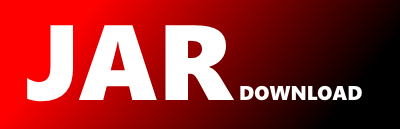
io.army.criteria.impl.PostgreSimpleValues Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.criteria.*;
import io.army.criteria.postgre.PostgreCtes;
import io.army.criteria.postgre.PostgreQuery;
import io.army.criteria.postgre.PostgreStatement;
import io.army.criteria.postgre.PostgreValues;
import io.army.dialect.Dialect;
import io.army.dialect.postgre.PostgreDialect;
import javax.annotation.Nullable;
import java.util.function.Consumer;
import java.util.function.Function;
/**
*
* This class is abstract implementation of {@link PostgreValues}.
*
* @since 1.0
*/
abstract class PostgreSimpleValues extends SimpleValues.WithSimpleValues<
I,
PostgreCtes,
PostgreValues._ValuesSpec,
PostgreValues._ValuesLeftParenSpec,
PostgreValues._OrderByCommaSpec,
PostgreValues._LimitSpec,
PostgreValues._OffsetSpec,
PostgreValues._FetchSpec,
PostgreStatement._AsValuesClause,
PostgreValues._QueryWithComplexSpec>
implements PostgreValues._WithSpec,
PostgreValues._ValuesLeftParenSpec,
PostgreValues._OffsetSpec,
PostgreValues._OrderByCommaSpec,
PostgreValues {
/**
*
* create primary VALUES statement.
*
*/
static PostgreValues._WithSpec simpleValues() {
return new SimplePrimaryValues<>(null, null, SQLs::identity);
}
/**
* create primary VALUES statement for dispatcher.
*/
static PostgreValues._WithSpec fromDispatcher(ArmyStmtSpec spec,
Function super Values, I> function) {
return new SimplePrimaryValues<>(spec, null, function);
}
/**
* create sub VALUES statement for dispatcher.
*/
static PostgreValues._WithSpec fromSubDispatcher(ArmyStmtSpec spec,
Function super SubValues, I> function) {
return new SimpleSubValues<>(spec, null, function);
}
/**
* create sub VALUES statement.
*/
static PostgreValues._WithSpec subValues(CriteriaContext outerContext,
Function super SubValues, I> function) {
return new SimpleSubValues<>(null, outerContext, function);
}
private PostgreSimpleValues(@Nullable _WithClauseSpec spec, CriteriaContext context) {
super(spec, context);
}
@Override
public final PostgreQuery._StaticCteParensSpec<_ValuesSpec> with(String name) {
return PostgreQueries.complexCte(this.context, false, this::endStaticWithClause)
.comma(name);
}
@Override
public final PostgreQuery._StaticCteParensSpec<_ValuesSpec> withRecursive(String name) {
return PostgreQueries.complexCte(this.context, true, this::endStaticWithClause)
.comma(name);
}
@Override
public final _OrderBySpec values(Consumer consumer) {
consumer.accept(new RowConstructorImpl(this));
return this;
}
@Override
public final _PostgreValuesLeftParenClause values() {
return this;
}
@Override
final Dialect statementDialect() {
return PostgreDialect.POSTGRE15;
}
@Override
final PostgreCtes createCteBuilder(boolean recursive) {
return PostgreSupports.postgreCteBuilder(recursive, this.context);
}
@Override
final String columnAlias(int columnIndex) {
return "column" + (++columnIndex);
}
private static final class SimplePrimaryValues extends PostgreSimpleValues
implements ArmyValues {
private final Function super Values, I> function;
/**
* @param outerBracketContext outer bracket context
*/
private SimplePrimaryValues(@Nullable ArmyStmtSpec spec, @Nullable CriteriaContext outerBracketContext,
Function super Values, I> function) {
super(spec, CriteriaContexts.primaryValuesContext(spec, outerBracketContext));
this.function = function;
}
@Override
public _UnionOrderBySpec parens(Function<_WithSpec<_UnionOrderBySpec>, _UnionOrderBySpec> function) {
this.endStmtBeforeCommand();
final BracketValues bracket;
bracket = new BracketValues<>(this, this.function);
return function.apply(new SimplePrimaryValues<>(null, bracket.context, bracket::parensEnd));
}
@Override
I onAsValues() {
return this.function.apply(this);
}
@Override
_QueryWithComplexSpec createUnionValues(final _UnionType unionType) {
final Function unionFun;
unionFun = right -> this.function.apply(new UnionValues(this, unionType, right));
return new ValuesDispatcher<>(this.context, unionFun);
}
}//SimplePrimaryValues
private static final class SimpleSubValues extends PostgreSimpleValues
implements ArmySubValues {
private final Function super SubValues, I> function;
private SimpleSubValues(@Nullable ArmyStmtSpec spec, @Nullable CriteriaContext outerContext,
Function super SubValues, I> function) {
super(spec, CriteriaContexts.subValuesContext(spec, outerContext));
this.function = function;
}
@Override
public _UnionOrderBySpec parens(Function<_WithSpec<_UnionOrderBySpec>, _UnionOrderBySpec> function) {
this.endStmtBeforeCommand();
final BracketSubValues bracket;
bracket = new BracketSubValues<>(this, this.function);
return function.apply(PostgreSimpleValues.subValues(bracket.context, bracket::parensEnd));
}
@Override
I onAsValues() {
return this.function.apply(this);
}
@Override
_QueryWithComplexSpec createUnionValues(final _UnionType unionType) {
final Function unionFun;
unionFun = rowSet -> this.function.apply(new UnionSubValues(this, unionType, rowSet));
return new SubValuesDispatcher<>(this.context, unionFun);
}
}//SimpleSubValues
static abstract class PostgreBracketValues extends BracketRowSet<
I,
PostgreValues._UnionOrderBySpec,
PostgreValues._UnionOrderByCommaSpec,
PostgreValues._UnionLimitSpec,
PostgreValues._UnionOffsetSpec,
PostgreValues._UnionFetchSpec,
PostgreValues._AsValuesClause,
PostgreValues._QueryWithComplexSpec>
implements PostgreValues,
PostgreValues._UnionOrderBySpec,
PostgreValues._UnionOrderByCommaSpec,
PostgreValues._UnionOffsetSpec,
PostgreValues._UnionFetchSpec {
private PostgreBracketValues(ArmyStmtSpec spec) {
super(spec);
}
@Override
public final I asValues() {
return this.asQuery();
}
@Override
final Dialect statementDialect() {
return PostgreDialect.POSTGRE15;
}
}//PostgreBracketValues
private static final class BracketValues extends PostgreBracketValues
implements ArmyValues {
private final Function super Values, I> function;
private BracketValues(ArmyStmtSpec spec, Function super Values, I> function) {
super(spec);
this.function = function;
}
@Override
I onAsQuery() {
return this.function.apply(this);
}
@Override
_QueryWithComplexSpec createUnionRowSet(final _UnionType unionType) {
final Function unionFun;
unionFun = rowSet -> this.function.apply(new UnionValues(this, unionType, rowSet));
return new ValuesDispatcher<>(this.context, unionFun);
}
}//BracketValues
private static final class BracketSubValues extends PostgreBracketValues
implements ArmySubValues {
private final Function super SubValues, I> function;
private BracketSubValues(ArmyStmtSpec spec, Function super SubValues, I> function) {
super(spec);
this.function = function;
}
@Override
I onAsQuery() {
return this.function.apply(this);
}
@Override
_QueryWithComplexSpec createUnionRowSet(final _UnionType unionType) {
final Function unionFun;
unionFun = rowSet -> this.function.apply(new UnionSubValues(this, unionType, rowSet));
return new SubValuesDispatcher<>(this.context, unionFun);
}
}//BracketSubValues
private static abstract class PostgreValuesDispatcher
extends PostgreQueries.PostgreSelectClauseDispatcher<
I,
PostgreValues._QueryComplexSpec>
implements PostgreValues._QueryWithComplexSpec {
final Function function;
private PostgreValuesDispatcher(CriteriaContext leftContext, Function function) {
super(leftContext.getOuterContext(), leftContext);
this.function = function;
}
private PostgreValuesDispatcher(PostgreBracketValues> bracket, Function function) {
super(bracket.context, null);
this.function = function;
}
@Override
public final PostgreQuery._StaticCteParensSpec<_QueryComplexSpec> with(String name) {
return PostgreQueries.complexCte(this.context, false, this::endStaticWithClause)
.comma(name);
}
@Override
public final PostgreQuery._StaticCteParensSpec<_QueryComplexSpec> withRecursive(String name) {
return PostgreQueries.complexCte(this.context, true, this::endStaticWithClause)
.comma(name);
}
@Override
final PostgreCtes createCteBuilder(boolean recursive, CriteriaContext context) {
return PostgreSupports.postgreCteBuilder(recursive, context);
}
}//PostgreComplexValues
private static final class ValuesDispatcher extends PostgreValuesDispatcher {
private ValuesDispatcher(CriteriaContext leftContext, Function function) {
super(leftContext, function);
}
private ValuesDispatcher(BracketValues> bracket, Function function) {
super(bracket, function);
}
@Override
public _UnionOrderBySpec parens(Function<_QueryWithComplexSpec<_UnionOrderBySpec>, _UnionOrderBySpec> function) {
this.endDispatcher();
final BracketValues bracket;
bracket = new BracketValues<>(this, this.function);
return function.apply(new ValuesDispatcher<>(bracket, bracket::parensEnd));
}
@Override
public PostgreValues._OrderBySpec values(Consumer consumer) {
this.endDispatcher();
return PostgreSimpleValues.fromDispatcher(this, this.function)
.values(consumer);
}
@Override
public _PostgreValuesLeftParenClause values() {
this.endDispatcher();
return PostgreSimpleValues.fromDispatcher(this, this.function)
.values();
}
@Override
PostgreQueries createSelectClause() {
this.endDispatcher();
return PostgreQueries.fromDispatcher(this, this.function);
}
}//ValuesDispatcher
private static final class SubValuesDispatcher extends PostgreValuesDispatcher {
private SubValuesDispatcher(CriteriaContext leftContext, Function function) {
super(leftContext, function);
}
private SubValuesDispatcher(BracketSubValues> bracket, Function function) {
super(bracket, function);
}
@Override
public _UnionOrderBySpec parens(Function<_QueryWithComplexSpec<_UnionOrderBySpec>, _UnionOrderBySpec> function) {
this.endDispatcher();
final BracketSubValues bracket;
bracket = new BracketSubValues<>(this, this.function);
return function.apply(new SubValuesDispatcher<>(bracket, bracket::parensEnd));
}
@Override
public _OrderBySpec values(Consumer consumer) {
this.endDispatcher();
return PostgreSimpleValues.fromSubDispatcher(this, this.function)
.values(consumer);
}
@Override
public _PostgreValuesLeftParenClause values() {
this.endDispatcher();
return PostgreSimpleValues.fromSubDispatcher(this, this.function)
.values();
}
@Override
PostgreQueries createSelectClause() {
this.endDispatcher();
return PostgreQueries.fromSubDispatcher(this, this.function);
}
}//SubValuesDispatcher
}