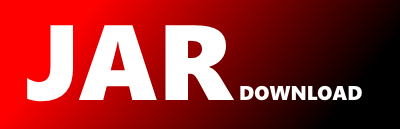
io.qameta.allure.model.TestResultContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of allure2-model-pojo Show documentation
Show all versions of allure2-model-pojo Show documentation
Module contains Allure2 model pojo
package io.qameta.allure.model;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for TestResultContainer complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="TestResultContainer">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <all>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="children" type="{urn:model.allure.qameta.io}Ids"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="descriptionHtml" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="befores" type="{urn:model.allure.qameta.io}Befores"/>
* <element name="afters" type="{urn:model.allure.qameta.io}Afters"/>
* <element name="links" type="{urn:model.allure.qameta.io}Links"/>
* </all>
* <attribute name="start" type="{http://www.w3.org/2001/XMLSchema}long" />
* <attribute name="stop" type="{http://www.w3.org/2001/XMLSchema}long" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "TestResultContainer", propOrder = {
})
public class TestResultContainer implements Serializable
{
private final static long serialVersionUID = 1L;
@XmlElement(required = true)
protected String id;
@XmlElement(required = true)
protected String name;
@XmlElementWrapper(required = true)
@XmlElement(name = "childId")
protected List children;
@XmlElement(required = true)
protected String description;
@XmlElement(required = true)
protected String descriptionHtml;
@XmlElementWrapper(required = true)
@XmlElement(name = "before")
protected List befores;
@XmlElementWrapper(required = true)
@XmlElement(name = "after")
protected List afters;
@XmlElementWrapper(required = true)
@XmlElement(name = "link")
protected List links;
@XmlAttribute(name = "start")
protected Long start;
@XmlAttribute(name = "stop")
protected Long stop;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the descriptionHtml property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescriptionHtml() {
return descriptionHtml;
}
/**
* Sets the value of the descriptionHtml property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescriptionHtml(String value) {
this.descriptionHtml = value;
}
/**
* Gets the value of the start property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getStart() {
return start;
}
/**
* Sets the value of the start property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setStart(Long value) {
this.start = value;
}
/**
* Gets the value of the stop property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getStop() {
return stop;
}
/**
* Sets the value of the stop property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setStop(Long value) {
this.stop = value;
}
public List getChildren() {
if (children == null) {
children = new ArrayList();
}
return children;
}
public void setChildren(List children) {
this.children = children;
}
public List getBefores() {
if (befores == null) {
befores = new ArrayList();
}
return befores;
}
public void setBefores(List befores) {
this.befores = befores;
}
public List getAfters() {
if (afters == null) {
afters = new ArrayList();
}
return afters;
}
public void setAfters(List afters) {
this.afters = afters;
}
public List getLinks() {
if (links == null) {
links = new ArrayList();
}
return links;
}
public void setLinks(List links) {
this.links = links;
}
public TestResultContainer withId(String value) {
setId(value);
return this;
}
public TestResultContainer withName(String value) {
setName(value);
return this;
}
public TestResultContainer withDescription(String value) {
setDescription(value);
return this;
}
public TestResultContainer withDescriptionHtml(String value) {
setDescriptionHtml(value);
return this;
}
public TestResultContainer withStart(Long value) {
setStart(value);
return this;
}
public TestResultContainer withStop(Long value) {
setStop(value);
return this;
}
public TestResultContainer withChildren(String... values) {
if (values!= null) {
for (String value: values) {
getChildren().add(value);
}
}
return this;
}
public TestResultContainer withChildren(Collection values) {
if (values!= null) {
getChildren().addAll(values);
}
return this;
}
public TestResultContainer withChildren(List children) {
setChildren(children);
return this;
}
public TestResultContainer withBefores(FixtureResult... values) {
if (values!= null) {
for (FixtureResult value: values) {
getBefores().add(value);
}
}
return this;
}
public TestResultContainer withBefores(Collection values) {
if (values!= null) {
getBefores().addAll(values);
}
return this;
}
public TestResultContainer withBefores(List befores) {
setBefores(befores);
return this;
}
public TestResultContainer withAfters(FixtureResult... values) {
if (values!= null) {
for (FixtureResult value: values) {
getAfters().add(value);
}
}
return this;
}
public TestResultContainer withAfters(Collection values) {
if (values!= null) {
getAfters().addAll(values);
}
return this;
}
public TestResultContainer withAfters(List afters) {
setAfters(afters);
return this;
}
public TestResultContainer withLinks(Link... values) {
if (values!= null) {
for (Link value: values) {
getLinks().add(value);
}
}
return this;
}
public TestResultContainer withLinks(Collection values) {
if (values!= null) {
getLinks().addAll(values);
}
return this;
}
public TestResultContainer withLinks(List links) {
setLinks(links);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy