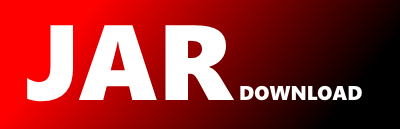
io.pyroscope.javaagent.config.AppName Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of agent Show documentation
Show all versions of agent Show documentation
The Java profiling agent for Pyroscope.io. Based on async-profiler.
package io.pyroscope.javaagent.config;
import org.jetbrains.annotations.NotNull;
import java.util.*;
public class AppName {
final String name;
final Map labels;
public AppName(String name, Map labels) {
this.name = name;
this.labels = Collections.unmodifiableMap(new TreeMap<>(labels));
}
@Override
public String toString() {
if (labels.isEmpty()) {
return name;
}
StringJoiner joinedLabels = new StringJoiner(",");
for (Map.Entry e : this.labels.entrySet()) {
joinedLabels.add((e.getKey().trim()) + "=" + (e.getValue().trim()));
}
return String.format("%s{%s}", name, joinedLabels);
}
public Builder newBuilder() {
return new Builder(name, labels);
}
public static class Builder {
private String name;
private Map labels;
public Builder(String name) {
this.name = name;
this.labels = new TreeMap<>();
}
public Builder(String name, Map labels) {
this.name = name;
this.labels = new TreeMap<>(labels);
}
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder addLabel(String k, String v) {
if (isValidLabel(k) || isValidLabel(v)) {
this.labels.put(k, v);
}
return this;
}
public Builder addLabels(Map labels) {
for (Map.Entry it : labels.entrySet()) {
addLabel(it.getKey(), it.getValue());
}
return this;
}
public AppName build() {
return new AppName(name, labels);
}
}
public static AppName parse(String appName) {
int l = appName.indexOf('{');
int r = appName.indexOf('}');
if (l != -1 && r != -1 && l < r) {
String name = appName.substring(0, l);
String strLabels = appName.substring(l + 1, r);
Map labelsMap = parseLabels(strLabels);
return new AppName(name, labelsMap);
} else {
return new AppName(appName, Collections.emptyMap());
}
}
@NotNull
public static Map parseLabels(String strLabels) {
String[] labels = strLabels.split(",");
Map labelMap = new HashMap<>();
for (String label : labels) {
String[] kv = label.split("=");
if (kv.length != 2) {
continue;
}
kv[0] = kv[0].trim();
kv[1] = kv[1].trim();
if (!isValidLabel(kv[0]) || !isValidLabel(kv[1])) {
continue;
}
labelMap.put(kv[0], kv[1]);
}
return labelMap;
}
public static boolean isValidLabel(String s) {
if (s.isEmpty()) {
return false;
}
for (int i = 0; i < s.length(); i++) {
int c = s.codePointAt(i);
if (c == '{' || c == '}' || c == ',' || c == '=' || c == ' ') {
return false;
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy