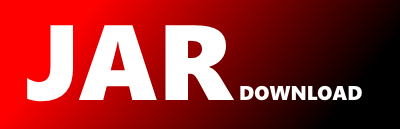
io.army.criteria.impl.PostgreWindowFunctions Maven / Gradle / Ivy
package io.army.criteria.impl;
import io.army.criteria.*;
import io.army.criteria.dialect.Window;
import io.army.criteria.postgre.PostgreWindow;
import io.army.criteria.standard.SQLFunction;
import io.army.mapping.*;
import io.army.mapping.array.DoubleArrayType;
import io.army.mapping.array.IntervalArrayType;
import io.army.mapping.optional.IntervalType;
import io.army.meta.TypeMeta;
import javax.annotation.Nullable;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Consumer;
/**
*
* Package class,This class hold window function and Aggregate function method.
*
* @see Window Functions tutorial
* @see Window Function synatx
* @see Window Functions list
* @see Window Function Processing
* @see Aggregate Functions tutorial
* @see Aggregate function syntax
* @see General-Purpose Aggregate Functions list
* @see Aggregate Functions for Statistics list
* @see Ordered-Set Aggregate Functions list
* @see Hypothetical-Set Aggregate Functions list
* @since 1.0
*/
abstract class PostgreWindowFunctions extends PostgreDocumentFunctions {
PostgreWindowFunctions() {
}
/**
*
* This interface representing postgre over clause.
*
*/
public interface _OverSpec extends Window._OverWindowClause {
}
/**
*
* This interface representing postgre aggregate function over clause.
*
*/
public interface _PgAggWindowFuncSpec extends _OverSpec,
SQLFunction._OuterClauseBeforeOver,
SQLFunction.AggregateFunction,
SimpleExpression {
}
/**
*
* This interface representing postgre aggregate function filter clause.
*
*/
interface _PgAggFuncFilterClause {
R filter(Consumer consumer);
R ifFilter(Consumer consumer);
}
/**
*
* This interface representing postgre aggregate function.
*
*/
public interface _PostgreAggregateFunction extends SimpleExpression,
_PgAggFuncFilterClause,
SQLFunction.AggregateFunction {
}
/**
*
* This interface is base interface of postgre window aggregate function.
*
*/
public interface _AggWindowFunc extends _PostgreAggregateFunction<_PgAggWindowFuncSpec>, _PgAggWindowFuncSpec {
}
/**
*
* This interface is base interface of postgre non-window aggregate function.
*
*/
public interface _PgAggFunc extends _PostgreAggregateFunction {
}
/**
*
* This interface representing postgre ordered-set aggregate function. This interface couldn't extends {@link _PgAggFunc}
*
*/
public interface _AggWithGroupClause {
_PgAggFunc withinGroup(Consumer consumer);
}
/*-------------------below window function-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see row_number () → bigint
* Returns the number of the current row within its partition, counting from 1.
*
*/
public static _OverSpec rowNumber() {
return PostgreFunctionUtils.zeroArgWindowFunc("row_number", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see rank () → bigint
* Returns the rank of the current row, with gaps; that is, the row_number of the first row in its peer group.
*
*/
public static _OverSpec rank() {
return PostgreFunctionUtils.zeroArgWindowFunc("rank", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see dense_rank () → bigint
* Returns the rank of the current row, without gaps; this function effectively counts peer groups.
*
*/
public static _OverSpec denseRank() {
return PostgreFunctionUtils.zeroArgWindowFunc("dense_rank", LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}
*
*
* @see percent_rank () → double precision
* Returns the relative rank of the current row, that is (rank - 1) / (total partition rows - 1). The value thus ranges from 0 to 1 inclusive.
*
*/
public static _OverSpec percentRank() {
return PostgreFunctionUtils.zeroArgWindowFunc("percent_rank", DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}
*
*
* @see cume_dist () → double precision
* Returns the cumulative distribution, that is (number of partition rows preceding or peers with current row) / (total partition rows). The value thus ranges from 1/N to 1.
*
*/
public static _OverSpec cumeDist() {
return PostgreFunctionUtils.zeroArgWindowFunc("cume_dist", DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @param func the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of func always is {@link IntegerType#INSTANCE}.
* @param value non-null,it will be passed to func as the second argument of func
* @see #ntile(Expression)
* @see ntile ( num_buckets integer ) → integer
* Returns an integer ranging from 1 to the argument value, dividing the partition as equally as possible.
*
*/
public static _OverSpec ntile(BiFunction func, T value) {
return ntile(func.apply(IntegerType.INSTANCE, value));
}
/**
*
* The {@link MappingType} of function return type: {@link IntegerType#INSTANCE}
*
*
* @see ntile ( num_buckets integer ) → integer
* Returns an integer ranging from 1 to the argument value, dividing the partition as equally as possible.
*
*/
public static _OverSpec ntile(Expression numBuckets) {
return PostgreFunctionUtils.oneArgWindowFunc("ntile", numBuckets, IntegerType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see lag ( value anycompatible [, offset integer [, default anycompatible ]] ) → anycompatible
* Returns value evaluated at the row that is offset rows before the current row within the partition; if there is no such row,
* instead returns default (which must be of a type compatible with value).
* Both offset and default are evaluated with respect to the current row. If omitted, offset defaults to 1 and default to NULL.
*
*/
public static _OverSpec lag(Expression value) {
return PostgreFunctionUtils.oneArgWindowFunc("lag", value, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see lag ( value anycompatible [, offset integer [, default anycompatible ]] ) → anycompatible
* Returns value evaluated at the row that is offset rows before the current row within the partition; if there is no such row,
* instead returns default (which must be of a type compatible with value).
* Both offset and default are evaluated with respect to the current row. If omitted, offset defaults to 1 and default to NULL.
*
*/
public static _OverSpec lag(Expression value, Expression offset) {
return PostgreFunctionUtils.twoArgWindowFunc("lag", value, offset, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see lag ( value anycompatible [, offset integer [, default anycompatible ]] ) → anycompatible
* Returns value evaluated at the row that is offset rows before the current row within the partition; if there is no such row,
* instead returns default (which must be of a type compatible with value).
* Both offset and default are evaluated with respect to the current row. If omitted, offset defaults to 1 and default to NULL.
*
*/
public static _OverSpec lag(Expression value, Expression offset, Expression defaultValue) {
return PostgreFunctionUtils.threeArgWindowFunc("lag", value, offset, defaultValue,
_returnType(value, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see lead ( value anycompatible [, offset integer [, default anycompatible ]] ) → anycompatible
* Returns value evaluated at the row that is offset rows after the current row within the partition;
* if there is no such row, instead returns default (which must be of a type compatible with value).
* Both offset and default are evaluated with respect to the current row. If omitted, offset defaults to 1 and default to NULL.
*
*/
public static _OverSpec lead(Expression value) {
return PostgreFunctionUtils.oneArgWindowFunc("lead", value, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see lead ( value anycompatible [, offset integer [, default anycompatible ]] ) → anycompatible
* Returns value evaluated at the row that is offset rows after the current row within the partition;
* if there is no such row, instead returns default (which must be of a type compatible with value).
* Both offset and default are evaluated with respect to the current row. If omitted, offset defaults to 1 and default to NULL.
*
*/
public static _OverSpec lead(Expression value, Expression offset) {
return PostgreFunctionUtils.twoArgWindowFunc("lead", value, offset, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see lead ( value anycompatible [, offset integer [, default anycompatible ]] ) → anycompatible
* Returns value evaluated at the row that is offset rows after the current row within the partition;
* if there is no such row, instead returns default (which must be of a type compatible with value).
* Both offset and default are evaluated with respect to the current row. If omitted, offset defaults to 1 and default to NULL.
*
*/
public static _OverSpec lead(Expression value, Expression offset, Expression defaultValue) {
return PostgreFunctionUtils.threeArgWindowFunc("lead", value, offset, defaultValue,
_returnType(value, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see first_value ( value anyelement ) → anyelement
* Returns value evaluated at the row that is the first row of the window frame.
*
*/
public static _OverSpec firstValue(Expression value) {
return PostgreFunctionUtils.oneArgWindowFunc("first_value", value, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see last_value ( value anyelement ) → anyelement
* Returns value evaluated at the row that is the last row of the window frame.
*
*/
public static _OverSpec lastValue(Expression value) {
return PostgreFunctionUtils.oneArgWindowFunc("last_value", value, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @param func the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of func always is {@link IntegerType#INSTANCE}.
* @param n non-null,it will be passed to func as the second argument of func
* @see #nthValue(Expression, Expression)
* @see nth_value ( value anyelement, n integer ) → anyelement
* Returns value evaluated at the row that is the n'th row of the window frame (counting from 1); returns NULL if there is no such row.
*
*/
public static _OverSpec nthValue(Expression value, BiFunction func, T n) {
return nthValue(value, func.apply(IntegerType.INSTANCE, n));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of value
*
*
* @see nth_value ( value anyelement, n integer ) → anyelement
* Returns value evaluated at the row that is the n'th row of the window frame (counting from 1); returns NULL if there is no such row.
*
*/
public static _OverSpec nthValue(Expression value, Expression n) {
return PostgreFunctionUtils.twoArgWindowFunc("nth_value", value, n, _returnType(value, Expressions::identityType));
}
/*-------------------below general-purpose Aggregate Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: the array {@link MappingType} of any
*
*
* @param modifier see {@link SQLs#DISTINCT} or {@link Postgres#DISTINCT}
* @see array_agg ( anynonarray ) → anyarray
* Collects all the input values, including nulls, into an array.
*
*/
public static _PgAggFunc arrayAgg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("array_agg", modifier, any, null, _returnType(any, MappingType::arrayTypeOfThis));
}
/**
*
* The {@link MappingType} of function return type: the array {@link MappingType} of any
*
*
* @see array_agg ( anynonarray ) → anyarray
* Collects all the input values, including nulls, into an array.
*
*/
public static _PgAggFunc arrayAgg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("array_agg", null, any, consumer, _returnType(any, MappingType::arrayTypeOfThis));
}
/**
*
* The {@link MappingType} of function return type: the array {@link MappingType} of any
*
*
* @param modifier see {@link SQLs#DISTINCT} or {@link Postgres#DISTINCT}
* @see array_agg ( anynonarray ) → anyarray
* Collects all the input values, including nulls, into an array.
*
*/
public static _PgAggFunc arrayAgg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("array_agg", modifier, any, consumer, _returnType(any, MappingType::arrayTypeOfThis));
}
/**
*
* The {@link MappingType} of function return type: the array {@link MappingType} of any
*
*
* @see array_agg ( anynonarray ) → anyarray
* Collects all the input values, including nulls, into an array.
*
*/
public static _AggWindowFunc arrayAgg(Expression any) {
return PostgreFunctionUtils.oneArgAggWindowFunc("array_agg", any,
_returnType(any, MappingType::arrayTypeOfThis)
);
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → {@link BigDecimalType#INSTANCE}
* - exp is decimal type → {@link BigDecimalType#INSTANCE}
* - exp is float type → {@link DoubleType#INSTANCE}
* - exp is interval type → {@link IntervalType#TEXT}
* - else → {@link TextType#INSTANCE}
*
*
*
* @see avg ( smallint ) → numeric
* avg ( integer ) → numeric
* avg ( bigint ) → numeric
* avg ( numeric ) → numeric
* avg ( real ) → double precision
* avg ( double precision ) → double precision
* avg ( interval ) → interval
*
*/
public static _PgAggFunc avg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("avg", modifier, any, null, _returnType(any, PostgreWindowFunctions::_avgType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → {@link BigDecimalType#INSTANCE}
* - exp is decimal type → {@link BigDecimalType#INSTANCE}
* - exp is float type → {@link DoubleType#INSTANCE}
* - exp is interval type → {@link IntervalType#TEXT}
* - else → {@link TextType#INSTANCE}
*
*
*
* @see avg ( smallint ) → numeric
* avg ( integer ) → numeric
* avg ( bigint ) → numeric
* avg ( numeric ) → numeric
* avg ( real ) → double precision
* avg ( double precision ) → double precision
* avg ( interval ) → interval
*
*/
public static _PgAggFunc avg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("avg", null, any, consumer, _returnType(any, PostgreWindowFunctions::_avgType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → {@link BigDecimalType#INSTANCE}
* - exp is decimal type → {@link BigDecimalType#INSTANCE}
* - exp is float type → {@link DoubleType#INSTANCE}
* - exp is interval type → {@link IntervalType#TEXT}
* - else → {@link TextType#INSTANCE}
*
*
*
* @see avg ( smallint ) → numeric
* avg ( integer ) → numeric
* avg ( bigint ) → numeric
* avg ( numeric ) → numeric
* avg ( real ) → double precision
* avg ( double precision ) → double precision
* avg ( interval ) → interval
*
*/
public static _PgAggFunc avg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("avg", modifier, any, consumer, _returnType(any, PostgreWindowFunctions::_avgType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → {@link BigDecimalType#INSTANCE}
* - exp is decimal type → {@link BigDecimalType#INSTANCE}
* - exp is float type → {@link DoubleType#INSTANCE}
* - exp is interval type → {@link IntervalType#TEXT}
* - else → {@link TextType#INSTANCE}
*
*
*
* @see avg ( smallint ) → numeric
* avg ( integer ) → numeric
* avg ( bigint ) → numeric
* avg ( numeric ) → numeric
* avg ( real ) → double precision
* avg ( double precision ) → double precision
* avg ( interval ) → interval
*
*/
public static _AggWindowFunc avg(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("avg", exp, _returnType(exp, PostgreWindowFunctions::_avgType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_and ( smallint ) → smallint
* bit_and ( integer ) → integer
* bit_and ( bigint ) → bigint
* bit_and ( bit ) → bit
* Computes the bitwise AND of all non-null input values.
*
*/
public static _PgAggFunc bitAnd(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("bit_and", modifier, any, null, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_and ( smallint ) → smallint
* bit_and ( integer ) → integer
* bit_and ( bigint ) → bigint
* bit_and ( bit ) → bit
* Computes the bitwise AND of all non-null input values.
*
*/
public static _PgAggFunc bitAnd(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bit_and", null, any, consumer, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_and ( smallint ) → smallint
* bit_and ( integer ) → integer
* bit_and ( bigint ) → bigint
* bit_and ( bit ) → bit
* Computes the bitwise AND of all non-null input values.
*
*/
public static _PgAggFunc bitAnd(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bit_and", modifier, any, consumer, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_and ( smallint ) → smallint
* bit_and ( integer ) → integer
* bit_and ( bigint ) → bigint
* bit_and ( bit ) → bit
* Computes the bitwise AND of all non-null input values.
*
*/
public static _AggWindowFunc bitAnd(Expression exp) {
return _bitWindowFunc("bit_and", exp);
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_or ( smallint ) → smallint
* bit_or ( integer ) → integer
* bit_or ( bigint ) → bigint
* bit_or ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _PgAggFunc bitOr(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("bit_or", modifier, any, null, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_or ( smallint ) → smallint
* bit_or ( integer ) → integer
* bit_or ( bigint ) → bigint
* bit_or ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _PgAggFunc bitOr(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bit_or", null, any, consumer, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_or ( smallint ) → smallint
* bit_or ( integer ) → integer
* bit_or ( bigint ) → bigint
* bit_or ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _PgAggFunc bitOr(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bit_or", modifier, any, consumer, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_or ( smallint ) → smallint
* bit_or ( integer ) → integer
* bit_or ( bigint ) → bigint
* bit_or ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _AggWindowFunc bitOr(Expression exp) {
return _bitWindowFunc("bit_or", exp);
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_xor ( smallint ) → smallint
* bit_xor ( integer ) → integer
* bit_xor ( bigint ) → bigint
* bit_xor ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _PgAggFunc bitXor(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("bit_xor", modifier, any, null, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_xor ( smallint ) → smallint
* bit_xor ( integer ) → integer
* bit_xor ( bigint ) → bigint
* bit_xor ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _PgAggFunc bitXor(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bit_xor", null, any, consumer, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_xor ( smallint ) → smallint
* bit_xor ( integer ) → integer
* bit_xor ( bigint ) → bigint
* bit_xor ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _PgAggFunc bitXor(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bit_xor", modifier, any, consumer, _returnType(any, PostgreWindowFunctions::_bitOpeType));
}
/**
*
* The {@link MappingType} of function return type:
* - exp is integer type → the {@link MappingType} of exp
* - exp is bit type → the {@link MappingType} of exp
* - else → {@link TextType#INSTANCE}
*
*
*
* @see bit_xor ( smallint ) → smallint
* bit_xor ( integer ) → integer
* bit_xor ( bigint ) → bigint
* bit_xor ( bit ) → bit
* Computes the bitwise OR of all non-null input values.
*
*/
public static _AggWindowFunc bitXor(Expression exp) {
return _bitWindowFunc("bit_xor", exp);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_and ( boolean ) → boolean
* Returns true if all non-null input values are true, otherwise false.
*
*/
public static _PgAggFunc boolAnd(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("bool_and", modifier, any, null, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_and ( boolean ) → boolean
* Returns true if all non-null input values are true, otherwise false.
*
*/
public static _PgAggFunc boolAnd(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bool_and", null, any, consumer, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_and ( boolean ) → boolean
* Returns true if all non-null input values are true, otherwise false.
*
*/
public static _PgAggFunc boolAnd(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bool_and", modifier, any, consumer, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_and ( boolean ) → boolean
* Returns true if all non-null input values are true, otherwise false.
*
*/
public static _AggWindowFunc boolAnd(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("bool_and", exp, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_or ( boolean ) → boolean
* Returns true if any non-null input value is true, otherwise false.
*
*/
public static _PgAggFunc boolOr(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("bool_or", modifier, any, null, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_or ( boolean ) → boolean
* Returns true if any non-null input value is true, otherwise false.
*
*/
public static _PgAggFunc boolOr(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bool_or", null, any, consumer, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_or ( boolean ) → boolean
* Returns true if any non-null input value is true, otherwise false.
*
*/
public static _PgAggFunc boolOr(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("bool_or", modifier, any, consumer, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see bool_or ( boolean ) → boolean
* Returns true if any non-null input value is true, otherwise false.
*
*/
public static _AggWindowFunc boolOr(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("bool_or", exp, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see count ( * ) → bigint
* Computes the number of input rows.
*
* @see #countAsterisk()
*/
public static _AggWindowFunc countAsterisk() {
return PostgreFunctionUtils.oneArgAggWindowFunc("count", SQLs._ASTERISK_EXP, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see count ( "any" ) → bigint
* Computes the number of input rows in which the input value is not null.
*
* @see #count(Expression)
*/
public static _PgAggFunc count(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("count", modifier, any, null, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see count ( "any" ) → bigint
* Computes the number of input rows in which the input value is not null.
*
* @see #count(Expression)
*/
public static _PgAggFunc count(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("count", null, any, consumer, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see count ( "any" ) → bigint
* Computes the number of input rows in which the input value is not null.
*
* @see #count(Expression)
*/
public static _PgAggFunc count(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("count", modifier, any, consumer, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see count ( "any" ) → bigint
* Computes the number of input rows in which the input value is not null.
*
* @see #count(Expression)
*/
public static _AggWindowFunc count(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("count", exp, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see every ( boolean ) → boolean
* This is the SQL standard's equivalent to bool_and.
*
* @see #boolAnd(Expression)
*/
public static _PgAggFunc every(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("every", modifier, any, null, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see every ( boolean ) → boolean
* This is the SQL standard's equivalent to bool_and.
*
* @see #boolAnd(Expression)
*/
public static _PgAggFunc every(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("every", null, any, consumer, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see every ( boolean ) → boolean
* This is the SQL standard's equivalent to bool_and.
*
* @see #boolAnd(Expression)
*/
public static _PgAggFunc every(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("every", modifier, any, consumer, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link BooleanType#INSTANCE}.
*
*
* @see every ( boolean ) → boolean
* This is the SQL standard's equivalent to bool_and.
*
* @see #boolAnd(Expression)
*/
public static _AggWindowFunc every(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("every", exp, BooleanType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_agg ( anyelement ) → json
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _PgAggFunc jsonAgg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("json_agg", modifier, any, null, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_agg ( anyelement ) → json
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _PgAggFunc jsonAgg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("json_agg", null, any, consumer, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_agg ( anyelement ) → json
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _PgAggFunc jsonAgg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("json_agg", modifier, any, consumer, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_agg ( anyelement ) → json
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _AggWindowFunc jsonAgg(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("json_agg", exp, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_agg ( anyelement ) → jsonb_agg
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _PgAggFunc jsonbAgg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("jsonb_agg", modifier, any, null, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_agg ( anyelement ) → jsonb_agg
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _PgAggFunc jsonbAgg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("jsonb_agg", null, any, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_agg ( anyelement ) → jsonb_agg
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _PgAggFunc jsonbAgg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("jsonb_agg", modifier, any, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_agg ( anyelement ) → jsonb_agg
* Collects all the input values, including nulls, into a JSON array. Values are converted to JSON as per to_json or to_jsonb.
*
*/
public static _AggWindowFunc jsonbAgg(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("jsonb_agg", exp, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _PgAggFunc jsonObjectAgg(@Nullable SQLs.ArgDistinct modifier, Expression key, Expression value) {
return PostgreFunctionUtils.twoArgAggFunc("json_object_agg", modifier, key, value, null, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _PgAggFunc jsonObjectAgg(Expression key, Expression value, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("json_object_agg", null, key, value, consumer, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _PgAggFunc jsonObjectAgg(@Nullable SQLs.ArgDistinct modifier, Expression key, Expression value,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("json_object_agg", modifier, key, value, consumer, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @param keyFunc the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of keyFunc always is {@link TextType#INSTANCE}.
* @param key non-null,it will be passed to keyFunc as the second argument of keyFunc
* @param valueFunc the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of valueFunc always is {@link TextType#INSTANCE}.
* @param value non-null,it will be passed to valueFunc as the second argument of valueFunc
* @see #jsonObjectAgg(Expression, Expression)
* @see json_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _AggWindowFunc jsonObjectAgg(BiFunction keyFunc, K key,
BiFunction valueFunc, V value) {
return jsonObjectAgg(keyFunc.apply(TextType.INSTANCE, key), valueFunc.apply(TextType.INSTANCE, value));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonType#TEXT}.
*
*
* @see json_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _AggWindowFunc jsonObjectAgg(Expression key, Expression value) {
return PostgreFunctionUtils.twoArgAggWindowFunc("json_object_agg", key, value, JsonType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _PgAggFunc jsonbObjectAgg(@Nullable SQLs.ArgDistinct modifier, Expression key, Expression value) {
return PostgreFunctionUtils.twoArgAggFunc("jsonb_object_agg", modifier, key, value, null, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _PgAggFunc jsonbObjectAgg(Expression key, Expression value, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("jsonb_object_agg", null, key, value, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _PgAggFunc jsonbObjectAgg(@Nullable SQLs.ArgDistinct modifier, Expression key, Expression value,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("jsonb_object_agg", modifier, key, value, consumer, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @param keyFunc the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of keyFunc always is {@link TextType#INSTANCE}.
* @param key non-null,it will be passed to keyFunc as the second argument of keyFunc
* @param valueFunc the reference of method,Note: it's the reference of method,not lambda. Valid method:
*
* - {@link SQLs#param(TypeInfer, Object)}
* - {@link SQLs#literal(TypeInfer, Object)}
* - {@link SQLs#namedParam(TypeInfer, String)} ,used only in INSERT( or batch update/delete ) syntax
* - {@link SQLs#namedLiteral(TypeInfer, String)} ,used only in INSERT( or batch update/delete in multi-statement) syntax
* - developer custom method
*
.
* The first argument of valueFunc always is {@link TextType#INSTANCE}.
* @param value non-null,it will be passed to valueFunc as the second argument of valueFunc
* @see #jsonbObjectAgg(Expression, Expression)
* @see json_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _AggWindowFunc jsonbObjectAgg(BiFunction keyFunc, K key,
BiFunction valueFunc, V value) {
return jsonbObjectAgg(keyFunc.apply(TextType.INSTANCE, key), valueFunc.apply(TextType.INSTANCE, value));
}
/**
*
* The {@link MappingType} of function return type: {@link JsonbType#TEXT}.
*
*
* @see jsonb_object_agg ( key "any", value "any" ) → json
* Collects all the key/value pairs into a JSON object. Key arguments are coerced to text; value arguments are converted as per to_json or to_jsonb. Values can be null, but not keys.
*
*/
public static _AggWindowFunc jsonbObjectAgg(Expression key, Expression value) {
return PostgreFunctionUtils.twoArgAggWindowFunc("jsonb_object_agg", key, value, JsonbType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see max ( see text ) → same as input type
* Computes the maximum of the non-null input values. Available for any numeric, string, date/time, or enum type, as well as inet, interval, money, oid, pg_lsn, tid, xid8, and arrays of any of these types.
*
*/
public static _PgAggFunc max(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("max", modifier, exp, null, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see max ( see text ) → same as input type
* Computes the maximum of the non-null input values. Available for any numeric, string, date/time, or enum type, as well as inet, interval, money, oid, pg_lsn, tid, xid8, and arrays of any of these types.
*
*/
public static _AggWindowFunc max(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("max", exp, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see min ( see text ) → same as input type
* Computes the minimum of the non-null input values. Available for any numeric, string, date/time, or enum type, as well as inet, interval, money, oid, pg_lsn, tid, xid8, and arrays of any of these types.
*
*/
public static _PgAggFunc min(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("min", modifier, exp, null, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see min ( see text ) → same as input type
* Computes the minimum of the non-null input values. Available for any numeric, string, date/time, or enum type, as well as inet, interval, money, oid, pg_lsn, tid, xid8, and arrays of any of these types.
*
*/
public static _AggWindowFunc min(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("min", exp, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_agg ( value anyrange ) → anymultirange
* range_agg ( value anymultirange ) → anymultirange
* Computes the union of the non-null input values.
*
*/
public static _PgAggFunc rangeAgg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("range_agg", modifier, any, null,
_returnType(any, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_agg ( value anyrange ) → anymultirange
* range_agg ( value anymultirange ) → anymultirange
* Computes the union of the non-null input values.
*
*/
public static _PgAggFunc rangeAgg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("range_agg", null, any, consumer,
_returnType(any, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_agg ( value anyrange ) → anymultirange
* range_agg ( value anymultirange ) → anymultirange
* Computes the union of the non-null input values.
*
*/
public static _PgAggFunc rangeAgg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("range_agg", modifier, any, consumer,
_returnType(any, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_agg ( value anyrange ) → anymultirange
* range_agg ( value anymultirange ) → anymultirange
* Computes the union of the non-null input values.
*
*/
public static _AggWindowFunc rangeAgg(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("range_agg", exp, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_intersect_agg ( value anyrange ) → anymultirange
* range_intersect_agg ( value anymultirange ) → anymultirange
* Computes the intersection of the non-null input values.
*
*/
public static _PgAggFunc rangeIntersectAgg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("range_intersect_agg", modifier, any, null,
_returnType(any, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_intersect_agg ( value anyrange ) → anymultirange
* range_intersect_agg ( value anymultirange ) → anymultirange
* Computes the intersection of the non-null input values.
*
*/
public static _PgAggFunc rangeIntersectAgg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("range_intersect_agg", null, any, consumer,
_returnType(any, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_intersect_agg ( value anyrange ) → anymultirange
* range_intersect_agg ( value anymultirange ) → anymultirange
* Computes the intersection of the non-null input values.
*
*/
public static _PgAggFunc rangeIntersectAgg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("range_intersect_agg", modifier, any, consumer,
_returnType(any, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see range_intersect_agg ( value anyrange ) → anymultirange
* range_intersect_agg ( value anymultirange ) → anymultirange
* Computes the intersection of the non-null input values.
*
*/
public static _AggWindowFunc rangeIntersectAgg(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("range_intersect_agg", exp, _returnType(exp, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see string_agg ( value text, delimiter text ) → text
* string_agg ( value bytea, delimiter bytea ) → bytea
* Concatenates the non-null input values into a string. Each value after the first is preceded by the corresponding delimiter (if it's not null).
*
*/
public static _PgAggFunc stringAgg(@Nullable SQLs.ArgDistinct modifier, Expression value, Expression delimiter) {
return PostgreFunctionUtils.twoArgAggFunc("string_agg", modifier, value, delimiter, null,
_returnType(value, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see string_agg ( value text, delimiter text ) → text
* string_agg ( value bytea, delimiter bytea ) → bytea
* Concatenates the non-null input values into a string. Each value after the first is preceded by the corresponding delimiter (if it's not null).
*
*/
public static _PgAggFunc stringAgg(Expression value, Expression delimiter, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("string_agg", null, value, delimiter, consumer,
_returnType(value, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see string_agg ( value text, delimiter text ) → text
* string_agg ( value bytea, delimiter bytea ) → bytea
* Concatenates the non-null input values into a string. Each value after the first is preceded by the corresponding delimiter (if it's not null).
*
*/
public static _PgAggFunc stringAgg(@Nullable SQLs.ArgDistinct modifier, Expression value, Expression delimiter,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("string_agg", modifier, value, delimiter, consumer,
_returnType(value, Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of exp.
*
*
* @see string_agg ( value text, delimiter text ) → text
* string_agg ( value bytea, delimiter bytea ) → bytea
* Concatenates the non-null input values into a string. Each value after the first is preceded by the corresponding delimiter (if it's not null).
*
*/
public static _AggWindowFunc stringAgg(Expression value, Expression delimiter) {
return PostgreFunctionUtils.twoArgAggWindowFunc("string_agg", value, delimiter, _returnType(value, Expressions::identityType));
}
/**
*
* The {@link MappingType} of function return type:
*
* - If exp is {@link ByteType},then {@link ShortType}
* - Else if exp is {@link ShortType},then {@link IntegerType}
* - Else if exp is {@link MediumIntType},then {@link IntegerType}
* - Else if exp is {@link LongType},then {@link BigIntegerType}
* - Else if exp is {@link BigDecimalType},then {@link BigDecimalType}
* - Else if exp is {@link FloatType},then {@link FloatType}
* - Else if exp is sql float type,then {@link DoubleType}
* - Else he {@link MappingType} of exp
*
*
*
* @see sum ( smallint ) → bigint
* sum ( integer ) → bigint
* sum ( bigint ) → numeric
* sum ( numeric ) → numeric
* sum ( real ) → real
* sum ( double precision ) → double precision
* sum ( interval ) → interval
* sum ( money ) → money
* Computes the sum of the non-null input values.
*
*/
public static _PgAggFunc sum(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("sum", modifier, exp, null, _returnType(exp, Functions::_sumType));
}
/**
*
* The {@link MappingType} of function return type:
*
* - If exp is {@link ByteType},then {@link ShortType}
* - Else if exp is {@link ShortType},then {@link IntegerType}
* - Else if exp is {@link MediumIntType},then {@link IntegerType}
* - Else if exp is {@link LongType},then {@link BigIntegerType}
* - Else if exp is {@link BigDecimalType},then {@link BigDecimalType}
* - Else if exp is {@link FloatType},then {@link FloatType}
* - Else if exp is sql float type,then {@link DoubleType}
* - Else he {@link MappingType} of exp
*
*
*
* @see sum ( smallint ) → bigint
* sum ( integer ) → bigint
* sum ( bigint ) → numeric
* sum ( numeric ) → numeric
* sum ( real ) → real
* sum ( double precision ) → double precision
* sum ( interval ) → interval
* sum ( money ) → money
* Computes the sum of the non-null input values.
*
*/
public static _AggWindowFunc sum(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("sum", exp, _returnType(exp, Functions::_sumType));
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}.
*
*
* @see xmlagg ( xml ) → xml
* Concatenates the non-null XML input values
*
*/
public static _PgAggFunc xmlAgg(@Nullable SQLs.ArgDistinct modifier, Expression any) {
return PostgreFunctionUtils.oneArgAggFunc("xmlagg", modifier, any, null, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}.
*
*
* @see xmlagg ( xml ) → xml
* Concatenates the non-null XML input values
*
*/
public static _PgAggFunc xmlAgg(Expression any, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("xmlagg", null, any, consumer, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}.
*
*
* @see xmlagg ( xml ) → xml
* Concatenates the non-null XML input values
*
*/
public static _PgAggFunc xmlAgg(@Nullable SQLs.ArgDistinct modifier, Expression any,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("xmlagg", modifier, any, consumer, XmlType.TEXT);
}
/**
*
* The {@link MappingType} of function return type: {@link XmlType#TEXT}.
*
*
* @see xmlagg ( xml ) → xml
* Concatenates the non-null XML input values
*
*/
public static _AggWindowFunc xmlAgg(Expression xml) {
return PostgreFunctionUtils.oneArgAggWindowFunc("xmlagg", xml, XmlType.TEXT);
}
/*-------------------below Aggregate Functions for Statistics-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see corr ( Y double precision, X double precision ) → double precision
* Computes the correlation coefficient.
*
*/
public static _PgAggFunc corr(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("corr", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see corr ( Y double precision, X double precision ) → double precision
* Computes the correlation coefficient.
*
*/
public static _PgAggFunc corr(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("corr", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see corr ( Y double precision, X double precision ) → double precision
* Computes the correlation coefficient.
*
*/
public static _PgAggFunc corr(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("corr", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see corr ( Y double precision, X double precision ) → double precision
* Computes the correlation coefficient.
*
*/
public static _AggWindowFunc corr(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("corr", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_pop ( Y double precision, X double precision ) → double precision
* Computes the population covariance.
*
*/
public static _PgAggFunc covarPop(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("covar_pop", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_pop ( Y double precision, X double precision ) → double precision
* Computes the population covariance.
*
*/
public static _PgAggFunc covarPop(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("covar_pop", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_pop ( Y double precision, X double precision ) → double precision
* Computes the population covariance.
*
*/
public static _PgAggFunc covarPop(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("covar_pop", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_pop ( Y double precision, X double precision ) → double precision
* Computes the population covariance.
*
*/
public static _AggWindowFunc covarPop(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("covar_pop", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_samp ( Y double precision, X double precision ) → double precision
* Computes the sample covariance.
*
*/
public static _PgAggFunc covarSamp(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("covar_samp", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_samp ( Y double precision, X double precision ) → double precision
* Computes the sample covariance.
*
*/
public static _PgAggFunc covarSamp(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("covar_samp", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_samp ( Y double precision, X double precision ) → double precision
* Computes the sample covariance.
*
*/
public static _PgAggFunc covarSamp(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("covar_samp", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see covar_samp ( Y double precision, X double precision ) → double precision
* Computes the sample covariance.
*
*/
public static _AggWindowFunc covarSamp(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("covar_samp", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgx ( Y double precision, X double precision ) → double precision
* Computes the average of the independent variable, sum(X)/N.
*
*/
public static _PgAggFunc regrAvgx(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_avgx", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgx ( Y double precision, X double precision ) → double precision
* Computes the average of the independent variable, sum(X)/N.
*
*/
public static _PgAggFunc regrAvgx(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_avgx", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgx ( Y double precision, X double precision ) → double precision
* Computes the average of the independent variable, sum(X)/N.
*
*/
public static _PgAggFunc regrAvgx(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_avgx", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgx ( Y double precision, X double precision ) → double precision
* Computes the average of the independent variable, sum(X)/N.
*
*/
public static _AggWindowFunc regrAvgx(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_avgx", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgy ( Y double precision, X double precision ) → double precision
* Computes the average of the dependent variable, sum(Y)/N.
*
*/
public static _PgAggFunc regrAvgy(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_avgy", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgy ( Y double precision, X double precision ) → double precision
* Computes the average of the dependent variable, sum(Y)/N.
*
*/
public static _PgAggFunc regrAvgy(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_avgy", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgy ( Y double precision, X double precision ) → double precision
* Computes the average of the dependent variable, sum(Y)/N.
*
*/
public static _PgAggFunc regrAvgy(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_avgy", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_avgy ( Y double precision, X double precision ) → double precision
* Computes the average of the dependent variable, sum(Y)/N.
*
*/
public static _AggWindowFunc regrAvgy(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_avgy", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see regr_count ( Y double precision, X double precision ) → bigint
* Computes the number of rows in which both inputs are non-null.
*
*/
public static _PgAggFunc regrCount(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_count", modifier, y, x, null, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see regr_count ( Y double precision, X double precision ) → bigint
* Computes the number of rows in which both inputs are non-null.
*
*/
public static _PgAggFunc regrCount(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_count", null, y, x, consumer, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see regr_count ( Y double precision, X double precision ) → bigint
* Computes the number of rows in which both inputs are non-null.
*
*/
public static _PgAggFunc regrCount(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_count", modifier, y, x, consumer, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}.
*
*
* @see regr_count ( Y double precision, X double precision ) → bigint
* Computes the number of rows in which both inputs are non-null.
*
*/
public static _AggWindowFunc regrCount(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_count", y, x, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_intercept ( Y double precision, X double precision ) → double precision
* Computes the y-intercept of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _PgAggFunc regrIntercept(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_intercept", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_intercept ( Y double precision, X double precision ) → double precision
* Computes the y-intercept of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _PgAggFunc regrIntercept(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_intercept", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_intercept ( Y double precision, X double precision ) → double precision
* Computes the y-intercept of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _PgAggFunc regrIntercept(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_intercept", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_intercept ( Y double precision, X double precision ) → double precision
* Computes the y-intercept of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _AggWindowFunc regrIntercept(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_intercept", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_r2 ( Y double precision, X double precision ) → double precision
* Computes the square of the correlation coefficient.
*
*/
public static _PgAggFunc regrR2(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_r2", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_r2 ( Y double precision, X double precision ) → double precision
* Computes the square of the correlation coefficient.
*
*/
public static _PgAggFunc regrR2(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_r2", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_r2 ( Y double precision, X double precision ) → double precision
* Computes the square of the correlation coefficient.
*
*/
public static _PgAggFunc regrR2(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_r2", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_r2 ( Y double precision, X double precision ) → double precision
* Computes the square of the correlation coefficient.
*
*/
public static _AggWindowFunc regrR2(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_r2", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_slope ( Y double precision, X double precision ) → double precision
* Computes the slope of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _PgAggFunc regrSlope(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_slope", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
* /**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_slope ( Y double precision, X double precision ) → double precision
* Computes the slope of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _PgAggFunc regrSlope(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_slope", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_slope ( Y double precision, X double precision ) → double precision
* Computes the slope of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _PgAggFunc regrSlope(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_slope", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_slope ( Y double precision, X double precision ) → double precision
* Computes the slope of the least-squares-fit linear equation determined by the (X, Y) pairs.
*
*/
public static _AggWindowFunc regrSlope(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_slope", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxx ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the independent variable, sum(X^2) - sum(X)^2/N.
*
*/
public static _PgAggFunc regrSxx(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_sxx", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxx ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the independent variable, sum(X^2) - sum(X)^2/N.
*
*/
public static _PgAggFunc regrSxx(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_sxx", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxx ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the independent variable, sum(X^2) - sum(X)^2/N.
*
*/
public static _PgAggFunc regrSxx(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_sxx", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxx ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the independent variable, sum(X^2) - sum(X)^2/N.
*
*/
public static _AggWindowFunc regrSxx(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_sxx", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxy ( Y double precision, X double precision ) → double precision
* Computes the “sum of products” of independent times dependent variables, sum(X*Y) - sum(X) * sum(Y)/N.
*
*/
public static _PgAggFunc regrSxy(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_sxy", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxy ( Y double precision, X double precision ) → double precision
* Computes the “sum of products” of independent times dependent variables, sum(X*Y) - sum(X) * sum(Y)/N.
*
*/
public static _PgAggFunc regrSxy(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_sxy", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxy ( Y double precision, X double precision ) → double precision
* Computes the “sum of products” of independent times dependent variables, sum(X*Y) - sum(X) * sum(Y)/N.
*
*/
public static _PgAggFunc regrSxy(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_sxy", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_sxy ( Y double precision, X double precision ) → double precision
* Computes the “sum of products” of independent times dependent variables, sum(X*Y) - sum(X) * sum(Y)/N.
*
*/
public static _AggWindowFunc regrSxy(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_sxy", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_syy ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the dependent variable, sum(Y^2) - sum(Y)^2/N.
*
*/
public static _PgAggFunc regrSyy(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggFunc("regr_syy", modifier, y, x, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_syy ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the dependent variable, sum(Y^2) - sum(Y)^2/N.
*
*/
public static _PgAggFunc regrSyy(Expression y, Expression x, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_syy", null, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_syy ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the dependent variable, sum(Y^2) - sum(Y)^2/N.
*
*/
public static _PgAggFunc regrSyy(@Nullable SQLs.ArgDistinct modifier, Expression y, Expression x,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.twoArgAggFunc("regr_syy", modifier, y, x, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see regr_syy ( Y double precision, X double precision ) → double precision
* Computes the “sum of squares” of the dependent variable, sum(Y^2) - sum(Y)^2/N.
*
*/
public static _AggWindowFunc regrSyy(Expression y, Expression x) {
return PostgreFunctionUtils.twoArgAggWindowFunc("regr_syy", y, x, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for stddev_samp.
*
*/
public static _PgAggFunc stdDev(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("stddev", modifier, exp, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for stddev_samp.
*
*/
public static _PgAggFunc stdDev(Expression exp, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("stddev", null, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for stddev_samp.
*
*/
public static _PgAggFunc stdDev(@Nullable SQLs.ArgDistinct modifier, Expression exp,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("stddev", modifier, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for stddev_samp.
*
*/
public static _AggWindowFunc stdDev(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("stddev", exp, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population standard deviation of the input values.
*
*/
public static _PgAggFunc stdDevPop(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("stddev_pop", modifier, exp, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population standard deviation of the input values.
*
*/
public static _PgAggFunc stdDevPop(Expression exp, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("stddev_pop", null, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population standard deviation of the input values.
*
*/
public static _PgAggFunc stdDevPop(@Nullable SQLs.ArgDistinct modifier, Expression exp,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("stddev_pop", modifier, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population standard deviation of the input values.
*
*/
public static _AggWindowFunc stdDevPop(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("stddev_pop", exp, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample standard deviation of the input values.
*
*/
public static _PgAggFunc stdDevSamp(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("stddev_samp", modifier, exp, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample standard deviation of the input values.
*
*/
public static _PgAggFunc stdDevSamp(Expression exp, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("stddev_samp", null, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample standard deviation of the input values.
*
*/
public static _PgAggFunc stdDevSamp(@Nullable SQLs.ArgDistinct modifier, Expression exp,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("stddev_samp", modifier, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see stddev_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample standard deviation of the input values.
*
*/
public static _AggWindowFunc stdDevSamp(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("stddev_samp", exp, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see variance ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for var_samp.
*
*/
public static _PgAggFunc variance(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("variance", modifier, exp, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see variance ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for var_samp.
*
*/
public static _PgAggFunc variance(Expression exp, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("variance", null, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see variance ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for var_samp.
*
*/
public static _PgAggFunc variance(@Nullable SQLs.ArgDistinct modifier, Expression exp,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("variance", modifier, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see variance ( numeric_type ) → double precision for real or double precision, otherwise numeric
* This is a historical alias for var_samp.
*
*/
public static _AggWindowFunc variance(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("variance", exp, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population variance of the input values (square of the population standard deviation).
*
*/
public static _PgAggFunc varPop(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("var_pop", modifier, exp, null, DoubleType.INSTANCE);
}
/**
* /**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population variance of the input values (square of the population standard deviation).
*
*/
public static _PgAggFunc varPop(Expression exp, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("var_pop", null, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population variance of the input values (square of the population standard deviation).
*
*/
public static _PgAggFunc varPop(@Nullable SQLs.ArgDistinct modifier, Expression exp,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("var_pop", modifier, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_pop ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the population variance of the input values (square of the population standard deviation).
*
*/
public static _AggWindowFunc varPop(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("var_pop", exp, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample variance of the input values (square of the sample standard deviation).
*
*/
public static _PgAggFunc varSamp(@Nullable SQLs.ArgDistinct modifier, Expression exp) {
return PostgreFunctionUtils.oneArgAggFunc("var_samp", modifier, exp, null, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample variance of the input values (square of the sample standard deviation).
*
*/
public static _PgAggFunc varSamp(Expression exp, Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("var_samp", null, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample variance of the input values (square of the sample standard deviation).
*
*/
public static _PgAggFunc varSamp(@Nullable SQLs.ArgDistinct modifier, Expression exp,
Consumer consumer) {
ContextStack.assertNonNull(consumer);
return PostgreFunctionUtils.oneArgAggFunc("var_samp", modifier, exp, consumer, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}.
*
*
* @see var_samp ( numeric_type ) → double precision for real or double precision, otherwise numeric
* Computes the sample variance of the input values (square of the sample standard deviation).
*
*/
public static _AggWindowFunc varSamp(Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc("var_samp", exp, DoubleType.INSTANCE);
}
/*-------------------below Ordered-Set Aggregate Functions -------------------*/
/**
*
* The {@link MappingType} of function return type: the {@link MappingType} of order by clause first item.
*
*
* @see mode () WITHIN GROUP ( ORDER BY anyelement ) → anyelement
* Computes the mode, the most frequent value of the aggregated argument (arbitrarily choosing the first one if there are multiple equally-frequent values). The aggregated argument must be of a sortable type.
*
*/
public static _AggWithGroupClause mode() {
return PostgreFunctionUtils.zeroArgWithGroupAggFunc("mode",
PostgreFunctionUtils.unaryOrderSetType(Expressions::identityType)
);
}
/**
*
* The {@link MappingType} of function return type:
* - If fraction is double array type and order by clause first item is sql double type type,then {@link DoubleType#INSTANCE}
* - If fraction is sql double array type and order by clause first item is sql interval type,then {@link IntervalType#TEXT}
* - If fraction is sql double type and order by clause first item is sql double type type,then {@link DoubleArrayType#LINEAR}
* - If fraction is sql double type and order by clause first item is sql interval type,then {@link IntervalArrayType#LINEAR}
* - Else {@link TextType#INSTANCE}
*
*
*
* @see percentile_cont ( fraction double precision ) WITHIN GROUP ( ORDER BY double precision ) → double precision
*
*/
public static _AggWithGroupClause percentileCont(final Expression fraction) {
return PostgreFunctionUtils.oneArgWithGroupAggFunc("percentile_cont", fraction,
PostgreFunctionUtils.biOrderedSetType(fraction, PostgreWindowFunctions::_percentileType)
);
}
/**
*
* The {@link MappingType} of function return type:
* - If fraction is double array type ,then it is the {@link MappingType} of order by clause first item
* - If fraction is double array type ,then it is the array {@link MappingType} of order by clause first item
* - Else {@link TextType#INSTANCE}
*
*
*
* @see percentile_disc ( fraction double precision ) WITHIN GROUP ( ORDER BY anyelement ) → anyelement
* percentile_disc ( fractions double precision[] ) WITHIN GROUP ( ORDER BY anyelement ) → anyarray
*
*/
public static _AggWithGroupClause percentileDisc(final Expression fraction) {
return PostgreFunctionUtils.oneArgWithGroupAggFunc("percentile_disc", fraction,
PostgreFunctionUtils.biOrderedSetType(fraction, PostgreWindowFunctions::_percentileType)
);
}
/*-------------------below Hypothetical-Set Aggregate Functions-------------------*/
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see rank ( args ) WITHIN GROUP ( ORDER BY sorted_args ) → bigint
*
*/
public static _AggWithGroupClause rank(Expression args) {
return PostgreFunctionUtils.oneArgWithGroupAggFunc("rank", args, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link LongType#INSTANCE}
*
*
* @see dense_rank ( args ) WITHIN GROUP ( ORDER BY sorted_args ) → bigint
*
*/
public static _AggWithGroupClause denseRank(Expression args) {
return PostgreFunctionUtils.oneArgWithGroupAggFunc("dense_rank", args, LongType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}
*
*
* @see percent_rank ( args ) WITHIN GROUP ( ORDER BY sorted_args ) → double precision
*
*/
public static _AggWithGroupClause percentRank(Expression args) {
return PostgreFunctionUtils.oneArgWithGroupAggFunc("percent_rank", args, DoubleType.INSTANCE);
}
/**
*
* The {@link MappingType} of function return type: {@link DoubleType#INSTANCE}
*
*
* @see cume_dist ( args ) WITHIN GROUP ( ORDER BY sorted_args ) → double precision
*
*/
public static _AggWithGroupClause cumeDist(Expression args) {
return PostgreFunctionUtils.oneArgWithGroupAggFunc("cume_dist", args, DoubleType.INSTANCE);
}
/*-------------------below user-defined WITH GROUP aggregate function-------------------*/
/**
* user-defined WITH GROUP aggregate function
*/
public static _AggWithGroupClause myWithGroupAggFunc(String name, TypeMeta returnType) {
return PostgreFunctionUtils.zeroArgMyWithGroupAggFunc(name, returnType);
}
/**
* user-defined WITH GROUP aggregate function
*/
public static _AggWithGroupClause myWithGroupAggFunc(String name, Expression one, TypeMeta returnType) {
return PostgreFunctionUtils.oneArgMyWithGroupAggFunc(name, one, returnType);
}
/**
* user-defined WITH GROUP aggregate function
*/
public static _AggWithGroupClause myWithGroupAggFunc(String name, List argList, TypeMeta returnType) {
return PostgreFunctionUtils.multiArgMyWithGroupAggFunc(name, FunctionUtils.expList(name, argList), returnType);
}
/*-------------------below private method-------------------*/
/**
* @see #bitAnd(Expression)
* @see #bitOr(Expression)
* @see #bitXor(Expression)
*/
private static _AggWindowFunc _bitWindowFunc(String name, Expression exp) {
return PostgreFunctionUtils.oneArgAggWindowFunc(name, exp,
_returnType(exp, PostgreWindowFunctions::_bitOpeType)
);
}
/**
* @see #avg(Expression)
*/
private static MappingType _avgType(final MappingType type) {
final MappingType returnType;
if (type instanceof MappingType.SqlIntegerType || type instanceof MappingType.SqlDecimalType) {
returnType = BigDecimalType.INSTANCE;
} else if (type instanceof MappingType.SqlFloatType) {
returnType = DoubleType.INSTANCE;
} else if (type instanceof MappingType.SqlIntervalType) {
returnType = IntervalType.TEXT;
} else {
returnType = TextType.INSTANCE;
}
return returnType;
}
/**
* @see #bitAnd(Expression)
* @see #bitOr(Expression)
*/
private static MappingType _bitOpeType(final MappingType type) {
final MappingType returnType;
if (type instanceof MappingType.SqlIntegerType || type instanceof MappingType.SqlBitType) {
returnType = type;
} else {
returnType = TextType.INSTANCE;
}
return returnType;
}
/**
* @see #percentileCont(Expression)
*/
private static MappingType _percentileType(final MappingType fractionType, final MappingType type) {
final MappingType returnType;
if (fractionType instanceof MappingType.SqlArrayType) {
returnType = type.arrayTypeOfThis();
} else if (type instanceof MappingType.SqlFloatType) {
returnType = type;
} else if (type instanceof MappingType.SqlIntervalType) {
returnType = type;
} else {
returnType = TextType.INSTANCE;
}
return returnType;
}
}