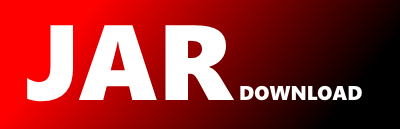
io.army.criteria.impl.PostgreDocumentFunctions Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.criteria.*;
import io.army.dialect._DialectUtils;
import io.army.mapping.*;
import io.army.mapping.array.TextArrayType;
import io.army.mapping.array.XmlArrayType;
import io.army.mapping.optional.JsonPathType;
import io.army.mapping.postgre.PostgreTsQueryType;
import io.army.mapping.postgre.PostgreTsVectorType;
import io.army.meta.TableMeta;
import io.army.util.ArrayUtils;
import io.army.util._Collections;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Consumer;
/**
*
* This class hold tsvectory/ tsquery/xml /json/jsonb function method.
* * @since 1.0
*/
//@SuppressWarnings("unused")
abstract class PostgreDocumentFunctions extends PostgreMiscellaneous2Functions {
/**
* package constructor
*/
PostgreDocumentFunctions() {
}
public interface XmlNameSpaces extends Item {
}
public interface NullTreatMode extends Expression {
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextArrayType} with one dimension array.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #arrayToTsVector(Expression)
* @see array_to_tsvector ( text[] ) → tsvector
*/
public static SimpleExpression arrayToTsVector(BiFunction funcRef, T value) {
return arrayToTsVector(funcRef.apply(TextArrayType.from(String[].class), value));
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see array_to_tsvector ( text[] ) → tsvector
*/
public static SimpleExpression arrayToTsVector(Expression exp) {
return FunctionUtils.oneArgFunc("ARRAY_TO_TSVECTOR", exp, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #plainToTsQuery(Expression)
* @see plainto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression plainToTsQuery(BiFunction funcRefForQuery, T query) {
return plainToTsQuery(funcRefForQuery.apply(TextType.INSTANCE, query));
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see plainto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression plainToTsQuery(Expression query) {
return FunctionUtils.oneArgFunc("PLAINTO_TSQUERY", query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForConfig the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForConfig always is {@link StringType#INSTANCE}.
* @param config non-null,it will be passed to funcRefForConfig as the second argument of funcRefForConfig
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #plainToTsQuery(Expression, Expression)
* @see plainto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression plainToTsQuery(BiFunction funcRefForConfig, T config,
BiFunction funcRefForQuery, U query) {
return plainToTsQuery(funcRefForConfig.apply(StringType.INSTANCE, config),
funcRefForQuery.apply(TextType.INSTANCE, query)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see plainto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression plainToTsQuery(Expression config, Expression query) {
return FunctionUtils.twoArgFunc("PLAINTO_TSQUERY", config, query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* he first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #phraseToTsQuery(Expression)
* @see phraseto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression phraseToTsQuery(BiFunction funcRefForQuery, T query) {
return phraseToTsQuery(funcRefForQuery.apply(TextType.INSTANCE, query));
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see phraseto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression phraseToTsQuery(Expression query) {
return FunctionUtils.oneArgFunc("PHRASETO_TSQUERY", query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForConfig the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForConfig always is {@link StringType#INSTANCE}.
* @param config non-null,it will be passed to funcRefForConfig as the second argument of funcRefForConfig
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* he first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #phraseToTsQuery(Expression, Expression)
* @see phraseto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression phraseToTsQuery(BiFunction funcRefForConfig, T config,
BiFunction funcRefForQuery, U query) {
return phraseToTsQuery(funcRefForConfig.apply(StringType.INSTANCE, config),
funcRefForQuery.apply(TextType.INSTANCE, query)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see phraseto_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression phraseToTsQuery(Expression config, Expression query) {
return FunctionUtils.twoArgFunc("PHRASETO_TSQUERY", config, query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #webSearchToTsQuery(Expression)
* @see websearch_to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression webSearchToTsQuery(BiFunction funcRefForQuery, T query) {
return webSearchToTsQuery(funcRefForQuery.apply(TextType.INSTANCE, query));
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see websearch_to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression webSearchToTsQuery(Expression query) {
return FunctionUtils.oneArgFunc("WEBSEARCH_TO_TSQUERY", query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForConfig the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForConfig always is {@link StringType#INSTANCE}.
* @param config non-null,it will be passed to funcRefForConfig as the second argument of funcRefForConfig
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #webSearchToTsQuery(Expression, Expression)
* @see websearch_to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression webSearchToTsQuery(BiFunction funcRefForConfig, T config,
BiFunction funcRefForQuery, U query) {
return webSearchToTsQuery(funcRefForConfig.apply(StringType.INSTANCE, config),
funcRefForQuery.apply(TextType.INSTANCE, query)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see websearch_to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression webSearchToTsQuery(Expression config, Expression query) {
return FunctionUtils.twoArgFunc("WEBSEARCH_TO_TSQUERY", config, query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType}
* *
*
* @see querytree ( tsquery ) → text
*/
public static SimpleExpression queryTree(Expression tsQuery) {
return FunctionUtils.oneArgFunc("QUERYTREE", tsQuery, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRefForVector the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVector always is {@link PostgreTsVectorType#INSTANCE}.
* @param vector non-null,it will be passed to funcRefForVector as the second argument of funcRefForVector
* @param funcRefForWeight the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForWeight always is {@link CharacterType#INSTANCE}.
* @param weight non-null,it will be passed to funcRefForWeight as the second argument of funcRefForWeight
* @see #setWeight(Expression, Expression)
* @see setweight ( vector tsvector, weight "char" ) → tsvector
* Assigns the specified weight to each element of the vector.
* setweight('fat:2,4 cat:3 rat:5B'::tsvector, 'A') → 'cat':3A 'fat':2A,4A 'rat':5A
*
*/
public static SimpleExpression setWeight(BiFunction funcRefForVector, T vector,
BiFunction funcRefForWeight, U weight) {
return setWeight(funcRefForVector.apply(PostgreTsVectorType.INSTANCE, vector),
funcRefForWeight.apply(CharacterType.INSTANCE, weight)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see setweight ( vector tsvector, weight "char" ) → tsvector
* Assigns the specified weight to each element of the vector.
* setweight('fat:2,4 cat:3 rat:5B'::tsvector, 'A') → 'cat':3A 'fat':2A,4A 'rat':5A
*
*/
public static SimpleExpression setWeight(Expression vector, Expression weight) {
return FunctionUtils.twoArgFunc("SETWEIGHT", vector, weight, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRefForVector the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVector always is {@link PostgreTsVectorType#INSTANCE}.
* @param vector non-null,it will be passed to funcRefForVector as the second argument of funcRefForVector
* @param funcRefForWeight the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForWeight always is {@link CharacterType#INSTANCE}.
* @param weight non-null,it will be passed to funcRefForWeight as the second argument of funcRefForWeight
* @param funcRefForLexemes the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForLexemes always is {@link TextArrayType} with one dimension array.
* @param lexemes non-null,it will be passed to funcRefForLexemes as the second argument of funcRefForLexemes
* @see #setWeight(Expression, Expression, Expression)
* @see setweight ( vector tsvector, weight "char", lexemes text[] ) → tsvector
* Assigns the specified weight to elements of the vector that are listed in lexemes. The strings in lexemes are taken as lexemes as-is, without further
* processing. Strings that do not match any lexeme in vector are ignored.
* setweight('fat:2,4 cat:3 rat:5,6B'::tsvector, 'A', '{cat,rat}') → 'cat':3A 'fat':2,4 'rat':5A,6A
*
*/
public static SimpleExpression setWeight(BiFunction funcRefForVector, T vector,
BiFunction funcRefForWeight, U weight,
BiFunction funcRefForLexemes, V lexemes) {
return setWeight(funcRefForVector.apply(PostgreTsVectorType.INSTANCE, vector),
funcRefForWeight.apply(CharacterType.INSTANCE, weight),
funcRefForLexemes.apply(TextArrayType.from(String[].class), lexemes)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see setweight ( vector tsvector, weight "char", lexemes text[] ) → tsvector
* Assigns the specified weight to elements of the vector that are listed in lexemes. The strings in lexemes are taken as lexemes as-is, without further
* processing. Strings that do not match any lexeme in vector are ignored.
* setweight('fat:2,4 cat:3 rat:5,6B'::tsvector, 'A', '{cat,rat}') → 'cat':3A 'fat':2,4 'rat':5A,6A
*
*/
public static SimpleExpression setWeight(Expression vector, Expression weight, Expression lexemes) {
return FunctionUtils.threeArgFunc("SETWEIGHT", vector, weight, lexemes, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link PostgreTsVectorType} with one dimension array.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #strip(Expression)
* @see array_to_tsvector ( text[] ) → tsvector
*/
public static SimpleExpression strip(BiFunction funcRef, T value) {
return strip(funcRef.apply(PostgreTsVectorType.INSTANCE, value));
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see strip ( tsvector ) → tsvector
* Removes positions and weights from the tsvector.
* strip('fat:2,4 cat:3 rat:5A'::tsvector) → 'cat' 'fat' 'rat'
*
*/
public static SimpleExpression strip(Expression tsVector) {
return FunctionUtils.oneArgFunc("STRIP", tsVector, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #toTsQuery(Expression)
* @see to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression toTsQuery(BiFunction funcRefForQuery, T query) {
return toTsQuery(funcRefForQuery.apply(TextType.INSTANCE, query));
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression toTsQuery(Expression query) {
return FunctionUtils.oneArgFunc("TO_TSQUERY", query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @param funcRefForConfig the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForConfig always is {@link StringType#INSTANCE}.
* @param config non-null,it will be passed to funcRefForConfig as the second argument of funcRefForConfig
* @param funcRefForQuery the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForQuery always is {@link TextType#INSTANCE}.
* @param query non-null,it will be passed to funcRefForQuery as the second argument of funcRefForQuery
* @see #toTsQuery(Expression, Expression)
* @see to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression toTsQuery(BiFunction funcRefForConfig, T config,
BiFunction funcRefForQuery, U query) {
return toTsQuery(funcRefForConfig.apply(StringType.INSTANCE, config),
funcRefForQuery.apply(TextType.INSTANCE, query)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see to_tsquery ( [ config regconfig, ] query text ) → tsquery
*/
public static SimpleExpression toTsQuery(Expression config, Expression query) {
return FunctionUtils.twoArgFunc("TO_TSQUERY", config, query, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see #toTsVector(Expression, Expression)
* @see to_tsvector ( [ config regconfig, ] document text ) → tsvector
*/
public static SimpleExpression toTsVector(Expression document) {
return FunctionUtils.oneArgFunc("TO_TSVECTOR", document, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see to_tsvector ( [ config regconfig, ] document text ) → tsvector
*/
public static SimpleExpression toTsVector(Expression config, Expression document) {
return FunctionUtils.twoArgFunc("TO_TSVECTOR", config, document, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRefForDocument the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForDocument always is {@link JsonType#TEXT}.
* @param document non-null,it will be passed to funcRefForDocument as the second argument of funcRefForDocument
* @param funcRefForFilter the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForFilter always is {@link JsonbType#TEXT} .
* @param filter non-null,it will be passed to funcRefForFilter as the second argument of funcRefForFilter
* @see #jsonToTsVector(Expression, Expression)
* @see json_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonToTsVector(BiFunction funcRefForDocument, T document,
BiFunction funcRefForFilter, U filter) {
return jsonToTsVector(funcRefForDocument.apply(JsonType.TEXT, document),
funcRefForFilter.apply(JsonbType.TEXT, filter)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see json_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonToTsVector(Expression document, Expression filter) {
return FunctionUtils.twoArgFunc("JSON_TO_TSVECTOR", document, filter, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRefForConfig the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForConfig always is {@link StringType#INSTANCE}.
* @param config non-null,it will be passed to funcRefForConfig as the second argument of funcRefForConfig
* @param funcRefForDocument the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForDocument always is {@link JsonType#TEXT}.
* @param document non-null,it will be passed to funcRefForDocument as the second argument of funcRefForDocument
* @param funcRefForFilter the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForFilter always is {@link JsonbType#TEXT} .
* @param filter non-null,it will be passed to funcRefForFilter as the second argument of funcRefForFilter
* @see #jsonToTsVector(Expression, Expression, Expression)
* @see json_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonToTsVector(BiFunction funcRefForConfig, T config,
BiFunction funcRefForDocument, U document,
BiFunction funcRefForFilter, V filter) {
return jsonToTsVector(funcRefForConfig.apply(StringType.INSTANCE, config),
funcRefForDocument.apply(JsonType.TEXT, document),
funcRefForFilter.apply(JsonbType.TEXT, filter)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see json_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonToTsVector(Expression config, Expression document, Expression filter) {
return FunctionUtils.threeArgFunc("JSON_TO_TSVECTOR", config, document, filter, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRefForDocument the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForDocument always is {@link JsonType#TEXT}.
* @param document non-null,it will be passed to funcRefForDocument as the second argument of funcRefForDocument
* @param funcRefForFilter the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForFilter always is {@link JsonbType#TEXT} .
* @param filter non-null,it will be passed to funcRefForFilter as the second argument of funcRefForFilter
* @see #jsonbToTsVector(Expression, Expression)
* @see jsonb_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonbToTsVector(BiFunction funcRefForDocument, T document,
BiFunction funcRefForFilter, U filter) {
return jsonbToTsVector(funcRefForDocument.apply(JsonType.TEXT, document),
funcRefForFilter.apply(JsonbType.TEXT, filter)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see jsonb_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonbToTsVector(Expression document, Expression filter) {
return FunctionUtils.twoArgFunc("JSONB_TO_TSVECTOR", document, filter, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @param funcRefForConfig the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForConfig always is {@link StringType#INSTANCE}.
* @param config non-null,it will be passed to funcRefForConfig as the second argument of funcRefForConfig
* @param funcRefForDocument the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForDocument always is {@link JsonType#TEXT}.
* @param document non-null,it will be passed to funcRefForDocument as the second argument of funcRefForDocument
* @param funcRefForFilter the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* the first argument of funcRefForFilter always is {@link JsonbType#TEXT} .
* @param filter non-null,it will be passed to funcRefForFilter as the second argument of funcRefForFilter
* @see #jsonbToTsVector(Expression, Expression, Expression)
* @see jsonb_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonbToTsVector(BiFunction funcRefForConfig, T config,
BiFunction funcRefForDocument, U document,
BiFunction funcRefForFilter, V filter) {
return jsonbToTsVector(funcRefForConfig.apply(StringType.INSTANCE, config),
funcRefForDocument.apply(JsonType.TEXT, document),
funcRefForFilter.apply(JsonbType.TEXT, filter)
);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see jsonb_to_tsvector ( [ config regconfig, ] document json, filter jsonb ) → tsvector
*/
public static SimpleExpression jsonbToTsVector(Expression config, Expression document, Expression filter) {
return FunctionUtils.threeArgFunc("JSONB_TO_TSVECTOR", config, document, filter, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see ts_delete ( vector tsvector, lexeme text ) → tsvector
* @see ts_delete ( vector tsvector, lexemes text[] ) → tsvector
*/
public static SimpleExpression tsDelete(Expression tsVector, Expression lexeme) {
return FunctionUtils.twoArgFunc("TS_DELETE", tsVector, lexeme, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsVectorType}
* *
*
* @see ts_filter ( vector tsvector, weights "char"[] ) → tsvector
*/
public static SimpleExpression tsFilter(Expression tsVector, Expression lexeme) {
return FunctionUtils.twoArgFunc("TS_FILTER", tsVector, lexeme, PostgreTsVectorType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType}
* *
*
* @see ts_headline ( [ config regconfig, ] document text, query tsquery [, options text ] ) → text
* @see ts_headline ( [ config regconfig, ] document json, query tsquery [, options text ] ) → text
* @see ts_headline ( [ config regconfig, ] document jsonb, query tsquery [, options text ] ) → text
*/
public static SimpleExpression tsHeadline(Expression config, Expression document, Expression query, Expression options) {
return FunctionUtils.fourArgFunc("TS_HEADLINE", config, document, query, options, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType}
* *
*
* @see ts_headline ( [ config regconfig, ] document text, query tsquery [, options text ] ) → text
* @see ts_headline ( [ config regconfig, ] document json, query tsquery [, options text ] ) → text
* @see ts_headline ( [ config regconfig, ] document jsonb, query tsquery [, options text ] ) → text
*/
public static SimpleExpression tsHeadline(Expression exp1, Expression exp2, Expression exp3) {
return FunctionUtils.threeArgFunc("TS_HEADLINE", exp1, exp2, exp3, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType}
* *
*
* @see ts_headline ( [ config regconfig, ] document text, query tsquery [, options text ] ) → text
* @see ts_headline ( [ config regconfig, ] document json, query tsquery [, options text ] ) → text
* @see ts_headline ( [ config regconfig, ] document jsonb, query tsquery [, options text ] ) → text
*/
public static SimpleExpression tsHeadline(Expression document, Expression query) {
return FunctionUtils.twoArgFunc("TS_HEADLINE", document, query, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link FloatType}
* *
*
* @see ts_rank ( [ weights real[], ] vector tsvector, query tsquery [, normalization integer ] ) → real
*/
public static SimpleExpression tsRank(Expression weights, Expression vector, Expression query, Expression normalization) {
return FunctionUtils.fourArgFunc("TS_RANK", weights, vector, query, normalization, FloatType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link FloatType}
* *
*
* @see ts_rank ( [ weights real[], ] vector tsvector, query tsquery [, normalization integer ] ) → real
*/
public static SimpleExpression tsRank(Expression exp1, Expression exp2, Expression exp3) {
return FunctionUtils.threeArgFunc("TS_RANK", exp1, exp2, exp3, FloatType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link FloatType}
* *
*
* @see ts_rank ( [ weights real[], ] vector tsvector, query tsquery [, normalization integer ] ) → real
*/
public static SimpleExpression tsRank(Expression vector, Expression query) {
return FunctionUtils.twoArgFunc("TS_RANK", vector, query, FloatType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link FloatType}
* *
*
* @see ts_rank_cd ( [ weights real[], ] vector tsvector, query tsquery [, normalization integer ] ) → real
*/
public static SimpleExpression tsRankCd(Expression weights, Expression vector, Expression query, Expression normalization) {
return FunctionUtils.fourArgFunc("TS_RANK_CD", weights, vector, query, normalization, FloatType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link FloatType}
* *
*
* @see ts_rank_cd ( [ weights real[], ] vector tsvector, query tsquery [, normalization integer ] ) → real
*/
public static SimpleExpression tsRankCd(Expression exp1, Expression exp2, Expression exp3) {
return FunctionUtils.threeArgFunc("TS_RANK_CD", exp1, exp2, exp3, FloatType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link FloatType}
* *
*
* @see ts_rank_cd ( [ weights real[], ] vector tsvector, query tsquery [, normalization integer ] ) → real
*/
public static SimpleExpression tsRankCd(Expression vector, Expression query) {
return FunctionUtils.twoArgFunc("TS_RANK_CD", vector, query, FloatType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see ts_rewrite ( query tsquery, target tsquery, substitute tsquery ) → tsquery
*/
public static SimpleExpression tsRewrite(Expression query, Expression target, Expression substitute) {
return FunctionUtils.threeArgFunc("TS_REWRITE", query, target, substitute, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see ts_rewrite ( query tsquery, select text ) → tsquery
*/
public static SimpleExpression tsRewrite(Expression query, Expression select) {
return FunctionUtils.twoArgFunc("TS_REWRITE", query, select, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see tsquery_phrase ( query1 tsquery, query2 tsquery ) → tsquery
*/
public static SimpleExpression tsQueryPhrase(Expression query1, Expression query2) {
return FunctionUtils.twoArgFunc("TSQUERY_PHRASE", query1, query2, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreTsQueryType}
* *
*
* @see tsquery_phrase ( query1 tsquery, query2 tsquery, distance integer ) → tsquery
*/
public static SimpleExpression tsQueryPhrase(Expression query1, Expression query2, Expression distance) {
return FunctionUtils.threeArgFunc("TSQUERY_PHRASE", query1, query2, distance, PostgreTsQueryType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextArrayType} with one dimension.
* *
*
* @see tsvector_to_array ( tsvector ) → text[]
*/
public static SimpleExpression tsVectorToArray(Expression tsVector) {
return FunctionUtils.oneArgFunc("TSVECTOR_TO_ARRAY", tsVector, TextArrayType.from(String[].class));
}
/*-------------------below XML function -------------------*/
/**
* @param option {@link Postgres#DOCUMENT} or {@link Postgres#CONTENT}
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextType#INSTANCE}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #xmlParse(SQLs.DocumentValueOption, Expression)
* @see XMLPARSE ( { DOCUMENT | CONTENT } value)
*
*/
public static SimpleExpression xmlParse(final SQLs.DocumentValueOption option, BiFunction funcRef, String value) {
return xmlParse(option, funcRef.apply(TextType.INSTANCE, value));
}
/**
* @param option {@link Postgres#DOCUMENT} or {@link Postgres#CONTENT}
* @see XMLPARSE ( { DOCUMENT | CONTENT } value)
*
*/
public static SimpleExpression xmlParse(final SQLs.DocumentValueOption option, final Expression value) {
final String name = "XMLPARSE";
if (!(option == Postgres.DOCUMENT || option == Postgres.CONTENT)) {
throw CriteriaUtils.funcArgError(name, option);
} else if (!(value instanceof FunctionArg.SingleFunctionArg)) {
throw CriteriaUtils.funcArgError(name, value);
}
return FunctionUtils.complexArgFunc(name, XmlType.TEXT, option, value);
}
/**
* @param option {@link Postgres#DOCUMENT} or {@link Postgres#CONTENT}
* @see XMLSERIALIZE ( { DOCUMENT | CONTENT } value AS type )
*
*/
public static SimpleExpression xmlSerialize(final SQLs.DocumentValueOption option, final Expression value, final SQLs.WordAs as,
final MappingType type) {
final String name = "XMLSERIALIZE";
if (!(option == Postgres.DOCUMENT || option == Postgres.CONTENT)) {
throw CriteriaUtils.funcArgError(name, option);
} else if (!(value instanceof FunctionArg.SingleFunctionArg)) {
throw CriteriaUtils.funcArgError(name, value);
} else if (as != SQLs.AS) {
throw CriteriaUtils.funcArgError(name, as);
}
return FunctionUtils.complexArgFunc(name, XmlType.TEXT, option, value, as, type);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see Xmlforest
*
*/
public SimpleExpression xmlForest(Consumer consumer) {
final PostgreFunctionUtils.XmlNamedElementPart part;
part = PostgreFunctionUtils.xmlForest();
consumer.accept(part);
return part.endNamedPart();
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType}
* *
*
* @see xmlcomment ( text ) → xml
*/
public static SimpleExpression xmlComment(Expression exp) {
return FunctionUtils.oneArgFunc("XMLCOMMENT", exp, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType}
* *
*
* @see xmlconcat ( xml [, ...] ) → xml
*/
public static SimpleExpression xmlConcat(Expression xmls) {
return FunctionUtils.oneOrMultiArgFunc("XMLCONCAT", xmls, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType}
* *
*
* @see xmlconcat ( xml [, ...] ) → xml
*/
public static SimpleExpression xmlConcat(Expression xml1, Expression... xml2) {
return FunctionUtils.oneAndRestFunc("XMLCONCAT", XmlType.TEXT, xml1, xml2);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType}
* *
*
* @see xmlconcat ( xml [, ...] ) → xml
*/
public static SimpleExpression xmlConcat(List xmlList) {
return FunctionUtils.multiArgFunc("XMLCONCAT", xmlList, XmlType.TEXT);
}
/**
*
* Note:This function cannot exist independently,see {@link #xmlElement(Postgres.WordName, String, XmlAttributes, Expression...)}
* *
*
* @see 9.15.1.3. Xmlelement
* xmlelement ( NAME name [, XMLATTRIBUTES ( attvalue [ AS attname ] [, ...] ) ] [, content [, ...]] ) → xml
*
*/
public static XmlAttributes xmlAttributes(Consumer consumer) {
final PostgreFunctionUtils.XmlNamedElementPart part;
part = PostgreFunctionUtils.xmlAttributes();
consumer.accept(part);
return part.endNamedPart();
}
/**
* @param wordName see {@link Postgres#NAME}
* @param name The nam items shown in the syntax are simple identifiers, not values.
* @param attributes see {@link #xmlAttributes(Consumer)}
* @see 9.15.1.3. Xmlelement
* xmlelement ( NAME name [, XMLATTRIBUTES ( attvalue [ AS attname ] [, ...] ) ] [, content [, ...]] ) → xml
*
*/
public static SimpleExpression xmlElement(Postgres.WordName wordName, String name, XmlAttributes attributes,
Expression... contents) {
ContextStack.assertNonNull(attributes);
ContextStack.assertNonNull(contents);
return _xmlElement(wordName, name, attributes, c -> {
for (Expression content : contents) {
c.accept(FuncWord.COMMA);
c.accept(content);
}
});
}
/**
* @param wordName see {@link Postgres#NAME}
* @param name The nam items shown in the syntax are simple identifiers, not values.
* @see 9.15.1.3. Xmlelement
* xmlelement ( NAME name [, XMLATTRIBUTES ( attvalue [ AS attname ] [, ...] ) ] [, content [, ...]] ) → xml
*
*/
public static SimpleExpression xmlElement(Postgres.WordName wordName, String name, Expression... contents) {
ContextStack.assertNonNull(contents);
return _xmlElement(wordName, name, null, c -> {
for (Expression content : contents) {
c.accept(FuncWord.COMMA);
c.accept(content);
}
});
}
/**
* @param wordName see {@link Postgres#NAME}
* @param name The nam items shown in the syntax are simple identifiers, not values.
* @param attributes see {@link #xmlAttributes(Consumer)}
* @see 9.15.1.3. Xmlelement
* xmlelement ( NAME name [, XMLATTRIBUTES ( attvalue [ AS attname ] [, ...] ) ] [, content [, ...]] ) → xml
*
*/
public static SimpleExpression xmlElement(Postgres.WordName wordName, String name, XmlAttributes attributes,
List contentList) {
ContextStack.assertNonNull(attributes);
return _xmlElement(wordName, name, attributes, c -> {
for (Expression content : contentList) {
c.accept(FuncWord.COMMA);
c.accept(content);
}
});
}
/**
* @param wordName see {@link Postgres#NAME}
* @param name The nam items shown in the syntax are simple identifiers, not values.
* @see 9.15.1.3. Xmlelement
* xmlelement ( NAME name [, XMLATTRIBUTES ( attvalue [ AS attname ] [, ...] ) ] [, content [, ...]] ) → xml
*
*/
public static SimpleExpression xmlElement(Postgres.WordName wordName, String name,
List contentList) {
return _xmlElement(wordName, name, null, c -> {
for (Expression content : contentList) {
c.accept(FuncWord.COMMA);
c.accept(content);
}
});
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlPi(Postgres.WordName, String, Expression)
* @see xmlpi ( NAME name [, content ] ) → xml
*
*/
public static SimpleExpression xmlPi(Postgres.WordName wordName, String name) {
return _xmlPi(wordName, name, null);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlPi(Postgres.WordName, String)
* @see xmlpi ( NAME name [, content ] ) → xml
*
*/
public static SimpleExpression xmlPi(Postgres.WordName wordName, String name,
BiFunction funcRef, String content) {
final Expression contentExp;
contentExp = funcRef.apply(StringType.INSTANCE, content);
ContextStack.assertNonNull(contentExp);
return _xmlPi(wordName, name, contentExp);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlPi(Postgres.WordName, String)
* @see xmlpi ( NAME name [, content ] ) → xml
*
*/
public static SimpleExpression xmlPi(Postgres.WordName wordName, String name, Expression content) {
ContextStack.assertNonNull(content);
return _xmlPi(wordName, name, content);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlRoot(Expression, WordVersion, Expression)
* @see #xmlRoot(Expression, WordVersion, WordsNoValue, WordStandalone, StandaloneOption)
* @see #xmlRoot(Expression, WordVersion, Expression, WordStandalone, StandaloneOption)
* @see xmlroot ( xml, VERSION {text|NO VALUE} [, STANDALONE {YES|NO|NO VALUE} ] ) → xml
*
*/
public static SimpleExpression xmlRoot(Expression xml, WordVersion version, WordsNoValue noValue) {
return _xmlRoot(xml, version, noValue, Postgres.STANDALONE, null);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlRoot(Expression, WordVersion, WordsNoValue)
* @see #xmlRoot(Expression, WordVersion, WordsNoValue, WordStandalone, StandaloneOption)
* @see #xmlRoot(Expression, WordVersion, Expression, WordStandalone, StandaloneOption)
* @see xmlroot ( xml, VERSION {text|NO VALUE} [, STANDALONE {YES|NO|NO VALUE} ] ) → xml
*
*/
public static SimpleExpression xmlRoot(Expression xml, WordVersion version, Expression text) {
return _xmlRoot(xml, version, text, Postgres.STANDALONE, null);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlRoot(Expression, WordVersion, WordsNoValue)
* @see #xmlRoot(Expression, WordVersion, Expression)
* @see #xmlRoot(Expression, WordVersion, Expression, WordStandalone, StandaloneOption)
* @see xmlroot ( xml, VERSION {text|NO VALUE} [, STANDALONE {YES|NO|NO VALUE} ] ) → xml
*
*/
public static SimpleExpression xmlRoot(Expression xml, WordVersion version, WordsNoValue noValue,
WordStandalone standalone, StandaloneOption option) {
ContextStack.assertNonNull(option);
return _xmlRoot(xml, version, noValue, standalone, option);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see #xmlRoot(Expression, WordVersion, WordsNoValue)
* @see #xmlRoot(Expression, WordVersion, Expression)
* @see #xmlRoot(Expression, WordVersion, WordsNoValue, WordStandalone, StandaloneOption)
* @see xmlroot ( xml, VERSION {text|NO VALUE} [, STANDALONE {YES|NO|NO VALUE} ] ) → xml
*
*/
public static SimpleExpression xmlRoot(Expression xml, WordVersion version, Expression text,
WordStandalone standalone, StandaloneOption option) {
ContextStack.assertNonNull(option);
return _xmlRoot(xml, version, text, standalone, option);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see xmlagg ( xml ) → xml
*
*/
public static SimpleExpression xmlAgg(Expression xml) {
return FunctionUtils.oneArgFunc("XMLAGG", xml, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}
* *
*
* @see xmlagg ( xml ) → xml
*
*/
public static SimpleExpression xmlAgg(Expression xml, Consumer consumer) {
final FunctionUtils.OrderByOptionClause clause;
clause = FunctionUtils.orderByOptionClause();
consumer.accept(clause);
clause.endOrderByClauseIfNeed();
final SimpleExpression func;
final String name = "XMLAGG";
if (clause.orderByList().size() == 0) {
func = FunctionUtils.oneArgFunc(name, xml, XmlType.TEXT);
} else {
func = FunctionUtils.complexArgFunc(name, XmlType.TEXT, xml, clause);
}
return func;
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see #xmlExists(Expression, WordPassing, PassingOption, Expression)
* @see #xmlExists(Expression, WordPassing, Expression, PassingOption)
* @see #xmlExists(Expression, WordPassing, PassingOption, Expression, PassingOption)
* @see XMLEXISTS ( text PASSING [BY {REF|VALUE}] xml [BY {REF|VALUE}] ) → boolean
*
*/
public static SimplePredicate xmlExists(Expression text, WordPassing passing, Expression xml) {
return _xmlExists(text, passing, null, xml, null);
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see #xmlExists(Expression, WordPassing, Expression)
* @see #xmlExists(Expression, WordPassing, PassingOption, Expression)
* @see #xmlExists(Expression, WordPassing, PassingOption, Expression, PassingOption)
* @see XMLEXISTS ( text PASSING [BY {REF|VALUE}] xml [BY {REF|VALUE}] ) → boolean
*
*/
public static SimplePredicate xmlExists(Expression text, WordPassing passing, Expression xml,
PassingOption xmlOption) {
ContextStack.assertNonNull(xmlOption);
return _xmlExists(text, passing, null, xml, xmlOption);
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see #xmlExists(Expression, WordPassing, Expression)
* @see #xmlExists(Expression, WordPassing, Expression, PassingOption)
* @see #xmlExists(Expression, WordPassing, PassingOption, Expression, PassingOption)
* @see XMLEXISTS ( text PASSING [BY {REF|VALUE}] xml [BY {REF|VALUE}] ) → boolean
*
*/
public static SimplePredicate xmlExists(Expression text, WordPassing passing, PassingOption textOption,
Expression xml) {
ContextStack.assertNonNull(textOption);
return _xmlExists(text, passing, textOption, xml, null);
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see #xmlExists(Expression, WordPassing, Expression)
* @see #xmlExists(Expression, WordPassing, PassingOption, Expression)
* @see #xmlExists(Expression, WordPassing, Expression, PassingOption)
* @see XMLEXISTS ( text PASSING [BY {REF|VALUE}] xml [BY {REF|VALUE}] ) → boolean
*
*/
public static SimplePredicate xmlExists(Expression text, WordPassing passing, PassingOption textOption,
Expression xml, PassingOption xmlOption) {
ContextStack.assertNonNull(textOption);
ContextStack.assertNonNull(xmlOption);
return _xmlExists(text, passing, textOption, xml, xmlOption);
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see xml_is_well_formed ( text ) → boolean
*
*/
public static SimplePredicate xmlIsWellFormed(Expression text) {
return FunctionUtils.oneArgPredicateFunc("XML_IS_WELL_FORMED", text);
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see xml_is_well_formed_document ( text ) → boolean
*
*/
public static SimplePredicate xmlIsWellFormedDocument(Expression text) {
return FunctionUtils.oneArgPredicateFunc("XML_IS_WELL_FORMED_DOCUMENT", text);
}
/**
*
* The {@link MappingType} of function return type: {@link Boolean}
* *
*
* @see xml_is_well_formed_content ( text ) → boolean
*
*/
public static SimplePredicate xmlIsWellFormedContent(Expression text) {
return FunctionUtils.oneArgPredicateFunc("XML_IS_WELL_FORMED_CONTENT", text);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see #xpath(Expression, Expression, Expression)
* @see xpath ( xpath text, xml xml [, nsarray text[] ] ) → xml[]
*
*/
public static SimpleExpression xpath(Expression xpath, Expression xml) {
return FunctionUtils.twoArgFunc("XPATH", xpath, xml, XmlArrayType.TEXT_LINEAR);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see xpath ( xpath text, xml xml [, nsarray text[] ] ) → xml[]
*
*/
public static SimpleExpression xpath(Expression xpath, Expression xml, Expression nsArray) {
return FunctionUtils.threeArgFunc("XPATH", xpath, xml, nsArray, XmlArrayType.TEXT_LINEAR);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}
* *
*
* @see #xpathExists(Expression, Expression, Expression)
* @see xpath_exists ( xpath text, xml xml [, nsarray text[] ] ) → boolean
*
*/
public static SimplePredicate xpathExists(Expression xpath, Expression xml) {
return FunctionUtils.twoArgPredicateFunc("XPATH_EXISTS", xpath, xml);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}
* *
*
* @see #xpathExists(Expression, Expression)
* @see xpath_exists ( xpath text, xml xml [, nsarray text[] ] ) → boolean
*
*/
public static SimplePredicate xpathExists(Expression xpath, Expression xml, Expression nsArray) {
return FunctionUtils.threeArgPredicateFunc("XPATH_EXISTS", xpath, xml, nsArray);
}
/**
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextType#INSTANCE}.
* @param namespaceUri non-null,it will be passed to funcRef as the second argument of funcRef
* @param as see {@link SQLs#AS}
* @param namespaceName a simple identifier
* @throws CriteriaException throw when :
* - {@link Expression} returned by funcRef isn't operable {@link Expression},for example {@link SQLs#DEFAULT}
* - namespaceName isn't simple identifier
*
* @see #xmlNamespaces(Expression, SQLs.WordAs, String)
* @see XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] )
*
*/
public static XmlNameSpaces xmlNamespaces(BiFunction funcRef, String namespaceUri, SQLs.WordAs as, String namespaceName) {
return xmlNamespaces(funcRef.apply(TextType.INSTANCE, namespaceUri), as, namespaceName);
}
/**
* @param namespaceUri a text expression
* @param as see {@link SQLs#AS}
* @param namespaceName a simple identifier
* @see #xmlNamespaces(BiFunction, String, SQLs.WordAs, String)
* @see #xmlNamespaces(Consumer)
* @see XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] )
*
*/
public static XmlNameSpaces xmlNamespaces(Expression namespaceUri, SQLs.WordAs as, String namespaceName) {
final PostgreFunctionUtils.XmlNamedElementPart clause;
clause = PostgreFunctionUtils.xmlNamespaces();
clause.accept(namespaceUri, as, namespaceName);
return clause.endNamedPart();
}
/**
* @see #xmlNamespaces(BiFunction, String, SQLs.WordAs, String)
* @see #xmlNamespaces(Consumer)
* @see XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] )
*
*/
public static XmlNameSpaces xmlNamespaces(Consumer consumer) {
final PostgreFunctionUtils.XmlNamedElementPart clause;
clause = PostgreFunctionUtils.xmlNamespaces();
consumer.accept(clause);
return clause.endNamedPart();
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(Expression rowExp, WordPassing passing, Expression docExp,
Consumer consumer) {
return _xmlTable(null, rowExp, passing, null, docExp, null, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(XmlNameSpaces nameSpaces, Expression rowExp, WordPassing passing,
Expression docExp, Consumer consumer) {
ContextStack.assertNonNull(nameSpaces);
return _xmlTable(nameSpaces, rowExp, passing, null, docExp, null, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(Expression rowExp, WordPassing passing, PassingOption rowOption,
Expression docExp, Consumer consumer) {
ContextStack.assertNonNull(rowOption);
return _xmlTable(null, rowExp, passing, rowOption, docExp, null, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(Expression rowExp, WordPassing passing, Expression docExp,
PassingOption docOption,
Consumer consumer) {
ContextStack.assertNonNull(docOption);
return _xmlTable(null, rowExp, passing, null, docExp, docOption, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(XmlNameSpaces nameSpaces, Expression rowExp, WordPassing passing,
PassingOption rowOption, Expression docExp,
Consumer consumer) {
ContextStack.assertNonNull(nameSpaces);
ContextStack.assertNonNull(rowOption);
return _xmlTable(nameSpaces, rowExp, passing, rowOption, docExp, null, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(XmlNameSpaces nameSpaces, Expression rowExp, WordPassing passing,
Expression docExp, PassingOption docOption,
Consumer consumer) {
ContextStack.assertNonNull(nameSpaces);
ContextStack.assertNonNull(docOption);
return _xmlTable(nameSpaces, rowExp, passing, null, docExp, docOption, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(Expression rowExp, WordPassing passing, PassingOption rowOption,
Expression docExp, PassingOption docOption,
Consumer consumer) {
ContextStack.assertNonNull(rowOption);
ContextStack.assertNonNull(docOption);
return _xmlTable(null, rowExp, passing, rowOption, docExp, docOption, consumer);
}
/**
*
* XMLTABLE (
* [ XMLNAMESPACES ( namespace_uri AS namespace_name [, ...] ), ]
* row_expression PASSING [BY {REF|VALUE}] document_expression [BY {REF|VALUE}]
* COLUMNS name { type [PATH column_expression] [DEFAULT default_expression] [NOT NULL | NULL]
* | FOR ORDINALITY }
* [, ...]
* ) → setof record
*
*
* @see #xmlTable(Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(Expression, WordPassing, Expression, PassingOption, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, Consumer)
* @see #xmlTable(XmlNameSpaces, Expression, WordPassing, PassingOption, Expression, PassingOption, Consumer)
* @see XMLTABLE
*/
public static _TabularFunction xmlTable(XmlNameSpaces nameSpaces, Expression rowExp, WordPassing passing,
PassingOption rowOption, Expression docExp, PassingOption docOption,
Consumer consumer) {
ContextStack.assertNonNull(nameSpaces);
ContextStack.assertNonNull(rowOption);
ContextStack.assertNonNull(docOption);
return _xmlTable(nameSpaces, rowExp, passing, rowOption, docExp, docOption, consumer);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param table will output strings identifying tables using the usual notation, including optional schema qualification and double quotes
* @see #tableToXml(Expression, Expression, Expression, Expression)
* @see table_to_xml
*/
public static SimpleExpression tableToXml(TableMeta> table, Expression nulls, Expression tableForest,
Expression targetNs) {
return tableToXml(PostgreFunctionUtils.tableNameExp(table), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see table_to_xml
*/
public static SimpleExpression tableToXml(Expression table, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("TABLE_TO_XML", table, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param query will output literal sql
* @see query_to_xml
*/
public static SimpleExpression queryToXml(Select query, Visible visible, Expression nulls, Expression tableForest,
Expression targetNs) {
return queryToXml(PostgreFunctionUtils.queryStringExp(query, visible), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param query will output literal sql
* @see query_to_xml
*/
public static SimpleExpression queryToXml(Select query, Expression nulls, Expression tableForest,
Expression targetNs) {
return queryToXml(PostgreFunctionUtils.queryStringExp(query, Visible.ONLY_VISIBLE), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see query_to_xml
*/
public static SimpleExpression queryToXml(Expression query, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("QUERY_TO_XML", query, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see cursor_to_xml
*/
public static SimpleExpression cursorToXml(Expression cursor, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("CURSOR_TO_XML", cursor, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param table will output strings identifying tables using the usual notation, including optional schema qualification and double quotes
* @see #tableToXmlSchema(Expression, Expression, Expression, Expression)
* @see table_to_xmlschema
*/
public static SimpleExpression tableToXmlSchema(TableMeta> table, Expression nulls, Expression tableForest,
Expression targetNs) {
return tableToXmlSchema(PostgreFunctionUtils.tableNameExp(table), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see table_to_xmlschema
*/
public static SimpleExpression tableToXmlSchema(Expression table, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("TABLE_TO_XMLSCHEMA", table, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param query will output literal sql
* @see query_to_xmlschema
*/
public static SimpleExpression queryToXmlSchema(Select query, Visible visible, Expression nulls, Expression tableForest,
Expression targetNs) {
return queryToXmlSchema(PostgreFunctionUtils.queryStringExp(query, visible), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param query will output literal sql
* @see query_to_xmlschema
*/
public static SimpleExpression queryToXmlSchema(Select query, Expression nulls, Expression tableForest,
Expression targetNs) {
return queryToXmlSchema(PostgreFunctionUtils.queryStringExp(query, Visible.ONLY_VISIBLE), nulls, tableForest,
targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see query_to_xmlschema
*/
public static SimpleExpression queryToXmlSchema(Expression query, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("QUERY_TO_XMLSCHEMA", query, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see cursor_to_xmlschema
*/
public static SimpleExpression cursorToXmlSchema(Expression cursor, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("CURSOR_TO_XMLSCHEMA", cursor, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param table will output strings identifying tables using the usual notation, including optional schema qualification and double quotes
* @see #tableToXmlAndXmlSchema(Expression, Expression, Expression, Expression)
* @see table_to_xml_and_xmlschema
*/
public static SimpleExpression tableToXmlAndXmlSchema(TableMeta> table, Expression nulls, Expression tableForest,
Expression targetNs) {
return tableToXmlAndXmlSchema(PostgreFunctionUtils.tableNameExp(table), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see table_to_xml_and_xmlschema
*/
public static SimpleExpression tableToXmlAndXmlSchema(Expression table, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("TABLE_TO_XML_AND_XMLSCHEMA", table, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param query will output literal sql
* @see query_to_xml_and_xmlschema
*/
public static SimpleExpression queryToXmlAndXmlSchema(Select query, Visible visible, Expression nulls, Expression tableForest,
Expression targetNs) {
return queryToXmlAndXmlSchema(PostgreFunctionUtils.queryStringExp(query, visible), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @param query will output literal sql
* @see query_to_xml_and_xmlschema
*/
public static SimpleExpression queryToXmlAndXmlSchema(Select query, Expression nulls, Expression tableForest,
Expression targetNs) {
return queryToXmlAndXmlSchema(PostgreFunctionUtils.queryStringExp(query, Visible.ONLY_VISIBLE), nulls, tableForest, targetNs);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see query_to_xml_and_xmlschema
*/
public static SimpleExpression queryToXmlAndXmlSchema(Expression query, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("QUERY_TO_XML_AND_XMLSCHEMA", query, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see schema_to_xml
*/
public static SimpleExpression schemaToXml(Expression schema, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("SCHEMA_TO_XML", schema, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see schema_to_xmlschema
*/
public static SimpleExpression schemaToXmlSchema(Expression schema, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("SCHEMA_TO_XMLSCHEMA", schema, nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see schema_to_xml_and_xmlschema
*/
public static SimpleExpression schemaToXmlAndXmlSchema(Expression schema, Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.fourArgFunc("SCHEMA_TO_XML_AND_XMLSCHEMA", schema, nulls, tableForest, targetNs,
XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see database_to_xml
*/
public static SimpleExpression databaseToXml(Expression nulls, Expression tableForest, Expression targetNs) {
return FunctionUtils.threeArgFunc("DATABASE_TO_XML", nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see database_to_xmlschema
*/
public static SimpleExpression databaseToXmlSchema(Expression nulls, Expression tableForest, Expression targetNs) {
return FunctionUtils.threeArgFunc("DATABASE_TO_XMLSCHEMA", nulls, tableForest, targetNs, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlArrayType#TEXT_LINEAR}
* *
*
* @see database_to_xml_and_xmlschema
*/
public static SimpleExpression databaseToXmlAndXmlSchema(Expression nulls, Expression tableForest,
Expression targetNs) {
return FunctionUtils.threeArgFunc("DATABASE_TO_XML_AND_XMLSCHEMA", nulls, tableForest, targetNs,
XmlType.TEXT);
}
/*-------------------below JSON function -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param arg valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see to_json ( anyelement ) → json
*/
public static SimpleExpression toJson(Object arg) {
return FunctionUtils.oneArgRowElementFunc("to_json", arg, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
*
* @param arg valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see to_jsonb ( anyelement ) → jsonb
*/
public static SimpleExpression toJsonb(Object arg) {
return FunctionUtils.oneArgRowElementFunc("to_jsonb", arg, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @see #arrayToJson(Expression, Expression)
* @see array_to_json ( anyarray [, boolean ] ) → json
* Converts an SQL array to a JSON array. The behavior is the same as to_json except that line feeds will be added between top-level array elements if the optional boolean parameter is true.
*
*/
public static SimpleExpression arrayToJson(Expression array) {
return FunctionUtils.oneArgFunc("array_to_json", array, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @param lineFeed in most case ,{@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see array_to_json ( anyarray [, boolean ] ) → json
* Converts an SQL array to a JSON array. The behavior is the same as to_json except that line feeds will be added between top-level array elements if the optional boolean parameter is true.
*
*/
public static SimpleExpression arrayToJson(Expression array, Expression lineFeed) {
return FunctionUtils.twoArgFunc("array_to_json", array, lineFeed, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
*
* @param record valid type:
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see row_to_json ( record [, boolean ] ) → json
* Converts an SQL composite value to a JSON object. The behavior is the same as to_json except that line feeds will be added between top-level elements if the optional boolean parameter is true.
*
*/
public static SimpleExpression rowToJson(RowElement record) {
return FunctionUtils.oneArgRowElementFunc("row_to_json", record, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
*
* @param record valid type:
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param lineFeed in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see row_to_json ( record [, boolean ] ) → json
* Converts an SQL composite value to a JSON object. The behavior is the same as to_json except that line feeds will be added between top-level elements if the optional boolean parameter is true.
*
*/
public static SimpleExpression rowToJson(RowElement record, Expression lineFeed) {
return FunctionUtils.twoArgRowElementFunc("row_to_json", record, lineFeed, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* create a empty json array
* *
*
* @see json_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildArray() {
return FunctionUtils.zeroArgFunc("json_build_array", JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param arg valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see json_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildArray(Object arg) {
return FunctionUtils.oneArgRowElementFunc("json_build_array", arg, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param arg1 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param arg2 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see json_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildArray(Object arg1, Object arg2) {
return FunctionUtils.twoArgRowElementFunc("json_build_array", arg1, arg2, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param arg1 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param arg2 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param arg3 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param variadic valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see json_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildArray(Object arg1, Object arg2, Object arg3, Object... variadic) {
return FunctionUtils.threeAndRestRowElementFunc("json_build_array", JsonType.TEXT, arg1, arg2, arg3, variadic);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param consumer valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)
* @see SQLs#space(String, SQLs.SymbolPeriod, TableMeta)
* @see #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)
* @see #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)
* @see json_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildArray(Consumer> consumer) {
return FunctionUtils.rowElementFunc("json_build_array", false, consumer, JsonType.TEXT);
}
/*-------------------below jsonb_build_array-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
* create a empty jsonb array
* *
*
* @see jsonb_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildArray() {
return FunctionUtils.zeroArgFunc("jsonb_build_array", JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param arg valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see jsonb_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildArray(Object arg) {
return FunctionUtils.oneArgRowElementFunc("jsonb_build_array", arg, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
*
* @param arg1 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param arg2 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see jsonb_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildArray(Object arg1, Object arg2) {
return FunctionUtils.twoArgRowElementFunc("jsonb_build_array", arg1, arg2, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
*
* @param arg1 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param arg2 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param arg3 valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @param variadic valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see jsonb_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildArray(Object arg1, Object arg2, Object arg3, Object... variadic) {
return FunctionUtils.threeAndRestRowElementFunc("jsonb_build_array", JsonbType.TEXT, arg1, arg2, arg3, variadic);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
* This method don't support {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)},because it will output non-mapping column .
* you should use
*
* - {@link #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
* - {@link #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)}
*
* *
*
* @param consumer valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
*
* @throws CriteriaException throw when arg type error , but probably defer if arg is {@link SQLs#refThis(String, String)}
* @see SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)
* @see SQLs#space(String, SQLs.SymbolPeriod, TableMeta)
* @see #jsonBuildObject(String, SQLs.SymbolPeriod, TableMeta)
* @see #jsonbBuildObject(String, SQLs.SymbolPeriod, TableMeta)
* @see jsonb_build_array ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildArray(Consumer> consumer) {
return FunctionUtils.rowElementFunc("jsonb_build_array", false, consumer, JsonbType.TEXT);
}
public static SimpleExpression jsonBuildObject() {
return FunctionUtils.zeroArgFunc("json_build_object", JsonType.TEXT);
}
public static SimpleExpression jsonbBuildObject() {
return FunctionUtils.zeroArgFunc("jsonb_build_object", JsonbType.TEXT);
}
public static SimpleExpression jsonBuildObject(String tableAlias, SQLs.SymbolPeriod period, TableMeta> table) {
return FunctionUtils.oneArgObjectElementFunc("json_build_object", ContextStack.peek().row(tableAlias, period, table),
JsonType.TEXT);
}
public static SimpleExpression jsonBuildObject(String derivedAlias, SQLs.SymbolPeriod period, SQLs.SymbolAsterisk asterisk) {
return FunctionUtils.oneArgObjectElementFunc("json_build_object", ContextStack.peek().row(derivedAlias, period, asterisk),
JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @param consumer value valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)}
*
* @see json_build_object ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildObject(Consumer consumer) {
return FunctionUtils.objectElementFunc("json_build_object", false, consumer, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @param space see {@link SQLs#SPACE}
* @param consumer value valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)}
*
* @see json_build_object ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonBuildObject(SQLs.SymbolSpace space, Consumer consumer) {
return FunctionUtils.objectElementFunc(space, "json_build_object", false, consumer, JsonType.TEXT);
}
public static SimpleExpression jsonbBuildObject(String tableAlias, SQLs.SymbolPeriod period, TableMeta> table) {
return FunctionUtils.oneArgObjectElementFunc("jsonb_build_object", ContextStack.peek().row(tableAlias, period, table),
JsonbType.TEXT);
}
public static SimpleExpression jsonbBuildObject(String derivedAlias, SQLs.SymbolPeriod period, SQLs.SymbolAsterisk asterisk) {
return FunctionUtils.oneArgObjectElementFunc("jsonb_build_object", ContextStack.peek().row(derivedAlias, period, asterisk),
JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* @param consumer value valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)}
*
* @see jsonb_build_object ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildObject(Consumer consumer) {
return FunctionUtils.objectElementFunc("jsonb_build_object", false, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* @param space see {@link SQLs#SPACE}
* @param consumer value valid type:
* - constant
* - {@link RowExpression}
* - {@link Expression}
* - {@link SQLs#row(Object)}
* - {@link SQLs#row(Consumer)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, SQLs.SymbolAsterisk)}
* - {@link SQLs#space(String, SQLs.SymbolPeriod, TableMeta)}
*
* @see jsonb_build_object ( VARIADIC "any" ) → json
* Builds a possibly-heterogeneously-typed JSON array out of a variadic argument list. Each argument is converted as per to_json or to_jsonb.
*
*/
public static SimpleExpression jsonbBuildObject(SQLs.SymbolSpace space, Consumer consumer) {
return FunctionUtils.objectElementFunc(space, "jsonb_build_object", false, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @see json_object ( text[] ) → json
* Builds a JSON object out of a text array. The array must have either exactly one dimension with an even number of members, in which case they are taken
* as alternating key/value pairs, or two dimensions such that each inner array has exactly two elements, which are taken as a key/value pair. All values are
* converted to JSON strings.
*
*/
public static SimpleExpression jsonObject(Expression pairArray) {
return FunctionUtils.oneArgFunc("json_object", pairArray, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextArrayType#LINEAR}.
* @see #jsonObject(Expression)
* @see json_object ( text[] ) → json
* Builds a JSON object out of a text array. The array must have either exactly one dimension with an even number of members, in which case they are taken
* as alternating key/value pairs, or two dimensions such that each inner array has exactly two elements, which are taken as a key/value pair. All values are
* converted to JSON strings.
*
*/
public static SimpleExpression jsonObject(BiFunction funcRef,
Consumer consumer) {
return FunctionUtils.staticStringObjectStringFunc("json_object", false, funcRef, TextArrayType.LINEAR, consumer,
JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextArrayType#LINEAR}.
* @see #jsonObject(Expression)
* @see json_object ( text[] ) → json
* Builds a JSON object out of a text array. The array must have either exactly one dimension with an even number of members, in which case they are taken
* as alternating key/value pairs, or two dimensions such that each inner array has exactly two elements, which are taken as a key/value pair. All values are
* converted to JSON strings.
*
*/
public static SimpleExpression jsonObject(SQLs.SymbolSpace space, final BiFunction funcRef,
final Consumer consumer) {
return FunctionUtils.dynamicStringObjectStringFunc("json_object", space, false, funcRef, TextArrayType.LINEAR, consumer,
JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* @see jsonb_object ( text[] ) → json
* Builds a JSON object out of a text array. The array must have either exactly one dimension with an even number of members, in which case they are taken
* as alternating key/value pairs, or two dimensions such that each inner array has exactly two elements, which are taken as a key/value pair. All values are
* converted to JSON strings.
*
*/
public static SimpleExpression jsonbObject(Expression pairArray) {
return FunctionUtils.oneArgFunc("jsonb_object", pairArray, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextArrayType#LINEAR}.
* @see #jsonbObject(Expression)
* @see jsonb_object ( text[] ) → json
* Builds a JSON object out of a text array. The array must have either exactly one dimension with an even number of members, in which case they are taken
* as alternating key/value pairs, or two dimensions such that each inner array has exactly two elements, which are taken as a key/value pair. All values are
* converted to JSON strings.
*
*/
public static SimpleExpression jsonbObject(BiFunction funcRef,
Consumer consumer) {
return FunctionUtils.staticStringObjectStringFunc("jsonb_object", false, funcRef, TextArrayType.LINEAR, consumer,
JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link TextArrayType#LINEAR}.
* @see #jsonbObject(Expression)
* @see jsonb_object ( text[] ) → json
* Builds a JSON object out of a text array. The array must have either exactly one dimension with an even number of members, in which case they are taken
* as alternating key/value pairs, or two dimensions such that each inner array has exactly two elements, which are taken as a key/value pair. All values are
* converted to JSON strings.
*
*/
public static SimpleExpression jsonbObject(SQLs.SymbolSpace space, final BiFunction funcRef,
final Consumer consumer) {
return FunctionUtils.dynamicStringObjectStringFunc("jsonb_object", space, false, funcRef, TextArrayType.LINEAR, consumer,
JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}
* *
*
* @see json_object ( keys text[], values text[] ) → json
* This form of json_object takes keys and values pairwise from separate text arrays. Otherwise it is identical to the one-argument form.
* json_object('{a,b}', '{1,2}') → {"a": "1", "b": "2"}
*
*/
public static SimpleExpression jsonObject(Expression keyArray, Expression valueArray) {
return FunctionUtils.twoArgFunc("json_object", keyArray, valueArray, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}
* *
*
* @see jsonb_object ( keys text[], values text[] ) → json
* This form of json_object takes keys and values pairwise from separate text arrays. Otherwise it is identical to the one-argument form.
* json_object('{a,b}', '{1,2}') → {"a": "1", "b": "2"}
*
*/
public static SimpleExpression jsonbObject(Expression keyArray, Expression valueArray) {
return FunctionUtils.twoArgFunc("jsonb_object", keyArray, valueArray, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* Default Selection alias is 'value'.
* *
* select * from json_array_elements('[1,true, [2,false]]') →
*
* value
* -----------
* 1
* true
* [2,false]
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonArrayElements(Expression)
* @see json_array_elements ( json ) → setof json
* Expands the top-level JSON array into a set of JSON values.
*
*/
public static _ColumnWithOrdinalityFunction jsonArrayElements(final BiFunction funcRef, T value) {
return jsonArrayElements(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* Default Selection alias is 'value'.
* *
* select * from json_array_elements('[1,true, [2,false]]') →
*
* value
* -----------
* 1
* true
* [2,false]
*
*
* @see json_array_elements ( json ) → setof json
* Expands the top-level JSON array into a set of JSON values.
*
*/
public static _ColumnWithOrdinalityFunction jsonArrayElements(final Expression json) {
return DialectFunctionUtils.oneArgColumnFunction("JSON_ARRAY_ELEMENTS", json, "value", JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* Default Selection alias is 'value'.
* *
* select * from jsonb_array_elements('[1,true, [2,false]]') →
*
* value
* -----------
* 1
* true
* [2,false]
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonbArrayElements(Expression)
* @see jsonb_array_elements ( json ) → setof json
* Expands the top-level JSON array into a set of JSON values.
*
*/
public static _ColumnWithOrdinalityFunction jsonbArrayElements(final BiFunction funcRef, T value) {
return jsonbArrayElements(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* Default Selection alias is 'value'.
* *
* select * from jsonb_array_elements('[1,true, [2,false]]') →
*
* value
* -----------
* 1
* true
* [2,false]
*
*
* @see jsonb_array_elements ( json ) → setof json
* Expands the top-level JSON array into a set of JSON values.
*
*/
public static _ColumnWithOrdinalityFunction jsonbArrayElements(final Expression json) {
return DialectFunctionUtils.oneArgColumnFunction("JSONB_ARRAY_ELEMENTS", json, "value", JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* Default Selection alias is 'value'.
* *
* select * from json_array_elements_text('["foo", "bar"]') →
*
* value
* -----------
* foo
* bar
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonArrayElementsText(Expression)
* @see json_array_elements_text ( json ) → setof text
* Expands the top-level JSON array into a set of JSON values.
*
*/
public static _ColumnWithOrdinalityFunction jsonArrayElementsText(final BiFunction funcRef, T value) {
return jsonArrayElementsText(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* Default Selection alias is 'value'.
* *
* select * from json_array_elements_text('["foo", "bar"]') →
*
* value
* -----------
* foo
* bar
*
*
* @see json_array_elements_text ( json ) → setof text
* Expands the top-level JSON array into a set of text values.
*
*/
public static _ColumnWithOrdinalityFunction jsonArrayElementsText(final Expression json) {
return DialectFunctionUtils.oneArgColumnFunction("JSON_ARRAY_ELEMENTS_TEXT", json, "value", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* Default Selection alias is 'value'.
* *
* select * from jsonb_array_elements_text('["foo", "bar"]') →
*
* value
* -----------
* foo
* bar
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonbArrayElementsText(Expression)
* @see jsonb_array_elements_text ( json ) → setof text
* Expands the top-level JSON array into a set of JSON values.
*
*/
public static _ColumnWithOrdinalityFunction jsonbArrayElementsText(final BiFunction funcRef, T value) {
return jsonbArrayElementsText(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* Default Selection alias is 'value'.
* *
* select * from jsonb_array_elements_text('["foo", "bar"]') →
*
* value
* -----------
* foo
* bar
*
*
* @see jsonb_array_elements_text ( json ) → setof text
* Expands the top-level JSON array into a set of text values.
*
*/
public static _ColumnWithOrdinalityFunction jsonbArrayElementsText(final Expression json) {
return DialectFunctionUtils.oneArgColumnFunction("JSONB_ARRAY_ELEMENTS_TEXT", json, "value", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}.
* *
*
* @see json_array_length ( json ) → integer
* Returns the number of elements in the top-level JSON array.
*
*/
public static SimpleExpression jsonArrayLength(Expression json) {
return FunctionUtils.oneArgFunc("JSON_ARRAY_LENGTH", json, IntegerType.INSTANCE);
}
/**
*
The {@link MappingType} of function return type: {@link IntegerType}.
*
* @see jsonb_array_length ( json ) → integer
* Returns the number of elements in the top-level JSON array.
*
*/
public static SimpleExpression jsonbArrayLength(Expression jsonb) {
return FunctionUtils.oneArgFunc("JSONB_ARRAY_LENGTH", jsonb, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link JsonType#TEXT}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select * from json_each('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonEach(Expression)
* @see json_each ( json ) → setof record ( key text, value json )
* Expands the top-level JSON object into a set of key/value pairs.
*
*/
public static _TabularWithOrdinalityFunction jsonEach(BiFunction funcRef, T value) {
return jsonEach(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link JsonType#TEXT}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select * from json_each('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @see #jsonEach(Expression)
* @see json_each ( json ) → setof record ( key text, value json )
* Expands the top-level JSON object into a set of key/value pairs.
*
*/
public static _TabularWithOrdinalityFunction jsonEach(final Expression json) {
final List fieldList = _Collections.arrayList(2);
fieldList.add(ArmySelections.forName("key", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("value", JsonType.TEXT));
return DialectFunctionUtils.oneArgTabularFunc("JSON_EACH", json, fieldList);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link JsonbType#TEXT}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
*
* select * from jsonb_each('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonbEach(Expression)
* @see jsonb_each ( json ) → setof record ( key text, value json )
* Expands the top-level JSON object into a set of key/value pairs.
*
*/
public static _TabularWithOrdinalityFunction jsonbEach(BiFunction funcRef, T value) {
return jsonbEach(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link JsonbType#TEXT}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
*
* select * from jsonb_each('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @see #jsonEach(Expression)
* @see jsonb_each ( json ) → setof record ( key text, value json )
* Expands the top-level JSON object into a set of key/value pairs.
*
*/
public static _TabularWithOrdinalityFunction jsonbEach(final Expression json) {
final List fieldList = _Collections.arrayList(2);
fieldList.add(ArmySelections.forName("key", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("value", JsonbType.TEXT));
return DialectFunctionUtils.oneArgTabularFunc("JSONB_EACH", json, fieldList);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select * from json_each_text('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonEachText(Expression)
* @see json_each_text ( json ) → setof record ( key text, value text )
* Expands the top-level JSON object into a set of key/value pairs. The returned values will be of type text.
*
*/
public static _TabularWithOrdinalityFunction jsonEachText(BiFunction funcRef, T value) {
return jsonEachText(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select * from json_each_text('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @see #jsonEachText(BiFunction, Object)
* @see json_each_text ( json ) → setof record ( key text, value text )
* Expands the top-level JSON object into a set of key/value pairs. The returned values will be of type text.
*
*/
public static _TabularWithOrdinalityFunction jsonEachText(final Expression json) {
final List fieldList = _Collections.arrayList(2);
fieldList.add(ArmySelections.forName("key", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("value", TextType.INSTANCE));
return DialectFunctionUtils.oneArgTabularFunc("JSON_EACH_TEXT", json, fieldList);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link TextType}
* - value : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select * from jsonb_each_text('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonbEachText(Expression)
* @see jsonb_each_text ( json ) → setof record ( key text, value text )
* Expands the top-level JSON object into a set of key/value pairs. The returned values will be of type text.
*
*/
public static _TabularWithOrdinalityFunction jsonbEachText(BiFunction funcRef, T value) {
return jsonbEachText(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* The {@link MappingType} of fields of derived table :
* - key : {@link StringType}
* - value : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select * from jsonb_each_text('{"a":"foo", "b":"bar"}') →
*
* key | value
* -----+-------
* a | "foo"
* b | "bar"
*
*
* @see #jsonbEachText(BiFunction, Object)
* @see jsonb_each_text ( json ) → setof record ( key text, value text )
* Expands the top-level JSON object into a set of key/value pairs. The returned values will be of type text.
*
*/
public static _TabularWithOrdinalityFunction jsonbEachText(final Expression json) {
final List fieldList = _Collections.arrayList(2);
fieldList.add(ArmySelections.forName("key", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("value", TextType.INSTANCE));
return DialectFunctionUtils.oneArgTabularFunc("JSONB_EACH_TEXT", json, fieldList);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* *
*
* @param firstPath should be {@link TextType} or {@link StringType} type.
* @param rest each element should be {@link TextType} or {@link StringType} type.
* @see json_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonExtractPath(Expression fromJson, Expression firstPath, Expression... rest) {
final String name = "JSON_EXTRACT_PATH";
return FunctionUtils.oneAndAtLeastFunc(name, JsonType.TEXT, fromJson, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* *
*
* @param consumer should be {@link TextType} or {@link StringType} type.
* @see json_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonExtractPath(Expression fromJson, Consumer> consumer) {
final String name = "JSON_EXTRACT_PATH";
return FunctionUtils.oneAndConsumer(name, true, fromJson, consumer, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* *
*
* @param funcRefForJson the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForJson always is {@link JsonType#TEXT}.
* @param json non-null,it will be passed to funcRefForJson as the second argument of funcRefForJson
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link NoCastTextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see json_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonExtractPath(BiFunction funcRefForJson, T json,
BiFunction, Expression> funcRefForPath,
String firstPath, String... rest) {
return jsonExtractPath(funcRefForJson.apply(JsonType.TEXT, json), funcRefForPath, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link NoCastTextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see json_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonExtractPath(Expression fromJson, BiFunction, Expression> funcRef,
String firstPath, String... rest) {
final String name = "JSON_EXTRACT_PATH";
final List pathElemList;
pathElemList = ArrayUtils.unmodifiableListOf(firstPath, rest);
return FunctionUtils.oneAndMulti(name, fromJson, funcRef.apply(NoCastTextType.INSTANCE, pathElemList), JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link NoCastTextType#INSTANCE}.
* @see json_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonExtractPath(Expression fromJson, BiFunction, Expression> funcRef,
Consumer> consumer) {
return FunctionUtils.oneAndMulti("JSON_EXTRACT_PATH", fromJson,
funcRef.apply(NoCastTextType.INSTANCE, CriteriaUtils.stringList(null, true, consumer)),
JsonType.TEXT
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param firstPath should be {@link TextType} or {@link StringType} type.
* @param rest each element should be {@link TextType} or {@link StringType} type.
* @see jsonb_extract_path ( from_json jsonb, VARIADIC path_elems text[] ) → jsonb
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonbExtractPath(Expression fromJson, Expression firstPath, Expression... rest) {
final String name = "JSONB_EXTRACT_PATH";
return FunctionUtils.oneAndAtLeastFunc(name, JsonbType.TEXT, fromJson, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param consumer should be {@link TextType} or {@link StringType} type.
* @see jsonb_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonbExtractPath(Expression fromJson, Consumer> consumer) {
final String name = "JSONB_EXTRACT_PATH";
return FunctionUtils.oneAndConsumer(name, true, fromJson, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForJson the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForJson always is {@link JsonbType#TEXT}.
* @param json non-null,it will be passed to funcRefForJson as the second argument of funcRefForJson
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link NoCastTextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see jsonb_extract_path ( from_json jsonb, VARIADIC path_elems text[] ) → jsonb
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonbExtractPath(BiFunction funcRefForJson, T json,
BiFunction, Expression> funcRefForPath,
String firstPath, String... rest) {
return jsonbExtractPath(funcRefForJson.apply(JsonbType.TEXT, json), funcRefForPath, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link NoCastTextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see jsonb_extract_path ( from_json jsonb, VARIADIC path_elems text[] ) → jsonb
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonbExtractPath(Expression fromJson, BiFunction, Expression> funcRef,
String firstPath, String... rest) {
final String name = "JSONB_EXTRACT_PATH";
final List pathElemList;
pathElemList = ArrayUtils.unmodifiableListOf(firstPath, rest);
return FunctionUtils.oneAndMulti(name, fromJson, funcRef.apply(NoCastTextType.INSTANCE, pathElemList), JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link NoCastTextType#INSTANCE}.
* @see jsonb_extract_path ( from_json json, VARIADIC path_elems text[] ) → json
* Extracts JSON sub-object at the specified path. (This is functionally equivalent to the #> operator, but writing the path out as a variadic list can be more convenient in some cases.)
*
*/
public static SimpleExpression jsonbExtractPath(Expression fromJson, BiFunction, Expression> funcRef,
Consumer> consumer) {
return FunctionUtils.oneAndMulti("JSONB_EXTRACT_PATH", fromJson,
funcRef.apply(NoCastTextType.INSTANCE, CriteriaUtils.stringList(null, true, consumer)),
JsonbType.TEXT
);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param firstPath should be {@link TextType} or {@link StringType} type.
* @param rest each element should be {@link TextType} or {@link StringType} type.
* @see json_extract_path_text ( from_json json, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonExtractPathText(Expression fromJson, Expression firstPath, Expression... rest) {
final String name = "JSON_EXTRACT_PATH_TEXT";
return FunctionUtils.oneAndAtLeastFunc(name, TextType.INSTANCE, fromJson, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param funcRefForJson the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForJson always is {@link JsonType#TEXT}.
* @param json non-null,it will be passed to funcRefForJson as the second argument of funcRefForJson
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see json_extract_path_text ( from_json json, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonExtractPathText(BiFunction funcRefForJson, T json,
BiFunction, Expression> funcRefForPath,
String firstPath, String... rest) {
return jsonExtractPathText(funcRefForJson.apply(JsonType.TEXT, json), funcRefForPath, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link NoCastTextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see json_extract_path_text ( from_json json, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonExtractPathText(Expression fromJson, BiFunction, Expression> funcRef,
String firstPath, String... rest) {
final String name = "JSON_EXTRACT_PATH_TEXT";
final List pathElemList;
pathElemList = ArrayUtils.unmodifiableListOf(firstPath, rest);
return FunctionUtils.oneAndMulti(name, fromJson, funcRef.apply(NoCastTextType.INSTANCE, pathElemList), TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param consumer should be {@link TextType} or {@link StringType} type.
* @see json_extract_path_text ( from_json json, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonExtractPathText(Expression fromJson, Consumer> consumer) {
return FunctionUtils.oneAndConsumer("JSON_EXTRACT_PATH_TEXT", true, fromJson, consumer, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link NoCastTextType#INSTANCE}.
* @see json_extract_path_text ( from_json json, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonExtractPathText(Expression fromJson, BiFunction, Expression> funcRef,
Consumer> consumer) {
return FunctionUtils.oneAndMulti("JSON_EXTRACT_PATH_TEXT", fromJson,
funcRef.apply(NoCastTextType.INSTANCE, CriteriaUtils.stringList(null, true, consumer)),
TextType.INSTANCE
);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param firstPath should be {@link TextType} or {@link StringType} type.
* @param rest each element should be {@link TextType} or {@link StringType} type.
* @see jsonb_extract_path_text ( from_json jsonb, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonbExtractPathText(Expression fromJson, Expression firstPath, Expression... rest) {
final String name = "JSONB_EXTRACT_PATH_TEXT";
return FunctionUtils.oneAndAtLeastFunc(name, TextType.INSTANCE, fromJson, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param funcRefForJson the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForJson always is {@link JsonbType#TEXT}.
* @param json non-null,it will be passed to funcRefForJson as the second argument of funcRefForJson
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see jsonb_extract_path_text ( from_json jsonb, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonbExtractPathText(BiFunction funcRefForJson, T json,
BiFunction, Expression> funcRefForPath,
String firstPath, String... rest) {
return jsonbExtractPathText(funcRefForJson.apply(JsonbType.TEXT, json), funcRefForPath, firstPath, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRef always is {@link NoCastTextType#INSTANCE}.
* @param firstPath firstPath and rest will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @param rest rest and firstPath will be collected to unmodified {@link List} and passed to funcRef as the second argument of funcRef
* @see jsonb_extract_path_text ( from_json jsonb, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonbExtractPathText(Expression fromJson, BiFunction, Expression> funcRef,
String firstPath, String... rest) {
final String name = "JSONB_EXTRACT_PATH_TEXT";
final List pathElemList;
pathElemList = ArrayUtils.unmodifiableListOf(firstPath, rest);
return FunctionUtils.oneAndMulti(name, fromJson, funcRef.apply(NoCastTextType.INSTANCE, pathElemList), TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
* *
*
* @param consumer should be {@link TextType} or {@link StringType} type.
* @see jsonb_extract_path_text ( from_json jsonb, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonbExtractPathText(Expression fromJson, Consumer> consumer) {
return FunctionUtils.oneAndConsumer("JSONB_EXTRACT_PATH_TEXT", true, fromJson, consumer, TextType.INSTANCE);
}
/**
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#rowParam(TypeInfer, Collection)}
* - {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link NoCastTextType#INSTANCE}.
* @see jsonb_extract_path_text ( from_json jsonb, VARIADIC path_elems text[] ) → text
* Extracts JSON sub-object at the specified path as text. (This is functionally equivalent to the #>> operator.)
*
*/
public static SimpleExpression jsonbExtractPathText(Expression fromJson, BiFunction, Expression> funcRef,
Consumer> consumer) {
return FunctionUtils.oneAndMulti("JSONB_EXTRACT_PATH_TEXT", fromJson,
funcRef.apply(NoCastTextType.INSTANCE, CriteriaUtils.stringList(null, true, consumer)),
TextType.INSTANCE
);
}
/*-------------------below json_object_keys-------------------*/
/**
*
* The {@link MappingType} of fields of derived table :
* - function alias(is specified by AS clause) : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select keys.keys from json_object_keys('{"f1":"abc","f2":{"f3":"a", "f4":"b"}}') as keys →
*
* keys
* ------------------
* f1
* f2
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonObjectKeys(Expression)
* @see json_object_keys ( json ) → setof text
* Returns the set of keys in the top-level JSON object.
*
*/
public static _ColumnWithOrdinalityFunction jsonObjectKeys(BiFunction funcRef, T value) {
return jsonObjectKeys(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* The {@link MappingType} of fields of derived table :
* - function alias(is specified by AS clause) : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select keys.keys from json_object_keys('{"f1":"abc","f2":{"f3":"a", "f4":"b"}}') as keys →
*
* keys
* ------------------
* f1
* f2
*
*
* @see json_object_keys ( json ) → setof text
* Returns the set of keys in the top-level JSON object.
*
*/
public static _ColumnWithOrdinalityFunction jsonObjectKeys(Expression json) {
return DialectFunctionUtils.oneArgColumnFunction("JSON_OBJECT_KEYS", json, null, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - function alias(is specified by AS clause) : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select keys.keys from json_object_keys('{"f1":"abc","f2":{"f3":"a", "f4":"b"}}') as keys →
*
* keys
* ------------------
* f1
* f2
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @see #jsonbObjectKeys(Expression)
* @see jsonb_object_keys ( json ) → setof text
* Returns the set of keys in the top-level JSON object.
*
*/
public static _ColumnWithOrdinalityFunction jsonbObjectKeys(BiFunction funcRef, T value) {
return jsonbObjectKeys(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* The {@link MappingType} of fields of derived table :
* - function alias(is specified by AS clause) : {@link TextType}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
* select keys.keys from json_object_keys('{"f1":"abc","f2":{"f3":"a", "f4":"b"}}') as keys →
*
* keys
* ------------------
* f1
* f2
*
*
* @see jsonb_object_keys ( json ) → setof text
* Returns the set of keys in the top-level JSON object.
*
*/
public static _ColumnWithOrdinalityFunction jsonbObjectKeys(Expression json) {
return DialectFunctionUtils.oneArgColumnFunction("JSONB_OBJECT_KEYS", json, null, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - fields follow {@link MappingType.SqlCompositeType#fieldList()}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
*
* @param base composite type expression.
* @see json_populate_record ( base anyelement, from_json json ) → anyelement
*
*
*/
public static _TabularWithOrdinalityFunction jsonPopulateRecord(final Expression base, final Expression json) {
return _jsonbPopulateRecordFunc("JSON_POPULATE_RECORD", base, json);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - fields follow {@link MappingType.SqlCompositeType#fieldList()}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
* *
*
* @param base composite type expression.
* @see jsonb_populate_record ( base anyelement, from_json json ) → anyelement
*
*
*/
public static _TabularWithOrdinalityFunction jsonbPopulateRecord(final Expression base, final Expression json) {
return _jsonbPopulateRecordFunc("JSONB_POPULATE_RECORD", base, json);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - fields follow {@link MappingType.SqlCompositeType#fieldList()}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
*
* @param base composite type expression.
* @see json_populate_recordset ( base anyelement, from_json json ) → anyelement
*
*
*/
public static _TabularWithOrdinalityFunction jsonPopulateRecordSet(final Expression base, final Expression json) {
return _jsonbPopulateRecordFunc("JSON_POPULATE_RECORDSET", base, json);
}
/**
*
* The {@link MappingType} of fields of derived table :
* - fields follow {@link MappingType.SqlCompositeType#fieldList()}
* - ordinality (optioinal) : {@link IntegerType}. see {@link _WithOrdinalityClause#withOrdinality()}
*
*
* @param base composite type expression.
* @see jsonb_populate_recordset ( base anyelement, from_json json ) → anyelement
*
*
*/
public static _TabularWithOrdinalityFunction jsonbPopulateRecordSet(final Expression base, final Expression json) {
return _jsonbPopulateRecordFunc("JSONB_POPULATE_RECORDSET", base, json);
}
/**
*
* Expands the top-level JSON object to a row having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) The output record is filled from fields of the JSON object, in the same way as described
* above for json[b]_populate_record. Since there is no input record value, unmatched columns are always filled
* with nulls.
*
* create type myrowtype as (a int, b text);
*
* select * from json_to_record('{"a":1,"b":[1,2,3],"c":[1,2,3],"e":"bar","r": {"a": 123, "b": "a b c"}}') as x(a int, b text, c int[], d text, r myrowtype) →
*
* a | b | c | d | r
* ---+---------+---------+---+---------------
* 1 | [1,2,3] | {1,2,3} | | (123,"a b c")
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* @see #jsonToRecord(Expression)
* @see json_to_record ( json ) → record
*
*
*/
public static UndoneFunction jsonToRecord(BiFunction funcRef, final T value) {
return jsonToRecord(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* Expands the top-level JSON object to a row having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) The output record is filled from fields of the JSON object, in the same way as described
* above for json[b]_populate_record. Since there is no input record value, unmatched columns are always filled
* with nulls.
*
* create type myrowtype as (a int, b text);
*
* select * from json_to_record('{"a":1,"b":[1,2,3],"c":[1,2,3],"e":"bar","r": {"a": 123, "b": "a b c"}}') as x(a int, b text, c int[], d text, r myrowtype) →
*
* a | b | c | d | r
* ---+---------+---------+---+---------------
* 1 | [1,2,3] | {1,2,3} | | (123,"a b c")
*
*
* @see json_to_record ( json ) → record
*
*
*/
public static UndoneFunction jsonToRecord(final Expression json) {
return DialectFunctionUtils.oneArgUndoneFunc("JSON_TO_RECORD", json);
}
/**
*
* Expands the top-level JSON object to a row having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) The output record is filled from fields of the JSON object, in the same way as described
* above for json[b]_populate_record. Since there is no input record value, unmatched columns are always filled
* with nulls.
*
* create type myrowtype as (a int, b text);
*
* select * from json_to_record('{"a":1,"b":[1,2,3],"c":[1,2,3],"e":"bar","r": {"a": 123, "b": "a b c"}}') as x(a int, b text, c int[], d text, r myrowtype) →
*
* a | b | c | d | r
* ---+---------+---------+---+---------------
* 1 | [1,2,3] | {1,2,3} | | (123,"a b c")
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* @see #jsonbToRecord(Expression)
* @see jsonb_to_record ( jsonb ) → record
*
*
*/
public static UndoneFunction jsonbToRecord(BiFunction funcRef, final T value) {
return jsonbToRecord(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* Expands the top-level JSON object to a row having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) The output record is filled from fields of the JSON object, in the same way as described
* above for json[b]_populate_record. Since there is no input record value, unmatched columns are always filled
* with nulls.
*
* create type myrowtype as (a int, b text);
*
* select * from json_to_record('{"a":1,"b":[1,2,3],"c":[1,2,3],"e":"bar","r": {"a": 123, "b": "a b c"}}') as x(a int, b text, c int[], d text, r myrowtype) →
*
* a | b | c | d | r
* ---+---------+---------+---+---------------
* 1 | [1,2,3] | {1,2,3} | | (123,"a b c")
*
*
* @see jsonb_to_record ( jsonb ) → record
*
*
*/
public static UndoneFunction jsonbToRecord(final Expression json) {
return DialectFunctionUtils.oneArgUndoneFunc("JSONB_TO_RECORD", json);
}
/**
*
* Expands the top-level JSON array of objects to a set of rows having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) Each element of the JSON array is processed as described above for json[b]_populate_record.
*
* select * from json_to_recordset('[{"a":1,"b":"foo"}, {"a":"2","c":"bar"}]') as x(a int, b text) →
*
* a | b
* ---+-----
* 1 | foo
* 2 |
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* @see #jsonToRecordSet(Expression)
* @see json_to_recordset ( json ) → setof record
*
*
*/
public static UndoneFunction jsonToRecordSet(BiFunction funcRef, final T value) {
return jsonToRecordSet(funcRef.apply(JsonType.TEXT, value));
}
/**
*
* Expands the top-level JSON array of objects to a set of rows having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) Each element of the JSON array is processed as described above for json[b]_populate_record.
*
* select * from json_to_recordset('[{"a":1,"b":"foo"}, {"a":"2","c":"bar"}]') as x(a int, b text) →
*
* a | b
* ---+-----
* 1 | foo
* 2 |
*
*
* @see json_to_recordset ( json ) → setof record
*
*
*/
public static UndoneFunction jsonToRecordSet(final Expression json) {
return DialectFunctionUtils.oneArgUndoneFunc("JSON_TO_RECORDSET", json);
}
/**
*
* Expands the top-level JSON array of objects to a set of rows having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) Each element of the JSON array is processed as described above for json[b]_populate_record.
*
* select * from json_to_recordset('[{"a":1,"b":"foo"}, {"a":"2","c":"bar"}]') as x(a int, b text) →
*
* a | b
* ---+-----
* 1 | foo
* 2 |
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonbType#TEXT}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* @see #jsonbToRecordSet(Expression)
* @see jsonb_to_recordset ( jsonb ) → setof record
*
*
*/
public static UndoneFunction jsonbToRecordSet(BiFunction funcRef, final T value) {
return jsonbToRecordSet(funcRef.apply(JsonbType.TEXT, value));
}
/**
*
* Expands the top-level JSON array of objects to a set of rows having the composite type defined by an AS clause.
* (As with all functions returning record, the calling query must explicitly define the structure of the record
* with an AS clause.) Each element of the JSON array is processed as described above for json[b]_populate_record.
*
* select * from json_to_recordset('[{"a":1,"b":"foo"}, {"a":"2","c":"bar"}]') as x(a int, b text) →
*
* a | b
* ---+-----
* 1 | foo
* 2 |
*
*
* @see jsonb_to_recordset ( jsonb ) → setof record
*
*
*/
public static UndoneFunction jsonbToRecordSet(final Expression json) {
return DialectFunctionUtils.oneArgUndoneFunc("JSONB_TO_RECORDSET", json);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @param createIfMissing in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbSet(Expression, Expression, Expression, Expression)
* @see jsonb_set ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSet(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue, Expression createIfMissing) {
return jsonbSet(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue),
createIfMissing
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param createIfMissing in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see jsonb_set ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSet(Expression jsonb, Expression path, Expression newValue,
Expression createIfMissing) {
return FunctionUtils.fourArgFunc("JSONB_SET", jsonb, path, newValue, createIfMissing, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbSet(Expression, Expression, Expression)
* @see jsonb_set ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSet(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue) {
return jsonbSet(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue)
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @see #jsonbSet(Expression, Expression, Expression, Expression)
* @see jsonb_set ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSet(Expression jsonb, Expression path, Expression newValue) {
return FunctionUtils.threeArgFunc("JSONB_SET", jsonb, path, newValue, JsonbType.TEXT);
}
/*-------------------below jsonb_set_lax -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @param createIfMissing in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbSetLax(Expression, Expression, Expression, Expression)
* @see jsonb_set_lax ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean [, null_value_treatment text ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSetLax(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue, Expression createIfMissing) {
return jsonbSetLax(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue),
createIfMissing
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param createIfMissing in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see jsonb_set_lax ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean [, null_value_treatment text ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSetLax(Expression jsonb, Expression path, Expression newValue,
Expression createIfMissing) {
return FunctionUtils.fourArgFunc("JSONB_SET_LAX", jsonb, path, newValue, createIfMissing, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbSetLax(Expression, Expression, Expression)
* @see jsonb_set_lax ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean [, null_value_treatment text ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSetLax(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue) {
return jsonbSetLax(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue)
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @see #jsonbSetLax(Expression, Expression, Expression, Expression)
* @see jsonb_set_lax ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean [, null_value_treatment text ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSetLax(Expression jsonb, Expression path, Expression newValue) {
return FunctionUtils.threeArgFunc("JSONB_SET_LAX", jsonb, path, newValue, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @param createIfMissing in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @param nullTreatMode must be one of
* - {@link Postgres#RAISE_EXCEPTION}
* - {@link Postgres#USE_JSON_NULL}
* - {@link Postgres#DELETE_KEY}
* - {@link Postgres#RETURN_TARGET}
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbSetLax(Expression, Expression, Expression, Expression, Expression)
* @see jsonb_set_lax ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean [, null_value_treatment text ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSetLax(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue, Expression createIfMissing, NullTreatMode nullTreatMode) {
return jsonbSetLax(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue), createIfMissing,
nullTreatMode
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param createIfMissing in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @param nullTreatMode is one of
* - {@link Postgres#RAISE_EXCEPTION}
* - {@link Postgres#USE_JSON_NULL}
* - {@link Postgres#DELETE_KEY}
* - {@link Postgres#RETURN_TARGET}
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbSetLax(Expression, Expression, Expression, Expression)
* @see jsonb_set_lax ( target jsonb, path text[], new_value jsonb [, create_if_missing boolean [, null_value_treatment text ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbSetLax(Expression jsonb, Expression path, Expression newValue,
Expression createIfMissing, Expression nullTreatMode) {
return FunctionUtils.fiveArgFunc("JSONB_SET_LAX", jsonb, path, newValue,
createIfMissing, nullTreatMode,
JsonbType.TEXT
);
}
/*-------------------below jsonb_insert-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @param insertAfter in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbInsert(Expression, Expression, Expression, Expression)
* @see jsonb_insert ( target jsonb, path text[], new_value jsonb [, insert_after boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbInsert(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue, Expression insertAfter) {
return jsonbInsert(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue),
insertAfter
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param insertAfter in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see jsonb_insert ( target jsonb, path text[], new_value jsonb [, insert_after boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbInsert(Expression jsonb, Expression path, Expression newValue,
Expression insertAfter) {
return FunctionUtils.fourArgFunc("JSONB_INSERT", jsonb, path, newValue, insertAfter, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link TextArrayType#LINEAR}.
* @param paths non-null nad non-empty,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForValue the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForValue always is {@link JsonbType#TEXT}.
* @param newValue non-null,it will be passed to funcRefForValue as the second argument of funcRefForValue
* @throws CriteriaException throw when Operand isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbInsert(Expression, Expression, Expression)
* @see jsonb_insert ( target jsonb, path text[], new_value jsonb [, insert_after boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbInsert(Expression jsonb, BiFunction funcRefForPath,
T paths, BiFunction funcRefForValue,
U newValue) {
return jsonbInsert(jsonb, funcRefForPath.apply(TextArrayType.LINEAR, paths),
funcRefForValue.apply(JsonbType.TEXT, newValue)
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbInsert(Expression, Expression, Expression, Expression)
* @see jsonb_insert ( target jsonb, path text[], new_value jsonb [, insert_after boolean ] ) → jsonb
*
*
*/
public static SimpleExpression jsonbInsert(Expression jsonb, Expression path, Expression newValue) {
return FunctionUtils.threeArgFunc("JSONB_INSERT", jsonb, path, newValue, JsonbType.TEXT);
}
/*-------------------below json_strip_nulls-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see json_strip_nulls ( json ) → json
*
*
*/
public static SimpleExpression jsonStripNulls(Expression json) {
return FunctionUtils.oneArgFunc("JSON_STRIP_NULLS", json, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_strip_nulls ( jsonb ) → jsonb
*
*
*/
public static SimpleExpression jsonbStripNulls(Expression json) {
return FunctionUtils.oneArgFunc("JSONB_STRIP_NULLS", json, JsonbType.TEXT);
}
/*-------------------below jsonb_path_exists -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExists(Expression, Expression)
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, BiFunction funcRefForPath,
T path) {
return jsonbPathExists(target, funcRefForPath.apply(JsonPathType.INSTANCE, path));
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, Expression path) {
return FunctionUtils.twoArgPredicateFunc("JSONB_PATH_EXISTS", target, path);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExists(Expression, Expression, Expression)
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, BiFunction funcRefForPath,
T path, Expression vars) {
return jsonbPathExists(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExists(Expression, Expression, Expression)
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars) {
return jsonbPathExists(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars)
);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, Expression path, Expression vars) {
return FunctionUtils.threeArgPredicateFunc("JSONB_PATH_EXISTS", target, path, vars);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExists(Expression, Expression, Expression, Expression)
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, BiFunction funcRefForPath,
T path, Expression vars, Expression silent) {
return jsonbPathExists(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars, silent);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExists(Expression, Expression, Expression, Expression)
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars, Expression silent) {
return jsonbPathExists(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars), silent
);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_exists ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExists(Expression target, Expression path, Expression vars, Expression silent) {
return FunctionUtils.fourArgPredicateFunc("JSONB_PATH_EXISTS", target, path, vars, silent);
}
/*-------------------below jsonb_path_match-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathMatch(Expression, Expression)
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, BiFunction funcRefForPath,
String path) {
return jsonbPathMatch(target, funcRefForPath.apply(JsonPathType.INSTANCE, path));
}
/*-------------------below jsonb_path_match-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathMatch(Expression, Expression)
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, BiFunction funcRefForPath, T path) {
return jsonbPathMatch(target, funcRefForPath.apply(JsonPathType.INSTANCE, path));
}
/**
* The {@link MappingType} of function return type: {@link BooleanType}.
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, Expression path) {
return FunctionUtils.twoArgPredicateFunc("JSONB_PATH_MATCH", target, path);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathMatch(Expression, Expression, Expression)
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, BiFunction funcRefForPath,
T path, Expression vars) {
return jsonbPathMatch(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars);
}
/**
* The {@link MappingType} of function return type: {@link BooleanType}.
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathMatch(Expression, Expression, Expression, Expression)
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, BiFunction funcRefForPath,
T path, Expression vars, Expression silent) {
return jsonbPathMatch(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars, silent);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathMatch(Expression, Expression, Expression)
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars) {
return jsonbPathMatch(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars)
);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, Expression path, Expression vars) {
return FunctionUtils.threeArgPredicateFunc("JSONB_PATH_MATCH", target, path, vars);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathMatch(Expression, Expression, Expression, Expression)
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars,
Expression silent) {
return jsonbPathMatch(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars), silent
);
}
/**
* The {@link MappingType} of function return type: {@link BooleanType}.
*
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_match ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathMatch(Expression target, Expression path, Expression vars, Expression silent) {
return FunctionUtils.fourArgPredicateFunc("JSONB_PATH_MATCH", target, path, vars, silent);
}
/*-------------------below jsonb_path_query-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQuery(Expression, Expression)
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, BiFunction funcRefForPath,
T path) {
return jsonbPathQuery(target, funcRefForPath.apply(JsonPathType.INSTANCE, path));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, Expression path) {
return DialectFunctionUtils.twoArgColumnFunction("JSONB_PATH_QUERY", target, path,
null, JsonbType.TEXT
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQuery(Expression, Expression, Expression)
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, BiFunction funcRefForPath,
T path, Expression vars) {
return jsonbPathQuery(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQuery(Expression, Expression, Expression)
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars) {
return jsonbPathQuery(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars)
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, Expression path, Expression vars) {
return DialectFunctionUtils.threeArgColumnFunction("JSONB_PATH_QUERY", target, path, vars,
null, JsonbType.TEXT
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQuery(Expression, Expression, Expression, Expression)
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, BiFunction funcRefForPath,
T path, Expression vars, Expression silent) {
return jsonbPathQuery(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars, silent);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQuery(Expression, Expression, Expression, Expression)
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars,
U vars, Expression silent) {
return jsonbPathQuery(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars), silent
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* this filed name of function is specified by AS clause.
* *
* jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
* Returns all JSON items returned by the JSON path for the specified JSON value. The optional vars
* and silent arguments act the same as for jsonb_path_exists.
*
* select q.q from jsonb_path_query('{"a":[1,2,3,4,5]}', '$.a[*] ? (@ >= $min && @ <= $max)', '{"min":2, "max":4}') as q →
*
* q
* ------------------
* 2
* 3
* 4
*
*
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → setof jsonb
*
*
*/
public static _ColumnWithOrdinalityFunction jsonbPathQuery(Expression target, Expression path, Expression vars,
Expression silent) {
return DialectFunctionUtils.fourArgColumnFunction("JSONB_PATH_QUERY", target, path, vars, silent,
null, JsonbType.TEXT
);
}
/*-------------------below jsonb_path_query_array-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryArray(Expression, Expression)
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, BiFunction funcRefForPath,
T path) {
return jsonbPathQueryArray(target, funcRefForPath.apply(JsonPathType.INSTANCE, path));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, Expression path) {
return FunctionUtils.twoArgFunc("JSONB_PATH_QUERY_ARRAY", target, path, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryArray(Expression, Expression, Expression)
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, BiFunction funcRefForPath,
T path, Expression vars) {
return jsonbPathQueryArray(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryArray(Expression, Expression, Expression)
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars) {
return jsonbPathQueryArray(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars)
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, Expression path, Expression vars) {
return FunctionUtils.threeArgFunc("JSONB_PATH_QUERY_ARRAY", target, path, vars, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryArray(Expression, Expression, Expression, Expression)
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, BiFunction funcRefForPath,
T path, Expression vars, Expression silent) {
return jsonbPathQueryArray(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars, silent);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryArray(Expression, Expression, Expression, Expression)
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars,
Expression silent) {
return jsonbPathQueryArray(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars), silent
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query_array ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryArray(Expression target, Expression path, Expression vars, Expression silent) {
return FunctionUtils.fourArgFunc("JSONB_PATH_QUERY_ARRAY", target, path, vars, silent, JsonbType.TEXT);
}
/*-------------------below jsonb_path_query_first-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryFirst(Expression, Expression)
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, BiFunction funcRefForPath,
T path) {
return jsonbPathQueryFirst(target, funcRefForPath.apply(JsonPathType.INSTANCE, path));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, Expression path) {
return FunctionUtils.twoArgFunc("JSONB_PATH_QUERY_FIRST", target, path, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryFirst(Expression, Expression, Expression)
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, BiFunction funcRefForPath,
T path, Expression vars) {
return jsonbPathQueryFirst(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryFirst(Expression, Expression, Expression)
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars) {
return jsonbPathQueryFirst(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars)
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, Expression path, Expression vars) {
return FunctionUtils.threeArgFunc("JSONB_PATH_QUERY_FIRST", target, path, vars, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryFirst(Expression, Expression, Expression, Expression)
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, BiFunction funcRefForPath,
T path, Expression vars, Expression silent) {
return jsonbPathQueryFirst(target, funcRefForPath.apply(JsonPathType.INSTANCE, path), vars, silent);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathQueryFirst(Expression, Expression, Expression, Expression)
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars,
Expression silent) {
return jsonbPathQueryFirst(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars), silent
);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
* *
*
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_query_first ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → jsonb
*
*
*/
public static SimpleExpression jsonbPathQueryFirst(Expression target, Expression path, Expression vars,
Expression silent) {
return FunctionUtils.fourArgFunc("JSONB_PATH_QUERY_FIRST", target, path, vars, silent, JsonbType.TEXT);
}
/*-------------------below jsonb_path_exists_tz-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExistsTz(Expression, Expression)
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, BiFunction funcRef, T path) {
return jsonbPathExistsTz(target, funcRef.apply(JsonPathType.INSTANCE, path));
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, Expression path) {
return FunctionUtils.twoArgPredicateFunc("JSONB_PATH_EXISTS_TZ", target, path);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExistsTz(Expression, Expression, Expression)
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, BiFunction funcRef,
T path, Expression vars) {
return jsonbPathExistsTz(target, funcRef.apply(JsonPathType.INSTANCE, path), vars);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExistsTz(Expression, Expression, Expression)
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars) {
return jsonbPathExistsTz(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars)
);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, Expression path, Expression vars) {
return FunctionUtils.threeArgPredicateFunc("JSONB_PATH_EXISTS_TZ", target, path, vars);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRef as the second argument of funcRef
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExistsTz(Expression, Expression, Expression, Expression)
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, BiFunction funcRef,
T path, Expression vars, Expression silent) {
return jsonbPathExistsTz(target, funcRef.apply(JsonPathType.INSTANCE, path), vars, silent);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param funcRefForPath the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForPath always is {@link JsonPathType#INSTANCE}.
* @param path non-null,it will be passed to funcRefForPath as the second argument of funcRefForPath
* @param funcRefForVars the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForVars always is {@link JsonbType#TEXT}.
* @param vars non-null,it will be passed to funcRefForVars as the second argument of funcRefForVars
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see #jsonbPathExistsTz(Expression, Expression, Expression, Expression)
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean
*
*
*/
public static SimplePredicate jsonbPathExistsTz(Expression target, BiFunction funcRefForPath,
T path, BiFunction funcRefForVars, U vars,
Expression silent) {
return jsonbPathExistsTz(target, funcRefForPath.apply(JsonPathType.INSTANCE, path),
funcRefForVars.apply(JsonbType.TEXT, vars), silent
);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}.
* *
*
* @param silent in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @throws CriteriaException throw when argument isn't operable {@link Expression},for example {@link SQLs#DEFAULT},
* {@link SQLs#rowParam(TypeInfer, Collection)}
* @see jsonb_path_exists_tz ( target jsonb, path jsonpath [, vars jsonb [, silent boolean ]] ) → boolean