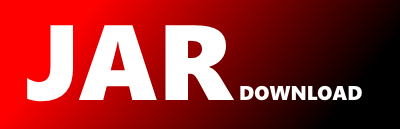
io.army.criteria.impl.PostgreDualBooleanOperator Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.dialect.Database;
import io.army.util._Exceptions;
enum PostgreDualBooleanOperator implements Operator.SqlDualBooleanOperator {
/**
* @see text ^@ text → boolean
*/
CARET_AT(" ^@"),
/**
* @see text ~ text → boolean
* String matches regular expression, case sensitively
*/
TILDE(" ~"),
/**
* @see text !~ text → boolean
* String does not match regular expression, case sensitively
*/
NOT_TILDE(" !~"),
/**
* @see text ~* text → boolean
* String matches regular expression, case insensitively
*/
TILDE_STAR(" ~*"),
/**
* @see text !~* text → boolean
* String does not match regular expression, case insensitively
*/
NOT_TILDE_STAR(" !~*"),
/**
* @see geometric_type @> geometric_type → boolean
* Does first object contain second? Available for these pairs of types: (box, point), (box, box), (path, point), (polygon, point), (polygon, polygon), (circle, point), (circle, circle).
*
*/
AT_GT(" @>"),
/**
* @see geometric_type <@ geometric_type → boolean
* Is first object contained in or on second? Available for these pairs of types: (point, box), (point, lseg), (point, line), (point, path), (point, polygon), (point, circle), (box, box), (lseg, box), (lseg, line), (polygon, polygon), (circle, circle).
*/
LT_AT(" <@"),
/**
* @see geometric_type << geometric_type → boolean
* Is first object strictly left of second? Available for point, box, polygon, circle.
* inet << inet → boolean
* Is subnet strictly contained by subnet? This operator, and the next four, test for subnet inclusion. They consider only the network parts of the two
* addresses (ignoring any bits to the right of the netmasks) and determine whether one network is identical to or a subnet of the other.
* inet '192.168.1.5' << inet '192.168.1/24' → t
* inet '192.168.0.5' << inet '192.168.1/24' → f
* inet '192.168.1/24' << inet '192.168.1/24' → f
*
* @see inet << inet → boolean
* Is subnet strictly contained by subnet? This operator, and the next four, test for subnet inclusion. They consider only the network parts of the two
* addresses (ignoring any bits to the right of the netmasks) and determine whether one network is identical to or a subnet of the other.
* inet '192.168.1.5' << inet '192.168.1/24' → t
* inet '192.168.0.5' << inet '192.168.1/24' → f
* inet '192.168.1/24' << inet '192.168.1/24' → f
*
*/
LT_LT(" <<"),
/**
* @see geometric_type >> geometric_type → boolean
* Is first object strictly right of second? Available for point, box, polygon, circle.
*/
GT_GT(" >>"),
/**
* @see inet <<= inet → boolean
* Is subnet contained by or equal to subnet?
* inet '192.168.1/24' <<= inet '192.168.1/24' → t
*
*/
LT_LT_EQUAL(" <<="),
/**
* @see inet >>= inet → boolean
* Does subnet contain or equal subnet?
* inet '192.168.1/24' >>= inet '192.168.1/24' → t
*
*/
GT_GT_EQUAL(" >>="),
/**
* double ampersand
*
* @see geometric_type && geometric_type → boolean
* Do these objects overlap? (One point in common makes this true.) Available for box, polygon, circle.
*/
DOUBLE_AMP(" &&"),
/**
* @see geometric_type &< geometric_type → boolean
* Does first object not extend to the right of second? Available for box, polygon, circle.
*/
AMP_LT(" &<"),
/**
* @see geometric_type &> geometric_type → boolean
* Does first object not extend to the left of second? Available for box, polygon, circle.
*/
AMP_GT(" &>"),
/**
* @see geometric_type <<| geometric_type → boolean
* Is first object strictly below second? Available for point, box, polygon, circle.
*/
LT_LT_VERTICAL(" <<|"),
/**
* @see geometric_type |>> geometric_type → boolean
* Is first object strictly above second? Available for point, box, polygon, circle.
*/
VERTICAL_GT_GT(" |>>"),
/**
* @see geometric_type &<| geometric_type → boolean
* Does first object not extend above second? Available for box, polygon, circle.
*/
AMP_LT_VERTICAL(" &<|"),
/**
* @see geometric_type |&> geometric_type → boolean
* Does first object not extend below second? Available for box, polygon, circle.
*/
VERTICAL_AMP_GT(" |&>"),
/**
* @see box <^ box → boolean
* Is first object below second (allows edges to touch)?
*/
LT_CARET(" <^"),
/**
* @see box >^ box → boolean
* Is first object above second (allows edges to touch)?
*/
GT_CARET(" >^"),
/**
* @see jsonb ? text → boolean
* Does the text string exist as a top-level key or array element within the JSON value?
* '{"a":1, "b":2}'::jsonb ? 'b' → t
* '["a", "b", "c"]'::jsonb ? 'b' → t
*
*/
QUESTION(" ?"),
/**
* @see geometric_type ?# geometric_type → boolean
* Is first object above second (allows edges to touch)?
*/
QUESTION_POUND(" ?#"),
/**
* @see point ?- point → boolean
* Are points horizontally aligned (that is, have same y coordinate)?
*/
QUESTION_HYPHEN(" ?-"),
/**
* @see jsonb ?& text[] → boolean
* Do all of the strings in the text array exist as top-level keys or array elements?
* '["a", "b", "c"]'::jsonb ?& array['a', 'b'] → t
*
*/
QUESTION_AMP(" ?&"),
/**
* @see point ?| point → boolean
* Are points vertically aligned (that is, have same x coordinate)?
*/
QUESTION_VERTICAL(" ?|"),
/**
* @see line ?-| line → boolean
* lseg ?-| lseg → boolean
*/
QUESTION_HYPHEN_VERTICAL(" ?-|"),
/**
* @see line ?|| line → boolean
* lseg ?|| lseg → boolean
*/
QUESTION_VERTICAL_VERTICAL(" ?||"),
/**
* @see geometric_type ~= geometric_type → boolean
* Are these objects the same? Available for point, box, polygon, circle.
*
*/
TILDE_EQUAL(" ~="),
/**
* @see anyrange -|- anyrange → boolean
* Are the ranges adjacent?.
* numrange(1.1,2.2) -|- numrange(2.2,3.3) → t
*
*/
HYPHEN_VERTICAL_HYPHEN(" -|-"),
/**
* @see jsonb @? jsonpath → boolean
* Does JSON path return any item for the specified JSON value?
* '{"a":[1,2,3,4,5]}'::jsonb @? '$.a[*] ? (@ > 2)' → t
*
*/
AT_QUESTION(" @?"),
/**
* @see tsvector @@ tsquery → boolean
* tsquery @@ tsvector → boolean
* text @@ tsquery → boolean
*
*/
DOUBLE_AT(" @@"),
/**
* @see tsvector @@@ tsquery → boolean
* tsquery @@@ tsvector → boolean
*
*/
TRIPLE_AT(" @@@"),
/**
* @see row_constructor IS DISTINCT FROM row_constructor
*
*/
IS_DISTINCT_FROM(" IS DISTINCT FROM"),
/**
* @see row_constructor IS NOT DISTINCT FROM row_constructor
*
*/
IS_NOT_DISTINCT_FROM(" IS NOT DISTINCT FROM");
private final String spaceOperator;
PostgreDualBooleanOperator(String spaceOperator) {
this.spaceOperator = spaceOperator;
}
@Override
public final String spaceRender() {
return this.spaceOperator;
}
@Override
public final Database database() {
return Database.PostgreSQL;
}
@Override
public final String spaceRender(final Database database) {
if (database != Database.PostgreSQL) {
throw _Exceptions.operatorError(this, database);
}
return this.spaceOperator;
}
@Override
public final String toString() {
return CriteriaUtils.enumToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy