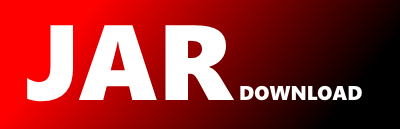
io.army.criteria.impl.PostgreMiscellaneousFunctions Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.criteria.*;
import io.army.mapping.*;
import io.army.mapping.array.BooleanArrayType;
import io.army.mapping.array.StringArrayType;
import io.army.mapping.array.TextArrayType;
import io.army.mapping.postgre.PostgreCidrType;
import io.army.mapping.postgre.PostgreInetType;
import io.army.mapping.postgre.PostgrePgLsnType;
import io.army.util.ArrayUtils;
import io.army.util._Collections;
import java.util.List;
import java.util.function.BiFunction;
/**
*
* package class.
*
* @since 1.0
*/
abstract class PostgreMiscellaneousFunctions extends PostgreGeometricFunctions {
/**
* package constructor
*/
PostgreMiscellaneousFunctions() {
}
/*-------------------below Comparison Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see Comparison Functions
*/
public static SimpleExpression numNonNulls(Expression first, Expression... rest) {
return FunctionUtils.multiArgFunc("num_nonnulls", IntegerType.INSTANCE, first, rest);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see Comparison Functions
*/
public static SimpleExpression numNulls(Expression first, Expression... rest) {
return FunctionUtils.multiArgFunc("num_nulls", IntegerType.INSTANCE, first, rest);
}
/*-------------------below Mathematical Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see cbrt(double precision)
*/
public static SimpleExpression cbrt(Expression exp) {
return FunctionUtils.oneArgFunc("cbrt", exp, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of y
*
*
* @see div ( y numeric, x numeric )
*/
public static SimpleExpression div(Expression y, Expression x) {
return FunctionUtils.twoArgFunc("div", y, x, y.typeMeta());
}
/**
*
* The {@link MappingType} of function return type: {@link BigDecimalType}
*
*
* @see factorial ( bigint ) → numeric
*/
public static SimpleExpression factorial(Expression exp) {
return FunctionUtils.oneArgFunc("factorial", exp, BigDecimalType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link MappingType} of exp
*
*
* @see floor ( numeric ) → numeric,floor ( double precision ) → double precision
*/
public static SimpleExpression floor(final Expression exp) {
return FunctionUtils.oneArgFunc("floor", exp, exp.typeMeta());
}
/**
*
* The {@link MappingType} of function return type: {@link MappingType} of exp1
*
*
* @see gcd ( numeric_type, numeric_type ) → numeric_type
*/
public static SimpleExpression gcd(Expression exp1, Expression exp2) {
return FunctionUtils.twoArgFunc("gcd", exp1, exp2, exp1.typeMeta());
}
/**
*
* The {@link MappingType} of function return type: {@link MappingType} of exp1
*
*
* @see lcm ( numeric_type, numeric_type ) → numeric_type
*/
public static SimpleExpression lcm(Expression exp1, Expression exp2) {
return FunctionUtils.twoArgFunc("lcm", exp1, exp2, exp1.typeMeta());
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see min_scale ( numeric ) → integer
*/
public static SimpleExpression minScale(final Expression exp) {
return FunctionUtils.oneArgFunc("min_scale", exp, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
*
* - If the {@link MappingType} of exp is float number type,then {@link DoubleType}
* - Else {@link BigDecimalType}
*
*
*
* @see power ( a numeric, b numeric ) → numeric,power ( a double precision, b double precision ) → double precision
*/
public static SimpleExpression power(final Expression x, final Expression y) {
return FunctionUtils.twoArgFunc("power", x, y, _returnType(x, Functions::_numberOrDecimal));
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see scale ( numeric ) → integer
*/
public static SimpleExpression scale(final Expression x, final Expression y) {
return FunctionUtils.twoArgFunc("scale", x, y, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BigDecimalType}
*
*
* @see trim_scale ( numeric ) → numeric
*/
public static SimpleExpression trimScale(final Expression exp) {
return FunctionUtils.oneArgFunc("trim_scale", exp, BigDecimalType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:The {@link MappingType} of exp
*
*
* @see trunc ( numeric ) → numeric,trunc ( double precision ) → double precision
* @see trunc ( macaddr ) → macaddr
* trunc ( macaddr8 ) → macaddr8
*
*/
public static SimpleExpression trunc(final Expression exp) {
return FunctionUtils.oneArgFunc("trunc", exp, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: {@link BigDecimalType}
*
*
* @see trunc ( v numeric, s integer ) → numeric
*/
public static SimpleExpression trunc(final Expression v, final Expression s) {
return FunctionUtils.twoArgFunc("trunc", v, s, BigDecimalType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see width_bucket ( operand numeric, low numeric, high numeric, count integer ) → integer,width_bucket ( operand double precision, low double precision, high double precision, count integer ) → integer
*/
public static SimpleExpression widthBucket(final Expression operand, final Expression low, Expression high, Expression count) {
return FunctionUtils.fourArgFunc("width_bucket", operand, low, high, count, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see width_bucket ( operand anycompatible, thresholds anycompatiblearray ) → integer
*/
public static SimpleExpression widthBucket(final Expression operand, final Expression thresholds) {
return FunctionUtils.twoArgFunc("width_bucket", operand, thresholds, IntegerType.INSTANCE);
}
/*-------------------below Random Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see random ( ) → double precision
*/
public static SimpleExpression random() {
return FunctionUtils.zeroArgFunc("random", DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see setseed ( double precision ) → void
*/
public static SimpleExpression setSeed(Expression exp) {
return FunctionUtils.oneArgFunc("setseed", exp, StringType.INSTANCE);
}
/*-------------------below Trigonometric Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see acosd ( double precision ) → double precision
*/
public static SimpleExpression acosd(final Expression expr) {
return FunctionUtils.oneArgFunc("acosd", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see asind ( double precision ) → double precision
*/
public static SimpleExpression asind(final Expression expr) {
return FunctionUtils.oneArgFunc("asind", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see atand ( double precision ) → double precision
*/
public static SimpleExpression atand(final Expression expr) {
return FunctionUtils.oneArgFunc("atand", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see atan2 ( y double precision, x double precision ) → double precision
*/
public static SimpleExpression atan2(Expression y, Expression x) {
return FunctionUtils.twoArgFunc("atan2", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see atan2d ( y double precision, x double precision ) → double precision
*/
public static SimpleExpression atan2d(Expression y, Expression x) {
return FunctionUtils.twoArgFunc("atan2d", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see cosd ( double precision ) → double precision
*/
public static SimpleExpression cosd(final Expression expr) {
return FunctionUtils.oneArgFunc("cosd", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see cotd ( double precision ) → double precision
*/
public static SimpleExpression cotd(final Expression expr) {
return FunctionUtils.oneArgFunc("cotd", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see sind ( double precision ) → double precision
*/
public static SimpleExpression sind(final Expression expr) {
return FunctionUtils.oneArgFunc("sind", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see tand ( double precision ) → double precision
*/
public static SimpleExpression tand(final Expression expr) {
return FunctionUtils.oneArgFunc("tand", expr, DoubleType.INSTANCE);
}
/*-------------------below Hyperbolic Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see sinh ( double precision ) → double precision
*/
public static SimpleExpression sinh(final Expression expr) {
return FunctionUtils.oneArgFunc("sinh", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see cosh ( double precision ) → double precision
*/
public static SimpleExpression cosh(final Expression expr) {
return FunctionUtils.oneArgFunc("cosh", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see tanh ( double precision ) → double precision
*/
public static SimpleExpression tanh(final Expression expr) {
return FunctionUtils.oneArgFunc("tanh", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see asinh ( double precision ) → double precision
*/
public static SimpleExpression asinh(final Expression expr) {
return FunctionUtils.oneArgFunc("asinh", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see acosh ( double precision ) → double precision
*/
public static SimpleExpression acosh(final Expression expr) {
return FunctionUtils.oneArgFunc("acosh", expr, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType}
*
*
* @see atanh ( double precision ) → double precision
*/
public static SimpleExpression atanh(final Expression expr) {
return FunctionUtils.oneArgFunc("atanh", expr, DoubleType.INSTANCE);
}
/*-------------------below Data Type Formatting Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see to_char ( timestamp, text ) → text
* to_char ( timestamp with time zone, text ) → text
* to_char ( interval, text ) → text
* to_char ( numeric_type, text ) → text
*
*/
public static SimpleExpression toChar(Expression exp, Expression format) {
return FunctionUtils.twoArgFunc("to_char", exp, format, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LocalDateType}
*
*
* @see to_date ( text, text ) → date
*/
public static SimpleExpression toDate(Expression exp, Expression format) {
return FunctionUtils.twoArgFunc("to_date", exp, format, LocalDateType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BigDecimalType}
*
*
* @see to_number ( text, text ) → numeric
*/
public static SimpleExpression toNumber(Expression exp, Expression format) {
return FunctionUtils.twoArgFunc("to_number", exp, format, BigDecimalType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link OffsetDateTimeType}
*
*
* @see to_timestamp ( text, text ) → timestamp with time zone
*/
public static SimpleExpression toTimestamp(Expression exp, Expression format) {
return FunctionUtils.twoArgFunc("to_timestamp", exp, format, OffsetDateTimeType.INSTANCE);
}
/*-------------------below Date/Time Functions and Operators-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see age ( timestamp ) → interval
*/
public static SimpleExpression age(Expression timestamp) {
return FunctionUtils.oneArgFunc("age", timestamp, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see age ( timestamp, timestamp ) → interval
*/
public static SimpleExpression age(Expression timestamp1, Expression timestamp2) {
return FunctionUtils.twoArgFunc("age", timestamp1, timestamp2, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}
*
*
* @see isfinite ( date ) → boolean
* isfinite ( timestamp ) → boolean
* isfinite ( interval ) → boolean
*
*/
public static IPredicate isFinite(Expression exp) {
return FunctionUtils.oneArgPredicateFunc("isfinite", exp);
}
/*-------------------below Delaying Execution function -------------------*/
/*-------------------below Enum Support Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see enum_first ( anyenum ) → anyenum
*/
public static SimpleExpression enumFirst(Expression anyEnum) {
return FunctionUtils.oneArgFunc("enum_first", anyEnum, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see enum_first ( anyenum ) → anyenum
*/
public static SimpleExpression enumFirst(Expression anyEnum, MappingType returnType) {
final String name = "enum_first";
if (!Enum.class.isAssignableFrom(returnType.javaType())) {
throw CriteriaUtils.errorCustomReturnType(name, returnType);
}
return FunctionUtils.oneArgFunc(name, anyEnum, returnType);
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see enum_last ( anyenum ) → anyenum
*/
public static SimpleExpression enumLast(Expression anyEnum) {
return FunctionUtils.oneArgFunc("enum_last", anyEnum, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see enum_last ( anyenum ) → anyenum
*/
public static SimpleExpression enumLast(Expression anyEnum, MappingType returnType) {
final String name = "enum_last";
if (!Enum.class.isAssignableFrom(returnType.javaType())) {
throw CriteriaUtils.errorCustomReturnType(name, returnType);
}
return FunctionUtils.oneArgFunc(name, anyEnum, returnType);
}
/**
*
* The {@link MappingType} of function return type: {@link StringArrayType}
*
*
* @see enum_range ( anyenum ) → anyarray
*/
public static SimpleExpression enumRange(Expression anyEnum) {
return FunctionUtils.oneArgFunc("enum_range", anyEnum, StringArrayType.from(String[].class));
}
/**
*
* The {@link MappingType} of function return type: {@link StringArrayType}
*
*
* @see enum_range ( anyenum ) → anyarray
*/
public static SimpleExpression enumRange(Expression anyEnum, MappingType returnType) {
final String name = "enum_range";
final Class> javaType;
javaType = returnType.javaType();
if (!javaType.isArray() || !javaType.getComponentType().isEnum()) {
throw CriteriaUtils.errorCustomReturnType(name, returnType);
}
return FunctionUtils.oneArgFunc(name, anyEnum, returnType);
}
/**
*
* The {@link MappingType} of function return type: {@link StringArrayType}
*
*
* @see enum_range ( anyenum, anyenum ) → anyarray
*/
public static SimpleExpression enumRange(Expression leftEnum, Expression rightEnum) {
return FunctionUtils.twoArgFunc("enum_range", leftEnum, rightEnum, StringArrayType.from(String[].class));
}
public static SimpleExpression enumRange(Expression leftEnum, Expression rightEnum, MappingType returnType) {
final String name = "enum_range";
final Class> javaType;
javaType = returnType.javaType();
if (!javaType.isArray() || !javaType.getComponentType().isEnum()) {
throw CriteriaUtils.errorCustomReturnType(name, returnType);
}
return FunctionUtils.twoArgFunc(name, leftEnum, rightEnum, returnType);
}
/*-------------------below IP Address Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see abbrev ( inet ) → text
* abbrev ( cidr ) → text
*
*/
public static SimpleExpression abbrev(Expression exp) {
return FunctionUtils.oneArgFunc("abbrev", exp, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreInetType}
*
*
* @see broadcast ( inet ) → inet
* Computes the broadcast address for the address's network.
*
*/
public static SimpleExpression broadcast(Expression inet) {
return FunctionUtils.oneArgFunc("broadcast", inet, PostgreInetType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see family ( inet ) → integer
* Returns the address's family: 4 for IPv4, 6 for IPv6.
* family(inet '::1') → 6
*
*/
public static SimpleExpression family(Expression inet) {
return FunctionUtils.oneArgFunc("family", inet, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see host ( inet ) → text
* Returns the IP address as text, ignoring the netmask.
* host(inet '192.168.1.0/24') → 192.168.1.0
*
*/
public static SimpleExpression host(Expression inet) {
return FunctionUtils.oneArgFunc("host", inet, StringType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreInetType}
*
*
* @see hostmask ( inet ) → inet
* Computes the host mask for the address's network.
* hostmask(inet '192.168.23.20/30') → 0.0.0.3
*
*/
public static SimpleExpression hostmask(Expression inet) {
return FunctionUtils.oneArgFunc("hostmask", inet, PostgreInetType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreCidrType}
*
*
* @see inet_merge ( inet, inet ) → cidr
* Computes the smallest network that includes both of the given networks.
* inet_merge(inet '192.168.1.5/24', inet '192.168.2.5/24') → 192.168.0.0/22
*
*/
public static SimpleExpression inetMerge(Expression exp1, Expression exp2) {
return FunctionUtils.twoArgFunc("inet_merge", exp1, exp2, PostgreCidrType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType}
*
*
* @see inet_same_family ( inet, inet ) → boolean
* Tests whether the addresses belong to the same IP family.
* inet_same_family(inet '192.168.1.5/24', inet '::1') → f
*
*/
public static SimplePredicate inetSameFamily(Expression exp1, Expression exp2) {
return FunctionUtils.twoArgPredicateFunc("inet_same_family", exp1, exp2);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType}
*
*
* @see masklen ( inet ) → integer
* Returns the netmask length in bits.
* masklen(inet '192.168.1.5/24') → 24
*
*/
public static SimpleExpression maskLen(Expression inet) {
return FunctionUtils.oneArgFunc("masklen", inet, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreInetType}
*
*
* @see netmask ( inet ) → inet
* Computes the network mask for the address's network.
* netmask(inet '192.168.1.5/24') → 255.255.255.0
*
*/
public static SimpleExpression netmask(Expression inet) {
return FunctionUtils.oneArgFunc("netmask", inet, PostgreInetType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link PostgreCidrType}
*
*
* @see network ( inet ) → cidr
* Returns the network part of the address, zeroing out whatever is to the right of the netmask. (This is equivalent to casting the value to cidr.)
* network(inet '192.168.1.5/24') → 192.168.1.0/24
*
*/
public static SimpleExpression network(Expression inet) {
return FunctionUtils.oneArgFunc("network", inet, PostgreCidrType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
* - If exp1 type is {@link PostgreInetType},then {@link PostgreInetType}
* - If exp1 type is {@link PostgreCidrType},then {@link PostgreCidrType}
* - Else The {@link MappingType} of exp1
*
*
*
* @param funcRef the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of funcRef always is {@link IntegerType#INSTANCE}.
* @param value non-null,it will be passed to funcRef as the second argument of funcRef.
* @see #setMaskLen(Expression, Expression)
* @see set_masklen ( inet, integer ) → inet
* Sets the netmask length for an inet value. The address part does not change.
* set_masklen(inet '192.168.1.5/24', 16) → 192.168.1.5/16
*
*/
public static SimpleExpression setMaskLen(Expression exp1, BiFunction funcRef, T value) {
return setMaskLen(exp1, funcRef.apply(IntegerType.INSTANCE, value));
}
/**
*
* The {@link MappingType} of function return type:
* - If exp1 type is {@link PostgreInetType},then {@link PostgreInetType}
* - If exp1 type is {@link PostgreCidrType},then {@link PostgreCidrType}
* - Else The {@link MappingType} of exp1
*
*
*
* @see set_masklen ( inet, integer ) → inet
* Sets the netmask length for an inet value. The address part does not change.
* set_masklen(inet '192.168.1.5/24', 16) → 192.168.1.5/16
*
*/
public static SimpleExpression setMaskLen(Expression exp1, Expression exp2) {
return FunctionUtils.twoArgFunc("set_masklen", exp1, exp2, _returnType(exp1, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: {@link StringType}
*
*
* @see text ( inet ) → text
* Returns the unabbreviated IP address and netmask length as text. (This has the same result as an explicit cast to text.)
* text(inet '192.168.1.5') → 192.168.1.5/32
*
*/
public static SimpleExpression text(Expression inet) {
return FunctionUtils.oneArgFunc("text", inet, StringType.INSTANCE);
}
/*-------------------below MAC Address Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: The {@link MappingType} of macAddr8
*
*
* @see macaddr8_set7bit ( macaddr8 ) → macaddr8
* Sets the 7th bit of the address to one, creating what is known as modified EUI-64, for inclusion in an IPv6 address.
* macaddr8_set7bit(macaddr8 '00:34:56:ab:cd:ef') → 02:34:56:ff:fe:ab:cd:ef
*
*/
public static SimpleExpression macAddr8Set7bit(Expression macAddr8) {
return FunctionUtils.oneArgFunc("macaddr8_set7bit", macAddr8, _returnType(macAddr8, Expressions::identityType));
}
/*-------------------below Comment Information Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see col_description ( table oid, column integer ) → text
*
*/
public static SimpleExpression colDescription(Expression table, Expression column) {
return FunctionUtils.twoArgFunc("col_description", table, column, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see obj_description ( object oid, catalog name ) → text
*
*/
public static SimpleExpression objDescription(Expression object, Expression catalog) {
return FunctionUtils.twoArgFunc("obj_description", object, catalog, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see obj_description ( object oid ) → text
*
*/
public static SimpleExpression objDescription(Expression object) {
return FunctionUtils.oneArgFunc("obj_description", object, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see shobj_description ( object oid, catalog name ) → text
*
*/
public static SimpleExpression shObjDescription(Expression object, Expression catalog) {
return FunctionUtils.twoArgFunc("shobj_description", object, catalog, TextType.INSTANCE);
}
/*-------------------below Transaction ID and Snapshot Information Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see pg_current_xact_id () → xid8
*
*/
public static SimpleExpression pgCurrentXactId() {
return FunctionUtils.zeroArgFunc("pg_current_xact_id", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see pg_current_xact_id_if_assigned () → xid8
*
*/
public static SimpleExpression pgCurrentXactIdIfAssigned() {
//TODO xid8 is binary ?
return FunctionUtils.zeroArgFunc("pg_current_xact_id_if_assigned", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_xact_status ( xid8 ) → text
*
*/
public static SimpleExpression pgXactStatus(Expression xid8) {
return FunctionUtils.oneArgFunc("pg_xact_status", xid8, TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* @see pg_current_snapshot () → pg_snapshot
*
*/
public static SimpleExpression pgCurrentSnapshot() {
//TODO pg_snapshot what type?
return FunctionUtils.zeroArgFunc("pg_current_snapshot", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
* - "Anonymous field" ( you must use as clause definite filed name) : {@link LongType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_snapshot_xip ( pg_snapshot ) → setof xid8
*
*/
public static _ColumnWithOrdinalityFunction pgSnapshotXip(Expression pgSnapshot) {
return DialectFunctionUtils.oneArgColumnFunction("pg_snapshot_xip", pgSnapshot, null, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see pg_snapshot_xmax ( pg_snapshot ) → xid8
*
*/
public static SimpleExpression pgSnapshotXMax(Expression pgSnapshot) {
return FunctionUtils.oneArgFunc("pg_snapshot_xmax", pgSnapshot, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see pg_snapshot_xmin ( pg_snapshot ) → xid8
*
*/
public static SimpleExpression pgSnapshotXMin(Expression pgSnapshot) {
return FunctionUtils.oneArgFunc("pg_snapshot_xmin", pgSnapshot, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* @see pg_visible_in_snapshot ( xid8, pg_snapshot ) → boolean
*
*/
public static SimplePredicate pgVisibleInSnapshot(Expression xid8, Expression pgSnapshot) {
return FunctionUtils.twoArgPredicateFunc("pg_visible_in_snapshot", xid8, pgSnapshot);
}
/*-------------------below Deprecated Transaction ID and Snapshot Information Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* Deprecated as of postgre 13
*
*
* @see txid_current () → bigint
* See pg_current_xact_id().
*
*/
public static SimpleExpression txidCurrent() {
return FunctionUtils.zeroArgFunc("txid_current", LongType.INSTANCE);
}
/**
*
The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
Deprecated as of postgre 13
*
* @see txid_current_if_assigned () → bigint
* See pg_current_xact_id_if_assigned().
*
*/
public static SimpleExpression txidCurrentIfAssigned() {
return FunctionUtils.zeroArgFunc("txid_current_if_assigned", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* Deprecated as of postgre 13
*
*
* @see txid_current_snapshot () → txid_snapshot
* See pg_current_snapshot().
*
*/
public static SimpleExpression txidCurrentSnapshot() {
//TODO what type is txid_snapshot ?
return FunctionUtils.zeroArgFunc("txid_current_snapshot", TextType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
* - "Anonymous field" ( you must use as clause definite filed name) : {@link LongType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* Deprecated as of postgre 13
*
*
* @see txid_snapshot_xip ( txid_snapshot ) → setof bigint
* see pg_snapshot_xip()
*
*/
public static _ColumnWithOrdinalityFunction txidSnapshotXip(Expression txidSnapshot) {
return DialectFunctionUtils.oneArgColumnFunction("txid_snapshot_xip", txidSnapshot, null, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* Deprecated as of postgre 13
*
*
* @see txid_snapshot_xmax ( txid_snapshot ) → bigint
* See pg_snapshot_xmax().
*
*/
public static SimpleExpression txidSnapshotXMax(Expression txidSnapshot) {
return FunctionUtils.oneArgFunc("txid_snapshot_xmax", txidSnapshot, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* Deprecated as of postgre 13
*
*
* @see txid_snapshot_xmin ( txid_snapshot ) → bigint
* See pg_snapshot_xmin().
*
*/
public static SimpleExpression txidSnapshotXMin(Expression txidSnapshot) {
return FunctionUtils.oneArgFunc("txid_snapshot_xmin", txidSnapshot, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}
*
*
* Deprecated as of postgre 13
*
*
* @see txid_visible_in_snapshot ( bigint, txid_snapshot ) → boolean
* See pg_visible_in_snapshot().
*
*/
public static SimplePredicate txidVisibleInSnapshot(Expression exp1, Expression exp2) {
return FunctionUtils.twoArgPredicateFunc("txid_visible_in_snapshot", exp1, exp2);
}
/**
*
* The {@link MappingType} of function return type: {@link TextType#INSTANCE}
*
*
* Deprecated as of postgre 13
*
*
* @see txid_status ( bigint ) → text
* See pg_xact_status().
*
*/
public static SimpleExpression txidStatus(Expression exp) {
return FunctionUtils.oneArgFunc("txid_status", exp, TextType.INSTANCE);
}
/*-------------------below Committed Transaction Information Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: {@link OffsetDateTimeType#INSTANCE}
*
*
* @see pg_xact_commit_timestamp ( xid ) → timestamp with time zone
*
*/
public static SimpleExpression pgXactCommitTimestamp(Expression xid) {
return FunctionUtils.oneArgFunc("pg_xact_commit_timestamp", xid, OffsetDateTimeType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type:
*
* - timestamp : {@link OffsetDateTimeType#INSTANCE}
* - roident : {@link LongType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_xact_commit_timestamp_origin ( xid ) → record ( timestamp timestamp with time zone, roident oid)
*
*/
public static _TabularWithOrdinalityFunction pgXactCommitTimestampOrigin(Expression xid) {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("timestamp", OffsetDateTimeType.INSTANCE),
ArmySelections.forName("roident", LongType.INSTANCE)
);
return DialectFunctionUtils.oneArgTabularFunc("pg_xact_commit_timestamp_origin", xid, fieldList);
}
/**
*
* The {@link MappingType} of function return type:
*
* - xid : {@link IntegerType#INSTANCE}
* - timestamp : {@link OffsetDateTimeType#INSTANCE}
* - roident : {@link LongType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_last_committed_xact () → record ( xid xid, timestamp timestamp with time zone, roident oid )
*
*/
public static _TabularWithOrdinalityFunction pgLastCommittedXact() {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("xid", IntegerType.INSTANCE),
ArmySelections.forName("timestamp", OffsetDateTimeType.INSTANCE),
ArmySelections.forName("roident", LongType.INSTANCE)
);
return DialectFunctionUtils.zeroArgTabularFunc("pg_last_committed_xact", fieldList);
}
/*-------------------below Control Data Functions-------------------*/
/**
*
* The {@link MappingType} of function return type:
*
* - checkpoint_lsn : {@link PostgrePgLsnType#LONG}
* - redo_lsn : {@link PostgrePgLsnType#LONG}
* - redo_wal_file : {@link TextType#INSTANCE}
* - timeline_id : {@link IntegerType#INSTANCE}
*
* - prev_timeline_id : {@link IntegerType#INSTANCE}
* - full_page_writes : {@link BooleanType#INSTANCE}
* - next_xid : {@link TextType#INSTANCE}
* - next_oid : {@link LongType#INSTANCE}
*
* - next_multixact_id : {@link IntegerType#INSTANCE}
* - next_multi_offset : {@link IntegerType#INSTANCE}
* - oldest_xid : {@link IntegerType#INSTANCE}
* - oldest_xid_dbid : {@link LongType#INSTANCE}
*
* - oldest_active_xid : {@link IntegerType#INSTANCE}
* - oldest_multi_xid : {@link IntegerType#INSTANCE}
* - oldest_multi_dbid : {@link LongType#INSTANCE}
* - oldest_commit_ts_xid : {@link IntegerType#INSTANCE}
*
* - newest_commit_ts_xid : {@link IntegerType#INSTANCE}
* - checkpoint_time : {@link OffsetDateTimeType#INSTANCE}
*
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_control_checkpoint () → record
*
* @see pg_control_checkpoint Output Columns
*
*/
public static _TabularWithOrdinalityFunction pgControlCheckpoint() {
final List fieldList;
fieldList = _Collections.arrayList(18);
fieldList.add(ArmySelections.forName("checkpoint_lsn", PostgrePgLsnType.LONG));
fieldList.add(ArmySelections.forName("redo_lsn", PostgrePgLsnType.LONG));
fieldList.add(ArmySelections.forName("redo_wal_file", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("timeline_id", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("prev_timeline_id", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("full_page_writes", BooleanType.INSTANCE));
fieldList.add(ArmySelections.forName("next_xid", TextType.INSTANCE));
fieldList.add(ArmySelections.forName("next_oid", LongType.INSTANCE));
fieldList.add(ArmySelections.forName("next_multixact_id", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("next_multi_offset", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("oldest_xid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("oldest_xid_dbid", LongType.INSTANCE));
fieldList.add(ArmySelections.forName("oldest_active_xid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("oldest_multi_xid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("oldest_multi_dbid", LongType.INSTANCE));
fieldList.add(ArmySelections.forName("oldest_commit_ts_xid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("newest_commit_ts_xid", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("checkpoint_time", OffsetDateTimeType.INSTANCE));
return DialectFunctionUtils.zeroArgTabularFunc("pg_control_checkpoint", fieldList);
}
/**
* The {@link MappingType} of function return type:
*
* - pg_control_version : {@link IntegerType#INSTANCE}
* - catalog_version_no : {@link IntegerType#INSTANCE}
* - system_identifier : {@link LongType#INSTANCE}
* - pg_control_last_modified : {@link OffsetDateTimeType#INSTANCE}
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_control_system () → record
*
* @see pg_control_system Output Columns
*
*/
public static _TabularWithOrdinalityFunction pgControlSystem() {
final List fieldList;
fieldList = ArrayUtils.of(
ArmySelections.forName("pg_control_version", IntegerType.INSTANCE),
ArmySelections.forName("catalog_version_no", IntegerType.INSTANCE),
ArmySelections.forName("system_identifier", LongType.INSTANCE),
ArmySelections.forName("pg_control_last_modified", OffsetDateTimeType.INSTANCE)
);
return DialectFunctionUtils.zeroArgTabularFunc("pg_control_system", fieldList);
}
/**
*
* The {@link MappingType} of function return type:
*
* - max_data_alignment : {@link IntegerType#INSTANCE}
* - database_block_size : {@link IntegerType#INSTANCE}
* - blocks_per_segment : {@link IntegerType#INSTANCE}
* - wal_block_size : {@link IntegerType#INSTANCE}
*
* - bytes_per_wal_segment : {@link IntegerType#INSTANCE}
* - max_identifier_length : {@link IntegerType#INSTANCE}
* - max_index_columns : {@link IntegerType#INSTANCE}
* - max_toast_chunk_size : {@link IntegerType#INSTANCE}
*
* - large_object_chunk_size : {@link IntegerType#INSTANCE}
* - float8_pass_by_value : {@link BooleanType#INSTANCE}
* - data_page_checksum_version : {@link IntegerType#INSTANCE}
*
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_control_init () → record
*
* @see pg_control_init Output Columns
*
*/
public static _TabularWithOrdinalityFunction pgControlInit() {
final List fieldList;
fieldList = _Collections.arrayList(11);
fieldList.add(ArmySelections.forName("max_data_alignment", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("database_block_size", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("blocks_per_segment", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("wal_block_size", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("bytes_per_wal_segment", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("max_identifier_length", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("max_index_columns", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("max_toast_chunk_size", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("large_object_chunk_size", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("float8_pass_by_value", BooleanType.INSTANCE));
fieldList.add(ArmySelections.forName("data_page_checksum_version", IntegerType.INSTANCE));
return DialectFunctionUtils.zeroArgTabularFunc("pg_control_init", fieldList);
}
/**
*
* The {@link MappingType} of function return type:
*
* - min_recovery_end_lsn : {@link PostgrePgLsnType#LONG}
* - min_recovery_end_timeline : {@link IntegerType#INSTANCE}
* - backup_start_lsn : {@link PostgrePgLsnType#LONG}
* - backup_end_lsn : {@link PostgrePgLsnType#LONG}
*
* - end_of_backup_record_required : {@link BooleanType#INSTANCE}
*
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see pg_control_recovery () → record
*
* @see pg_control_recovery Output Columns
*
*/
public static _TabularWithOrdinalityFunction pgControlRecovery() {
final List fieldList;
fieldList = _Collections.arrayList(5);
fieldList.add(ArmySelections.forName("min_recovery_end_lsn", PostgrePgLsnType.LONG));
fieldList.add(ArmySelections.forName("min_recovery_end_timeline", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("backup_start_lsn", PostgrePgLsnType.LONG));
fieldList.add(ArmySelections.forName("backup_end_lsn", PostgrePgLsnType.LONG));
fieldList.add(ArmySelections.forName("end_of_backup_record_required", BooleanType.INSTANCE));
return DialectFunctionUtils.zeroArgTabularFunc("pg_control_recovery", fieldList);
}
/*-------------------below Trigger Functions TODO ? -------------------*/
/*-------------------below Event Trigger Functions TODO ? -------------------*/
/*-------------------below Statistics Information Functions-------------------*/
/**
*
* The {@link MappingType} of function return type:
*
* - index : {@link IntegerType#INSTANCE}
* - values : {@link TextArrayType#LINEAR}
* - nulls : {@link BooleanArrayType#PRIMITIVE_LINEAR}
* - frequency : {@link DoubleType#INSTANCE}
*
* - base_frequency : {@link DoubleType#INSTANCE}
*
* - ordinality (optional) : {@link LongType#INSTANCE} ,see {@link io.army.criteria.impl.Functions._WithOrdinalityClause}
*
*
*
* @see Statistics Information Functions
*
*/
public static _TabularWithOrdinalityFunction pgMcvListItems(Expression pgMcvList) {
final List fieldList = _Collections.arrayList(5);
fieldList.add(ArmySelections.forName("index", IntegerType.INSTANCE));
fieldList.add(ArmySelections.forName("values", TextArrayType.LINEAR));
fieldList.add(ArmySelections.forName("nulls", BooleanArrayType.PRIMITIVE_LINEAR));
fieldList.add(ArmySelections.forName("frequency", DoubleType.INSTANCE));
fieldList.add(ArmySelections.forName("base_frequency", DoubleType.INSTANCE));
return DialectFunctionUtils.oneArgTabularFunc("pg_mcv_list_items", pgMcvList, fieldList);
}
/*-------------------below sampling methods-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link VoidType#VOID}
*
*
* @see TABLESAMPLE sampling_method ( argument [, ...] ) [ REPEATABLE ( seed ) ]
*
*/
public static Expression bernoulli(Expression fraction) {
return FunctionUtils.oneArgFunc("BERNOULLI", fraction, VoidType.VOID);
}
/**
*
* The {@link MappingType} of function return type: {@link VoidType#VOID}
*
*
* @see TABLESAMPLE sampling_method ( argument [, ...] ) [ REPEATABLE ( seed ) ]
*
*/
public static Expression system(Expression fraction) {
return FunctionUtils.oneArgFunc("SYSTEM", fraction, VoidType.VOID);
}
}