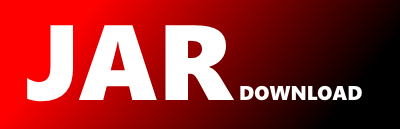
io.qameta.allure.PluginConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of allure-plugin-api Show documentation
Show all versions of allure-plugin-api Show documentation
Module allure-plugin-api of Allure Framework.
package io.qameta.allure;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for PluginConfiguration complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PluginConfiguration">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="extensions">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="extension" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="jsFiles">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="jsFile" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="cssFiles">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="cssFile" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PluginConfiguration", propOrder = {
"id",
"name",
"description",
"extensions",
"jsFiles",
"cssFiles"
})
public class PluginConfiguration implements Serializable
{
private final static long serialVersionUID = 1L;
@XmlElement(required = true)
protected String id;
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String description;
@XmlElementWrapper(required = true)
@XmlElement(name = "extension")
protected List extensions;
@XmlElementWrapper(required = true)
@XmlElement(name = "jsFile")
protected List jsFiles;
@XmlElementWrapper(required = true)
@XmlElement(name = "cssFile")
protected List cssFiles;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
public List getExtensions() {
if (extensions == null) {
extensions = new ArrayList();
}
return extensions;
}
public void setExtensions(List extensions) {
this.extensions = extensions;
}
public List getJsFiles() {
if (jsFiles == null) {
jsFiles = new ArrayList();
}
return jsFiles;
}
public void setJsFiles(List jsFiles) {
this.jsFiles = jsFiles;
}
public List getCssFiles() {
if (cssFiles == null) {
cssFiles = new ArrayList();
}
return cssFiles;
}
public void setCssFiles(List cssFiles) {
this.cssFiles = cssFiles;
}
public PluginConfiguration withId(String value) {
setId(value);
return this;
}
public PluginConfiguration withName(String value) {
setName(value);
return this;
}
public PluginConfiguration withDescription(String value) {
setDescription(value);
return this;
}
public PluginConfiguration withExtensions(String... values) {
if (values!= null) {
for (String value: values) {
getExtensions().add(value);
}
}
return this;
}
public PluginConfiguration withExtensions(Collection values) {
if (values!= null) {
getExtensions().addAll(values);
}
return this;
}
public PluginConfiguration withExtensions(List extensions) {
setExtensions(extensions);
return this;
}
public PluginConfiguration withJsFiles(String... values) {
if (values!= null) {
for (String value: values) {
getJsFiles().add(value);
}
}
return this;
}
public PluginConfiguration withJsFiles(Collection values) {
if (values!= null) {
getJsFiles().addAll(values);
}
return this;
}
public PluginConfiguration withJsFiles(List jsFiles) {
setJsFiles(jsFiles);
return this;
}
public PluginConfiguration withCssFiles(String... values) {
if (values!= null) {
for (String value: values) {
getCssFiles().add(value);
}
}
return this;
}
public PluginConfiguration withCssFiles(Collection values) {
if (values!= null) {
getCssFiles().addAll(values);
}
return this;
}
public PluginConfiguration withCssFiles(List cssFiles) {
setCssFiles(cssFiles);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy