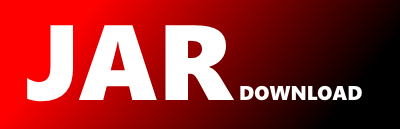
tech.confio.ics23.LengthOp Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: confio/proofs.proto
package tech.confio.ics23;
/**
*
**
*LengthOp defines how to process the key and value of the LeafOp
*to include length information. After encoding the length with the given
*algorithm, the length will be prepended to the key and value bytes.
*(Each one with it's own encoded length)
*
*
* Protobuf enum {@code ics23.LengthOp}
*/
public enum LengthOp
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* NO_PREFIX don't include any length info
*
*
* NO_PREFIX = 0;
*/
NO_PREFIX(0),
/**
*
* VAR_PROTO uses protobuf (and go-amino) varint encoding of the length
*
*
* VAR_PROTO = 1;
*/
VAR_PROTO(1),
/**
*
* VAR_RLP uses rlp int encoding of the length
*
*
* VAR_RLP = 2;
*/
VAR_RLP(2),
/**
*
* FIXED32_BIG uses big-endian encoding of the length as a 32 bit integer
*
*
* FIXED32_BIG = 3;
*/
FIXED32_BIG(3),
/**
*
* FIXED32_LITTLE uses little-endian encoding of the length as a 32 bit integer
*
*
* FIXED32_LITTLE = 4;
*/
FIXED32_LITTLE(4),
/**
*
* FIXED64_BIG uses big-endian encoding of the length as a 64 bit integer
*
*
* FIXED64_BIG = 5;
*/
FIXED64_BIG(5),
/**
*
* FIXED64_LITTLE uses little-endian encoding of the length as a 64 bit integer
*
*
* FIXED64_LITTLE = 6;
*/
FIXED64_LITTLE(6),
/**
*
* REQUIRE_32_BYTES is like NONE, but will fail if the input is not exactly 32 bytes (sha256 output)
*
*
* REQUIRE_32_BYTES = 7;
*/
REQUIRE_32_BYTES(7),
/**
*
* REQUIRE_64_BYTES is like NONE, but will fail if the input is not exactly 64 bytes (sha512 output)
*
*
* REQUIRE_64_BYTES = 8;
*/
REQUIRE_64_BYTES(8),
UNRECOGNIZED(-1),
;
/**
*
* NO_PREFIX don't include any length info
*
*
* NO_PREFIX = 0;
*/
public static final int NO_PREFIX_VALUE = 0;
/**
*
* VAR_PROTO uses protobuf (and go-amino) varint encoding of the length
*
*
* VAR_PROTO = 1;
*/
public static final int VAR_PROTO_VALUE = 1;
/**
*
* VAR_RLP uses rlp int encoding of the length
*
*
* VAR_RLP = 2;
*/
public static final int VAR_RLP_VALUE = 2;
/**
*
* FIXED32_BIG uses big-endian encoding of the length as a 32 bit integer
*
*
* FIXED32_BIG = 3;
*/
public static final int FIXED32_BIG_VALUE = 3;
/**
*
* FIXED32_LITTLE uses little-endian encoding of the length as a 32 bit integer
*
*
* FIXED32_LITTLE = 4;
*/
public static final int FIXED32_LITTLE_VALUE = 4;
/**
*
* FIXED64_BIG uses big-endian encoding of the length as a 64 bit integer
*
*
* FIXED64_BIG = 5;
*/
public static final int FIXED64_BIG_VALUE = 5;
/**
*
* FIXED64_LITTLE uses little-endian encoding of the length as a 64 bit integer
*
*
* FIXED64_LITTLE = 6;
*/
public static final int FIXED64_LITTLE_VALUE = 6;
/**
*
* REQUIRE_32_BYTES is like NONE, but will fail if the input is not exactly 32 bytes (sha256 output)
*
*
* REQUIRE_32_BYTES = 7;
*/
public static final int REQUIRE_32_BYTES_VALUE = 7;
/**
*
* REQUIRE_64_BYTES is like NONE, but will fail if the input is not exactly 64 bytes (sha512 output)
*
*
* REQUIRE_64_BYTES = 8;
*/
public static final int REQUIRE_64_BYTES_VALUE = 8;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LengthOp valueOf(int value) {
return forNumber(value);
}
public static LengthOp forNumber(int value) {
switch (value) {
case 0: return NO_PREFIX;
case 1: return VAR_PROTO;
case 2: return VAR_RLP;
case 3: return FIXED32_BIG;
case 4: return FIXED32_LITTLE;
case 5: return FIXED64_BIG;
case 6: return FIXED64_LITTLE;
case 7: return REQUIRE_32_BYTES;
case 8: return REQUIRE_64_BYTES;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LengthOp> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LengthOp findValueByNumber(int number) {
return LengthOp.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return tech.confio.ics23.Proofs.getDescriptor().getEnumTypes().get(1);
}
private static final LengthOp[] VALUES = values();
public static LengthOp valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private LengthOp(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ics23.LengthOp)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy