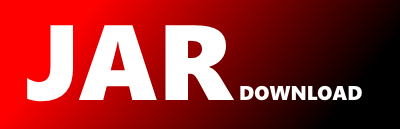
io.army.criteria.impl.PostgreMiscellaneous2Functions Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.criteria.*;
import io.army.mapping.*;
import io.army.mapping.array.IntegerArrayType;
import io.army.mapping.array.ShortArrayType;
import io.army.mapping.array.TextArrayType;
import io.army.mapping.postgre.PostgreAclItemType;
import io.army.mapping.postgre.PostgreInetType;
import io.army.mapping.postgre.PostgreRangeType;
import io.army.mapping.postgre.PostgreTsVectorType;
import io.army.mapping.postgre.array.PostgreAclItemArrayType;
import io.army.util.ArrayUtils;
import io.army.util._Collections;
import javax.annotation.Nullable;
import java.util.Collection;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.UnaryOperator;
abstract class PostgreMiscellaneous2Functions extends PostgreMiscellaneousFunctions {
/**
* package constructor
*/
PostgreMiscellaneous2Functions() {
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see get_current_ts_config ( ) → regconfig
*/
public static SimpleExpression getCurrentTsConfig() {
return FunctionUtils.zeroArgFunc("GET_CURRENT_TS_CONFIG", StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see numnode ( tsquery ) → integer
*/
public static SimpleExpression numNode(Expression exp) {
return FunctionUtils.oneArgFunc("NUMNODE", exp, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function returned fields type:
* - alias {@link TextType}
* - description {@link TextType}
* - token {@link TextType}
* - dictionaries {@link TextArrayType#LINEAR}
* - dictionary {@link TextType}
* - lexemes {@link TextArrayType#LINEAR}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see ts_debug ( [ config regconfig, ] document text ) → setof record ( alias text, description text, token text, dictionaries regdictionary[], dictionary regdictionary, lexemes text[] )
* Extracts and normalizes tokens from the document according to the specified or default text search configuration, and returns information about how each token was processed.
* ts_debug('english', 'The Brightest supernovaes') → (asciiword,"Word, all ASCII",The,{english_stem},english_stem,{})
*
*/
public static _TabularWithOrdinalityFunction tsDebug(Expression document) {
return _tsDebug(null, document);
}
/**
*
* The {@link MappingType} of function returned fields type:
* - alias {@link TextType}
* - description {@link TextType}
* - token {@link TextType}
* - dictionaries {@link TextArrayType#LINEAR}
* - dictionary {@link TextType}
* - lexemes {@link TextArrayType#LINEAR}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see ts_debug ( [ config regconfig, ] document text ) → setof record ( alias text, description text, token text, dictionaries regdictionary[], dictionary regdictionary, lexemes text[] )
* Extracts and normalizes tokens from the document according to the specified or default text search configuration, and returns information about how each token was processed.
* ts_debug('english', 'The Brightest supernovaes') → (asciiword,"Word, all ASCII",The,{english_stem},english_stem,{})
*
*/
public static _TabularWithOrdinalityFunction tsDebug(Expression config, Expression document) {
return _tsDebug(config, document);
}
/**
*
* The {@link MappingType} of function return type: {@link TextArrayType#LINEAR}.
*
*
* @see ts_lexize ( dict regdictionary, token text ) → text[]
*/
public static SimpleExpression tsLexize(Expression dict, Expression token) {
return FunctionUtils.twoArgFunc("TS_LEXIZE", dict, token, TextArrayType.LINEAR);
}
/**
*
* The {@link MappingType} of function returned fields type:
* - tokid {@link IntegerType}
* - token {@link TextType}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see ts_parse ( parser_name text, document text ) → setof record ( tokid integer, token text )
* Extracts tokens from the document using the named parser.
* ts_parse('default', 'foo - bar') → (1,foo)
* ts_parse ( parser_oid oid, document text ) → setof record ( tokid integer, token text )
* Extracts tokens from the document using a parser specified by OID.
* ts_parse(3722, 'foo - bar') → (1,foo)
*
*/
public static _TabularWithOrdinalityFunction tsParse(Expression parserName, Expression document) {
final List fieldList = _Collections.arrayList(2);
fieldList.add(ArmySelections.forName("tokid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("token", TextType.INSTANCE));
return DialectFunctionUtils.twoArgTabularFunc("TS_PARSE", parserName, document, fieldList);
}
/**
*
* The {@link MappingType} of function returned fields type:
* - tokid {@link IntegerType}
* - alias {@link TextType}
* - description {@link TextType}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see ts_token_type ( parser_name text ) → setof record ( tokid integer, alias text, description text )
* Returns a table that describes each type of token the named parser can recognize.
* ts_token_type('default') → (1,asciiword,"Word, all ASCII")
* ts_token_type ( parser_oid oid ) → setof record ( tokid integer, alias text, description text )
* Returns a table that describes each type of token a parser specified by OID can recognize.
* ts_token_type(3722) → (1,asciiword,"Word, all ASCII")
*
*/
public static _TabularWithOrdinalityFunction tsTokenType(Expression exp) {
final List fieldList = _Collections.arrayList(3);
fieldList.add(ArmySelections.forName("tokid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("alias", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("description", TextType.INSTANCE));
return DialectFunctionUtils.oneArgTabularFunc("TS_TOKEN_TYPE", exp, fieldList);
}
/**
*
* The {@link MappingType} of function returned fields type:
* - word {@link TextType}
* - ndoc {@link IntegerType}
* - nentry {@link IntegerType}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see #tsStat(Expression, Expression)
* @see ts_stat ( sqlquery text [, weights text ] ) → setof record ( word text, ndoc integer, nentry integer )
* Executes the sqlquery, which must return a single tsvector column, and returns statistics about each distinct lexeme contained in the data.
* ts_stat('SELECT vector FROM apod') → (foo,10,15)
*
*/
public static _TabularWithOrdinalityFunction tsStat(Expression sqlQuery) {
return _tsStat(sqlQuery, null);
}
/**
*
* The {@link MappingType} of function returned fields type:
* - word {@link TextType}
* - ndoc {@link IntegerType}
* - nentry {@link IntegerType}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see #tsStat(Expression)
* @see ts_stat ( sqlquery text [, weights text ] ) → setof record ( word text, ndoc integer, nentry integer )
* Executes the sqlquery, which must return a single tsvector column, and returns statistics about each distinct lexeme contained in the data.
* ts_stat('SELECT vector FROM apod') → (foo,10,15)
*
*/
public static _TabularWithOrdinalityFunction tsStat(Expression sqlQuery, Expression weights) {
return _tsStat(sqlQuery, weights);
}
/**
*
* The {@link MappingType} of function return type: {@link UUIDType}
*
*
* @see UUID Functions
*/
public static SimpleExpression genRandomUuid() {
return FunctionUtils.zeroArgFunc("GEN_RANDOM_UUID", UUIDType.INSTANCE);
}
/*-------------------below Sequence Manipulation Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link LongType}
*
*
* @see nextval ( regclass ) → bigint
*/
public static SimpleExpression nextVal(Expression exp) {
return FunctionUtils.oneArgFunc("NEXTVAL", exp, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType}
*
* @see #setVal(Expression, Expression, Expression)
* @see setval ( regclass, bigint [, boolean ] ) → bigint
*/
public static SimpleExpression setVal(Expression regClass, Expression value) {
return FunctionUtils.twoArgFunc("SETVAL", regClass, value, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType}
*
*
* @param isCalled in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see #setVal(Expression, Expression)
* @see setval ( regclass, bigint [, boolean ] ) → bigint
*/
public static SimpleExpression setVal(Expression regClass, Expression value, Expression isCalled) {
return FunctionUtils.threeArgFunc("SETVAL", regClass, value, isCalled, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType}
*
*
* @see currval ( regclass ) → bigint
*/
public static SimpleExpression currVal(Expression exp) {
return FunctionUtils.oneArgFunc("CURRVAL", exp, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType}
*
*
* @see lastval ( regclass ) → bigint
*/
public static SimpleExpression lastVal(Expression exp) {
return FunctionUtils.oneArgFunc("LASTVAL", exp, LongType.INSTANCE);
}
/*-------------------below Conditional Expressions-------------------*/
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of firstValue
*
*
* @throws CriteriaException throw when
* - firstValue isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
* - firstValue is multi value {@link Expression},eg: {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - the element of rest isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see COALESCE(value [, ...])
*/
public static SimpleExpression coalesce(Expression firstValue, Expression... rest) {
return FunctionUtils.oneAndRestFunc("COALESCE", _returnType(firstValue, Expressions::identityType),
firstValue, rest
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of fist argument
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see COALESCE(value [, ...])
*/
public static SimpleExpression coalesce(Consumer> consumer) {
return FunctionUtils.consumerAndFirstTypeFunc("COALESCE", consumer);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of firstValue
*
*
* @throws CriteriaException throw when
* - firstValue isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
* - firstValue is multi value {@link Expression},eg: {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - the element of rest isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see GREATEST(value [, ...])
*/
public static SimpleExpression greatest(Expression firstValue, Expression... rest) {
return FunctionUtils.oneAndRestFunc("GREATEST", _returnType(firstValue, Expressions::identityType),
firstValue, rest
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of fist argument
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see GREATEST(value [, ...])
*/
public static SimpleExpression greatest(Consumer> consumer) {
return FunctionUtils.consumerAndFirstTypeFunc("GREATEST", consumer);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of firstValue
*
*
* @throws CriteriaException throw when
* - firstValue isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
* - firstValue is multi value {@link Expression},eg: {@link SQLs#rowLiteral(TypeInfer, Collection)}
* - the element of rest isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see LEAST(value [, ...])
*/
public static SimpleExpression least(Expression firstValue, Expression... rest) {
return FunctionUtils.oneAndRestFunc("LEAST", _returnType(firstValue, Expressions::identityType),
firstValue, rest
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of fist argument
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see LEAST(value [, ...])
*/
public static SimpleExpression least(Consumer> consumer) {
return FunctionUtils.consumerAndFirstTypeFunc("LEAST", consumer);
}
/*-------------------below Array Functions-------------------*/
/**
* The {@link MappingType} of function return type: the {@link MappingType} of fist anyCompatibleArray
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_append ( anycompatiblearray, anycompatible ) → anycompatiblearray
*/
public static SimpleExpression arrayAppend(Expression anyCompatibleArray, Expression anyCompatible) {
return FunctionUtils.twoArgFunc("ARRAY_APPEND", anyCompatibleArray, anyCompatible,
_returnType(anyCompatibleArray, Expressions::identityType)
);
}
/**
* The {@link MappingType} of function return type: the {@link MappingType} of fist anyCompatibleArray1
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_cat ( anycompatiblearray, anycompatiblearray ) → anycompatiblearray
*/
public static SimpleExpression arrayCat(Expression anyCompatibleArray1, Expression anyCompatibleArray2) {
return FunctionUtils.twoArgFunc("ARRAY_CAT", anyCompatibleArray1, anyCompatibleArray2,
_returnType(anyCompatibleArray1, Expressions::identityType)
);
}
/**
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_dims ( anyarray ) → text
*/
public static SimpleExpression arrayDims(Expression anyArray) {
return FunctionUtils.oneArgFunc("ARRAY_DIMS", anyArray, TextType.INSTANCE);
}
/**
* The {@link MappingType} of function return type: the array type of {@link MappingType} of anyElement.
*
* @param funcRefForDimension the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForDimension always is {@link IntegerArrayType#LINEAR}.
* @param dimensions non-null,it will be passed to funcRefForDimension as the second argument of funcRefForDimension
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_fill ( anyelement, integer[] [, integer[] ] ) → anyarray
*/
public static SimpleExpression arrayFill(Expression anyElement, BiFunction funcRefForDimension,
T dimensions) {
return _arrayFill(anyElement, funcRefForDimension.apply(IntegerArrayType.LINEAR, dimensions), null);
}
/**
* The {@link MappingType} of function return type: the array type of {@link MappingType} of anyElement.
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_fill ( anyelement, integer[] [, integer[] ] ) → anyarray
*/
public static SimpleExpression arrayFill(Expression anyElement, Expression dimensions) {
return _arrayFill(anyElement, dimensions, null);
}
/**
*
* The {@link MappingType} of function return type: the array type of {@link MappingType} of anyElement.
*
*
* @param funcRefForDimension the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForDimension always is {@link IntegerArrayType#LINEAR}.
* @param dimensions non-null,it will be passed to funcRefForDimension as the second argument of funcRefForDimension
* @param funcRefForBound the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRefForBound always is {@link IntegerArrayType#LINEAR}.
* @param bounds non-null,it will be passed to funcRefForBound as the second argument of funcRefForBound
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_fill ( anyelement, integer[] [, integer[] ] ) → anyarray
*/
public static SimpleExpression arrayFill(Expression anyElement, BiFunction funcRefForDimension,
T dimensions, BiFunction funcRefForBound, U bounds) {
return _arrayFill(anyElement, funcRefForDimension.apply(IntegerArrayType.LINEAR, dimensions),
funcRefForBound.apply(IntegerArrayType.LINEAR, bounds)
);
}
/**
* The {@link MappingType} of function return type: the array type of {@link MappingType} of anyElement.
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_fill ( anyelement, integer[] [, integer[] ] ) → anyarray
*/
public static SimpleExpression arrayFill(Expression anyElement, Expression dimensions, Expression bounds) {
ContextStack.assertNonNull(bounds);
return _arrayFill(anyElement, dimensions, bounds);
}
/**
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link IntegerType#INSTANCE}.
* @param dimension non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayLength(Expression, Expression)
* @see array_length ( anyarray, integer ) → integer
*/
public static SimpleExpression arrayLength(Expression anyArray, BiFunction funcRef,
T dimension) {
return arrayLength(anyArray, funcRef.apply(IntegerType.INSTANCE, dimension));
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_length ( anyarray, integer ) → integer
*/
public static SimpleExpression arrayLength(Expression anyArray, Expression dimension) {
return FunctionUtils.twoArgFunc("ARRAY_LENGTH", anyArray, dimension, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayUpper(Expression, Expression)
* @see array_lower ( anyarray, integer ) → integer
*/
public static SimpleExpression arrayLower(Expression anyArray, Expression dimension) {
return FunctionUtils.twoArgFunc("ARRAY_LOWER", anyArray, dimension, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_ndims ( anyarray ) → integer
*/
public static SimpleExpression arrayNDims(Expression anyArray) {
return FunctionUtils.oneArgFunc("ARRAY_NDIMS", anyArray, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayPosition(Expression, Expression, Expression)
* @see array_position ( anycompatiblearray, anycompatible [, integer ] ) → integer
*/
public static SimpleExpression arrayPosition(Expression anyCompatibleArray, Expression anyCompatible) {
return FunctionUtils.twoArgFunc("ARRAY_POSITION", anyCompatibleArray, anyCompatible, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - {@link SQLs#encodingParam(TypeInfer, Object)},used when only this is instance of {@link TableField} and {@link TableField#codec()} is true
* - {@link SQLs#encodingLiteral(TypeInfer, Object)},used when only this is instance of {@link TableField} and {@link TableField#codec()} is true
* - {@link SQLs#encodingNamedParam(TypeInfer, String)} ,used when only this is instance of {@link TableField} and {@link TableField#codec()} is true
* and in INSERT( or batch update/delete ) syntax
* - {@link SQLs#encodingNamedLiteral(TypeInfer, String)} ,used when only this is instance of {@link TableField} and {@link TableField#codec()} is true
* and in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link IntegerType#INSTANCE}.
* @param subscript non-null,it will be passed to funcRef as the second argument of funcRef
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayPosition(Expression, Expression, Expression)
* @see array_position ( anycompatiblearray, anycompatible [, integer ] ) → integer
*/
public static SimpleExpression arrayPosition(Expression anyCompatibleArray, Expression anyCompatible,
BiFunction funcRef, T subscript) {
return arrayPosition(anyCompatibleArray, anyCompatible, funcRef.apply(IntegerType.INSTANCE, subscript));
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_position ( anycompatiblearray, anycompatible [, integer ] ) → integer
*/
public static SimpleExpression arrayPosition(Expression anyCompatibleArray, Expression anyCompatible,
Expression subscript) {
return FunctionUtils.threeArgFunc("ARRAY_POSITION", anyCompatibleArray, anyCompatible, subscript,
IntegerType.INSTANCE
);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerArrayType#LINEAR}
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_positions ( anycompatiblearray, anycompatible ) → integer[]
*/
public static SimpleExpression arrayPositions(Expression anyCompatibleArray, Expression anyCompatible) {
return FunctionUtils.twoArgFunc("ARRAY_POSITIONS", anyCompatibleArray, anyCompatible, IntegerArrayType.LINEAR);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of anyCompatibleArray.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_prepend ( anycompatible, anycompatiblearray ) → anycompatiblearray
*/
public static SimpleExpression arrayPrepend(Expression anyCompatible, Expression anyCompatibleArray) {
return FunctionUtils.twoArgFunc("ARRAY_PREPEND", anyCompatible, anyCompatibleArray,
_returnType(anyCompatibleArray, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of anyCompatibleArray.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_remove ( anycompatiblearray, anycompatible ) → anycompatiblearray
*/
public static SimpleExpression arrayRemove(Expression anyCompatibleArray, Expression anyCompatible) {
return FunctionUtils.twoArgFunc("ARRAY_REMOVE", anyCompatibleArray, anyCompatible,
_returnType(anyCompatibleArray, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of anyCompatibleArray.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_replace ( anycompatiblearray, anycompatible, anycompatible ) → anycompatiblearray
*/
public static SimpleExpression arrayReplace(Expression anyCompatibleArray, Expression anyCompatible,
Expression replacement) {
return FunctionUtils.threeArgFunc("ARRAY_REPLACE", anyCompatibleArray, anyCompatible, replacement,
_returnType(anyCompatibleArray, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see array_to_string ( array anyarray, delimiter text [, null_string text ] ) → text
*/
public static SimpleExpression arrayToString(Expression array, Expression delimiter, Expression nullString) {
return FunctionUtils.threeArgFunc("ARRAY_TO_STRING", array, delimiter, nullString, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayLower(Expression, Expression)
* @see array_upper ( anyarray, integer ) → integer
*/
public static SimpleExpression arrayUpper(Expression anyArray, Expression dimension) {
return FunctionUtils.twoArgFunc("ARRAY_UPPER", anyArray, dimension, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayLower(Expression, Expression)
* @see cardinality ( anyarray ) → integer
*/
public static SimpleExpression cardinality(Expression anyArray) {
return FunctionUtils.oneArgFunc("CARDINALITY", anyArray, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of array.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see #arrayLower(Expression, Expression)
* @see trim_array ( array anyarray, n integer ) → anyarray
*/
public static SimpleExpression trimArray(Expression array, Expression n) {
return FunctionUtils.twoArgFunc("TRIM_ARRAY", array, n, _returnType(array, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function returned fields type:
* - lexeme {@link TextType}
* - positions {@link ShortArrayType} with one dimension
* - weights {@link TextType}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
*
* select * from unnest('cat:3 fat:2,4 rat:5A'::tsvector) →
*
* lexeme | positions | weights
* --------+-----------+---------
* cat | {3} | {D}
* fat | {2,4} | {D,D}
* rat | {5} | {A}
*
*
*
*
* If exp is array,then the {@link MappingType} of function returned is the {@link MappingType} of the element.
*
* unnest ( anyarray ) → setof anyelement
*
* Expands an array into a set of rows. The array's elements are read out in storage order.
*
* unnest(ARRAY[1,2]) →
*
* 1
* 2
* unnest(ARRAY[['foo','bar'],['baz','quux']]) →
*
* foo
* bar
* baz
* quux
*
*
*
* @see unnest ( tsvector ) → setof record ( lexeme text, positions smallint[], weights text )
* Expands a tsvector into a set of rows, one per lexeme
*
* @see unnest ( anyarray ) → setof anyelement
* Expands an array into a set of rows. The array's elements are read out in storage order.
*
* @see unnest ( anymultirange ) → setof anyrange
* Expands a multirange into a set of ranges. The ranges are read out in storage order (ascending).
* unnest('{[1,2), [3,4)}'::int4multirange) →
* [1,2)
* [3,4)
*
*/
public static _TabularWithOrdinalityFunction unnest(final Expression exp) {
final String name = "UNNEST";
final MappingType type;
type = exp.typeMeta().mappingType();
final _TabularWithOrdinalityFunction func;
if (type instanceof MappingType.SqlArrayType) {
func = DialectFunctionUtils.oneArgColumnFunction(name, exp, null, CriteriaUtils.arrayUnderlyingType(type));
} else if (type instanceof PostgreTsVectorType) {
final List fieldList = _Collections.arrayList(3);
fieldList.add(ArmySelections.forName("lexeme", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("positions", ShortArrayType.from(Short[].class)));
fieldList.add(ArmySelections.forName("weights", TextType.INSTANCE));
func = DialectFunctionUtils.oneArgTabularFunc(name, exp, fieldList);
} else if (type instanceof PostgreRangeType.MultiRangeType) {
func = DialectFunctionUtils.oneArgColumnFunction(name, exp, null,
((PostgreRangeType.MultiRangeType) type).rangeType());
} else {
throw CriteriaUtils.funcArgError(name, exp);
}
return func;
}
/**
*
* If exp is array,then the {@link MappingType} of function returned is the {@link MappingType} of the element.
*
* unnest ( anyarray ) → setof anyelement
*
* Expands an array into a set of rows. The array's elements are read out in storage order.
*
* unnest(ARRAY[1,2]) →
*
* 1
* 2
* unnest(ARRAY[['foo','bar'],['baz','quux']]) →
*
* foo
* bar
* baz
* quux
*
*
*
* @see unnest ( anyarray ) → setof anyelement
* Expands an array into a set of rows. The array's elements are read out in storage order.
*
*/
public static _ColumnWithOrdinalityFunction unnest(final ArrayExpression exp) {
return DialectFunctionUtils.oneArgColumnFunction("UNNEST", exp, null,
CriteriaUtils.arrayUnderlyingType(exp.typeMeta().mappingType())
);
}
/**
*
* If exp is array,then the {@link MappingType} of function returned is the {@link MappingType} of the element.
*
* select * from unnest(ARRAY[1,2], ARRAY['foo','bar','baz']) as x(a,b) →
*
* a | b
* ---+-----
* 1 | foo
* 2 | bar
* | baz
*
*
*
*
* @see unnest ( anyarray, anyarray [, ... ] ) → setof anyelement, anyelement [, ... ]
* Expands multiple arrays (possibly of different data types) into a set of rows. If the arrays are not all the same length then the shorter ones are padded with NULLs. This form is only allowed in a query's FROM clause;
*
*/
public static _TabularWithOrdinalityFunction unnest(ArrayExpression array1, ArrayExpression array2) {
final List fieldList = _Collections.arrayList(2);
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(array1.typeMeta())));
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(array2.typeMeta())));
return DialectFunctionUtils.twoArgTabularFunc("UNNEST", array1, array2, fieldList);
}
/**
*
* If exp is array,then the {@link MappingType} of function returned is the {@link MappingType} of the element.
*
* select * from unnest(ARRAY[1,2], ARRAY['foo','bar','baz']) as x(a,b) →
*
* a | b
* ---+-----
* 1 | foo
* 2 | bar
* | baz
*
*
*
*
* @see unnest ( anyarray, anyarray [, ... ] ) → setof anyelement, anyelement [, ... ]
* Expands multiple arrays (possibly of different data types) into a set of rows. If the arrays are not all the same length then the shorter ones are padded with NULLs. This form is only allowed in a query's FROM clause;
*
*/
public static _TabularWithOrdinalityFunction unnest(ArrayExpression array1, ArrayExpression array2,
ArrayExpression array3, ArrayExpression... restArray) {
final String name = "UNNEST";
final List fieldList = _Collections.arrayList(3 + restArray.length);
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(array1.typeMeta())));
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(array2.typeMeta())));
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(array3.typeMeta())));
final _TabularWithOrdinalityFunction func;
if (restArray.length == 0) {
func = DialectFunctionUtils.threeArgTabularFunc(name, array1, array2, array3, fieldList);
} else {
final List argList = _Collections.arrayList(3 + restArray.length);
argList.add((ArmyExpression) array1);
argList.add((ArmyExpression) array2);
argList.add((ArmyExpression) array3);
for (ArrayExpression array : restArray) {
argList.add((ArmyExpression) array);
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(array.typeMeta())));
}
func = DialectFunctionUtils.multiArgTabularFunc(name, argList, fieldList);
}
return func;
}
/**
*
* If exp is array,then the {@link MappingType} of function returned is the {@link MappingType} of the element.
*
* select * from unnest(ARRAY[1,2], ARRAY['foo','bar','baz']) as x(a,b) →
*
* a | b
* ---+-----
* 1 | foo
* 2 | bar
* | baz
*
*
*
*
* @see unnest ( anyarray, anyarray [, ... ] ) → setof anyelement, anyelement [, ... ]
* Expands multiple arrays (possibly of different data types) into a set of rows. If the arrays are not all the same length then the shorter ones are padded with NULLs. This form is only allowed in a query's FROM clause;
*
*/
public static _TabularWithOrdinalityFunction unnest(final Consumer> consumer) {
final String name = "UNNEST";
final List argList = _Collections.arrayList();
final List fieldList = _Collections.arrayList();
consumer.accept(exp -> {
argList.add((ArmyExpression) exp);
fieldList.add(ArmySelections.forAnonymous(CriteriaUtils.arrayUnderlyingType(exp.typeMeta())));
});
if (argList.size() == 0) {
throw CriteriaUtils.dontAddAnyItem();
}
return DialectFunctionUtils.multiArgTabularFunc(name, argList, fieldList);
}
/*-------------------below Range/Multirange Functions and Operators -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see isempty ( anyrange ) → boolean
* Is the range empty?
* isempty(numrange(1.1,2.2)) → f
*
*/
public static SimplePredicate isEmpty(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("ISEMPTY", exp);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see lower_inc ( anyrange ) → boolean
* Is the range's lower bound inclusive?
* lower_inc(numrange(1.1,2.2)) → t
*
*/
public static SimplePredicate lowerInc(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("LOWER_INC", exp);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see upper_inc ( anyrange ) → boolean
* Is the range's upper bound inclusive?
* upper_inc(numrange(1.1,2.2)) → t
*
*/
public static SimplePredicate upperInc(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("UPPER_INC", exp);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see lower_inf ( anyrange ) → boolean
* Is the range's lower bound infinite?
* lower_inf(numrange(1.1,2.2)) → t
*
*/
public static SimplePredicate lowerInf(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("LOWER_INF", exp);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see upper_inf ( anyrange ) → boolean
* Is the range's upper bound infinite?
* upper_inf(numrange(1.1,2.2)) → t
*
*/
public static SimplePredicate upperInf(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("UPPER_INF", exp);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of range1.
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see range_merge ( anyrange, anyrange ) → anyrange
* Computes the smallest range that includes both of the given ranges.
* range_merge('[1,2)'::int4range, '[3,4)'::int4range) → [1,4)
*
*/
public static SimpleExpression rangeMerge(Expression range1, Expression range2) {
return FunctionUtils.twoArgFunc("RANGE_MERGE", range1, range2, _returnType(range1, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type:
* - If anyRange is {@link PostgreRangeType.SingleRangeType} ,then the multi range of the {@link MappingType} of anyRange.
* - Else {@link TextType#INSTANCE}
*
*
*
* @throws CriteriaException throw when
* - the element of consumer isn't operable {@link Expression},eg:{@link SQLs#DEFAULT}
*
* @see multirange ( anyrange ) → anymultirange
* Returns a multirange containing just the given range.
* multirange('[1,2)'::int4range) → {[1,2)}
*
*/
public static SimpleExpression multiRange(final Expression anyRange) {
final UnaryOperator func;
func = t -> {
final MappingType type;
if (t instanceof PostgreRangeType.SingleRangeType) {
type = ((PostgreRangeType.SingleRangeType) t).multiRangeType();
} else {
type = TextType.INSTANCE;
}
return type;
};
return FunctionUtils.oneArgFunc("multirange", anyRange, _returnType(anyRange, func));
}
/*-------------------below Series Generating Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of start
*
*
* @see Set Returning Functions
*
*/
public static _ColumnWithOrdinalityFunction generateSeries(Expression start, Expression stop) {
return DialectFunctionUtils.twoArgColumnFunction("generate_series", start, stop, null,
_returnType(start, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of start
*
*
* @see Set Returning Functions
*
*/
public static _ColumnWithOrdinalityFunction generateSeries(Expression start, Expression stop, Expression step) {
return DialectFunctionUtils.threeArgColumnFunction("generate_series", start, stop, step, null,
_returnType(start, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE} rt
*
*
* @see Subscript Generating Functions
*
*/
public static _ColumnWithOrdinalityFunction generateSubscripts(Expression array, Expression dim) {
return DialectFunctionUtils.twoArgColumnFunction("generate_subscripts", array, dim, null,
IntegerType.INSTANCE
);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @param reverse in mose case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see Subscript Generating Functions
*
*/
public static _ColumnWithOrdinalityFunction generateSubscripts(Expression array, Expression dim, Expression reverse) {
return DialectFunctionUtils.threeArgColumnFunction("generate_subscripts", array, dim, reverse, null,
IntegerType.INSTANCE
);
}
/*-------------------below System Information Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see current_database () → name
*
*/
public static SimpleExpression currentDatabase() {
return FunctionUtils.zeroArgFunc("current_database", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see current_query () → text
*
*/
public static SimpleExpression currentQuery() {
return FunctionUtils.zeroArgFunc("current_query", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see currentSchema () → name
*
*/
public static SimpleExpression currentSchema() {
return FunctionUtils.zeroArgFunc("current_schema", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextArrayType#LINEAR}
*
*
* @param includeImplicit in mose case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see current_schemas ( include_implicit boolean ) → name[]
*
*/
public static SimpleExpression currentSchema(Expression includeImplicit) {
return FunctionUtils.oneArgFunc("current_schema", includeImplicit, TextArrayType.LINEAR);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreInetType#INSTANCE}
*
*
* @see inet_client_addr () → inet
*
*/
public static SimpleExpression inetClientAddr() {
return FunctionUtils.zeroArgFunc("inet_client_addr", PostgreInetType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @see inet_client_port () → integer
*
*/
public static SimpleExpression inetClientPort() {
return FunctionUtils.zeroArgFunc("inet_client_port", IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreInetType#INSTANCE}
*
*
* @see inet_server_addr () → inet
*
*/
public static SimpleExpression inetServerAddr() {
return FunctionUtils.zeroArgFunc("inet_server_addr", PostgreInetType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @see inet_server_port () → integer
*
*/
public static SimpleExpression inetServerPort() {
return FunctionUtils.zeroArgFunc("inet_server_port", IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @see pg_backend_pid () → integer
*
*/
public static SimpleExpression pgBackendPid() {
return FunctionUtils.zeroArgFunc("pg_backend_pid", IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerArrayType#PRIMITIVE_LINEAR}
*
*
* @see pg_blocking_pids ( integer ) → integer[]
*
*/
public static SimpleExpression pgBlockingPids(Expression exp) {
return FunctionUtils.oneArgFunc("pg_blocking_pids", exp, IntegerArrayType.PRIMITIVE_LINEAR);
}
/**
*
* The {@link MappingType} of function return type: {@link OffsetDateTimeType#INSTANCE}
*
*
* @see pg_conf_load_time () → timestamp with time zone
*
*/
public static SimpleExpression pgConfLoadTime() {
return FunctionUtils.zeroArgFunc("pg_conf_load_time", OffsetDateTimeType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_current_logfile ( [ text ] ) → text
*
*/
public static SimpleExpression pgCurrentLogFile() {
return FunctionUtils.zeroArgFunc("pg_current_logfile", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_current_logfile ( [ text ] ) → text
*
*/
public static SimpleExpression pgCurrentLogFile(Expression exp) {
return FunctionUtils.oneArgFunc("pg_current_logfile", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see pg_my_temp_schema () → oid
*
*/
public static SimpleExpression pgMyTempSchema() {
return FunctionUtils.zeroArgFunc("pg_my_temp_schema", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_is_other_temp_schema ( oid ) → boolean
*
*/
public static SimplePredicate pgIsOtherTempSchema(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("pg_is_other_temp_schema", exp);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_jit_available () → boolean
*
*/
public static SimplePredicate pgJitAvailable() {
return FunctionUtils.zeroArgFuncPredicate("pg_jit_available");
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_listening_channels () → setof text
*
*/
public static _ColumnWithOrdinalityFunction pgListeningChannels() {
return DialectFunctionUtils.zeroArgColumnFunction("pg_listening_channels", null, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}
*
*
* @see pg_notification_queue_usage () → double precision
*
*/
public static SimpleExpression pgNotificationQueueUsage() {
return FunctionUtils.zeroArgFunc("pg_notification_queue_usage", DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link OffsetDateTimeType#INSTANCE}
*
*
* @see pg_postmaster_start_time () → timestamp with time zone
*
*/
public static SimpleExpression pgPostMasterStartTime() {
return FunctionUtils.zeroArgFunc("pg_postmaster_start_time", OffsetDateTimeType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerArrayType#PRIMITIVE_LINEAR}
*
*
* @see pg_safe_snapshot_blocking_pids ( integer ) → integer[]
*
*/
public static SimpleExpression pgSafeSnapshotBlockingPids(Expression exp) {
return FunctionUtils.oneArgFunc("pg_safe_snapshot_blocking_pids", exp, IntegerArrayType.PRIMITIVE_LINEAR);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @see pg_trigger_depth () → integer
*
*/
public static SimpleExpression pgTriggerDepth() {
return FunctionUtils.zeroArgFunc("pg_trigger_depth", IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see version () → text
*
*/
public static SimpleExpression version() {
return FunctionUtils.zeroArgFunc("version", TextType.INSTANCE);
}
/*-------------------below Access Privilege Inquiry Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_any_column_privilege ( [ user name or oid, ] table text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasAnyColumnPrivilege(Expression table, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_any_column_privilege", table, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_any_column_privilege ( [ user name or oid, ] table text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasAnyColumnPrivilege(Expression user, Expression table, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_any_column_privilege", user, table, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_column_privilege ( [ user name or oid, ] table text or oid, column text or smallint, privilege text ) → boolean
*
*/
public static SimplePredicate hasColumnPrivilege(Expression table, Expression column, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_column_privilege", table, column, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_column_privilege ( [ user name or oid, ] table text or oid, column text or smallint, privilege text ) → boolean
*
*/
public static SimplePredicate hasColumnPrivilege(Expression user, Expression table, Expression column, Expression privilege) {
return FunctionUtils.fourArgPredicateFunc("has_column_privilege", user, table, column, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_database_privilege ( [ user name or oid, ] database text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasDatabasePrivilege(Expression database, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_database_privilege", database, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_database_privilege ( [ user name or oid, ] database text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasDatabasePrivilege(Expression user, Expression database, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_database_privilege", user, database, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_foreign_data_wrapper_privilege ( [ user name or oid, ] fdw text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasForeignDataWrapperPrivilege(Expression fdw, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_foreign_data_wrapper_privilege", fdw, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_foreign_data_wrapper_privilege ( [ user name or oid, ] fdw text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasForeignDataWrapperPrivilege(Expression user, Expression fdw, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_foreign_data_wrapper_privilege", user, fdw, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_function_privilege ( [ user name or oid, ] function text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasFunctionPrivilege(Expression function, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_function_privilege", function, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_function_privilege ( [ user name or oid, ] function text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasFunctionPrivilege(Expression user, Expression function, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_function_privilege", user, function, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_language_privilege ( [ user name or oid, ] language text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasLanguagePrivilege(Expression language, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_language_privilege", language, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_language_privilege ( [ user name or oid, ] language text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasLanguagePrivilege(Expression user, Expression language, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_language_privilege", user, language, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_parameter_privilege ( [ user name or oid, ] parameter text, privilege text ) → boolean
*
*/
public static SimplePredicate hasParameterPrivilege(Expression parameter, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_parameter_privilege", parameter, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_parameter_privilege ( [ user name or oid, ] parameter text, privilege text ) → boolean
*
*/
public static SimplePredicate hasParameterPrivilege(Expression user, Expression parameter, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_parameter_privilege", user, parameter, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_schema_privilege ( [ user name or oid, ] schema text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasSchemaPrivilege(Expression schema, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_schema_privilege", schema, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_schema_privilege ( [ user name or oid, ] schema text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasSchemaPrivilege(Expression user, Expression schema, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_schema_privilege", user, schema, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_sequence_privilege ( [ user name or oid, ] sequence text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasSequencePrivilege(Expression sequence, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_sequence_privilege", sequence, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_sequence_privilege ( [ user name or oid, ] sequence text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasSequencePrivilege(Expression user, Expression sequence, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_sequence_privilege", user, sequence, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_server_privilege ( [ user name or oid, ] server text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasServerPrivilege(Expression server, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_server_privilege", server, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_server_privilege ( [ user name or oid, ] server text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasServerPrivilege(Expression user, Expression server, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_server_privilege", user, server, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_table_privilege ( [ user name or oid, ] table text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasTablePrivilege(Expression table, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_table_privilege", table, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_table_privilege ( [ user name or oid, ] table text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasTablePrivilege(Expression user, Expression table, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_table_privilege", user, table, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_tablespace_privilege ( [ user name or oid, ] tablespace text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasTablespacePrivilege(Expression tablespace, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_tablespace_privilege", tablespace, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_tablespace_privilege ( [ user name or oid, ] tablespace text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasTablespacePrivilege(Expression user, Expression tablespace, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_tablespace_privilege", user, tablespace, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_type_privilege ( [ user name or oid, ] type text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasTypePrivilege(Expression type, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("has_type_privilege", type, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see has_type_privilege ( [ user name or oid, ] type text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate hasTypePrivilege(Expression user, Expression type, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("has_type_privilege", user, type, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_has_role ( [ user name or oid, ] role text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate pgHasRole(Expression role, Expression privilege) {
return FunctionUtils.twoArgPredicateFunc("pg_has_role", role, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_has_role ( [ user name or oid, ] role text or oid, privilege text ) → boolean
*
*/
public static SimplePredicate pgHasRole(Expression user, Expression role, Expression privilege) {
return FunctionUtils.threeArgPredicateFunc("pg_has_role", user, role, privilege);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see row_security_active ( table text or oid ) → boolean
*
*/
public static SimplePredicate rowSecurityActive(Expression table) {
return FunctionUtils.oneArgPredicateFunc("row_security_active", table);
}
/*-------------------below aclitem Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link PostgreAclItemArrayType#LINEAR}
*
*
* @see acldefault ( type "char", ownerId oid ) → aclitem[]
*
*/
public static SimpleExpression aclDefault(Expression type, Expression ownerId) {
return FunctionUtils.twoArgFunc("acldefault", type, ownerId, PostgreAclItemArrayType.LINEAR);
}
/**
*
* The {@link MappingType} of function return type:
* - grantor : {@link LongType#INSTANCE}
* - grantee : {@link LongType#INSTANCE}
* - privilege_type : {@link TextType#INSTANCE}
* - is_grantable : {@link BooleanType#INSTANCE}
*
*
*
* @see aclexplode ( aclitem[] ) → setof record ( grantor oid, grantee oid, privilege_type text, is_grantable boolean )
*
*/
public static _TabularWithOrdinalityFunction aclExplode(Expression exp) {
final List fieldList = _Collections.arrayList(4);
fieldList.add(ArmySelections.forName("grantor", LongType.INSTANCE));
fieldList.add(ArmySelections.forName("grantee", LongType.INSTANCE));
fieldList.add(ArmySelections.forName("privilege_type", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("is_grantable", BooleanType.INSTANCE));
return DialectFunctionUtils.oneArgTabularFunc("aclexplode", exp, fieldList);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreAclItemType#TEXT}
*
*
* @param isGrantable in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see makeaclitem ( grantee oid, grantor oid, privileges text, is_grantable boolean ) → aclitem
*
*/
public static SimpleExpression makeAclItem(Expression grantee, Expression grantor, Expression privileges, Expression isGrantable) {
return FunctionUtils.fourArgFunc("makeaclitem", grantee, grantor, privileges, isGrantable, PostgreAclItemType.TEXT);
}
/*-------------------below Schema Visibility Inquiry Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_collation_is_visible ( collation oid ) → boolean
*
*/
public static SimplePredicate pgCollationIsVisible(Expression collation) {
return FunctionUtils.oneArgPredicateFunc("pg_collation_is_visible", collation);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_conversion_is_visible ( conversion oid ) → boolean
*
*/
public static SimplePredicate pgConversionIsVisible(Expression conversion) {
return FunctionUtils.oneArgPredicateFunc("pg_conversion_is_visible", conversion);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_function_is_visible ( function oid ) → boolean
*
*/
public static SimplePredicate pgFunctionIsVisible(Expression function) {
return FunctionUtils.oneArgPredicateFunc("pg_function_is_visible", function);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_opclass_is_visible ( opclass oid ) → boolean
*
*/
public static SimplePredicate pgOpClassIsVisible(Expression opclass) {
return FunctionUtils.oneArgPredicateFunc("pg_opclass_is_visible", opclass);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_operator_is_visible ( operator oid ) → boolean
*
*/
public static SimplePredicate pgOperatorIsVisible(Expression operator) {
return FunctionUtils.oneArgPredicateFunc("pg_operator_is_visible", operator);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_opfamily_is_visible ( opclass oid ) → boolean
*
*/
public static SimplePredicate pgOpFamilyIsVisible(Expression opClass) {
return FunctionUtils.oneArgPredicateFunc("pg_opfamily_is_visible", opClass);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_statistics_obj_is_visible ( stat oid ) → boolean
*
*/
public static SimplePredicate pgStatisticsObjIsVisible(Expression stat) {
return FunctionUtils.oneArgPredicateFunc("pg_statistics_obj_is_visible", stat);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_table_is_visible ( table oid ) → boolean
*
*/
public static SimplePredicate pgTableIsVisible(Expression table) {
return FunctionUtils.oneArgPredicateFunc("pg_table_is_visible", table);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_ts_config_is_visible ( config oid ) → boolean
*
*/
public static SimplePredicate pgTsConfigIsVisible(Expression config) {
return FunctionUtils.oneArgPredicateFunc("pg_ts_config_is_visible", config);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_ts_dict_is_visible ( dict oid ) → boolean
*
*/
public static SimplePredicate pgTsDictIsVisible(Expression dict) {
return FunctionUtils.oneArgPredicateFunc("pg_ts_dict_is_visible", dict);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_ts_parser_is_visible ( parser oid ) → boolean
*
*/
public static SimplePredicate pgTsParserIsVisible(Expression parser) {
return FunctionUtils.oneArgPredicateFunc("pg_ts_parser_is_visible", parser);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_ts_template_is_visible ( template oid ) → boolean
*
*/
public static SimplePredicate pgTsTemplateIsVisible(Expression template) {
return FunctionUtils.oneArgPredicateFunc("pg_ts_template_is_visible", template);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_type_is_visible ( type oid ) → boolean
*
*/
public static SimplePredicate pgTypeIsVisible(Expression type) {
return FunctionUtils.oneArgPredicateFunc("pg_type_is_visible", type);
}
/*-------------------below System Catalog Information Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see format_type ( type oid, typemod integer ) → text
*
*/
public static SimpleExpression formatType(Expression type, Expression typeMode) {
return FunctionUtils.twoArgFunc("format_type", type, typeMode, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @see pg_char_to_encoding ( encoding name ) → integer
*
*/
public static SimpleExpression pgCharToEncoding(Expression encoding) {
return FunctionUtils.oneArgFunc("pg_char_to_encoding", encoding, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_encoding_to_char ( encoding integer ) → name
*
*/
public static SimpleExpression pgEncodingToChar(Expression encoding) {
return FunctionUtils.oneArgFunc("pg_encoding_to_char", encoding, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
* - fktable : {@link TextType#INSTANCE}
* - fkcols : {@link TextArrayType#LINEAR}
* - pktable : {@link TextType#INSTANCE}
* - pkcols : {@link TextArrayType#LINEAR}
* - is_array : {@link BooleanType#INSTANCE}
* - is_opt : {@link BooleanType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_get_catalog_foreign_keys () → setof record ( fktable regclass, fkcols text[], pktable regclass, pkcols text[], is_array boolean, is_opt boolean )
*
*/
public static _TabularWithOrdinalityFunction pgGetCatalogForeignKeys() {
final List fieldList = _Collections.arrayList(6);
fieldList.add(ArmySelections.forName("fktable", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("fkcols", TextArrayType.LINEAR));
fieldList.add(ArmySelections.forName("pktable", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("pkcols", TextArrayType.LINEAR));
fieldList.add(ArmySelections.forName("is_array", BooleanType.INSTANCE));
fieldList.add(ArmySelections.forName("is_opt", BooleanType.INSTANCE));
return DialectFunctionUtils.zeroArgTabularFunc("pg_get_catalog_foreign_keys", fieldList);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_constraintdef ( constraint oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetConstraintDef(Expression constraint) {
return FunctionUtils.oneArgFunc("pg_get_constraintdef", constraint, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @param pretty in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see pg_get_constraintdef ( constraint oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetConstraintDef(Expression constraint, Expression pretty) {
return FunctionUtils.twoArgFunc("pg_get_constraintdef", constraint, pretty, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @param pretty in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see pg_get_expr ( expr pg_node_tree, relation oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetExpr(Expression expr, Expression relation, Expression pretty) {
// TODO hwo to pg_node_tree ?
return FunctionUtils.threeArgFunc("pg_get_expr", expr, relation, pretty, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_functiondef ( func oid ) → text
*
*/
public static SimpleExpression pgGetFunctionDef(Expression func) {
return FunctionUtils.oneArgFunc("pg_get_functiondef", func, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_function_arguments ( func oid ) → text
*
*/
public static SimpleExpression pgGetFunctionArguments(Expression func) {
return FunctionUtils.oneArgFunc("pg_get_function_arguments", func, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_function_identity_arguments ( func oid ) → text
*
*/
public static SimpleExpression pgGetFunctionIdentityArguments(Expression func) {
return FunctionUtils.oneArgFunc("pg_get_function_identity_arguments", func, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_function_result ( func oid ) → text
*
*/
public static SimpleExpression pgGetFunctionResult(Expression func) {
return FunctionUtils.oneArgFunc("pg_get_function_result", func, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_indexdef ( index oid [, column integer, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetIndexDef(Expression func) {
return FunctionUtils.oneArgFunc("pg_get_indexdef", func, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @param pretty in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see pg_get_indexdef ( index oid [, column integer, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetIndexDef(Expression func, Expression column, Expression pretty) {
return FunctionUtils.threeArgFunc("pg_get_indexdef", func, column, pretty, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
* - word : {@link TextType#INSTANCE}
* - catcode : {@link CharacterType#INSTANCE}
* - barelabel : {@link BooleanType#INSTANCE}
* - catdesc : {@link TextType#INSTANCE}
* - baredesc : {@link TextType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_get_keywords () → setof record ( word text, catcode "char", barelabel boolean, catdesc text, baredesc text )
*
*/
public static _TabularWithOrdinalityFunction pgGetKeywords() {
final List fieldList = _Collections.arrayList(5);
fieldList.add(ArmySelections.forName("word", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("catcode", CharacterType.INSTANCE));
fieldList.add(ArmySelections.forName("barelabel", BooleanType.INSTANCE));
fieldList.add(ArmySelections.forName("catdesc", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("baredesc", TextType.INSTANCE));
return DialectFunctionUtils.zeroArgTabularFunc("pg_get_keywords", fieldList);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_ruledef ( rule oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetRuleDef(Expression rule) {
return FunctionUtils.oneArgFunc("pg_get_ruledef", rule, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @param pretty in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see pg_get_ruledef ( rule oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetRuleDef(Expression rule, Expression pretty) {
return FunctionUtils.twoArgFunc("pg_get_ruledef", rule, pretty, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_serial_sequence ( table text, column text ) → text
*
*/
public static SimpleExpression pgGetSerialSequence(Expression table, Expression column) {
return FunctionUtils.twoArgFunc("pg_get_serial_sequence", table, column, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_statisticsobjdef ( statobj oid ) → text
*
*/
public static SimpleExpression pgGetStatisticsObjDef(Expression statObj) {
return FunctionUtils.oneArgFunc("pg_get_statisticsobjdef", statObj, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_triggerdef ( trigger oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetTriggerDef(Expression trigger) {
return FunctionUtils.oneArgFunc("pg_get_triggerdef", trigger, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @param pretty in most case {@link SQLs#TRUE} or {@link SQLs#FALSE}
* @see pg_get_triggerdef ( trigger oid [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetTriggerDef(Expression trigger, Expression pretty) {
return FunctionUtils.twoArgFunc("pg_get_triggerdef", trigger, pretty, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_userbyid ( role oid ) → name
*
*/
public static SimpleExpression pgGetUserById(Expression role) {
return FunctionUtils.oneArgFunc("pg_get_userbyid", role, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_viewdef ( view oid [, pretty boolean ] ) → text
* pg_get_viewdef ( view oid, wrap_column integer ) → text
* pg_get_viewdef ( view text [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetViewDef(Expression view) {
return FunctionUtils.oneArgFunc("pg_get_viewdef", view, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_get_viewdef ( view oid [, pretty boolean ] ) → text
* pg_get_viewdef ( view oid, wrap_column integer ) → text
* pg_get_viewdef ( view text [, pretty boolean ] ) → text
*
*/
public static SimpleExpression pgGetViewDef(Expression view, Expression exp) {
return FunctionUtils.twoArgFunc("pg_get_viewdef", view, exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_index_column_has_property ( index regclass, column integer, property text ) → boolean
*
*/
public static SimplePredicate pgIndexColumnHasProperty(Expression index, Expression column, Expression property) {
return FunctionUtils.threeArgPredicateFunc("pg_index_column_has_property", index, column, property);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_index_has_property ( index regclass, property text ) → boolean
*
*/
public static SimplePredicate pgIndexHasProperty(Expression index, Expression property) {
return FunctionUtils.twoArgPredicateFunc("pg_index_has_property", index, property);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_indexam_has_property ( am oid, property text ) → boolean
*
*/
public static SimplePredicate pgIndexAmHasProperty(Expression am, Expression property) {
return FunctionUtils.twoArgPredicateFunc("pg_indexam_has_property", am, property);
}
/**
*
* The {@link MappingType} of function return type:
* - option_name : {@link TextType#INSTANCE}
* - option_value : {@link TextType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_options_to_table ( options_array text[] ) → setof record ( option_name text, option_value text )
*
*/
public static _TabularWithOrdinalityFunction pgOptionsToTable(Expression optionsArray) {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("option_name", TextType.INSTANCE),
ArmySelections.forName("option_value", TextType.INSTANCE)
);
return DialectFunctionUtils.oneArgTabularFunc("pg_options_to_table", optionsArray, fieldList);
}
/**
*
* The {@link MappingType} of function return type: {@link TextArrayType#LINEAR}
*
*
* @see pg_settings_get_flags ( guc text ) → text[]
*
*/
public static SimpleExpression pgSettingsGetFlags(Expression guc) {
return FunctionUtils.oneArgFunc("pg_settings_get_flags", guc, TextArrayType.LINEAR);
}
/**
*
* The {@link MappingType} of function return type:
* - "Anonymous field" ( you must use as clause definite filed name) : {@link LongType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_tablespace_databases ( tablespace oid ) → setof oid
*
*/
public static _ColumnWithOrdinalityFunction pgTablespaceDatabases(Expression tablespace) {
return DialectFunctionUtils.oneArgColumnFunction("pg_tablespace_databases", tablespace, null, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_tablespace_location ( tablespace oid ) → text
*
*/
public static SimpleExpression pgTablespaceLocation(Expression tablespace) {
return FunctionUtils.oneArgFunc("pg_tablespace_location", tablespace, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_typeof ( "any" ) → regtype
*
*/
public static SimpleExpression pgTypeOf(Expression any) {
return FunctionUtils.oneArgFunc("pg_typeof", any, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see COLLATION FOR ( "any" ) → text
*
*/
public static SimpleExpression collationSpaceFor(Expression any) {
return FunctionUtils.oneArgFunc("COLLATION FOR", any, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regclass ( text ) → regclass
*
*/
public static SimpleExpression toRegClass(Expression exp) {
return FunctionUtils.oneArgFunc("to_regclass", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regcollation ( text ) → regcollation
*
*/
public static SimpleExpression toRegCollation(Expression exp) {
return FunctionUtils.oneArgFunc("to_regcollation", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regnamespace ( text ) → regnamespace
*
*/
public static SimpleExpression toRegNamespace(Expression exp) {
return FunctionUtils.oneArgFunc("to_regnamespace", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see o_regoper ( text ) → regoper
*
*/
public static SimpleExpression toRegOper(Expression exp) {
return FunctionUtils.oneArgFunc("to_regoper", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regoperator ( text ) → regoperator
*
*/
public static SimpleExpression toRegOperator(Expression exp) {
return FunctionUtils.oneArgFunc("to_regoperator", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regproc ( text ) → regproc
*
*/
public static SimpleExpression toRegProc(Expression exp) {
return FunctionUtils.oneArgFunc("to_regproc", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regprocedure ( text ) → regprocedure
*
*/
public static SimpleExpression toRegProcedure(Expression exp) {
return FunctionUtils.oneArgFunc("to_regprocedure", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regrole ( text ) → regrole
*
*/
public static SimpleExpression toRegRole(Expression exp) {
return FunctionUtils.oneArgFunc("to_regrole", exp, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see to_regtype ( text ) → regtype
*
*/
public static SimpleExpression toRegType(Expression exp) {
return FunctionUtils.oneArgFunc("to_regtype", exp, TextType.INSTANCE);
}
/*-------------------below Object Information and Addressing Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_describe_object ( classid oid, objid oid, objsubid integer ) → text
*
*/
public static SimpleExpression pgDescribeObject(Expression classId, Expression objId, Expression objSubId) {
return FunctionUtils.threeArgFunc("pg_describe_object", classId, objId, objSubId, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
*
* - type : {@link TextType#INSTANCE}
* - schema : {@link TextType#INSTANCE}
* - name : {@link TextType#INSTANCE}
* - identity : {@link TextType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_identify_object ( classid oid, objid oid, objsubid integer ) → record ( type text, schema text, name text, identity text )
*
*/
public static _TabularWithOrdinalityFunction pgIdentifyObject(Expression classId, Expression objId, Expression objSubId) {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("type", TextType.INSTANCE),
ArmySelections.forName("schema", TextType.INSTANCE),
ArmySelections.forName("name", TextType.INSTANCE),
ArmySelections.forName("identity", TextType.INSTANCE)
);
return DialectFunctionUtils.threeArgTabularFunc("pg_identify_object", classId, objId, objSubId, fieldList);
}
/**
*
* The {@link MappingType} of function return type:
*
* - type : {@link TextType#INSTANCE}
* - object_names : {@link TextArrayType#LINEAR}
* - object_args : {@link TextArrayType#LINEAR}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_identify_object_as_address ( classid oid, objid oid, objsubid integer ) → record ( type text, object_names text[], object_args text[] )
*
*/
public static _TabularWithOrdinalityFunction pgIdentifyObjectAsAddress(Expression classId, Expression objId, Expression objSubId) {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("type", TextType.INSTANCE),
ArmySelections.forName("object_names", TextArrayType.LINEAR),
ArmySelections.forName("object_args", TextArrayType.LINEAR)
);
return DialectFunctionUtils.threeArgTabularFunc("pg_identify_object_as_address", classId, objId, objSubId, fieldList);
}
/**
*
* The {@link MappingType} of function return type:
*
* - classid : {@link LongType#INSTANCE}
* - objid : {@link LongType#INSTANCE}
* - objsubid : {@link IntegerType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_get_object_address ( type text, object_names text[], object_args text[] ) → record ( classid oid, objid oid, objsubid integer )
*
*/
public static _TabularWithOrdinalityFunction pgGetObjectAddress(Expression type, Expression objectNames, Expression objectArgs) {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("classid", LongType.INSTANCE),
ArmySelections.forName("objid", LongType.INSTANCE),
ArmySelections.forName("objsubid", IntegerType.INSTANCE)
);
return DialectFunctionUtils.threeArgTabularFunc("pg_get_object_address", type, objectNames, objectArgs, fieldList);
}
/*-------------------below private method -------------------*/
/**
* @see #tsDebug(Expression)
* @see #tsDebug(Expression, Expression)
*/
private static _TabularWithOrdinalityFunction _tsDebug(final @Nullable Expression config, final Expression document) {
final List fieldList = _Collections.arrayList(6);
fieldList.add(ArmySelections.forName("alias", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("description", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("token", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("dictionaries", TextArrayType.LINEAR));
fieldList.add(ArmySelections.forName("dictionary", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("lexemes", TextArrayType.LINEAR));
final String name = "ts_debug";
final _TabularWithOrdinalityFunction func;
if (config == null) {
func = DialectFunctionUtils.oneArgTabularFunc(name, document, fieldList);
} else {
func = DialectFunctionUtils.twoArgTabularFunc(name, config, document, fieldList);
}
return func;
}
/**
*
* The {@link MappingType} of function returned fields type:
* - word {@link TextType}
* - ndoc {@link IntegerType}
* - nentry {@link IntegerType}
* - ordinality (this is optional) {@link LongType},see {@link _WithOrdinalityClause#withOrdinality()}
*
*
*
* @see #tsStat(Expression)
* @see #tsStat(Expression, Expression)
* @see ts_stat ( sqlquery text [, weights text ] ) → setof record ( word text, ndoc integer, nentry integer )
* Executes the sqlquery, which must return a single tsvector column, and returns statistics about each distinct lexeme contained in the data.
* ts_stat('SELECT vector FROM apod') → (foo,10,15)
*
*/
private static _TabularWithOrdinalityFunction _tsStat(final Expression sqlQuery, final @Nullable Expression weights) {
final List fieldList = _Collections.arrayList(3);
fieldList.add(ArmySelections.forName("word", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("ndoc", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("nentry", IntegerType.INSTANCE));
final String name = "ts_stat";
_TabularWithOrdinalityFunction func;
if (weights == null) {
func = DialectFunctionUtils.oneArgTabularFunc(name, sqlQuery, fieldList);
} else {
func = DialectFunctionUtils.twoArgTabularFunc(name, sqlQuery, weights, fieldList);
}
return func;
}
/**
* @see #arrayFill(Expression, Expression)
* @see #arrayFill(Expression, Expression, Expression)
*/
private static SimpleExpression _arrayFill(final Expression anyElement, final Expression dimensions,
final @Nullable Expression bounds) {
final String name = "array_fill";
final SimpleExpression func;
if (bounds == null) {
func = FunctionUtils.twoArgFunc(name, anyElement, dimensions,
_returnType(anyElement, MappingType::arrayTypeOfThis)
);
} else {
func = FunctionUtils.threeArgFunc(name, anyElement, dimensions, bounds,
_returnType(anyElement, MappingType::arrayTypeOfThis)
);
}
return func;
}
}