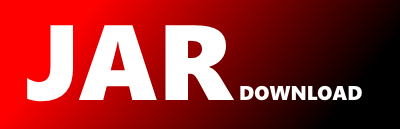
io.army.jdbd.JdbdExecutorSupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of army-jdbd Show documentation
Show all versions of army-jdbd Show documentation
Army jdbc module,provide jdbd executor.
The newest version!
/*
* Copyright 2023-2043 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.army.jdbd;
import io.army.criteria.Selection;
import io.army.reactive.ReactiveMultiResultSpec;
import io.army.reactive.executor.ReactiveExecutorSupport;
import io.army.session.DataAccessException;
import io.army.session.Option;
import io.army.session.record.CurrentRecord;
import io.army.session.record.FieldType;
import io.army.session.record.KeyType;
import io.army.session.record.ResultStates;
import io.army.sqltype.DataType;
import io.army.util._Collections;
import io.army.util._Exceptions;
import io.jdbd.result.ResultRowMeta;
import reactor.core.publisher.Flux;
import javax.annotation.Nullable;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.Function;
import java.util.function.Supplier;
abstract class JdbdExecutorSupport extends ReactiveExecutorSupport {
JdbdExecutorSupport() {
}
private static abstract class JdbdRecordMeta extends ArmyResultRecordMeta {
final JdbdStmtExecutor executor;
final ResultRowMeta meta;
private Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy