {
clientState_ = null;
} else {
clientState_ = null;
clientStateBuilder_ = null;
}
if (consensusStateBuilder_ == null) {
consensusState_ = null;
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
signer_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClient_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClient getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgCreateClient.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClient build() {
ibc.core.client.v1.Tx.MsgCreateClient result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClient buildPartial() {
ibc.core.client.v1.Tx.MsgCreateClient result = new ibc.core.client.v1.Tx.MsgCreateClient(this);
if (clientStateBuilder_ == null) {
result.clientState_ = clientState_;
} else {
result.clientState_ = clientStateBuilder_.build();
}
if (consensusStateBuilder_ == null) {
result.consensusState_ = consensusState_;
} else {
result.consensusState_ = consensusStateBuilder_.build();
}
result.signer_ = signer_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgCreateClient) {
return mergeFrom((ibc.core.client.v1.Tx.MsgCreateClient)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgCreateClient other) {
if (other == ibc.core.client.v1.Tx.MsgCreateClient.getDefaultInstance()) return this;
if (other.hasClientState()) {
mergeClientState(other.getClientState());
}
if (other.hasConsensusState()) {
mergeConsensusState(other.getConsensusState());
}
if (!other.getSigner().isEmpty()) {
signer_ = other.signer_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgCreateClient parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgCreateClient) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.Any clientState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> clientStateBuilder_;
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public boolean hasClientState() {
return clientStateBuilder_ != null || clientState_ != null;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.Any getClientState() {
if (clientStateBuilder_ == null) {
return clientState_ == null ? com.google.protobuf.Any.getDefaultInstance() : clientState_;
} else {
return clientStateBuilder_.getMessage();
}
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder setClientState(com.google.protobuf.Any value) {
if (clientStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientState_ = value;
onChanged();
} else {
clientStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder setClientState(
com.google.protobuf.Any.Builder builderForValue) {
if (clientStateBuilder_ == null) {
clientState_ = builderForValue.build();
onChanged();
} else {
clientStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder mergeClientState(com.google.protobuf.Any value) {
if (clientStateBuilder_ == null) {
if (clientState_ != null) {
clientState_ =
com.google.protobuf.Any.newBuilder(clientState_).mergeFrom(value).buildPartial();
} else {
clientState_ = value;
}
onChanged();
} else {
clientStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder clearClientState() {
if (clientStateBuilder_ == null) {
clientState_ = null;
onChanged();
} else {
clientState_ = null;
clientStateBuilder_ = null;
}
return this;
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.Any.Builder getClientStateBuilder() {
onChanged();
return getClientStateFieldBuilder().getBuilder();
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.AnyOrBuilder getClientStateOrBuilder() {
if (clientStateBuilder_ != null) {
return clientStateBuilder_.getMessageOrBuilder();
} else {
return clientState_ == null ?
com.google.protobuf.Any.getDefaultInstance() : clientState_;
}
}
/**
*
* light client state
*
*
* .google.protobuf.Any client_state = 1 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getClientStateFieldBuilder() {
if (clientStateBuilder_ == null) {
clientStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getClientState(),
getParentForChildren(),
isClean());
clientState_ = null;
}
return clientStateBuilder_;
}
private com.google.protobuf.Any consensusState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> consensusStateBuilder_;
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public boolean hasConsensusState() {
return consensusStateBuilder_ != null || consensusState_ != null;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.Any getConsensusState() {
if (consensusStateBuilder_ == null) {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
} else {
return consensusStateBuilder_.getMessage();
}
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder setConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consensusState_ = value;
onChanged();
} else {
consensusStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder setConsensusState(
com.google.protobuf.Any.Builder builderForValue) {
if (consensusStateBuilder_ == null) {
consensusState_ = builderForValue.build();
onChanged();
} else {
consensusStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder mergeConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (consensusState_ != null) {
consensusState_ =
com.google.protobuf.Any.newBuilder(consensusState_).mergeFrom(value).buildPartial();
} else {
consensusState_ = value;
}
onChanged();
} else {
consensusStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder clearConsensusState() {
if (consensusStateBuilder_ == null) {
consensusState_ = null;
onChanged();
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
return this;
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.Any.Builder getConsensusStateBuilder() {
onChanged();
return getConsensusStateFieldBuilder().getBuilder();
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
if (consensusStateBuilder_ != null) {
return consensusStateBuilder_.getMessageOrBuilder();
} else {
return consensusState_ == null ?
com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
}
/**
*
* consensus state associated with the client that corresponds to a given
* height.
*
*
* .google.protobuf.Any consensus_state = 2 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getConsensusStateFieldBuilder() {
if (consensusStateBuilder_ == null) {
consensusStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getConsensusState(),
getParentForChildren(),
isClean());
consensusState_ = null;
}
return consensusStateBuilder_;
}
private java.lang.Object signer_ = "";
/**
*
* signer address
*
*
* string signer = 3;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder setSigner(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
signer_ = value;
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder clearSigner() {
signer_ = getDefaultInstance().getSigner();
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder setSignerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
signer_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgCreateClient)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgCreateClient)
private static final ibc.core.client.v1.Tx.MsgCreateClient DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgCreateClient();
}
public static ibc.core.client.v1.Tx.MsgCreateClient getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgCreateClient parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgCreateClient(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClient getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgCreateClientResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgCreateClientResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgCreateClientResponse defines the Msg/CreateClient response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgCreateClientResponse}
*/
public static final class MsgCreateClientResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgCreateClientResponse)
MsgCreateClientResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgCreateClientResponse.newBuilder() to construct.
private MsgCreateClientResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgCreateClientResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgCreateClientResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClientResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClientResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgCreateClientResponse.class, ibc.core.client.v1.Tx.MsgCreateClientResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgCreateClientResponse)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgCreateClientResponse other = (ibc.core.client.v1.Tx.MsgCreateClientResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgCreateClientResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgCreateClientResponse defines the Msg/CreateClient response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgCreateClientResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgCreateClientResponse)
ibc.core.client.v1.Tx.MsgCreateClientResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClientResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClientResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgCreateClientResponse.class, ibc.core.client.v1.Tx.MsgCreateClientResponse.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgCreateClientResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgCreateClientResponse_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClientResponse getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgCreateClientResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClientResponse build() {
ibc.core.client.v1.Tx.MsgCreateClientResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClientResponse buildPartial() {
ibc.core.client.v1.Tx.MsgCreateClientResponse result = new ibc.core.client.v1.Tx.MsgCreateClientResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgCreateClientResponse) {
return mergeFrom((ibc.core.client.v1.Tx.MsgCreateClientResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgCreateClientResponse other) {
if (other == ibc.core.client.v1.Tx.MsgCreateClientResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgCreateClientResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgCreateClientResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgCreateClientResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgCreateClientResponse)
private static final ibc.core.client.v1.Tx.MsgCreateClientResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgCreateClientResponse();
}
public static ibc.core.client.v1.Tx.MsgCreateClientResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgCreateClientResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgCreateClientResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgCreateClientResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgUpdateClientOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgUpdateClient)
com.google.protobuf.MessageOrBuilder {
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
java.lang.String getClientId();
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
boolean hasHeader();
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
com.google.protobuf.Any getHeader();
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
com.google.protobuf.AnyOrBuilder getHeaderOrBuilder();
/**
*
* signer address
*
*
* string signer = 3;
*/
java.lang.String getSigner();
/**
*
* signer address
*
*
* string signer = 3;
*/
com.google.protobuf.ByteString
getSignerBytes();
}
/**
*
* MsgUpdateClient defines an sdk.Msg to update a IBC client state using
* the given header.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpdateClient}
*/
public static final class MsgUpdateClient extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgUpdateClient)
MsgUpdateClientOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgUpdateClient.newBuilder() to construct.
private MsgUpdateClient(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgUpdateClient() {
clientId_ = "";
signer_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgUpdateClient(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
case 18: {
com.google.protobuf.Any.Builder subBuilder = null;
if (header_ != null) {
subBuilder = header_.toBuilder();
}
header_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(header_);
header_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
signer_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpdateClient.class, ibc.core.client.v1.Tx.MsgUpdateClient.Builder.class);
}
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object clientId_;
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HEADER_FIELD_NUMBER = 2;
private com.google.protobuf.Any header_;
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public boolean hasHeader() {
return header_ != null;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public com.google.protobuf.Any getHeader() {
return header_ == null ? com.google.protobuf.Any.getDefaultInstance() : header_;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public com.google.protobuf.AnyOrBuilder getHeaderOrBuilder() {
return getHeader();
}
public static final int SIGNER_FIELD_NUMBER = 3;
private volatile java.lang.Object signer_;
/**
*
* signer address
*
*
* string signer = 3;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getClientIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, clientId_);
}
if (header_ != null) {
output.writeMessage(2, getHeader());
}
if (!getSignerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, signer_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getClientIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, clientId_);
}
if (header_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getHeader());
}
if (!getSignerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, signer_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgUpdateClient)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgUpdateClient other = (ibc.core.client.v1.Tx.MsgUpdateClient) obj;
boolean result = true;
result = result && getClientId()
.equals(other.getClientId());
result = result && (hasHeader() == other.hasHeader());
if (hasHeader()) {
result = result && getHeader()
.equals(other.getHeader());
}
result = result && getSigner()
.equals(other.getSigner());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
if (hasHeader()) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeader().hashCode();
}
hash = (37 * hash) + SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getSigner().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpdateClient parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgUpdateClient prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgUpdateClient defines an sdk.Msg to update a IBC client state using
* the given header.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpdateClient}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgUpdateClient)
ibc.core.client.v1.Tx.MsgUpdateClientOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpdateClient.class, ibc.core.client.v1.Tx.MsgUpdateClient.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgUpdateClient.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
clientId_ = "";
if (headerBuilder_ == null) {
header_ = null;
} else {
header_ = null;
headerBuilder_ = null;
}
signer_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClient_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClient getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgUpdateClient.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClient build() {
ibc.core.client.v1.Tx.MsgUpdateClient result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClient buildPartial() {
ibc.core.client.v1.Tx.MsgUpdateClient result = new ibc.core.client.v1.Tx.MsgUpdateClient(this);
result.clientId_ = clientId_;
if (headerBuilder_ == null) {
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
result.signer_ = signer_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgUpdateClient) {
return mergeFrom((ibc.core.client.v1.Tx.MsgUpdateClient)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgUpdateClient other) {
if (other == ibc.core.client.v1.Tx.MsgUpdateClient.getDefaultInstance()) return this;
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (other.hasHeader()) {
mergeHeader(other.getHeader());
}
if (!other.getSigner().isEmpty()) {
signer_ = other.signer_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgUpdateClient parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgUpdateClient) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object clientId_ = "";
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private com.google.protobuf.Any header_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> headerBuilder_;
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public boolean hasHeader() {
return headerBuilder_ != null || header_ != null;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public com.google.protobuf.Any getHeader() {
if (headerBuilder_ == null) {
return header_ == null ? com.google.protobuf.Any.getDefaultInstance() : header_;
} else {
return headerBuilder_.getMessage();
}
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public Builder setHeader(com.google.protobuf.Any value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
header_ = value;
onChanged();
} else {
headerBuilder_.setMessage(value);
}
return this;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public Builder setHeader(
com.google.protobuf.Any.Builder builderForValue) {
if (headerBuilder_ == null) {
header_ = builderForValue.build();
onChanged();
} else {
headerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public Builder mergeHeader(com.google.protobuf.Any value) {
if (headerBuilder_ == null) {
if (header_ != null) {
header_ =
com.google.protobuf.Any.newBuilder(header_).mergeFrom(value).buildPartial();
} else {
header_ = value;
}
onChanged();
} else {
headerBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = null;
onChanged();
} else {
header_ = null;
headerBuilder_ = null;
}
return this;
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public com.google.protobuf.Any.Builder getHeaderBuilder() {
onChanged();
return getHeaderFieldBuilder().getBuilder();
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
public com.google.protobuf.AnyOrBuilder getHeaderOrBuilder() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilder();
} else {
return header_ == null ?
com.google.protobuf.Any.getDefaultInstance() : header_;
}
}
/**
*
* header to update the light client
*
*
* .google.protobuf.Any header = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getHeader(),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private java.lang.Object signer_ = "";
/**
*
* signer address
*
*
* string signer = 3;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder setSigner(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
signer_ = value;
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder clearSigner() {
signer_ = getDefaultInstance().getSigner();
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder setSignerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
signer_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgUpdateClient)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgUpdateClient)
private static final ibc.core.client.v1.Tx.MsgUpdateClient DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgUpdateClient();
}
public static ibc.core.client.v1.Tx.MsgUpdateClient getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgUpdateClient parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgUpdateClient(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClient getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgUpdateClientResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgUpdateClientResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgUpdateClientResponse defines the Msg/UpdateClient response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpdateClientResponse}
*/
public static final class MsgUpdateClientResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgUpdateClientResponse)
MsgUpdateClientResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgUpdateClientResponse.newBuilder() to construct.
private MsgUpdateClientResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgUpdateClientResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgUpdateClientResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClientResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClientResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpdateClientResponse.class, ibc.core.client.v1.Tx.MsgUpdateClientResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgUpdateClientResponse)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgUpdateClientResponse other = (ibc.core.client.v1.Tx.MsgUpdateClientResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgUpdateClientResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgUpdateClientResponse defines the Msg/UpdateClient response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpdateClientResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgUpdateClientResponse)
ibc.core.client.v1.Tx.MsgUpdateClientResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClientResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClientResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpdateClientResponse.class, ibc.core.client.v1.Tx.MsgUpdateClientResponse.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgUpdateClientResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpdateClientResponse_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClientResponse getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgUpdateClientResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClientResponse build() {
ibc.core.client.v1.Tx.MsgUpdateClientResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClientResponse buildPartial() {
ibc.core.client.v1.Tx.MsgUpdateClientResponse result = new ibc.core.client.v1.Tx.MsgUpdateClientResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgUpdateClientResponse) {
return mergeFrom((ibc.core.client.v1.Tx.MsgUpdateClientResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgUpdateClientResponse other) {
if (other == ibc.core.client.v1.Tx.MsgUpdateClientResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgUpdateClientResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgUpdateClientResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgUpdateClientResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgUpdateClientResponse)
private static final ibc.core.client.v1.Tx.MsgUpdateClientResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgUpdateClientResponse();
}
public static ibc.core.client.v1.Tx.MsgUpdateClientResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgUpdateClientResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgUpdateClientResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpdateClientResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgUpgradeClientOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgUpgradeClient)
com.google.protobuf.MessageOrBuilder {
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
java.lang.String getClientId();
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
boolean hasClientState();
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
com.google.protobuf.Any getClientState();
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
com.google.protobuf.AnyOrBuilder getClientStateOrBuilder();
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
boolean hasConsensusState();
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
com.google.protobuf.Any getConsensusState();
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder();
/**
*
* proof that old chain committed to new client
*
*
* bytes proof_upgrade_client = 4 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_client\""];
*/
com.google.protobuf.ByteString getProofUpgradeClient();
/**
*
* proof that old chain committed to new consensus state
*
*
* bytes proof_upgrade_consensus_state = 5 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_consensus_state\""];
*/
com.google.protobuf.ByteString getProofUpgradeConsensusState();
/**
*
* signer address
*
*
* string signer = 6;
*/
java.lang.String getSigner();
/**
*
* signer address
*
*
* string signer = 6;
*/
com.google.protobuf.ByteString
getSignerBytes();
}
/**
*
* MsgUpgradeClient defines an sdk.Msg to upgrade an IBC client to a new client state
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpgradeClient}
*/
public static final class MsgUpgradeClient extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgUpgradeClient)
MsgUpgradeClientOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgUpgradeClient.newBuilder() to construct.
private MsgUpgradeClient(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgUpgradeClient() {
clientId_ = "";
proofUpgradeClient_ = com.google.protobuf.ByteString.EMPTY;
proofUpgradeConsensusState_ = com.google.protobuf.ByteString.EMPTY;
signer_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgUpgradeClient(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
case 18: {
com.google.protobuf.Any.Builder subBuilder = null;
if (clientState_ != null) {
subBuilder = clientState_.toBuilder();
}
clientState_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientState_);
clientState_ = subBuilder.buildPartial();
}
break;
}
case 26: {
com.google.protobuf.Any.Builder subBuilder = null;
if (consensusState_ != null) {
subBuilder = consensusState_.toBuilder();
}
consensusState_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(consensusState_);
consensusState_ = subBuilder.buildPartial();
}
break;
}
case 34: {
proofUpgradeClient_ = input.readBytes();
break;
}
case 42: {
proofUpgradeConsensusState_ = input.readBytes();
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
signer_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpgradeClient.class, ibc.core.client.v1.Tx.MsgUpgradeClient.Builder.class);
}
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object clientId_;
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_STATE_FIELD_NUMBER = 2;
private com.google.protobuf.Any clientState_;
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public boolean hasClientState() {
return clientState_ != null;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.Any getClientState() {
return clientState_ == null ? com.google.protobuf.Any.getDefaultInstance() : clientState_;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.AnyOrBuilder getClientStateOrBuilder() {
return getClientState();
}
public static final int CONSENSUS_STATE_FIELD_NUMBER = 3;
private com.google.protobuf.Any consensusState_;
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public boolean hasConsensusState() {
return consensusState_ != null;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.Any getConsensusState() {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
return getConsensusState();
}
public static final int PROOF_UPGRADE_CLIENT_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString proofUpgradeClient_;
/**
*
* proof that old chain committed to new client
*
*
* bytes proof_upgrade_client = 4 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_client\""];
*/
public com.google.protobuf.ByteString getProofUpgradeClient() {
return proofUpgradeClient_;
}
public static final int PROOF_UPGRADE_CONSENSUS_STATE_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString proofUpgradeConsensusState_;
/**
*
* proof that old chain committed to new consensus state
*
*
* bytes proof_upgrade_consensus_state = 5 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_consensus_state\""];
*/
public com.google.protobuf.ByteString getProofUpgradeConsensusState() {
return proofUpgradeConsensusState_;
}
public static final int SIGNER_FIELD_NUMBER = 6;
private volatile java.lang.Object signer_;
/**
*
* signer address
*
*
* string signer = 6;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
}
}
/**
*
* signer address
*
*
* string signer = 6;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getClientIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, clientId_);
}
if (clientState_ != null) {
output.writeMessage(2, getClientState());
}
if (consensusState_ != null) {
output.writeMessage(3, getConsensusState());
}
if (!proofUpgradeClient_.isEmpty()) {
output.writeBytes(4, proofUpgradeClient_);
}
if (!proofUpgradeConsensusState_.isEmpty()) {
output.writeBytes(5, proofUpgradeConsensusState_);
}
if (!getSignerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, signer_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getClientIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, clientId_);
}
if (clientState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getClientState());
}
if (consensusState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getConsensusState());
}
if (!proofUpgradeClient_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, proofUpgradeClient_);
}
if (!proofUpgradeConsensusState_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, proofUpgradeConsensusState_);
}
if (!getSignerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, signer_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgUpgradeClient)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgUpgradeClient other = (ibc.core.client.v1.Tx.MsgUpgradeClient) obj;
boolean result = true;
result = result && getClientId()
.equals(other.getClientId());
result = result && (hasClientState() == other.hasClientState());
if (hasClientState()) {
result = result && getClientState()
.equals(other.getClientState());
}
result = result && (hasConsensusState() == other.hasConsensusState());
if (hasConsensusState()) {
result = result && getConsensusState()
.equals(other.getConsensusState());
}
result = result && getProofUpgradeClient()
.equals(other.getProofUpgradeClient());
result = result && getProofUpgradeConsensusState()
.equals(other.getProofUpgradeConsensusState());
result = result && getSigner()
.equals(other.getSigner());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
if (hasClientState()) {
hash = (37 * hash) + CLIENT_STATE_FIELD_NUMBER;
hash = (53 * hash) + getClientState().hashCode();
}
if (hasConsensusState()) {
hash = (37 * hash) + CONSENSUS_STATE_FIELD_NUMBER;
hash = (53 * hash) + getConsensusState().hashCode();
}
hash = (37 * hash) + PROOF_UPGRADE_CLIENT_FIELD_NUMBER;
hash = (53 * hash) + getProofUpgradeClient().hashCode();
hash = (37 * hash) + PROOF_UPGRADE_CONSENSUS_STATE_FIELD_NUMBER;
hash = (53 * hash) + getProofUpgradeConsensusState().hashCode();
hash = (37 * hash) + SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getSigner().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgUpgradeClient prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgUpgradeClient defines an sdk.Msg to upgrade an IBC client to a new client state
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpgradeClient}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgUpgradeClient)
ibc.core.client.v1.Tx.MsgUpgradeClientOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpgradeClient.class, ibc.core.client.v1.Tx.MsgUpgradeClient.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgUpgradeClient.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
clientId_ = "";
if (clientStateBuilder_ == null) {
clientState_ = null;
} else {
clientState_ = null;
clientStateBuilder_ = null;
}
if (consensusStateBuilder_ == null) {
consensusState_ = null;
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
proofUpgradeClient_ = com.google.protobuf.ByteString.EMPTY;
proofUpgradeConsensusState_ = com.google.protobuf.ByteString.EMPTY;
signer_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClient_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClient getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgUpgradeClient.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClient build() {
ibc.core.client.v1.Tx.MsgUpgradeClient result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClient buildPartial() {
ibc.core.client.v1.Tx.MsgUpgradeClient result = new ibc.core.client.v1.Tx.MsgUpgradeClient(this);
result.clientId_ = clientId_;
if (clientStateBuilder_ == null) {
result.clientState_ = clientState_;
} else {
result.clientState_ = clientStateBuilder_.build();
}
if (consensusStateBuilder_ == null) {
result.consensusState_ = consensusState_;
} else {
result.consensusState_ = consensusStateBuilder_.build();
}
result.proofUpgradeClient_ = proofUpgradeClient_;
result.proofUpgradeConsensusState_ = proofUpgradeConsensusState_;
result.signer_ = signer_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgUpgradeClient) {
return mergeFrom((ibc.core.client.v1.Tx.MsgUpgradeClient)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgUpgradeClient other) {
if (other == ibc.core.client.v1.Tx.MsgUpgradeClient.getDefaultInstance()) return this;
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (other.hasClientState()) {
mergeClientState(other.getClientState());
}
if (other.hasConsensusState()) {
mergeConsensusState(other.getConsensusState());
}
if (other.getProofUpgradeClient() != com.google.protobuf.ByteString.EMPTY) {
setProofUpgradeClient(other.getProofUpgradeClient());
}
if (other.getProofUpgradeConsensusState() != com.google.protobuf.ByteString.EMPTY) {
setProofUpgradeConsensusState(other.getProofUpgradeConsensusState());
}
if (!other.getSigner().isEmpty()) {
signer_ = other.signer_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgUpgradeClient parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgUpgradeClient) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object clientId_ = "";
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private com.google.protobuf.Any clientState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> clientStateBuilder_;
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public boolean hasClientState() {
return clientStateBuilder_ != null || clientState_ != null;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.Any getClientState() {
if (clientStateBuilder_ == null) {
return clientState_ == null ? com.google.protobuf.Any.getDefaultInstance() : clientState_;
} else {
return clientStateBuilder_.getMessage();
}
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder setClientState(com.google.protobuf.Any value) {
if (clientStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientState_ = value;
onChanged();
} else {
clientStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder setClientState(
com.google.protobuf.Any.Builder builderForValue) {
if (clientStateBuilder_ == null) {
clientState_ = builderForValue.build();
onChanged();
} else {
clientStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder mergeClientState(com.google.protobuf.Any value) {
if (clientStateBuilder_ == null) {
if (clientState_ != null) {
clientState_ =
com.google.protobuf.Any.newBuilder(clientState_).mergeFrom(value).buildPartial();
} else {
clientState_ = value;
}
onChanged();
} else {
clientStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public Builder clearClientState() {
if (clientStateBuilder_ == null) {
clientState_ = null;
onChanged();
} else {
clientState_ = null;
clientStateBuilder_ = null;
}
return this;
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.Any.Builder getClientStateBuilder() {
onChanged();
return getClientStateFieldBuilder().getBuilder();
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
public com.google.protobuf.AnyOrBuilder getClientStateOrBuilder() {
if (clientStateBuilder_ != null) {
return clientStateBuilder_.getMessageOrBuilder();
} else {
return clientState_ == null ?
com.google.protobuf.Any.getDefaultInstance() : clientState_;
}
}
/**
*
* upgraded client state
*
*
* .google.protobuf.Any client_state = 2 [(.gogoproto.moretags) = "yaml:\"client_state\""];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getClientStateFieldBuilder() {
if (clientStateBuilder_ == null) {
clientStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getClientState(),
getParentForChildren(),
isClean());
clientState_ = null;
}
return clientStateBuilder_;
}
private com.google.protobuf.Any consensusState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> consensusStateBuilder_;
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public boolean hasConsensusState() {
return consensusStateBuilder_ != null || consensusState_ != null;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.Any getConsensusState() {
if (consensusStateBuilder_ == null) {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
} else {
return consensusStateBuilder_.getMessage();
}
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder setConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consensusState_ = value;
onChanged();
} else {
consensusStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder setConsensusState(
com.google.protobuf.Any.Builder builderForValue) {
if (consensusStateBuilder_ == null) {
consensusState_ = builderForValue.build();
onChanged();
} else {
consensusStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder mergeConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (consensusState_ != null) {
consensusState_ =
com.google.protobuf.Any.newBuilder(consensusState_).mergeFrom(value).buildPartial();
} else {
consensusState_ = value;
}
onChanged();
} else {
consensusStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public Builder clearConsensusState() {
if (consensusStateBuilder_ == null) {
consensusState_ = null;
onChanged();
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
return this;
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.Any.Builder getConsensusStateBuilder() {
onChanged();
return getConsensusStateFieldBuilder().getBuilder();
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
if (consensusStateBuilder_ != null) {
return consensusStateBuilder_.getMessageOrBuilder();
} else {
return consensusState_ == null ?
com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
}
/**
*
* upgraded consensus state, only contains enough information to serve as a basis of trust in update logic
*
*
* .google.protobuf.Any consensus_state = 3 [(.gogoproto.moretags) = "yaml:\"consensus_state\""];
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getConsensusStateFieldBuilder() {
if (consensusStateBuilder_ == null) {
consensusStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getConsensusState(),
getParentForChildren(),
isClean());
consensusState_ = null;
}
return consensusStateBuilder_;
}
private com.google.protobuf.ByteString proofUpgradeClient_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* proof that old chain committed to new client
*
*
* bytes proof_upgrade_client = 4 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_client\""];
*/
public com.google.protobuf.ByteString getProofUpgradeClient() {
return proofUpgradeClient_;
}
/**
*
* proof that old chain committed to new client
*
*
* bytes proof_upgrade_client = 4 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_client\""];
*/
public Builder setProofUpgradeClient(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proofUpgradeClient_ = value;
onChanged();
return this;
}
/**
*
* proof that old chain committed to new client
*
*
* bytes proof_upgrade_client = 4 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_client\""];
*/
public Builder clearProofUpgradeClient() {
proofUpgradeClient_ = getDefaultInstance().getProofUpgradeClient();
onChanged();
return this;
}
private com.google.protobuf.ByteString proofUpgradeConsensusState_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* proof that old chain committed to new consensus state
*
*
* bytes proof_upgrade_consensus_state = 5 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_consensus_state\""];
*/
public com.google.protobuf.ByteString getProofUpgradeConsensusState() {
return proofUpgradeConsensusState_;
}
/**
*
* proof that old chain committed to new consensus state
*
*
* bytes proof_upgrade_consensus_state = 5 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_consensus_state\""];
*/
public Builder setProofUpgradeConsensusState(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proofUpgradeConsensusState_ = value;
onChanged();
return this;
}
/**
*
* proof that old chain committed to new consensus state
*
*
* bytes proof_upgrade_consensus_state = 5 [(.gogoproto.moretags) = "yaml:\"proof_upgrade_consensus_state\""];
*/
public Builder clearProofUpgradeConsensusState() {
proofUpgradeConsensusState_ = getDefaultInstance().getProofUpgradeConsensusState();
onChanged();
return this;
}
private java.lang.Object signer_ = "";
/**
*
* signer address
*
*
* string signer = 6;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* signer address
*
*
* string signer = 6;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* signer address
*
*
* string signer = 6;
*/
public Builder setSigner(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
signer_ = value;
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 6;
*/
public Builder clearSigner() {
signer_ = getDefaultInstance().getSigner();
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 6;
*/
public Builder setSignerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
signer_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgUpgradeClient)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgUpgradeClient)
private static final ibc.core.client.v1.Tx.MsgUpgradeClient DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgUpgradeClient();
}
public static ibc.core.client.v1.Tx.MsgUpgradeClient getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgUpgradeClient parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgUpgradeClient(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClient getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgUpgradeClientResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgUpgradeClientResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgUpgradeClientResponse defines the Msg/UpgradeClient response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpgradeClientResponse}
*/
public static final class MsgUpgradeClientResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgUpgradeClientResponse)
MsgUpgradeClientResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgUpgradeClientResponse.newBuilder() to construct.
private MsgUpgradeClientResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgUpgradeClientResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgUpgradeClientResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpgradeClientResponse.class, ibc.core.client.v1.Tx.MsgUpgradeClientResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgUpgradeClientResponse)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgUpgradeClientResponse other = (ibc.core.client.v1.Tx.MsgUpgradeClientResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgUpgradeClientResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgUpgradeClientResponse defines the Msg/UpgradeClient response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgUpgradeClientResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgUpgradeClientResponse)
ibc.core.client.v1.Tx.MsgUpgradeClientResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgUpgradeClientResponse.class, ibc.core.client.v1.Tx.MsgUpgradeClientResponse.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgUpgradeClientResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClientResponse getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgUpgradeClientResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClientResponse build() {
ibc.core.client.v1.Tx.MsgUpgradeClientResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClientResponse buildPartial() {
ibc.core.client.v1.Tx.MsgUpgradeClientResponse result = new ibc.core.client.v1.Tx.MsgUpgradeClientResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgUpgradeClientResponse) {
return mergeFrom((ibc.core.client.v1.Tx.MsgUpgradeClientResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgUpgradeClientResponse other) {
if (other == ibc.core.client.v1.Tx.MsgUpgradeClientResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgUpgradeClientResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgUpgradeClientResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgUpgradeClientResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgUpgradeClientResponse)
private static final ibc.core.client.v1.Tx.MsgUpgradeClientResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgUpgradeClientResponse();
}
public static ibc.core.client.v1.Tx.MsgUpgradeClientResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgUpgradeClientResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgUpgradeClientResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgUpgradeClientResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgSubmitMisbehaviourOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgSubmitMisbehaviour)
com.google.protobuf.MessageOrBuilder {
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
java.lang.String getClientId();
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
boolean hasMisbehaviour();
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
com.google.protobuf.Any getMisbehaviour();
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
com.google.protobuf.AnyOrBuilder getMisbehaviourOrBuilder();
/**
*
* signer address
*
*
* string signer = 3;
*/
java.lang.String getSigner();
/**
*
* signer address
*
*
* string signer = 3;
*/
com.google.protobuf.ByteString
getSignerBytes();
}
/**
*
* MsgSubmitMisbehaviour defines an sdk.Msg type that submits Evidence for
* light client misbehaviour.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgSubmitMisbehaviour}
*/
public static final class MsgSubmitMisbehaviour extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgSubmitMisbehaviour)
MsgSubmitMisbehaviourOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgSubmitMisbehaviour.newBuilder() to construct.
private MsgSubmitMisbehaviour(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgSubmitMisbehaviour() {
clientId_ = "";
signer_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgSubmitMisbehaviour(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
case 18: {
com.google.protobuf.Any.Builder subBuilder = null;
if (misbehaviour_ != null) {
subBuilder = misbehaviour_.toBuilder();
}
misbehaviour_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(misbehaviour_);
misbehaviour_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
signer_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.class, ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.Builder.class);
}
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object clientId_;
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MISBEHAVIOUR_FIELD_NUMBER = 2;
private com.google.protobuf.Any misbehaviour_;
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public boolean hasMisbehaviour() {
return misbehaviour_ != null;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public com.google.protobuf.Any getMisbehaviour() {
return misbehaviour_ == null ? com.google.protobuf.Any.getDefaultInstance() : misbehaviour_;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public com.google.protobuf.AnyOrBuilder getMisbehaviourOrBuilder() {
return getMisbehaviour();
}
public static final int SIGNER_FIELD_NUMBER = 3;
private volatile java.lang.Object signer_;
/**
*
* signer address
*
*
* string signer = 3;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getClientIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, clientId_);
}
if (misbehaviour_ != null) {
output.writeMessage(2, getMisbehaviour());
}
if (!getSignerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, signer_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getClientIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, clientId_);
}
if (misbehaviour_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getMisbehaviour());
}
if (!getSignerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, signer_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgSubmitMisbehaviour)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgSubmitMisbehaviour other = (ibc.core.client.v1.Tx.MsgSubmitMisbehaviour) obj;
boolean result = true;
result = result && getClientId()
.equals(other.getClientId());
result = result && (hasMisbehaviour() == other.hasMisbehaviour());
if (hasMisbehaviour()) {
result = result && getMisbehaviour()
.equals(other.getMisbehaviour());
}
result = result && getSigner()
.equals(other.getSigner());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
if (hasMisbehaviour()) {
hash = (37 * hash) + MISBEHAVIOUR_FIELD_NUMBER;
hash = (53 * hash) + getMisbehaviour().hashCode();
}
hash = (37 * hash) + SIGNER_FIELD_NUMBER;
hash = (53 * hash) + getSigner().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgSubmitMisbehaviour prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgSubmitMisbehaviour defines an sdk.Msg type that submits Evidence for
* light client misbehaviour.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgSubmitMisbehaviour}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgSubmitMisbehaviour)
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.class, ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
clientId_ = "";
if (misbehaviourBuilder_ == null) {
misbehaviour_ = null;
} else {
misbehaviour_ = null;
misbehaviourBuilder_ = null;
}
signer_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviour getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviour build() {
ibc.core.client.v1.Tx.MsgSubmitMisbehaviour result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviour buildPartial() {
ibc.core.client.v1.Tx.MsgSubmitMisbehaviour result = new ibc.core.client.v1.Tx.MsgSubmitMisbehaviour(this);
result.clientId_ = clientId_;
if (misbehaviourBuilder_ == null) {
result.misbehaviour_ = misbehaviour_;
} else {
result.misbehaviour_ = misbehaviourBuilder_.build();
}
result.signer_ = signer_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgSubmitMisbehaviour) {
return mergeFrom((ibc.core.client.v1.Tx.MsgSubmitMisbehaviour)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgSubmitMisbehaviour other) {
if (other == ibc.core.client.v1.Tx.MsgSubmitMisbehaviour.getDefaultInstance()) return this;
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (other.hasMisbehaviour()) {
mergeMisbehaviour(other.getMisbehaviour());
}
if (!other.getSigner().isEmpty()) {
signer_ = other.signer_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgSubmitMisbehaviour parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgSubmitMisbehaviour) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object clientId_ = "";
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
* client unique identifier
*
*
* string client_id = 1 [(.gogoproto.moretags) = "yaml:\"client_id\""];
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private com.google.protobuf.Any misbehaviour_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> misbehaviourBuilder_;
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public boolean hasMisbehaviour() {
return misbehaviourBuilder_ != null || misbehaviour_ != null;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public com.google.protobuf.Any getMisbehaviour() {
if (misbehaviourBuilder_ == null) {
return misbehaviour_ == null ? com.google.protobuf.Any.getDefaultInstance() : misbehaviour_;
} else {
return misbehaviourBuilder_.getMessage();
}
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public Builder setMisbehaviour(com.google.protobuf.Any value) {
if (misbehaviourBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
misbehaviour_ = value;
onChanged();
} else {
misbehaviourBuilder_.setMessage(value);
}
return this;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public Builder setMisbehaviour(
com.google.protobuf.Any.Builder builderForValue) {
if (misbehaviourBuilder_ == null) {
misbehaviour_ = builderForValue.build();
onChanged();
} else {
misbehaviourBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public Builder mergeMisbehaviour(com.google.protobuf.Any value) {
if (misbehaviourBuilder_ == null) {
if (misbehaviour_ != null) {
misbehaviour_ =
com.google.protobuf.Any.newBuilder(misbehaviour_).mergeFrom(value).buildPartial();
} else {
misbehaviour_ = value;
}
onChanged();
} else {
misbehaviourBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public Builder clearMisbehaviour() {
if (misbehaviourBuilder_ == null) {
misbehaviour_ = null;
onChanged();
} else {
misbehaviour_ = null;
misbehaviourBuilder_ = null;
}
return this;
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public com.google.protobuf.Any.Builder getMisbehaviourBuilder() {
onChanged();
return getMisbehaviourFieldBuilder().getBuilder();
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
public com.google.protobuf.AnyOrBuilder getMisbehaviourOrBuilder() {
if (misbehaviourBuilder_ != null) {
return misbehaviourBuilder_.getMessageOrBuilder();
} else {
return misbehaviour_ == null ?
com.google.protobuf.Any.getDefaultInstance() : misbehaviour_;
}
}
/**
*
* misbehaviour used for freezing the light client
*
*
* .google.protobuf.Any misbehaviour = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getMisbehaviourFieldBuilder() {
if (misbehaviourBuilder_ == null) {
misbehaviourBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getMisbehaviour(),
getParentForChildren(),
isClean());
misbehaviour_ = null;
}
return misbehaviourBuilder_;
}
private java.lang.Object signer_ = "";
/**
*
* signer address
*
*
* string signer = 3;
*/
public java.lang.String getSigner() {
java.lang.Object ref = signer_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
signer_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public com.google.protobuf.ByteString
getSignerBytes() {
java.lang.Object ref = signer_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
signer_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder setSigner(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
signer_ = value;
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder clearSigner() {
signer_ = getDefaultInstance().getSigner();
onChanged();
return this;
}
/**
*
* signer address
*
*
* string signer = 3;
*/
public Builder setSignerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
signer_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgSubmitMisbehaviour)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgSubmitMisbehaviour)
private static final ibc.core.client.v1.Tx.MsgSubmitMisbehaviour DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgSubmitMisbehaviour();
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviour getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgSubmitMisbehaviour parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgSubmitMisbehaviour(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviour getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MsgSubmitMisbehaviourResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.client.v1.MsgSubmitMisbehaviourResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* MsgSubmitMisbehaviourResponse defines the Msg/SubmitMisbehaviour response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgSubmitMisbehaviourResponse}
*/
public static final class MsgSubmitMisbehaviourResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.client.v1.MsgSubmitMisbehaviourResponse)
MsgSubmitMisbehaviourResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MsgSubmitMisbehaviourResponse.newBuilder() to construct.
private MsgSubmitMisbehaviourResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MsgSubmitMisbehaviourResponse() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MsgSubmitMisbehaviourResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.class, ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse)) {
return super.equals(obj);
}
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse other = (ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse) obj;
boolean result = true;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MsgSubmitMisbehaviourResponse defines the Msg/SubmitMisbehaviour response type.
*
*
* Protobuf type {@code ibc.core.client.v1.MsgSubmitMisbehaviourResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.client.v1.MsgSubmitMisbehaviourResponse)
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.class, ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.Builder.class);
}
// Construct using ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.client.v1.Tx.internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_descriptor;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse getDefaultInstanceForType() {
return ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse build() {
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse buildPartial() {
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse result = new ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse) {
return mergeFrom((ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse other) {
if (other == ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.client.v1.MsgSubmitMisbehaviourResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.client.v1.MsgSubmitMisbehaviourResponse)
private static final ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse();
}
public static ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MsgSubmitMisbehaviourResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MsgSubmitMisbehaviourResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.client.v1.Tx.MsgSubmitMisbehaviourResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgCreateClient_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgCreateClient_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgCreateClientResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgCreateClientResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgUpdateClient_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgUpdateClient_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgUpdateClientResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgUpdateClientResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgUpgradeClient_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgUpgradeClient_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\033ibc/core/client/v1/tx.proto\022\022ibc.core." +
"client.v1\032\024gogoproto/gogo.proto\032\031google/" +
"protobuf/any.proto\032\037ibc/core/client/v1/c" +
"lient.proto\"\273\001\n\017MsgCreateClient\022C\n\014clien" +
"t_state\030\001 \001(\0132\024.google.protobuf.AnyB\027\362\336\037" +
"\023yaml:\"client_state\"\022I\n\017consensus_state\030" +
"\002 \001(\0132\024.google.protobuf.AnyB\032\362\336\037\026yaml:\"c" +
"onsensus_state\"\022\016\n\006signer\030\003 \001(\t:\010\350\240\037\000\210\240\037" +
"\000\"\031\n\027MsgCreateClientResponse\"z\n\017MsgUpdat" +
"eClient\022\'\n\tclient_id\030\001 \001(\tB\024\362\336\037\020yaml:\"cl" +
"ient_id\"\022$\n\006header\030\002 \001(\0132\024.google.protob" +
"uf.Any\022\016\n\006signer\030\003 \001(\t:\010\350\240\037\000\210\240\037\000\"\031\n\027MsgU" +
"pdateClientResponse\"\365\002\n\020MsgUpgradeClient" +
"\022\'\n\tclient_id\030\001 \001(\tB\024\362\336\037\020yaml:\"client_id" +
"\"\022C\n\014client_state\030\002 \001(\0132\024.google.protobu" +
"f.AnyB\027\362\336\037\023yaml:\"client_state\"\022I\n\017consen" +
"sus_state\030\003 \001(\0132\024.google.protobuf.AnyB\032\362" +
"\336\037\026yaml:\"consensus_state\"\022=\n\024proof_upgra" +
"de_client\030\004 \001(\014B\037\362\336\037\033yaml:\"proof_upgrade" +
"_client\"\022O\n\035proof_upgrade_consensus_stat" +
"e\030\005 \001(\014B(\362\336\037$yaml:\"proof_upgrade_consens" +
"us_state\"\022\016\n\006signer\030\006 \001(\t:\010\350\240\037\000\210\240\037\000\"\032\n\030M" +
"sgUpgradeClientResponse\"\206\001\n\025MsgSubmitMis" +
"behaviour\022\'\n\tclient_id\030\001 \001(\tB\024\362\336\037\020yaml:\"" +
"client_id\"\022*\n\014misbehaviour\030\002 \001(\0132\024.googl" +
"e.protobuf.Any\022\016\n\006signer\030\003 \001(\t:\010\350\240\037\000\210\240\037\000" +
"\"\037\n\035MsgSubmitMisbehaviourResponse2\242\003\n\003Ms" +
"g\022`\n\014CreateClient\022#.ibc.core.client.v1.M" +
"sgCreateClient\032+.ibc.core.client.v1.MsgC" +
"reateClientResponse\022`\n\014UpdateClient\022#.ib" +
"c.core.client.v1.MsgUpdateClient\032+.ibc.c" +
"ore.client.v1.MsgUpdateClientResponse\022c\n" +
"\rUpgradeClient\022$.ibc.core.client.v1.MsgU" +
"pgradeClient\032,.ibc.core.client.v1.MsgUpg" +
"radeClientResponse\022r\n\022SubmitMisbehaviour" +
"\022).ibc.core.client.v1.MsgSubmitMisbehavi" +
"our\0321.ibc.core.client.v1.MsgSubmitMisbeh" +
"aviourResponseB9Z7github.com/cosmos/cosm" +
"os-sdk/x/ibc/core/02-client/typesb\006proto" +
"3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
ibc.core.client.v1.Client.getDescriptor(),
}, assigner);
internal_static_ibc_core_client_v1_MsgCreateClient_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ibc_core_client_v1_MsgCreateClient_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgCreateClient_descriptor,
new java.lang.String[] { "ClientState", "ConsensusState", "Signer", });
internal_static_ibc_core_client_v1_MsgCreateClientResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ibc_core_client_v1_MsgCreateClientResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgCreateClientResponse_descriptor,
new java.lang.String[] { });
internal_static_ibc_core_client_v1_MsgUpdateClient_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ibc_core_client_v1_MsgUpdateClient_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgUpdateClient_descriptor,
new java.lang.String[] { "ClientId", "Header", "Signer", });
internal_static_ibc_core_client_v1_MsgUpdateClientResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_ibc_core_client_v1_MsgUpdateClientResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgUpdateClientResponse_descriptor,
new java.lang.String[] { });
internal_static_ibc_core_client_v1_MsgUpgradeClient_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_ibc_core_client_v1_MsgUpgradeClient_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgUpgradeClient_descriptor,
new java.lang.String[] { "ClientId", "ClientState", "ConsensusState", "ProofUpgradeClient", "ProofUpgradeConsensusState", "Signer", });
internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgUpgradeClientResponse_descriptor,
new java.lang.String[] { });
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviour_descriptor,
new java.lang.String[] { "ClientId", "Misbehaviour", "Signer", });
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_client_v1_MsgSubmitMisbehaviourResponse_descriptor,
new java.lang.String[] { });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.equal);
registry.add(com.google.protobuf.GoGoProtos.goprotoGetters);
registry.add(com.google.protobuf.GoGoProtos.moretags);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
ibc.core.client.v1.Client.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy