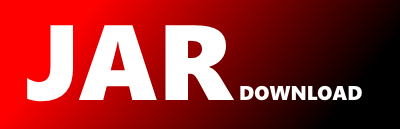
io.qameta.allure.entity.ObjectFactory Maven / Gradle / Ivy
Show all versions of allure-plugin-api Show documentation
package io.qameta.allure.entity;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the io.qameta.allure.entity package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _TestResult_QNAME = new QName("urn:entity.allure.qameta.io", "testResult");
private final static QName _StageResult_QNAME = new QName("urn:entity.allure.qameta.io", "stageResult");
private final static QName _ExecutorInfo_QNAME = new QName("urn:entity.allure.qameta.io", "executorInfo");
private final static QName _Link_QNAME = new QName("urn:entity.allure.qameta.io", "link");
private final static QName _Time_QNAME = new QName("urn:entity.allure.qameta.io", "time");
private final static QName _StatusDetails_QNAME = new QName("urn:entity.allure.qameta.io", "statusDetails");
private final static QName _Step_QNAME = new QName("urn:entity.allure.qameta.io", "step");
private final static QName _Attachment_QNAME = new QName("urn:entity.allure.qameta.io", "attachment");
private final static QName _Parameter_QNAME = new QName("urn:entity.allure.qameta.io", "parameter");
private final static QName _EnvironmentItem_QNAME = new QName("urn:entity.allure.qameta.io", "environmentItem");
private final static QName _Statistic_QNAME = new QName("urn:entity.allure.qameta.io", "statistic");
private final static QName _Label_QNAME = new QName("urn:entity.allure.qameta.io", "label");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: io.qameta.allure.entity
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link TestResult }
*
*/
public TestResult createTestResult() {
return new TestResult();
}
/**
* Create an instance of {@link StageResult }
*
*/
public StageResult createStageResult() {
return new StageResult();
}
/**
* Create an instance of {@link ExecutorInfo }
*
*/
public ExecutorInfo createExecutorInfo() {
return new ExecutorInfo();
}
/**
* Create an instance of {@link Link }
*
*/
public Link createLink() {
return new Link();
}
/**
* Create an instance of {@link Time }
*
*/
public Time createTime() {
return new Time();
}
/**
* Create an instance of {@link StatusDetails }
*
*/
public StatusDetails createStatusDetails() {
return new StatusDetails();
}
/**
* Create an instance of {@link Step }
*
*/
public Step createStep() {
return new Step();
}
/**
* Create an instance of {@link Attachment }
*
*/
public Attachment createAttachment() {
return new Attachment();
}
/**
* Create an instance of {@link Parameter }
*
*/
public Parameter createParameter() {
return new Parameter();
}
/**
* Create an instance of {@link EnvironmentItem }
*
*/
public EnvironmentItem createEnvironmentItem() {
return new EnvironmentItem();
}
/**
* Create an instance of {@link Statistic }
*
*/
public Statistic createStatistic() {
return new Statistic();
}
/**
* Create an instance of {@link Label }
*
*/
public Label createLabel() {
return new Label();
}
/**
* Create an instance of {@link GroupTime }
*
*/
public GroupTime createGroupTime() {
return new GroupTime();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TestResult }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:entity.allure.qameta.io", name = "testResult")
public JAXBElement createTestResult(TestResult value) {
return new JAXBElement(_TestResult_QNAME, TestResult.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link StageResult }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:entity.allure.qameta.io", name = "stageResult")
public JAXBElement createStageResult(StageResult value) {
return new JAXBElement(_StageResult_QNAME, StageResult.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ExecutorInfo }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:entity.allure.qameta.io", name = "executorInfo")
public JAXBElement createExecutorInfo(ExecutorInfo value) {
return new JAXBElement(_ExecutorInfo_QNAME, ExecutorInfo.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Link }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:entity.allure.qameta.io", name = "link")
public JAXBElement createLink(Link value) {
return new JAXBElement(_Link_QNAME, Link.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link Time }{@code >}}
*
*/
@XmlElementDecl(namespace = "urn:entity.allure.qameta.io", name = "time")
public JAXBElement