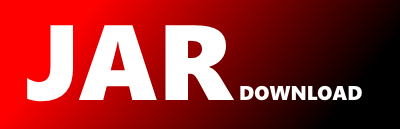
io.army.criteria.impl.PostgreSyntax Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.criteria.*;
import io.army.dialect._Constant;
import io.army.mapping.*;
import io.army.mapping.array.TextArrayType;
import io.army.meta.FieldMeta;
import io.army.util.ArrayUtils;
import io.army.util._StringUtils;
import java.util.Collection;
import java.util.Collections;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
/**
*
* Package class
*
* @since 1.0
*/
abstract class PostgreSyntax extends PostgreWindowFunctions {
/**
* Package constructor
*/
PostgreSyntax() {
}
/**
*
* Static array constructor, array is {@link TextArrayType#LINEAR} type.
*
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array() {
return Expressions.array();
}
/**
*
* Static array constructor
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array(Object element) {
SQLs._ArrayConstructorSpec array;
if (element instanceof SubQuery) {
array = Expressions.array((SubQuery) element);
} else {
array = Expressions.array(Expressions::nonNullFirstArrayType, Collections.singletonList(element));
}
return array;
}
/**
*
* Static array constructor, array is {@link TextArrayType#LINEAR} type.
*
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array(Object one, Object two) {
return Expressions.array(Expressions::nonNullFirstArrayType, ArrayUtils.of(one, two));
}
/**
*
* Static array constructor, array is {@link TextArrayType#LINEAR} type.
*
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array(Object one, Object two, Object three) {
return Expressions.array(Expressions::nonNullFirstArrayType, ArrayUtils.of(one, two, three));
}
/**
*
* Static array constructor, array is {@link TextArrayType#LINEAR} type.
*
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array(Object one, Object two, Object three, Object four) {
return Expressions.array(Expressions::nonNullFirstArrayType,
ArrayUtils.of(one, two, three, four)
);
}
/**
*
* Static array constructor, array is {@link TextArrayType#LINEAR} type.
*
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array(Object one, Object two, Object three, Object four, Object five,
Object... rest) {
return Expressions.array(Expressions::nonNullFirstArrayType,
ArrayUtils.of(one, two, three, four, five, rest)
);
}
/**
*
* Dynamic array constructor, if empty,then array is {@link TextArrayType#LINEAR} type.
*
*
* @see Array Constructors
*/
public static SQLs._ArrayConstructorSpec array(Consumer> consumer) {
return Expressions.array(Expressions::nonNullFirstArrayType, consumer);
}
public static Expression excluded(FieldMeta> field) {
return ContextStack.peek().insertValueField(field, PostgreExcludedField::excludedField);
}
/**
* @param expression couldn't be multi-value parameter/literal, for example {@link SQLs#rowParam(TypeInfer, Collection)}
* @see Using Positional Notation
* @see Using Named Notation
* @see make_interval ( [ years int [, months int [, weeks int [, days int [, hours int [, mins int [, secs double precision ]]]]]]] ) → interval
*/
public static Expression namedNotation(String name, Expression expression) {
return FunctionUtils.namedNotation(name, expression);
}
/**
* @param valueOperator couldn't return multi-value parameter/literal, for example {@link SQLs#rowParam(TypeInfer, Collection)}
* @see Using Positional Notation
* @see Using Named Notation
* @see make_interval ( [ years int [, months int [, weeks int [, days int [, hours int [, mins int [, secs double precision ]]]]]]] ) → interval
*/
public static Expression namedNotation(String name, Function valueOperator, T value) {
return FunctionUtils.namedNotation(name, valueOperator.apply(value));
}
/**
*
* create empty row. non-empty row see {@link SQLs#row(Object)}
*
*
* @see SQLs#row(Object)
* @see SQLs#row(SubQuery)
* @see SQLs#row(Consumer)
* @see Row Constructors
*/
public static RowExpression row() {
return RowExpressions.emptyRow();
}
/*-------------------below operator method -------------------*/
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp
*
*
* @see Absolute value operator
*/
public static Expression at(Expression operand) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.AT, operand, Expressions::identityType);
}
/**
* @see @-@ geometric_type → double precision
* Computes the total length. Available for lseg, path.
*
*/
public static Expression atHyphenAt(Expression operand) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.AT_HYPHEN_AT, operand, PostgreExpressions::atHyphenAtType);
}
/**
* @see @@ geometric_type → point
* Computes the center point. Available for box, lseg, polygon, circle.
*
*/
public static Expression atAt(Expression operand) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.AT_AT, operand, PostgreExpressions::atAtType);
}
/**
* @see # geometric_type → integer
* Returns the number of points. Available for path, polygon.
*
*/
public static Expression pound(Expression operand) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.POUND, operand, PostgreExpressions::unaryPoundType);
}
/*-------------------below dual operator -------------------*/
/**
*
Create PostgreSQL-style typecast expression. Format : 'string'::type .
* This method is used for postgre dialect type, for example : text, regclass .
*
*
NOTE: {@link LiteralExpression#typeMeta()} always is {@link NoCastTextType#INSTANCE}
*
*
examples :
*
* Postgres.space("my_seq",Postgres.DOUBLE_COLON,"regclass")
* Postgres.space('my_seq',DOUBLE_COLON,"regclass")
* Postgres.space('QinArmy',DOUBLE_COLON,"text")
*
*
* @param literal text literal
* @param doubleColon must be {@link Postgres#DOUBLE_COLON}
* @param typeName not key word , a simple sql identifier.
* @return a {@link LiteralExpression} whose {@link LiteralExpression#typeMeta()} always is {@link NoCastTextType#INSTANCE}
* @throws CriteriaException throw when
* - literal error,here is delay , throw when parsing
* - typeName error,here is delay , throw when parsing
* - dialect isn't {@link io.army.dialect.postgre.PostgreDialect},here is delay , throw when parsing
*
*/
public static LiteralExpression space(String literal, Postgres.DoubleColon doubleColon, String typeName) {
if (doubleColon != Postgres.DOUBLE_COLON) {
throw CriteriaUtils.errorSymbol(doubleColon);
}
return PostgreDoubleColonCastExpression.cast(literal, typeName);
}
/**
* @see geometric_type # geometric_type → point
* Computes the point of intersection, or NULL if there is none. Available for lseg, line.
*
*/
public static CompoundExpression pound(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.POUND, right, PostgreExpressions::dualPoundType);
}
/**
* @see geometric_type <-> geometric_type → double precision
* Computes the distance between the objects. Available for all seven geometric types, for all combinations of point with another geometric type, and for these additional pairs of types: (box, lseg), (lseg, line), (polygon, circle) (and the commutator cases).
*
*/
public static CompoundExpression ltHyphenGt(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.LT_HYPHEN_GT, right, PostgreExpressions::ltHyphenGtType);
}
/**
* @see numeric_type + numeric_type → numeric_type
*/
public static CompoundExpression plus(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.PLUS, right, PostgreExpressions::plusType);
}
/**
* @see numeric_type - numeric_type → numeric_type
* Subtraction
* @see jsonb - text → jsonb
* jsonb - text[] → jsonb
* jsonb - integer → jsonb
*
*/
public static CompoundExpression minus(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.MINUS, right, PostgreExpressions::minusType);
}
/**
* @see numeric_type * numeric_type → numeric_type
* Multiplication
*/
public static CompoundExpression times(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.TIMES, right, PostgreExpressions::timesType);
}
/**
* @see numeric_type / numeric_type → numeric_type
* Division (for integral types, division truncates the result towards zero)
*/
public static CompoundExpression divide(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.DIVIDE, right, PostgreExpressions::divideType);
}
/**
* @see numeric_type % numeric_type → numeric_type
* Modulo (remainder); available for smallint, integer, bigint, and numeric
*/
public static CompoundExpression mode(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.MOD, right, Expressions::mathExpType);
}
/**
*
* The {@link MappingType} of function return type: follow
* private static MappingType caretResultType(final MappingType left, final MappingType right) {
* final MappingType returnType;
* if (left instanceof MappingType.IntegerOrDecimalType
* && right instanceof MappingType.IntegerOrDecimalType) {
* returnType = BigDecimalType.INSTANCE;
* } else {
* returnType = DoubleType.INSTANCE;
* }
* return returnType;
* }
*
*
* @see numeric ^ numeric → numeric
* double precision ^ double precision → double precision
* Exponentiation
*/
public static CompoundExpression caret(final Expression left, final Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.EXPONENTIATION, right, PostgreSyntax::caretResultType);
}
/**
* @see #doubleAmp(Expression, Expression)
* @see tsquery && tsquery → tsquery
* ANDs two tsquerys together, producing a query that matches documents that match both input queries.
*
*/
public static CompoundExpression ampAmp(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.DOUBLE_AMP, right, PostgreExpressions::doubleAmpType);
}
/**
* @see json -> integer → json
* jsonb -> integer → jsonb
* Extracts n'th element of JSON array (array elements are indexed from zero, but negative integers count from the end).
* '[{"a":"foo"},{"b":"bar"},{"c":"baz"}]'::json -> 2 → {"c":"baz"}
*
*/
public static CompoundExpression hyphenGt(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.HYPHEN_GT, right, PostgreExpressions::hyphenGtType);
}
/**
* @see json ->> integer → text
* jsonb ->> integer → text
* Extracts n'th element of JSON array, as text.
* '[1,2,3]'::json ->> 2 → 3
* json ->> text → text
* jsonb ->> text → text
*
*/
public static CompoundExpression hyphenGtGt(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.HYPHEN_GT_GT, right, PostgreExpressions::hyphenGtGtType);
}
/**
* @see jsonb #- text[] → jsonb
* Deletes the field or array element at the specified path, where path elements can be either field keys or array indexes.
* '["a", {"b":1}]'::jsonb #- '{1,b}' → ["a", {}]
*
*/
public static CompoundExpression poundHyphen(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.POUND_HYPHEN, right, PostgreExpressions::poundHyphenType);
}
/**
* @see json #> text[] → json
* jsonb #> text[] → jsonb
* Extracts JSON sub-object at the specified path, where path elements can be either field keys or array indexes.
* '{"a": {"b": ["foo","bar"]}}'::json #> '{a,b,1}' → "bar"
*
*/
public static CompoundExpression poundGt(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.POUND_GT, right, PostgreExpressions::poundGtType);
}
/**
* @see json #>> text[] → text
* jsonb #>> text[] → text
* Extracts JSON sub-object at the specified path as text.
* '{"a": {"b": ["foo","bar"]}}'::json #>> '{a,b,1}' → bar
*
*/
public static CompoundExpression poundGtGt(Expression left, Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.POUND_GT_GT, right, PostgreExpressions::poundGtGtType);
}
/**
*
* The {@link MappingType} of operator return type: follow
* private static MappingType doubleVerticalType(final MappingType left, final MappingType right) {
* final MappingType returnType;
* if (left instanceof MappingType.SqlStringType || right instanceof MappingType.SqlStringType) {
* returnType = TextType.INSTANCE;
* } else if (left instanceof MappingType.SqlBinaryType || right instanceof MappingType.SqlBinaryType) {
* if (left instanceof MappingType.SqlBitType || right instanceof MappingType.SqlBitType) {
* throw CriteriaUtils.dualOperandError(DualOperator.DOUBLE_VERTICAL, left, right);
* }
* returnType = PrimitiveByteArrayType.INSTANCE;
* } else if (left instanceof MappingType.SqlBitType || right instanceof MappingType.SqlBitType) {
* returnType = BitSetType.INSTANCE;
* } else {
* throw CriteriaUtils.dualOperandError(DualOperator.DOUBLE_VERTICAL, left, right);
* }
* return returnType;
* }
*
*
*
* @param left not {@link SQLs#DEFAULT} etc.
* @param right not {@link SQLs#DEFAULT} etc.
* @see Expression#space(BiFunction, Object)
* @see SimpleExpression#space(BiFunction, BiFunction, Object)
* @see bit || bit → bit
* @see text || text → text
* text || anynonarray → text
* anynonarray || text → text
*
* @see bytea || bytea → bytea
* @see tsvector || tsvector → tsvector
* @see tsquery || tsquery → tsquery
* @see tsquery || tsquery → tsquery
* @see jsonb || jsonb → jsonb
* @see anycompatiblearray || anycompatiblearray → anycompatiblearray
* @see anycompatible || anycompatiblearray → anycompatiblearray
*/
public static CompoundExpression doubleVertical(final Expression left, final Expression right) {
return Expressions.dialectDualExp(left, DualExpOperator.DOUBLE_VERTICAL, right, PostgreExpressions::doubleVerticalType);
}
/**
*
* The {@link MappingType} of function return type:{@link DoubleType}
*
*
* @see |/ double precision → double precision
* Square root
*
*/
public static Expression verticalSlash(final Expression exp) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.VERTICAL_SLASH, exp, Expressions::doubleType);
}
/**
* @see !! tsquery → tsquery
* Negates a tsquery, producing a query that matches documents that do not match the input query.
*
*/
public static Expression doubleExclamation(final Expression exp) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.DOUBLE_EXCLAMATION, exp, Expressions::identityType);
}
/**
*
* The {@link MappingType} of function return type:{@link DoubleType}
*
*
* @see ||/ double precision → double precision
* Cube root
*
*/
public static Expression doubleVerticalSlash(final Expression operand) {
return PostgreExpressions.unaryExpression(PostgreUnaryExpOperator.DOUBLE_VERTICAL_SLASH, operand, Expressions::doubleType);
}
/**
* @see ?- line → boolean
* ?- lseg → boolean
* Is line horizontal?
*
*/
public static IPredicate questionHyphen(Expression operand) {
return PostgreExpressions.unaryPredicate(PostgreBooleanUnaryOperator.QUESTION_HYPHEN, operand);
}
/**
* @see ?| line → boolean
* ?| lseg → boolean
* Is line vertical?
*
*/
public static IPredicate questionVertical(Expression operand) {
return PostgreExpressions.unaryPredicate(PostgreBooleanUnaryOperator.QUESTION_VERTICAL, operand);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type @> geometric_type → boolean
* Does first object contain second? Available for these pairs of types: (box, point), (box, box), (path, point), (polygon, point), (polygon, polygon), (circle, point), (circle, circle).
*
* @see tsquery @> tsquery → boolean
*
* @see jsonb @> jsonb → boolean
*
* @see anyarray @> anyarray → boolean
*
*/
public static CompoundPredicate atGt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.AT_GT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see jsonb @? jsonpath → boolean
* Does JSON path return any item for the specified JSON value?
* '{"a":[1,2,3,4,5]}'::jsonb @? '$.a[*] ? (@ > 2)' → t
*
*/
public static CompoundPredicate atQuestion(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.AT_QUESTION, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type <@ geometric_type → boolean
* Is first object contained in or on second? Available for these pairs of types: (point, box), (point, lseg), (point, line), (point, path), (point, polygon), (point, circle), (box, box), (lseg, box), (lseg, line), (polygon, polygon), (circle, circle).
*
* @see tsquery <@ tsquery → boolean
*
* @see jsonb <@ jsonb → boolean
*
* @see anyarray <@ anyarray → boolean
*
*/
public static CompoundPredicate ltAt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.LT_AT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type && geometric_type → boolean
* Do these objects overlap? (One point in common makes this true.) Available for box, polygon, circle.
* @see inet && inet → boolean
* Does either subnet contain or equal the other?
* inet '192.168.1/24' && inet '192.168.1.80/28' → t
* inet '192.168.1/24' && inet '192.168.2.0/28' → f
*
* @see anyarray && anyarray → boolean
*
*/
public static CompoundPredicate doubleAmp(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.DOUBLE_AMP, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type << geometric_type → boolean
* Is first object strictly left of second? Available for point, box, polygon, circle.
* inet << inet → boolean
* Is subnet strictly contained by subnet? This operator, and the next four, test for subnet inclusion. They consider only the network parts of the two
* addresses (ignoring any bits to the right of the netmasks) and determine whether one network is identical to or a subnet of the other.
* inet '192.168.1.5' << inet '192.168.1/24' → t
* inet '192.168.0.5' << inet '192.168.1/24' → f
* inet '192.168.1/24' << inet '192.168.1/24' → f
*
* @see inet << inet → boolean
* Is subnet strictly contained by subnet? This operator, and the next four, test for subnet inclusion. They consider only the network parts of the two
* addresses (ignoring any bits to the right of the netmasks) and determine whether one network is identical to or a subnet of the other.
* inet '192.168.1.5' << inet '192.168.1/24' → t
* inet '192.168.0.5' << inet '192.168.1/24' → f
* inet '192.168.1/24' << inet '192.168.1/24' → f
*
*/
public static CompoundPredicate ltLt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.LT_LT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type >> geometric_type → boolean
* Is first object strictly right of second? Available for point, box, polygon, circle.
*/
public static CompoundPredicate gtGt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.GT_GT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see inet <<= inet → boolean
* Is subnet contained by or equal to subnet?
* inet '192.168.1/24' <<= inet '192.168.1/24' → t
*
*/
public static CompoundPredicate ltLtEqual(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.LT_LT_EQUAL, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see inet >>= inet → boolean
* Does subnet contain or equal subnet?
* inet '192.168.1/24' >>= inet '192.168.1/24' → t
*
*/
public static CompoundPredicate gtGtEqual(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.GT_GT_EQUAL, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type &< geometric_type → boolean
* Does first object not extend to the right of second? Available for box, polygon, circle.
*/
public static CompoundPredicate ampLt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.AMP_LT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type &> geometric_type → boolean
* Does first object not extend to the left of second? Available for box, polygon, circle.
*/
public static CompoundPredicate ampGt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.AMP_GT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type <<| geometric_type → boolean
* Is first object strictly below second? Available for point, box, polygon, circle.
*/
public static CompoundPredicate ltLtVertical(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.LT_LT_VERTICAL, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type |>> geometric_type → boolean
* Is first object strictly above second? Available for point, box, polygon, circle.
*/
public static CompoundPredicate verticalGtGt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.VERTICAL_GT_GT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type &<| geometric_type → boolean
* Does first object not extend above second? Available for box, polygon, circle.
*/
public static CompoundPredicate ampLtVertical(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.AMP_LT_VERTICAL, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type |&> geometric_type → boolean
* Does first object not extend below second? Available for box, polygon, circle.
*/
public static CompoundPredicate verticalAmpGt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.VERTICAL_AMP_GT, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see box <^ box → boolean
* Is first object below second (allows edges to touch)?
*/
public static CompoundPredicate ltCaret(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.LT_CARET, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see box >^ box → boolean
* Is first object above second (allows edges to touch)?
*/
public static CompoundPredicate gtCaret(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.GT_CARET, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see jsonb ? text → boolean
* Does the text string exist as a top-level key or array element within the JSON value?
* '{"a":1, "b":2}'::jsonb ? 'b' → t
* '["a", "b", "c"]'::jsonb ? 'b' → t
*
*/
public static CompoundPredicate question(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see geometric_type ?# geometric_type → boolean
* Is first object above second (allows edges to touch)?
*/
public static CompoundPredicate questionPound(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION_POUND, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see jsonb ?& text[] → boolean
* Do all of the strings in the text array exist as top-level keys or array elements?
* '["a", "b", "c"]'::jsonb ?& array['a', 'b'] → t
*
*/
public static CompoundPredicate questionAmp(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION_AMP, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see point ?- point → boolean
* Are points horizontally aligned (that is, have same y coordinate)?
*/
public static CompoundPredicate questionHyphen(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION_HYPHEN, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see point ?| point → boolean
* Are points vertically aligned (that is, have same x coordinate)?
* @see jsonb ?| text[] → boolean
* Do any of the strings in the text array exist as top-level keys or array elements?
* '{"a":1, "b":2, "c":3}'::jsonb ?| array['b', 'd'] → t
*
*/
public static CompoundPredicate questionVertical(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION_VERTICAL, right);
}
/**
* @see Expression#whiteSpace(BiFunction, Object)
* @see SimpleExpression#whiteSpace(BiFunction, BiFunction, Object)
* @see line ?-| line → boolean
* lseg ?-| lseg → boolean
*/
public static CompoundPredicate questionHyphenVertical(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION_HYPHEN_VERTICAL, right);
}
/**
* @see line ?|| line → boolean
* lseg ?|| lseg → boolean
*/
public static CompoundPredicate questionVerticalVertical(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.QUESTION_VERTICAL_VERTICAL, right);
}
/**
* @see geometric_type ~= geometric_type → boolean
* Are these objects the same? Available for point, box, polygon, circle.
*
*/
public static CompoundPredicate tildeEqual(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.TILDE_EQUAL, right);
}
/**
* @see tsvector @@ tsquery → boolean
* tsquery @@ tsvector → boolean
* text @@ tsquery → boolean
*
* @see jsonb @@ jsonpath → boolean
* tReturns the result of a JSON path predicate check for the specified JSON value. Only the first item of the result is taken into account. If the result is not Boolean, then NULL is returned.
* '{"a":[1,2,3,4,5]}'::jsonb @@ '$.a[*] > 2' → t
*
*/
public static CompoundPredicate doubleAt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.DOUBLE_AT, right);
}
/**
* @see tsvector @@@ tsquery → boolean
* tsquery @@@ tsvector → boolean
*
*/
public static CompoundPredicate tripleAt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.TRIPLE_AT, right);
}
/**
*
* The {@link MappingType} of operator return type: {@link BooleanType} .
*
*
* @param left not {@link SQLs#DEFAULT} etc.
* @see Expression#space(BiFunction, Object)
* @see SimpleExpression#space(BiFunction, BiFunction, Object)
* @see Postgres#startsWith(Expression, Expression)
* @see text ^@ text → boolean
*/
public static CompoundPredicate caretAt(Expression left, Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.CARET_AT, right);
}
/**
*
* The {@link MappingType} of function return type:{@link BooleanType}
*
*
* @see Postgres#regexpLike(Expression, Expression)
* @see Postgres#regexpLike(Expression, Expression, Expression)
* @see text ~ text → boolean
* String matches regular expression, case sensitively
*/
public static CompoundPredicate tilde(final Expression left, final Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.TILDE, right);
}
/**
*
* The {@link MappingType} of function return type:{@link BooleanType}
*
*
* @see Postgres#regexpLike(Expression, Expression)
* @see Postgres#regexpLike(Expression, Expression, Expression)
* @see text !~ text → boolean
* String does not match regular expression, case sensitively
*/
public static CompoundPredicate notTilde(final Expression left, final Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.NOT_TILDE, right);
}
/**
*
* The {@link MappingType} of function return type:{@link BooleanType}
*
*
* @see text ~* text → boolean
* String matches regular expression, case insensitively
*/
public static CompoundPredicate tildeStar(final Expression left, final Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.TILDE_STAR, right);
}
/**
*
* The {@link MappingType} of function return type:{@link BooleanType}
*
*
* @see text !~* text → boolean
* String does not match regular expression, case insensitively
*/
public static CompoundPredicate notTildeStar(final Expression left, final Expression right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.NOT_TILDE_STAR, right);
}
/**
*
* OVERLAPS operator
*
*
* @see OVERLAPS operato
*/
public static Postgres._PeriodOverlapsClause period(final Expression start, final Expression endOrLength) {
return PostgreExpressions.overlaps(start, endOrLength);
}
/**
*
* OVERLAPS operator
*
*
* @see OVERLAPS operato
*/
public static Postgres._PeriodOverlapsClause period(Expression start, BiFunction valueOperator, T value) {
return period(start, valueOperator.apply(start, value));
}
/**
*
* OVERLAPS operator
*
*
* @see OVERLAPS operato
*/
public static Postgres._PeriodOverlapsClause period(BiFunction valueOperator, T value, Expression endOrLength) {
return period(valueOperator.apply(endOrLength, value), endOrLength);
}
/**
*
* OVERLAPS operator
*
*
* @see OVERLAPS operato
*/
public static Postgres._PeriodOverlapsClause period(TypeInfer type, BiFunction valueOperator, Object start, Object endOrLength) {
return period(valueOperator.apply(type, start), valueOperator.apply(type, endOrLength));
}
/**
*
* AT TIME ZONE operator,The {@link MappingType} of operator return type:
*
* - If The {@link MappingType} of source is {@link MappingType.SqlLocalDateTimeType},then {@link OffsetDateTimeType}
* - If The {@link MappingType} of source is {@link MappingType.SqlOffsetDateTimeType},then {@link LocalDateTimeType}
* - If The {@link MappingType} of source is {@link MappingType.SqlLocalTimeType},then {@link OffsetTimeType}
* - If The {@link MappingType} of source is {@link MappingType.SqlOffsetTimeType},then {@link LocalTimeType}
* - Else raise {@link CriteriaException}
*
*
*
* @param source non-multi value parameter/literal
* @param zone non-multi value parameter/literal
* @throws CriteriaException throw when
* - source is multi value parameter/literal
* - zone is multi value parameter/literal
* - The {@link MappingType} of source error
*
* @see AT TIME ZONE Variants
*/
public static CompoundExpression atTimeZone(final Expression source, final Expression zone) {
return Expressions.dialectDualExp(source, DualExpOperator.AT_TIME_ZONE, zone, PostgreSyntax::atTimeZoneType);
}
/**
* @see SIMILAR TO Regular Expressions
*/
public static CompoundPredicate similarTo(Expression exp, Expression pattern) {
return Expressions.likePredicate(exp, DualBooleanOperator.SIMILAR_TO, pattern, SQLs.ESCAPE, null);
}
/**
* @see SIMILAR TO Regular Expressions
*/
public static CompoundPredicate similarTo(Expression exp, Expression pattern, SQLs.WordEscape escape, Expression escapeChar) {
return Expressions.likePredicate(exp, DualBooleanOperator.SIMILAR_TO, pattern, escape, escapeChar);
}
/**
* @see SIMILAR TO Regular Expressions
*/
public static CompoundPredicate notSimilarTo(Expression exp, Expression pattern) {
return Expressions.likePredicate(exp, DualBooleanOperator.NOT_SIMILAR_TO, pattern, SQLs.ESCAPE, null);
}
/**
* @see SIMILAR TO Regular Expressions
*/
public static CompoundPredicate notSimilarTo(Expression exp, Expression pattern, SQLs.WordEscape escape, Expression escapeChar) {
return Expressions.likePredicate(exp, DualBooleanOperator.NOT_SIMILAR_TO, pattern, escape, escapeChar);
}
/**
* @see row_constructor IS DISTINCT FROM row_constructor
*
*/
public static CompoundPredicate isDistinctFrom(T left, T right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.IS_DISTINCT_FROM, right);
}
/**
* @see row_constructor IS NOT DISTINCT FROM row_constructor
*
*/
public static CompoundPredicate isNotDistinctFrom(T left, T right) {
return PostgreExpressions.dualPredicate(left, PostgreDualBooleanOperator.IS_NOT_DISTINCT_FROM, right);
}
/**
* @param word see
* - {@link SQLs#ALL}
* - {@link SQLs#SOME}
* - {@link SQLs#ANY}
*
* @see ANY/SOME (array)
*/
public static CompoundPredicate equal(Expression left, SQLs.QuantifiedWord word, ArrayExpression array) {
return Expressions.compareQueryPredicate(left, DualBooleanOperator.EQUAL, word, array);
}
/**
* @param word see
* - {@link SQLs#ALL}
* - {@link SQLs#SOME}
* - {@link SQLs#ANY}
*
* @see ANY/SOME (array)
*/
public static CompoundPredicate notEqual(Expression left, SQLs.QuantifiedWord word, ArrayExpression array) {
return Expressions.compareQueryPredicate(left, DualBooleanOperator.NOT_EQUAL, word, array);
}
/**
* @param word see
* - {@link SQLs#ALL}
* - {@link SQLs#SOME}
* - {@link SQLs#ANY}
*
* @see ANY/SOME (array)
*/
public static CompoundPredicate less(Expression left, SQLs.QuantifiedWord word, ArrayExpression array) {
return Expressions.compareQueryPredicate(left, DualBooleanOperator.LESS, word, array);
}
/**
* @param word see
* - {@link SQLs#ALL}
* - {@link SQLs#SOME}
* - {@link SQLs#ANY}
*
* @see ANY/SOME (array)
*/
public static CompoundPredicate lessEqual(Expression left, SQLs.QuantifiedWord word, ArrayExpression array) {
return Expressions.compareQueryPredicate(left, DualBooleanOperator.LESS_EQUAL, word, array);
}
/**
* @param word see
* - {@link SQLs#ALL}
* - {@link SQLs#SOME}
* - {@link SQLs#ANY}
*
* @see ANY/SOME (array)
*/
public static CompoundPredicate greater(Expression left, SQLs.QuantifiedWord word, ArrayExpression array) {
return Expressions.compareQueryPredicate(left, DualBooleanOperator.GREATER, word, array);
}
/**
* @param word see
* - {@link SQLs#ALL}
* - {@link SQLs#SOME}
* - {@link SQLs#ANY}
*
* @see ANY/SOME (array)
*/
public static CompoundPredicate greaterEqual(Expression left, SQLs.QuantifiedWord word, ArrayExpression array) {
return Expressions.compareQueryPredicate(left, DualBooleanOperator.GREATER_EQUAL, word, array);
}
/**
* @see Collation Expressions
*/
public static SimpleResultExpression collate(Expression expr, String collation) {
return Expressions.collateExp(expr, collation);
}
/**
* @see grouping_element
*/
public static GroupByItem.ExpressionGroup parens() {
return Expressions.emptyParens();
}
/**
* @see grouping_element
*/
public static GroupByItem.ExpressionGroup parens(Expression exp) {
return (GroupByItem.ExpressionGroup) Expressions.parens(null, exp);
}
/**
* @see grouping_element
*/
public static GroupByItem.ExpressionGroup parens(Expression exp1, Expression exp2) {
return (GroupByItem.ExpressionGroup) Expressions.parens(null, exp1, exp2);
}
/**
* @see grouping_element
*/
public static GroupByItem.ExpressionGroup parens(Expression exp1, Expression exp2, Expression exp3,
Expression... rest) {
return (GroupByItem.ExpressionGroup) Expressions.parens(null, exp1, exp2, exp3, rest);
}
/**
* @see grouping_element
*/
public static GroupByItem.ExpressionGroup parens(Consumer> consumer) {
return (GroupByItem.ExpressionGroup) Expressions.parens(null, consumer);
}
/**
* @see grouping_element
*/
public static GroupByItem rollup(GroupByItem.ExpressionItem exp) {
return Expressions.parens(Expressions.GroupingModifier.ROLLUP, exp);
}
/**
* @see grouping_element
*/
public static GroupByItem rollup(GroupByItem.ExpressionItem exp1, GroupByItem.ExpressionItem exp2) {
return Expressions.parens(Expressions.GroupingModifier.ROLLUP, exp1, exp2);
}
/**
* @see grouping_element
*/
public static GroupByItem rollup(GroupByItem.ExpressionItem exp1, GroupByItem.ExpressionItem exp2,
GroupByItem.ExpressionItem exp3, GroupByItem.ExpressionItem... rest) {
return Expressions.parens(Expressions.GroupingModifier.ROLLUP, exp1, exp2, exp3, rest);
}
/**
* @see grouping_element
*/
public static GroupByItem rollup(Consumer> consumer) {
return Expressions.parens(Expressions.GroupingModifier.ROLLUP, consumer);
}
/**
* @see grouping_element
*/
public static GroupByItem cube(GroupByItem.ExpressionItem exp) {
return Expressions.parens(Expressions.GroupingModifier.CUBE, exp);
}
/**
* @see grouping_element
*/
public static GroupByItem cube(GroupByItem.ExpressionItem exp1, GroupByItem.ExpressionItem exp2) {
return Expressions.parens(Expressions.GroupingModifier.CUBE, exp1, exp2);
}
/**
* @see grouping_element
*/
public static GroupByItem cube(GroupByItem.ExpressionItem exp1, GroupByItem.ExpressionItem exp2,
GroupByItem.ExpressionItem exp3, GroupByItem.ExpressionItem... rest) {
return Expressions.parens(Expressions.GroupingModifier.CUBE, exp1, exp2, exp3, rest);
}
/**
* @see grouping_element
*/
public static GroupByItem cube(Consumer> consumer) {
return Expressions.parens(Expressions.GroupingModifier.CUBE, consumer);
}
/**
* @see grouping_element
*/
public static GroupByItem groupingSets(GroupByItem item) {
return Expressions.parens(Expressions.GroupingModifier.GROUPING_SETS, item);
}
/**
* @see grouping_element
*/
public static GroupByItem groupingSets(GroupByItem item1, GroupByItem item2) {
return Expressions.parens(Expressions.GroupingModifier.GROUPING_SETS, item1, item2);
}
/**
* @see grouping_element
*/
public static GroupByItem groupingSets(GroupByItem item1, GroupByItem item2, GroupByItem item3, GroupByItem... rest) {
return Expressions.parens(Expressions.GroupingModifier.GROUPING_SETS, item1, item2, item3, rest);
}
/**
* @see grouping_element
*/
public static GroupByItem groupingSets(Consumer> consumer) {
return Expressions.parens(Expressions.GroupingModifier.GROUPING_SETS, consumer);
}
/**
* @see ROWS FROM
*/
public static _TabularWithOrdinalityFunction rowsFrom(Consumer consumer) {
return PostgreFunctionUtils.rowsFrom(consumer);
}
/**
* @see ROWS FROM
*/
public static _TabularWithOrdinalityFunction rowsFrom(SQLs.SymbolSpace space, Consumer consumer) {
return PostgreFunctionUtils.rowsFrom(space, consumer);
}
/*-------------------below package method -------------------*/
static String keyWordToString(Enum> keyWordEnum) {
return _StringUtils.builder()
.append(Postgres.class.getSimpleName())
.append(_Constant.PERIOD)
.append(keyWordEnum.name())
.toString();
}
/*-------------------below private method -------------------*/
/**
* @see #atTimeZone(Expression, Expression)
*/
private static MappingType atTimeZoneType(final MappingType left, final MappingType right) {
final MappingType returnType;
if (left instanceof MappingType.SqlLocalDateTimeType) {
returnType = OffsetDateTimeType.INSTANCE;
} else if (left instanceof MappingType.SqlOffsetDateTimeType) {
returnType = LocalDateTimeType.INSTANCE;
} else if (left instanceof MappingType.SqlLocalTimeType) {
returnType = OffsetTimeType.INSTANCE;
} else if (left instanceof MappingType.SqlOffsetTimeType) {
returnType = LocalTimeType.INSTANCE;
} else {
String m = String.format("AT TIME ZONE operator don't support %s", left);
throw ContextStack.clearStackAndCriteriaError(m);
}
return returnType;
}
/**
* @see #caret(Expression, Expression)
*/
private static MappingType caretResultType(final MappingType left, final MappingType right) {
final MappingType returnType;
if (left instanceof MappingType.SqlIntegerOrDecimalType
&& right instanceof MappingType.SqlIntegerOrDecimalType) {
returnType = BigDecimalType.INSTANCE;
} else {
returnType = DoubleType.INSTANCE;
}
return returnType;
}
}