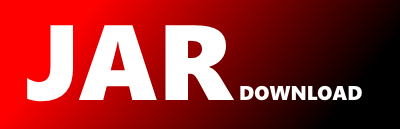
ibc.core.connection.v1.QueryOuterClass Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ibc/core/connection/v1/query.proto
package ibc.core.connection.v1;
public final class QueryOuterClass {
private QueryOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface QueryConnectionRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
java.lang.String getConnectionId();
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
com.google.protobuf.ByteString
getConnectionIdBytes();
}
/**
*
* QueryConnectionRequest is the request type for the Query/Connection RPC
* method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionRequest}
*/
public static final class QueryConnectionRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionRequest)
QueryConnectionRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionRequest.newBuilder() to construct.
private QueryConnectionRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionRequest() {
connectionId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
connectionId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.Builder.class);
}
public static final int CONNECTION_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object connectionId_;
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public java.lang.String getConnectionId() {
java.lang.Object ref = connectionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionId_ = s;
return s;
}
}
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public com.google.protobuf.ByteString
getConnectionIdBytes() {
java.lang.Object ref = connectionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getConnectionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, connectionId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getConnectionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, connectionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest) obj;
boolean result = true;
result = result && getConnectionId()
.equals(other.getConnectionId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONNECTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getConnectionId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionRequest is the request type for the Query/Connection RPC
* method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionRequest)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
connectionId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionRequest_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest(this);
result.connectionId_ = connectionId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest.getDefaultInstance()) return this;
if (!other.getConnectionId().isEmpty()) {
connectionId_ = other.connectionId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object connectionId_ = "";
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public java.lang.String getConnectionId() {
java.lang.Object ref = connectionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public com.google.protobuf.ByteString
getConnectionIdBytes() {
java.lang.Object ref = connectionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public Builder setConnectionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
connectionId_ = value;
onChanged();
return this;
}
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public Builder clearConnectionId() {
connectionId_ = getDefaultInstance().getConnectionId();
onChanged();
return this;
}
/**
*
* connection unique identifier
*
*
* string connection_id = 1;
*/
public Builder setConnectionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
connectionId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionRequest)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
boolean hasConnection();
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
ibc.core.connection.v1.Connection.ConnectionEnd getConnection();
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
ibc.core.connection.v1.Connection.ConnectionEndOrBuilder getConnectionOrBuilder();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryConnectionResponse is the response type for the Query/Connection RPC
* method. Besides the connection end, it includes a proof and the height from
* which the proof was retrieved.
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionResponse}
*/
public static final class QueryConnectionResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionResponse)
QueryConnectionResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionResponse.newBuilder() to construct.
private QueryConnectionResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionResponse() {
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
ibc.core.connection.v1.Connection.ConnectionEnd.Builder subBuilder = null;
if (connection_ != null) {
subBuilder = connection_.toBuilder();
}
connection_ = input.readMessage(ibc.core.connection.v1.Connection.ConnectionEnd.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(connection_);
connection_ = subBuilder.buildPartial();
}
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.Builder.class);
}
public static final int CONNECTION_FIELD_NUMBER = 1;
private ibc.core.connection.v1.Connection.ConnectionEnd connection_;
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public boolean hasConnection() {
return connection_ != null;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public ibc.core.connection.v1.Connection.ConnectionEnd getConnection() {
return connection_ == null ? ibc.core.connection.v1.Connection.ConnectionEnd.getDefaultInstance() : connection_;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public ibc.core.connection.v1.Connection.ConnectionEndOrBuilder getConnectionOrBuilder() {
return getConnection();
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (connection_ != null) {
output.writeMessage(1, getConnection());
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (connection_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConnection());
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse) obj;
boolean result = true;
result = result && (hasConnection() == other.hasConnection());
if (hasConnection()) {
result = result && getConnection()
.equals(other.getConnection());
}
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConnection()) {
hash = (37 * hash) + CONNECTION_FIELD_NUMBER;
hash = (53 * hash) + getConnection().hashCode();
}
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionResponse is the response type for the Query/Connection RPC
* method. Besides the connection end, it includes a proof and the height from
* which the proof was retrieved.
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionResponse)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (connectionBuilder_ == null) {
connection_ = null;
} else {
connection_ = null;
connectionBuilder_ = null;
}
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionResponse_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse(this);
if (connectionBuilder_ == null) {
result.connection_ = connection_;
} else {
result.connection_ = connectionBuilder_.build();
}
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse.getDefaultInstance()) return this;
if (other.hasConnection()) {
mergeConnection(other.getConnection());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private ibc.core.connection.v1.Connection.ConnectionEnd connection_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.connection.v1.Connection.ConnectionEnd, ibc.core.connection.v1.Connection.ConnectionEnd.Builder, ibc.core.connection.v1.Connection.ConnectionEndOrBuilder> connectionBuilder_;
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public boolean hasConnection() {
return connectionBuilder_ != null || connection_ != null;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public ibc.core.connection.v1.Connection.ConnectionEnd getConnection() {
if (connectionBuilder_ == null) {
return connection_ == null ? ibc.core.connection.v1.Connection.ConnectionEnd.getDefaultInstance() : connection_;
} else {
return connectionBuilder_.getMessage();
}
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public Builder setConnection(ibc.core.connection.v1.Connection.ConnectionEnd value) {
if (connectionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
connection_ = value;
onChanged();
} else {
connectionBuilder_.setMessage(value);
}
return this;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public Builder setConnection(
ibc.core.connection.v1.Connection.ConnectionEnd.Builder builderForValue) {
if (connectionBuilder_ == null) {
connection_ = builderForValue.build();
onChanged();
} else {
connectionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public Builder mergeConnection(ibc.core.connection.v1.Connection.ConnectionEnd value) {
if (connectionBuilder_ == null) {
if (connection_ != null) {
connection_ =
ibc.core.connection.v1.Connection.ConnectionEnd.newBuilder(connection_).mergeFrom(value).buildPartial();
} else {
connection_ = value;
}
onChanged();
} else {
connectionBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public Builder clearConnection() {
if (connectionBuilder_ == null) {
connection_ = null;
onChanged();
} else {
connection_ = null;
connectionBuilder_ = null;
}
return this;
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public ibc.core.connection.v1.Connection.ConnectionEnd.Builder getConnectionBuilder() {
onChanged();
return getConnectionFieldBuilder().getBuilder();
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
public ibc.core.connection.v1.Connection.ConnectionEndOrBuilder getConnectionOrBuilder() {
if (connectionBuilder_ != null) {
return connectionBuilder_.getMessageOrBuilder();
} else {
return connection_ == null ?
ibc.core.connection.v1.Connection.ConnectionEnd.getDefaultInstance() : connection_;
}
}
/**
*
* connection associated with the request identifier
*
*
* .ibc.core.connection.v1.ConnectionEnd connection = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.connection.v1.Connection.ConnectionEnd, ibc.core.connection.v1.Connection.ConnectionEnd.Builder, ibc.core.connection.v1.Connection.ConnectionEndOrBuilder>
getConnectionFieldBuilder() {
if (connectionBuilder_ == null) {
connectionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.connection.v1.Connection.ConnectionEnd, ibc.core.connection.v1.Connection.ConnectionEnd.Builder, ibc.core.connection.v1.Connection.ConnectionEndOrBuilder>(
getConnection(),
getParentForChildren(),
isClean());
connection_ = null;
}
return connectionBuilder_;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionResponse)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
boolean hasPagination();
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
cosmos.base.query.v1beta1.Pagination.PageRequest getPagination();
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder();
}
/**
*
* QueryConnectionsRequest is the request type for the Query/Connections RPC
* method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionsRequest}
*/
public static final class QueryConnectionsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionsRequest)
QueryConnectionsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionsRequest.newBuilder() to construct.
private QueryConnectionsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionsRequest() {
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.Builder.class);
}
public static final int PAGINATION_FIELD_NUMBER = 1;
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_;
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
return getPagination();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (pagination_ != null) {
output.writeMessage(1, getPagination());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getPagination());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest) obj;
boolean result = true;
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionsRequest is the request type for the Query/Connections RPC
* method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionsRequest)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsRequest_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest(this);
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest.getDefaultInstance()) return this;
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cosmos.base.query.v1beta1.Pagination.PageRequest pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder> paginationBuilder_;
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageRequest.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageRequest value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageRequest.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequest.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
public cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageRequest.getDefaultInstance() : pagination_;
}
}
/**
* .cosmos.base.query.v1beta1.PageRequest pagination = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageRequest, cosmos.base.query.v1beta1.Pagination.PageRequest.Builder, cosmos.base.query.v1beta1.Pagination.PageRequestOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionsRequest)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
java.util.List
getConnectionsList();
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
ibc.core.connection.v1.Connection.IdentifiedConnection getConnections(int index);
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
int getConnectionsCount();
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
java.util.List extends ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder>
getConnectionsOrBuilderList();
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder getConnectionsOrBuilder(
int index);
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
boolean hasPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponse getPagination();
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getHeight();
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder();
}
/**
*
* QueryConnectionsResponse is the response type for the Query/Connections RPC
* method.
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionsResponse}
*/
public static final class QueryConnectionsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionsResponse)
QueryConnectionsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionsResponse.newBuilder() to construct.
private QueryConnectionsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionsResponse() {
connections_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
connections_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
connections_.add(
input.readMessage(ibc.core.connection.v1.Connection.IdentifiedConnection.parser(), extensionRegistry));
break;
}
case 18: {
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(cosmos.base.query.v1beta1.Pagination.PageResponse.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (height_ != null) {
subBuilder = height_.toBuilder();
}
height_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(height_);
height_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
connections_ = java.util.Collections.unmodifiableList(connections_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.Builder.class);
}
private int bitField0_;
public static final int CONNECTIONS_FIELD_NUMBER = 1;
private java.util.List connections_;
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public java.util.List getConnectionsList() {
return connections_;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public java.util.List extends ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder>
getConnectionsOrBuilderList() {
return connections_;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public int getConnectionsCount() {
return connections_.size();
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnection getConnections(int index) {
return connections_.get(index);
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder getConnectionsOrBuilder(
int index) {
return connections_.get(index);
}
public static final int PAGINATION_FIELD_NUMBER = 2;
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
return getPagination();
}
public static final int HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height height_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
return getHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < connections_.size(); i++) {
output.writeMessage(1, connections_.get(i));
}
if (pagination_ != null) {
output.writeMessage(2, getPagination());
}
if (height_ != null) {
output.writeMessage(3, getHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < connections_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, connections_.get(i));
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPagination());
}
if (height_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse) obj;
boolean result = true;
result = result && getConnectionsList()
.equals(other.getConnectionsList());
result = result && (hasPagination() == other.hasPagination());
if (hasPagination()) {
result = result && getPagination()
.equals(other.getPagination());
}
result = result && (hasHeight() == other.hasHeight());
if (hasHeight()) {
result = result && getHeight()
.equals(other.getHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConnectionsCount() > 0) {
hash = (37 * hash) + CONNECTIONS_FIELD_NUMBER;
hash = (53 * hash) + getConnectionsList().hashCode();
}
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
if (hasHeight()) {
hash = (37 * hash) + HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionsResponse is the response type for the Query/Connections RPC
* method.
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionsResponse)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getConnectionsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (connectionsBuilder_ == null) {
connections_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
connectionsBuilder_.clear();
}
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
if (heightBuilder_ == null) {
height_ = null;
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionsResponse_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (connectionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
connections_ = java.util.Collections.unmodifiableList(connections_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.connections_ = connections_;
} else {
result.connections_ = connectionsBuilder_.build();
}
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
if (heightBuilder_ == null) {
result.height_ = height_;
} else {
result.height_ = heightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse.getDefaultInstance()) return this;
if (connectionsBuilder_ == null) {
if (!other.connections_.isEmpty()) {
if (connections_.isEmpty()) {
connections_ = other.connections_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConnectionsIsMutable();
connections_.addAll(other.connections_);
}
onChanged();
}
} else {
if (!other.connections_.isEmpty()) {
if (connectionsBuilder_.isEmpty()) {
connectionsBuilder_.dispose();
connectionsBuilder_ = null;
connections_ = other.connections_;
bitField0_ = (bitField0_ & ~0x00000001);
connectionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getConnectionsFieldBuilder() : null;
} else {
connectionsBuilder_.addAllMessages(other.connections_);
}
}
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
if (other.hasHeight()) {
mergeHeight(other.getHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List connections_ =
java.util.Collections.emptyList();
private void ensureConnectionsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
connections_ = new java.util.ArrayList(connections_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.connection.v1.Connection.IdentifiedConnection, ibc.core.connection.v1.Connection.IdentifiedConnection.Builder, ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder> connectionsBuilder_;
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public java.util.List getConnectionsList() {
if (connectionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(connections_);
} else {
return connectionsBuilder_.getMessageList();
}
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public int getConnectionsCount() {
if (connectionsBuilder_ == null) {
return connections_.size();
} else {
return connectionsBuilder_.getCount();
}
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnection getConnections(int index) {
if (connectionsBuilder_ == null) {
return connections_.get(index);
} else {
return connectionsBuilder_.getMessage(index);
}
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder setConnections(
int index, ibc.core.connection.v1.Connection.IdentifiedConnection value) {
if (connectionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionsIsMutable();
connections_.set(index, value);
onChanged();
} else {
connectionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder setConnections(
int index, ibc.core.connection.v1.Connection.IdentifiedConnection.Builder builderForValue) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.set(index, builderForValue.build());
onChanged();
} else {
connectionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder addConnections(ibc.core.connection.v1.Connection.IdentifiedConnection value) {
if (connectionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionsIsMutable();
connections_.add(value);
onChanged();
} else {
connectionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder addConnections(
int index, ibc.core.connection.v1.Connection.IdentifiedConnection value) {
if (connectionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionsIsMutable();
connections_.add(index, value);
onChanged();
} else {
connectionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder addConnections(
ibc.core.connection.v1.Connection.IdentifiedConnection.Builder builderForValue) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.add(builderForValue.build());
onChanged();
} else {
connectionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder addConnections(
int index, ibc.core.connection.v1.Connection.IdentifiedConnection.Builder builderForValue) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.add(index, builderForValue.build());
onChanged();
} else {
connectionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder addAllConnections(
java.lang.Iterable extends ibc.core.connection.v1.Connection.IdentifiedConnection> values) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, connections_);
onChanged();
} else {
connectionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder clearConnections() {
if (connectionsBuilder_ == null) {
connections_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
connectionsBuilder_.clear();
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public Builder removeConnections(int index) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.remove(index);
onChanged();
} else {
connectionsBuilder_.remove(index);
}
return this;
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnection.Builder getConnectionsBuilder(
int index) {
return getConnectionsFieldBuilder().getBuilder(index);
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder getConnectionsOrBuilder(
int index) {
if (connectionsBuilder_ == null) {
return connections_.get(index); } else {
return connectionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public java.util.List extends ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder>
getConnectionsOrBuilderList() {
if (connectionsBuilder_ != null) {
return connectionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(connections_);
}
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnection.Builder addConnectionsBuilder() {
return getConnectionsFieldBuilder().addBuilder(
ibc.core.connection.v1.Connection.IdentifiedConnection.getDefaultInstance());
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public ibc.core.connection.v1.Connection.IdentifiedConnection.Builder addConnectionsBuilder(
int index) {
return getConnectionsFieldBuilder().addBuilder(
index, ibc.core.connection.v1.Connection.IdentifiedConnection.getDefaultInstance());
}
/**
*
* list of stored connections of the chain.
*
*
* repeated .ibc.core.connection.v1.IdentifiedConnection connections = 1;
*/
public java.util.List
getConnectionsBuilderList() {
return getConnectionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.connection.v1.Connection.IdentifiedConnection, ibc.core.connection.v1.Connection.IdentifiedConnection.Builder, ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder>
getConnectionsFieldBuilder() {
if (connectionsBuilder_ == null) {
connectionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
ibc.core.connection.v1.Connection.IdentifiedConnection, ibc.core.connection.v1.Connection.IdentifiedConnection.Builder, ibc.core.connection.v1.Connection.IdentifiedConnectionOrBuilder>(
connections_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
connections_ = null;
}
return connectionsBuilder_;
}
private cosmos.base.query.v1beta1.Pagination.PageResponse pagination_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder> paginationBuilder_;
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder setPagination(
cosmos.base.query.v1beta1.Pagination.PageResponse.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder mergePagination(cosmos.base.query.v1beta1.Pagination.PageResponse value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
cosmos.base.query.v1beta1.Pagination.PageResponse.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponse.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
public cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
cosmos.base.query.v1beta1.Pagination.PageResponse.getDefaultInstance() : pagination_;
}
}
/**
*
* pagination response
*
*
* .cosmos.base.query.v1beta1.PageResponse pagination = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cosmos.base.query.v1beta1.Pagination.PageResponse, cosmos.base.query.v1beta1.Pagination.PageResponse.Builder, cosmos.base.query.v1beta1.Pagination.PageResponseOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
private ibc.core.client.v1.Client.Height height_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> heightBuilder_;
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasHeight() {
return heightBuilder_ != null || height_ != null;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getHeight() {
if (heightBuilder_ == null) {
return height_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
} else {
return heightBuilder_.getMessage();
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
height_ = value;
onChanged();
} else {
heightBuilder_.setMessage(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (heightBuilder_ == null) {
height_ = builderForValue.build();
onChanged();
} else {
heightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeHeight(ibc.core.client.v1.Client.Height value) {
if (heightBuilder_ == null) {
if (height_ != null) {
height_ =
ibc.core.client.v1.Client.Height.newBuilder(height_).mergeFrom(value).buildPartial();
} else {
height_ = value;
}
onChanged();
} else {
heightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearHeight() {
if (heightBuilder_ == null) {
height_ = null;
onChanged();
} else {
height_ = null;
heightBuilder_ = null;
}
return this;
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getHeightBuilder() {
onChanged();
return getHeightFieldBuilder().getBuilder();
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getHeightOrBuilder() {
if (heightBuilder_ != null) {
return heightBuilder_.getMessageOrBuilder();
} else {
return height_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : height_;
}
}
/**
*
* query block height
*
*
* .ibc.core.client.v1.Height height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getHeightFieldBuilder() {
if (heightBuilder_ == null) {
heightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getHeight(),
getParentForChildren(),
isClean());
height_ = null;
}
return heightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionsResponse)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryClientConnectionsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryClientConnectionsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
java.lang.String getClientId();
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
com.google.protobuf.ByteString
getClientIdBytes();
}
/**
*
* QueryClientConnectionsRequest is the request type for the
* Query/ClientConnections RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryClientConnectionsRequest}
*/
public static final class QueryClientConnectionsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryClientConnectionsRequest)
QueryClientConnectionsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryClientConnectionsRequest.newBuilder() to construct.
private QueryClientConnectionsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryClientConnectionsRequest() {
clientId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryClientConnectionsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.Builder.class);
}
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object clientId_;
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getClientIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, clientId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getClientIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, clientId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest other = (ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest) obj;
boolean result = true;
result = result && getClientId()
.equals(other.getClientId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryClientConnectionsRequest is the request type for the
* Query/ClientConnections RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryClientConnectionsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryClientConnectionsRequest)
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
clientId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest build() {
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest result = new ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest(this);
result.clientId_ = clientId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest.getDefaultInstance()) return this;
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object clientId_ = "";
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
* client identifier associated with a connection
*
*
* string client_id = 1;
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryClientConnectionsRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryClientConnectionsRequest)
private static final ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryClientConnectionsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryClientConnectionsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryClientConnectionsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryClientConnectionsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
java.util.List
getConnectionPathsList();
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
int getConnectionPathsCount();
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
java.lang.String getConnectionPaths(int index);
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
com.google.protobuf.ByteString
getConnectionPathsBytes(int index);
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryClientConnectionsResponse is the response type for the
* Query/ClientConnections RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryClientConnectionsResponse}
*/
public static final class QueryClientConnectionsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryClientConnectionsResponse)
QueryClientConnectionsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryClientConnectionsResponse.newBuilder() to construct.
private QueryClientConnectionsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryClientConnectionsResponse() {
connectionPaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryClientConnectionsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
connectionPaths_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
connectionPaths_.add(s);
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
connectionPaths_ = connectionPaths_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.Builder.class);
}
private int bitField0_;
public static final int CONNECTION_PATHS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList connectionPaths_;
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public com.google.protobuf.ProtocolStringList
getConnectionPathsList() {
return connectionPaths_;
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public int getConnectionPathsCount() {
return connectionPaths_.size();
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public java.lang.String getConnectionPaths(int index) {
return connectionPaths_.get(index);
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public com.google.protobuf.ByteString
getConnectionPathsBytes(int index) {
return connectionPaths_.getByteString(index);
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < connectionPaths_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, connectionPaths_.getRaw(i));
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < connectionPaths_.size(); i++) {
dataSize += computeStringSizeNoTag(connectionPaths_.getRaw(i));
}
size += dataSize;
size += 1 * getConnectionPathsList().size();
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse other = (ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse) obj;
boolean result = true;
result = result && getConnectionPathsList()
.equals(other.getConnectionPathsList());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConnectionPathsCount() > 0) {
hash = (37 * hash) + CONNECTION_PATHS_FIELD_NUMBER;
hash = (53 * hash) + getConnectionPathsList().hashCode();
}
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryClientConnectionsResponse is the response type for the
* Query/ClientConnections RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryClientConnectionsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryClientConnectionsResponse)
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
connectionPaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse build() {
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse result = new ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
connectionPaths_ = connectionPaths_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.connectionPaths_ = connectionPaths_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse.getDefaultInstance()) return this;
if (!other.connectionPaths_.isEmpty()) {
if (connectionPaths_.isEmpty()) {
connectionPaths_ = other.connectionPaths_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConnectionPathsIsMutable();
connectionPaths_.addAll(other.connectionPaths_);
}
onChanged();
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList connectionPaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureConnectionPathsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
connectionPaths_ = new com.google.protobuf.LazyStringArrayList(connectionPaths_);
bitField0_ |= 0x00000001;
}
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public com.google.protobuf.ProtocolStringList
getConnectionPathsList() {
return connectionPaths_.getUnmodifiableView();
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public int getConnectionPathsCount() {
return connectionPaths_.size();
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public java.lang.String getConnectionPaths(int index) {
return connectionPaths_.get(index);
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public com.google.protobuf.ByteString
getConnectionPathsBytes(int index) {
return connectionPaths_.getByteString(index);
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public Builder setConnectionPaths(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionPathsIsMutable();
connectionPaths_.set(index, value);
onChanged();
return this;
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public Builder addConnectionPaths(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionPathsIsMutable();
connectionPaths_.add(value);
onChanged();
return this;
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public Builder addAllConnectionPaths(
java.lang.Iterable values) {
ensureConnectionPathsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, connectionPaths_);
onChanged();
return this;
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public Builder clearConnectionPaths() {
connectionPaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* slice of all the connection paths associated with a client.
*
*
* repeated string connection_paths = 1;
*/
public Builder addConnectionPathsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureConnectionPathsIsMutable();
connectionPaths_.add(value);
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was generated
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryClientConnectionsResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryClientConnectionsResponse)
private static final ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryClientConnectionsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryClientConnectionsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryClientConnectionsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionClientStateRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionClientStateRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
java.lang.String getConnectionId();
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
com.google.protobuf.ByteString
getConnectionIdBytes();
}
/**
*
* QueryConnectionClientStateRequest is the request type for the
* Query/ConnectionClientState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionClientStateRequest}
*/
public static final class QueryConnectionClientStateRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionClientStateRequest)
QueryConnectionClientStateRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionClientStateRequest.newBuilder() to construct.
private QueryConnectionClientStateRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionClientStateRequest() {
connectionId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionClientStateRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
connectionId_ = s;
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.Builder.class);
}
public static final int CONNECTION_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object connectionId_;
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public java.lang.String getConnectionId() {
java.lang.Object ref = connectionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionId_ = s;
return s;
}
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public com.google.protobuf.ByteString
getConnectionIdBytes() {
java.lang.Object ref = connectionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getConnectionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, connectionId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getConnectionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, connectionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest) obj;
boolean result = true;
result = result && getConnectionId()
.equals(other.getConnectionId());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONNECTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getConnectionId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionClientStateRequest is the request type for the
* Query/ConnectionClientState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionClientStateRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionClientStateRequest)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
connectionId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest(this);
result.connectionId_ = connectionId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest.getDefaultInstance()) return this;
if (!other.getConnectionId().isEmpty()) {
connectionId_ = other.connectionId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object connectionId_ = "";
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public java.lang.String getConnectionId() {
java.lang.Object ref = connectionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public com.google.protobuf.ByteString
getConnectionIdBytes() {
java.lang.Object ref = connectionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public Builder setConnectionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
connectionId_ = value;
onChanged();
return this;
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public Builder clearConnectionId() {
connectionId_ = getDefaultInstance().getConnectionId();
onChanged();
return this;
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public Builder setConnectionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
connectionId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionClientStateRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionClientStateRequest)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionClientStateRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionClientStateRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionClientStateResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionClientStateResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
boolean hasIdentifiedClientState();
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
ibc.core.client.v1.Client.IdentifiedClientState getIdentifiedClientState();
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder getIdentifiedClientStateOrBuilder();
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryConnectionClientStateResponse is the response type for the
* Query/ConnectionClientState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionClientStateResponse}
*/
public static final class QueryConnectionClientStateResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionClientStateResponse)
QueryConnectionClientStateResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionClientStateResponse.newBuilder() to construct.
private QueryConnectionClientStateResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionClientStateResponse() {
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionClientStateResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
ibc.core.client.v1.Client.IdentifiedClientState.Builder subBuilder = null;
if (identifiedClientState_ != null) {
subBuilder = identifiedClientState_.toBuilder();
}
identifiedClientState_ = input.readMessage(ibc.core.client.v1.Client.IdentifiedClientState.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifiedClientState_);
identifiedClientState_ = subBuilder.buildPartial();
}
break;
}
case 18: {
proof_ = input.readBytes();
break;
}
case 26: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.Builder.class);
}
public static final int IDENTIFIED_CLIENT_STATE_FIELD_NUMBER = 1;
private ibc.core.client.v1.Client.IdentifiedClientState identifiedClientState_;
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public boolean hasIdentifiedClientState() {
return identifiedClientState_ != null;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientState getIdentifiedClientState() {
return identifiedClientState_ == null ? ibc.core.client.v1.Client.IdentifiedClientState.getDefaultInstance() : identifiedClientState_;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder getIdentifiedClientStateOrBuilder() {
return getIdentifiedClientState();
}
public static final int PROOF_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 3;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (identifiedClientState_ != null) {
output.writeMessage(1, getIdentifiedClientState());
}
if (!proof_.isEmpty()) {
output.writeBytes(2, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(3, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (identifiedClientState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getIdentifiedClientState());
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse) obj;
boolean result = true;
result = result && (hasIdentifiedClientState() == other.hasIdentifiedClientState());
if (hasIdentifiedClientState()) {
result = result && getIdentifiedClientState()
.equals(other.getIdentifiedClientState());
}
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasIdentifiedClientState()) {
hash = (37 * hash) + IDENTIFIED_CLIENT_STATE_FIELD_NUMBER;
hash = (53 * hash) + getIdentifiedClientState().hashCode();
}
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionClientStateResponse is the response type for the
* Query/ConnectionClientState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionClientStateResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionClientStateResponse)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (identifiedClientStateBuilder_ == null) {
identifiedClientState_ = null;
} else {
identifiedClientState_ = null;
identifiedClientStateBuilder_ = null;
}
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse(this);
if (identifiedClientStateBuilder_ == null) {
result.identifiedClientState_ = identifiedClientState_;
} else {
result.identifiedClientState_ = identifiedClientStateBuilder_.build();
}
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse.getDefaultInstance()) return this;
if (other.hasIdentifiedClientState()) {
mergeIdentifiedClientState(other.getIdentifiedClientState());
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private ibc.core.client.v1.Client.IdentifiedClientState identifiedClientState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.IdentifiedClientState, ibc.core.client.v1.Client.IdentifiedClientState.Builder, ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder> identifiedClientStateBuilder_;
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public boolean hasIdentifiedClientState() {
return identifiedClientStateBuilder_ != null || identifiedClientState_ != null;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientState getIdentifiedClientState() {
if (identifiedClientStateBuilder_ == null) {
return identifiedClientState_ == null ? ibc.core.client.v1.Client.IdentifiedClientState.getDefaultInstance() : identifiedClientState_;
} else {
return identifiedClientStateBuilder_.getMessage();
}
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder setIdentifiedClientState(ibc.core.client.v1.Client.IdentifiedClientState value) {
if (identifiedClientStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifiedClientState_ = value;
onChanged();
} else {
identifiedClientStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder setIdentifiedClientState(
ibc.core.client.v1.Client.IdentifiedClientState.Builder builderForValue) {
if (identifiedClientStateBuilder_ == null) {
identifiedClientState_ = builderForValue.build();
onChanged();
} else {
identifiedClientStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder mergeIdentifiedClientState(ibc.core.client.v1.Client.IdentifiedClientState value) {
if (identifiedClientStateBuilder_ == null) {
if (identifiedClientState_ != null) {
identifiedClientState_ =
ibc.core.client.v1.Client.IdentifiedClientState.newBuilder(identifiedClientState_).mergeFrom(value).buildPartial();
} else {
identifiedClientState_ = value;
}
onChanged();
} else {
identifiedClientStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public Builder clearIdentifiedClientState() {
if (identifiedClientStateBuilder_ == null) {
identifiedClientState_ = null;
onChanged();
} else {
identifiedClientState_ = null;
identifiedClientStateBuilder_ = null;
}
return this;
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientState.Builder getIdentifiedClientStateBuilder() {
onChanged();
return getIdentifiedClientStateFieldBuilder().getBuilder();
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
public ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder getIdentifiedClientStateOrBuilder() {
if (identifiedClientStateBuilder_ != null) {
return identifiedClientStateBuilder_.getMessageOrBuilder();
} else {
return identifiedClientState_ == null ?
ibc.core.client.v1.Client.IdentifiedClientState.getDefaultInstance() : identifiedClientState_;
}
}
/**
*
* client state associated with the channel
*
*
* .ibc.core.client.v1.IdentifiedClientState identified_client_state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.IdentifiedClientState, ibc.core.client.v1.Client.IdentifiedClientState.Builder, ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder>
getIdentifiedClientStateFieldBuilder() {
if (identifiedClientStateBuilder_ == null) {
identifiedClientStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.IdentifiedClientState, ibc.core.client.v1.Client.IdentifiedClientState.Builder, ibc.core.client.v1.Client.IdentifiedClientStateOrBuilder>(
getIdentifiedClientState(),
getParentForChildren(),
isClean());
identifiedClientState_ = null;
}
return identifiedClientStateBuilder_;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 2;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 3 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionClientStateResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionClientStateResponse)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionClientStateResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionClientStateResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionClientStateResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionConsensusStateRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionConsensusStateRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
java.lang.String getConnectionId();
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
com.google.protobuf.ByteString
getConnectionIdBytes();
/**
* uint64 revision_number = 2;
*/
long getRevisionNumber();
/**
* uint64 revision_height = 3;
*/
long getRevisionHeight();
}
/**
*
* QueryConnectionConsensusStateRequest is the request type for the
* Query/ConnectionConsensusState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionConsensusStateRequest}
*/
public static final class QueryConnectionConsensusStateRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionConsensusStateRequest)
QueryConnectionConsensusStateRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionConsensusStateRequest.newBuilder() to construct.
private QueryConnectionConsensusStateRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionConsensusStateRequest() {
connectionId_ = "";
revisionNumber_ = 0L;
revisionHeight_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionConsensusStateRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
connectionId_ = s;
break;
}
case 16: {
revisionNumber_ = input.readUInt64();
break;
}
case 24: {
revisionHeight_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.Builder.class);
}
public static final int CONNECTION_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object connectionId_;
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public java.lang.String getConnectionId() {
java.lang.Object ref = connectionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionId_ = s;
return s;
}
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public com.google.protobuf.ByteString
getConnectionIdBytes() {
java.lang.Object ref = connectionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REVISION_NUMBER_FIELD_NUMBER = 2;
private long revisionNumber_;
/**
* uint64 revision_number = 2;
*/
public long getRevisionNumber() {
return revisionNumber_;
}
public static final int REVISION_HEIGHT_FIELD_NUMBER = 3;
private long revisionHeight_;
/**
* uint64 revision_height = 3;
*/
public long getRevisionHeight() {
return revisionHeight_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getConnectionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, connectionId_);
}
if (revisionNumber_ != 0L) {
output.writeUInt64(2, revisionNumber_);
}
if (revisionHeight_ != 0L) {
output.writeUInt64(3, revisionHeight_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getConnectionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, connectionId_);
}
if (revisionNumber_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, revisionNumber_);
}
if (revisionHeight_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, revisionHeight_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest) obj;
boolean result = true;
result = result && getConnectionId()
.equals(other.getConnectionId());
result = result && (getRevisionNumber()
== other.getRevisionNumber());
result = result && (getRevisionHeight()
== other.getRevisionHeight());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONNECTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getConnectionId().hashCode();
hash = (37 * hash) + REVISION_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRevisionNumber());
hash = (37 * hash) + REVISION_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRevisionHeight());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionConsensusStateRequest is the request type for the
* Query/ConnectionConsensusState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionConsensusStateRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionConsensusStateRequest)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
connectionId_ = "";
revisionNumber_ = 0L;
revisionHeight_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest(this);
result.connectionId_ = connectionId_;
result.revisionNumber_ = revisionNumber_;
result.revisionHeight_ = revisionHeight_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest.getDefaultInstance()) return this;
if (!other.getConnectionId().isEmpty()) {
connectionId_ = other.connectionId_;
onChanged();
}
if (other.getRevisionNumber() != 0L) {
setRevisionNumber(other.getRevisionNumber());
}
if (other.getRevisionHeight() != 0L) {
setRevisionHeight(other.getRevisionHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object connectionId_ = "";
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public java.lang.String getConnectionId() {
java.lang.Object ref = connectionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
connectionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public com.google.protobuf.ByteString
getConnectionIdBytes() {
java.lang.Object ref = connectionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
connectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public Builder setConnectionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
connectionId_ = value;
onChanged();
return this;
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public Builder clearConnectionId() {
connectionId_ = getDefaultInstance().getConnectionId();
onChanged();
return this;
}
/**
*
* connection identifier
*
*
* string connection_id = 1 [(.gogoproto.moretags) = "yaml:\"connection_id\""];
*/
public Builder setConnectionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
connectionId_ = value;
onChanged();
return this;
}
private long revisionNumber_ ;
/**
* uint64 revision_number = 2;
*/
public long getRevisionNumber() {
return revisionNumber_;
}
/**
* uint64 revision_number = 2;
*/
public Builder setRevisionNumber(long value) {
revisionNumber_ = value;
onChanged();
return this;
}
/**
* uint64 revision_number = 2;
*/
public Builder clearRevisionNumber() {
revisionNumber_ = 0L;
onChanged();
return this;
}
private long revisionHeight_ ;
/**
* uint64 revision_height = 3;
*/
public long getRevisionHeight() {
return revisionHeight_;
}
/**
* uint64 revision_height = 3;
*/
public Builder setRevisionHeight(long value) {
revisionHeight_ = value;
onChanged();
return this;
}
/**
* uint64 revision_height = 3;
*/
public Builder clearRevisionHeight() {
revisionHeight_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionConsensusStateRequest)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionConsensusStateRequest)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionConsensusStateRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionConsensusStateRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface QueryConnectionConsensusStateResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ibc.core.connection.v1.QueryConnectionConsensusStateResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
boolean hasConsensusState();
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
com.google.protobuf.Any getConsensusState();
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder();
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
java.lang.String getClientId();
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
com.google.protobuf.ByteString getProof();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
boolean hasProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.Height getProofHeight();
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder();
}
/**
*
* QueryConnectionConsensusStateResponse is the response type for the
* Query/ConnectionConsensusState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionConsensusStateResponse}
*/
public static final class QueryConnectionConsensusStateResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ibc.core.connection.v1.QueryConnectionConsensusStateResponse)
QueryConnectionConsensusStateResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use QueryConnectionConsensusStateResponse.newBuilder() to construct.
private QueryConnectionConsensusStateResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private QueryConnectionConsensusStateResponse() {
clientId_ = "";
proof_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private QueryConnectionConsensusStateResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.Any.Builder subBuilder = null;
if (consensusState_ != null) {
subBuilder = consensusState_.toBuilder();
}
consensusState_ = input.readMessage(com.google.protobuf.Any.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(consensusState_);
consensusState_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
case 26: {
proof_ = input.readBytes();
break;
}
case 34: {
ibc.core.client.v1.Client.Height.Builder subBuilder = null;
if (proofHeight_ != null) {
subBuilder = proofHeight_.toBuilder();
}
proofHeight_ = input.readMessage(ibc.core.client.v1.Client.Height.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(proofHeight_);
proofHeight_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.Builder.class);
}
public static final int CONSENSUS_STATE_FIELD_NUMBER = 1;
private com.google.protobuf.Any consensusState_;
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public boolean hasConsensusState() {
return consensusState_ != null;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.Any getConsensusState() {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
return getConsensusState();
}
public static final int CLIENT_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object clientId_;
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROOF_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString proof_;
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
public static final int PROOF_HEIGHT_FIELD_NUMBER = 4;
private ibc.core.client.v1.Client.Height proofHeight_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
return getProofHeight();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (consensusState_ != null) {
output.writeMessage(1, getConsensusState());
}
if (!getClientIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, clientId_);
}
if (!proof_.isEmpty()) {
output.writeBytes(3, proof_);
}
if (proofHeight_ != null) {
output.writeMessage(4, getProofHeight());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (consensusState_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsensusState());
}
if (!getClientIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, clientId_);
}
if (!proof_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, proof_);
}
if (proofHeight_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getProofHeight());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse)) {
return super.equals(obj);
}
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse other = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse) obj;
boolean result = true;
result = result && (hasConsensusState() == other.hasConsensusState());
if (hasConsensusState()) {
result = result && getConsensusState()
.equals(other.getConsensusState());
}
result = result && getClientId()
.equals(other.getClientId());
result = result && getProof()
.equals(other.getProof());
result = result && (hasProofHeight() == other.hasProofHeight());
if (hasProofHeight()) {
result = result && getProofHeight()
.equals(other.getProofHeight());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsensusState()) {
hash = (37 * hash) + CONSENSUS_STATE_FIELD_NUMBER;
hash = (53 * hash) + getConsensusState().hashCode();
}
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
hash = (37 * hash) + PROOF_FIELD_NUMBER;
hash = (53 * hash) + getProof().hashCode();
if (hasProofHeight()) {
hash = (37 * hash) + PROOF_HEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getProofHeight().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* QueryConnectionConsensusStateResponse is the response type for the
* Query/ConnectionConsensusState RPC method
*
*
* Protobuf type {@code ibc.core.connection.v1.QueryConnectionConsensusStateResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ibc.core.connection.v1.QueryConnectionConsensusStateResponse)
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.class, ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.Builder.class);
}
// Construct using ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (consensusStateBuilder_ == null) {
consensusState_ = null;
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
clientId_ = "";
proof_ = com.google.protobuf.ByteString.EMPTY;
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return ibc.core.connection.v1.QueryOuterClass.internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_descriptor;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse getDefaultInstanceForType() {
return ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.getDefaultInstance();
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse build() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse buildPartial() {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse result = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse(this);
if (consensusStateBuilder_ == null) {
result.consensusState_ = consensusState_;
} else {
result.consensusState_ = consensusStateBuilder_.build();
}
result.clientId_ = clientId_;
result.proof_ = proof_;
if (proofHeightBuilder_ == null) {
result.proofHeight_ = proofHeight_;
} else {
result.proofHeight_ = proofHeightBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse) {
return mergeFrom((ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse other) {
if (other == ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse.getDefaultInstance()) return this;
if (other.hasConsensusState()) {
mergeConsensusState(other.getConsensusState());
}
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (other.getProof() != com.google.protobuf.ByteString.EMPTY) {
setProof(other.getProof());
}
if (other.hasProofHeight()) {
mergeProofHeight(other.getProofHeight());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.google.protobuf.Any consensusState_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder> consensusStateBuilder_;
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public boolean hasConsensusState() {
return consensusStateBuilder_ != null || consensusState_ != null;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.Any getConsensusState() {
if (consensusStateBuilder_ == null) {
return consensusState_ == null ? com.google.protobuf.Any.getDefaultInstance() : consensusState_;
} else {
return consensusStateBuilder_.getMessage();
}
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder setConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consensusState_ = value;
onChanged();
} else {
consensusStateBuilder_.setMessage(value);
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder setConsensusState(
com.google.protobuf.Any.Builder builderForValue) {
if (consensusStateBuilder_ == null) {
consensusState_ = builderForValue.build();
onChanged();
} else {
consensusStateBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder mergeConsensusState(com.google.protobuf.Any value) {
if (consensusStateBuilder_ == null) {
if (consensusState_ != null) {
consensusState_ =
com.google.protobuf.Any.newBuilder(consensusState_).mergeFrom(value).buildPartial();
} else {
consensusState_ = value;
}
onChanged();
} else {
consensusStateBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public Builder clearConsensusState() {
if (consensusStateBuilder_ == null) {
consensusState_ = null;
onChanged();
} else {
consensusState_ = null;
consensusStateBuilder_ = null;
}
return this;
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.Any.Builder getConsensusStateBuilder() {
onChanged();
return getConsensusStateFieldBuilder().getBuilder();
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
public com.google.protobuf.AnyOrBuilder getConsensusStateOrBuilder() {
if (consensusStateBuilder_ != null) {
return consensusStateBuilder_.getMessageOrBuilder();
} else {
return consensusState_ == null ?
com.google.protobuf.Any.getDefaultInstance() : consensusState_;
}
}
/**
*
* consensus state associated with the channel
*
*
* .google.protobuf.Any consensus_state = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>
getConsensusStateFieldBuilder() {
if (consensusStateBuilder_ == null) {
consensusStateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Any, com.google.protobuf.Any.Builder, com.google.protobuf.AnyOrBuilder>(
getConsensusState(),
getParentForChildren(),
isClean());
consensusState_ = null;
}
return consensusStateBuilder_;
}
private java.lang.Object clientId_ = "";
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
*
* client ID associated with the consensus state
*
*
* string client_id = 2;
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString proof_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public com.google.protobuf.ByteString getProof() {
return proof_;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public Builder setProof(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
proof_ = value;
onChanged();
return this;
}
/**
*
* merkle proof of existence
*
*
* bytes proof = 3;
*/
public Builder clearProof() {
proof_ = getDefaultInstance().getProof();
onChanged();
return this;
}
private ibc.core.client.v1.Client.Height proofHeight_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder> proofHeightBuilder_;
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public boolean hasProofHeight() {
return proofHeightBuilder_ != null || proofHeight_ != null;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height getProofHeight() {
if (proofHeightBuilder_ == null) {
return proofHeight_ == null ? ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
} else {
return proofHeightBuilder_.getMessage();
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
proofHeight_ = value;
onChanged();
} else {
proofHeightBuilder_.setMessage(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder setProofHeight(
ibc.core.client.v1.Client.Height.Builder builderForValue) {
if (proofHeightBuilder_ == null) {
proofHeight_ = builderForValue.build();
onChanged();
} else {
proofHeightBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder mergeProofHeight(ibc.core.client.v1.Client.Height value) {
if (proofHeightBuilder_ == null) {
if (proofHeight_ != null) {
proofHeight_ =
ibc.core.client.v1.Client.Height.newBuilder(proofHeight_).mergeFrom(value).buildPartial();
} else {
proofHeight_ = value;
}
onChanged();
} else {
proofHeightBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public Builder clearProofHeight() {
if (proofHeightBuilder_ == null) {
proofHeight_ = null;
onChanged();
} else {
proofHeight_ = null;
proofHeightBuilder_ = null;
}
return this;
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.Height.Builder getProofHeightBuilder() {
onChanged();
return getProofHeightFieldBuilder().getBuilder();
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
public ibc.core.client.v1.Client.HeightOrBuilder getProofHeightOrBuilder() {
if (proofHeightBuilder_ != null) {
return proofHeightBuilder_.getMessageOrBuilder();
} else {
return proofHeight_ == null ?
ibc.core.client.v1.Client.Height.getDefaultInstance() : proofHeight_;
}
}
/**
*
* height at which the proof was retrieved
*
*
* .ibc.core.client.v1.Height proof_height = 4 [(.gogoproto.nullable) = false];
*/
private com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>
getProofHeightFieldBuilder() {
if (proofHeightBuilder_ == null) {
proofHeightBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
ibc.core.client.v1.Client.Height, ibc.core.client.v1.Client.Height.Builder, ibc.core.client.v1.Client.HeightOrBuilder>(
getProofHeight(),
getParentForChildren(),
isClean());
proofHeight_ = null;
}
return proofHeightBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ibc.core.connection.v1.QueryConnectionConsensusStateResponse)
}
// @@protoc_insertion_point(class_scope:ibc.core.connection.v1.QueryConnectionConsensusStateResponse)
private static final ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse();
}
public static ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public QueryConnectionConsensusStateResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new QueryConnectionConsensusStateResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public ibc.core.connection.v1.QueryOuterClass.QueryConnectionConsensusStateResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\"ibc/core/connection/v1/query.proto\022\026ib" +
"c.core.connection.v1\032\024gogoproto/gogo.pro" +
"to\032*cosmos/base/query/v1beta1/pagination" +
".proto\032\037ibc/core/client/v1/client.proto\032" +
"\'ibc/core/connection/v1/connection.proto" +
"\032\034google/api/annotations.proto\032\031google/p" +
"rotobuf/any.proto\"/\n\026QueryConnectionRequ" +
"est\022\025\n\rconnection_id\030\001 \001(\t\"\233\001\n\027QueryConn" +
"ectionResponse\0229\n\nconnection\030\001 \001(\0132%.ibc" +
".core.connection.v1.ConnectionEnd\022\r\n\005pro" +
"of\030\002 \001(\014\0226\n\014proof_height\030\003 \001(\0132\032.ibc.cor" +
"e.client.v1.HeightB\004\310\336\037\000\"U\n\027QueryConnect" +
"ionsRequest\022:\n\npagination\030\001 \001(\0132&.cosmos" +
".base.query.v1beta1.PageRequest\"\314\001\n\030Quer" +
"yConnectionsResponse\022A\n\013connections\030\001 \003(" +
"\0132,.ibc.core.connection.v1.IdentifiedCon" +
"nection\022;\n\npagination\030\002 \001(\0132\'.cosmos.bas" +
"e.query.v1beta1.PageResponse\0220\n\006height\030\003" +
" \001(\0132\032.ibc.core.client.v1.HeightB\004\310\336\037\000\"2" +
"\n\035QueryClientConnectionsRequest\022\021\n\tclien" +
"t_id\030\001 \001(\t\"\201\001\n\036QueryClientConnectionsRes" +
"ponse\022\030\n\020connection_paths\030\001 \003(\t\022\r\n\005proof" +
"\030\002 \001(\014\0226\n\014proof_height\030\003 \001(\0132\032.ibc.core." +
"client.v1.HeightB\004\310\336\037\000\"T\n!QueryConnectio" +
"nClientStateRequest\022/\n\rconnection_id\030\001 \001" +
"(\tB\030\362\336\037\024yaml:\"connection_id\"\"\267\001\n\"QueryCo" +
"nnectionClientStateResponse\022J\n\027identifie" +
"d_client_state\030\001 \001(\0132).ibc.core.client.v" +
"1.IdentifiedClientState\022\r\n\005proof\030\002 \001(\014\0226" +
"\n\014proof_height\030\003 \001(\0132\032.ibc.core.client.v" +
"1.HeightB\004\310\336\037\000\"\211\001\n$QueryConnectionConsen" +
"susStateRequest\022/\n\rconnection_id\030\001 \001(\tB\030" +
"\362\336\037\024yaml:\"connection_id\"\022\027\n\017revision_num" +
"ber\030\002 \001(\004\022\027\n\017revision_height\030\003 \001(\004\"\260\001\n%Q" +
"ueryConnectionConsensusStateResponse\022-\n\017" +
"consensus_state\030\001 \001(\0132\024.google.protobuf." +
"Any\022\021\n\tclient_id\030\002 \001(\t\022\r\n\005proof\030\003 \001(\014\0226\n" +
"\014proof_height\030\004 \001(\0132\032.ibc.core.client.v1" +
".HeightB\004\310\336\037\0002\251\010\n\005Query\022\257\001\n\nConnection\022." +
".ibc.core.connection.v1.QueryConnectionR" +
"equest\032/.ibc.core.connection.v1.QueryCon" +
"nectionResponse\"@\202\323\344\223\002:\0228/ibc/core/conne" +
"ction/v1beta1/connections/{connection_id" +
"}\022\242\001\n\013Connections\022/.ibc.core.connection." +
"v1.QueryConnectionsRequest\0320.ibc.core.co" +
"nnection.v1.QueryConnectionsResponse\"0\202\323" +
"\344\223\002*\022(/ibc/core/connection/v1beta1/conne" +
"ctions\022\307\001\n\021ClientConnections\0225.ibc.core." +
"connection.v1.QueryClientConnectionsRequ" +
"est\0326.ibc.core.connection.v1.QueryClient" +
"ConnectionsResponse\"C\202\323\344\223\002=\022;/ibc/core/c" +
"onnection/v1beta1/client_connections/{cl" +
"ient_id}\022\335\001\n\025ConnectionClientState\0229.ibc" +
".core.connection.v1.QueryConnectionClien" +
"tStateRequest\032:.ibc.core.connection.v1.Q" +
"ueryConnectionClientStateResponse\"M\202\323\344\223\002" +
"G\022E/ibc/core/connection/v1beta1/connecti" +
"ons/{connection_id}/client_state\022\236\002\n\030Con" +
"nectionConsensusState\022<.ibc.core.connect" +
"ion.v1.QueryConnectionConsensusStateRequ" +
"est\032=.ibc.core.connection.v1.QueryConnec" +
"tionConsensusStateResponse\"\204\001\202\323\344\223\002~\022|/ib" +
"c/core/connection/v1beta1/connections/{c" +
"onnection_id}/consensus_state/revision/{" +
"revision_number}/height/{revision_height" +
"}B=Z;github.com/cosmos/cosmos-sdk/x/ibc/" +
"core/03-connection/typesb\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.protobuf.GoGoProtos.getDescriptor(),
cosmos.base.query.v1beta1.Pagination.getDescriptor(),
ibc.core.client.v1.Client.getDescriptor(),
ibc.core.connection.v1.Connection.getDescriptor(),
com.google.api.AnnotationsProto.getDescriptor(),
com.google.protobuf.AnyProto.getDescriptor(),
}, assigner);
internal_static_ibc_core_connection_v1_QueryConnectionRequest_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ibc_core_connection_v1_QueryConnectionRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionRequest_descriptor,
new java.lang.String[] { "ConnectionId", });
internal_static_ibc_core_connection_v1_QueryConnectionResponse_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ibc_core_connection_v1_QueryConnectionResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionResponse_descriptor,
new java.lang.String[] { "Connection", "Proof", "ProofHeight", });
internal_static_ibc_core_connection_v1_QueryConnectionsRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ibc_core_connection_v1_QueryConnectionsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionsRequest_descriptor,
new java.lang.String[] { "Pagination", });
internal_static_ibc_core_connection_v1_QueryConnectionsResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_ibc_core_connection_v1_QueryConnectionsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionsResponse_descriptor,
new java.lang.String[] { "Connections", "Pagination", "Height", });
internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryClientConnectionsRequest_descriptor,
new java.lang.String[] { "ClientId", });
internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryClientConnectionsResponse_descriptor,
new java.lang.String[] { "ConnectionPaths", "Proof", "ProofHeight", });
internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionClientStateRequest_descriptor,
new java.lang.String[] { "ConnectionId", });
internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionClientStateResponse_descriptor,
new java.lang.String[] { "IdentifiedClientState", "Proof", "ProofHeight", });
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateRequest_descriptor,
new java.lang.String[] { "ConnectionId", "RevisionNumber", "RevisionHeight", });
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ibc_core_connection_v1_QueryConnectionConsensusStateResponse_descriptor,
new java.lang.String[] { "ConsensusState", "ClientId", "Proof", "ProofHeight", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(com.google.protobuf.GoGoProtos.moretags);
registry.add(com.google.protobuf.GoGoProtos.nullable);
registry.add(com.google.api.AnnotationsProto.http);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
com.google.protobuf.GoGoProtos.getDescriptor();
cosmos.base.query.v1beta1.Pagination.getDescriptor();
ibc.core.client.v1.Client.getDescriptor();
ibc.core.connection.v1.Connection.getDescriptor();
com.google.api.AnnotationsProto.getDescriptor();
com.google.protobuf.AnyProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy